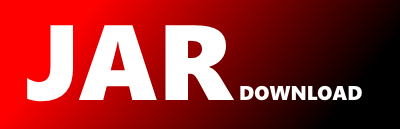
ftl.FreshMarkerParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of freshmarker Show documentation
Show all versions of freshmarker Show documentation
A simple, small but powerful template engine based loosely on the FreeMarker syntax. FreshMarker is
implemented in Java 21 and supports the `java.time` API and Records.
/* Generated by: CongoCC Parser Generator. FreshMarkerParser.java */
package ftl;
import java.util.*;
import java.io.*;
import java.io.IOException;
import java.io.PrintStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.charset.Charset;
import java.util.Arrays;
import java.util.ArrayList;
import java.util.Collections;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.ListIterator;
import java.util.concurrent.CancellationException;
import ftl.Token.TokenType;
import static ftl.Token.TokenType.*;
import ftl.ast.Expression;
import ftl.ast.OrExpression;
import ftl.ast.AndExpression;
import ftl.ast.EqualityExpression;
import ftl.ast.RelationalExpression;
import ftl.ast.RangeExpression;
import ftl.ast.AdditiveExpression;
import ftl.ast.MultiplicativeExpression;
import ftl.ast.UnaryPlusMinusExpression;
import ftl.ast.NotExpression;
import ftl.ast.BuiltinVariable;
import ftl.ast.DefaultToExpression;
import ftl.ast.PrimaryExpression;
import ftl.ast.BaseExpression;
import ftl.ast.DotKey;
import ftl.ast.DynamicKey;
import ftl.ast.MethodInvoke;
import ftl.ast.BuiltIn;
import ftl.ast.Exists;
import ftl.ast.ListLiteral;
import ftl.ast.StringLiteral;
import ftl.ast.HashLiteral;
import ftl.ast.NumberLiteral;
import ftl.ast.BooleanLiteral;
import ftl.ast.NullLiteral;
import ftl.ast.Parenthesis;
import ftl.ast.NamedArgsList;
import ftl.ast.PositionalArgsList;
import ftl.ast.IdentifierOrStringLiteral;
import ftl.ast.Interpolation;
import ftl.ast.Assignment;
import ftl.ast.VarInstruction;
import ftl.ast.SettingInstruction;
import ftl.ast.CommentBlock;
import ftl.ast.BreakInstruction;
import ftl.ast.ReturnInstruction;
import ftl.ast.OutputFormatBlock;
import ftl.ast.ImportInstruction;
import ftl.ast.IfStatement;
import ftl.ast.ElseIfBlock;
import ftl.ast.ElseBlock;
import ftl.ast.SwitchInstruction;
import ftl.ast.CaseInstruction;
import ftl.ast.DefaultInstruction;
import ftl.ast.ListInstruction;
import ftl.ast.NestedInstruction;
import ftl.ast.MacroDefinition;
import ftl.ast.ParameterList;
import ftl.ast.LooseTagEnd;
import ftl.ast.DirectiveEnd;
import ftl.ast.Text;
import ftl.ast.UserDirective;
import ftl.ast.Block;
import ftl.ast.Root;
public class FreshMarkerParser {
static final int UNLIMITED = Integer.MAX_VALUE;
// The last token successfully "consumed"
Token lastConsumedToken;
private TokenType nextTokenType;
// Normally null when parsing, populated when doing lookahead
private Token currentLookaheadToken;
private int remainingLookahead;
private boolean hitFailure;
private boolean passedPredicate;
private int passedPredicateThreshold = -1;
private String currentlyParsedProduction;
private String currentLookaheadProduction;
private int lookaheadRoutineNesting;
private final boolean legacyGlitchyLookahead = false;
private final Token DUMMY_START_TOKEN = new Token();
private boolean cancelled;
public void cancel() {
cancelled = true;
}
public boolean isCancelled() {
return cancelled;
}
/** Generated Lexer. */
private FreshMarkerLexer token_source;
public void setInputSource(String inputSource) {
token_source.setInputSource(inputSource);
}
String getInputSource() {
return token_source.getInputSource();
}
//=================================
// Generated constructors
//=================================
public FreshMarkerParser(String inputSource, CharSequence content) {
this(new FreshMarkerLexer(inputSource, content));
}
public FreshMarkerParser(CharSequence content) {
this("input", content);
}
/**
* @param inputSource just the name of the input source (typically the filename) that
* will be used in error messages and so on.
* @param path The location (typically the filename) from which to get the input to parse
*/
public FreshMarkerParser(String inputSource, Path path) throws IOException {
this(inputSource, TokenSource.stringFromBytes(Files.readAllBytes(path)));
}
public FreshMarkerParser(String inputSource, Path path, Charset charset) throws IOException {
this(inputSource, TokenSource.stringFromBytes(Files.readAllBytes(path), charset));
}
/**
* @param path The location (typically the filename) from which to get the input to parse
*/
public FreshMarkerParser(Path path) throws IOException {
this(path.toString(), path);
}
/** Constructor with user supplied Lexer. */
public FreshMarkerParser(FreshMarkerLexer lexer) {
token_source = lexer;
lastConsumedToken = DUMMY_START_TOKEN;
lastConsumedToken.setTokenSource(lexer);
}
/**
* Set the starting line/column for location reporting.
* By default, this is 1,1.
*/
public void setStartingPos(int startingLine, int startingColumn) {
token_source.setStartingPos(startingLine, startingColumn);
}
// this method is for testing only.
public boolean getLegacyGlitchyLookahead() {
return legacyGlitchyLookahead;
}
// If the next token is cached, it returns that
// Otherwise, it goes to the token_source, i.e. the Lexer.
private Token nextToken(final Token tok) {
Token result = token_source.getNextToken(tok);
while (result.isUnparsed()) {
result = token_source.getNextToken(result);
}
nextTokenType = null;
return result;
}
/**
* @return the next Token off the stream. This is the same as #getToken(1)
*/
public final Token getNextToken() {
return getToken(1);
}
/**
* @param index how many tokens to look ahead
* @return the specific regular (i.e. parsed) Token index ahead/behind in the stream.
* If we are in a lookahead, it looks ahead from the currentLookaheadToken
* Otherwise, it is the lastConsumedToken. If you pass in a negative
* number it goes backward.
*/
public final Token getToken(final int index) {
Token t = currentLookaheadToken == null ? lastConsumedToken : currentLookaheadToken;
for (int i = 0; i < index; i++) {
t = nextToken(t);
}
for (int i = 0; i > index; i--) {
t = t.getPrevious();
if (t == null) break;
}
return t;
}
private TokenType nextTokenType() {
if (nextTokenType == null) {
nextTokenType = nextToken(lastConsumedToken).getType();
}
return nextTokenType;
}
boolean activateTokenTypes(TokenType...types) {
if (token_source.activeTokenTypes == null) return false;
boolean result = false;
for (TokenType tt : types) {
result |= token_source.activeTokenTypes.add(tt);
}
if (result) {
token_source.reset(getToken(0));
nextTokenType = null;
}
return result;
}
boolean deactivateTokenTypes(TokenType...types) {
boolean result = false;
if (token_source.activeTokenTypes == null) {
token_source.activeTokenTypes = EnumSet.allOf(TokenType.class);
}
for (TokenType tt : types) {
result |= token_source.activeTokenTypes.remove(tt);
}
if (result) {
token_source.reset(getToken(0));
nextTokenType = null;
}
return result;
}
private static final HashMap> enumSetCache = new HashMap<>();
private static EnumSet tokenTypeSet(TokenType first, TokenType...rest) {
TokenType[] key = new TokenType[1 + rest.length];
key[0] = first;
if (rest.length > 0) {
System.arraycopy(rest, 0, key, 1, rest.length);
}
Arrays.sort(key);
if (enumSetCache.containsKey(key)) {
return enumSetCache.get(key);
}
EnumSet result = (rest.length == 0) ? EnumSet.of(first) : EnumSet.of(first, rest);
enumSetCache.put(key, result);
return result;
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:179:1
final public Node Input() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Input";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:179:1
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:180:4
pushOntoCallStack("Input", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 180, 4);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:181:4
consumeToken(EOF);
// Code for CodeBlock specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:182:4
return rootNode();
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:187:1
final public void Expression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Expression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:187:1
Expression thisProduction = null;
if (buildTree) {
thisProduction = new Expression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:188:5
pushOntoCallStack("Expression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 188, 5);
try {
OrExpression();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:191:1
final public void OrExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "OrExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:191:1
OrExpression thisProduction = null;
if (buildTree) {
thisProduction = new OrExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:192:5
pushOntoCallStack("OrExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 192, 5);
try {
AndExpression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:193:5
while (true) {
if (!(nextTokenType() == OR || nextTokenType == XOR || nextTokenType == OR2)) break;
if (nextTokenType() == OR) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:194:10
consumeToken(OR);
} else if (nextTokenType() == OR2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:194:15
consumeToken(OR2);
} else if (nextTokenType() == XOR) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:194:21
consumeToken(XOR);
} else {
pushOntoCallStack("OrExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 194, 10);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$194$10, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:194:28
pushOntoCallStack("OrExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 194, 28);
try {
AndExpression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:198:1
final public void AndExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "AndExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:198:1
AndExpression thisProduction = null;
if (buildTree) {
thisProduction = new AndExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:199:5
pushOntoCallStack("AndExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 199, 5);
try {
EqualityExpression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:200:5
while (true) {
if (!(nextTokenType() == AND || nextTokenType == AND2)) break;
if (nextTokenType() == AND) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:201:10
consumeToken(AND);
} else if (nextTokenType() == AND2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:201:16
consumeToken(AND2);
} else {
pushOntoCallStack("AndExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 201, 10);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$201$10, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:201:24
pushOntoCallStack("AndExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 201, 24);
try {
EqualityExpression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:205:1
final public void EqualityExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "EqualityExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:205:1
EqualityExpression thisProduction = null;
if (buildTree) {
thisProduction = new EqualityExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:206:5
pushOntoCallStack("EqualityExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 206, 5);
try {
RelationalExpression();
} finally {
popCallStack();
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:207:5
if (nextTokenType() == EQUALS || nextTokenType == DOUBLE_EQUALS || nextTokenType == NOT_EQUALS) {
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:208:10
consumeToken(EQUALS);
} else if (nextTokenType() == DOUBLE_EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:208:19
consumeToken(DOUBLE_EQUALS);
} else if (nextTokenType() == NOT_EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:208:35
consumeToken(NOT_EQUALS);
} else {
pushOntoCallStack("EqualityExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 208, 10);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$208$10, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:209:9
pushOntoCallStack("EqualityExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 209, 9);
try {
RelationalExpression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:213:1
final public void RelationalExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "RelationalExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:213:1
RelationalExpression thisProduction = null;
if (buildTree) {
thisProduction = new RelationalExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:214:5
pushOntoCallStack("RelationalExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 214, 5);
try {
RangeExpression();
} finally {
popCallStack();
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:215:5
if (first_set$FEL_ccc$216$9.contains(nextTokenType())) {
if (nextTokenType() == GT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:216:10
consumeToken(GT);
} else if (nextTokenType() == GTE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:216:15
consumeToken(GTE);
} else if (nextTokenType() == LT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:216:21
consumeToken(LT);
} else if (nextTokenType() == LTE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:216:26
consumeToken(LTE);
} else if (nextTokenType() == ALT_GT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:216:32
consumeToken(ALT_GT);
} else if (nextTokenType() == ALT_GTE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:216:41
consumeToken(ALT_GTE);
} else if (nextTokenType() == ALT_LTE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:216:51
consumeToken(ALT_LTE);
} else if (nextTokenType() == ALT_LT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:216:61
consumeToken(ALT_LT);
} else {
pushOntoCallStack("RelationalExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 216, 10);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$216$10, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:217:9
pushOntoCallStack("RelationalExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 217, 9);
try {
RangeExpression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:221:1
final public void RangeExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "RangeExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:221:1
RangeExpression thisProduction = null;
if (buildTree) {
thisProduction = new RangeExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:222:5
pushOntoCallStack("RangeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 222, 5);
try {
AdditiveExpression();
} finally {
popCallStack();
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:223:5
if (nextTokenType() == DOT_DOT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:224:8
consumeToken(DOT_DOT);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:225:8
if (scan$FEL_ccc$226$11()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:226:11
pushOntoCallStack("RangeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 226, 11);
try {
AdditiveExpression();
} finally {
popCallStack();
}
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:231:1
final public void AdditiveExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "AdditiveExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:231:1
AdditiveExpression thisProduction = null;
if (buildTree) {
thisProduction = new AdditiveExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:232:5
pushOntoCallStack("AdditiveExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 232, 5);
try {
MultiplicativeExpression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:233:5
while (true) {
if (!(nextTokenType() == PLUS || nextTokenType == MINUS)) break;
if (nextTokenType() == PLUS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:234:13
consumeToken(PLUS);
} else if (nextTokenType() == MINUS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:234:20
consumeToken(MINUS);
} else {
pushOntoCallStack("AdditiveExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 234, 13);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$234$13, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:235:12
pushOntoCallStack("AdditiveExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 235, 12);
try {
MultiplicativeExpression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:239:1
final public void MultiplicativeExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "MultiplicativeExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:239:1
MultiplicativeExpression thisProduction = null;
if (buildTree) {
thisProduction = new MultiplicativeExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:240:5
pushOntoCallStack("MultiplicativeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 240, 5);
try {
UnaryExpression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:241:5
while (true) {
if (!(nextTokenType() == TIMES || nextTokenType == DIVIDE || nextTokenType == PERCENT)) break;
if (nextTokenType() == TIMES) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:242:12
consumeToken(TIMES);
} else if (nextTokenType() == DIVIDE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:242:20
consumeToken(DIVIDE);
} else if (nextTokenType() == PERCENT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:242:29
consumeToken(PERCENT);
} else {
pushOntoCallStack("MultiplicativeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 242, 12);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$242$12, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:243:11
pushOntoCallStack("MultiplicativeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 243, 11);
try {
UnaryExpression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
private static final EnumSet UnaryExpression_FIRST_SET = UnaryExpression_FIRST_SET_init();
private static EnumSet UnaryExpression_FIRST_SET_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:248:1
final public void UnaryExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "UnaryExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:248:1
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:249:5
if (nextTokenType() == PLUS || nextTokenType == MINUS) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:249:5
pushOntoCallStack("UnaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 249, 5);
try {
UnaryPlusMinusExpression();
} finally {
popCallStack();
}
} else if (nextTokenType() == EXCLAM) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:251:5
pushOntoCallStack("UnaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 251, 5);
try {
NotExpression();
} finally {
popCallStack();
}
} else if (first_set$FEL_ccc$253$5.contains(nextTokenType())) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:253:5
pushOntoCallStack("UnaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 253, 5);
try {
DefaultToExpression();
} finally {
popCallStack();
}
} else {
pushOntoCallStack("UnaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 249, 5);
throw new ParseException(lastConsumedToken, UnaryExpression_FIRST_SET, parsingStack);
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:256:1
final public void UnaryPlusMinusExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "UnaryPlusMinusExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:256:1
UnaryPlusMinusExpression thisProduction = null;
if (buildTree) {
thisProduction = new UnaryPlusMinusExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == PLUS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:257:6
consumeToken(PLUS);
} else if (nextTokenType() == MINUS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:257:13
consumeToken(MINUS);
} else {
pushOntoCallStack("UnaryPlusMinusExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 257, 6);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$257$6, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:257:22
pushOntoCallStack("UnaryPlusMinusExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 257, 22);
try {
DefaultToExpression();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:260:1
final public void NotExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "NotExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:260:1
NotExpression thisProduction = null;
if (buildTree) {
thisProduction = new NotExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:261:5
consumeToken(EXCLAM);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:262:5
pushOntoCallStack("NotExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 262, 5);
try {
DefaultToExpression();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:265:1
final public void BuiltinVariable() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "BuiltinVariable";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:265:1
BuiltinVariable thisProduction = null;
if (buildTree) {
thisProduction = new BuiltinVariable();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:266:5
consumeToken(DOT);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:266:10
consumeToken(IDENTIFIER);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:269:1
final public void DefaultToExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "DefaultToExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:269:1
DefaultToExpression thisProduction = null;
if (buildTree) {
thisProduction = new DefaultToExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:270:5
pushOntoCallStack("DefaultToExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 270, 5);
try {
PrimaryExpression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:271:5
while (true) {
if (!((getToken(2).getType() != IDENTIFIER || getToken(3).getType() != EQUALS) && scan$FEL_ccc$273$9())) break;
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:274:12
consumeToken(EXCLAM);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:274:20
pushOntoCallStack("DefaultToExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 274, 20);
try {
PrimaryExpression();
} finally {
popCallStack();
}
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:276:5
if (nextTokenType() == EXCLAM) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:277:8
consumeToken(EXCLAM);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:281:1
final public void PrimaryExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "PrimaryExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:281:1
PrimaryExpression thisProduction = null;
if (buildTree) {
thisProduction = new PrimaryExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:282:3
pushOntoCallStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 282, 3);
try {
BaseExpression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:283:3
while (true) {
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:284:7
if (nextTokenType() == DOT) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:284:7
pushOntoCallStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 284, 7);
try {
DotKey();
} finally {
popCallStack();
}
} else if (nextTokenType() == DOUBLE_COLON) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:286:7
pushOntoCallStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 286, 7);
try {
MethodReference();
} finally {
popCallStack();
}
} else if (nextTokenType() == OPEN_BRACKET) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:288:7
pushOntoCallStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 288, 7);
try {
DynamicKey();
} finally {
popCallStack();
}
} else if (nextTokenType() == OPEN_PAREN) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:290:7
pushOntoCallStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 290, 7);
try {
MethodInvoke();
} finally {
popCallStack();
}
} else if (nextTokenType() == BUILT_IN) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:292:7
pushOntoCallStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 292, 7);
try {
BuiltIn();
} finally {
popCallStack();
}
} else if (nextTokenType() == EXISTS_OPERATOR) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:294:7
pushOntoCallStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 294, 7);
try {
Exists();
} finally {
popCallStack();
}
} else {
break;
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
private static final EnumSet BaseExpression_FIRST_SET = BaseExpression_FIRST_SET_init();
private static EnumSet BaseExpression_FIRST_SET_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:298:1
final public void BaseExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "BaseExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:298:1
BaseExpression thisProduction = null;
if (buildTree) {
thisProduction = new BaseExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:299:5
if (nextTokenType() == IDENTIFIER) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:299:5
consumeToken(IDENTIFIER);
} else if (nextTokenType() == INTEGER || nextTokenType == DECIMAL) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:301:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 301, 5);
try {
NumberLiteral();
} finally {
popCallStack();
}
} else if (nextTokenType() == OPEN_BRACE) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:303:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 303, 5);
try {
HashLiteral();
} finally {
popCallStack();
}
} else if (nextTokenType() == STRING_LITERAL) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:305:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 305, 5);
try {
StringLiteral();
} finally {
popCallStack();
}
} else if (nextTokenType() == TRUE || nextTokenType == FALSE) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:307:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 307, 5);
try {
BooleanLiteral();
} finally {
popCallStack();
}
} else if (nextTokenType() == NULL) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:309:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 309, 5);
try {
NullLiteral();
} finally {
popCallStack();
}
} else if (nextTokenType() == OPEN_BRACKET) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:311:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 311, 5);
try {
ListLiteral();
} finally {
popCallStack();
}
} else if (nextTokenType() == OPEN_PAREN) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:313:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 313, 5);
try {
Parenthesis();
} finally {
popCallStack();
}
} else if (nextTokenType() == DOT) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:315:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 315, 5);
try {
BuiltinVariable();
} finally {
popCallStack();
}
} else {
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 299, 5);
throw new ParseException(lastConsumedToken, BaseExpression_FIRST_SET, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:319:1
final public void DotKey() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "DotKey";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:319:1
DotKey thisProduction = null;
if (buildTree) {
thisProduction = new DotKey();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:320:5
consumeToken(DOT);
if (nextTokenType() == IDENTIFIER) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:321:6
consumeToken(IDENTIFIER);
} else if (nextTokenType() == TIMES) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:321:19
consumeToken(TIMES);
} else {
pushOntoCallStack("DotKey", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 321, 6);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$321$6, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:324:1
final public void MethodReference() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "MethodReference";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:324:1
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:325:5
consumeToken(DOUBLE_COLON);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:326:5
consumeToken(IDENTIFIER);
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:329:1
final public void DynamicKey() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "DynamicKey";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:329:1
DynamicKey thisProduction = null;
if (buildTree) {
thisProduction = new DynamicKey();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:330:5
consumeToken(OPEN_BRACKET);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:330:19
pushOntoCallStack("DynamicKey", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 330, 19);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:330:29
consumeToken(CLOSE_BRACKET);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:333:1
final public void MethodInvoke() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "MethodInvoke";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:333:1
MethodInvoke thisProduction = null;
if (buildTree) {
thisProduction = new MethodInvoke();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:334:3
consumeToken(OPEN_PAREN);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:334:15
if (first_set$FEL_ccc$334$16.contains(nextTokenType())) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:334:16
pushOntoCallStack("MethodInvoke", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 334, 16);
try {
ArgsList();
} finally {
popCallStack();
}
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:334:25
consumeToken(CLOSE_PAREN);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:337:1
final public void BuiltIn() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "BuiltIn";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:337:1
BuiltIn thisProduction = null;
if (buildTree) {
thisProduction = new BuiltIn();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:5
consumeToken(BUILT_IN);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:15
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:27
if (nextTokenType() == OPEN_PAREN) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:28
consumeToken(OPEN_PAREN);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:40
if (first_set$FEL_ccc$338$41.contains(nextTokenType())) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:41
pushOntoCallStack("BuiltIn", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 338, 41);
try {
ArgsList();
} finally {
popCallStack();
}
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:50
consumeToken(CLOSE_PAREN);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:341:1
final public void Exists() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Exists";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:341:1
Exists thisProduction = null;
if (buildTree) {
thisProduction = new Exists();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:342:5
consumeToken(EXISTS_OPERATOR);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:345:1
final public void ListLiteral() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "ListLiteral";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:345:1
ListLiteral thisProduction = null;
if (buildTree) {
thisProduction = new ListLiteral();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:346:5
consumeToken(OPEN_BRACKET);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:5
if (first_set$FEL_ccc$347$6.contains(nextTokenType())) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:6
pushOntoCallStack("ListLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 347, 6);
try {
Expression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:17
while (true) {
if (!(first_set$FEL_ccc$347$18.contains(nextTokenType()))) break;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:18
if (nextTokenType() == COMMA) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:19
consumeToken(COMMA);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:27
pushOntoCallStack("ListLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 347, 27);
try {
Expression();
} finally {
popCallStack();
}
}
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:5
consumeToken(CLOSE_BRACKET);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:351:1
final public void StringLiteral() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "StringLiteral";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:351:1
StringLiteral thisProduction = null;
if (buildTree) {
thisProduction = new StringLiteral();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:352:5
consumeToken(STRING_LITERAL);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:356:1
final public void HashLiteral() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "HashLiteral";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:356:1
HashLiteral thisProduction = null;
if (buildTree) {
thisProduction = new HashLiteral();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:357:5
consumeToken(OPEN_BRACE);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:358:5
if (first_set$FEL_ccc$359$8.contains(nextTokenType())) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:359:8
pushOntoCallStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 359, 8);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:359:19
consumeToken(COLON);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:359:27
pushOntoCallStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 359, 27);
try {
Expression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:8
while (true) {
if (!(nextTokenType() == COMMA)) break;
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:9
consumeToken(COMMA);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:17
pushOntoCallStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 360, 17);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:28
consumeToken(COLON);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:36
pushOntoCallStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 360, 36);
try {
Expression();
} finally {
popCallStack();
}
}
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:362:5
consumeToken(CLOSE_BRACE);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
private static final EnumSet NumberLiteral_FIRST_SET = tokenTypeSet(INTEGER, DECIMAL);
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:365:1
final public void NumberLiteral() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "NumberLiteral";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:365:1
NumberLiteral thisProduction = null;
if (buildTree) {
thisProduction = new NumberLiteral();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:366:5
if (nextTokenType() == INTEGER) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:366:5
consumeToken(INTEGER);
} else if (nextTokenType() == DECIMAL) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:366:15
consumeToken(DECIMAL);
} else {
pushOntoCallStack("NumberLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 366, 5);
throw new ParseException(lastConsumedToken, NumberLiteral_FIRST_SET, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
private static final EnumSet BooleanLiteral_FIRST_SET = tokenTypeSet(TRUE, FALSE);
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:369:1
final public void BooleanLiteral() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "BooleanLiteral";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:369:1
BooleanLiteral thisProduction = null;
if (buildTree) {
thisProduction = new BooleanLiteral();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:370:5
if (nextTokenType() == TRUE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:370:5
consumeToken(TRUE);
} else if (nextTokenType() == FALSE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:370:12
consumeToken(FALSE);
} else {
pushOntoCallStack("BooleanLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 370, 5);
throw new ParseException(lastConsumedToken, BooleanLiteral_FIRST_SET, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:373:1
final public void NullLiteral() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "NullLiteral";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:373:1
NullLiteral thisProduction = null;
if (buildTree) {
thisProduction = new NullLiteral();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:373:15
consumeToken(NULL);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:375:1
final public void Parenthesis() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Parenthesis";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:375:1
Parenthesis thisProduction = null;
if (buildTree) {
thisProduction = new Parenthesis();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:376:5
consumeToken(OPEN_PAREN);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:376:18
pushOntoCallStack("Parenthesis", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 376, 18);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:376:29
consumeToken(CLOSE_PAREN);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:379:1
final public void ArgsList() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "ArgsList";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:379:1
if (scan$FEL_ccc$381$9()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:382:9
pushOntoCallStack("ArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 382, 9);
try {
NamedArgsList();
} finally {
popCallStack();
}
} else if (first_set$FEL_ccc$384$9.contains(nextTokenType())) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:384:9
pushOntoCallStack("ArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 384, 9);
try {
PositionalArgsList();
} finally {
popCallStack();
}
} else {
pushOntoCallStack("ArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 381, 9);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$381$9, parsingStack);
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:388:1
final public void NamedArgsList() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "NamedArgsList";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:388:1
NamedArgsList thisProduction = null;
if (buildTree) {
thisProduction = new NamedArgsList();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:389:5
consumeToken(IDENTIFIER);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:390:5
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:391:5
pushOntoCallStack("NamedArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 391, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:392:5
while (true) {
if (!(nextTokenType() == COMMA || nextTokenType == IDENTIFIER)) break;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:393:8
if (nextTokenType() == COMMA) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:393:9
consumeToken(COMMA);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:394:8
consumeToken(IDENTIFIER);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:395:8
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:396:8
pushOntoCallStack("NamedArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 396, 8);
try {
Expression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:400:1
final public void PositionalArgsList() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "PositionalArgsList";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:400:1
PositionalArgsList thisProduction = null;
if (buildTree) {
thisProduction = new PositionalArgsList();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:401:5
pushOntoCallStack("PositionalArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 401, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:402:5
while (true) {
if (!(first_set$FEL_ccc$403$8.contains(nextTokenType()))) break;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:403:8
if (nextTokenType() == COMMA) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:403:9
consumeToken(COMMA);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:404:8
pushOntoCallStack("PositionalArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 404, 8);
try {
Expression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
private static final EnumSet IdentifierOrStringLiteral_FIRST_SET = tokenTypeSet(IDENTIFIER, STRING_LITERAL);
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:233:1
final public void IdentifierOrStringLiteral() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "IdentifierOrStringLiteral";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:233:1
IdentifierOrStringLiteral thisProduction = null;
if (buildTree) {
thisProduction = new IdentifierOrStringLiteral();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:234:5
if (nextTokenType() == IDENTIFIER) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:234:5
consumeToken(IDENTIFIER);
} else if (nextTokenType() == STRING_LITERAL) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:236:5
pushOntoCallStack("IdentifierOrStringLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 236, 5);
try {
StringLiteral();
} finally {
popCallStack();
}
} else {
pushOntoCallStack("IdentifierOrStringLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 234, 5);
throw new ParseException(lastConsumedToken, IdentifierOrStringLiteral_FIRST_SET, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:239:1
final public void Interpolation() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Interpolation";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:239:1
Interpolation thisProduction = null;
if (buildTree) {
thisProduction = new Interpolation();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:240:5
consumeToken(INTERPOLATE);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:240:18
pushOntoCallStack("Interpolation", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 240, 18);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:240:28
consumeToken(CLOSE_BRACE);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:243:1
final public void Assignment() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Assignment";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:243:1
Assignment thisProduction = null;
if (buildTree) {
thisProduction = new Assignment();
openNodeScope(thisProduction);
}
Token t;
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:247:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:247:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 247, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$247$6, parsingStack);
}
if (nextTokenType() == SET) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:249:8
t = consumeToken(SET);
} else if (nextTokenType() == ASSIGN) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:250:8
t = consumeToken(ASSIGN);
} else if (nextTokenType() == LOCAL) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:251:8
t = consumeToken(LOCAL);
} else if (nextTokenType() == GLOBAL) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:252:8
t = consumeToken(GLOBAL);
} else {
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 249, 8);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$249$8, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:254:5
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:255:5
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 255, 5);
try {
IdentifierOrStringLiteral();
} finally {
popCallStack();
}
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:258:8
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:258:16
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 258, 16);
try {
Expression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:259:8
while (true) {
if (!(nextTokenType() == COMMA || nextTokenType == IDENTIFIER || nextTokenType == STRING_LITERAL)) break;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:259:9
if (nextTokenType() == COMMA) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:259:10
consumeToken(COMMA);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:259:19
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 259, 19);
try {
IdentifierOrStringLiteral();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:259:45
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:259:54
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 259, 54);
try {
Expression();
} finally {
popCallStack();
}
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:260:8
if (nextTokenType() == IN) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:260:9
consumeToken(IN);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:260:14
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 260, 14);
try {
Expression();
} finally {
popCallStack();
}
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:261:8
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 261, 8);
try {
LooseTagEnd();
} finally {
popCallStack();
}
} else if (nextTokenType() == IN || nextTokenType == CLOSE_TAG) {
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:265:11
if (nextTokenType() == IN) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:265:12
consumeToken(IN);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:265:17
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 265, 17);
try {
Expression();
} finally {
popCallStack();
}
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:266:10
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:267:10
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 267, 10);
try {
Block();
} finally {
popCallStack();
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:268:10
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 268, 10);
try {
DirectiveEnd(t.toString());
} finally {
popCallStack();
}
} else {
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 257, 7);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$257$7, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:1
final public void Var() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Var";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:1
VarInstruction thisProduction = null;
if (buildTree) {
thisProduction = new VarInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:274:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:274:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Var", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 274, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$274$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:275:5
consumeToken(VAR);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:275:10
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:276:5
pushOntoCallStack("Var", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 276, 5);
try {
IdentifierOrStringLiteral();
} finally {
popCallStack();
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:276:31
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:276:32
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:276:41
pushOntoCallStack("Var", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 276, 41);
try {
Expression();
} finally {
popCallStack();
}
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:5
while (true) {
if (!(nextTokenType() == COMMA || nextTokenType == IDENTIFIER || nextTokenType == STRING_LITERAL)) break;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:6
if (nextTokenType() == COMMA) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:7
consumeToken(COMMA);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:15
pushOntoCallStack("Var", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 277, 15);
try {
IdentifierOrStringLiteral();
} finally {
popCallStack();
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:41
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:42
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:51
pushOntoCallStack("Var", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 277, 51);
try {
Expression();
} finally {
popCallStack();
}
}
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:278:5
pushOntoCallStack("Var", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 278, 5);
try {
LooseTagEnd();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:1
final public void Setting() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Setting";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:1
SettingInstruction thisProduction = null;
if (buildTree) {
thisProduction = new SettingInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:282:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:282:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Setting", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 282, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$282$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:283:5
consumeToken(SETTING);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:283:14
consumeToken(BLANK);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:284:5
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:284:18
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:284:19
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:284:28
pushOntoCallStack("Setting", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 284, 28);
try {
Expression();
} finally {
popCallStack();
}
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:5
while (true) {
if (!(nextTokenType() == COMMA || nextTokenType == IDENTIFIER || nextTokenType == STRING_LITERAL)) break;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:6
if (nextTokenType() == COMMA) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:7
consumeToken(COMMA);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:15
pushOntoCallStack("Setting", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 285, 15);
try {
IdentifierOrStringLiteral();
} finally {
popCallStack();
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:41
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:42
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:51
pushOntoCallStack("Setting", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 285, 51);
try {
Expression();
} finally {
popCallStack();
}
}
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:286:5
pushOntoCallStack("Setting", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 286, 5);
try {
LooseTagEnd();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:289:1
final public void Comment() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Comment";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:289:1
CommentBlock thisProduction = null;
if (buildTree) {
thisProduction = new CommentBlock();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:290:5
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:290:27
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Comment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 290, 5);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$290$5, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:291:4
consumeToken(COMMENT);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:292:4
consumeToken(END_COMMENT);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:295:1
final public void Break() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Break";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:295:1
BreakInstruction thisProduction = null;
if (buildTree) {
thisProduction = new BreakInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:296:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:296:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Break", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 296, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$296$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:297:5
consumeToken(BREAK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:298:5
pushOntoCallStack("Break", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 298, 5);
try {
LooseTagEnd();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:301:1
final public void Return() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Return";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:301:1
ReturnInstruction thisProduction = null;
if (buildTree) {
thisProduction = new ReturnInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:302:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:302:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Return", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 302, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$302$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:303:5
consumeToken(RETURN);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:304:5
if (nextTokenType() == BLANK) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:304:6
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:304:13
pushOntoCallStack("Return", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 304, 13);
try {
Expression();
} finally {
popCallStack();
}
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:305:5
pushOntoCallStack("Return", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 305, 5);
try {
LooseTagEnd();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:308:1
final public void OutputFormatBlock() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "OutputFormatBlock";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:308:1
OutputFormatBlock thisProduction = null;
if (buildTree) {
thisProduction = new OutputFormatBlock();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:309:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:309:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("OutputFormatBlock", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 309, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$309$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:310:5
consumeToken(OUTPUT_FORMAT);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:311:5
consumeToken(BLANK);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:312:5
consumeToken(STRING_LITERAL);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:313:5
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:314:5
pushOntoCallStack("OutputFormatBlock", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 314, 5);
try {
Block();
} finally {
popCallStack();
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:315:5
pushOntoCallStack("OutputFormatBlock", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 315, 5);
try {
DirectiveEnd("outputformat");
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:318:1
final public void Import() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Import";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:318:1
ImportInstruction thisProduction = null;
if (buildTree) {
thisProduction = new ImportInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:319:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:319:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Import", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 319, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$319$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:320:5
consumeToken(IMPORT);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:320:13
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:321:5
pushOntoCallStack("Import", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 321, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:322:5
consumeToken(AS);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:323:5
consumeToken(IDENTIFIER);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:324:5
pushOntoCallStack("Import", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 324, 5);
try {
LooseTagEnd();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:327:1
final public void If() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "If";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:327:1
IfStatement thisProduction = null;
if (buildTree) {
thisProduction = new IfStatement();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:328:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:328:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 328, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$328$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:329:5
consumeToken(IF);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:329:9
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:330:5
pushOntoCallStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 330, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:331:5
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:332:5
pushOntoCallStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 332, 5);
try {
Block();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:333:5
while (true) {
if (!(scan$FTL_ccc$333$7())) break;
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:333:7
pushOntoCallStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 333, 7);
try {
ElseIf();
} finally {
popCallStack();
}
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:334:5
if (scan$FTL_ccc$335$8()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:335:8
pushOntoCallStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 335, 8);
try {
Else();
} finally {
popCallStack();
}
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:337:5
pushOntoCallStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 337, 5);
try {
DirectiveEnd("if");
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:340:1
final public void ElseIf() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "ElseIf";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:340:1
ElseIfBlock thisProduction = null;
if (buildTree) {
thisProduction = new ElseIfBlock();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:341:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:341:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("ElseIf", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 341, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$341$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:342:5
consumeToken(ELSEIF);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:344:5
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:345:5
pushOntoCallStack("ElseIf", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 345, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:346:5
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:347:5
pushOntoCallStack("ElseIf", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 347, 5);
try {
Block();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:350:1
final public void Else() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Else";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:350:1
ElseBlock thisProduction = null;
if (buildTree) {
thisProduction = new ElseBlock();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:351:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:351:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Else", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 351, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$351$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:352:5
consumeToken(ELSE);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:354:5
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:355:5
pushOntoCallStack("Else", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 355, 5);
try {
Block();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:358:1
final public void Switch() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Switch";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:358:1
SwitchInstruction thisProduction = null;
if (buildTree) {
thisProduction = new SwitchInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:359:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:359:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 359, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$359$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:360:5
consumeToken(SWITCH);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:361:5
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:362:5
pushOntoCallStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 362, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:363:5
consumeToken(CLOSE_TAG);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:364:5
if (nextTokenType() == WHITESPACE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:364:6
consumeToken(WHITESPACE);
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:365:5
while (true) {
if (!(scan$FTL_ccc$365$6())) break;
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:365:6
pushOntoCallStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 365, 6);
try {
Case();
} finally {
popCallStack();
}
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:366:5
if (scan$FTL_ccc$367$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:367:13
pushOntoCallStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 367, 13);
try {
Default();
} finally {
popCallStack();
}
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:369:5
pushOntoCallStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 369, 5);
try {
DirectiveEnd("switch");
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:372:1
final public void Case() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Case";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:372:1
CaseInstruction thisProduction = null;
if (buildTree) {
thisProduction = new CaseInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:373:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:373:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Case", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 373, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$373$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:374:5
consumeToken(CASE);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:376:5
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:377:5
pushOntoCallStack("Case", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 377, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:377:16
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:377:28
pushOntoCallStack("Case", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 377, 28);
try {
Block();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:380:1
final public void Default() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Default";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:380:1
DefaultInstruction thisProduction = null;
if (buildTree) {
thisProduction = new DefaultInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:381:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:381:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Default", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 381, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$381$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:382:5
consumeToken(DEFAULT);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:382:14
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:383:5
pushOntoCallStack("Default", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 383, 5);
try {
Block();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:386:1
final public void List() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "List";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:386:1
ListInstruction thisProduction = null;
if (buildTree) {
thisProduction = new ListInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:387:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:387:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 387, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$387$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:388:5
consumeToken(LIST);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:388:11
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:389:5
pushOntoCallStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 389, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:390:5
consumeToken(AS);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:391:5
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:392:5
if (nextTokenType() == COMMA || nextTokenType == SORTED) {
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:393:7
if (nextTokenType() == SORTED) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:393:9
consumeToken(SORTED);
if (nextTokenType() == ASCENDING) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:393:19
consumeToken(ASCENDING);
} else if (nextTokenType() == DESCENDING) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:393:33
consumeToken(DESCENDING);
} else {
pushOntoCallStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 393, 19);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$393$19, parsingStack);
}
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:394:7
consumeToken(COMMA);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:394:15
consumeToken(IDENTIFIER);
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:396:5
if (nextTokenType() == WITH) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:396:7
consumeToken(WITH);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:396:14
consumeToken(IDENTIFIER);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:397:5
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:398:5
pushOntoCallStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 398, 5);
try {
Block();
} finally {
popCallStack();
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:399:5
pushOntoCallStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 399, 5);
try {
DirectiveEnd("list");
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:402:1
final public void Nested() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Nested";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:402:1
NestedInstruction thisProduction = null;
if (buildTree) {
thisProduction = new NestedInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:403:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:403:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Nested", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 403, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$403$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:404:5
consumeToken(NESTED);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:405:5
if (nextTokenType() == BLANK) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:405:6
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:405:13
pushOntoCallStack("Nested", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 405, 13);
try {
PositionalArgsList();
} finally {
popCallStack();
}
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:406:5
pushOntoCallStack("Nested", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 406, 5);
try {
LooseTagEnd();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:409:1
final public void MacroDefinition() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "MacroDefinition";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:409:1
MacroDefinition thisProduction = null;
if (buildTree) {
thisProduction = new MacroDefinition();
openNodeScope(thisProduction);
}
Token t;
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:413:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:413:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("MacroDefinition", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 413, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$413$6, parsingStack);
}
if (nextTokenType() == MACRO) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:415:7
t = consumeToken(MACRO);
} else if (nextTokenType() == FUNCTION) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:417:7
t = consumeToken(FUNCTION);
} else {
pushOntoCallStack("MacroDefinition", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 415, 7);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$415$7, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:419:5
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:420:5
pushOntoCallStack("MacroDefinition", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 420, 5);
try {
IdentifierOrStringLiteral();
} finally {
popCallStack();
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:421:5
if (nextTokenType() == OPEN_PAREN) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:421:6
consumeToken(OPEN_PAREN);
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:422:5
if (nextTokenType() == IDENTIFIER) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:422:6
pushOntoCallStack("MacroDefinition", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 422, 6);
try {
ParameterList();
} finally {
popCallStack();
}
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:423:5
if (nextTokenType() == CLOSE_PAREN) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:423:6
consumeToken(CLOSE_PAREN);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:424:5
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:425:5
pushOntoCallStack("MacroDefinition", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 425, 5);
try {
Block();
} finally {
popCallStack();
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:426:5
pushOntoCallStack("MacroDefinition", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 426, 5);
try {
DirectiveEnd(t.toString());
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:429:1
final public void ParameterList() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "ParameterList";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:429:1
ParameterList thisProduction = null;
if (buildTree) {
thisProduction = new ParameterList();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:433:5
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:434:5
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:434:6
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:434:7
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:434:15
pushOntoCallStack("ParameterList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 434, 15);
try {
Expression();
} finally {
popCallStack();
}
} else if (nextTokenType() == ELLIPSIS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:434:29
consumeToken(ELLIPSIS);
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:435:5
while (true) {
if (!(nextTokenType() == COMMA || nextTokenType == IDENTIFIER)) break;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:436:8
if (nextTokenType() == COMMA) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:436:9
consumeToken(COMMA);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:437:8
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:438:8
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:439:11
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:439:12
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:439:20
pushOntoCallStack("ParameterList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 439, 20);
try {
Expression();
} finally {
popCallStack();
}
} else if (nextTokenType() == ELLIPSIS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:441:11
consumeToken(ELLIPSIS);
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
private static final EnumSet LooseTagEnd_FIRST_SET = tokenTypeSet(CLOSE_TAG, CLOSE_EMPTY_TAG);
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:447:1
final public void LooseTagEnd() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "LooseTagEnd";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:447:1
LooseTagEnd thisProduction = null;
if (buildTree) {
thisProduction = new LooseTagEnd();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:448:5
if (nextTokenType() == CLOSE_TAG) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:448:5
consumeToken(CLOSE_TAG);
} else if (nextTokenType() == CLOSE_EMPTY_TAG) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:450:5
consumeToken(CLOSE_EMPTY_TAG);
} else {
pushOntoCallStack("LooseTagEnd", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 448, 5);
throw new ParseException(lastConsumedToken, LooseTagEnd_FIRST_SET, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
private static final EnumSet DirectiveEnd_FIRST_SET = tokenTypeSet(END_DIRECTIVE1, END_DIRECTIVE2);
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:453:1
final public void DirectiveEnd(String name) {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "DirectiveEnd";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:453:1
DirectiveEnd thisProduction = null;
if (buildTree) {
thisProduction = new DirectiveEnd();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:454:5
if (nextTokenType() == END_DIRECTIVE1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:454:5
consumeToken(END_DIRECTIVE1);
} else if (nextTokenType() == END_DIRECTIVE2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:454:22
consumeToken(END_DIRECTIVE2);
// Code for CodeBlock specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:455:5
String tag = lastConsumedToken.toString();
tag = tag.substring(3);
tag = tag.substring(0, tag.length() - 1).trim();
if (tag.length() != 0 && !name.equals(tag)) {
throw new ParseException("Expecting closing tag for " + name);
}
} else {
pushOntoCallStack("DirectiveEnd", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 454, 5);
throw new ParseException(lastConsumedToken, DirectiveEnd_FIRST_SET, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:465:1
final public void Text() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Text";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:465:1
Text thisProduction = null;
if (buildTree) {
thisProduction = new Text();
openNodeScope(thisProduction);
}
Token t;
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for OneOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:469:5
while (true) {
if (nextTokenType() == PRINTABLE_CHARS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:470:8
t = consumeToken(PRINTABLE_CHARS);
} else if (nextTokenType() == WHITESPACE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:471:8
t = consumeToken(WHITESPACE);
} else if (nextTokenType() == SPECIAL_CHAR) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:472:8
t = consumeToken(SPECIAL_CHAR);
} else {
pushOntoCallStack("Text", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 470, 8);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$470$8, parsingStack);
}
if (!(nextTokenType() == WHITESPACE || nextTokenType == SPECIAL_CHAR || nextTokenType == PRINTABLE_CHARS)) break;
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:476:1
final public void TopLevelDirective() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "TopLevelDirective";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:476:1
if (scan$FTL_ccc$478$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:478:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 478, 13);
try {
Assignment();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$479$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:479:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 479, 13);
try {
Comment();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$480$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:480:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 480, 13);
try {
If();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$481$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:481:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 481, 13);
try {
List();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$482$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:482:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 482, 13);
try {
Import();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$483$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:483:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 483, 13);
try {
MacroDefinition();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$484$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:484:6
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 484, 6);
try {
Switch();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$485$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:485:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 485, 13);
try {
Setting();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$486$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:486:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 486, 13);
try {
Var();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$487$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:487:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 487, 13);
try {
Break();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$488$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:488:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 488, 13);
try {
Return();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$489$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:489:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 489, 13);
try {
Nested();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$490$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:490:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 490, 13);
try {
OutputFormatBlock();
} finally {
popCallStack();
}
} else {
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 478, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$478$6, parsingStack);
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:494:1
final public void UserDirective() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "UserDirective";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:494:1
UserDirective thisProduction = null;
if (buildTree) {
thisProduction = new UserDirective();
openNodeScope(thisProduction);
}
Token startToken;
token_source.noWhitespaceInExpression = true;
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == USER_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:500:9
startToken = consumeToken(USER_DIRECTIVE_OPEN1);
} else if (nextTokenType() == USER_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:502:9
startToken = consumeToken(USER_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 500, 9);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$500$9, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:504:5
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:504:18
if (nextTokenType() == DOT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:504:20
consumeToken(DOT);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:504:27
consumeToken(IDENTIFIER);
}
// Code for CodeBlock specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:505:5
token_source.noWhitespaceInExpression = false;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:506:5
if (nextTokenType() == BLANK) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:506:6
consumeToken(BLANK);
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:507:5
if (first_set$FTL_ccc$507$6.contains(nextTokenType())) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:507:6
pushOntoCallStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 507, 6);
try {
ArgsList();
} finally {
popCallStack();
}
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:508:5
if (nextTokenType() == SEMICOLON) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:508:6
consumeToken(SEMICOLON);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:508:18
if (nextTokenType() == IDENTIFIER) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:508:19
pushOntoCallStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 508, 19);
try {
ParameterList();
} finally {
popCallStack();
}
}
}
if (nextTokenType() == CLOSE_EMPTY_TAG) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:510:8
consumeToken(CLOSE_EMPTY_TAG);
} else if (nextTokenType() == GT || nextTokenType == CLOSE_TAG) {
if (nextTokenType() == CLOSE_TAG) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:513:13
consumeToken(CLOSE_TAG);
} else if (nextTokenType() == GT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:513:27
consumeToken(GT);
} else {
pushOntoCallStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 513, 13);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$513$13, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:514:11
pushOntoCallStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 514, 11);
try {
Block();
} finally {
popCallStack();
}
if (nextTokenType() == END_USER_DIRECTIVE1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:515:12
consumeToken(END_USER_DIRECTIVE1);
} else if (nextTokenType() == END_USER_DIRECTIVE2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:515:34
consumeToken(END_USER_DIRECTIVE2);
} else {
pushOntoCallStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 515, 12);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$515$12, parsingStack);
}
} else {
pushOntoCallStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 510, 8);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$510$8, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:520:1
final public void Block() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Block";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:520:1
Block thisProduction = null;
if (buildTree) {
thisProduction = new Block();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:521:3
while (true) {
if (!(scan$FTL_ccc$522$5())) break;
if (nextTokenType() == WHITESPACE || nextTokenType == SPECIAL_CHAR || nextTokenType == PRINTABLE_CHARS) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:524:6
pushOntoCallStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 524, 6);
try {
Text();
} finally {
popCallStack();
}
} else if (nextTokenType() == INTERPOLATE) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:526:6
pushOntoCallStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 526, 6);
try {
Interpolation();
} finally {
popCallStack();
}
} else if (nextTokenType() == USER_DIRECTIVE_OPEN1 || nextTokenType == USER_DIRECTIVE_OPEN2) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:528:6
pushOntoCallStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 528, 6);
try {
UserDirective();
} finally {
popCallStack();
}
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN1 || nextTokenType == FTL_DIRECTIVE_OPEN2) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:530:6
pushOntoCallStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 530, 6);
try {
TopLevelDirective();
} finally {
popCallStack();
}
} else {
pushOntoCallStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 524, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$524$6, parsingStack);
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:535:1
final public void Root() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Root";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:535:1
Root thisProduction = null;
if (buildTree) {
thisProduction = new Root();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:536:5
if (nextTokenType() == WHITESPACE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:536:6
consumeToken(WHITESPACE);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:537:5
pushOntoCallStack("Root", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 537, 5);
try {
Block();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:538:5
consumeToken(EOF);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
private static final EnumSet first_set$FEL_ccc$194$10 = tokenTypeSet(OR, XOR, OR2);
private static final EnumSet first_set$FEL_ccc$201$10 = tokenTypeSet(AND, AND2);
private static final EnumSet first_set$FEL_ccc$208$10 = tokenTypeSet(EQUALS, DOUBLE_EQUALS, NOT_EQUALS);
private static final EnumSet first_set$FEL_ccc$216$9 = first_set$FEL_ccc$216$9_init();
private static EnumSet first_set$FEL_ccc$216$9_init() {
return tokenTypeSet(LT, GT, LTE, GTE, ALT_GT, ALT_LT, ALT_GTE, ALT_LTE);
}
private static final EnumSet first_set$FEL_ccc$216$10 = first_set$FEL_ccc$216$10_init();
private static EnumSet first_set$FEL_ccc$216$10_init() {
return tokenTypeSet(LT, GT, LTE, GTE, ALT_GT, ALT_LT, ALT_GTE, ALT_LTE);
}
private static final EnumSet first_set$FEL_ccc$234$13 = tokenTypeSet(PLUS, MINUS);
private static final EnumSet first_set$FEL_ccc$242$12 = tokenTypeSet(TIMES, DIVIDE, PERCENT);
private static final EnumSet first_set$FEL_ccc$253$5 = first_set$FEL_ccc$253$5_init();
private static EnumSet first_set$FEL_ccc$253$5_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$257$6 = tokenTypeSet(PLUS, MINUS);
private static final EnumSet first_set$FEL_ccc$321$6 = tokenTypeSet(TIMES, IDENTIFIER);
private static final EnumSet first_set$FEL_ccc$334$16 = first_set$FEL_ccc$334$16_init();
private static EnumSet first_set$FEL_ccc$334$16_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$338$41 = first_set$FEL_ccc$338$41_init();
private static EnumSet first_set$FEL_ccc$338$41_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$347$6 = first_set$FEL_ccc$347$6_init();
private static EnumSet first_set$FEL_ccc$347$6_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$347$18 = first_set$FEL_ccc$347$18_init();
private static EnumSet first_set$FEL_ccc$347$18_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, COMMA, EXCLAM, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$359$8 = first_set$FEL_ccc$359$8_init();
private static EnumSet first_set$FEL_ccc$359$8_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$381$9 = first_set$FEL_ccc$381$9_init();
private static EnumSet first_set$FEL_ccc$381$9_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$384$9 = first_set$FEL_ccc$384$9_init();
private static EnumSet first_set$FEL_ccc$384$9_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$403$8 = first_set$FEL_ccc$403$8_init();
private static EnumSet first_set$FEL_ccc$403$8_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, COMMA, EXCLAM, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FTL_ccc$247$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$249$8 = tokenTypeSet(SET, LOCAL, GLOBAL, ASSIGN);
private static final EnumSet first_set$FTL_ccc$257$7 = tokenTypeSet(EQUALS, IN, CLOSE_TAG);
private static final EnumSet first_set$FTL_ccc$274$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$282$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$290$5 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$296$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$302$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$309$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$319$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$328$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$341$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$351$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$359$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$373$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$381$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$387$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$393$19 = tokenTypeSet(ASCENDING, DESCENDING);
private static final EnumSet first_set$FTL_ccc$403$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$413$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$415$7 = tokenTypeSet(MACRO, FUNCTION);
private static final EnumSet first_set$FTL_ccc$470$8 = tokenTypeSet(WHITESPACE, SPECIAL_CHAR, PRINTABLE_CHARS);
private static final EnumSet first_set$FTL_ccc$478$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$500$9 = tokenTypeSet(USER_DIRECTIVE_OPEN1, USER_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$507$6 = first_set$FTL_ccc$507$6_init();
private static EnumSet first_set$FTL_ccc$507$6_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FTL_ccc$510$8 = tokenTypeSet(GT, CLOSE_TAG, CLOSE_EMPTY_TAG);
private static final EnumSet first_set$FTL_ccc$513$13 = tokenTypeSet(GT, CLOSE_TAG);
private static final EnumSet first_set$FTL_ccc$515$12 = tokenTypeSet(END_USER_DIRECTIVE1, END_USER_DIRECTIVE2);
private static final EnumSet first_set$FTL_ccc$524$6 = first_set$FTL_ccc$524$6_init();
private static EnumSet first_set$FTL_ccc$524$6_init() {
return tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2, USER_DIRECTIVE_OPEN1, USER_DIRECTIVE_OPEN2, INTERPOLATE, WHITESPACE, SPECIAL_CHAR, PRINTABLE_CHARS);
}
private boolean scanToken(TokenType expectedType, TokenType...additionalTypes) {
Token peekedToken = nextToken(currentLookaheadToken);
TokenType type = peekedToken.getType();
if (type != expectedType) {
boolean matched = false;
for (TokenType tt : additionalTypes) {
if (type == tt) {
matched = true;
break;
}
}
if (!matched) return false;
}
--remainingLookahead;
currentLookaheadToken = peekedToken;
return true;
}
private boolean scanToken(EnumSet types) {
Token peekedToken = nextToken(currentLookaheadToken);
TokenType type = peekedToken.getType();
if (!types.contains(type)) return false;
--remainingLookahead;
currentLookaheadToken = peekedToken;
return true;
}
//====================================
// Lookahead Routines
//====================================
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:194:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$194$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:194:10
if (!scanToken(OR, XOR, OR2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:194:28
// NonTerminal AndExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:194:28
pushOntoLookaheadStack("OrExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 194, 28);
currentLookaheadProduction = "AndExpression";
try {
if (!check$AndExpression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:201:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$201$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:201:10
if (!scanToken(AND, AND2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:201:24
// NonTerminal EqualityExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:201:24
pushOntoLookaheadStack("AndExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 201, 24);
currentLookaheadProduction = "EqualityExpression";
try {
if (!check$EqualityExpression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:208:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$208$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:208:10
if (!scanToken(EQUALS, DOUBLE_EQUALS, NOT_EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:209:9
// NonTerminal RelationalExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:209:9
pushOntoLookaheadStack("EqualityExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 209, 9);
currentLookaheadProduction = "RelationalExpression";
try {
if (!check$RelationalExpression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:216:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$216$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:216:10
if (!scanToken(first_set$FEL_ccc$216$10)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:217:9
// NonTerminal RangeExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:217:9
pushOntoLookaheadStack("RelationalExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 217, 9);
currentLookaheadProduction = "RangeExpression";
try {
if (!check$RangeExpression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:224:8
// BuildScanRoutine macro
private boolean check$FEL_ccc$224$8(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:224:8
if (!scanToken(DOT_DOT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:225:8
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$226$11(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:226:11
// BuildScanRoutine macro
private boolean check$FEL_ccc$226$11(boolean scanToEnd) {
int prevPassedPredicateThreshold = this.passedPredicateThreshold;
this.passedPredicateThreshold = -1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:226:11
// NonTerminal AdditiveExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:226:11
pushOntoLookaheadStack("RangeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 226, 11);
currentLookaheadProduction = "AdditiveExpression";
try {
if (!check$AdditiveExpression(true)) return false;
} finally {
popLookaheadStack();
}
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
} finally {
lookaheadRoutineNesting--;
if (remainingLookahead <= this.passedPredicateThreshold) {
passedPredicate = true;
this.passedPredicateThreshold = prevPassedPredicateThreshold;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:234:12
// BuildScanRoutine macro
private boolean check$FEL_ccc$234$12(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:234:13
if (!scanToken(PLUS, MINUS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:235:12
// NonTerminal MultiplicativeExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:235:12
pushOntoLookaheadStack("AdditiveExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 235, 12);
currentLookaheadProduction = "MultiplicativeExpression";
try {
if (!check$MultiplicativeExpression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:242:11
// BuildScanRoutine macro
private boolean check$FEL_ccc$242$11(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:242:12
if (!scanToken(TIMES, DIVIDE, PERCENT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:243:11
// NonTerminal UnaryExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:243:11
pushOntoLookaheadStack("MultiplicativeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 243, 11);
currentLookaheadProduction = "UnaryExpression";
try {
if (!check$UnaryExpression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:249:5
// BuildScanRoutine macro
private boolean check$FEL_ccc$249$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:249:5
// NonTerminal UnaryPlusMinusExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:249:5
pushOntoLookaheadStack("UnaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 249, 5);
currentLookaheadProduction = "UnaryPlusMinusExpression";
try {
if (!check$UnaryPlusMinusExpression(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:251:5
// BuildScanRoutine macro
private boolean check$FEL_ccc$251$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:251:5
// NonTerminal NotExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:251:5
pushOntoLookaheadStack("UnaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 251, 5);
currentLookaheadProduction = "NotExpression";
try {
if (!check$NotExpression(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:253:5
// BuildScanRoutine macro
private boolean check$FEL_ccc$253$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:253:5
// NonTerminal DefaultToExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:253:5
pushOntoLookaheadStack("UnaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 253, 5);
currentLookaheadProduction = "DefaultToExpression";
try {
if (!check$DefaultToExpression(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:273:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$273$9(boolean scanToEnd) {
int prevPassedPredicateThreshold = this.passedPredicateThreshold;
this.passedPredicateThreshold = -1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:274:12
if (!scanToken(EXCLAM)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:274:20
// NonTerminal PrimaryExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:274:20
pushOntoLookaheadStack("DefaultToExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 274, 20);
currentLookaheadProduction = "PrimaryExpression";
try {
if (!check$PrimaryExpression(true)) return false;
} finally {
popLookaheadStack();
}
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
} finally {
lookaheadRoutineNesting--;
if (remainingLookahead <= this.passedPredicateThreshold) {
passedPredicate = true;
this.passedPredicateThreshold = prevPassedPredicateThreshold;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:284:7
// BuildScanRoutine macro
private boolean check$FEL_ccc$284$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:284:7
Token token2 = currentLookaheadToken;
int remainingLookahead2 = remainingLookahead;
boolean hitFailure2 = hitFailure;
boolean passedPredicate2 = passedPredicate;
try {
passedPredicate = false;
if (!check$FEL_ccc$284$7$(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$286$7(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$288$7(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$290$7(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$292$7(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!scanToken(EXISTS_OPERATOR)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
return false;
}
}
}
}
}
}
} finally {
passedPredicate = passedPredicate2;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:284:7
// BuildScanRoutine macro
private boolean check$FEL_ccc$284$7$(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:284:7
// NonTerminal DotKey at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:284:7
pushOntoLookaheadStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 284, 7);
currentLookaheadProduction = "DotKey";
try {
if (!check$DotKey(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:286:7
// BuildScanRoutine macro
private boolean check$FEL_ccc$286$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:286:7
// NonTerminal MethodReference at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:286:7
pushOntoLookaheadStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 286, 7);
currentLookaheadProduction = "MethodReference";
try {
if (!check$MethodReference(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:288:7
// BuildScanRoutine macro
private boolean check$FEL_ccc$288$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:288:7
// NonTerminal DynamicKey at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:288:7
pushOntoLookaheadStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 288, 7);
currentLookaheadProduction = "DynamicKey";
try {
if (!check$DynamicKey(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:290:7
// BuildScanRoutine macro
private boolean check$FEL_ccc$290$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:290:7
// NonTerminal MethodInvoke at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:290:7
pushOntoLookaheadStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 290, 7);
currentLookaheadProduction = "MethodInvoke";
try {
if (!check$MethodInvoke(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:292:7
// BuildScanRoutine macro
private boolean check$FEL_ccc$292$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:292:7
// NonTerminal BuiltIn at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:292:7
pushOntoLookaheadStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 292, 7);
currentLookaheadProduction = "BuiltIn";
try {
if (!check$BuiltIn(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:303:5
// BuildScanRoutine macro
private boolean check$FEL_ccc$303$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:303:5
// NonTerminal HashLiteral at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:303:5
pushOntoLookaheadStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 303, 5);
currentLookaheadProduction = "HashLiteral";
try {
if (!check$HashLiteral(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:311:5
// BuildScanRoutine macro
private boolean check$FEL_ccc$311$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:311:5
// NonTerminal ListLiteral at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:311:5
pushOntoLookaheadStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 311, 5);
currentLookaheadProduction = "ListLiteral";
try {
if (!check$ListLiteral(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:313:5
// BuildScanRoutine macro
private boolean check$FEL_ccc$313$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:313:5
// NonTerminal Parenthesis at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:313:5
pushOntoLookaheadStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 313, 5);
currentLookaheadProduction = "Parenthesis";
try {
if (!check$Parenthesis(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:315:5
// BuildScanRoutine macro
private boolean check$FEL_ccc$315$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:315:5
// NonTerminal BuiltinVariable at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:315:5
pushOntoLookaheadStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 315, 5);
currentLookaheadProduction = "BuiltinVariable";
try {
if (!check$BuiltinVariable(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:334:16
// BuildScanRoutine macro
private boolean check$FEL_ccc$334$16(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:334:16
// NonTerminal ArgsList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:334:16
pushOntoLookaheadStack("MethodInvoke", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 334, 16);
currentLookaheadProduction = "ArgsList";
try {
if (!check$ArgsList(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:28
// BuildScanRoutine macro
private boolean check$FEL_ccc$338$28(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:28
if (!scanToken(OPEN_PAREN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:40
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$338$41(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:50
if (!scanToken(CLOSE_PAREN)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:41
// BuildScanRoutine macro
private boolean check$FEL_ccc$338$41(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:41
// NonTerminal ArgsList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:41
pushOntoLookaheadStack("BuiltIn", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 338, 41);
currentLookaheadProduction = "ArgsList";
try {
if (!check$ArgsList(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:6
// BuildScanRoutine macro
private boolean check$FEL_ccc$347$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:6
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:6
pushOntoLookaheadStack("ListLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 347, 6);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:17
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$347$18(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:18
// BuildScanRoutine macro
private boolean check$FEL_ccc$347$18(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:18
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(COMMA)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:27
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:27
pushOntoLookaheadStack("ListLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 347, 27);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:359:8
// BuildScanRoutine macro
private boolean check$FEL_ccc$359$8(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:359:8
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:359:8
pushOntoLookaheadStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 359, 8);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:359:19
if (!scanToken(COLON)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:359:27
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:359:27
pushOntoLookaheadStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 359, 27);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:8
boolean passedPredicate6 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token7 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$360$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token7;
break;
}
}
} finally {
passedPredicate = passedPredicate6;
}
hitFailure = false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$360$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:9
if (!scanToken(COMMA)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:17
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:17
pushOntoLookaheadStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 360, 17);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:28
if (!scanToken(COLON)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:36
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:36
pushOntoLookaheadStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 360, 36);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:381:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$381$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2147483647;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
if (remainingLookahead <= 0) {
passedPredicate = true;
return !hitFailure;
}
if (!check$FEL_ccc$381$14(true)) return false;
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:382:9
// NonTerminal NamedArgsList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:382:9
pushOntoLookaheadStack("ArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 382, 9);
currentLookaheadProduction = "NamedArgsList";
try {
if (!check$NamedArgsList(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:384:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$384$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:384:9
// NonTerminal PositionalArgsList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:384:9
pushOntoLookaheadStack("ArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 384, 9);
currentLookaheadProduction = "PositionalArgsList";
try {
if (!check$PositionalArgsList(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:393:8
// BuildScanRoutine macro
private boolean check$FEL_ccc$393$8(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:393:8
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(COMMA)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:394:8
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:395:8
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:396:8
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:396:8
pushOntoLookaheadStack("NamedArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 396, 8);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:403:8
// BuildScanRoutine macro
private boolean check$FEL_ccc$403$8(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:403:8
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(COMMA)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:404:8
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:404:8
pushOntoLookaheadStack("PositionalArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 404, 8);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:257:7
// BuildScanRoutine macro
private boolean check$FTL_ccc$257$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:258:8
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:258:16
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:258:16
pushOntoLookaheadStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 258, 16);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:259:8
boolean passedPredicate7 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token8 = currentLookaheadToken;
passedPredicate = false;
if (!check$FTL_ccc$259$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token8;
break;
}
}
} finally {
passedPredicate = passedPredicate7;
}
hitFailure = false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:260:8
Token token10 = currentLookaheadToken;
boolean passedPredicate10 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$260$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token10;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate10;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:261:8
if (!scanToken(CLOSE_TAG, CLOSE_EMPTY_TAG)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:259:9
// BuildScanRoutine macro
private boolean check$FTL_ccc$259$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:259:9
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(COMMA)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:259:19
if (!scanToken(IDENTIFIER, STRING_LITERAL)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:259:45
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:259:54
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:259:54
pushOntoLookaheadStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 259, 54);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:260:9
// BuildScanRoutine macro
private boolean check$FTL_ccc$260$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:260:9
if (!scanToken(IN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:260:14
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:260:14
pushOntoLookaheadStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 260, 14);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:264:7
// BuildScanRoutine macro
private boolean check$FTL_ccc$264$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:265:11
Token token5 = currentLookaheadToken;
boolean passedPredicate5 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$265$12(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate5;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:266:10
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:267:10
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:267:10
pushOntoLookaheadStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 267, 10);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:268:10
if (!scanToken(END_DIRECTIVE1, END_DIRECTIVE2)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:265:12
// BuildScanRoutine macro
private boolean check$FTL_ccc$265$12(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:265:12
if (!scanToken(IN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:265:17
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:265:17
pushOntoLookaheadStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 265, 17);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:276:32
// BuildScanRoutine macro
private boolean check$FTL_ccc$276$32(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:276:32
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:276:41
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:276:41
pushOntoLookaheadStack("Var", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 276, 41);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$277$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:6
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(COMMA)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:15
if (!scanToken(IDENTIFIER, STRING_LITERAL)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:41
Token token6 = currentLookaheadToken;
boolean passedPredicate6 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$277$42(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token6;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate6;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:42
// BuildScanRoutine macro
private boolean check$FTL_ccc$277$42(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:42
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:51
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:51
pushOntoLookaheadStack("Var", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 277, 51);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:284:19
// BuildScanRoutine macro
private boolean check$FTL_ccc$284$19(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:284:19
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:284:28
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:284:28
pushOntoLookaheadStack("Setting", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 284, 28);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$285$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:6
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(COMMA)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:15
if (!scanToken(IDENTIFIER, STRING_LITERAL)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:41
Token token6 = currentLookaheadToken;
boolean passedPredicate6 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$285$42(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token6;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate6;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:42
// BuildScanRoutine macro
private boolean check$FTL_ccc$285$42(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:42
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:51
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:51
pushOntoLookaheadStack("Setting", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 285, 51);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:304:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$304$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:304:6
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:304:13
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:304:13
pushOntoLookaheadStack("Return", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 304, 13);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:333:7
// BuildScanRoutine macro
private boolean check$FTL_ccc$333$7(boolean scanToEnd) {
int prevPassedPredicateThreshold = this.passedPredicateThreshold;
this.passedPredicateThreshold = -1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:333:7
// NonTerminal ElseIf at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:333:7
pushOntoLookaheadStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 333, 7);
currentLookaheadProduction = "ElseIf";
try {
if (!check$ElseIf(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (remainingLookahead <= this.passedPredicateThreshold) {
passedPredicate = true;
this.passedPredicateThreshold = prevPassedPredicateThreshold;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:335:8
// BuildScanRoutine macro
private boolean check$FTL_ccc$335$8(boolean scanToEnd) {
int prevPassedPredicateThreshold = this.passedPredicateThreshold;
this.passedPredicateThreshold = -1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:335:8
// NonTerminal Else at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:335:8
pushOntoLookaheadStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 335, 8);
currentLookaheadProduction = "Else";
try {
if (!check$Else(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (remainingLookahead <= this.passedPredicateThreshold) {
passedPredicate = true;
this.passedPredicateThreshold = prevPassedPredicateThreshold;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:365:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$365$6(boolean scanToEnd) {
int prevPassedPredicateThreshold = this.passedPredicateThreshold;
this.passedPredicateThreshold = -1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:365:6
// NonTerminal Case at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:365:6
pushOntoLookaheadStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 365, 6);
currentLookaheadProduction = "Case";
try {
if (!check$Case(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (remainingLookahead <= this.passedPredicateThreshold) {
passedPredicate = true;
this.passedPredicateThreshold = prevPassedPredicateThreshold;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:367:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$367$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:367:13
// NonTerminal Default at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:367:13
pushOntoLookaheadStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 367, 13);
currentLookaheadProduction = "Default";
try {
if (!check$Default(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:393:7
// BuildScanRoutine macro
private boolean check$FTL_ccc$393$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:393:7
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$393$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:394:7
if (!scanToken(COMMA)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:394:15
if (!scanToken(IDENTIFIER)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:393:9
// BuildScanRoutine macro
private boolean check$FTL_ccc$393$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:393:9
if (!scanToken(SORTED)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:393:19
if (!scanToken(ASCENDING, DESCENDING)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:396:7
// BuildScanRoutine macro
private boolean check$FTL_ccc$396$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:396:7
if (!scanToken(WITH)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:396:14
if (!scanToken(IDENTIFIER)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:405:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$405$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:405:6
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:405:13
// NonTerminal PositionalArgsList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:405:13
pushOntoLookaheadStack("Nested", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 405, 13);
currentLookaheadProduction = "PositionalArgsList";
try {
if (!check$PositionalArgsList(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:422:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$422$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:422:6
// NonTerminal ParameterList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:422:6
pushOntoLookaheadStack("MacroDefinition", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 422, 6);
currentLookaheadProduction = "ParameterList";
try {
if (!check$ParameterList(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:434:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$434$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:434:6
Token token2 = currentLookaheadToken;
int remainingLookahead2 = remainingLookahead;
boolean hitFailure2 = hitFailure;
boolean passedPredicate2 = passedPredicate;
try {
passedPredicate = false;
if (!check$FTL_ccc$434$6$(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!scanToken(ELLIPSIS)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
return false;
}
}
} finally {
passedPredicate = passedPredicate2;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:434:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$434$6$(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:434:7
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:434:15
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:434:15
pushOntoLookaheadStack("ParameterList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 434, 15);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:436:8
// BuildScanRoutine macro
private boolean check$FTL_ccc$436$8(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:436:8
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(COMMA)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:437:8
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:438:8
Token token6 = currentLookaheadToken;
boolean passedPredicate6 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$439$11(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token6;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate6;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:439:11
// BuildScanRoutine macro
private boolean check$FTL_ccc$439$11(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:439:11
Token token2 = currentLookaheadToken;
int remainingLookahead2 = remainingLookahead;
boolean hitFailure2 = hitFailure;
boolean passedPredicate2 = passedPredicate;
try {
passedPredicate = false;
if (!check$FTL_ccc$439$11$(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!scanToken(ELLIPSIS)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
return false;
}
}
} finally {
passedPredicate = passedPredicate2;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:439:11
// BuildScanRoutine macro
private boolean check$FTL_ccc$439$11$(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:439:12
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:439:20
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:439:20
pushOntoLookaheadStack("ParameterList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 439, 20);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:478:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$478$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:478:13
// NonTerminal Assignment at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:478:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 478, 13);
currentLookaheadProduction = "Assignment";
try {
if (!check$Assignment(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:479:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$479$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:479:13
// NonTerminal Comment at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:479:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 479, 13);
currentLookaheadProduction = "Comment";
try {
if (!check$Comment(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:480:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$480$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:480:13
// NonTerminal If at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:480:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 480, 13);
currentLookaheadProduction = "If";
try {
if (!check$If(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:481:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$481$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:481:13
// NonTerminal List at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:481:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 481, 13);
currentLookaheadProduction = "List";
try {
if (!check$List(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:482:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$482$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:482:13
// NonTerminal Import at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:482:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 482, 13);
currentLookaheadProduction = "Import";
try {
if (!check$Import(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:483:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$483$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:483:13
// NonTerminal MacroDefinition at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:483:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 483, 13);
currentLookaheadProduction = "MacroDefinition";
try {
if (!check$MacroDefinition(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:484:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$484$6(boolean scanToEnd) {
int prevPassedPredicateThreshold = this.passedPredicateThreshold;
this.passedPredicateThreshold = -1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:484:6
// NonTerminal Switch at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:484:6
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 484, 6);
currentLookaheadProduction = "Switch";
try {
if (!check$Switch(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (remainingLookahead <= this.passedPredicateThreshold) {
passedPredicate = true;
this.passedPredicateThreshold = prevPassedPredicateThreshold;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:485:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$485$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:485:13
// NonTerminal Setting at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:485:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 485, 13);
currentLookaheadProduction = "Setting";
try {
if (!check$Setting(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:486:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$486$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:486:13
// NonTerminal Var at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:486:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 486, 13);
currentLookaheadProduction = "Var";
try {
if (!check$Var(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:487:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$487$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:487:13
// NonTerminal Break at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:487:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 487, 13);
currentLookaheadProduction = "Break";
try {
if (!check$Break(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:488:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$488$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:488:13
// NonTerminal Return at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:488:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 488, 13);
currentLookaheadProduction = "Return";
try {
if (!check$Return(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:489:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$489$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:489:13
// NonTerminal Nested at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:489:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 489, 13);
currentLookaheadProduction = "Nested";
try {
if (!check$Nested(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:490:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$490$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:490:13
// NonTerminal OutputFormatBlock at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:490:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 490, 13);
currentLookaheadProduction = "OutputFormatBlock";
try {
if (!check$OutputFormatBlock(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:504:20
// BuildScanRoutine macro
private boolean check$FTL_ccc$504$20(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:504:20
if (!scanToken(DOT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:504:27
if (!scanToken(IDENTIFIER)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:507:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$507$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:507:6
// NonTerminal ArgsList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:507:6
pushOntoLookaheadStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 507, 6);
currentLookaheadProduction = "ArgsList";
try {
if (!check$ArgsList(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:508:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$508$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:508:6
if (!scanToken(SEMICOLON)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:508:18
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$508$19(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:508:19
// BuildScanRoutine macro
private boolean check$FTL_ccc$508$19(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:508:19
// NonTerminal ParameterList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:508:19
pushOntoLookaheadStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 508, 19);
currentLookaheadProduction = "ParameterList";
try {
if (!check$ParameterList(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:512:8
// BuildScanRoutine macro
private boolean check$FTL_ccc$512$8(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:513:13
if (!scanToken(GT, CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:514:11
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:514:11
pushOntoLookaheadStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 514, 11);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:515:12
if (!scanToken(END_USER_DIRECTIVE1, END_USER_DIRECTIVE2)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:522:5
// BuildScanRoutine macro
private boolean check$FTL_ccc$522$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:524:6
Token token4 = currentLookaheadToken;
int remainingLookahead4 = remainingLookahead;
boolean hitFailure4 = hitFailure;
boolean passedPredicate4 = passedPredicate;
try {
passedPredicate = false;
if (!check$FTL_ccc$524$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$526$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$528$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$530$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
return false;
}
}
}
}
} finally {
passedPredicate = passedPredicate4;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:524:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$524$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:524:6
// NonTerminal Text at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:524:6
pushOntoLookaheadStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 524, 6);
currentLookaheadProduction = "Text";
try {
if (!check$Text(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:526:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$526$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:526:6
// NonTerminal Interpolation at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:526:6
pushOntoLookaheadStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 526, 6);
currentLookaheadProduction = "Interpolation";
try {
if (!check$Interpolation(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:528:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$528$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:528:6
// NonTerminal UserDirective at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:528:6
pushOntoLookaheadStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 528, 6);
currentLookaheadProduction = "UserDirective";
try {
if (!check$UserDirective(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:530:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$530$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:530:6
// NonTerminal TopLevelDirective at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:530:6
pushOntoLookaheadStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 530, 6);
currentLookaheadProduction = "TopLevelDirective";
try {
if (!check$TopLevelDirective(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:226:11
private boolean scan$FEL_ccc$226$11() {
remainingLookahead = UNLIMITED;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:226:11
// NonTerminal AdditiveExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:226:11
pushOntoLookaheadStack("RangeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 226, 11);
currentLookaheadProduction = "AdditiveExpression";
try {
if (!check$AdditiveExpression(true)) return false;
} finally {
popLookaheadStack();
}
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:273:9
private boolean scan$FEL_ccc$273$9() {
remainingLookahead = UNLIMITED;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:274:12
if (!scanToken(EXCLAM)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:274:20
// NonTerminal PrimaryExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:274:20
pushOntoLookaheadStack("DefaultToExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 274, 20);
currentLookaheadProduction = "PrimaryExpression";
try {
if (!check$PrimaryExpression(true)) return false;
} finally {
popLookaheadStack();
}
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:381:9
private boolean scan$FEL_ccc$381$9() {
remainingLookahead = UNLIMITED;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
if (remainingLookahead <= 0) {
passedPredicate = true;
return !hitFailure;
}
if (!check$FEL_ccc$381$14(true)) return false;
// End BuildPredicateCode macro
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:333:7
private boolean scan$FTL_ccc$333$7() {
remainingLookahead = UNLIMITED;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:333:7
// NonTerminal ElseIf at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:333:7
pushOntoLookaheadStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 333, 7);
currentLookaheadProduction = "ElseIf";
try {
if (!check$ElseIf(false)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:335:8
private boolean scan$FTL_ccc$335$8() {
remainingLookahead = UNLIMITED;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:335:8
// NonTerminal Else at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:335:8
pushOntoLookaheadStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 335, 8);
currentLookaheadProduction = "Else";
try {
if (!check$Else(false)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:365:6
private boolean scan$FTL_ccc$365$6() {
remainingLookahead = UNLIMITED;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:365:6
// NonTerminal Case at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:365:6
pushOntoLookaheadStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 365, 6);
currentLookaheadProduction = "Case";
try {
if (!check$Case(false)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:367:6
private boolean scan$FTL_ccc$367$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:367:13
// NonTerminal Default at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:367:13
pushOntoLookaheadStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 367, 13);
currentLookaheadProduction = "Default";
try {
if (!check$Default(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:478:6
private boolean scan$FTL_ccc$478$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:478:13
// NonTerminal Assignment at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:478:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 478, 13);
currentLookaheadProduction = "Assignment";
try {
if (!check$Assignment(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:479:6
private boolean scan$FTL_ccc$479$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:479:13
// NonTerminal Comment at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:479:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 479, 13);
currentLookaheadProduction = "Comment";
try {
if (!check$Comment(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:480:6
private boolean scan$FTL_ccc$480$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:480:13
// NonTerminal If at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:480:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 480, 13);
currentLookaheadProduction = "If";
try {
if (!check$If(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:481:6
private boolean scan$FTL_ccc$481$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:481:13
// NonTerminal List at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:481:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 481, 13);
currentLookaheadProduction = "List";
try {
if (!check$List(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:482:6
private boolean scan$FTL_ccc$482$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:482:13
// NonTerminal Import at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:482:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 482, 13);
currentLookaheadProduction = "Import";
try {
if (!check$Import(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:483:6
private boolean scan$FTL_ccc$483$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:483:13
// NonTerminal MacroDefinition at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:483:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 483, 13);
currentLookaheadProduction = "MacroDefinition";
try {
if (!check$MacroDefinition(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:484:6
private boolean scan$FTL_ccc$484$6() {
remainingLookahead = UNLIMITED;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:484:6
// NonTerminal Switch at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:484:6
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 484, 6);
currentLookaheadProduction = "Switch";
try {
if (!check$Switch(false)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:485:6
private boolean scan$FTL_ccc$485$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:485:13
// NonTerminal Setting at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:485:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 485, 13);
currentLookaheadProduction = "Setting";
try {
if (!check$Setting(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:486:6
private boolean scan$FTL_ccc$486$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:486:13
// NonTerminal Var at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:486:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 486, 13);
currentLookaheadProduction = "Var";
try {
if (!check$Var(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:487:6
private boolean scan$FTL_ccc$487$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:487:13
// NonTerminal Break at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:487:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 487, 13);
currentLookaheadProduction = "Break";
try {
if (!check$Break(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:488:6
private boolean scan$FTL_ccc$488$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:488:13
// NonTerminal Return at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:488:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 488, 13);
currentLookaheadProduction = "Return";
try {
if (!check$Return(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:489:6
private boolean scan$FTL_ccc$489$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:489:13
// NonTerminal Nested at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:489:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 489, 13);
currentLookaheadProduction = "Nested";
try {
if (!check$Nested(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:490:6
private boolean scan$FTL_ccc$490$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:490:13
// NonTerminal OutputFormatBlock at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:490:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 490, 13);
currentLookaheadProduction = "OutputFormatBlock";
try {
if (!check$OutputFormatBlock(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:522:5
private boolean scan$FTL_ccc$522$5() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:524:6
Token token4 = currentLookaheadToken;
int remainingLookahead4 = remainingLookahead;
boolean hitFailure4 = hitFailure;
boolean passedPredicate4 = passedPredicate;
try {
passedPredicate = false;
if (!check$FTL_ccc$524$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$526$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$528$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$530$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
return false;
}
}
}
}
} finally {
passedPredicate = passedPredicate4;
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// lookahead routine for lookahead at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:381:9
private boolean check$FEL_ccc$381$14(boolean scanToEnd) {
int prevRemainingLookahead = remainingLookahead;
boolean prevHitFailure = hitFailure;
Token prevScanAheadToken = currentLookaheadToken;
try {
lookaheadRoutineNesting++;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:381:14
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:381:26
if (!scanToken(EQUALS)) return false;
return !hitFailure;
} finally {
lookaheadRoutineNesting--;
currentLookaheadToken = prevScanAheadToken;
remainingLookahead = prevRemainingLookahead;
hitFailure = prevHitFailure;
}
}
// BuildProductionLookaheadMethod macro
private boolean check$Expression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:188:5
// NonTerminal OrExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:188:5
pushOntoLookaheadStack("Expression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 188, 5);
currentLookaheadProduction = "OrExpression";
try {
if (!check$OrExpression(false)) return false;
} finally {
popLookaheadStack();
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$OrExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:192:5
// NonTerminal AndExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:192:5
pushOntoLookaheadStack("OrExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 192, 5);
currentLookaheadProduction = "AndExpression";
try {
if (!check$AndExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:193:5
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$194$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$AndExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:199:5
// NonTerminal EqualityExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:199:5
pushOntoLookaheadStack("AndExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 199, 5);
currentLookaheadProduction = "EqualityExpression";
try {
if (!check$EqualityExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:200:5
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$201$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$EqualityExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:206:5
// NonTerminal RelationalExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:206:5
pushOntoLookaheadStack("EqualityExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 206, 5);
currentLookaheadProduction = "RelationalExpression";
try {
if (!check$RelationalExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:207:5
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$208$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$RelationalExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:214:5
// NonTerminal RangeExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:214:5
pushOntoLookaheadStack("RelationalExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 214, 5);
currentLookaheadProduction = "RangeExpression";
try {
if (!check$RangeExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:215:5
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$216$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$RangeExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:222:5
// NonTerminal AdditiveExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:222:5
pushOntoLookaheadStack("RangeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 222, 5);
currentLookaheadProduction = "AdditiveExpression";
try {
if (!check$AdditiveExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:223:5
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$224$8(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$AdditiveExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:232:5
// NonTerminal MultiplicativeExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:232:5
pushOntoLookaheadStack("AdditiveExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 232, 5);
currentLookaheadProduction = "MultiplicativeExpression";
try {
if (!check$MultiplicativeExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:233:5
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$234$12(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$MultiplicativeExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:240:5
// NonTerminal UnaryExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:240:5
pushOntoLookaheadStack("MultiplicativeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 240, 5);
currentLookaheadProduction = "UnaryExpression";
try {
if (!check$UnaryExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:241:5
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$242$11(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$UnaryExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:249:5
Token token2 = currentLookaheadToken;
int remainingLookahead2 = remainingLookahead;
boolean hitFailure2 = hitFailure;
boolean passedPredicate2 = passedPredicate;
try {
passedPredicate = false;
if (!check$FEL_ccc$249$5(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$251$5(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$253$5(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
return false;
}
}
}
} finally {
passedPredicate = passedPredicate2;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$UnaryPlusMinusExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:257:6
if (!scanToken(PLUS, MINUS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:257:22
// NonTerminal DefaultToExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:257:22
pushOntoLookaheadStack("UnaryPlusMinusExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 257, 22);
currentLookaheadProduction = "DefaultToExpression";
try {
if (!check$DefaultToExpression(true)) return false;
} finally {
popLookaheadStack();
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$NotExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:261:5
if (!scanToken(EXCLAM)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:262:5
// NonTerminal DefaultToExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:262:5
pushOntoLookaheadStack("NotExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 262, 5);
currentLookaheadProduction = "DefaultToExpression";
try {
if (!check$DefaultToExpression(true)) return false;
} finally {
popLookaheadStack();
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$BuiltinVariable(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:266:5
if (!scanToken(DOT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:266:10
if (!scanToken(IDENTIFIER)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$DefaultToExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:270:5
// NonTerminal PrimaryExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:270:5
pushOntoLookaheadStack("DefaultToExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 270, 5);
currentLookaheadProduction = "PrimaryExpression";
try {
if (!check$PrimaryExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:271:5
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$273$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:276:5
Token token7 = currentLookaheadToken;
boolean passedPredicate7 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(EXCLAM)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token7;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate7;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$PrimaryExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:282:3
// NonTerminal BaseExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:282:3
pushOntoLookaheadStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 282, 3);
currentLookaheadProduction = "BaseExpression";
try {
if (!check$BaseExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:283:3
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$284$7(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$BaseExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:299:5
Token token2 = currentLookaheadToken;
int remainingLookahead2 = remainingLookahead;
boolean hitFailure2 = hitFailure;
boolean passedPredicate2 = passedPredicate;
try {
passedPredicate = false;
if (!scanToken(IDENTIFIER)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!scanToken(INTEGER, DECIMAL)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$303$5(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!scanToken(STRING_LITERAL)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!scanToken(TRUE, FALSE)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!scanToken(NULL)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$311$5(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$313$5(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$315$5(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
return false;
}
}
}
}
}
}
}
}
}
} finally {
passedPredicate = passedPredicate2;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$DotKey(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:320:5
if (!scanToken(DOT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:321:6
if (!scanToken(TIMES, IDENTIFIER)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$MethodReference(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:325:5
if (!scanToken(DOUBLE_COLON)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:326:5
if (!scanToken(IDENTIFIER)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$DynamicKey(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:330:5
if (!scanToken(OPEN_BRACKET)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:330:19
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:330:19
pushOntoLookaheadStack("DynamicKey", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 330, 19);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:330:29
if (!scanToken(CLOSE_BRACKET)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$MethodInvoke(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:334:3
if (!scanToken(OPEN_PAREN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:334:15
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$334$16(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:334:25
if (!scanToken(CLOSE_PAREN)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$BuiltIn(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:5
if (!scanToken(BUILT_IN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:15
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:27
Token token5 = currentLookaheadToken;
boolean passedPredicate5 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$338$28(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate5;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$ListLiteral(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:346:5
if (!scanToken(OPEN_BRACKET)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:5
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$347$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:5
if (!scanToken(CLOSE_BRACKET)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$HashLiteral(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:357:5
if (!scanToken(OPEN_BRACE)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:358:5
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$359$8(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:362:5
if (!scanToken(CLOSE_BRACE)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Parenthesis(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:376:5
if (!scanToken(OPEN_PAREN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:376:18
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:376:18
pushOntoLookaheadStack("Parenthesis", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 376, 18);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:376:29
if (!scanToken(CLOSE_PAREN)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$ArgsList(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:381:9
Token token4 = currentLookaheadToken;
int remainingLookahead4 = remainingLookahead;
boolean hitFailure4 = hitFailure;
boolean passedPredicate4 = passedPredicate;
try {
passedPredicate = false;
if (!check$FEL_ccc$381$9(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$384$9(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
return false;
}
}
} finally {
passedPredicate = passedPredicate4;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$NamedArgsList(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:389:5
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:390:5
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:391:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:391:5
pushOntoLookaheadStack("NamedArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 391, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:392:5
boolean passedPredicate6 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token7 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$393$8(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token7;
break;
}
}
} finally {
passedPredicate = passedPredicate6;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$PositionalArgsList(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:401:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:401:5
pushOntoLookaheadStack("PositionalArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 401, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:402:5
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$403$8(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Interpolation(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:240:5
if (!scanToken(INTERPOLATE)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:240:18
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:240:18
pushOntoLookaheadStack("Interpolation", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 240, 18);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:240:28
if (!scanToken(CLOSE_BRACE)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Assignment(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:247:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:249:8
if (!scanToken(SET, LOCAL, GLOBAL, ASSIGN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:254:5
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:255:5
if (!scanToken(IDENTIFIER, STRING_LITERAL)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:257:7
Token token10 = currentLookaheadToken;
int remainingLookahead10 = remainingLookahead;
boolean hitFailure10 = hitFailure;
boolean passedPredicate10 = passedPredicate;
try {
passedPredicate = false;
if (!check$FTL_ccc$257$7(false)) {
currentLookaheadToken = token10;
remainingLookahead = remainingLookahead10;
hitFailure = hitFailure10;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$264$7(false)) {
currentLookaheadToken = token10;
remainingLookahead = remainingLookahead10;
hitFailure = hitFailure10;
return false;
}
}
} finally {
passedPredicate = passedPredicate10;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Var(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:274:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:275:5
if (!scanToken(VAR)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:275:10
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:276:5
if (!scanToken(IDENTIFIER, STRING_LITERAL)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:276:31
Token token8 = currentLookaheadToken;
boolean passedPredicate8 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$276$32(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token8;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate8;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:5
boolean passedPredicate10 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token11 = currentLookaheadToken;
passedPredicate = false;
if (!check$FTL_ccc$277$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token11;
break;
}
}
} finally {
passedPredicate = passedPredicate10;
}
hitFailure = false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:278:5
if (!scanToken(CLOSE_TAG, CLOSE_EMPTY_TAG)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Setting(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:282:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:283:5
if (!scanToken(SETTING)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:283:14
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:284:5
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:284:18
Token token8 = currentLookaheadToken;
boolean passedPredicate8 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$284$19(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token8;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate8;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:5
boolean passedPredicate10 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token11 = currentLookaheadToken;
passedPredicate = false;
if (!check$FTL_ccc$285$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token11;
break;
}
}
} finally {
passedPredicate = passedPredicate10;
}
hitFailure = false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:286:5
if (!scanToken(CLOSE_TAG, CLOSE_EMPTY_TAG)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Comment(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:290:5
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:291:4
if (!scanToken(COMMENT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:292:4
if (!scanToken(END_COMMENT)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Break(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:296:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:297:5
if (!scanToken(BREAK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:298:5
if (!scanToken(CLOSE_TAG, CLOSE_EMPTY_TAG)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Return(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:302:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:303:5
if (!scanToken(RETURN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:304:5
Token token6 = currentLookaheadToken;
boolean passedPredicate6 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$304$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token6;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate6;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:305:5
if (!scanToken(CLOSE_TAG, CLOSE_EMPTY_TAG)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$OutputFormatBlock(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:309:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:310:5
if (!scanToken(OUTPUT_FORMAT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:311:5
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:312:5
if (!scanToken(STRING_LITERAL)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:313:5
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:314:5
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:314:5
pushOntoLookaheadStack("OutputFormatBlock", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 314, 5);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:315:5
if (!scanToken(END_DIRECTIVE1, END_DIRECTIVE2)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Import(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:319:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:320:5
if (!scanToken(IMPORT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:320:13
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:321:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:321:5
pushOntoLookaheadStack("Import", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 321, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:322:5
if (!scanToken(AS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:323:5
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:324:5
if (!scanToken(CLOSE_TAG, CLOSE_EMPTY_TAG)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$If(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:328:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:329:5
if (!scanToken(IF)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:329:9
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:330:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:330:5
pushOntoLookaheadStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 330, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:331:5
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:332:5
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:332:5
pushOntoLookaheadStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 332, 5);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:333:5
boolean passedPredicate10 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token11 = currentLookaheadToken;
passedPredicate = false;
if (!check$FTL_ccc$333$7(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token11;
break;
}
}
} finally {
passedPredicate = passedPredicate10;
}
hitFailure = false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:334:5
Token token13 = currentLookaheadToken;
boolean passedPredicate13 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$335$8(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token13;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate13;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:337:5
if (!scanToken(END_DIRECTIVE1, END_DIRECTIVE2)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$ElseIf(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:341:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:342:5
if (!scanToken(ELSEIF)) return false;
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:344:5
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:345:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:345:5
pushOntoLookaheadStack("ElseIf", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 345, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:346:5
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:347:5
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:347:5
pushOntoLookaheadStack("ElseIf", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 347, 5);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Else(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:351:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:352:5
if (!scanToken(ELSE)) return false;
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:354:5
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:355:5
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:355:5
pushOntoLookaheadStack("Else", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 355, 5);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Switch(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:359:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:360:5
if (!scanToken(SWITCH)) return false;
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:361:5
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:362:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:362:5
pushOntoLookaheadStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 362, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:363:5
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:364:5
Token token9 = currentLookaheadToken;
boolean passedPredicate9 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(WHITESPACE)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token9;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate9;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:365:5
boolean passedPredicate11 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token12 = currentLookaheadToken;
passedPredicate = false;
if (!check$FTL_ccc$365$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token12;
break;
}
}
} finally {
passedPredicate = passedPredicate11;
}
hitFailure = false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:366:5
Token token14 = currentLookaheadToken;
boolean passedPredicate14 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$367$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token14;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate14;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:369:5
if (!scanToken(END_DIRECTIVE1, END_DIRECTIVE2)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Case(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:373:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:374:5
if (!scanToken(CASE)) return false;
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:376:5
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:377:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:377:5
pushOntoLookaheadStack("Case", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 377, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:377:16
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:377:28
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:377:28
pushOntoLookaheadStack("Case", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 377, 28);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Default(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:381:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:382:5
if (!scanToken(DEFAULT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:382:14
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:383:5
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:383:5
pushOntoLookaheadStack("Default", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 383, 5);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$List(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:387:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:388:5
if (!scanToken(LIST)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:388:11
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:389:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:389:5
pushOntoLookaheadStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 389, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:390:5
if (!scanToken(AS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:391:5
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:392:5
Token token10 = currentLookaheadToken;
boolean passedPredicate10 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$393$7(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token10;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate10;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:396:5
Token token12 = currentLookaheadToken;
boolean passedPredicate12 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$396$7(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token12;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate12;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:397:5
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:398:5
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:398:5
pushOntoLookaheadStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 398, 5);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:399:5
if (!scanToken(END_DIRECTIVE1, END_DIRECTIVE2)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Nested(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:403:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:404:5
if (!scanToken(NESTED)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:405:5
Token token6 = currentLookaheadToken;
boolean passedPredicate6 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$405$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token6;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate6;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:406:5
if (!scanToken(CLOSE_TAG, CLOSE_EMPTY_TAG)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$MacroDefinition(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:413:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:415:7
if (!scanToken(MACRO, FUNCTION)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:419:5
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:420:5
if (!scanToken(IDENTIFIER, STRING_LITERAL)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:421:5
Token token9 = currentLookaheadToken;
boolean passedPredicate9 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(OPEN_PAREN)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token9;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate9;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:422:5
Token token11 = currentLookaheadToken;
boolean passedPredicate11 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$422$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token11;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate11;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:423:5
Token token13 = currentLookaheadToken;
boolean passedPredicate13 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(CLOSE_PAREN)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token13;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate13;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:424:5
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:425:5
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:425:5
pushOntoLookaheadStack("MacroDefinition", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 425, 5);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:426:5
if (!scanToken(END_DIRECTIVE1, END_DIRECTIVE2)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$ParameterList(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:433:5
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:434:5
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$434$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:435:5
boolean passedPredicate6 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token7 = currentLookaheadToken;
passedPredicate = false;
if (!check$FTL_ccc$436$8(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token7;
break;
}
}
} finally {
passedPredicate = passedPredicate6;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Text(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for OneOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:469:5
if (!scanToken(WHITESPACE, SPECIAL_CHAR, PRINTABLE_CHARS)) return false;
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!scanToken(WHITESPACE, SPECIAL_CHAR, PRINTABLE_CHARS)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$TopLevelDirective(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:478:6
Token token4 = currentLookaheadToken;
int remainingLookahead4 = remainingLookahead;
boolean hitFailure4 = hitFailure;
boolean passedPredicate4 = passedPredicate;
try {
passedPredicate = false;
if (!check$FTL_ccc$478$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$479$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$480$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$481$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$482$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$483$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$484$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$485$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$486$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$487$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$488$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$489$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$490$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
return false;
}
}
}
}
}
}
}
}
}
}
}
}
}
} finally {
passedPredicate = passedPredicate4;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$UserDirective(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:500:9
if (!scanToken(USER_DIRECTIVE_OPEN1, USER_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:504:5
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:504:18
Token token6 = currentLookaheadToken;
boolean passedPredicate6 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$504$20(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token6;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate6;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for CodeBlock specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:505:5
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:506:5
Token token9 = currentLookaheadToken;
boolean passedPredicate9 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(BLANK)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token9;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate9;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:507:5
Token token11 = currentLookaheadToken;
boolean passedPredicate11 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$507$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token11;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate11;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:508:5
Token token13 = currentLookaheadToken;
boolean passedPredicate13 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$508$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token13;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate13;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:510:8
Token token16 = currentLookaheadToken;
int remainingLookahead16 = remainingLookahead;
boolean hitFailure16 = hitFailure;
boolean passedPredicate16 = passedPredicate;
try {
passedPredicate = false;
if (!scanToken(CLOSE_EMPTY_TAG)) {
currentLookaheadToken = token16;
remainingLookahead = remainingLookahead16;
hitFailure = hitFailure16;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$512$8(false)) {
currentLookaheadToken = token16;
remainingLookahead = remainingLookahead16;
hitFailure = hitFailure16;
return false;
}
}
} finally {
passedPredicate = passedPredicate16;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Block(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:521:3
boolean passedPredicate3 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token4 = currentLookaheadToken;
passedPredicate = false;
if (!check$FTL_ccc$522$5(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
break;
}
}
} finally {
passedPredicate = passedPredicate3;
}
hitFailure = false;
return true;
}
private ArrayList parsingStack = new ArrayList<>();
private final ArrayList lookaheadStack = new ArrayList<>();
private void pushOntoCallStack(String methodName, String fileName, int line, int column) {
parsingStack.add(new NonTerminalCall("FreshMarkerParser", token_source, fileName, methodName, line, column));
}
private void popCallStack() {
NonTerminalCall ntc = parsingStack.remove(parsingStack.size() - 1);
this.currentlyParsedProduction = ntc.productionName;
}
private void restoreCallStack(int prevSize) {
while (parsingStack.size() > prevSize) {
popCallStack();
}
}
private void pushOntoLookaheadStack(String methodName, String fileName, int line, int column) {
lookaheadStack.add(new NonTerminalCall("FreshMarkerParser", token_source, fileName, methodName, line, column));
}
private void popLookaheadStack() {
NonTerminalCall ntc = lookaheadStack.remove(lookaheadStack.size() - 1);
this.currentLookaheadProduction = ntc.productionName;
}
void dumpLookaheadStack(PrintStream ps) {
ListIterator it = lookaheadStack.listIterator(lookaheadStack.size());
while (it.hasPrevious()) {
it.previous().dump(ps);
}
}
void dumpCallStack(PrintStream ps) {
ListIterator it = parsingStack.listIterator(parsingStack.size());
while (it.hasPrevious()) {
it.previous().dump(ps);
}
}
void dumpLookaheadCallStack(PrintStream ps) {
ps.println("Current Parser Production is: " + currentlyParsedProduction);
ps.println("Current Lookahead Production is: " + currentLookaheadProduction);
ps.println("---Lookahead Stack---");
dumpLookaheadStack(ps);
ps.println("---Call Stack---");
dumpCallStack(ps);
}
public boolean isParserTolerant() {
return false;
}
public void setParserTolerant(boolean tolerantParsing) {
if (tolerantParsing) {
throw new UnsupportedOperationException("This parser was not built with that feature!");
}
}
private Token consumeToken(TokenType expectedType) {
Token nextToken = nextToken(lastConsumedToken);
if (nextToken.getType() != expectedType) {
nextToken = handleUnexpectedTokenType(expectedType, nextToken);
}
this.lastConsumedToken = nextToken;
this.nextTokenType = null;
if (buildTree && tokensAreNodes) {
lastConsumedToken.open();
pushNode(lastConsumedToken);
lastConsumedToken.close();
}
return lastConsumedToken;
}
private Token handleUnexpectedTokenType(TokenType expectedType, Token nextToken) {
throw new ParseException(nextToken, EnumSet.of(expectedType), parsingStack);
}
private boolean buildTree = true;
private boolean tokensAreNodes = true;
private boolean unparsedTokensAreNodes = false;
public boolean isTreeBuildingEnabled() {
return buildTree;
}
public void setUnparsedTokensAreNodes(boolean unparsedTokensAreNodes) {
this.unparsedTokensAreNodes = unparsedTokensAreNodes;
}
public void setTokensAreNodes(boolean tokensAreNodes) {
this.tokensAreNodes = tokensAreNodes;
}
NodeScope currentNodeScope = new NodeScope();
/**
* @return the root node of the AST. It only makes sense to call
* this after a successful parse.
*/
public Node rootNode() {
return currentNodeScope.rootNode();
}
/**
* push a node onto the top of the node stack
* @param n the node to push
*/
public void pushNode(Node n) {
currentNodeScope.add(n);
}
/**
* @return the node on the top of the stack, and remove it from the
* stack.
*/
public Node popNode() {
return currentNodeScope.pop();
}
/**
* @return the node currently on the top of the tree-building stack.
*/
public Node peekNode() {
return currentNodeScope.peek();
}
/**
* Puts the node on the top of the stack. However, unlike pushNode()
* it replaces the node that is currently on the top of the stack.
* This is effectively equivalent to popNode() followed by pushNode(n)
* @param n the node to poke
*/
public void pokeNode(Node n) {
currentNodeScope.poke(n);
}
/**
* @return the number of Nodes on the tree-building stack in the current node
* scope.
*/
public int nodeArity() {
return currentNodeScope.size();
}
private void clearNodeScope() {
currentNodeScope.clear();
}
private void openNodeScope(Node n) {
new NodeScope();
if (n != null) {
n.setTokenSource(lastConsumedToken.getTokenSource());
// We set the begin/end offsets based on the ending location
// of the last consumed token. So, we start with a Node
// of length zero. Typically this is overridden in the
// closeNodeScope() method, unless this node has no children
n.setBeginOffset(lastConsumedToken.getEndOffset());
n.setEndOffset(n.getBeginOffset());
n.setTokenSource(this.token_source);
n.open();
}
}
/* A definite node is constructed from a specified number of
* children. That number of nodes are popped from the stack and
* made the children of the definite node. Then the definite node
* is pushed on to the stack.
* @param n is the node whose scope is being closed
* @param num is the number of child nodes to pop as children
* @return @{code true}
*/
private boolean closeNodeScope(Node n, int num) {
n.setBeginOffset(lastConsumedToken.getEndOffset());
n.setEndOffset(lastConsumedToken.getEndOffset());
currentNodeScope.close();
ArrayList nodes = new ArrayList<>();
for (int i = 0; i < num; i++) {
nodes.add(popNode());
}
Collections.reverse(nodes);
for (Node child : nodes) {
if (child.getInputSource() == n.getInputSource()) {
n.setBeginOffset(child.getBeginOffset());
break;
}
}
for (Node child : nodes) {
if (unparsedTokensAreNodes && child instanceof Token) {
Token tok = (Token) child;
while (tok.previousCachedToken() != null && tok.previousCachedToken().isUnparsed()) {
tok = tok.previousCachedToken();
}
boolean locationSet = false;
while (tok.isUnparsed()) {
n.add(tok);
if (!locationSet && tok.getInputSource() == n.getInputSource() && tok.getBeginOffset() < n.getBeginOffset()) {
n.setBeginOffset(tok.getBeginOffset());
locationSet = true;
}
tok = tok.nextCachedToken();
}
}
if (child.getInputSource() == n.getInputSource()) {
n.setEndOffset(child.getEndOffset());
}
n.add(child);
}
n.close();
pushNode(n);
return true;
}
/**
* A conditional node is constructed if the condition is true. All
* the nodes that have been pushed since the node was opened are
* made children of the conditional node, which is then pushed
* on to the stack. If the condition is false the node is not
* constructed and they are left on the stack.
*/
private boolean closeNodeScope(Node n, boolean condition) {
if (n == null || !condition) {
currentNodeScope.close();
return false;
}
return closeNodeScope(n, nodeArity());
}
public boolean getBuildTree() {
return buildTree;
}
public void setBuildTree(boolean buildTree) {
this.buildTree = buildTree;
}
@SuppressWarnings("serial")
class NodeScope extends ArrayList {
NodeScope parentScope;
NodeScope() {
this.parentScope = FreshMarkerParser.this.currentNodeScope;
FreshMarkerParser.this.currentNodeScope = this;
}
boolean isRootScope() {
return parentScope == null;
}
Node rootNode() {
NodeScope ns = this;
while (ns.parentScope != null) {
ns = ns.parentScope;
}
return ns.isEmpty() ? null : ns.get(0);
}
Node peek() {
if (isEmpty()) {
return parentScope == null ? null : parentScope.peek();
}
return get(size() - 1);
}
Node pop() {
return isEmpty() ? parentScope.pop() : remove(size() - 1);
}
void poke(Node n) {
if (isEmpty()) {
parentScope.poke(n);
} else {
set(size() - 1, n);
}
}
void close() {
parentScope.addAll(this);
FreshMarkerParser.this.currentNodeScope = parentScope;
}
int nestingLevel() {
int result = 0;
NodeScope parent = this;
while (parent.parentScope != null) {
result++;
parent = parent.parentScope;
}
return result;
}
public NodeScope clone() {
NodeScope clone = (NodeScope) super.clone();
if (parentScope != null) {
clone.parentScope = parentScope.clone();
}
return clone;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy