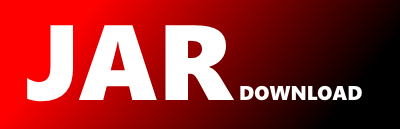
de.saumya.mojo.gem.HelpMojo Maven / Gradle / Ivy
package de.saumya.mojo.gem;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
/**
* Display help information on gem-maven-plugin.
Call mvn gem:help -Ddetail=true -Dgoal=<goal-name>
to display parameter details.
*
* @version generated on Fri Nov 28 12:18:07 GMT 2014
* @author org.apache.maven.tools.plugin.generator.PluginHelpGenerator (version 2.5.1)
* @goal help
* @requiresProject false
*/
public class HelpMojo
extends AbstractMojo
{
/**
* If true
, display all settable properties for each goal.
*
* @parameter expression="${detail}" default-value="false"
*/
private boolean detail;
/**
* The name of the goal for which to show help. If unspecified, all goals will be displayed.
*
* @parameter expression="${goal}"
*/
private java.lang.String goal;
/**
* The maximum length of a display line, should be positive.
*
* @parameter expression="${lineLength}" default-value="80"
*/
private int lineLength;
/**
* The number of spaces per indentation level, should be positive.
*
* @parameter expression="${indentSize}" default-value="2"
*/
private int indentSize;
/** {@inheritDoc} */
public void execute()
throws MojoExecutionException
{
if ( lineLength <= 0 )
{
getLog().warn( "The parameter 'lineLength' should be positive, using '80' as default." );
lineLength = 80;
}
if ( indentSize <= 0 )
{
getLog().warn( "The parameter 'indentSize' should be positive, using '2' as default." );
indentSize = 2;
}
StringBuffer sb = new StringBuffer();
append( sb, "de.saumya.mojo:gem-maven-plugin:1.0.7", 0 );
append( sb, "", 0 );
append( sb, "Gem Maven Mojo", 0 );
append( sb, "shared dependencies and plugins for the mojos", 1 );
append( sb, "", 0 );
if ( goal == null || goal.length() <= 0 )
{
append( sb, "This plugin has 11 goals:", 0 );
append( sb, "", 0 );
}
if ( goal == null || goal.length() <= 0 || "exec".equals( goal ) )
{
append( sb, "gem:exec", 0 );
append( sb, "executes a ruby script in context of the gems from pom. the arguments for jruby are build like this: ${jruby.args} ${exec.file} ${exec.args} ${args}\nto execute an inline script the exec parameters are ignored.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "args", 2 );
append( sb, "common arguments\nCommand line -Dargs=...", 3 );
append( sb, "", 0 );
append( sb, "binDirectory", 2 );
append( sb, "directory of JRuby bin path to use when forking JRuby.\nCommand line -Dgem.binDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "env", 2 );
append( sb, "environment values passed on to the jruby process. needs jrubyFork true.\n", 3 );
append( sb, "", 0 );
append( sb, "execArgLines", 2 );
append( sb, "an array of arguments which can contain spaces for the ruby script given through file parameter.", 3 );
append( sb, "", 0 );
append( sb, "execArgs", 2 );
append( sb, "arguments separated by whitespaces for the ruby script given through file parameter. no quoting or escaping possible - if needed use execArglines instead.\nCommand line -Dexec.args=...", 3 );
append( sb, "", 0 );
append( sb, "file", 2 );
append( sb, "ruby file which gets executed in context of the given gems..\nCommand line -Dexec.file=...", 3 );
append( sb, "", 0 );
append( sb, "filename", 2 );
append( sb, "ruby file found on search path which gets executed. the search path includes the executable scripts which were installed via the given gem-artifacts.\nCommand line -Dexec.filename=...", 3 );
append( sb, "", 0 );
append( sb, "gemHome (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of gem home to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.home=...", 3 );
append( sb, "", 0 );
append( sb, "gemHomes", 2 );
append( sb, "map different install locations for rubygems (GEM_HOME) to a directory. examples are the different scopes like provided and test as well when installed inside a plugin declaration, where the key is the artifactId of the plugin.\n", 3 );
append( sb, "", 0 );
append( sb, "gemPath (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of JRuby path to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.path=...", 3 );
append( sb, "", 0 );
append( sb, "gemUseSystem (Default: false)", 2 );
append( sb, "use system gems instead of setting up GemPath/GemHome inside the build directory and ignores any set gemHome and gemPath. you need to have both GEM_HOME and GEM_PATH environment variable set to make it work.\nCommand line -Dgem.useSystem=...", 3 );
append( sb, "", 0 );
append( sb, "includeGemsInResources", 2 );
append( sb, "EXPERIMENTAL this gives the scope of the gems which shall be included to resources. flag whether to include all gems to resources, i.e. to classpath or not. the difference to the includeRubygemsInResources is that it does not depend on rubygems during runtime since the required_path of the gems gets added to resources. note that it expect the required_path of the gem to be lib which is the default BUT that is not true for all gems. in this sense this feature is incomplete and might not work for you !!! IMPORTANT: it only adds the gems with provided scope since they are packed with the jar and then the pom.xml will not have them (since they are marked \'provided\') as transitive dependencies. this feature can be helpful in situations where the classloader does not work for rubygems due to rubygems uses file system globs to find the gems and this only works if the classloader reveals the jar url of its jars (i.e. URLClassLoader). for example OSGi classloader can not work with rubygems !!\nCommand line -Dgem.includeGemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeLibDirectoryInResources (Default: false)", 2 );
append( sb, "\nCommand line -Dgem.includeLibDirectoryInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeOpenSSL (Default: true)", 2 );
append( sb, "flag whether to include open-ssl gem or not\nCommand line -Dgem.includeOpenSSL=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInResources (Default: false)", 2 );
append( sb, "flag whether to include all gems to resources, i.e. to classpath or not\nCommand line -Dgem.includeRubygemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInTestResources (Default: true)", 2 );
append( sb, "flag whether to include all gems to test-resources, i.e. to test-classpath or not\nCommand line -Dgem.includeRubygemsInTestResources=...", 3 );
append( sb, "", 0 );
append( sb, "installRDoc (Default: false)", 2 );
append( sb, "flag whether to install rdocs of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "installRI (Default: false)", 2 );
append( sb, "flag whether to install ri of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyFork (Default: true)", 2 );
append( sb, "fork the JRuby execution.\nCommand line -Djruby.fork=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyJvmArgs", 2 );
append( sb, "jvm arguments for the java command executing jruby\nCommand line -Djruby.jvmargs=...", 3 );
append( sb, "", 0 );
append( sb, "jrubySwitches", 2 );
append( sb, "switches for the jruby command, like \'--1.9\'\nCommand line -Djruby.switches=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVerbose (Default: false)", 2 );
append( sb, "verbose jruby related output\nCommand line -Djruby.verbose=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVersion", 2 );
append( sb, "if the pom.xml has no runtime dependency to a jruby-complete.jar then this version is used to resolve the jruby-complete dependency from the local/remote maven repository. it overwrites the jruby version from the dependencies if any. i.e. you can easily switch jruby version from the commandline !\ndefault: 1.7.12\nCommand line -Djruby.version=...", 3 );
append( sb, "", 0 );
append( sb, "launchDirectory (Default: ${project.basedir})", 2 );
append( sb, "the launch directory for the JRuby execution.\nCommand line -Djruby.launchDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "libDirectory (Default: lib)", 2 );
append( sb, "directory with ruby sources - added to ruby loadpath only\nCommand line -Djruby.lib=...", 3 );
append( sb, "", 0 );
append( sb, "outputFile", 2 );
append( sb, "output file where the standard out will be written\nCommand line -Dexec.outputFile=...", 3 );
append( sb, "", 0 );
append( sb, "plugin", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "reference to maven project for internal use.", 3 );
append( sb, "", 0 );
append( sb, "rubySourceDirectory (Default: src/main/ruby)", 2 );
append( sb, "directory with ruby sources - added to java classpath and ruby loadpath\nCommand line -Djruby.sourceDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "script", 2 );
append( sb, "ruby code from the pom configuration part which gets executed.\nCommand line -Dexec.script=...", 3 );
append( sb, "", 0 );
append( sb, "supportNative (Default: false)", 2 );
append( sb, "flag to indicate to setup jruby\'s native support for C-extensions\nCommand line -Dgem.supportNative=...", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "gem".equals( goal ) )
{
append( sb, "gem:gem", 0 );
append( sb, "goal to run gem with the given arguments.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "args", 2 );
append( sb, "common arguments\nCommand line -Dargs=...", 3 );
append( sb, "", 0 );
append( sb, "binDirectory", 2 );
append( sb, "directory of JRuby bin path to use when forking JRuby.\nCommand line -Dgem.binDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "env", 2 );
append( sb, "environment values passed on to the jruby process. needs jrubyFork true.\n", 3 );
append( sb, "", 0 );
append( sb, "gemArgs (Default: ${gem.args})", 2 );
append( sb, "arguments for the gem command of JRuby.\nCommand line -Dgem.args=...", 3 );
append( sb, "", 0 );
append( sb, "gemHome (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of gem home to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.home=...", 3 );
append( sb, "", 0 );
append( sb, "gemHomes", 2 );
append( sb, "map different install locations for rubygems (GEM_HOME) to a directory. examples are the different scopes like provided and test as well when installed inside a plugin declaration, where the key is the artifactId of the plugin.\n", 3 );
append( sb, "", 0 );
append( sb, "gemPath (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of JRuby path to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.path=...", 3 );
append( sb, "", 0 );
append( sb, "gemUseSystem (Default: false)", 2 );
append( sb, "use system gems instead of setting up GemPath/GemHome inside the build directory and ignores any set gemHome and gemPath. you need to have both GEM_HOME and GEM_PATH environment variable set to make it work.\nCommand line -Dgem.useSystem=...", 3 );
append( sb, "", 0 );
append( sb, "includeGemsInResources", 2 );
append( sb, "EXPERIMENTAL this gives the scope of the gems which shall be included to resources. flag whether to include all gems to resources, i.e. to classpath or not. the difference to the includeRubygemsInResources is that it does not depend on rubygems during runtime since the required_path of the gems gets added to resources. note that it expect the required_path of the gem to be lib which is the default BUT that is not true for all gems. in this sense this feature is incomplete and might not work for you !!! IMPORTANT: it only adds the gems with provided scope since they are packed with the jar and then the pom.xml will not have them (since they are marked \'provided\') as transitive dependencies. this feature can be helpful in situations where the classloader does not work for rubygems due to rubygems uses file system globs to find the gems and this only works if the classloader reveals the jar url of its jars (i.e. URLClassLoader). for example OSGi classloader can not work with rubygems !!\nCommand line -Dgem.includeGemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeLibDirectoryInResources (Default: false)", 2 );
append( sb, "\nCommand line -Dgem.includeLibDirectoryInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeOpenSSL (Default: true)", 2 );
append( sb, "flag whether to include open-ssl gem or not\nCommand line -Dgem.includeOpenSSL=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInResources (Default: false)", 2 );
append( sb, "flag whether to include all gems to resources, i.e. to classpath or not\nCommand line -Dgem.includeRubygemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInTestResources (Default: true)", 2 );
append( sb, "flag whether to include all gems to test-resources, i.e. to test-classpath or not\nCommand line -Dgem.includeRubygemsInTestResources=...", 3 );
append( sb, "", 0 );
append( sb, "installRDoc (Default: false)", 2 );
append( sb, "flag whether to install rdocs of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "installRI (Default: false)", 2 );
append( sb, "flag whether to install ri of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyFork (Default: true)", 2 );
append( sb, "fork the JRuby execution.\nCommand line -Djruby.fork=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyJvmArgs", 2 );
append( sb, "jvm arguments for the java command executing jruby\nCommand line -Djruby.jvmargs=...", 3 );
append( sb, "", 0 );
append( sb, "jrubySwitches", 2 );
append( sb, "switches for the jruby command, like \'--1.9\'\nCommand line -Djruby.switches=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVerbose (Default: false)", 2 );
append( sb, "verbose jruby related output\nCommand line -Djruby.verbose=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVersion", 2 );
append( sb, "if the pom.xml has no runtime dependency to a jruby-complete.jar then this version is used to resolve the jruby-complete dependency from the local/remote maven repository. it overwrites the jruby version from the dependencies if any. i.e. you can easily switch jruby version from the commandline !\ndefault: 1.7.12\nCommand line -Djruby.version=...", 3 );
append( sb, "", 0 );
append( sb, "launchDirectory (Default: ${project.basedir})", 2 );
append( sb, "the launch directory for the JRuby execution.\nCommand line -Djruby.launchDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "libDirectory (Default: lib)", 2 );
append( sb, "directory with ruby sources - added to ruby loadpath only\nCommand line -Djruby.lib=...", 3 );
append( sb, "", 0 );
append( sb, "plugin", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "reference to maven project for internal use.", 3 );
append( sb, "", 0 );
append( sb, "rubySourceDirectory (Default: src/main/ruby)", 2 );
append( sb, "directory with ruby sources - added to java classpath and ruby loadpath\nCommand line -Djruby.sourceDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "supportNative (Default: false)", 2 );
append( sb, "flag to indicate to setup jruby\'s native support for C-extensions\nCommand line -Dgem.supportNative=...", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "gemify".equals( goal ) )
{
append( sb, "gem:gemify", 0 );
append( sb, "goal to convert that artifact into a gem.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "args", 2 );
append( sb, "common arguments\nCommand line -Dargs=...", 3 );
append( sb, "", 0 );
append( sb, "artifactId (Default: ${artifactId})", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "binDirectory", 2 );
append( sb, "directory of JRuby bin path to use when forking JRuby.\nCommand line -Dgem.binDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "buildDirectory (Default: ${project.build.directory})", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "env", 2 );
append( sb, "environment values passed on to the jruby process. needs jrubyFork true.\n", 3 );
append( sb, "", 0 );
append( sb, "gemHome (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of gem home to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.home=...", 3 );
append( sb, "", 0 );
append( sb, "gemHomes", 2 );
append( sb, "map different install locations for rubygems (GEM_HOME) to a directory. examples are the different scopes like provided and test as well when installed inside a plugin declaration, where the key is the artifactId of the plugin.\n", 3 );
append( sb, "", 0 );
append( sb, "gemify (Default: ${project.build.directory}/gemify)", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "gemPath (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of JRuby path to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.path=...", 3 );
append( sb, "", 0 );
append( sb, "gemUseSystem (Default: false)", 2 );
append( sb, "use system gems instead of setting up GemPath/GemHome inside the build directory and ignores any set gemHome and gemPath. you need to have both GEM_HOME and GEM_PATH environment variable set to make it work.\nCommand line -Dgem.useSystem=...", 3 );
append( sb, "", 0 );
append( sb, "groupId (Default: ${groupId})", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "includeGemsInResources", 2 );
append( sb, "EXPERIMENTAL this gives the scope of the gems which shall be included to resources. flag whether to include all gems to resources, i.e. to classpath or not. the difference to the includeRubygemsInResources is that it does not depend on rubygems during runtime since the required_path of the gems gets added to resources. note that it expect the required_path of the gem to be lib which is the default BUT that is not true for all gems. in this sense this feature is incomplete and might not work for you !!! IMPORTANT: it only adds the gems with provided scope since they are packed with the jar and then the pom.xml will not have them (since they are marked \'provided\') as transitive dependencies. this feature can be helpful in situations where the classloader does not work for rubygems due to rubygems uses file system globs to find the gems and this only works if the classloader reveals the jar url of its jars (i.e. URLClassLoader). for example OSGi classloader can not work with rubygems !!\nCommand line -Dgem.includeGemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeLibDirectoryInResources (Default: false)", 2 );
append( sb, "\nCommand line -Dgem.includeLibDirectoryInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeOpenSSL (Default: true)", 2 );
append( sb, "flag whether to include open-ssl gem or not\nCommand line -Dgem.includeOpenSSL=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInResources (Default: false)", 2 );
append( sb, "flag whether to include all gems to resources, i.e. to classpath or not\nCommand line -Dgem.includeRubygemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInTestResources (Default: true)", 2 );
append( sb, "flag whether to include all gems to test-resources, i.e. to test-classpath or not\nCommand line -Dgem.includeRubygemsInTestResources=...", 3 );
append( sb, "", 0 );
append( sb, "installRDoc (Default: false)", 2 );
append( sb, "flag whether to install rdocs of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "installRI (Default: false)", 2 );
append( sb, "flag whether to install ri of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyFork (Default: true)", 2 );
append( sb, "fork the JRuby execution.\nCommand line -Djruby.fork=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyJvmArgs", 2 );
append( sb, "jvm arguments for the java command executing jruby\nCommand line -Djruby.jvmargs=...", 3 );
append( sb, "", 0 );
append( sb, "jrubySwitches", 2 );
append( sb, "switches for the jruby command, like \'--1.9\'\nCommand line -Djruby.switches=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVerbose (Default: false)", 2 );
append( sb, "verbose jruby related output\nCommand line -Djruby.verbose=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVersion", 2 );
append( sb, "if the pom.xml has no runtime dependency to a jruby-complete.jar then this version is used to resolve the jruby-complete dependency from the local/remote maven repository. it overwrites the jruby version from the dependencies if any. i.e. you can easily switch jruby version from the commandline !\ndefault: 1.7.12\nCommand line -Djruby.version=...", 3 );
append( sb, "", 0 );
append( sb, "launchDirectory (Default: ${project.basedir})", 2 );
append( sb, "the launch directory for the JRuby execution.\nCommand line -Djruby.launchDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "libDirectory (Default: lib)", 2 );
append( sb, "directory with ruby sources - added to ruby loadpath only\nCommand line -Djruby.lib=...", 3 );
append( sb, "", 0 );
append( sb, "plugin", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "reference to maven project for internal use.", 3 );
append( sb, "", 0 );
append( sb, "rubySourceDirectory (Default: src/main/ruby)", 2 );
append( sb, "directory with ruby sources - added to java classpath and ruby loadpath\nCommand line -Djruby.sourceDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "skipGemInstall (Default: ${skipGemInstall})", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "supportNative (Default: false)", 2 );
append( sb, "flag to indicate to setup jruby\'s native support for C-extensions\nCommand line -Dgem.supportNative=...", 3 );
append( sb, "", 0 );
append( sb, "version (Default: ${version})", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "help".equals( goal ) )
{
append( sb, "gem:help", 0 );
append( sb, "Display help information on gem-maven-plugin.\nCall\n\u00a0\u00a0mvn\u00a0gem:help\u00a0-Ddetail=true\u00a0-Dgoal=\nto display parameter details.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "detail (Default: false)", 2 );
append( sb, "If true, display all settable properties for each goal.", 3 );
append( sb, "", 0 );
append( sb, "goal", 2 );
append( sb, "The name of the goal for which to show help. If unspecified, all goals will be displayed.", 3 );
append( sb, "", 0 );
append( sb, "indentSize (Default: 2)", 2 );
append( sb, "The number of spaces per indentation level, should be positive.", 3 );
append( sb, "", 0 );
append( sb, "lineLength (Default: 80)", 2 );
append( sb, "The maximum length of a display line, should be positive.", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "initialize".equals( goal ) )
{
append( sb, "gem:initialize", 0 );
append( sb, "(no description available)", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "args", 2 );
append( sb, "common arguments\nCommand line -Dargs=...", 3 );
append( sb, "", 0 );
append( sb, "binDirectory", 2 );
append( sb, "directory of JRuby bin path to use when forking JRuby.\nCommand line -Dgem.binDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "env", 2 );
append( sb, "environment values passed on to the jruby process. needs jrubyFork true.\n", 3 );
append( sb, "", 0 );
append( sb, "gemHome (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of gem home to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.home=...", 3 );
append( sb, "", 0 );
append( sb, "gemHomes", 2 );
append( sb, "map different install locations for rubygems (GEM_HOME) to a directory. examples are the different scopes like provided and test as well when installed inside a plugin declaration, where the key is the artifactId of the plugin.\n", 3 );
append( sb, "", 0 );
append( sb, "gemPath (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of JRuby path to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.path=...", 3 );
append( sb, "", 0 );
append( sb, "gemUseSystem (Default: false)", 2 );
append( sb, "use system gems instead of setting up GemPath/GemHome inside the build directory and ignores any set gemHome and gemPath. you need to have both GEM_HOME and GEM_PATH environment variable set to make it work.\nCommand line -Dgem.useSystem=...", 3 );
append( sb, "", 0 );
append( sb, "includeGemsInResources", 2 );
append( sb, "EXPERIMENTAL this gives the scope of the gems which shall be included to resources. flag whether to include all gems to resources, i.e. to classpath or not. the difference to the includeRubygemsInResources is that it does not depend on rubygems during runtime since the required_path of the gems gets added to resources. note that it expect the required_path of the gem to be lib which is the default BUT that is not true for all gems. in this sense this feature is incomplete and might not work for you !!! IMPORTANT: it only adds the gems with provided scope since they are packed with the jar and then the pom.xml will not have them (since they are marked \'provided\') as transitive dependencies. this feature can be helpful in situations where the classloader does not work for rubygems due to rubygems uses file system globs to find the gems and this only works if the classloader reveals the jar url of its jars (i.e. URLClassLoader). for example OSGi classloader can not work with rubygems !!\nCommand line -Dgem.includeGemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeLibDirectoryInResources (Default: false)", 2 );
append( sb, "\nCommand line -Dgem.includeLibDirectoryInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeOpenSSL (Default: true)", 2 );
append( sb, "flag whether to include open-ssl gem or not\nCommand line -Dgem.includeOpenSSL=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInResources (Default: false)", 2 );
append( sb, "flag whether to include all gems to resources, i.e. to classpath or not\nCommand line -Dgem.includeRubygemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInTestResources (Default: true)", 2 );
append( sb, "flag whether to include all gems to test-resources, i.e. to test-classpath or not\nCommand line -Dgem.includeRubygemsInTestResources=...", 3 );
append( sb, "", 0 );
append( sb, "installRDoc (Default: false)", 2 );
append( sb, "flag whether to install rdocs of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "installRI (Default: false)", 2 );
append( sb, "flag whether to install ri of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyFork (Default: true)", 2 );
append( sb, "fork the JRuby execution.\nCommand line -Djruby.fork=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyJvmArgs", 2 );
append( sb, "jvm arguments for the java command executing jruby\nCommand line -Djruby.jvmargs=...", 3 );
append( sb, "", 0 );
append( sb, "jrubySwitches", 2 );
append( sb, "switches for the jruby command, like \'--1.9\'\nCommand line -Djruby.switches=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVerbose (Default: false)", 2 );
append( sb, "verbose jruby related output\nCommand line -Djruby.verbose=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVersion", 2 );
append( sb, "if the pom.xml has no runtime dependency to a jruby-complete.jar then this version is used to resolve the jruby-complete dependency from the local/remote maven repository. it overwrites the jruby version from the dependencies if any. i.e. you can easily switch jruby version from the commandline !\ndefault: 1.7.12\nCommand line -Djruby.version=...", 3 );
append( sb, "", 0 );
append( sb, "launchDirectory (Default: ${project.basedir})", 2 );
append( sb, "the launch directory for the JRuby execution.\nCommand line -Djruby.launchDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "libDirectory (Default: lib)", 2 );
append( sb, "directory with ruby sources - added to ruby loadpath only\nCommand line -Djruby.lib=...", 3 );
append( sb, "", 0 );
append( sb, "plugin", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "reference to maven project for internal use.", 3 );
append( sb, "", 0 );
append( sb, "rubySourceDirectory (Default: src/main/ruby)", 2 );
append( sb, "directory with ruby sources - added to java classpath and ruby loadpath\nCommand line -Djruby.sourceDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "supportNative (Default: false)", 2 );
append( sb, "flag to indicate to setup jruby\'s native support for C-extensions\nCommand line -Dgem.supportNative=...", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "install".equals( goal ) )
{
append( sb, "gem:install", 0 );
append( sb, "goal to locally install a given gem", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "args", 2 );
append( sb, "common arguments\nCommand line -Dargs=...", 3 );
append( sb, "", 0 );
append( sb, "binDirectory", 2 );
append( sb, "directory of JRuby bin path to use when forking JRuby.\nCommand line -Dgem.binDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "env", 2 );
append( sb, "environment values passed on to the jruby process. needs jrubyFork true.\n", 3 );
append( sb, "", 0 );
append( sb, "gem (Default: ${gem})", 2 );
append( sb, "gem file to install locally.\nNote: this will install the gem in ${gem.home} so in general that is only useful if some other goal does something with it\nCommand line -Dgem=...", 3 );
append( sb, "", 0 );
append( sb, "gemHome (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of gem home to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.home=...", 3 );
append( sb, "", 0 );
append( sb, "gemHomes", 2 );
append( sb, "map different install locations for rubygems (GEM_HOME) to a directory. examples are the different scopes like provided and test as well when installed inside a plugin declaration, where the key is the artifactId of the plugin.\n", 3 );
append( sb, "", 0 );
append( sb, "gemPath (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of JRuby path to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.path=...", 3 );
append( sb, "", 0 );
append( sb, "gemUseSystem (Default: false)", 2 );
append( sb, "use system gems instead of setting up GemPath/GemHome inside the build directory and ignores any set gemHome and gemPath. you need to have both GEM_HOME and GEM_PATH environment variable set to make it work.\nCommand line -Dgem.useSystem=...", 3 );
append( sb, "", 0 );
append( sb, "includeGemsInResources", 2 );
append( sb, "EXPERIMENTAL this gives the scope of the gems which shall be included to resources. flag whether to include all gems to resources, i.e. to classpath or not. the difference to the includeRubygemsInResources is that it does not depend on rubygems during runtime since the required_path of the gems gets added to resources. note that it expect the required_path of the gem to be lib which is the default BUT that is not true for all gems. in this sense this feature is incomplete and might not work for you !!! IMPORTANT: it only adds the gems with provided scope since they are packed with the jar and then the pom.xml will not have them (since they are marked \'provided\') as transitive dependencies. this feature can be helpful in situations where the classloader does not work for rubygems due to rubygems uses file system globs to find the gems and this only works if the classloader reveals the jar url of its jars (i.e. URLClassLoader). for example OSGi classloader can not work with rubygems !!\nCommand line -Dgem.includeGemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeLibDirectoryInResources (Default: false)", 2 );
append( sb, "\nCommand line -Dgem.includeLibDirectoryInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeOpenSSL (Default: true)", 2 );
append( sb, "flag whether to include open-ssl gem or not\nCommand line -Dgem.includeOpenSSL=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInResources (Default: false)", 2 );
append( sb, "flag whether to include all gems to resources, i.e. to classpath or not\nCommand line -Dgem.includeRubygemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInTestResources (Default: true)", 2 );
append( sb, "flag whether to include all gems to test-resources, i.e. to test-classpath or not\nCommand line -Dgem.includeRubygemsInTestResources=...", 3 );
append( sb, "", 0 );
append( sb, "installArgs (Default: ${install.args})", 2 );
append( sb, "arguments for the \'gem install\' command.\nCommand line -Dinstall.args=...", 3 );
append( sb, "", 0 );
append( sb, "installRDoc (Default: false)", 2 );
append( sb, "flag whether to install rdocs of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "installRI (Default: false)", 2 );
append( sb, "flag whether to install ri of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyFork (Default: true)", 2 );
append( sb, "fork the JRuby execution.\nCommand line -Djruby.fork=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyJvmArgs", 2 );
append( sb, "jvm arguments for the java command executing jruby\nCommand line -Djruby.jvmargs=...", 3 );
append( sb, "", 0 );
append( sb, "jrubySwitches", 2 );
append( sb, "switches for the jruby command, like \'--1.9\'\nCommand line -Djruby.switches=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVerbose (Default: false)", 2 );
append( sb, "verbose jruby related output\nCommand line -Djruby.verbose=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVersion", 2 );
append( sb, "if the pom.xml has no runtime dependency to a jruby-complete.jar then this version is used to resolve the jruby-complete dependency from the local/remote maven repository. it overwrites the jruby version from the dependencies if any. i.e. you can easily switch jruby version from the commandline !\ndefault: 1.7.12\nCommand line -Djruby.version=...", 3 );
append( sb, "", 0 );
append( sb, "launchDirectory (Default: ${project.basedir})", 2 );
append( sb, "the launch directory for the JRuby execution.\nCommand line -Djruby.launchDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "libDirectory (Default: lib)", 2 );
append( sb, "directory with ruby sources - added to ruby loadpath only\nCommand line -Djruby.lib=...", 3 );
append( sb, "", 0 );
append( sb, "plugin", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "reference to maven project for internal use.", 3 );
append( sb, "", 0 );
append( sb, "rubySourceDirectory (Default: src/main/ruby)", 2 );
append( sb, "directory with ruby sources - added to java classpath and ruby loadpath\nCommand line -Djruby.sourceDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "supportNative (Default: false)", 2 );
append( sb, "flag to indicate to setup jruby\'s native support for C-extensions\nCommand line -Dgem.supportNative=...", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "irb".equals( goal ) )
{
append( sb, "gem:irb", 0 );
append( sb, "maven wrpper around IRB.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "args", 2 );
append( sb, "common arguments\nCommand line -Dargs=...", 3 );
append( sb, "", 0 );
append( sb, "binDirectory", 2 );
append( sb, "directory of JRuby bin path to use when forking JRuby.\nCommand line -Dgem.binDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "env", 2 );
append( sb, "environment values passed on to the jruby process. needs jrubyFork true.\n", 3 );
append( sb, "", 0 );
append( sb, "gemHome (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of gem home to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.home=...", 3 );
append( sb, "", 0 );
append( sb, "gemHomes", 2 );
append( sb, "map different install locations for rubygems (GEM_HOME) to a directory. examples are the different scopes like provided and test as well when installed inside a plugin declaration, where the key is the artifactId of the plugin.\n", 3 );
append( sb, "", 0 );
append( sb, "gemPath (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of JRuby path to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.path=...", 3 );
append( sb, "", 0 );
append( sb, "gemUseSystem (Default: false)", 2 );
append( sb, "use system gems instead of setting up GemPath/GemHome inside the build directory and ignores any set gemHome and gemPath. you need to have both GEM_HOME and GEM_PATH environment variable set to make it work.\nCommand line -Dgem.useSystem=...", 3 );
append( sb, "", 0 );
append( sb, "includeGemsInResources", 2 );
append( sb, "EXPERIMENTAL this gives the scope of the gems which shall be included to resources. flag whether to include all gems to resources, i.e. to classpath or not. the difference to the includeRubygemsInResources is that it does not depend on rubygems during runtime since the required_path of the gems gets added to resources. note that it expect the required_path of the gem to be lib which is the default BUT that is not true for all gems. in this sense this feature is incomplete and might not work for you !!! IMPORTANT: it only adds the gems with provided scope since they are packed with the jar and then the pom.xml will not have them (since they are marked \'provided\') as transitive dependencies. this feature can be helpful in situations where the classloader does not work for rubygems due to rubygems uses file system globs to find the gems and this only works if the classloader reveals the jar url of its jars (i.e. URLClassLoader). for example OSGi classloader can not work with rubygems !!\nCommand line -Dgem.includeGemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeLibDirectoryInResources (Default: false)", 2 );
append( sb, "\nCommand line -Dgem.includeLibDirectoryInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeOpenSSL (Default: true)", 2 );
append( sb, "flag whether to include open-ssl gem or not\nCommand line -Dgem.includeOpenSSL=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInResources (Default: false)", 2 );
append( sb, "flag whether to include all gems to resources, i.e. to classpath or not\nCommand line -Dgem.includeRubygemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInTestResources (Default: true)", 2 );
append( sb, "flag whether to include all gems to test-resources, i.e. to test-classpath or not\nCommand line -Dgem.includeRubygemsInTestResources=...", 3 );
append( sb, "", 0 );
append( sb, "installRDoc (Default: false)", 2 );
append( sb, "flag whether to install rdocs of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "installRI (Default: false)", 2 );
append( sb, "flag whether to install ri of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "irbArgs", 2 );
append( sb, "arguments for the irb command.\nCommand line -Dirb.args=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyFork (Default: true)", 2 );
append( sb, "fork the JRuby execution.\nCommand line -Djruby.fork=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyJvmArgs", 2 );
append( sb, "jvm arguments for the java command executing jruby\nCommand line -Djruby.jvmargs=...", 3 );
append( sb, "", 0 );
append( sb, "jrubySwitches", 2 );
append( sb, "switches for the jruby command, like \'--1.9\'\nCommand line -Djruby.switches=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVerbose (Default: false)", 2 );
append( sb, "verbose jruby related output\nCommand line -Djruby.verbose=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVersion", 2 );
append( sb, "if the pom.xml has no runtime dependency to a jruby-complete.jar then this version is used to resolve the jruby-complete dependency from the local/remote maven repository. it overwrites the jruby version from the dependencies if any. i.e. you can easily switch jruby version from the commandline !\ndefault: 1.7.12\nCommand line -Djruby.version=...", 3 );
append( sb, "", 0 );
append( sb, "launchDirectory (Default: ${project.basedir})", 2 );
append( sb, "the launch directory for the JRuby execution.\nCommand line -Djruby.launchDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "libDirectory (Default: lib)", 2 );
append( sb, "directory with ruby sources - added to ruby loadpath only\nCommand line -Djruby.lib=...", 3 );
append( sb, "", 0 );
append( sb, "plugin", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "reference to maven project for internal use.", 3 );
append( sb, "", 0 );
append( sb, "rubySourceDirectory (Default: src/main/ruby)", 2 );
append( sb, "directory with ruby sources - added to java classpath and ruby loadpath\nCommand line -Djruby.sourceDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "supportNative (Default: false)", 2 );
append( sb, "flag to indicate to setup jruby\'s native support for C-extensions\nCommand line -Dgem.supportNative=...", 3 );
append( sb, "", 0 );
append( sb, "swing (Default: false)", 2 );
append( sb, "launch IRB in a swing window.", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "package".equals( goal ) )
{
append( sb, "gem:package", 0 );
append( sb, "goal to convert that artifact into a gem or uses a given gemspec to build a gem.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "args", 2 );
append( sb, "common arguments\nCommand line -Dargs=...", 3 );
append( sb, "", 0 );
append( sb, "bindir", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "binDirectory", 2 );
append( sb, "directory of JRuby bin path to use when forking JRuby.\nCommand line -Dgem.binDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "buildDirectory", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "date", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "env", 2 );
append( sb, "environment values passed on to the jruby process. needs jrubyFork true.\n", 3 );
append( sb, "", 0 );
append( sb, "executables", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "extensions", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "extraFiles", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "extraRdocFiles", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "gemHome (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of gem home to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.home=...", 3 );
append( sb, "", 0 );
append( sb, "gemHomes", 2 );
append( sb, "map different install locations for rubygems (GEM_HOME) to a directory. examples are the different scopes like provided and test as well when installed inside a plugin declaration, where the key is the artifactId of the plugin.\n", 3 );
append( sb, "", 0 );
append( sb, "gemHook (Default: gem_hook.rb)", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "gemPath (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of JRuby path to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.path=...", 3 );
append( sb, "", 0 );
append( sb, "gemspec (Default: ${gemspec})", 2 );
append( sb, "the gemspec to use for building the gem\nCommand line -Dgemspec=...", 3 );
append( sb, "", 0 );
append( sb, "gemspecOverwrite (Default: ${gemspec.overwrite})", 2 );
append( sb, "use the pom to generate a gemspec and overwrite the one in lauchDirectory.\nCommand line -Dgemspec.overwrite=...", 3 );
append( sb, "", 0 );
append( sb, "gemUseSystem (Default: false)", 2 );
append( sb, "use system gems instead of setting up GemPath/GemHome inside the build directory and ignores any set gemHome and gemPath. you need to have both GEM_HOME and GEM_PATH environment variable set to make it work.\nCommand line -Dgem.useSystem=...", 3 );
append( sb, "", 0 );
append( sb, "includeDependencies (Default: false)", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "includeGemsInResources", 2 );
append( sb, "EXPERIMENTAL this gives the scope of the gems which shall be included to resources. flag whether to include all gems to resources, i.e. to classpath or not. the difference to the includeRubygemsInResources is that it does not depend on rubygems during runtime since the required_path of the gems gets added to resources. note that it expect the required_path of the gem to be lib which is the default BUT that is not true for all gems. in this sense this feature is incomplete and might not work for you !!! IMPORTANT: it only adds the gems with provided scope since they are packed with the jar and then the pom.xml will not have them (since they are marked \'provided\') as transitive dependencies. this feature can be helpful in situations where the classloader does not work for rubygems due to rubygems uses file system globs to find the gems and this only works if the classloader reveals the jar url of its jars (i.e. URLClassLoader). for example OSGi classloader can not work with rubygems !!\nCommand line -Dgem.includeGemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeLibDirectoryInResources (Default: false)", 2 );
append( sb, "\nCommand line -Dgem.includeLibDirectoryInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeOpenSSL (Default: true)", 2 );
append( sb, "flag whether to include open-ssl gem or not\nCommand line -Dgem.includeOpenSSL=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInResources (Default: false)", 2 );
append( sb, "flag whether to include all gems to resources, i.e. to classpath or not\nCommand line -Dgem.includeRubygemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInTestResources (Default: true)", 2 );
append( sb, "flag whether to include all gems to test-resources, i.e. to test-classpath or not\nCommand line -Dgem.includeRubygemsInTestResources=...", 3 );
append( sb, "", 0 );
append( sb, "installRDoc (Default: false)", 2 );
append( sb, "flag whether to install rdocs of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "installRI (Default: false)", 2 );
append( sb, "flag whether to install ri of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyFork (Default: true)", 2 );
append( sb, "fork the JRuby execution.\nCommand line -Djruby.fork=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyJvmArgs", 2 );
append( sb, "jvm arguments for the java command executing jruby\nCommand line -Djruby.jvmargs=...", 3 );
append( sb, "", 0 );
append( sb, "jrubySwitches", 2 );
append( sb, "switches for the jruby command, like \'--1.9\'\nCommand line -Djruby.switches=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVerbose (Default: false)", 2 );
append( sb, "verbose jruby related output\nCommand line -Djruby.verbose=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVersion", 2 );
append( sb, "if the pom.xml has no runtime dependency to a jruby-complete.jar then this version is used to resolve the jruby-complete dependency from the local/remote maven repository. it overwrites the jruby version from the dependencies if any. i.e. you can easily switch jruby version from the commandline !\ndefault: 1.7.12\nCommand line -Djruby.version=...", 3 );
append( sb, "", 0 );
append( sb, "launchDirectory (Default: ${project.basedir})", 2 );
append( sb, "the launch directory for the JRuby execution.\nCommand line -Djruby.launchDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "libDirectory (Default: lib)", 2 );
append( sb, "directory with ruby sources - added to ruby loadpath only\nCommand line -Djruby.lib=...", 3 );
append( sb, "", 0 );
append( sb, "platform", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "plugin", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "postInstallMessage", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "reference to maven project for internal use.", 3 );
append( sb, "", 0 );
append( sb, "rdocOptions", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "requiredRubygemsVersion", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "requiredRubyVersion", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "requirePaths", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "rubyforgeProject", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "rubygemsVersion", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "rubySourceDirectory (Default: src/main/ruby)", 2 );
append( sb, "directory with ruby sources - added to java classpath and ruby loadpath\nCommand line -Djruby.sourceDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "supportNative (Default: false)", 2 );
append( sb, "flag to indicate to setup jruby\'s native support for C-extensions\nCommand line -Dgem.supportNative=...", 3 );
append( sb, "", 0 );
append( sb, "useRepositoryLayout (Default: false)", 2 );
append( sb, "use repository layout for included dependencies", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "pom".equals( goal ) )
{
append( sb, "gem:pom", 0 );
append( sb, "goal to converts a gemspec file into pom.xml.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "args", 2 );
append( sb, "common arguments\nCommand line -Dargs=...", 3 );
append( sb, "", 0 );
append( sb, "env", 2 );
append( sb, "environment values passed on to the jruby process. needs jrubyFork true.\n", 3 );
append( sb, "", 0 );
append( sb, "force (Default: ${pom.force})", 2 );
append( sb, "force overwrite of an existing pom\nCommand line -Dpom.force=...", 3 );
append( sb, "", 0 );
append( sb, "gemfile (Default: Gemfile)", 2 );
append( sb, "use Gemfile to generate a pom\nCommand line -Dpom.gemfile=...", 3 );
append( sb, "", 0 );
append( sb, "gemspec (Default: ${pom.gemspec})", 2 );
append( sb, "use a gemspec file to generate a pom\nCommand line -Dpom.gemspec=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyFork (Default: true)", 2 );
append( sb, "fork the JRuby execution.\nCommand line -Djruby.fork=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyJvmArgs", 2 );
append( sb, "jvm arguments for the java command executing jruby\nCommand line -Djruby.jvmargs=...", 3 );
append( sb, "", 0 );
append( sb, "jrubySwitches", 2 );
append( sb, "switches for the jruby command, like \'--1.9\'\nCommand line -Djruby.switches=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVerbose (Default: false)", 2 );
append( sb, "verbose jruby related output\nCommand line -Djruby.verbose=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVersion", 2 );
append( sb, "if the pom.xml has no runtime dependency to a jruby-complete.jar then this version is used to resolve the jruby-complete dependency from the local/remote maven repository. it overwrites the jruby version from the dependencies if any. i.e. you can easily switch jruby version from the commandline !\ndefault: 1.7.12\nCommand line -Djruby.version=...", 3 );
append( sb, "", 0 );
append( sb, "launchDirectory (Default: ${project.basedir})", 2 );
append( sb, "the launch directory for the JRuby execution.\nCommand line -Djruby.launchDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "libDirectory (Default: lib)", 2 );
append( sb, "directory with ruby sources - added to ruby loadpath only\nCommand line -Djruby.lib=...", 3 );
append( sb, "", 0 );
append( sb, "plugin", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "pom (Default: pom.xml)", 2 );
append( sb, "the pom file to generate\nCommand line -Dpom=...", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "reference to maven project for internal use.", 3 );
append( sb, "", 0 );
append( sb, "rubySourceDirectory (Default: src/main/ruby)", 2 );
append( sb, "directory with ruby sources - added to java classpath and ruby loadpath\nCommand line -Djruby.sourceDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "skipGeneration", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "tmpPom (Default: ${project.build.directory}/pom.xml)", 2 );
append( sb, "temporary store generated pom.", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "push".equals( goal ) )
{
append( sb, "gem:push", 0 );
append( sb, "goal to push a given gem or a gem artifact to rubygems.org via the command \'gem push {gemfile}\'", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "args", 2 );
append( sb, "common arguments\nCommand line -Dargs=...", 3 );
append( sb, "", 0 );
append( sb, "binDirectory", 2 );
append( sb, "directory of JRuby bin path to use when forking JRuby.\nCommand line -Dgem.binDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "env", 2 );
append( sb, "environment values passed on to the jruby process. needs jrubyFork true.\n", 3 );
append( sb, "", 0 );
append( sb, "gem", 2 );
append( sb, "arguments for the ruby script given through file parameter.\nCommand line -Dgem=...", 3 );
append( sb, "", 0 );
append( sb, "gemHome (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of gem home to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.home=...", 3 );
append( sb, "", 0 );
append( sb, "gemHomes", 2 );
append( sb, "map different install locations for rubygems (GEM_HOME) to a directory. examples are the different scopes like provided and test as well when installed inside a plugin declaration, where the key is the artifactId of the plugin.\n", 3 );
append( sb, "", 0 );
append( sb, "gemPath (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of JRuby path to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.path=...", 3 );
append( sb, "", 0 );
append( sb, "gemUseSystem (Default: false)", 2 );
append( sb, "use system gems instead of setting up GemPath/GemHome inside the build directory and ignores any set gemHome and gemPath. you need to have both GEM_HOME and GEM_PATH environment variable set to make it work.\nCommand line -Dgem.useSystem=...", 3 );
append( sb, "", 0 );
append( sb, "includeGemsInResources", 2 );
append( sb, "EXPERIMENTAL this gives the scope of the gems which shall be included to resources. flag whether to include all gems to resources, i.e. to classpath or not. the difference to the includeRubygemsInResources is that it does not depend on rubygems during runtime since the required_path of the gems gets added to resources. note that it expect the required_path of the gem to be lib which is the default BUT that is not true for all gems. in this sense this feature is incomplete and might not work for you !!! IMPORTANT: it only adds the gems with provided scope since they are packed with the jar and then the pom.xml will not have them (since they are marked \'provided\') as transitive dependencies. this feature can be helpful in situations where the classloader does not work for rubygems due to rubygems uses file system globs to find the gems and this only works if the classloader reveals the jar url of its jars (i.e. URLClassLoader). for example OSGi classloader can not work with rubygems !!\nCommand line -Dgem.includeGemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeLibDirectoryInResources (Default: false)", 2 );
append( sb, "\nCommand line -Dgem.includeLibDirectoryInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeOpenSSL (Default: true)", 2 );
append( sb, "flag whether to include open-ssl gem or not\nCommand line -Dgem.includeOpenSSL=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInResources (Default: false)", 2 );
append( sb, "flag whether to include all gems to resources, i.e. to classpath or not\nCommand line -Dgem.includeRubygemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInTestResources (Default: true)", 2 );
append( sb, "flag whether to include all gems to test-resources, i.e. to test-classpath or not\nCommand line -Dgem.includeRubygemsInTestResources=...", 3 );
append( sb, "", 0 );
append( sb, "installRDoc (Default: false)", 2 );
append( sb, "flag whether to install rdocs of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "installRI (Default: false)", 2 );
append( sb, "flag whether to install ri of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyFork (Default: true)", 2 );
append( sb, "fork the JRuby execution.\nCommand line -Djruby.fork=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyJvmArgs", 2 );
append( sb, "jvm arguments for the java command executing jruby\nCommand line -Djruby.jvmargs=...", 3 );
append( sb, "", 0 );
append( sb, "jrubySwitches", 2 );
append( sb, "switches for the jruby command, like \'--1.9\'\nCommand line -Djruby.switches=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVerbose (Default: false)", 2 );
append( sb, "verbose jruby related output\nCommand line -Djruby.verbose=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVersion", 2 );
append( sb, "if the pom.xml has no runtime dependency to a jruby-complete.jar then this version is used to resolve the jruby-complete dependency from the local/remote maven repository. it overwrites the jruby version from the dependencies if any. i.e. you can easily switch jruby version from the commandline !\ndefault: 1.7.12\nCommand line -Djruby.version=...", 3 );
append( sb, "", 0 );
append( sb, "launchDirectory (Default: ${project.basedir})", 2 );
append( sb, "the launch directory for the JRuby execution.\nCommand line -Djruby.launchDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "libDirectory (Default: lib)", 2 );
append( sb, "directory with ruby sources - added to ruby loadpath only\nCommand line -Djruby.lib=...", 3 );
append( sb, "", 0 );
append( sb, "plugin", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "reference to maven project for internal use.", 3 );
append( sb, "", 0 );
append( sb, "pushArgs", 2 );
append( sb, "arguments for the ruby script given through file parameter.\nCommand line -Dpush.args=...", 3 );
append( sb, "", 0 );
append( sb, "rubySourceDirectory (Default: src/main/ruby)", 2 );
append( sb, "directory with ruby sources - added to java classpath and ruby loadpath\nCommand line -Djruby.sourceDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "skip", 2 );
append( sb, "skip the pushng the gem\nCommand line -Dpush.skipp=...", 3 );
append( sb, "", 0 );
append( sb, "supportNative (Default: false)", 2 );
append( sb, "flag to indicate to setup jruby\'s native support for C-extensions\nCommand line -Dgem.supportNative=...", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "sets".equals( goal ) )
{
append( sb, "gem:sets", 0 );
append( sb, "installs a set of given gems without resolving any transitive dependencies", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "args", 2 );
append( sb, "common arguments\nCommand line -Dargs=...", 3 );
append( sb, "", 0 );
append( sb, "binDirectory", 2 );
append( sb, "directory of JRuby bin path to use when forking JRuby.\nCommand line -Dgem.binDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "env", 2 );
append( sb, "environment values passed on to the jruby process. needs jrubyFork true.\n", 3 );
append( sb, "", 0 );
append( sb, "gemHome (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of gem home to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.home=...", 3 );
append( sb, "", 0 );
append( sb, "gemHomes", 2 );
append( sb, "map different install locations for rubygems (GEM_HOME) to a directory. examples are the different scopes like provided and test as well when installed inside a plugin declaration, where the key is the artifactId of the plugin.\n", 3 );
append( sb, "", 0 );
append( sb, "gemPath (Default: ${project.build.directory}/rubygems)", 2 );
append( sb, "directory of JRuby path to use when forking JRuby. default will be ignored when gemUseSystem is true.\nCommand line -Dgem.path=...", 3 );
append( sb, "", 0 );
append( sb, "gems", 2 );
append( sb, "map of gemname to version, i.e. it is a \'list\' of gems with fixed version", 3 );
append( sb, "", 0 );
append( sb, "gemUseSystem (Default: false)", 2 );
append( sb, "use system gems instead of setting up GemPath/GemHome inside the build directory and ignores any set gemHome and gemPath. you need to have both GEM_HOME and GEM_PATH environment variable set to make it work.\nCommand line -Dgem.useSystem=...", 3 );
append( sb, "", 0 );
append( sb, "includeGemsInResources", 2 );
append( sb, "EXPERIMENTAL this gives the scope of the gems which shall be included to resources. flag whether to include all gems to resources, i.e. to classpath or not. the difference to the includeRubygemsInResources is that it does not depend on rubygems during runtime since the required_path of the gems gets added to resources. note that it expect the required_path of the gem to be lib which is the default BUT that is not true for all gems. in this sense this feature is incomplete and might not work for you !!! IMPORTANT: it only adds the gems with provided scope since they are packed with the jar and then the pom.xml will not have them (since they are marked \'provided\') as transitive dependencies. this feature can be helpful in situations where the classloader does not work for rubygems due to rubygems uses file system globs to find the gems and this only works if the classloader reveals the jar url of its jars (i.e. URLClassLoader). for example OSGi classloader can not work with rubygems !!\nCommand line -Dgem.includeGemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeLibDirectoryInResources (Default: false)", 2 );
append( sb, "\nCommand line -Dgem.includeLibDirectoryInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeOpenSSL (Default: true)", 2 );
append( sb, "flag whether to include open-ssl gem or not\nCommand line -Dgem.includeOpenSSL=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInResources (Default: false)", 2 );
append( sb, "flag whether to include all gems to resources, i.e. to classpath or not\nCommand line -Dgem.includeRubygemsInResources=...", 3 );
append( sb, "", 0 );
append( sb, "includeRubygemsInTestResources (Default: true)", 2 );
append( sb, "flag whether to include all gems to test-resources, i.e. to test-classpath or not\nCommand line -Dgem.includeRubygemsInTestResources=...", 3 );
append( sb, "", 0 );
append( sb, "installRDoc (Default: false)", 2 );
append( sb, "flag whether to install rdocs of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "installRI (Default: false)", 2 );
append( sb, "flag whether to install ri of the used gems or not\nCommand line -Dgem.installRDoc=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyFork (Default: true)", 2 );
append( sb, "fork the JRuby execution.\nCommand line -Djruby.fork=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyJvmArgs", 2 );
append( sb, "jvm arguments for the java command executing jruby\nCommand line -Djruby.jvmargs=...", 3 );
append( sb, "", 0 );
append( sb, "jrubySwitches", 2 );
append( sb, "switches for the jruby command, like \'--1.9\'\nCommand line -Djruby.switches=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVerbose (Default: false)", 2 );
append( sb, "verbose jruby related output\nCommand line -Djruby.verbose=...", 3 );
append( sb, "", 0 );
append( sb, "jrubyVersion", 2 );
append( sb, "if the pom.xml has no runtime dependency to a jruby-complete.jar then this version is used to resolve the jruby-complete dependency from the local/remote maven repository. it overwrites the jruby version from the dependencies if any. i.e. you can easily switch jruby version from the commandline !\ndefault: 1.7.12\nCommand line -Djruby.version=...", 3 );
append( sb, "", 0 );
append( sb, "launchDirectory (Default: ${project.basedir})", 2 );
append( sb, "the launch directory for the JRuby execution.\nCommand line -Djruby.launchDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "libDirectory (Default: lib)", 2 );
append( sb, "directory with ruby sources - added to ruby loadpath only\nCommand line -Djruby.lib=...", 3 );
append( sb, "", 0 );
append( sb, "plugin", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "reference to maven project for internal use.", 3 );
append( sb, "", 0 );
append( sb, "rubySourceDirectory (Default: src/main/ruby)", 2 );
append( sb, "directory with ruby sources - added to java classpath and ruby loadpath\nCommand line -Djruby.sourceDirectory=...", 3 );
append( sb, "", 0 );
append( sb, "scope (Default: compile)", 2 );
append( sb, "the scope under which the gems get installed", 3 );
append( sb, "", 0 );
append( sb, "supportNative (Default: false)", 2 );
append( sb, "flag to indicate to setup jruby\'s native support for C-extensions\nCommand line -Dgem.supportNative=...", 3 );
append( sb, "", 0 );
}
}
if ( getLog().isInfoEnabled() )
{
getLog().info( sb.toString() );
}
}
/**
* Repeat a String n
times to form a new string.
*
* @param str String to repeat
* @param repeat number of times to repeat str
* @return String with repeated String
* @throws NegativeArraySizeException if repeat < 0
* @throws NullPointerException if str is null
*/
private static String repeat( String str, int repeat )
{
StringBuffer buffer = new StringBuffer( repeat * str.length() );
for ( int i = 0; i < repeat; i++ )
{
buffer.append( str );
}
return buffer.toString();
}
/**
* Append a description to the buffer by respecting the indentSize and lineLength parameters.
* Note: The last character is always a new line.
*
* @param sb The buffer to append the description, not null
.
* @param description The description, not null
.
* @param indent The base indentation level of each line, must not be negative.
*/
private void append( StringBuffer sb, String description, int indent )
{
for ( Iterator it = toLines( description, indent, indentSize, lineLength ).iterator(); it.hasNext(); )
{
sb.append( it.next().toString() ).append( '\n' );
}
}
/**
* Splits the specified text into lines of convenient display length.
*
* @param text The text to split into lines, must not be null
.
* @param indent The base indentation level of each line, must not be negative.
* @param indentSize The size of each indentation, must not be negative.
* @param lineLength The length of the line, must not be negative.
* @return The sequence of display lines, never null
.
* @throws NegativeArraySizeException if indent < 0
*/
private static List toLines( String text, int indent, int indentSize, int lineLength )
{
List lines = new ArrayList();
String ind = repeat( "\t", indent );
String[] plainLines = text.split( "(\r\n)|(\r)|(\n)" );
for ( int i = 0; i < plainLines.length; i++ )
{
toLines( lines, ind + plainLines[i], indentSize, lineLength );
}
return lines;
}
/**
* Adds the specified line to the output sequence, performing line wrapping if necessary.
*
* @param lines The sequence of display lines, must not be null
.
* @param line The line to add, must not be null
.
* @param indentSize The size of each indentation, must not be negative.
* @param lineLength The length of the line, must not be negative.
*/
private static void toLines( List lines, String line, int indentSize, int lineLength )
{
int lineIndent = getIndentLevel( line );
StringBuffer buf = new StringBuffer( 256 );
String[] tokens = line.split( " +" );
for ( int i = 0; i < tokens.length; i++ )
{
String token = tokens[i];
if ( i > 0 )
{
if ( buf.length() + token.length() >= lineLength )
{
lines.add( buf.toString() );
buf.setLength( 0 );
buf.append( repeat( " ", lineIndent * indentSize ) );
}
else
{
buf.append( ' ' );
}
}
for ( int j = 0; j < token.length(); j++ )
{
char c = token.charAt( j );
if ( c == '\t' )
{
buf.append( repeat( " ", indentSize - buf.length() % indentSize ) );
}
else if ( c == '\u00A0' )
{
buf.append( ' ' );
}
else
{
buf.append( c );
}
}
}
lines.add( buf.toString() );
}
/**
* Gets the indentation level of the specified line.
*
* @param line The line whose indentation level should be retrieved, must not be null
.
* @return The indentation level of the line.
*/
private static int getIndentLevel( String line )
{
int level = 0;
for ( int i = 0; i < line.length() && line.charAt( i ) == '\t'; i++ )
{
level++;
}
for ( int i = level + 1; i <= level + 4 && i < line.length(); i++ )
{
if ( line.charAt( i ) == '\t' )
{
level++;
break;
}
}
return level;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy