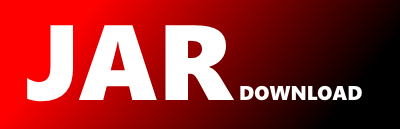
ftl.Token Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of freshmarker Show documentation
Show all versions of freshmarker Show documentation
A simple, small but powerful template engine based loosely on the FreeMarker syntax. FreshMarker is
implemented in Java 21 and supports the `java.time` API and Records.
/*
* Generated by: CongoCC Parser Generator. Token.java
*/
package ftl;
import org.freshmarker.core.ftl.FtlVisitor;
import org.freshmarker.core.ftl.ExpressionVisitor;
import ftl.ast.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
public class Token implements CharSequence, Node.TerminalNode {
public O accept(ExpressionVisitor visitor, I input) {
return (O) visitor.visit(this, input);
}
public O accept(FtlVisitor visitor, I input) {
return (O) visitor.visit(this, input);
}
public enum TokenType implements Node.NodeType {
EOF, _TOKEN_1, _TOKEN_2, _TOKEN_3, _TOKEN_4, _TOKEN_5, OPEN_PAREN, CLOSE_PAREN,
OPEN_BRACKET, CLOSE_BRACKET, OPEN_BRACE, CLOSE_BRACE, EQUALS, DOT, PLUS, MINUS,
PERCENT, LT, GT, COMMA, COLON, DOUBLE_COLON, SEMICOLON, EXCLAM, NOT, BUILT_IN,
DOUBLE_EQUALS, OR2, AND2, DOT_DOT, DOT_DOT_EXCLUSIVE, DOT_DOT_LENGTH, AS,
IN, ELLIPSIS, NULL, TRUE, FALSE, USING, WITH, SORTED, ASCENDING, DESCENDING,
LIMIT, FILTER, FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2, USER_DIRECTIVE_OPEN1,
USER_DIRECTIVE_OPEN2, INTERPOLATE, COMMENT, IF, SET, VAR, LIST, ELSE, CASE,
MACRO, LOCAL, BREAK, NESTED, SWITCH, IMPORT, DEFAULT, RETURN, GLOBAL, ASSIGN,
ELSEIF, FUNCTION, SETTING, BRICK, _INCLUDE, OUTPUT_FORMAT, EXP_WHITE_SPACE,
_TOKEN_74, TIMES, DIVIDE, OR, AND, XOR, NOT_EQUALS, EXISTS_OPERATOR, LTE,
GTE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL, WHITESPACE, SPECIAL_CHAR,
PRINTABLE_CHARS, END_DIRECTIVE1, END_DIRECTIVE2, END_USER_DIRECTIVE1, END_USER_DIRECTIVE2,
BLANK, CLOSE_TAG, CLOSE_EMPTY_TAG, _TOKEN_98, END_COMMENT, _TOKEN_100, NOPARSE_END,
DUMMY, INVALID;
public boolean isUndefined() {
return this == DUMMY;
}
public boolean isInvalid() {
return this == INVALID;
}
public boolean isEOF() {
return this == EOF;
}
}
private FreshMarkerLexer tokenSource;
private TokenType type = TokenType.DUMMY;
private int beginOffset;
private int endOffset;
private boolean unparsed;
private Node parent;
/**
* It would be extremely rare that an application
* programmer would use this method. It needs to
* be public because it is part of the ftl.Node interface.
*/
public void setBeginOffset(int beginOffset) {
this.beginOffset = beginOffset;
}
/**
* It would be extremely rare that an application
* programmer would use this method. It needs to
* be public because it is part of the ftl.Node interface.
*/
public void setEndOffset(int endOffset) {
this.endOffset = endOffset;
}
/**
* @return the FreshMarkerLexer object that handles
* location info for the tokens.
*/
public FreshMarkerLexer getTokenSource() {
return this.tokenSource;
}
/**
* It should be exceedingly rare that an application
* programmer needs to use this method.
*/
public void setTokenSource(TokenSource tokenSource) {
this.tokenSource = (FreshMarkerLexer) tokenSource;
}
public boolean isInvalid() {
return getType().isInvalid();
}
/**
* Return the TokenType of this Token object
*/
@Override
public TokenType getType() {
return type;
}
protected void setType(TokenType type) {
this.type = type;
}
/**
* @return whether this Token represent actual input or was it inserted somehow?
*/
public boolean isVirtual() {
return type == TokenType.EOF;
}
/**
* @return Did we skip this token in parsing?
*/
public boolean isSkipped() {
return false;
}
public int getBeginOffset() {
return beginOffset;
}
public int getEndOffset() {
return endOffset;
}
/**
* @return the string image of the token.
*/
@Override
/**
* @return the next _cached_ regular (i.e. parsed) token
* or null
*/
public final Token getNext() {
return getNextParsedToken();
}
/**
* @return the previous regular (i.e. parsed) token
* or null
*/
public final Token getPrevious() {
Token result = previousCachedToken();
while (result != null && result.isUnparsed()) {
result = result.previousCachedToken();
}
return result;
}
/**
* @return the next regular (i.e. parsed) token
*/
private Token getNextParsedToken() {
Token result = nextCachedToken();
while (result != null && result.isUnparsed()) {
result = result.nextCachedToken();
}
return result;
}
/**
* @return the next token of any sort (parsed or unparsed or invalid)
*/
public Token nextCachedToken() {
if (getType() == TokenType.EOF) return null;
FreshMarkerLexer tokenSource = getTokenSource();
return tokenSource != null ? (Token) tokenSource.nextCachedToken(getEndOffset()) : null;
}
public Token previousCachedToken() {
if (getTokenSource() == null) return null;
return (Token) getTokenSource().previousCachedToken(getBeginOffset());
}
Token getPreviousToken() {
return previousCachedToken();
}
public Token replaceType(TokenType type) {
Token result = newToken(type, getTokenSource(), getBeginOffset(), getEndOffset());
getTokenSource().cacheToken(result);
return result;
}
public String getSource() {
if (type == TokenType.EOF) return "";
FreshMarkerLexer ts = getTokenSource();
return ts == null ? null : ts.getText(getBeginOffset(), getEndOffset());
}
protected Token() {
}
public Token(TokenType type, FreshMarkerLexer tokenSource, int beginOffset, int endOffset) {
this.type = type;
this.tokenSource = tokenSource;
this.beginOffset = beginOffset;
this.endOffset = endOffset;
}
public boolean isUnparsed() {
return unparsed;
}
public void setUnparsed(boolean unparsed) {
this.unparsed = unparsed;
}
/**
* @return An iterator of the tokens preceding this one.
*/
public Iterator precedingTokens() {
return new Iterator() {
Token currentPoint = Token.this;
public boolean hasNext() {
return currentPoint.previousCachedToken() != null;
}
public Token next() {
Token previous = currentPoint.previousCachedToken();
if (previous == null) throw new java.util.NoSuchElementException("No previous token!");
return currentPoint = previous;
}
};
}
/**
* @return a list of the unparsed tokens preceding this one in the order they appear in the input
*/
public List precedingUnparsedTokens() {
List result = new ArrayList<>();
Token t = this.previousCachedToken();
while (t != null && t.isUnparsed()) {
result.add(t);
t = t.previousCachedToken();
}
Collections.reverse(result);
return result;
}
/**
* @return An iterator of the (cached) tokens that follow this one.
*/
public Iterator followingTokens() {
return new java.util.Iterator() {
Token currentPoint = Token.this;
public boolean hasNext() {
return currentPoint.nextCachedToken() != null;
}
public Token next() {
Token next = currentPoint.nextCachedToken();
if (next == null) throw new java.util.NoSuchElementException("No next token!");
return currentPoint = next;
}
};
}
public void copyLocationInfo(Token from) {
setTokenSource(from.getTokenSource());
setBeginOffset(from.getBeginOffset());
setEndOffset(from.getEndOffset());
}
public void copyLocationInfo(Token start, Token end) {
setTokenSource(start.getTokenSource());
if (tokenSource == null) setTokenSource(end.getTokenSource());
setBeginOffset(start.getBeginOffset());
setEndOffset(end.getEndOffset());
}
public static Token newToken(TokenType type, FreshMarkerLexer tokenSource, int beginOffset, int endOffset) {
switch(type) {
case ELSEIF :
return new ELSEIF(TokenType.ELSEIF, tokenSource, beginOffset, endOffset);
case VAR :
return new VAR(TokenType.VAR, tokenSource, beginOffset, endOffset);
case DOT_DOT_LENGTH :
return new DOT_DOT_LENGTH(TokenType.DOT_DOT_LENGTH, tokenSource, beginOffset, endOffset);
case LT :
return new LT(TokenType.LT, tokenSource, beginOffset, endOffset);
case END_COMMENT :
return new END_COMMENT(TokenType.END_COMMENT, tokenSource, beginOffset, endOffset);
case EXP_WHITE_SPACE :
return new EXP_WHITE_SPACE(TokenType.EXP_WHITE_SPACE, tokenSource, beginOffset, endOffset);
case DECIMAL :
return new DECIMAL(TokenType.DECIMAL, tokenSource, beginOffset, endOffset);
case MINUS :
return new MINUS(TokenType.MINUS, tokenSource, beginOffset, endOffset);
case END_USER_DIRECTIVE2 :
return new END_USER_DIRECTIVE2(TokenType.END_USER_DIRECTIVE2, tokenSource, beginOffset, endOffset);
case END_USER_DIRECTIVE1 :
return new END_USER_DIRECTIVE1(TokenType.END_USER_DIRECTIVE1, tokenSource, beginOffset, endOffset);
case OUTPUT_FORMAT :
return new OUTPUT_FORMAT(TokenType.OUTPUT_FORMAT, tokenSource, beginOffset, endOffset);
case OPEN_BRACE :
return new OPEN_BRACE(TokenType.OPEN_BRACE, tokenSource, beginOffset, endOffset);
case SEMICOLON :
return new SEMICOLON(TokenType.SEMICOLON, tokenSource, beginOffset, endOffset);
case WHITESPACE :
return new WHITESPACE(TokenType.WHITESPACE, tokenSource, beginOffset, endOffset);
case BREAK :
return new BREAK(TokenType.BREAK, tokenSource, beginOffset, endOffset);
case DESCENDING :
return new DESCENDING(TokenType.DESCENDING, tokenSource, beginOffset, endOffset);
case LOCAL :
return new LOCAL(TokenType.LOCAL, tokenSource, beginOffset, endOffset);
case ELSE :
return new ELSE(TokenType.ELSE, tokenSource, beginOffset, endOffset);
case SETTING :
return new SETTING(TokenType.SETTING, tokenSource, beginOffset, endOffset);
case INTERPOLATE :
return new INTERPOLATE(TokenType.INTERPOLATE, tokenSource, beginOffset, endOffset);
case IF :
return new IF(TokenType.IF, tokenSource, beginOffset, endOffset);
case SORTED :
return new SORTED(TokenType.SORTED, tokenSource, beginOffset, endOffset);
case INTEGER :
return new INTEGER(TokenType.INTEGER, tokenSource, beginOffset, endOffset);
case NULL :
return new NULL(TokenType.NULL, tokenSource, beginOffset, endOffset);
case IN :
return new IN(TokenType.IN, tokenSource, beginOffset, endOffset);
case DOUBLE_EQUALS :
return new DOUBLE_EQUALS(TokenType.DOUBLE_EQUALS, tokenSource, beginOffset, endOffset);
case PRINTABLE_CHARS :
return new PRINTABLE_CHARS(TokenType.PRINTABLE_CHARS, tokenSource, beginOffset, endOffset);
case DOT :
return new DOT(TokenType.DOT, tokenSource, beginOffset, endOffset);
case DOT_DOT_EXCLUSIVE :
return new DOT_DOT_EXCLUSIVE(TokenType.DOT_DOT_EXCLUSIVE, tokenSource, beginOffset, endOffset);
case TRUE :
return new TRUE(TokenType.TRUE, tokenSource, beginOffset, endOffset);
case FUNCTION :
return new FUNCTION(TokenType.FUNCTION, tokenSource, beginOffset, endOffset);
case OR2 :
return new OR2(TokenType.OR2, tokenSource, beginOffset, endOffset);
case CASE :
return new CASE(TokenType.CASE, tokenSource, beginOffset, endOffset);
case NOPARSE_END :
return new NOPARSE_END(TokenType.NOPARSE_END, tokenSource, beginOffset, endOffset);
case USING :
return new USING(TokenType.USING, tokenSource, beginOffset, endOffset);
case NOT :
return new NOT(TokenType.NOT, tokenSource, beginOffset, endOffset);
case AS :
return new AS(TokenType.AS, tokenSource, beginOffset, endOffset);
case OPEN_PAREN :
return new OPEN_PAREN(TokenType.OPEN_PAREN, tokenSource, beginOffset, endOffset);
case ELLIPSIS :
return new ELLIPSIS(TokenType.ELLIPSIS, tokenSource, beginOffset, endOffset);
case AND :
return new AND(TokenType.AND, tokenSource, beginOffset, endOffset);
case SWITCH :
return new SWITCH(TokenType.SWITCH, tokenSource, beginOffset, endOffset);
case XOR :
return new XOR(TokenType.XOR, tokenSource, beginOffset, endOffset);
case CLOSE_PAREN :
return new CLOSE_PAREN(TokenType.CLOSE_PAREN, tokenSource, beginOffset, endOffset);
case FTL_DIRECTIVE_OPEN1 :
return new FTL_DIRECTIVE_OPEN1(TokenType.FTL_DIRECTIVE_OPEN1, tokenSource, beginOffset, endOffset);
case LTE :
return new LTE(TokenType.LTE, tokenSource, beginOffset, endOffset);
case FTL_DIRECTIVE_OPEN2 :
return new FTL_DIRECTIVE_OPEN2(TokenType.FTL_DIRECTIVE_OPEN2, tokenSource, beginOffset, endOffset);
case LIST :
return new LIST(TokenType.LIST, tokenSource, beginOffset, endOffset);
case EXCLAM :
return new EXCLAM(TokenType.EXCLAM, tokenSource, beginOffset, endOffset);
case PLUS :
return new PLUS(TokenType.PLUS, tokenSource, beginOffset, endOffset);
case BUILT_IN :
return new BUILT_IN(TokenType.BUILT_IN, tokenSource, beginOffset, endOffset);
case EXISTS_OPERATOR :
return new EXISTS_OPERATOR(TokenType.EXISTS_OPERATOR, tokenSource, beginOffset, endOffset);
case SET :
return new SET(TokenType.SET, tokenSource, beginOffset, endOffset);
case AND2 :
return new AND2(TokenType.AND2, tokenSource, beginOffset, endOffset);
case SPECIAL_CHAR :
return new SPECIAL_CHAR(TokenType.SPECIAL_CHAR, tokenSource, beginOffset, endOffset);
case CLOSE_BRACKET :
return new CLOSE_BRACKET(TokenType.CLOSE_BRACKET, tokenSource, beginOffset, endOffset);
case LIMIT :
return new LIMIT(TokenType.LIMIT, tokenSource, beginOffset, endOffset);
case ASSIGN :
return new ASSIGN(TokenType.ASSIGN, tokenSource, beginOffset, endOffset);
case COMMENT :
return new COMMENT(TokenType.COMMENT, tokenSource, beginOffset, endOffset);
case DIVIDE :
return new DIVIDE(TokenType.DIVIDE, tokenSource, beginOffset, endOffset);
case DOT_DOT :
return new DOT_DOT(TokenType.DOT_DOT, tokenSource, beginOffset, endOffset);
case IMPORT :
return new IMPORT(TokenType.IMPORT, tokenSource, beginOffset, endOffset);
case RETURN :
return new RETURN(TokenType.RETURN, tokenSource, beginOffset, endOffset);
case CLOSE_BRACE :
return new CLOSE_BRACE(TokenType.CLOSE_BRACE, tokenSource, beginOffset, endOffset);
case USER_DIRECTIVE_OPEN2 :
return new USER_DIRECTIVE_OPEN2(TokenType.USER_DIRECTIVE_OPEN2, tokenSource, beginOffset, endOffset);
case GLOBAL :
return new GLOBAL(TokenType.GLOBAL, tokenSource, beginOffset, endOffset);
case IDENTIFIER :
return new IDENTIFIER(TokenType.IDENTIFIER, tokenSource, beginOffset, endOffset);
case USER_DIRECTIVE_OPEN1 :
return new USER_DIRECTIVE_OPEN1(TokenType.USER_DIRECTIVE_OPEN1, tokenSource, beginOffset, endOffset);
case COMMA :
return new COMMA(TokenType.COMMA, tokenSource, beginOffset, endOffset);
case FILTER :
return new FILTER(TokenType.FILTER, tokenSource, beginOffset, endOffset);
case OR :
return new OR(TokenType.OR, tokenSource, beginOffset, endOffset);
case EQUALS :
return new EQUALS(TokenType.EQUALS, tokenSource, beginOffset, endOffset);
case TIMES :
return new TIMES(TokenType.TIMES, tokenSource, beginOffset, endOffset);
case NOT_EQUALS :
return new NOT_EQUALS(TokenType.NOT_EQUALS, tokenSource, beginOffset, endOffset);
case OPEN_BRACKET :
return new OPEN_BRACKET(TokenType.OPEN_BRACKET, tokenSource, beginOffset, endOffset);
case BRICK :
return new BRICK(TokenType.BRICK, tokenSource, beginOffset, endOffset);
case COLON :
return new COLON(TokenType.COLON, tokenSource, beginOffset, endOffset);
case END_DIRECTIVE1 :
return new END_DIRECTIVE1(TokenType.END_DIRECTIVE1, tokenSource, beginOffset, endOffset);
case END_DIRECTIVE2 :
return new END_DIRECTIVE2(TokenType.END_DIRECTIVE2, tokenSource, beginOffset, endOffset);
case GT :
return new GT(TokenType.GT, tokenSource, beginOffset, endOffset);
case _INCLUDE :
return new _INCLUDE(TokenType._INCLUDE, tokenSource, beginOffset, endOffset);
case WITH :
return new WITH(TokenType.WITH, tokenSource, beginOffset, endOffset);
case BLANK :
return new BLANK(TokenType.BLANK, tokenSource, beginOffset, endOffset);
case DOUBLE_COLON :
return new DOUBLE_COLON(TokenType.DOUBLE_COLON, tokenSource, beginOffset, endOffset);
case ASCENDING :
return new ASCENDING(TokenType.ASCENDING, tokenSource, beginOffset, endOffset);
case PERCENT :
return new PERCENT(TokenType.PERCENT, tokenSource, beginOffset, endOffset);
case MACRO :
return new MACRO(TokenType.MACRO, tokenSource, beginOffset, endOffset);
case GTE :
return new GTE(TokenType.GTE, tokenSource, beginOffset, endOffset);
case FALSE :
return new FALSE(TokenType.FALSE, tokenSource, beginOffset, endOffset);
case NESTED :
return new NESTED(TokenType.NESTED, tokenSource, beginOffset, endOffset);
case STRING_LITERAL :
return new STRING_LITERAL(TokenType.STRING_LITERAL, tokenSource, beginOffset, endOffset);
case CLOSE_TAG :
return new CLOSE_TAG(TokenType.CLOSE_TAG, tokenSource, beginOffset, endOffset);
case CLOSE_EMPTY_TAG :
return new CLOSE_EMPTY_TAG(TokenType.CLOSE_EMPTY_TAG, tokenSource, beginOffset, endOffset);
case DEFAULT :
return new DEFAULT(TokenType.DEFAULT, tokenSource, beginOffset, endOffset);
case INVALID :
return new InvalidToken(tokenSource, beginOffset, endOffset);
default :
return new Token(type, tokenSource, beginOffset, endOffset);
}
}
public String getLocation() {
return getInputSource() + ":" + getBeginLine() + ":" + getBeginColumn();
}
public Node getParent() {
return parent;
}
public void setParent(Node parent) {
this.parent = parent;
}
public boolean isEmpty() {
return length() == 0;
}
public int length() {
return endOffset - beginOffset;
}
public CharSequence subSequence(int start, int end) {
return getTokenSource().subSequence(beginOffset + start, beginOffset + end);
}
public char charAt(int offset) {
return getTokenSource().charAt(beginOffset + offset);
}
/**
* @deprecated Use toString() instead
*/
@Deprecated
public String getImage() {
return getSource();
}
@Override
public String toString() {
return getSource();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy