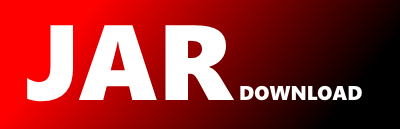
de.rwth.swc.coffee4j.model.report.ExecutionReporter Maven / Gradle / Ivy
package de.rwth.swc.coffee4j.model.report;
import de.rwth.swc.coffee4j.engine.report.ArgumentConverter;
import de.rwth.swc.coffee4j.engine.report.GenerationReporter;
import de.rwth.swc.coffee4j.engine.TestResult;
import de.rwth.swc.coffee4j.engine.characterization.FaultCharacterizationAlgorithm;
import de.rwth.swc.coffee4j.engine.generator.TestInputGroup;
import de.rwth.swc.coffee4j.engine.generator.TestInputGroupGenerator;
import de.rwth.swc.coffee4j.engine.report.Report;
import de.rwth.swc.coffee4j.engine.report.ReportLevel;
import de.rwth.swc.coffee4j.model.Combination;
import de.rwth.swc.coffee4j.model.InputParameterModel;
import de.rwth.swc.coffee4j.model.TestInputGroupContext;
import java.util.List;
/**
* The {@link InputParameterModel}-based equivalent to a
* {@link GenerationReporter}.
* Instead of {@link TestInputGroup}s this uses {@link TestInputGroupContext}s so
* that identifies can be converted using {@link ArgumentConverter}s. Additionally,
* this means that all test inputs are converted into a sensible format ({@link Combination}).
*
* The method in this class can be split into three categories. The first one deals with all lifecycle events
* from {@link TestInputGroupContext}s. The second one with actual execution of test inputs, and the third one with
* event reporting capabilities. Actual test execution reporting is not done per {@link TestInputGroupContext}, as
* caching results in some tests getting executed for multiple {@link TestInputGroupContext}s.
*
* All methods have empty default implementations so that each implementing class can choose what methods to
* override.
*/
public interface ExecutionReporter {
/**
* Called if a new {@link TestInputGroup} has been generated by a
* {@link TestInputGroupGenerator}. The generator is given inside
* the {@link TestInputGroupContext}.
*
* @param context all important information about one group
* @param testInputs the initially generated test inputs
*/
default void testInputGroupGenerated(TestInputGroupContext context, List testInputs) {
}
/**
* Called if a {@link TestInputGroup} has completely finished. This means
* fault characterization is finished (if it was enabled), and no more methods in this class will be called in
* reference to this context.
*
* @param context the context of the group which was finished
*/
default void testInputGroupFinished(TestInputGroupContext context) {
}
/**
* Called if a fault characterization started for a {@link TestInputGroup}.
* The process will end again when {@link #faultCharacterizationFinished(TestInputGroupContext, List)} is called.
*
* @param context the context of the group for which fault characterization started
* @param algorithm the algorithm which will be used to characterize faults. This is a reference to the real
* algorithm. Do not modify as this will most likely have unintended side effects
*/
default void faultCharacterizationStarted(TestInputGroupContext context, FaultCharacterizationAlgorithm algorithm) {
}
/**
* Called if fault characterization for a {@link TestInputGroup} is completely
* finished. This means {@link #faultCharacterizationTestInputsGenerated(TestInputGroupContext, List)} will never
* be called again for this context, and soon after this method {@link #testInputGroupFinished(TestInputGroupContext)}
* is called.
*
* @param context the context of the group for which fault characterization finished
* @param failureInducingCombinations all failure-inducing combinations found. The order may or may not be based
* on an algorithm internal probability metric of the combinations being
* failure-inducing
*/
default void faultCharacterizationFinished(TestInputGroupContext context, List failureInducingCombinations) {
}
/**
* Called if additional test inputs for the fault characterization process of one
* {@link TestInputGroup} have been generated by the algorithm given to
* {@link #faultCharacterizationStarted(TestInputGroupContext, FaultCharacterizationAlgorithm)}. This method can only be
* called (multiple times) between calls to
* {@link #faultCharacterizationStarted(TestInputGroupContext, FaultCharacterizationAlgorithm)} and
* {@link #faultCharacterizationFinished(TestInputGroupContext, List)}.
*
* @param context the context of the group for which additional test inputs were generated
* @param testInputs all additionally generated test inputs
*/
default void faultCharacterizationTestInputsGenerated(TestInputGroupContext context, List testInputs) {
}
/**
* Indicates the start of a test input execution.
*
* @param testInput the started test input
*/
default void testInputExecutionStarted(Combination testInput) {
}
/**
* Indicates the end of a test input execution.
*
* @param testInput the finished test input
* @param result the result of the test input
*/
default void testInputExecutionFinished(Combination testInput, TestResult result) {
}
/**
* Specifies the level of reports this reporter wants to get. Only reports with an equal of higher
* {@link ReportLevel} will be passed to {@link #report(ReportLevel, Report)}.
*
* @return the desired level of reports. The default method returns trace
*/
default ReportLevel getReportLevel() {
return ReportLevel.TRACE;
}
/**
* Called if any algorithm made a report for and event not covered by any of the life cycle callback methods.
*
* @param level the level of the report. Always higher than or equal to {@link #getReportLevel()}
* @param report the actual report with resolved arguments
*/
default void report(ReportLevel level, Report report) {
}
}