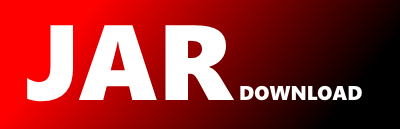
ftl.FreshMarkerParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of freshmarker Show documentation
Show all versions of freshmarker Show documentation
A simple, small but powerful template engine based loosely on the FreeMarker syntax. FreshMarker is
implemented in Java 21 and supports the `java.time` API and Records.
/* Generated by: CongoCC Parser Generator. FreshMarkerParser.java */
package ftl;
import java.util.*;
import java.io.*;
import java.io.IOException;
import java.io.PrintStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.charset.Charset;
import java.util.Arrays;
import java.util.ArrayList;
import java.util.Collections;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.ListIterator;
import java.util.concurrent.CancellationException;
import ftl.Token.TokenType;
import static ftl.Token.TokenType.*;
import ftl.ast.Expression;
import ftl.ast.OrExpression;
import ftl.ast.AndExpression;
import ftl.ast.EqualityExpression;
import ftl.ast.RelationalExpression;
import ftl.ast.RangeExpression;
import ftl.ast.AdditiveExpression;
import ftl.ast.MultiplicativeExpression;
import ftl.ast.UnaryPlusMinusExpression;
import ftl.ast.NotExpression;
import ftl.ast.BuiltinVariable;
import ftl.ast.DefaultToExpression;
import ftl.ast.PrimaryExpression;
import ftl.ast.BaseExpression;
import ftl.ast.DotKey;
import ftl.ast.DynamicKey;
import ftl.ast.MethodInvoke;
import ftl.ast.BuiltIn;
import ftl.ast.Exists;
import ftl.ast.ListLiteral;
import ftl.ast.StringLiteral;
import ftl.ast.HashLiteral;
import ftl.ast.NumberLiteral;
import ftl.ast.BooleanLiteral;
import ftl.ast.NullLiteral;
import ftl.ast.Parenthesis;
import ftl.ast.NamedArgsList;
import ftl.ast.PositionalArgsList;
import ftl.ast.Interpolation;
import ftl.ast.Assignment;
import ftl.ast.VarInstruction;
import ftl.ast.SettingInstruction;
import ftl.ast.CommentBlock;
import ftl.ast.BreakInstruction;
import ftl.ast.ReturnInstruction;
import ftl.ast.OutputFormatBlock;
import ftl.ast.BrickInstruction;
import ftl.ast.ImportInstruction;
import ftl.ast.IfStatement;
import ftl.ast.ElseIfBlock;
import ftl.ast.ElseBlock;
import ftl.ast.SwitchInstruction;
import ftl.ast.CaseInstruction;
import ftl.ast.DefaultInstruction;
import ftl.ast.ListInstruction;
import ftl.ast.NestedInstruction;
import ftl.ast.MacroDefinition;
import ftl.ast.ParameterList;
import ftl.ast.LooseTagEnd;
import ftl.ast.DirectiveEnd;
import ftl.ast.Text;
import ftl.ast.UserDirective;
import ftl.ast.Block;
import ftl.ast.Root;
public class FreshMarkerParser {
static final int UNLIMITED = Integer.MAX_VALUE;
// The last token successfully "consumed"
Token lastConsumedToken;
private TokenType nextTokenType;
// Normally null when parsing, populated when doing lookahead
private Token currentLookaheadToken;
private int remainingLookahead;
private boolean hitFailure;
private boolean passedPredicate;
private int passedPredicateThreshold = -1;
private String currentlyParsedProduction;
private String currentLookaheadProduction;
private int lookaheadRoutineNesting;
private final boolean legacyGlitchyLookahead = false;
private final Token DUMMY_START_TOKEN = new Token();
private boolean cancelled;
public void cancel() {
cancelled = true;
}
public boolean isCancelled() {
return cancelled;
}
/** Generated Lexer. */
private FreshMarkerLexer token_source;
public void setInputSource(String inputSource) {
token_source.setInputSource(inputSource);
}
String getInputSource() {
return token_source.getInputSource();
}
//=================================
// Generated constructors
//=================================
public FreshMarkerParser(String inputSource, CharSequence content) {
this(new FreshMarkerLexer(inputSource, content));
}
public FreshMarkerParser(CharSequence content) {
this("input", content);
}
/**
* @param inputSource just the name of the input source (typically the filename) that
* will be used in error messages and so on.
* @param path The location (typically the filename) from which to get the input to parse
*/
public FreshMarkerParser(String inputSource, Path path) throws IOException {
this(inputSource, TokenSource.stringFromBytes(Files.readAllBytes(path)));
}
public FreshMarkerParser(String inputSource, Path path, Charset charset) throws IOException {
this(inputSource, TokenSource.stringFromBytes(Files.readAllBytes(path), charset));
}
/**
* @param path The location (typically the filename) from which to get the input to parse
*/
public FreshMarkerParser(Path path) throws IOException {
this(path.toString(), path);
}
/** Constructor with user supplied Lexer. */
public FreshMarkerParser(FreshMarkerLexer lexer) {
token_source = lexer;
lastConsumedToken = DUMMY_START_TOKEN;
lastConsumedToken.setTokenSource(lexer);
}
/**
* Set the starting line/column for location reporting.
* By default, this is 1,1.
*/
public void setStartingPos(int startingLine, int startingColumn) {
token_source.setStartingPos(startingLine, startingColumn);
}
// this method is for testing only.
public boolean getLegacyGlitchyLookahead() {
return legacyGlitchyLookahead;
}
// If the next token is cached, it returns that
// Otherwise, it goes to the token_source, i.e. the Lexer.
private Token nextToken(final Token tok) {
Token result = token_source.getNextToken(tok);
while (result.isUnparsed()) {
result = token_source.getNextToken(result);
}
nextTokenType = null;
return result;
}
/**
* @return the next Token off the stream. This is the same as #getToken(1)
*/
public final Token getNextToken() {
return getToken(1);
}
/**
* @param index how many tokens to look ahead
* @return the specific regular (i.e. parsed) Token index ahead/behind in the stream.
* If we are in a lookahead, it looks ahead from the currentLookaheadToken
* Otherwise, it is the lastConsumedToken. If you pass in a negative
* number it goes backward.
*/
public final Token getToken(final int index) {
Token t = currentLookaheadToken == null ? lastConsumedToken : currentLookaheadToken;
for (int i = 0; i < index; i++) {
t = nextToken(t);
}
for (int i = 0; i > index; i--) {
t = t.getPrevious();
if (t == null) break;
}
return t;
}
private TokenType nextTokenType() {
if (nextTokenType == null) {
nextTokenType = nextToken(lastConsumedToken).getType();
}
return nextTokenType;
}
boolean activateTokenTypes(TokenType...types) {
if (token_source.activeTokenTypes == null) return false;
boolean result = false;
for (TokenType tt : types) {
result |= token_source.activeTokenTypes.add(tt);
}
if (result) {
token_source.reset(getToken(0));
nextTokenType = null;
}
return result;
}
boolean deactivateTokenTypes(TokenType...types) {
boolean result = false;
if (token_source.activeTokenTypes == null) {
token_source.activeTokenTypes = EnumSet.allOf(TokenType.class);
}
for (TokenType tt : types) {
result |= token_source.activeTokenTypes.remove(tt);
}
if (result) {
token_source.reset(getToken(0));
nextTokenType = null;
}
return result;
}
private static final HashMap> enumSetCache = new HashMap<>();
private static EnumSet tokenTypeSet(TokenType first, TokenType...rest) {
TokenType[] key = new TokenType[1 + rest.length];
key[0] = first;
if (rest.length > 0) {
System.arraycopy(rest, 0, key, 1, rest.length);
}
Arrays.sort(key);
if (enumSetCache.containsKey(key)) {
return enumSetCache.get(key);
}
EnumSet result = (rest.length == 0) ? EnumSet.of(first) : EnumSet.of(first, rest);
enumSetCache.put(key, result);
return result;
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:181:1
final public Node Input() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Input";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:181:1
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:182:4
pushOntoCallStack("Input", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 182, 4);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:183:4
consumeToken(EOF);
// Code for CodeBlock specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:184:4
return rootNode();
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:189:1
final public void Expression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Expression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:189:1
Expression thisProduction = null;
if (buildTree) {
thisProduction = new Expression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:190:5
pushOntoCallStack("Expression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 190, 5);
try {
OrExpression();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:193:1
final public void OrExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "OrExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:193:1
OrExpression thisProduction = null;
if (buildTree) {
thisProduction = new OrExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:194:5
pushOntoCallStack("OrExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 194, 5);
try {
AndExpression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:195:5
while (true) {
if (!(nextTokenType() == OR2 || nextTokenType == OR || nextTokenType == XOR)) break;
if (nextTokenType() == OR) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:196:10
consumeToken(OR);
} else if (nextTokenType() == OR2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:196:15
consumeToken(OR2);
} else if (nextTokenType() == XOR) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:196:21
consumeToken(XOR);
} else {
pushOntoCallStack("OrExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 196, 10);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$196$10, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:196:28
pushOntoCallStack("OrExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 196, 28);
try {
AndExpression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:200:1
final public void AndExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "AndExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:200:1
AndExpression thisProduction = null;
if (buildTree) {
thisProduction = new AndExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:201:5
pushOntoCallStack("AndExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 201, 5);
try {
EqualityExpression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:202:5
while (true) {
if (!(nextTokenType() == AND2 || nextTokenType == AND)) break;
if (nextTokenType() == AND) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:203:10
consumeToken(AND);
} else if (nextTokenType() == AND2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:203:16
consumeToken(AND2);
} else {
pushOntoCallStack("AndExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 203, 10);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$203$10, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:203:24
pushOntoCallStack("AndExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 203, 24);
try {
EqualityExpression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:207:1
final public void EqualityExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "EqualityExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:207:1
EqualityExpression thisProduction = null;
if (buildTree) {
thisProduction = new EqualityExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:208:5
pushOntoCallStack("EqualityExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 208, 5);
try {
RelationalExpression();
} finally {
popCallStack();
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:209:5
if (nextTokenType() == EQUALS || nextTokenType == DOUBLE_EQUALS || nextTokenType == NOT_EQUALS) {
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:210:10
consumeToken(EQUALS);
} else if (nextTokenType() == DOUBLE_EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:210:19
consumeToken(DOUBLE_EQUALS);
} else if (nextTokenType() == NOT_EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:210:35
consumeToken(NOT_EQUALS);
} else {
pushOntoCallStack("EqualityExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 210, 10);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$210$10, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:211:9
pushOntoCallStack("EqualityExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 211, 9);
try {
RelationalExpression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:215:1
final public void RelationalExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "RelationalExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:215:1
RelationalExpression thisProduction = null;
if (buildTree) {
thisProduction = new RelationalExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:216:5
pushOntoCallStack("RelationalExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 216, 5);
try {
RangeExpression();
} finally {
popCallStack();
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:217:5
if (nextTokenType() == LT || nextTokenType == GT || nextTokenType == LTE || nextTokenType == GTE) {
if (nextTokenType() == GT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:218:10
consumeToken(GT);
} else if (nextTokenType() == GTE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:218:15
consumeToken(GTE);
} else if (nextTokenType() == LT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:218:21
consumeToken(LT);
} else if (nextTokenType() == LTE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:218:26
consumeToken(LTE);
} else {
pushOntoCallStack("RelationalExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 218, 10);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$218$10, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:219:9
pushOntoCallStack("RelationalExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 219, 9);
try {
RangeExpression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:223:1
final public void RangeExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "RangeExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:223:1
RangeExpression thisProduction = null;
if (buildTree) {
thisProduction = new RangeExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:224:5
pushOntoCallStack("RangeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 224, 5);
try {
AdditiveExpression();
} finally {
popCallStack();
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:225:5
if (nextTokenType() == DOT_DOT || nextTokenType == DOT_DOT_EXCLUSIVE || nextTokenType == DOT_DOT_LENGTH) {
if (nextTokenType() == DOT_DOT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:226:9
consumeToken(DOT_DOT);
} else if (nextTokenType() == DOT_DOT_EXCLUSIVE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:226:19
consumeToken(DOT_DOT_EXCLUSIVE);
} else if (nextTokenType() == DOT_DOT_LENGTH) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:226:39
consumeToken(DOT_DOT_LENGTH);
} else {
pushOntoCallStack("RangeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 226, 9);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$226$9, parsingStack);
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:227:8
if (scan$FEL_ccc$228$11()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:228:11
pushOntoCallStack("RangeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 228, 11);
try {
AdditiveExpression();
} finally {
popCallStack();
}
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:233:1
final public void AdditiveExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "AdditiveExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:233:1
AdditiveExpression thisProduction = null;
if (buildTree) {
thisProduction = new AdditiveExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:234:5
pushOntoCallStack("AdditiveExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 234, 5);
try {
MultiplicativeExpression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:235:5
while (true) {
if (!(nextTokenType() == PLUS || nextTokenType == MINUS)) break;
if (nextTokenType() == PLUS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:236:13
consumeToken(PLUS);
} else if (nextTokenType() == MINUS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:236:20
consumeToken(MINUS);
} else {
pushOntoCallStack("AdditiveExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 236, 13);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$236$13, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:237:12
pushOntoCallStack("AdditiveExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 237, 12);
try {
MultiplicativeExpression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:241:1
final public void MultiplicativeExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "MultiplicativeExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:241:1
MultiplicativeExpression thisProduction = null;
if (buildTree) {
thisProduction = new MultiplicativeExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:242:5
pushOntoCallStack("MultiplicativeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 242, 5);
try {
UnaryExpression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:243:5
while (true) {
if (!(nextTokenType() == PERCENT || nextTokenType == TIMES || nextTokenType == DIVIDE)) break;
if (nextTokenType() == TIMES) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:244:12
consumeToken(TIMES);
} else if (nextTokenType() == DIVIDE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:244:20
consumeToken(DIVIDE);
} else if (nextTokenType() == PERCENT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:244:29
consumeToken(PERCENT);
} else {
pushOntoCallStack("MultiplicativeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 244, 12);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$244$12, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:245:11
pushOntoCallStack("MultiplicativeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 245, 11);
try {
UnaryExpression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
private static final EnumSet UnaryExpression_FIRST_SET = UnaryExpression_FIRST_SET_init();
private static EnumSet UnaryExpression_FIRST_SET_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NOT, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:250:1
final public void UnaryExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "UnaryExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:250:1
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:251:5
if (nextTokenType() == PLUS || nextTokenType == MINUS) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:251:5
pushOntoCallStack("UnaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 251, 5);
try {
UnaryPlusMinusExpression();
} finally {
popCallStack();
}
} else if (nextTokenType() == EXCLAM || nextTokenType == NOT) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:253:5
pushOntoCallStack("UnaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 253, 5);
try {
NotExpression();
} finally {
popCallStack();
}
} else if (first_set$FEL_ccc$255$5.contains(nextTokenType())) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:255:5
pushOntoCallStack("UnaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 255, 5);
try {
DefaultToExpression();
} finally {
popCallStack();
}
} else {
pushOntoCallStack("UnaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 251, 5);
throw new ParseException(lastConsumedToken, UnaryExpression_FIRST_SET, parsingStack);
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:258:1
final public void UnaryPlusMinusExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "UnaryPlusMinusExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:258:1
UnaryPlusMinusExpression thisProduction = null;
if (buildTree) {
thisProduction = new UnaryPlusMinusExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == PLUS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:259:6
consumeToken(PLUS);
} else if (nextTokenType() == MINUS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:259:13
consumeToken(MINUS);
} else {
pushOntoCallStack("UnaryPlusMinusExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 259, 6);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$259$6, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:259:22
pushOntoCallStack("UnaryPlusMinusExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 259, 22);
try {
DefaultToExpression();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:262:1
final public void NotExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "NotExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:262:1
NotExpression thisProduction = null;
if (buildTree) {
thisProduction = new NotExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == EXCLAM) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:263:6
consumeToken(EXCLAM);
} else if (nextTokenType() == NOT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:263:17
consumeToken(NOT);
} else {
pushOntoCallStack("NotExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 263, 6);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$263$6, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:264:5
pushOntoCallStack("NotExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 264, 5);
try {
DefaultToExpression();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:267:1
final public void BuiltinVariable() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "BuiltinVariable";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:267:1
BuiltinVariable thisProduction = null;
if (buildTree) {
thisProduction = new BuiltinVariable();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:268:5
consumeToken(DOT);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:268:10
consumeToken(IDENTIFIER);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:271:1
final public void DefaultToExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "DefaultToExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:271:1
DefaultToExpression thisProduction = null;
if (buildTree) {
thisProduction = new DefaultToExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:272:5
pushOntoCallStack("DefaultToExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 272, 5);
try {
PrimaryExpression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:273:5
while (true) {
if (!((getToken(2).getType() != IDENTIFIER || getToken(3).getType() != EQUALS) && scan$FEL_ccc$275$9())) break;
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:276:12
consumeToken(EXCLAM);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:276:20
pushOntoCallStack("DefaultToExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 276, 20);
try {
PrimaryExpression();
} finally {
popCallStack();
}
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:278:5
if (nextTokenType() == EXCLAM) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:279:8
consumeToken(EXCLAM);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:283:1
final public void PrimaryExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "PrimaryExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:283:1
PrimaryExpression thisProduction = null;
if (buildTree) {
thisProduction = new PrimaryExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:284:3
pushOntoCallStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 284, 3);
try {
BaseExpression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:285:3
while (true) {
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:286:7
if (nextTokenType() == DOT) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:286:7
pushOntoCallStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 286, 7);
try {
DotKey();
} finally {
popCallStack();
}
} else if (nextTokenType() == DOUBLE_COLON) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:288:7
pushOntoCallStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 288, 7);
try {
MethodReference();
} finally {
popCallStack();
}
} else if (nextTokenType() == OPEN_BRACKET) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:290:7
pushOntoCallStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 290, 7);
try {
DynamicKey();
} finally {
popCallStack();
}
} else if (nextTokenType() == OPEN_PAREN) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:292:7
pushOntoCallStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 292, 7);
try {
MethodInvoke();
} finally {
popCallStack();
}
} else if (nextTokenType() == BUILT_IN) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:294:7
pushOntoCallStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 294, 7);
try {
BuiltIn();
} finally {
popCallStack();
}
} else if (nextTokenType() == EXISTS_OPERATOR) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:296:7
pushOntoCallStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 296, 7);
try {
Exists();
} finally {
popCallStack();
}
} else {
break;
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
private static final EnumSet BaseExpression_FIRST_SET = BaseExpression_FIRST_SET_init();
private static EnumSet BaseExpression_FIRST_SET_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:300:1
final public void BaseExpression() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "BaseExpression";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:300:1
BaseExpression thisProduction = null;
if (buildTree) {
thisProduction = new BaseExpression();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:301:5
if (nextTokenType() == IDENTIFIER) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:301:5
consumeToken(IDENTIFIER);
} else if (nextTokenType() == INTEGER || nextTokenType == DECIMAL) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:303:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 303, 5);
try {
NumberLiteral();
} finally {
popCallStack();
}
} else if (nextTokenType() == OPEN_BRACE) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:305:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 305, 5);
try {
HashLiteral();
} finally {
popCallStack();
}
} else if (nextTokenType() == STRING_LITERAL) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:307:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 307, 5);
try {
StringLiteral();
} finally {
popCallStack();
}
} else if (nextTokenType() == TRUE || nextTokenType == FALSE) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:309:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 309, 5);
try {
BooleanLiteral();
} finally {
popCallStack();
}
} else if (nextTokenType() == NULL) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:311:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 311, 5);
try {
NullLiteral();
} finally {
popCallStack();
}
} else if (nextTokenType() == OPEN_BRACKET) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:313:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 313, 5);
try {
ListLiteral();
} finally {
popCallStack();
}
} else if (nextTokenType() == OPEN_PAREN) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:315:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 315, 5);
try {
Parenthesis();
} finally {
popCallStack();
}
} else if (nextTokenType() == DOT) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:317:5
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 317, 5);
try {
BuiltinVariable();
} finally {
popCallStack();
}
} else {
pushOntoCallStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 301, 5);
throw new ParseException(lastConsumedToken, BaseExpression_FIRST_SET, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:320:1
final public void DotKey() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "DotKey";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:320:1
DotKey thisProduction = null;
if (buildTree) {
thisProduction = new DotKey();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:321:5
consumeToken(DOT);
if (nextTokenType() == IDENTIFIER) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:322:6
consumeToken(IDENTIFIER);
} else if (nextTokenType() == TIMES) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:322:19
consumeToken(TIMES);
} else {
pushOntoCallStack("DotKey", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 322, 6);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$322$6, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:325:1
final public void MethodReference() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "MethodReference";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:325:1
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:326:5
consumeToken(DOUBLE_COLON);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:327:5
consumeToken(IDENTIFIER);
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:330:1
final public void DynamicKey() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "DynamicKey";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:330:1
DynamicKey thisProduction = null;
if (buildTree) {
thisProduction = new DynamicKey();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:331:5
consumeToken(OPEN_BRACKET);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:331:19
pushOntoCallStack("DynamicKey", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 331, 19);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:331:29
consumeToken(CLOSE_BRACKET);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:334:1
final public void MethodInvoke() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "MethodInvoke";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:334:1
MethodInvoke thisProduction = null;
if (buildTree) {
thisProduction = new MethodInvoke();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:335:3
consumeToken(OPEN_PAREN);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:335:15
if (first_set$FEL_ccc$335$16.contains(nextTokenType())) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:335:16
pushOntoCallStack("MethodInvoke", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 335, 16);
try {
ArgsList();
} finally {
popCallStack();
}
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:335:25
consumeToken(CLOSE_PAREN);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:1
final public void BuiltIn() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "BuiltIn";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:338:1
BuiltIn thisProduction = null;
if (buildTree) {
thisProduction = new BuiltIn();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:5
consumeToken(BUILT_IN);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:15
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:27
if (nextTokenType() == OPEN_PAREN) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:28
consumeToken(OPEN_PAREN);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:40
if (first_set$FEL_ccc$339$41.contains(nextTokenType())) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:41
pushOntoCallStack("BuiltIn", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 339, 41);
try {
ArgsList();
} finally {
popCallStack();
}
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:50
consumeToken(CLOSE_PAREN);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:342:1
final public void Exists() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Exists";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:342:1
Exists thisProduction = null;
if (buildTree) {
thisProduction = new Exists();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:343:5
consumeToken(EXISTS_OPERATOR);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:346:1
final public void ListLiteral() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "ListLiteral";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:346:1
ListLiteral thisProduction = null;
if (buildTree) {
thisProduction = new ListLiteral();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:5
consumeToken(OPEN_BRACKET);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:5
if (first_set$FEL_ccc$348$6.contains(nextTokenType())) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:6
pushOntoCallStack("ListLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 348, 6);
try {
Expression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:17
while (true) {
if (!(first_set$FEL_ccc$348$18.contains(nextTokenType()))) break;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:18
if (nextTokenType() == COMMA) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:19
consumeToken(COMMA);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:27
pushOntoCallStack("ListLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 348, 27);
try {
Expression();
} finally {
popCallStack();
}
}
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:349:5
consumeToken(CLOSE_BRACKET);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:352:1
final public void StringLiteral() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "StringLiteral";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:352:1
StringLiteral thisProduction = null;
if (buildTree) {
thisProduction = new StringLiteral();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:353:5
consumeToken(STRING_LITERAL);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:357:1
final public void HashLiteral() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "HashLiteral";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:357:1
HashLiteral thisProduction = null;
if (buildTree) {
thisProduction = new HashLiteral();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:358:5
consumeToken(OPEN_BRACE);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:359:5
if (first_set$FEL_ccc$360$8.contains(nextTokenType())) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:8
pushOntoCallStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 360, 8);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:19
consumeToken(COLON);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:27
pushOntoCallStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 360, 27);
try {
Expression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:361:8
while (true) {
if (!(nextTokenType() == COMMA)) break;
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:361:9
consumeToken(COMMA);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:361:17
pushOntoCallStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 361, 17);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:361:28
consumeToken(COLON);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:361:36
pushOntoCallStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 361, 36);
try {
Expression();
} finally {
popCallStack();
}
}
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:363:5
consumeToken(CLOSE_BRACE);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
private static final EnumSet NumberLiteral_FIRST_SET = tokenTypeSet(INTEGER, DECIMAL);
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:366:1
final public void NumberLiteral() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "NumberLiteral";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:366:1
NumberLiteral thisProduction = null;
if (buildTree) {
thisProduction = new NumberLiteral();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:367:5
if (nextTokenType() == INTEGER) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:367:5
consumeToken(INTEGER);
} else if (nextTokenType() == DECIMAL) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:367:15
consumeToken(DECIMAL);
} else {
pushOntoCallStack("NumberLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 367, 5);
throw new ParseException(lastConsumedToken, NumberLiteral_FIRST_SET, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
private static final EnumSet BooleanLiteral_FIRST_SET = tokenTypeSet(TRUE, FALSE);
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:370:1
final public void BooleanLiteral() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "BooleanLiteral";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:370:1
BooleanLiteral thisProduction = null;
if (buildTree) {
thisProduction = new BooleanLiteral();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:371:5
if (nextTokenType() == TRUE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:371:5
consumeToken(TRUE);
} else if (nextTokenType() == FALSE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:371:12
consumeToken(FALSE);
} else {
pushOntoCallStack("BooleanLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 371, 5);
throw new ParseException(lastConsumedToken, BooleanLiteral_FIRST_SET, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:374:1
final public void NullLiteral() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "NullLiteral";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:374:1
NullLiteral thisProduction = null;
if (buildTree) {
thisProduction = new NullLiteral();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:374:15
consumeToken(NULL);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:376:1
final public void Parenthesis() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Parenthesis";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:376:1
Parenthesis thisProduction = null;
if (buildTree) {
thisProduction = new Parenthesis();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:377:5
consumeToken(OPEN_PAREN);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:377:18
pushOntoCallStack("Parenthesis", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 377, 18);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:377:29
consumeToken(CLOSE_PAREN);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:380:1
final public void ArgsList() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "ArgsList";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:380:1
if (scan$FEL_ccc$382$9()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:383:9
pushOntoCallStack("ArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 383, 9);
try {
NamedArgsList();
} finally {
popCallStack();
}
} else if (first_set$FEL_ccc$385$9.contains(nextTokenType())) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:385:9
pushOntoCallStack("ArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 385, 9);
try {
PositionalArgsList();
} finally {
popCallStack();
}
} else {
pushOntoCallStack("ArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 382, 9);
throw new ParseException(lastConsumedToken, first_set$FEL_ccc$382$9, parsingStack);
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:389:1
final public void NamedArgsList() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "NamedArgsList";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:389:1
NamedArgsList thisProduction = null;
if (buildTree) {
thisProduction = new NamedArgsList();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:390:5
consumeToken(IDENTIFIER);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:391:5
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:392:5
pushOntoCallStack("NamedArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 392, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:393:5
while (true) {
if (!(nextTokenType() == COMMA || nextTokenType == IDENTIFIER)) break;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:394:8
if (nextTokenType() == COMMA) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:394:9
consumeToken(COMMA);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:395:8
consumeToken(IDENTIFIER);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:396:8
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:397:8
pushOntoCallStack("NamedArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 397, 8);
try {
Expression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:401:1
final public void PositionalArgsList() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "PositionalArgsList";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:401:1
PositionalArgsList thisProduction = null;
if (buildTree) {
thisProduction = new PositionalArgsList();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:402:5
pushOntoCallStack("PositionalArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 402, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:403:5
while (true) {
if (!(first_set$FEL_ccc$404$8.contains(nextTokenType()))) break;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:404:8
if (nextTokenType() == COMMA) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:404:9
consumeToken(COMMA);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:405:8
pushOntoCallStack("PositionalArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 405, 8);
try {
Expression();
} finally {
popCallStack();
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:235:1
final public void Interpolation() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Interpolation";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:235:1
Interpolation thisProduction = null;
if (buildTree) {
thisProduction = new Interpolation();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:236:5
consumeToken(INTERPOLATE);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:236:18
pushOntoCallStack("Interpolation", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 236, 18);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:236:28
consumeToken(CLOSE_BRACE);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:239:1
final public void Assignment() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Assignment";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:239:1
Assignment thisProduction = null;
if (buildTree) {
thisProduction = new Assignment();
openNodeScope(thisProduction);
}
Token t;
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:243:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:243:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 243, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$243$6, parsingStack);
}
if (nextTokenType() == SET) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:245:8
t = consumeToken(SET);
} else if (nextTokenType() == ASSIGN) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:246:8
t = consumeToken(ASSIGN);
} else if (nextTokenType() == LOCAL) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:247:8
t = consumeToken(LOCAL);
} else if (nextTokenType() == GLOBAL) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:248:8
t = consumeToken(GLOBAL);
} else {
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 245, 8);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$245$8, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:250:5
consumeToken(BLANK);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:251:5
consumeToken(IDENTIFIER);
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:254:8
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:254:16
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 254, 16);
try {
Expression();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:255:8
while (true) {
if (!(nextTokenType() == COMMA || nextTokenType == IDENTIFIER)) break;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:255:9
if (nextTokenType() == COMMA) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:255:10
consumeToken(COMMA);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:255:19
consumeToken(IDENTIFIER);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:255:32
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:255:41
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 255, 41);
try {
Expression();
} finally {
popCallStack();
}
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:256:8
if (nextTokenType() == IN) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:256:9
consumeToken(IN);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:256:14
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 256, 14);
try {
Expression();
} finally {
popCallStack();
}
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:257:8
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 257, 8);
try {
LooseTagEnd();
} finally {
popCallStack();
}
} else if (nextTokenType() == IN || nextTokenType == CLOSE_TAG) {
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:261:10
if (nextTokenType() == IN) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:261:11
consumeToken(IN);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:261:16
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 261, 16);
try {
Expression();
} finally {
popCallStack();
}
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:262:10
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:263:10
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 263, 10);
try {
Block();
} finally {
popCallStack();
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:264:10
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 264, 10);
try {
DirectiveEnd(t.toString());
} finally {
popCallStack();
}
} else {
pushOntoCallStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 253, 7);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$253$7, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:269:1
final public void Var() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Var";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:269:1
VarInstruction thisProduction = null;
if (buildTree) {
thisProduction = new VarInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:270:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:270:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Var", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 270, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$270$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:271:5
consumeToken(VAR);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:271:10
consumeToken(BLANK);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:272:5
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:272:18
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:272:19
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:272:28
pushOntoCallStack("Var", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 272, 28);
try {
Expression();
} finally {
popCallStack();
}
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:5
while (true) {
if (!(nextTokenType() == COMMA || nextTokenType == IDENTIFIER)) break;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:6
if (nextTokenType() == COMMA) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:7
consumeToken(COMMA);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:16
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:29
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:30
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:39
pushOntoCallStack("Var", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 273, 39);
try {
Expression();
} finally {
popCallStack();
}
}
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:274:5
pushOntoCallStack("Var", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 274, 5);
try {
LooseTagEnd();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:1
final public void Setting() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Setting";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:277:1
SettingInstruction thisProduction = null;
if (buildTree) {
thisProduction = new SettingInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:278:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:278:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Setting", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 278, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$278$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:279:5
consumeToken(SETTING);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:279:14
consumeToken(BLANK);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:280:5
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:280:18
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:280:19
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:280:28
pushOntoCallStack("Setting", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 280, 28);
try {
Expression();
} finally {
popCallStack();
}
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:5
while (true) {
if (!(nextTokenType() == COMMA || nextTokenType == IDENTIFIER)) break;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:6
if (nextTokenType() == COMMA) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:7
consumeToken(COMMA);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:16
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:29
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:30
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:39
pushOntoCallStack("Setting", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 281, 39);
try {
Expression();
} finally {
popCallStack();
}
}
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:282:5
pushOntoCallStack("Setting", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 282, 5);
try {
LooseTagEnd();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:1
final public void Comment() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Comment";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:285:1
CommentBlock thisProduction = null;
if (buildTree) {
thisProduction = new CommentBlock();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:286:5
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:286:27
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Comment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 286, 5);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$286$5, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:287:4
consumeToken(COMMENT);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:288:4
consumeToken(END_COMMENT);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:291:1
final public void Break() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Break";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:291:1
BreakInstruction thisProduction = null;
if (buildTree) {
thisProduction = new BreakInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:292:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:292:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Break", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 292, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$292$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:293:5
consumeToken(BREAK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:294:5
pushOntoCallStack("Break", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 294, 5);
try {
LooseTagEnd();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:297:1
final public void Return() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Return";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:297:1
ReturnInstruction thisProduction = null;
if (buildTree) {
thisProduction = new ReturnInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:298:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:298:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Return", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 298, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$298$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:299:5
consumeToken(RETURN);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:300:5
if (nextTokenType() == BLANK) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:300:6
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:300:13
pushOntoCallStack("Return", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 300, 13);
try {
Expression();
} finally {
popCallStack();
}
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:301:5
pushOntoCallStack("Return", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 301, 5);
try {
LooseTagEnd();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:304:1
final public void OutputFormatBlock() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "OutputFormatBlock";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:304:1
OutputFormatBlock thisProduction = null;
if (buildTree) {
thisProduction = new OutputFormatBlock();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:305:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:305:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("OutputFormatBlock", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 305, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$305$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:306:5
consumeToken(OUTPUT_FORMAT);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:307:5
consumeToken(BLANK);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:308:5
consumeToken(STRING_LITERAL);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:309:5
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:310:5
pushOntoCallStack("OutputFormatBlock", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 310, 5);
try {
Block();
} finally {
popCallStack();
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:311:5
pushOntoCallStack("OutputFormatBlock", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 311, 5);
try {
DirectiveEnd("outputformat");
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:314:1
final public void Brick() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Brick";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:314:1
BrickInstruction thisProduction = null;
if (buildTree) {
thisProduction = new BrickInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:315:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:315:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Brick", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 315, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$315$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:316:5
consumeToken(BRICK);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:317:5
consumeToken(BLANK);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:318:5
consumeToken(STRING_LITERAL);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:319:5
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:320:5
pushOntoCallStack("Brick", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 320, 5);
try {
Block();
} finally {
popCallStack();
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:321:5
pushOntoCallStack("Brick", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 321, 5);
try {
DirectiveEnd("brick");
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:324:1
final public void Import() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Import";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:324:1
ImportInstruction thisProduction = null;
if (buildTree) {
thisProduction = new ImportInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:325:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:325:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Import", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 325, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$325$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:326:5
consumeToken(IMPORT);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:326:13
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:327:5
pushOntoCallStack("Import", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 327, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:328:5
consumeToken(AS);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:329:5
consumeToken(IDENTIFIER);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:330:5
pushOntoCallStack("Import", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 330, 5);
try {
LooseTagEnd();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:333:1
final public void If() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "If";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:333:1
IfStatement thisProduction = null;
if (buildTree) {
thisProduction = new IfStatement();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:334:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:334:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 334, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$334$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:335:5
consumeToken(IF);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:335:9
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:336:5
pushOntoCallStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 336, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:337:5
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:338:5
pushOntoCallStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 338, 5);
try {
Block();
} finally {
popCallStack();
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:339:5
while (true) {
if (!(scan$FTL_ccc$339$7())) break;
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:339:7
pushOntoCallStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 339, 7);
try {
ElseIf();
} finally {
popCallStack();
}
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:340:5
if (scan$FTL_ccc$341$8()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:341:8
pushOntoCallStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 341, 8);
try {
Else();
} finally {
popCallStack();
}
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:343:5
pushOntoCallStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 343, 5);
try {
DirectiveEnd("if");
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:346:1
final public void ElseIf() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "ElseIf";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:346:1
ElseIfBlock thisProduction = null;
if (buildTree) {
thisProduction = new ElseIfBlock();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:347:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:347:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("ElseIf", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 347, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$347$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:348:5
consumeToken(ELSEIF);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:350:5
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:351:5
pushOntoCallStack("ElseIf", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 351, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:352:5
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:353:5
pushOntoCallStack("ElseIf", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 353, 5);
try {
Block();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:356:1
final public void Else() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Else";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:356:1
ElseBlock thisProduction = null;
if (buildTree) {
thisProduction = new ElseBlock();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:357:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:357:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Else", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 357, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$357$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:358:5
consumeToken(ELSE);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:360:5
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:361:5
pushOntoCallStack("Else", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 361, 5);
try {
Block();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:364:1
final public void Switch() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Switch";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:364:1
SwitchInstruction thisProduction = null;
if (buildTree) {
thisProduction = new SwitchInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:365:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:365:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 365, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$365$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:366:5
consumeToken(SWITCH);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:367:5
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:368:5
pushOntoCallStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 368, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:369:5
consumeToken(CLOSE_TAG);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:370:5
if (nextTokenType() == WHITESPACE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:370:6
consumeToken(WHITESPACE);
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:371:5
while (true) {
if (!(scan$FTL_ccc$371$6())) break;
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:371:6
pushOntoCallStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 371, 6);
try {
Case();
} finally {
popCallStack();
}
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:372:5
if (scan$FTL_ccc$373$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:373:13
pushOntoCallStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 373, 13);
try {
Default();
} finally {
popCallStack();
}
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:375:5
pushOntoCallStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 375, 5);
try {
DirectiveEnd("switch");
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:378:1
final public void Case() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Case";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:378:1
CaseInstruction thisProduction = null;
if (buildTree) {
thisProduction = new CaseInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:379:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:379:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Case", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 379, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$379$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:380:5
consumeToken(CASE);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:382:5
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:383:5
pushOntoCallStack("Case", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 383, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:383:16
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:383:28
pushOntoCallStack("Case", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 383, 28);
try {
Block();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:386:1
final public void Default() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Default";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:386:1
DefaultInstruction thisProduction = null;
if (buildTree) {
thisProduction = new DefaultInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:387:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:387:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Default", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 387, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$387$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:388:5
consumeToken(DEFAULT);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:388:14
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:389:5
pushOntoCallStack("Default", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 389, 5);
try {
Block();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:392:1
final public void List() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "List";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:392:1
ListInstruction thisProduction = null;
if (buildTree) {
thisProduction = new ListInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:393:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:393:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 393, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$393$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:394:5
consumeToken(LIST);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:394:11
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:395:5
pushOntoCallStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 395, 5);
try {
Expression();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:396:5
consumeToken(AS);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:397:5
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:398:5
if (nextTokenType() == COMMA || nextTokenType == SORTED) {
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:399:7
if (nextTokenType() == SORTED) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:399:9
consumeToken(SORTED);
if (nextTokenType() == ASCENDING) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:399:19
consumeToken(ASCENDING);
} else if (nextTokenType() == DESCENDING) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:399:33
consumeToken(DESCENDING);
} else {
pushOntoCallStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 399, 19);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$399$19, parsingStack);
}
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:400:7
consumeToken(COMMA);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:400:15
consumeToken(IDENTIFIER);
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:402:5
if (nextTokenType() == WITH) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:402:7
consumeToken(WITH);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:402:14
consumeToken(IDENTIFIER);
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:403:5
if (nextTokenType() == FILTER) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:403:7
consumeToken(FILTER);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:403:16
pushOntoCallStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 403, 16);
try {
Expression();
} finally {
popCallStack();
}
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:404:5
if (nextTokenType() == LIMIT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:404:7
consumeToken(LIMIT);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:404:15
pushOntoCallStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 404, 15);
try {
Expression();
} finally {
popCallStack();
}
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:405:5
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:406:5
pushOntoCallStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 406, 5);
try {
Block();
} finally {
popCallStack();
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:407:5
pushOntoCallStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 407, 5);
try {
DirectiveEnd("list");
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:410:1
final public void Nested() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Nested";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:410:1
NestedInstruction thisProduction = null;
if (buildTree) {
thisProduction = new NestedInstruction();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:411:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:411:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("Nested", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 411, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$411$6, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:412:5
consumeToken(NESTED);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:413:5
if (nextTokenType() == BLANK) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:413:6
consumeToken(BLANK);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:413:13
pushOntoCallStack("Nested", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 413, 13);
try {
PositionalArgsList();
} finally {
popCallStack();
}
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:414:5
pushOntoCallStack("Nested", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 414, 5);
try {
LooseTagEnd();
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, true);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:417:1
final public void MacroDefinition() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "MacroDefinition";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:417:1
MacroDefinition thisProduction = null;
if (buildTree) {
thisProduction = new MacroDefinition();
openNodeScope(thisProduction);
}
Token t;
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == FTL_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:421:6
consumeToken(FTL_DIRECTIVE_OPEN1);
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:421:28
consumeToken(FTL_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("MacroDefinition", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 421, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$421$6, parsingStack);
}
if (nextTokenType() == MACRO) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:423:7
t = consumeToken(MACRO);
} else if (nextTokenType() == FUNCTION) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:425:7
t = consumeToken(FUNCTION);
} else {
pushOntoCallStack("MacroDefinition", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 423, 7);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$423$7, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:427:5
consumeToken(BLANK);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:428:5
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:429:5
if (nextTokenType() == OPEN_PAREN) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:429:6
consumeToken(OPEN_PAREN);
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:430:5
if (nextTokenType() == IDENTIFIER) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:430:6
pushOntoCallStack("MacroDefinition", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 430, 6);
try {
ParameterList();
} finally {
popCallStack();
}
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:431:5
if (nextTokenType() == CLOSE_PAREN) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:431:6
consumeToken(CLOSE_PAREN);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:432:5
consumeToken(CLOSE_TAG);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:433:5
pushOntoCallStack("MacroDefinition", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 433, 5);
try {
Block();
} finally {
popCallStack();
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:434:5
pushOntoCallStack("MacroDefinition", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 434, 5);
try {
DirectiveEnd(t.toString());
} finally {
popCallStack();
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:437:1
final public void ParameterList() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "ParameterList";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:437:1
ParameterList thisProduction = null;
if (buildTree) {
thisProduction = new ParameterList();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:441:5
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:442:5
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:442:6
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:442:7
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:442:15
pushOntoCallStack("ParameterList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 442, 15);
try {
Expression();
} finally {
popCallStack();
}
} else if (nextTokenType() == ELLIPSIS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:442:29
consumeToken(ELLIPSIS);
}
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:443:5
while (true) {
if (!(nextTokenType() == COMMA || nextTokenType == IDENTIFIER)) break;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:444:8
if (nextTokenType() == COMMA) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:444:9
consumeToken(COMMA);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:445:8
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:446:8
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:447:11
if (nextTokenType() == EQUALS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:447:12
consumeToken(EQUALS);
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:447:20
pushOntoCallStack("ParameterList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 447, 20);
try {
Expression();
} finally {
popCallStack();
}
} else if (nextTokenType() == ELLIPSIS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:449:11
consumeToken(ELLIPSIS);
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
private static final EnumSet LooseTagEnd_FIRST_SET = tokenTypeSet(CLOSE_TAG, CLOSE_EMPTY_TAG);
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:455:1
final public void LooseTagEnd() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "LooseTagEnd";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:455:1
LooseTagEnd thisProduction = null;
if (buildTree) {
thisProduction = new LooseTagEnd();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:456:5
if (nextTokenType() == CLOSE_TAG) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:456:5
consumeToken(CLOSE_TAG);
} else if (nextTokenType() == CLOSE_EMPTY_TAG) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:458:5
consumeToken(CLOSE_EMPTY_TAG);
} else {
pushOntoCallStack("LooseTagEnd", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 456, 5);
throw new ParseException(lastConsumedToken, LooseTagEnd_FIRST_SET, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
private static final EnumSet DirectiveEnd_FIRST_SET = tokenTypeSet(END_DIRECTIVE1, END_DIRECTIVE2);
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:461:1
final public void DirectiveEnd(String name) {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "DirectiveEnd";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:461:1
DirectiveEnd thisProduction = null;
if (buildTree) {
thisProduction = new DirectiveEnd();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:462:5
if (nextTokenType() == END_DIRECTIVE1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:462:5
consumeToken(END_DIRECTIVE1);
} else if (nextTokenType() == END_DIRECTIVE2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:462:22
consumeToken(END_DIRECTIVE2);
// Code for CodeBlock specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:463:5
String tag = lastConsumedToken.toString();
tag = tag.substring(3);
tag = tag.substring(0, tag.length() - 1).trim();
if (tag.length() != 0 && !name.equals(tag)) {
throw new ParseException("Expecting closing tag for " + name);
}
} else {
pushOntoCallStack("DirectiveEnd", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 462, 5);
throw new ParseException(lastConsumedToken, DirectiveEnd_FIRST_SET, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:473:1
final public void Text() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Text";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:473:1
Text thisProduction = null;
if (buildTree) {
thisProduction = new Text();
openNodeScope(thisProduction);
}
Token t;
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for OneOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:477:5
while (true) {
if (nextTokenType() == PRINTABLE_CHARS) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:478:8
t = consumeToken(PRINTABLE_CHARS);
} else if (nextTokenType() == WHITESPACE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:479:8
t = consumeToken(WHITESPACE);
} else if (nextTokenType() == SPECIAL_CHAR) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:480:8
t = consumeToken(SPECIAL_CHAR);
} else {
pushOntoCallStack("Text", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 478, 8);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$478$8, parsingStack);
}
if (!(nextTokenType() == WHITESPACE || nextTokenType == SPECIAL_CHAR || nextTokenType == PRINTABLE_CHARS)) break;
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:484:1
final public void TopLevelDirective() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "TopLevelDirective";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:484:1
if (scan$FTL_ccc$486$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:486:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 486, 13);
try {
Assignment();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$487$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:487:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 487, 13);
try {
Comment();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$488$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:488:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 488, 13);
try {
If();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$489$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:489:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 489, 13);
try {
List();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$490$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:490:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 490, 13);
try {
Import();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$491$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:491:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 491, 13);
try {
MacroDefinition();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$492$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:492:6
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 492, 6);
try {
Switch();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$493$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:493:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 493, 13);
try {
Setting();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$494$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:494:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 494, 13);
try {
Var();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$495$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:495:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 495, 13);
try {
Break();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$496$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:496:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 496, 13);
try {
Return();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$497$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:497:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 497, 13);
try {
Nested();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$498$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:498:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 498, 13);
try {
OutputFormatBlock();
} finally {
popCallStack();
}
} else if (scan$FTL_ccc$499$6()) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:499:13
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 499, 13);
try {
Brick();
} finally {
popCallStack();
}
} else {
pushOntoCallStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 486, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$486$6, parsingStack);
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:503:1
final public void UserDirective() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "UserDirective";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:503:1
UserDirective thisProduction = null;
if (buildTree) {
thisProduction = new UserDirective();
openNodeScope(thisProduction);
}
Token startToken;
token_source.noWhitespaceInExpression = true;
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
if (nextTokenType() == USER_DIRECTIVE_OPEN1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:509:9
startToken = consumeToken(USER_DIRECTIVE_OPEN1);
} else if (nextTokenType() == USER_DIRECTIVE_OPEN2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:511:9
startToken = consumeToken(USER_DIRECTIVE_OPEN2);
} else {
pushOntoCallStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 509, 9);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$509$9, parsingStack);
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:513:5
consumeToken(IDENTIFIER);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:513:18
if (nextTokenType() == DOT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:513:20
consumeToken(DOT);
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:513:27
consumeToken(IDENTIFIER);
}
// Code for CodeBlock specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:514:5
token_source.noWhitespaceInExpression = false;
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:515:5
if (nextTokenType() == BLANK) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:515:6
consumeToken(BLANK);
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:516:5
if (first_set$FTL_ccc$516$6.contains(nextTokenType())) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:516:6
pushOntoCallStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 516, 6);
try {
ArgsList();
} finally {
popCallStack();
}
}
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:517:5
if (nextTokenType() == SEMICOLON) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:517:6
consumeToken(SEMICOLON);
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:517:18
if (nextTokenType() == IDENTIFIER) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:517:19
pushOntoCallStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 517, 19);
try {
ParameterList();
} finally {
popCallStack();
}
}
}
if (nextTokenType() == CLOSE_EMPTY_TAG) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:519:8
consumeToken(CLOSE_EMPTY_TAG);
} else if (nextTokenType() == GT || nextTokenType == CLOSE_TAG) {
if (nextTokenType() == CLOSE_TAG) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:522:13
consumeToken(CLOSE_TAG);
} else if (nextTokenType() == GT) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:522:27
consumeToken(GT);
} else {
pushOntoCallStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 522, 13);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$522$13, parsingStack);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:523:11
pushOntoCallStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 523, 11);
try {
Block();
} finally {
popCallStack();
}
if (nextTokenType() == END_USER_DIRECTIVE1) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:524:12
consumeToken(END_USER_DIRECTIVE1);
} else if (nextTokenType() == END_USER_DIRECTIVE2) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:524:34
consumeToken(END_USER_DIRECTIVE2);
} else {
pushOntoCallStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 524, 12);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$524$12, parsingStack);
}
} else {
pushOntoCallStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 519, 8);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$519$8, parsingStack);
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:529:1
final public void Block() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Block";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:529:1
Block thisProduction = null;
if (buildTree) {
thisProduction = new Block();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:530:3
while (true) {
if (!(scan$FTL_ccc$531$5())) break;
if (nextTokenType() == WHITESPACE || nextTokenType == SPECIAL_CHAR || nextTokenType == PRINTABLE_CHARS) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:533:6
pushOntoCallStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 533, 6);
try {
Text();
} finally {
popCallStack();
}
} else if (nextTokenType() == INTERPOLATE) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:535:6
pushOntoCallStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 535, 6);
try {
Interpolation();
} finally {
popCallStack();
}
} else if (nextTokenType() == USER_DIRECTIVE_OPEN1 || nextTokenType == USER_DIRECTIVE_OPEN2) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:537:6
pushOntoCallStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 537, 6);
try {
UserDirective();
} finally {
popCallStack();
}
} else if (nextTokenType() == FTL_DIRECTIVE_OPEN1 || nextTokenType == FTL_DIRECTIVE_OPEN2) {
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:539:6
pushOntoCallStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 539, 6);
try {
TopLevelDirective();
} finally {
popCallStack();
}
} else {
pushOntoCallStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 533, 6);
throw new ParseException(lastConsumedToken, first_set$FTL_ccc$533$6, parsingStack);
}
}
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:544:1
final public void Root() {
if (cancelled) throw new CancellationException();
this.currentlyParsedProduction = "Root";
// Code for BNFProduction specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:544:1
Root thisProduction = null;
if (buildTree) {
thisProduction = new Root();
openNodeScope(thisProduction);
}
ParseException parseException3 = null;
int callStackSize4 = parsingStack.size();
try {
// Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:545:5
if (nextTokenType() == WHITESPACE) {
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:545:6
consumeToken(WHITESPACE);
}
// Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:546:5
pushOntoCallStack("Root", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 546, 5);
try {
Block();
} finally {
popCallStack();
}
// Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:547:5
consumeToken(EOF);
} catch (ParseException e) {
parseException3 = e;
throw e;
} finally {
restoreCallStack(callStackSize4);
if (thisProduction != null) {
if (parseException3 == null) {
closeNodeScope(thisProduction, nodeArity() > 1);
} else {
clearNodeScope();
}
}
}
}
private static final EnumSet first_set$FEL_ccc$196$10 = tokenTypeSet(OR2, OR, XOR);
private static final EnumSet first_set$FEL_ccc$203$10 = tokenTypeSet(AND2, AND);
private static final EnumSet first_set$FEL_ccc$210$10 = tokenTypeSet(EQUALS, DOUBLE_EQUALS, NOT_EQUALS);
private static final EnumSet first_set$FEL_ccc$218$10 = tokenTypeSet(LT, GT, LTE, GTE);
private static final EnumSet first_set$FEL_ccc$226$9 = tokenTypeSet(DOT_DOT, DOT_DOT_EXCLUSIVE, DOT_DOT_LENGTH);
private static final EnumSet first_set$FEL_ccc$236$13 = tokenTypeSet(PLUS, MINUS);
private static final EnumSet first_set$FEL_ccc$244$12 = tokenTypeSet(PERCENT, TIMES, DIVIDE);
private static final EnumSet first_set$FEL_ccc$255$5 = first_set$FEL_ccc$255$5_init();
private static EnumSet first_set$FEL_ccc$255$5_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$259$6 = tokenTypeSet(PLUS, MINUS);
private static final EnumSet first_set$FEL_ccc$263$6 = tokenTypeSet(EXCLAM, NOT);
private static final EnumSet first_set$FEL_ccc$322$6 = tokenTypeSet(TIMES, IDENTIFIER);
private static final EnumSet first_set$FEL_ccc$335$16 = first_set$FEL_ccc$335$16_init();
private static EnumSet first_set$FEL_ccc$335$16_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NOT, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$339$41 = first_set$FEL_ccc$339$41_init();
private static EnumSet first_set$FEL_ccc$339$41_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NOT, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$348$6 = first_set$FEL_ccc$348$6_init();
private static EnumSet first_set$FEL_ccc$348$6_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NOT, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$348$18 = first_set$FEL_ccc$348$18_init();
private static EnumSet first_set$FEL_ccc$348$18_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, COMMA, EXCLAM, NOT, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$360$8 = first_set$FEL_ccc$360$8_init();
private static EnumSet first_set$FEL_ccc$360$8_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NOT, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$382$9 = first_set$FEL_ccc$382$9_init();
private static EnumSet first_set$FEL_ccc$382$9_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NOT, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$385$9 = first_set$FEL_ccc$385$9_init();
private static EnumSet first_set$FEL_ccc$385$9_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NOT, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FEL_ccc$404$8 = first_set$FEL_ccc$404$8_init();
private static EnumSet first_set$FEL_ccc$404$8_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, COMMA, EXCLAM, NOT, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FTL_ccc$243$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$245$8 = tokenTypeSet(SET, LOCAL, GLOBAL, ASSIGN);
private static final EnumSet first_set$FTL_ccc$253$7 = tokenTypeSet(EQUALS, IN, CLOSE_TAG);
private static final EnumSet first_set$FTL_ccc$270$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$278$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$286$5 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$292$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$298$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$305$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$315$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$325$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$334$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$347$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$357$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$365$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$379$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$387$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$393$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$399$19 = tokenTypeSet(ASCENDING, DESCENDING);
private static final EnumSet first_set$FTL_ccc$411$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$421$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$423$7 = tokenTypeSet(MACRO, FUNCTION);
private static final EnumSet first_set$FTL_ccc$478$8 = tokenTypeSet(WHITESPACE, SPECIAL_CHAR, PRINTABLE_CHARS);
private static final EnumSet first_set$FTL_ccc$486$6 = tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$509$9 = tokenTypeSet(USER_DIRECTIVE_OPEN1, USER_DIRECTIVE_OPEN2);
private static final EnumSet first_set$FTL_ccc$516$6 = first_set$FTL_ccc$516$6_init();
private static EnumSet first_set$FTL_ccc$516$6_init() {
return tokenTypeSet(OPEN_PAREN, OPEN_BRACKET, OPEN_BRACE, DOT, PLUS, MINUS, EXCLAM, NOT, NULL, TRUE, FALSE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL);
}
private static final EnumSet first_set$FTL_ccc$519$8 = tokenTypeSet(GT, CLOSE_TAG, CLOSE_EMPTY_TAG);
private static final EnumSet first_set$FTL_ccc$522$13 = tokenTypeSet(GT, CLOSE_TAG);
private static final EnumSet first_set$FTL_ccc$524$12 = tokenTypeSet(END_USER_DIRECTIVE1, END_USER_DIRECTIVE2);
private static final EnumSet first_set$FTL_ccc$533$6 = first_set$FTL_ccc$533$6_init();
private static EnumSet first_set$FTL_ccc$533$6_init() {
return tokenTypeSet(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2, USER_DIRECTIVE_OPEN1, USER_DIRECTIVE_OPEN2, INTERPOLATE, WHITESPACE, SPECIAL_CHAR, PRINTABLE_CHARS);
}
private boolean scanToken(TokenType expectedType, TokenType...additionalTypes) {
Token peekedToken = nextToken(currentLookaheadToken);
TokenType type = peekedToken.getType();
if (type != expectedType) {
boolean matched = false;
for (TokenType tt : additionalTypes) {
if (type == tt) {
matched = true;
break;
}
}
if (!matched) return false;
}
--remainingLookahead;
currentLookaheadToken = peekedToken;
return true;
}
private boolean scanToken(EnumSet types) {
Token peekedToken = nextToken(currentLookaheadToken);
TokenType type = peekedToken.getType();
if (!types.contains(type)) return false;
--remainingLookahead;
currentLookaheadToken = peekedToken;
return true;
}
//====================================
// Lookahead Routines
//====================================
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:196:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$196$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:196:10
if (!scanToken(OR2, OR, XOR)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:196:28
// NonTerminal AndExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:196:28
pushOntoLookaheadStack("OrExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 196, 28);
currentLookaheadProduction = "AndExpression";
try {
if (!check$AndExpression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:203:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$203$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:203:10
if (!scanToken(AND2, AND)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:203:24
// NonTerminal EqualityExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:203:24
pushOntoLookaheadStack("AndExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 203, 24);
currentLookaheadProduction = "EqualityExpression";
try {
if (!check$EqualityExpression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:210:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$210$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:210:10
if (!scanToken(EQUALS, DOUBLE_EQUALS, NOT_EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:211:9
// NonTerminal RelationalExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:211:9
pushOntoLookaheadStack("EqualityExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 211, 9);
currentLookaheadProduction = "RelationalExpression";
try {
if (!check$RelationalExpression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:218:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$218$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:218:10
if (!scanToken(LT, GT, LTE, GTE)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:219:9
// NonTerminal RangeExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:219:9
pushOntoLookaheadStack("RelationalExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 219, 9);
currentLookaheadProduction = "RangeExpression";
try {
if (!check$RangeExpression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:226:8
// BuildScanRoutine macro
private boolean check$FEL_ccc$226$8(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:226:9
if (!scanToken(DOT_DOT, DOT_DOT_EXCLUSIVE, DOT_DOT_LENGTH)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:227:8
Token token5 = currentLookaheadToken;
boolean passedPredicate5 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$228$11(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate5;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:228:11
// BuildScanRoutine macro
private boolean check$FEL_ccc$228$11(boolean scanToEnd) {
int prevPassedPredicateThreshold = this.passedPredicateThreshold;
this.passedPredicateThreshold = -1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:228:11
// NonTerminal AdditiveExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:228:11
pushOntoLookaheadStack("RangeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 228, 11);
currentLookaheadProduction = "AdditiveExpression";
try {
if (!check$AdditiveExpression(true)) return false;
} finally {
popLookaheadStack();
}
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
} finally {
lookaheadRoutineNesting--;
if (remainingLookahead <= this.passedPredicateThreshold) {
passedPredicate = true;
this.passedPredicateThreshold = prevPassedPredicateThreshold;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:236:12
// BuildScanRoutine macro
private boolean check$FEL_ccc$236$12(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:236:13
if (!scanToken(PLUS, MINUS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:237:12
// NonTerminal MultiplicativeExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:237:12
pushOntoLookaheadStack("AdditiveExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 237, 12);
currentLookaheadProduction = "MultiplicativeExpression";
try {
if (!check$MultiplicativeExpression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:244:11
// BuildScanRoutine macro
private boolean check$FEL_ccc$244$11(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:244:12
if (!scanToken(PERCENT, TIMES, DIVIDE)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:245:11
// NonTerminal UnaryExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:245:11
pushOntoLookaheadStack("MultiplicativeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 245, 11);
currentLookaheadProduction = "UnaryExpression";
try {
if (!check$UnaryExpression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:251:5
// BuildScanRoutine macro
private boolean check$FEL_ccc$251$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:251:5
// NonTerminal UnaryPlusMinusExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:251:5
pushOntoLookaheadStack("UnaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 251, 5);
currentLookaheadProduction = "UnaryPlusMinusExpression";
try {
if (!check$UnaryPlusMinusExpression(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:253:5
// BuildScanRoutine macro
private boolean check$FEL_ccc$253$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:253:5
// NonTerminal NotExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:253:5
pushOntoLookaheadStack("UnaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 253, 5);
currentLookaheadProduction = "NotExpression";
try {
if (!check$NotExpression(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:255:5
// BuildScanRoutine macro
private boolean check$FEL_ccc$255$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:255:5
// NonTerminal DefaultToExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:255:5
pushOntoLookaheadStack("UnaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 255, 5);
currentLookaheadProduction = "DefaultToExpression";
try {
if (!check$DefaultToExpression(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:275:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$275$9(boolean scanToEnd) {
int prevPassedPredicateThreshold = this.passedPredicateThreshold;
this.passedPredicateThreshold = -1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:276:12
if (!scanToken(EXCLAM)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:276:20
// NonTerminal PrimaryExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:276:20
pushOntoLookaheadStack("DefaultToExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 276, 20);
currentLookaheadProduction = "PrimaryExpression";
try {
if (!check$PrimaryExpression(true)) return false;
} finally {
popLookaheadStack();
}
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
} finally {
lookaheadRoutineNesting--;
if (remainingLookahead <= this.passedPredicateThreshold) {
passedPredicate = true;
this.passedPredicateThreshold = prevPassedPredicateThreshold;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:286:7
// BuildScanRoutine macro
private boolean check$FEL_ccc$286$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:286:7
Token token2 = currentLookaheadToken;
int remainingLookahead2 = remainingLookahead;
boolean hitFailure2 = hitFailure;
boolean passedPredicate2 = passedPredicate;
try {
passedPredicate = false;
if (!check$FEL_ccc$286$7$(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$288$7(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$290$7(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$292$7(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$294$7(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!scanToken(EXISTS_OPERATOR)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
return false;
}
}
}
}
}
}
} finally {
passedPredicate = passedPredicate2;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:286:7
// BuildScanRoutine macro
private boolean check$FEL_ccc$286$7$(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:286:7
// NonTerminal DotKey at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:286:7
pushOntoLookaheadStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 286, 7);
currentLookaheadProduction = "DotKey";
try {
if (!check$DotKey(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:288:7
// BuildScanRoutine macro
private boolean check$FEL_ccc$288$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:288:7
// NonTerminal MethodReference at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:288:7
pushOntoLookaheadStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 288, 7);
currentLookaheadProduction = "MethodReference";
try {
if (!check$MethodReference(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:290:7
// BuildScanRoutine macro
private boolean check$FEL_ccc$290$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:290:7
// NonTerminal DynamicKey at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:290:7
pushOntoLookaheadStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 290, 7);
currentLookaheadProduction = "DynamicKey";
try {
if (!check$DynamicKey(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:292:7
// BuildScanRoutine macro
private boolean check$FEL_ccc$292$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:292:7
// NonTerminal MethodInvoke at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:292:7
pushOntoLookaheadStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 292, 7);
currentLookaheadProduction = "MethodInvoke";
try {
if (!check$MethodInvoke(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:294:7
// BuildScanRoutine macro
private boolean check$FEL_ccc$294$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:294:7
// NonTerminal BuiltIn at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:294:7
pushOntoLookaheadStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 294, 7);
currentLookaheadProduction = "BuiltIn";
try {
if (!check$BuiltIn(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:305:5
// BuildScanRoutine macro
private boolean check$FEL_ccc$305$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:305:5
// NonTerminal HashLiteral at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:305:5
pushOntoLookaheadStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 305, 5);
currentLookaheadProduction = "HashLiteral";
try {
if (!check$HashLiteral(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:313:5
// BuildScanRoutine macro
private boolean check$FEL_ccc$313$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:313:5
// NonTerminal ListLiteral at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:313:5
pushOntoLookaheadStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 313, 5);
currentLookaheadProduction = "ListLiteral";
try {
if (!check$ListLiteral(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:315:5
// BuildScanRoutine macro
private boolean check$FEL_ccc$315$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:315:5
// NonTerminal Parenthesis at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:315:5
pushOntoLookaheadStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 315, 5);
currentLookaheadProduction = "Parenthesis";
try {
if (!check$Parenthesis(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:317:5
// BuildScanRoutine macro
private boolean check$FEL_ccc$317$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:317:5
// NonTerminal BuiltinVariable at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:317:5
pushOntoLookaheadStack("BaseExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 317, 5);
currentLookaheadProduction = "BuiltinVariable";
try {
if (!check$BuiltinVariable(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:335:16
// BuildScanRoutine macro
private boolean check$FEL_ccc$335$16(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:335:16
// NonTerminal ArgsList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:335:16
pushOntoLookaheadStack("MethodInvoke", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 335, 16);
currentLookaheadProduction = "ArgsList";
try {
if (!check$ArgsList(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:28
// BuildScanRoutine macro
private boolean check$FEL_ccc$339$28(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:28
if (!scanToken(OPEN_PAREN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:40
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$339$41(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:50
if (!scanToken(CLOSE_PAREN)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:41
// BuildScanRoutine macro
private boolean check$FEL_ccc$339$41(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:41
// NonTerminal ArgsList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:41
pushOntoLookaheadStack("BuiltIn", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 339, 41);
currentLookaheadProduction = "ArgsList";
try {
if (!check$ArgsList(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:6
// BuildScanRoutine macro
private boolean check$FEL_ccc$348$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:6
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:6
pushOntoLookaheadStack("ListLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 348, 6);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:17
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$348$18(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:18
// BuildScanRoutine macro
private boolean check$FEL_ccc$348$18(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:18
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(COMMA)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:27
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:27
pushOntoLookaheadStack("ListLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 348, 27);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:8
// BuildScanRoutine macro
private boolean check$FEL_ccc$360$8(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:8
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:8
pushOntoLookaheadStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 360, 8);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:19
if (!scanToken(COLON)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:27
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:360:27
pushOntoLookaheadStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 360, 27);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:361:8
boolean passedPredicate6 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token7 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$361$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token7;
break;
}
}
} finally {
passedPredicate = passedPredicate6;
}
hitFailure = false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:361:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$361$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:361:9
if (!scanToken(COMMA)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:361:17
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:361:17
pushOntoLookaheadStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 361, 17);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:361:28
if (!scanToken(COLON)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:361:36
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:361:36
pushOntoLookaheadStack("HashLiteral", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 361, 36);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:382:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$382$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2147483647;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
if (remainingLookahead <= 0) {
passedPredicate = true;
return !hitFailure;
}
if (!check$FEL_ccc$382$14(true)) return false;
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:383:9
// NonTerminal NamedArgsList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:383:9
pushOntoLookaheadStack("ArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 383, 9);
currentLookaheadProduction = "NamedArgsList";
try {
if (!check$NamedArgsList(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:385:9
// BuildScanRoutine macro
private boolean check$FEL_ccc$385$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:385:9
// NonTerminal PositionalArgsList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:385:9
pushOntoLookaheadStack("ArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 385, 9);
currentLookaheadProduction = "PositionalArgsList";
try {
if (!check$PositionalArgsList(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:394:8
// BuildScanRoutine macro
private boolean check$FEL_ccc$394$8(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:394:8
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(COMMA)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:395:8
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:396:8
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:397:8
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:397:8
pushOntoLookaheadStack("NamedArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 397, 8);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:404:8
// BuildScanRoutine macro
private boolean check$FEL_ccc$404$8(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:404:8
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(COMMA)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:405:8
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:405:8
pushOntoLookaheadStack("PositionalArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 405, 8);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:253:7
// BuildScanRoutine macro
private boolean check$FTL_ccc$253$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:254:8
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:254:16
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:254:16
pushOntoLookaheadStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 254, 16);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:255:8
boolean passedPredicate7 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token8 = currentLookaheadToken;
passedPredicate = false;
if (!check$FTL_ccc$255$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token8;
break;
}
}
} finally {
passedPredicate = passedPredicate7;
}
hitFailure = false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:256:8
Token token10 = currentLookaheadToken;
boolean passedPredicate10 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$256$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token10;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate10;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:257:8
if (!scanToken(CLOSE_TAG, CLOSE_EMPTY_TAG)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:255:9
// BuildScanRoutine macro
private boolean check$FTL_ccc$255$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:255:9
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(COMMA)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:255:19
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:255:32
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:255:41
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:255:41
pushOntoLookaheadStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 255, 41);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:256:9
// BuildScanRoutine macro
private boolean check$FTL_ccc$256$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:256:9
if (!scanToken(IN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:256:14
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:256:14
pushOntoLookaheadStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 256, 14);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:260:7
// BuildScanRoutine macro
private boolean check$FTL_ccc$260$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:261:10
Token token5 = currentLookaheadToken;
boolean passedPredicate5 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$261$11(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate5;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:262:10
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:263:10
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:263:10
pushOntoLookaheadStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 263, 10);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:264:10
if (!scanToken(END_DIRECTIVE1, END_DIRECTIVE2)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:261:11
// BuildScanRoutine macro
private boolean check$FTL_ccc$261$11(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:261:11
if (!scanToken(IN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:261:16
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:261:16
pushOntoLookaheadStack("Assignment", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 261, 16);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:272:19
// BuildScanRoutine macro
private boolean check$FTL_ccc$272$19(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:272:19
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:272:28
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:272:28
pushOntoLookaheadStack("Var", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 272, 28);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$273$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:6
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(COMMA)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:16
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:29
Token token6 = currentLookaheadToken;
boolean passedPredicate6 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$273$30(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token6;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate6;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:30
// BuildScanRoutine macro
private boolean check$FTL_ccc$273$30(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:30
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:39
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:39
pushOntoLookaheadStack("Var", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 273, 39);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:280:19
// BuildScanRoutine macro
private boolean check$FTL_ccc$280$19(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:280:19
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:280:28
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:280:28
pushOntoLookaheadStack("Setting", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 280, 28);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$281$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:6
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(COMMA)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:16
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:29
Token token6 = currentLookaheadToken;
boolean passedPredicate6 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$281$30(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token6;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate6;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:30
// BuildScanRoutine macro
private boolean check$FTL_ccc$281$30(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:30
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:39
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:39
pushOntoLookaheadStack("Setting", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 281, 39);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:300:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$300$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:300:6
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:300:13
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:300:13
pushOntoLookaheadStack("Return", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 300, 13);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:339:7
// BuildScanRoutine macro
private boolean check$FTL_ccc$339$7(boolean scanToEnd) {
int prevPassedPredicateThreshold = this.passedPredicateThreshold;
this.passedPredicateThreshold = -1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:339:7
// NonTerminal ElseIf at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:339:7
pushOntoLookaheadStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 339, 7);
currentLookaheadProduction = "ElseIf";
try {
if (!check$ElseIf(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (remainingLookahead <= this.passedPredicateThreshold) {
passedPredicate = true;
this.passedPredicateThreshold = prevPassedPredicateThreshold;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:341:8
// BuildScanRoutine macro
private boolean check$FTL_ccc$341$8(boolean scanToEnd) {
int prevPassedPredicateThreshold = this.passedPredicateThreshold;
this.passedPredicateThreshold = -1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:341:8
// NonTerminal Else at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:341:8
pushOntoLookaheadStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 341, 8);
currentLookaheadProduction = "Else";
try {
if (!check$Else(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (remainingLookahead <= this.passedPredicateThreshold) {
passedPredicate = true;
this.passedPredicateThreshold = prevPassedPredicateThreshold;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:371:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$371$6(boolean scanToEnd) {
int prevPassedPredicateThreshold = this.passedPredicateThreshold;
this.passedPredicateThreshold = -1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:371:6
// NonTerminal Case at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:371:6
pushOntoLookaheadStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 371, 6);
currentLookaheadProduction = "Case";
try {
if (!check$Case(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (remainingLookahead <= this.passedPredicateThreshold) {
passedPredicate = true;
this.passedPredicateThreshold = prevPassedPredicateThreshold;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:373:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$373$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:373:13
// NonTerminal Default at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:373:13
pushOntoLookaheadStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 373, 13);
currentLookaheadProduction = "Default";
try {
if (!check$Default(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:399:7
// BuildScanRoutine macro
private boolean check$FTL_ccc$399$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:399:7
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$399$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:400:7
if (!scanToken(COMMA)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:400:15
if (!scanToken(IDENTIFIER)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:399:9
// BuildScanRoutine macro
private boolean check$FTL_ccc$399$9(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:399:9
if (!scanToken(SORTED)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:399:19
if (!scanToken(ASCENDING, DESCENDING)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:402:7
// BuildScanRoutine macro
private boolean check$FTL_ccc$402$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:402:7
if (!scanToken(WITH)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:402:14
if (!scanToken(IDENTIFIER)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:403:7
// BuildScanRoutine macro
private boolean check$FTL_ccc$403$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:403:7
if (!scanToken(FILTER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:403:16
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:403:16
pushOntoLookaheadStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 403, 16);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:404:7
// BuildScanRoutine macro
private boolean check$FTL_ccc$404$7(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:404:7
if (!scanToken(LIMIT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:404:15
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:404:15
pushOntoLookaheadStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 404, 15);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:413:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$413$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:413:6
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:413:13
// NonTerminal PositionalArgsList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:413:13
pushOntoLookaheadStack("Nested", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 413, 13);
currentLookaheadProduction = "PositionalArgsList";
try {
if (!check$PositionalArgsList(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:430:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$430$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:430:6
// NonTerminal ParameterList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:430:6
pushOntoLookaheadStack("MacroDefinition", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 430, 6);
currentLookaheadProduction = "ParameterList";
try {
if (!check$ParameterList(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:442:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$442$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:442:6
Token token2 = currentLookaheadToken;
int remainingLookahead2 = remainingLookahead;
boolean hitFailure2 = hitFailure;
boolean passedPredicate2 = passedPredicate;
try {
passedPredicate = false;
if (!check$FTL_ccc$442$6$(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!scanToken(ELLIPSIS)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
return false;
}
}
} finally {
passedPredicate = passedPredicate2;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:442:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$442$6$(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:442:7
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:442:15
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:442:15
pushOntoLookaheadStack("ParameterList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 442, 15);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:444:8
// BuildScanRoutine macro
private boolean check$FTL_ccc$444$8(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:444:8
Token token3 = currentLookaheadToken;
boolean passedPredicate3 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(COMMA)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token3;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate3;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:445:8
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:446:8
Token token6 = currentLookaheadToken;
boolean passedPredicate6 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$447$11(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token6;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate6;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:447:11
// BuildScanRoutine macro
private boolean check$FTL_ccc$447$11(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:447:11
Token token2 = currentLookaheadToken;
int remainingLookahead2 = remainingLookahead;
boolean hitFailure2 = hitFailure;
boolean passedPredicate2 = passedPredicate;
try {
passedPredicate = false;
if (!check$FTL_ccc$447$11$(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!scanToken(ELLIPSIS)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
return false;
}
}
} finally {
passedPredicate = passedPredicate2;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:447:11
// BuildScanRoutine macro
private boolean check$FTL_ccc$447$11$(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:447:12
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:447:20
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:447:20
pushOntoLookaheadStack("ParameterList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 447, 20);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:486:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$486$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:486:13
// NonTerminal Assignment at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:486:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 486, 13);
currentLookaheadProduction = "Assignment";
try {
if (!check$Assignment(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:487:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$487$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:487:13
// NonTerminal Comment at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:487:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 487, 13);
currentLookaheadProduction = "Comment";
try {
if (!check$Comment(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:488:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$488$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:488:13
// NonTerminal If at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:488:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 488, 13);
currentLookaheadProduction = "If";
try {
if (!check$If(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:489:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$489$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:489:13
// NonTerminal List at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:489:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 489, 13);
currentLookaheadProduction = "List";
try {
if (!check$List(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:490:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$490$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:490:13
// NonTerminal Import at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:490:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 490, 13);
currentLookaheadProduction = "Import";
try {
if (!check$Import(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:491:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$491$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:491:13
// NonTerminal MacroDefinition at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:491:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 491, 13);
currentLookaheadProduction = "MacroDefinition";
try {
if (!check$MacroDefinition(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:492:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$492$6(boolean scanToEnd) {
int prevPassedPredicateThreshold = this.passedPredicateThreshold;
this.passedPredicateThreshold = -1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:492:6
// NonTerminal Switch at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:492:6
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 492, 6);
currentLookaheadProduction = "Switch";
try {
if (!check$Switch(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (remainingLookahead <= this.passedPredicateThreshold) {
passedPredicate = true;
this.passedPredicateThreshold = prevPassedPredicateThreshold;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:493:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$493$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:493:13
// NonTerminal Setting at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:493:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 493, 13);
currentLookaheadProduction = "Setting";
try {
if (!check$Setting(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:494:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$494$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:494:13
// NonTerminal Var at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:494:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 494, 13);
currentLookaheadProduction = "Var";
try {
if (!check$Var(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:495:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$495$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:495:13
// NonTerminal Break at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:495:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 495, 13);
currentLookaheadProduction = "Break";
try {
if (!check$Break(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:496:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$496$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:496:13
// NonTerminal Return at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:496:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 496, 13);
currentLookaheadProduction = "Return";
try {
if (!check$Return(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:497:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$497$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:497:13
// NonTerminal Nested at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:497:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 497, 13);
currentLookaheadProduction = "Nested";
try {
if (!check$Nested(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:498:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$498$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:498:13
// NonTerminal OutputFormatBlock at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:498:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 498, 13);
currentLookaheadProduction = "OutputFormatBlock";
try {
if (!check$OutputFormatBlock(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:499:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$499$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:499:13
// NonTerminal Brick at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:499:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 499, 13);
currentLookaheadProduction = "Brick";
try {
if (!check$Brick(true)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:513:20
// BuildScanRoutine macro
private boolean check$FTL_ccc$513$20(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:513:20
if (!scanToken(DOT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:513:27
if (!scanToken(IDENTIFIER)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:516:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$516$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:516:6
// NonTerminal ArgsList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:516:6
pushOntoLookaheadStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 516, 6);
currentLookaheadProduction = "ArgsList";
try {
if (!check$ArgsList(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:517:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$517$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:517:6
if (!scanToken(SEMICOLON)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:517:18
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$517$19(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:517:19
// BuildScanRoutine macro
private boolean check$FTL_ccc$517$19(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:517:19
// NonTerminal ParameterList at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:517:19
pushOntoLookaheadStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 517, 19);
currentLookaheadProduction = "ParameterList";
try {
if (!check$ParameterList(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:521:8
// BuildScanRoutine macro
private boolean check$FTL_ccc$521$8(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:522:13
if (!scanToken(GT, CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:523:11
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:523:11
pushOntoLookaheadStack("UserDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 523, 11);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:524:12
if (!scanToken(END_USER_DIRECTIVE1, END_USER_DIRECTIVE2)) return false;
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:531:5
// BuildScanRoutine macro
private boolean check$FTL_ccc$531$5(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 2;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:533:6
Token token4 = currentLookaheadToken;
int remainingLookahead4 = remainingLookahead;
boolean hitFailure4 = hitFailure;
boolean passedPredicate4 = passedPredicate;
try {
passedPredicate = false;
if (!check$FTL_ccc$533$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$535$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$537$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$539$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
return false;
}
}
}
}
} finally {
passedPredicate = passedPredicate4;
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:533:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$533$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:533:6
// NonTerminal Text at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:533:6
pushOntoLookaheadStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 533, 6);
currentLookaheadProduction = "Text";
try {
if (!check$Text(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:535:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$535$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:535:6
// NonTerminal Interpolation at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:535:6
pushOntoLookaheadStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 535, 6);
currentLookaheadProduction = "Interpolation";
try {
if (!check$Interpolation(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:537:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$537$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:537:6
// NonTerminal UserDirective at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:537:6
pushOntoLookaheadStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 537, 6);
currentLookaheadProduction = "UserDirective";
try {
if (!check$UserDirective(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// scanahead routine for expansion at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:539:6
// BuildScanRoutine macro
private boolean check$FTL_ccc$539$6(boolean scanToEnd) {
boolean reachedScanCode = false;
int passedPredicateThreshold = remainingLookahead - 1;
try {
lookaheadRoutineNesting++;
// BuildPredicateCode macro
// End BuildPredicateCode macro
reachedScanCode = true;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:539:6
// NonTerminal TopLevelDirective at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:539:6
pushOntoLookaheadStack("Block", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 539, 6);
currentLookaheadProduction = "TopLevelDirective";
try {
if (!check$TopLevelDirective(false)) return false;
} finally {
popLookaheadStack();
}
} finally {
lookaheadRoutineNesting--;
if (reachedScanCode && remainingLookahead <= passedPredicateThreshold) {
passedPredicate = true;
}
}
passedPredicate = false;
return true;
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:228:11
private boolean scan$FEL_ccc$228$11() {
remainingLookahead = UNLIMITED;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:228:11
// NonTerminal AdditiveExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:228:11
pushOntoLookaheadStack("RangeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 228, 11);
currentLookaheadProduction = "AdditiveExpression";
try {
if (!check$AdditiveExpression(true)) return false;
} finally {
popLookaheadStack();
}
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:275:9
private boolean scan$FEL_ccc$275$9() {
remainingLookahead = UNLIMITED;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:276:12
if (!scanToken(EXCLAM)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:276:20
// NonTerminal PrimaryExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:276:20
pushOntoLookaheadStack("DefaultToExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 276, 20);
currentLookaheadProduction = "PrimaryExpression";
try {
if (!check$PrimaryExpression(true)) return false;
} finally {
popLookaheadStack();
}
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:382:9
private boolean scan$FEL_ccc$382$9() {
remainingLookahead = UNLIMITED;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
if (remainingLookahead <= 0) {
passedPredicate = true;
return !hitFailure;
}
if (!check$FEL_ccc$382$14(true)) return false;
// End BuildPredicateCode macro
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:339:7
private boolean scan$FTL_ccc$339$7() {
remainingLookahead = UNLIMITED;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:339:7
// NonTerminal ElseIf at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:339:7
pushOntoLookaheadStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 339, 7);
currentLookaheadProduction = "ElseIf";
try {
if (!check$ElseIf(false)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:341:8
private boolean scan$FTL_ccc$341$8() {
remainingLookahead = UNLIMITED;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:341:8
// NonTerminal Else at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:341:8
pushOntoLookaheadStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 341, 8);
currentLookaheadProduction = "Else";
try {
if (!check$Else(false)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:371:6
private boolean scan$FTL_ccc$371$6() {
remainingLookahead = UNLIMITED;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:371:6
// NonTerminal Case at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:371:6
pushOntoLookaheadStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 371, 6);
currentLookaheadProduction = "Case";
try {
if (!check$Case(false)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:373:6
private boolean scan$FTL_ccc$373$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:373:13
// NonTerminal Default at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:373:13
pushOntoLookaheadStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 373, 13);
currentLookaheadProduction = "Default";
try {
if (!check$Default(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:486:6
private boolean scan$FTL_ccc$486$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:486:13
// NonTerminal Assignment at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:486:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 486, 13);
currentLookaheadProduction = "Assignment";
try {
if (!check$Assignment(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:487:6
private boolean scan$FTL_ccc$487$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:487:13
// NonTerminal Comment at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:487:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 487, 13);
currentLookaheadProduction = "Comment";
try {
if (!check$Comment(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:488:6
private boolean scan$FTL_ccc$488$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:488:13
// NonTerminal If at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:488:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 488, 13);
currentLookaheadProduction = "If";
try {
if (!check$If(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:489:6
private boolean scan$FTL_ccc$489$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:489:13
// NonTerminal List at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:489:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 489, 13);
currentLookaheadProduction = "List";
try {
if (!check$List(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:490:6
private boolean scan$FTL_ccc$490$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:490:13
// NonTerminal Import at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:490:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 490, 13);
currentLookaheadProduction = "Import";
try {
if (!check$Import(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:491:6
private boolean scan$FTL_ccc$491$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:491:13
// NonTerminal MacroDefinition at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:491:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 491, 13);
currentLookaheadProduction = "MacroDefinition";
try {
if (!check$MacroDefinition(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:492:6
private boolean scan$FTL_ccc$492$6() {
remainingLookahead = UNLIMITED;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:492:6
// NonTerminal Switch at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:492:6
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 492, 6);
currentLookaheadProduction = "Switch";
try {
if (!check$Switch(false)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:493:6
private boolean scan$FTL_ccc$493$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:493:13
// NonTerminal Setting at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:493:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 493, 13);
currentLookaheadProduction = "Setting";
try {
if (!check$Setting(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:494:6
private boolean scan$FTL_ccc$494$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:494:13
// NonTerminal Var at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:494:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 494, 13);
currentLookaheadProduction = "Var";
try {
if (!check$Var(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:495:6
private boolean scan$FTL_ccc$495$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:495:13
// NonTerminal Break at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:495:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 495, 13);
currentLookaheadProduction = "Break";
try {
if (!check$Break(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:496:6
private boolean scan$FTL_ccc$496$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:496:13
// NonTerminal Return at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:496:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 496, 13);
currentLookaheadProduction = "Return";
try {
if (!check$Return(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:497:6
private boolean scan$FTL_ccc$497$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:497:13
// NonTerminal Nested at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:497:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 497, 13);
currentLookaheadProduction = "Nested";
try {
if (!check$Nested(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:498:6
private boolean scan$FTL_ccc$498$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:498:13
// NonTerminal OutputFormatBlock at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:498:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 498, 13);
currentLookaheadProduction = "OutputFormatBlock";
try {
if (!check$OutputFormatBlock(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:499:6
private boolean scan$FTL_ccc$499$6() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:499:13
// NonTerminal Brick at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:499:13
pushOntoLookaheadStack("TopLevelDirective", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 499, 13);
currentLookaheadProduction = "Brick";
try {
if (!check$Brick(true)) return false;
} finally {
popLookaheadStack();
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// BuildPredicateRoutine: expansion at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:531:5
private boolean scan$FTL_ccc$531$5() {
remainingLookahead = 2;
currentLookaheadToken = lastConsumedToken;
final boolean scanToEnd = false;
try {
// BuildPredicateCode macro
// End BuildPredicateCode macro
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:533:6
Token token4 = currentLookaheadToken;
int remainingLookahead4 = remainingLookahead;
boolean hitFailure4 = hitFailure;
boolean passedPredicate4 = passedPredicate;
try {
passedPredicate = false;
if (!check$FTL_ccc$533$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$535$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$537$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$539$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
return false;
}
}
}
}
} finally {
passedPredicate = passedPredicate4;
}
return true;
} finally {
lookaheadRoutineNesting = 0;
currentLookaheadToken = null;
hitFailure = false;
}
}
// lookahead routine for lookahead at:
// C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:382:9
private boolean check$FEL_ccc$382$14(boolean scanToEnd) {
int prevRemainingLookahead = remainingLookahead;
boolean prevHitFailure = hitFailure;
Token prevScanAheadToken = currentLookaheadToken;
try {
lookaheadRoutineNesting++;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:382:14
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:382:26
if (!scanToken(EQUALS)) return false;
return !hitFailure;
} finally {
lookaheadRoutineNesting--;
currentLookaheadToken = prevScanAheadToken;
remainingLookahead = prevRemainingLookahead;
hitFailure = prevHitFailure;
}
}
// BuildProductionLookaheadMethod macro
private boolean check$Expression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:190:5
// NonTerminal OrExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:190:5
pushOntoLookaheadStack("Expression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 190, 5);
currentLookaheadProduction = "OrExpression";
try {
if (!check$OrExpression(false)) return false;
} finally {
popLookaheadStack();
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$OrExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:194:5
// NonTerminal AndExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:194:5
pushOntoLookaheadStack("OrExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 194, 5);
currentLookaheadProduction = "AndExpression";
try {
if (!check$AndExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:195:5
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$196$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$AndExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:201:5
// NonTerminal EqualityExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:201:5
pushOntoLookaheadStack("AndExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 201, 5);
currentLookaheadProduction = "EqualityExpression";
try {
if (!check$EqualityExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:202:5
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$203$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$EqualityExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:208:5
// NonTerminal RelationalExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:208:5
pushOntoLookaheadStack("EqualityExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 208, 5);
currentLookaheadProduction = "RelationalExpression";
try {
if (!check$RelationalExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:209:5
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$210$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$RelationalExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:216:5
// NonTerminal RangeExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:216:5
pushOntoLookaheadStack("RelationalExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 216, 5);
currentLookaheadProduction = "RangeExpression";
try {
if (!check$RangeExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:217:5
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$218$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$RangeExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:224:5
// NonTerminal AdditiveExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:224:5
pushOntoLookaheadStack("RangeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 224, 5);
currentLookaheadProduction = "AdditiveExpression";
try {
if (!check$AdditiveExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:225:5
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$226$8(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$AdditiveExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:234:5
// NonTerminal MultiplicativeExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:234:5
pushOntoLookaheadStack("AdditiveExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 234, 5);
currentLookaheadProduction = "MultiplicativeExpression";
try {
if (!check$MultiplicativeExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:235:5
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$236$12(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$MultiplicativeExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:242:5
// NonTerminal UnaryExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:242:5
pushOntoLookaheadStack("MultiplicativeExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 242, 5);
currentLookaheadProduction = "UnaryExpression";
try {
if (!check$UnaryExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:243:5
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$244$11(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$UnaryExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:251:5
Token token2 = currentLookaheadToken;
int remainingLookahead2 = remainingLookahead;
boolean hitFailure2 = hitFailure;
boolean passedPredicate2 = passedPredicate;
try {
passedPredicate = false;
if (!check$FEL_ccc$251$5(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$253$5(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$255$5(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
return false;
}
}
}
} finally {
passedPredicate = passedPredicate2;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$UnaryPlusMinusExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:259:6
if (!scanToken(PLUS, MINUS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:259:22
// NonTerminal DefaultToExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:259:22
pushOntoLookaheadStack("UnaryPlusMinusExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 259, 22);
currentLookaheadProduction = "DefaultToExpression";
try {
if (!check$DefaultToExpression(true)) return false;
} finally {
popLookaheadStack();
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$NotExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:263:6
if (!scanToken(EXCLAM, NOT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:264:5
// NonTerminal DefaultToExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:264:5
pushOntoLookaheadStack("NotExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 264, 5);
currentLookaheadProduction = "DefaultToExpression";
try {
if (!check$DefaultToExpression(true)) return false;
} finally {
popLookaheadStack();
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$BuiltinVariable(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:268:5
if (!scanToken(DOT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:268:10
if (!scanToken(IDENTIFIER)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$DefaultToExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:272:5
// NonTerminal PrimaryExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:272:5
pushOntoLookaheadStack("DefaultToExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 272, 5);
currentLookaheadProduction = "PrimaryExpression";
try {
if (!check$PrimaryExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:273:5
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$275$9(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:278:5
Token token7 = currentLookaheadToken;
boolean passedPredicate7 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(EXCLAM)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token7;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate7;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$PrimaryExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:284:3
// NonTerminal BaseExpression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:284:3
pushOntoLookaheadStack("PrimaryExpression", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 284, 3);
currentLookaheadProduction = "BaseExpression";
try {
if (!check$BaseExpression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:285:3
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$286$7(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$BaseExpression(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:301:5
Token token2 = currentLookaheadToken;
int remainingLookahead2 = remainingLookahead;
boolean hitFailure2 = hitFailure;
boolean passedPredicate2 = passedPredicate;
try {
passedPredicate = false;
if (!scanToken(IDENTIFIER)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!scanToken(INTEGER, DECIMAL)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$305$5(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!scanToken(STRING_LITERAL)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!scanToken(TRUE, FALSE)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!scanToken(NULL)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$313$5(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$315$5(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$317$5(false)) {
currentLookaheadToken = token2;
remainingLookahead = remainingLookahead2;
hitFailure = hitFailure2;
return false;
}
}
}
}
}
}
}
}
}
} finally {
passedPredicate = passedPredicate2;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$DotKey(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:321:5
if (!scanToken(DOT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:322:6
if (!scanToken(TIMES, IDENTIFIER)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$MethodReference(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:326:5
if (!scanToken(DOUBLE_COLON)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:327:5
if (!scanToken(IDENTIFIER)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$DynamicKey(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:331:5
if (!scanToken(OPEN_BRACKET)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:331:19
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:331:19
pushOntoLookaheadStack("DynamicKey", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 331, 19);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:331:29
if (!scanToken(CLOSE_BRACKET)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$MethodInvoke(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:335:3
if (!scanToken(OPEN_PAREN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:335:15
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$335$16(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:335:25
if (!scanToken(CLOSE_PAREN)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$BuiltIn(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:5
if (!scanToken(BUILT_IN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:15
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:339:27
Token token5 = currentLookaheadToken;
boolean passedPredicate5 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$339$28(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate5;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$ListLiteral(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:347:5
if (!scanToken(OPEN_BRACKET)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:348:5
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$348$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:349:5
if (!scanToken(CLOSE_BRACKET)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$HashLiteral(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:358:5
if (!scanToken(OPEN_BRACE)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:359:5
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FEL_ccc$360$8(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:363:5
if (!scanToken(CLOSE_BRACE)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Parenthesis(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:377:5
if (!scanToken(OPEN_PAREN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:377:18
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:377:18
pushOntoLookaheadStack("Parenthesis", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 377, 18);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:377:29
if (!scanToken(CLOSE_PAREN)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$ArgsList(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:382:9
Token token4 = currentLookaheadToken;
int remainingLookahead4 = remainingLookahead;
boolean hitFailure4 = hitFailure;
boolean passedPredicate4 = passedPredicate;
try {
passedPredicate = false;
if (!check$FEL_ccc$382$9(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FEL_ccc$385$9(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
return false;
}
}
} finally {
passedPredicate = passedPredicate4;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$NamedArgsList(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:390:5
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:391:5
if (!scanToken(EQUALS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:392:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:392:5
pushOntoLookaheadStack("NamedArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 392, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:393:5
boolean passedPredicate6 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token7 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$394$8(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token7;
break;
}
}
} finally {
passedPredicate = passedPredicate6;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$PositionalArgsList(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:402:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:402:5
pushOntoLookaheadStack("PositionalArgsList", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FEL.ccc", 402, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(false)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FEL.ccc:403:5
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!check$FEL_ccc$404$8(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Interpolation(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:236:5
if (!scanToken(INTERPOLATE)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:236:18
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:236:18
pushOntoLookaheadStack("Interpolation", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 236, 18);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:236:28
if (!scanToken(CLOSE_BRACE)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Assignment(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:243:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:245:8
if (!scanToken(SET, LOCAL, GLOBAL, ASSIGN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:250:5
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:251:5
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:253:7
Token token10 = currentLookaheadToken;
int remainingLookahead10 = remainingLookahead;
boolean hitFailure10 = hitFailure;
boolean passedPredicate10 = passedPredicate;
try {
passedPredicate = false;
if (!check$FTL_ccc$253$7(false)) {
currentLookaheadToken = token10;
remainingLookahead = remainingLookahead10;
hitFailure = hitFailure10;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$260$7(false)) {
currentLookaheadToken = token10;
remainingLookahead = remainingLookahead10;
hitFailure = hitFailure10;
return false;
}
}
} finally {
passedPredicate = passedPredicate10;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Var(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:270:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:271:5
if (!scanToken(VAR)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:271:10
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:272:5
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:272:18
Token token8 = currentLookaheadToken;
boolean passedPredicate8 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$272$19(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token8;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate8;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:273:5
boolean passedPredicate10 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token11 = currentLookaheadToken;
passedPredicate = false;
if (!check$FTL_ccc$273$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token11;
break;
}
}
} finally {
passedPredicate = passedPredicate10;
}
hitFailure = false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:274:5
if (!scanToken(CLOSE_TAG, CLOSE_EMPTY_TAG)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Setting(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:278:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:279:5
if (!scanToken(SETTING)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:279:14
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:280:5
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:280:18
Token token8 = currentLookaheadToken;
boolean passedPredicate8 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$280$19(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token8;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate8;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:281:5
boolean passedPredicate10 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token11 = currentLookaheadToken;
passedPredicate = false;
if (!check$FTL_ccc$281$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token11;
break;
}
}
} finally {
passedPredicate = passedPredicate10;
}
hitFailure = false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:282:5
if (!scanToken(CLOSE_TAG, CLOSE_EMPTY_TAG)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Comment(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:286:5
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:287:4
if (!scanToken(COMMENT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:288:4
if (!scanToken(END_COMMENT)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Break(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:292:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:293:5
if (!scanToken(BREAK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:294:5
if (!scanToken(CLOSE_TAG, CLOSE_EMPTY_TAG)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Return(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:298:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:299:5
if (!scanToken(RETURN)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:300:5
Token token6 = currentLookaheadToken;
boolean passedPredicate6 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$300$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token6;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate6;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:301:5
if (!scanToken(CLOSE_TAG, CLOSE_EMPTY_TAG)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$OutputFormatBlock(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:305:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:306:5
if (!scanToken(OUTPUT_FORMAT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:307:5
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:308:5
if (!scanToken(STRING_LITERAL)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:309:5
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:310:5
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:310:5
pushOntoLookaheadStack("OutputFormatBlock", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 310, 5);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:311:5
if (!scanToken(END_DIRECTIVE1, END_DIRECTIVE2)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Brick(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:315:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:316:5
if (!scanToken(BRICK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:317:5
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:318:5
if (!scanToken(STRING_LITERAL)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:319:5
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:320:5
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:320:5
pushOntoLookaheadStack("Brick", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 320, 5);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:321:5
if (!scanToken(END_DIRECTIVE1, END_DIRECTIVE2)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Import(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:325:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:326:5
if (!scanToken(IMPORT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:326:13
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:327:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:327:5
pushOntoLookaheadStack("Import", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 327, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:328:5
if (!scanToken(AS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:329:5
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:330:5
if (!scanToken(CLOSE_TAG, CLOSE_EMPTY_TAG)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$If(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:334:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:335:5
if (!scanToken(IF)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:335:9
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:336:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:336:5
pushOntoLookaheadStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 336, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:337:5
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:338:5
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:338:5
pushOntoLookaheadStack("If", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 338, 5);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:339:5
boolean passedPredicate10 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token11 = currentLookaheadToken;
passedPredicate = false;
if (!check$FTL_ccc$339$7(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token11;
break;
}
}
} finally {
passedPredicate = passedPredicate10;
}
hitFailure = false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:340:5
Token token13 = currentLookaheadToken;
boolean passedPredicate13 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$341$8(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token13;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate13;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:343:5
if (!scanToken(END_DIRECTIVE1, END_DIRECTIVE2)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$ElseIf(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:347:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:348:5
if (!scanToken(ELSEIF)) return false;
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:350:5
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:351:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:351:5
pushOntoLookaheadStack("ElseIf", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 351, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:352:5
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:353:5
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:353:5
pushOntoLookaheadStack("ElseIf", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 353, 5);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Else(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:357:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:358:5
if (!scanToken(ELSE)) return false;
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:360:5
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:361:5
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:361:5
pushOntoLookaheadStack("Else", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 361, 5);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Switch(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:365:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:366:5
if (!scanToken(SWITCH)) return false;
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:367:5
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:368:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:368:5
pushOntoLookaheadStack("Switch", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 368, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:369:5
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:370:5
Token token9 = currentLookaheadToken;
boolean passedPredicate9 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(WHITESPACE)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token9;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate9;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:371:5
boolean passedPredicate11 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token12 = currentLookaheadToken;
passedPredicate = false;
if (!check$FTL_ccc$371$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token12;
break;
}
}
} finally {
passedPredicate = passedPredicate11;
}
hitFailure = false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:372:5
Token token14 = currentLookaheadToken;
boolean passedPredicate14 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$373$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token14;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate14;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:375:5
if (!scanToken(END_DIRECTIVE1, END_DIRECTIVE2)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Case(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:379:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:380:5
if (!scanToken(CASE)) return false;
if (!scanToEnd && lookaheadStack.size() <= 1) {
if (lookaheadRoutineNesting == 0) {
remainingLookahead = 0;
} else if (lookaheadStack.size() == 1) {
passedPredicateThreshold = remainingLookahead;
}
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:382:5
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:383:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:383:5
pushOntoLookaheadStack("Case", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 383, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:383:16
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:383:28
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:383:28
pushOntoLookaheadStack("Case", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 383, 28);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Default(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:387:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:388:5
if (!scanToken(DEFAULT)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:388:14
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:389:5
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:389:5
pushOntoLookaheadStack("Default", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 389, 5);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$List(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:393:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:394:5
if (!scanToken(LIST)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:394:11
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:395:5
// NonTerminal Expression at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:395:5
pushOntoLookaheadStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 395, 5);
currentLookaheadProduction = "Expression";
try {
if (!check$Expression(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:396:5
if (!scanToken(AS)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:397:5
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:398:5
Token token10 = currentLookaheadToken;
boolean passedPredicate10 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$399$7(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token10;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate10;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:402:5
Token token12 = currentLookaheadToken;
boolean passedPredicate12 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$402$7(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token12;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate12;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:403:5
Token token14 = currentLookaheadToken;
boolean passedPredicate14 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$403$7(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token14;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate14;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:404:5
Token token16 = currentLookaheadToken;
boolean passedPredicate16 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$404$7(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token16;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate16;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:405:5
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:406:5
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:406:5
pushOntoLookaheadStack("List", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 406, 5);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:407:5
if (!scanToken(END_DIRECTIVE1, END_DIRECTIVE2)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Nested(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:411:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:412:5
if (!scanToken(NESTED)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:413:5
Token token6 = currentLookaheadToken;
boolean passedPredicate6 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$413$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token6;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate6;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:414:5
if (!scanToken(CLOSE_TAG, CLOSE_EMPTY_TAG)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$MacroDefinition(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:421:6
if (!scanToken(FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:423:7
if (!scanToken(MACRO, FUNCTION)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:427:5
if (!scanToken(BLANK)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:428:5
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:429:5
Token token9 = currentLookaheadToken;
boolean passedPredicate9 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(OPEN_PAREN)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token9;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate9;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:430:5
Token token11 = currentLookaheadToken;
boolean passedPredicate11 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$430$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token11;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate11;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:431:5
Token token13 = currentLookaheadToken;
boolean passedPredicate13 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(CLOSE_PAREN)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token13;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate13;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:432:5
if (!scanToken(CLOSE_TAG)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:433:5
// NonTerminal Block at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:433:5
pushOntoLookaheadStack("MacroDefinition", "C:\\Users\\r.kaiser\\IdeaProjects\\freshmarker\\src\\main\\ccc\\FreshMarker\\FTL.ccc", 433, 5);
currentLookaheadProduction = "Block";
try {
if (!check$Block(true)) return false;
} finally {
popLookaheadStack();
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for NonTerminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:434:5
if (!scanToken(END_DIRECTIVE1, END_DIRECTIVE2)) return false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$ParameterList(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:441:5
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:442:5
Token token4 = currentLookaheadToken;
boolean passedPredicate4 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$442$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate4;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:443:5
boolean passedPredicate6 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token7 = currentLookaheadToken;
passedPredicate = false;
if (!check$FTL_ccc$444$8(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token7;
break;
}
}
} finally {
passedPredicate = passedPredicate6;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Text(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for OneOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:477:5
if (!scanToken(WHITESPACE, SPECIAL_CHAR, PRINTABLE_CHARS)) return false;
boolean passedPredicate4 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token5 = currentLookaheadToken;
passedPredicate = false;
if (!scanToken(WHITESPACE, SPECIAL_CHAR, PRINTABLE_CHARS)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token5;
break;
}
}
} finally {
passedPredicate = passedPredicate4;
}
hitFailure = false;
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$TopLevelDirective(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:486:6
Token token4 = currentLookaheadToken;
int remainingLookahead4 = remainingLookahead;
boolean hitFailure4 = hitFailure;
boolean passedPredicate4 = passedPredicate;
try {
passedPredicate = false;
if (!check$FTL_ccc$486$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$487$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$488$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$489$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$490$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$491$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$492$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$493$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$494$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$495$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$496$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$497$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$498$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$499$6(false)) {
currentLookaheadToken = token4;
remainingLookahead = remainingLookahead4;
hitFailure = hitFailure4;
return false;
}
}
}
}
}
}
}
}
}
}
}
}
}
}
} finally {
passedPredicate = passedPredicate4;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$UserDirective(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:509:9
if (!scanToken(USER_DIRECTIVE_OPEN1, USER_DIRECTIVE_OPEN2)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for Terminal specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:513:5
if (!scanToken(IDENTIFIER)) return false;
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:513:18
Token token6 = currentLookaheadToken;
boolean passedPredicate6 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$513$20(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token6;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate6;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for CodeBlock specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:514:5
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:515:5
Token token9 = currentLookaheadToken;
boolean passedPredicate9 = passedPredicate;
passedPredicate = false;
try {
if (!scanToken(BLANK)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token9;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate9;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:516:5
Token token11 = currentLookaheadToken;
boolean passedPredicate11 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$516$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token11;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate11;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrOne specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:517:5
Token token13 = currentLookaheadToken;
boolean passedPredicate13 = passedPredicate;
passedPredicate = false;
try {
if (!check$FTL_ccc$517$6(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token13;
hitFailure = false;
}
} finally {
passedPredicate = passedPredicate13;
}
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ExpansionChoice specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:519:8
Token token16 = currentLookaheadToken;
int remainingLookahead16 = remainingLookahead;
boolean hitFailure16 = hitFailure;
boolean passedPredicate16 = passedPredicate;
try {
passedPredicate = false;
if (!scanToken(CLOSE_EMPTY_TAG)) {
currentLookaheadToken = token16;
remainingLookahead = remainingLookahead16;
hitFailure = hitFailure16;
if (passedPredicate && !legacyGlitchyLookahead) return false;
passedPredicate = false;
if (!check$FTL_ccc$521$8(false)) {
currentLookaheadToken = token16;
remainingLookahead = remainingLookahead16;
hitFailure = hitFailure16;
return false;
}
}
} finally {
passedPredicate = passedPredicate16;
}
return true;
}
// BuildProductionLookaheadMethod macro
private boolean check$Block(boolean scanToEnd) {
if (hitFailure) return false;
if (remainingLookahead <= 0) {
return true;
}
// Lookahead Code for ZeroOrMore specified at C:\Users\r.kaiser\IdeaProjects\freshmarker\src\main\ccc\FreshMarker\FTL.ccc:530:3
boolean passedPredicate3 = passedPredicate;
try {
while (remainingLookahead > 0 && !hitFailure) {
Token token4 = currentLookaheadToken;
passedPredicate = false;
if (!check$FTL_ccc$531$5(false)) {
if (passedPredicate && !legacyGlitchyLookahead) return false;
currentLookaheadToken = token4;
break;
}
}
} finally {
passedPredicate = passedPredicate3;
}
hitFailure = false;
return true;
}
private ArrayList parsingStack = new ArrayList<>();
private final ArrayList lookaheadStack = new ArrayList<>();
private void pushOntoCallStack(String methodName, String fileName, int line, int column) {
parsingStack.add(new NonTerminalCall("FreshMarkerParser", token_source, fileName, methodName, line, column));
}
private void popCallStack() {
NonTerminalCall ntc = parsingStack.remove(parsingStack.size() - 1);
this.currentlyParsedProduction = ntc.productionName;
}
private void restoreCallStack(int prevSize) {
while (parsingStack.size() > prevSize) {
popCallStack();
}
}
private void pushOntoLookaheadStack(String methodName, String fileName, int line, int column) {
lookaheadStack.add(new NonTerminalCall("FreshMarkerParser", token_source, fileName, methodName, line, column));
}
private void popLookaheadStack() {
NonTerminalCall ntc = lookaheadStack.remove(lookaheadStack.size() - 1);
this.currentLookaheadProduction = ntc.productionName;
}
void dumpLookaheadStack(PrintStream ps) {
ListIterator it = lookaheadStack.listIterator(lookaheadStack.size());
while (it.hasPrevious()) {
it.previous().dump(ps);
}
}
void dumpCallStack(PrintStream ps) {
ListIterator it = parsingStack.listIterator(parsingStack.size());
while (it.hasPrevious()) {
it.previous().dump(ps);
}
}
void dumpLookaheadCallStack(PrintStream ps) {
ps.println("Current Parser Production is: " + currentlyParsedProduction);
ps.println("Current Lookahead Production is: " + currentLookaheadProduction);
ps.println("---Lookahead Stack---");
dumpLookaheadStack(ps);
ps.println("---Call Stack---");
dumpCallStack(ps);
}
public boolean isParserTolerant() {
return false;
}
public void setParserTolerant(boolean tolerantParsing) {
if (tolerantParsing) {
throw new UnsupportedOperationException("This parser was not built with that feature!");
}
}
private Token consumeToken(TokenType expectedType) {
Token nextToken = nextToken(lastConsumedToken);
if (nextToken.getType() != expectedType) {
nextToken = handleUnexpectedTokenType(expectedType, nextToken);
}
this.lastConsumedToken = nextToken;
this.nextTokenType = null;
if (buildTree && tokensAreNodes) {
lastConsumedToken.open();
pushNode(lastConsumedToken);
lastConsumedToken.close();
}
return lastConsumedToken;
}
private Token handleUnexpectedTokenType(TokenType expectedType, Token nextToken) {
throw new ParseException(nextToken, EnumSet.of(expectedType), parsingStack);
}
private boolean buildTree = true;
private boolean tokensAreNodes = true;
private boolean unparsedTokensAreNodes = false;
public boolean isTreeBuildingEnabled() {
return buildTree;
}
public void setUnparsedTokensAreNodes(boolean unparsedTokensAreNodes) {
this.unparsedTokensAreNodes = unparsedTokensAreNodes;
}
public void setTokensAreNodes(boolean tokensAreNodes) {
this.tokensAreNodes = tokensAreNodes;
}
NodeScope currentNodeScope = new NodeScope();
/**
* @return the root node of the AST. It only makes sense to call
* this after a successful parse.
*/
public Node rootNode() {
return currentNodeScope.rootNode();
}
/**
* push a node onto the top of the node stack
* @param n the node to push
*/
public void pushNode(Node n) {
currentNodeScope.add(n);
}
/**
* @return the node on the top of the stack, and remove it from the
* stack.
*/
public Node popNode() {
return currentNodeScope.pop();
}
/**
* @return the node currently on the top of the tree-building stack.
*/
public Node peekNode() {
return currentNodeScope.peek();
}
/**
* Puts the node on the top of the stack. However, unlike pushNode()
* it replaces the node that is currently on the top of the stack.
* This is effectively equivalent to popNode() followed by pushNode(n)
* @param n the node to poke
*/
public void pokeNode(Node n) {
currentNodeScope.poke(n);
}
/**
* @return the number of Nodes on the tree-building stack in the current node
* scope.
*/
public int nodeArity() {
return currentNodeScope.size();
}
private void clearNodeScope() {
currentNodeScope.clear();
}
private void openNodeScope(Node n) {
new NodeScope();
if (n != null) {
n.setTokenSource(lastConsumedToken.getTokenSource());
// We set the begin/end offsets based on the ending location
// of the last consumed token. So, we start with a Node
// of length zero. Typically this is overridden in the
// closeNodeScope() method, unless this node has no children
n.setBeginOffset(lastConsumedToken.getEndOffset());
n.setEndOffset(n.getBeginOffset());
n.setTokenSource(this.token_source);
n.open();
}
}
/* A definite node is constructed from a specified number of
* children. That number of nodes are popped from the stack and
* made the children of the definite node. Then the definite node
* is pushed on to the stack.
* @param n is the node whose scope is being closed
* @param num is the number of child nodes to pop as children
* @return @{code true}
*/
private boolean closeNodeScope(Node n, int num) {
n.setBeginOffset(lastConsumedToken.getEndOffset());
n.setEndOffset(lastConsumedToken.getEndOffset());
currentNodeScope.close();
ArrayList nodes = new ArrayList<>();
for (int i = 0; i < num; i++) {
nodes.add(popNode());
}
Collections.reverse(nodes);
for (Node child : nodes) {
if (child.getInputSource() == n.getInputSource()) {
n.setBeginOffset(child.getBeginOffset());
break;
}
}
for (Node child : nodes) {
if (unparsedTokensAreNodes && child instanceof Token) {
Token tok = (Token) child;
while (tok.previousCachedToken() != null && tok.previousCachedToken().isUnparsed()) {
tok = tok.previousCachedToken();
}
boolean locationSet = false;
while (tok.isUnparsed()) {
n.add(tok);
if (!locationSet && tok.getInputSource() == n.getInputSource() && tok.getBeginOffset() < n.getBeginOffset()) {
n.setBeginOffset(tok.getBeginOffset());
locationSet = true;
}
tok = tok.nextCachedToken();
}
}
if (child.getInputSource() == n.getInputSource()) {
n.setEndOffset(child.getEndOffset());
}
n.add(child);
}
n.close();
pushNode(n);
return true;
}
/**
* A conditional node is constructed if the condition is true. All
* the nodes that have been pushed since the node was opened are
* made children of the conditional node, which is then pushed
* on to the stack. If the condition is false the node is not
* constructed and they are left on the stack.
*/
private boolean closeNodeScope(Node n, boolean condition) {
if (n == null || !condition) {
currentNodeScope.close();
return false;
}
return closeNodeScope(n, nodeArity());
}
public boolean getBuildTree() {
return buildTree;
}
public void setBuildTree(boolean buildTree) {
this.buildTree = buildTree;
}
@SuppressWarnings("serial")
class NodeScope extends ArrayList {
NodeScope parentScope;
NodeScope() {
this.parentScope = FreshMarkerParser.this.currentNodeScope;
FreshMarkerParser.this.currentNodeScope = this;
}
boolean isRootScope() {
return parentScope == null;
}
Node rootNode() {
NodeScope ns = this;
while (ns.parentScope != null) {
ns = ns.parentScope;
}
return ns.isEmpty() ? null : ns.get(0);
}
Node peek() {
if (isEmpty()) {
return parentScope == null ? null : parentScope.peek();
}
return get(size() - 1);
}
Node pop() {
return isEmpty() ? parentScope.pop() : remove(size() - 1);
}
void poke(Node n) {
if (isEmpty()) {
parentScope.poke(n);
} else {
set(size() - 1, n);
}
}
void close() {
parentScope.addAll(this);
FreshMarkerParser.this.currentNodeScope = parentScope;
}
int nestingLevel() {
int result = 0;
NodeScope parent = this;
while (parent.parentScope != null) {
result++;
parent = parent.parentScope;
}
return result;
}
public NodeScope clone() {
NodeScope clone = (NodeScope) super.clone();
if (parentScope != null) {
clone.parentScope = parentScope.clone();
}
return clone;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy