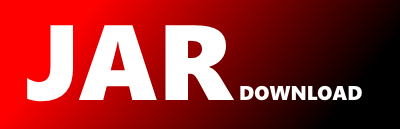
ftl.FreshMarkerLexer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of freshmarker Show documentation
Show all versions of freshmarker Show documentation
A simple, small but powerful template engine based loosely on the FreeMarker syntax. FreshMarker is
implemented in Java 21 and supports the `java.time` API and Records.
/* Generated by: CongoCC Parser Generator. FreshMarkerLexer.java */
package ftl;
import java.io.Reader;
import ftl.Token.TokenType;
import static ftl.Token.TokenType.*;
import java.io.IOException;
import java.util.*;
public class FreshMarkerLexer extends TokenSource {
boolean syntaxEstablished, olderSyntax;
boolean noWhitespaceInExpression, rightAfterHeader;
int parenthesisNesting, bracketNesting, braceNesting;
TokenType lastMatchedTokenType;
Token tokenHook(Token tok) {
if (tok.getType() == TokenType.EOF) return tok;
if (noWhitespaceInExpression && parenthesisNesting == 0 && braceNesting == 0 && tok.getPreviousToken() != null && tok.getPreviousToken().isUnparsed()) {
if (tok.getPreviousToken().toString().trim().length() == 0) {
Token copy = cloneToken(tok);
tok.copyLocationInfo(tok.getPreviousToken());
tok.setType(TokenType.BLANK);
//tok.setNext(copy);
noWhitespaceInExpression = false;
lastMatchedTokenType = tok.getType();
return tok;
}
}
int firstChar = tok.toString().codePointAt(0);
int lastChar = tok.toString().codePointAt(tok.toString().length() - 1);
boolean hasPointyDelimiter = firstChar == '<' || lastChar == '>';
if (rightAfterHeader) {
// chop off the newline after a <#ftl ...> header
rightAfterHeader = false;
if (tok.getType() == TokenType.WHITESPACE) {
String img = tok.toString();
int idx = img.indexOf('\n');
if (idx < 0) idx = img.indexOf('\r');
if (idx >= 0) {
tok.setBeginOffset(tok.getBeginOffset() + idx);
}
}
}
switch(tok.getType()) {
case USER_DIRECTIVE_OPEN1 : case USER_DIRECTIVE_OPEN2 : case FTL_DIRECTIVE_OPEN1 : case FTL_DIRECTIVE_OPEN2 :
if (syntaxEstablished) {
if (hasPointyDelimiter != olderSyntax) {
tok.setType(TokenType.PRINTABLE_CHARS);
} else {
if (tok.getType() == FTL_DIRECTIVE_OPEN1 || tok.getType() == FTL_DIRECTIVE_OPEN2) switchTo(LexicalState.FTL_DIRECTIVE);
else {
noWhitespaceInExpression = true;
switchTo(LexicalState.FTL_EXPRESSION);
}
}
} else {
olderSyntax = (firstChar == '<');
//activeTokenTypes.remove(FTL_D)
syntaxEstablished = true;
}
break;
case CLOSE_TAG : case CLOSE_EMPTY_TAG :
if (olderSyntax != hasPointyDelimiter) tok.setType(TokenType.PRINTABLE_CHARS);
else switchTo(LexicalState.TEMPLATE_TEXT);
break;
case END_DIRECTIVE1 : case END_DIRECTIVE2 :
if (olderSyntax != hasPointyDelimiter) tok.setType(TokenType.PRINTABLE_CHARS);
else switchTo(LexicalState.TEMPLATE_TEXT);
break;
case END_USER_DIRECTIVE1 : case END_USER_DIRECTIVE2 :
if (hasPointyDelimiter != olderSyntax) tok.setType(TokenType.PRINTABLE_CHARS);
break;
case GT : case GTE :
if (parenthesisNesting == 0 && olderSyntax) {
tok.setType(TokenType.CLOSE_TAG);
if (tok.getType() == TokenType.GTE) {
tok.truncate(1);
//tok.setImage(">");
//tok.setEndOffset(tok.getEndOffset()-1);
//bufferPosition = backup(bufferPosition,1);
//bufferPosition = tok.getEndOffset();
}
switchTo(LexicalState.TEMPLATE_TEXT);
}
break;
case OPEN_PAREN :
parenthesisNesting++;
break;
case CLOSE_PAREN :
parenthesisNesting--;
break;
case OPEN_BRACKET :
bracketNesting++;
break;
case CLOSE_BRACKET :
if (bracketNesting > 0) {
--bracketNesting;
} else if (!olderSyntax) {
tok.setType(TokenType.CLOSE_TAG);
switchTo(LexicalState.TEMPLATE_TEXT);
}
break;
case OPEN_BRACE :
braceNesting++;
break;
case CLOSE_BRACE :
if (braceNesting > 0) braceNesting--;
else switchTo(LexicalState.TEMPLATE_TEXT);
break;
case END_COMMENT :
if (hasPointyDelimiter != olderSyntax) tok.setType(TokenType.PRINTABLE_CHARS);
else switchTo(LexicalState.TEMPLATE_TEXT);
break;
default :
if (lastMatchedTokenType == TokenType.DOT && possibleIdentifier(tok.toString())) {
tok.setType(TokenType.IDENTIFIER);
}
}
lastMatchedTokenType = tok.getType();
return tok;
}
private boolean possibleIdentifier(String s) {
for (int c : s.codePoints().toArray()) {
if (c < 'a' || c > 'z') return false;
}
return true;
}
private Token cloneToken(Token t) {
Token result = Token.newToken(t.getType(), this, 0, 0);
result.copyLocationInfo(t);
return result;
}
static final int BUF_SIZE = 0x10000;
static String readFully(Reader reader) throws IOException {
char[] block = new char[BUF_SIZE];
int charsRead = reader.read(block);
if (charsRead < 0) {
throw new IOException("No input");
} else if (charsRead < BUF_SIZE) {
char[] result = new char[charsRead];
System.arraycopy(block, 0, result, 0, charsRead);
reader.close();
return new String(block, 0, charsRead);
}
StringBuilder buf = new StringBuilder();
buf.append(block);
do {
charsRead = reader.read(block);
if (charsRead > 0) {
buf.append(block, 0, charsRead);
}
}
while (charsRead == BUF_SIZE);
reader.close();
return buf.toString();
}
// Annoying kludge really...
static String readToEnd(Reader reader) {
try {
return readFully(reader);
} catch (IOException ioe) {
throw new RuntimeException(ioe);
}
}
private static MatcherHook MATCHER_HOOK;
// this cannot be initialize here, since hook must be set afterwards
public enum LexicalState {
TEMPLATE_TEXT, FTL_EXPRESSION, EXPRESSION_COMMENT, FTL_DIRECTIVE, IN_COMMENT,
NO_PARSE
}
LexicalState lexicalState = LexicalState.values()[0];
EnumSet activeTokenTypes = null;
// A lookup for lexical state transitions triggered by a certain token type
private static final EnumMap tokenTypeToLexicalStateMap = new EnumMap<>(TokenType.class);
// Token types that are "regular" tokens that participate in parsing,
// i.e. declared as TOKEN
static final EnumSet regularTokens = EnumSet.of(EOF, OPEN_PAREN, CLOSE_PAREN, OPEN_BRACKET, CLOSE_BRACKET, OPEN_BRACE, CLOSE_BRACE, EQUALS, DOT, PLUS, MINUS, PERCENT, LT, GT, COMMA, COLON, DOUBLE_COLON, SEMICOLON, EXCLAM, NOT, BUILT_IN, DOUBLE_EQUALS, OR2, AND2, DOT_DOT, DOT_DOT_EXCLUSIVE, DOT_DOT_LENGTH, AS, IN, ELLIPSIS, NULL, TRUE, FALSE, USING, WITH, SORTED, ASCENDING, DESCENDING, LIMIT, FILTER, FTL_DIRECTIVE_OPEN1, FTL_DIRECTIVE_OPEN2, USER_DIRECTIVE_OPEN1, USER_DIRECTIVE_OPEN2, INTERPOLATE, COMMENT, IF, SET, VAR, LIST, ELSE, CASE, MACRO, LOCAL, BREAK, NESTED, SWITCH, IMPORT, DEFAULT, RETURN, GLOBAL, ASSIGN, ELSEIF, FUNCTION, SETTING, BRICK, _INCLUDE, OUTPUT_FORMAT, TIMES, DIVIDE, OR, AND, XOR, NOT_EQUALS, EXISTS_OPERATOR, LTE, GTE, INTEGER, DECIMAL, IDENTIFIER, STRING_LITERAL, WHITESPACE, SPECIAL_CHAR, PRINTABLE_CHARS, END_DIRECTIVE1, END_DIRECTIVE2, END_USER_DIRECTIVE1, END_USER_DIRECTIVE2, BLANK, CLOSE_TAG, CLOSE_EMPTY_TAG, END_COMMENT, NOPARSE_END);
// Token types that do not participate in parsing
// i.e. declared as UNPARSED (or SPECIAL_TOKEN)
static final EnumSet unparsedTokens = EnumSet.of(_TOKEN_4, _TOKEN_5);
// Tokens that are skipped, i.e. SKIP
static final EnumSet skippedTokens = EnumSet.of(EXP_WHITE_SPACE);
// Tokens that correspond to a MORE, i.e. that are pending
// additional input
static final EnumSet moreTokens = EnumSet.of(_TOKEN_1, _TOKEN_2, _TOKEN_3, _TOKEN_74, _TOKEN_98, _TOKEN_100);
public FreshMarkerLexer(CharSequence input) {
this("input", input);
}
/**
* @param inputSource just the name of the input source (typically the filename)
* that will be used in error messages and so on.
* @param input the input
*/
public FreshMarkerLexer(String inputSource, CharSequence input) {
this(inputSource, input, LexicalState.TEMPLATE_TEXT, 1, 1);
}
/**
* @param inputSource just the name of the input source (typically the filename) that
* will be used in error messages and so on.
* @param input the input
* @param lexicalState The starting lexical state, may be null to indicate the default
* starting state
* @param line The line number at which we are starting for the purposes of location/error messages. In most
* normal usage, this is 1.
* @param column number at which we are starting for the purposes of location/error messages. In most normal
* usages this is 1.
*/
public FreshMarkerLexer(String inputSource, CharSequence input, LexicalState lexState, int startingLine, int startingColumn) {
super(inputSource, input, startingLine, startingColumn, 1, true, false, false, "");
if (lexicalState != null) switchTo(lexState);
}
public Token getNextToken(Token tok) {
return getNextToken(tok, this.activeTokenTypes);
}
/**
* The public method for getting the next token, that is
* called by FreshMarkerParser.
* It checks whether we have already cached
* the token after this one. If not, it finally goes
* to the NFA machinery
*/
public Token getNextToken(Token tok, EnumSet activeTokenTypes) {
if (tok == null) {
tok = tokenizeAt(0, null, activeTokenTypes);
cacheToken(tok);
return tok;
}
Token cachedToken = tok.nextCachedToken();
// If the cached next token is not currently active, we
// throw it away and go back to the FreshMarkerLexer
if (cachedToken != null && activeTokenTypes != null && !activeTokenTypes.contains(cachedToken.getType())) {
reset(tok);
cachedToken = null;
}
if (cachedToken == null) {
Token token = tokenizeAt(tok.getEndOffset(), null, activeTokenTypes);
cacheToken(token);
return token;
}
return cachedToken;
}
static class MatchInfo {
TokenType matchedType;
int matchLength;
@Override
public int hashCode() {
return Objects.hash(matchLength, matchedType);
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (getClass() != obj.getClass()) return false;
MatchInfo other = (MatchInfo) obj;
return matchLength == other.matchLength && matchedType == other.matchedType;
}
}
@FunctionalInterface
private interface MatcherHook {
MatchInfo apply(LexicalState lexicalState, CharSequence input, int position, EnumSet activeTokenTypes, NfaFunction[] nfaFunctions, BitSet currentStates, BitSet nextStates, MatchInfo matchInfo);
}
/**
* Core tokenization method. Note that this can be called from a static context.
* Hence the extra parameters that need to be passed in.
*/
static MatchInfo getMatchInfo(CharSequence input, int position, EnumSet activeTokenTypes, NfaFunction[] nfaFunctions, BitSet currentStates, BitSet nextStates, MatchInfo matchInfo) {
if (matchInfo == null) {
matchInfo = new MatchInfo();
}
if (position >= input.length()) {
matchInfo.matchedType = EOF;
matchInfo.matchLength = 0;
return matchInfo;
}
int start = position;
int matchLength = 0;
TokenType matchedType = TokenType.INVALID;
EnumSet alreadyMatchedTypes = EnumSet.noneOf(TokenType.class);
if (currentStates == null) currentStates = new BitSet(151);
else currentStates.clear();
if (nextStates == null) nextStates = new BitSet(151);
else nextStates.clear();
// the core NFA loop
do {
// Holder for the new type (if any) matched on this iteration
if (position > start) {
// What was nextStates on the last iteration
// is now the currentStates!
BitSet temp = currentStates;
currentStates = nextStates;
nextStates = temp;
nextStates.clear();
} else {
currentStates.set(0);
}
if (position >= input.length()) {
break;
}
int curChar = Character.codePointAt(input, position++);
if (curChar > 0xFFFF) position++;
int nextActive = currentStates.nextSetBit(0);
while (nextActive != -1) {
TokenType returnedType = nfaFunctions[nextActive].apply(curChar, nextStates, activeTokenTypes, alreadyMatchedTypes);
if (returnedType != null && (position - start > matchLength || returnedType.ordinal() < matchedType.ordinal())) {
matchedType = returnedType;
matchLength = position - start;
alreadyMatchedTypes.add(returnedType);
}
nextActive = currentStates.nextSetBit(nextActive + 1);
}
if (position >= input.length()) break;
}
while (!nextStates.isEmpty());
matchInfo.matchedType = matchedType;
matchInfo.matchLength = matchLength;
return matchInfo;
}
/**
* @param position The position at which to tokenize.
* @param lexicalState The lexical state in which to tokenize. If this is null, it is the instance variable #lexicalState
* @param activeTokenTypes The active token types. If this is null, they are all active.
* @return the Token at position
*/
final Token tokenizeAt(int position, LexicalState lexicalState, EnumSet activeTokenTypes) {
if (lexicalState == null) lexicalState = this.lexicalState;
int tokenBeginOffset = position;
boolean inMore = false;
int invalidRegionStart = -1;
Token matchedToken = null;
TokenType matchedType = null;
// The core tokenization loop
MatchInfo matchInfo = new MatchInfo();
BitSet currentStates = new BitSet(151);
BitSet nextStates = new BitSet(151);
while (matchedToken == null) {
// Get the NFA function table for the current lexical state.
// If we are in a MORE, there is some possibility that there
// was a lexical state change since the last iteration of this loop!
NfaFunction[] nfaFunctions = functionTableMap.get(lexicalState);
if (!inMore) tokenBeginOffset = position;
if (MATCHER_HOOK != null) {
matchInfo = MATCHER_HOOK.apply(lexicalState, this, position, activeTokenTypes, nfaFunctions, currentStates, nextStates, matchInfo);
if (matchInfo == null) {
matchInfo = getMatchInfo(this, position, activeTokenTypes, nfaFunctions, currentStates, nextStates, matchInfo);
}
} else {
matchInfo = getMatchInfo(this, position, activeTokenTypes, nfaFunctions, currentStates, nextStates, matchInfo);
}
matchedType = matchInfo.matchedType;
inMore = moreTokens.contains(matchedType);
position += matchInfo.matchLength;
LexicalState newState = tokenTypeToLexicalStateMap.get(matchedType);
if (newState != null) {
lexicalState = this.lexicalState = newState;
}
if (matchedType == TokenType.INVALID) {
if (invalidRegionStart == -1) {
invalidRegionStart = tokenBeginOffset;
}
int cp = Character.codePointAt(this, position);
++position;
if (cp > 0xFFFF) ++position;
continue;
}
if (invalidRegionStart != -1) {
return new InvalidToken(this, invalidRegionStart, tokenBeginOffset);
}
if (skippedTokens.contains(matchedType)) {
skipTokens(tokenBeginOffset, position);
} else if (regularTokens.contains(matchedType) || unparsedTokens.contains(matchedType)) {
matchedToken = Token.newToken(matchedType, this, tokenBeginOffset, position);
matchedToken.setUnparsed(!regularTokens.contains(matchedType));
}
}
doLexicalStateSwitch(matchedToken.getType());
matchedToken = tokenHook(matchedToken);
return matchedToken;
}
// Generate the map for lexical state transitions from the various token types
static {
tokenTypeToLexicalStateMap.put(_TOKEN_1, LexicalState.EXPRESSION_COMMENT);
tokenTypeToLexicalStateMap.put(_TOKEN_2, LexicalState.EXPRESSION_COMMENT);
tokenTypeToLexicalStateMap.put(_TOKEN_3, LexicalState.EXPRESSION_COMMENT);
tokenTypeToLexicalStateMap.put(_TOKEN_4, LexicalState.FTL_EXPRESSION);
tokenTypeToLexicalStateMap.put(_TOKEN_5, LexicalState.FTL_EXPRESSION);
tokenTypeToLexicalStateMap.put(FTL_DIRECTIVE_OPEN1, LexicalState.FTL_DIRECTIVE);
tokenTypeToLexicalStateMap.put(FTL_DIRECTIVE_OPEN2, LexicalState.FTL_DIRECTIVE);
tokenTypeToLexicalStateMap.put(USER_DIRECTIVE_OPEN1, LexicalState.FTL_EXPRESSION);
tokenTypeToLexicalStateMap.put(USER_DIRECTIVE_OPEN2, LexicalState.FTL_EXPRESSION);
tokenTypeToLexicalStateMap.put(INTERPOLATE, LexicalState.FTL_EXPRESSION);
tokenTypeToLexicalStateMap.put(COMMENT, LexicalState.IN_COMMENT);
tokenTypeToLexicalStateMap.put(BLANK, LexicalState.FTL_EXPRESSION);
tokenTypeToLexicalStateMap.put(CLOSE_TAG, LexicalState.TEMPLATE_TEXT);
tokenTypeToLexicalStateMap.put(CLOSE_EMPTY_TAG, LexicalState.TEMPLATE_TEXT);
tokenTypeToLexicalStateMap.put(NOPARSE_END, LexicalState.TEMPLATE_TEXT);
}
boolean doLexicalStateSwitch(TokenType tokenType) {
LexicalState newState = tokenTypeToLexicalStateMap.get(tokenType);
if (newState == null) return false;
return switchTo(newState);
}
/**
* Switch to specified lexical state.
* @param lexState the lexical state to switch to
* @return whether we switched (i.e. we weren't already in the desired lexical state)
*/
public boolean switchTo(LexicalState lexState) {
if (this.lexicalState != lexState) {
this.lexicalState = lexState;
return true;
}
return false;
}
// Reset the token source input
// to just after the Token passed in.
void reset(Token t, LexicalState state) {
uncacheTokens(t);
if (state != null) {
switchTo(state);
} else {
doLexicalStateSwitch(t.getType());
}
}
void reset(Token t) {
reset(t, null);
}
// NFA related code follows.
// The functional interface that represents
// the acceptance method of an NFA state
@FunctionalInterface
interface NfaFunction {
TokenType apply(int ch, BitSet bs, EnumSet validTypes, EnumSet alreadyMatchedTypes);
}
// A lookup of the NFA function tables for the respective lexical states.
private static final EnumMap functionTableMap = new EnumMap<>(LexicalState.class);
// Initialize the various NFA method tables
static {
TEMPLATE_TEXT.NFA_FUNCTIONS_init();
FTL_EXPRESSION.NFA_FUNCTIONS_init();
EXPRESSION_COMMENT.NFA_FUNCTIONS_init();
FTL_DIRECTIVE.NFA_FUNCTIONS_init();
IN_COMMENT.NFA_FUNCTIONS_init();
NO_PARSE.NFA_FUNCTIONS_init();
}
//The Nitty-gritty of the NFA code follows.
/**
* Holder class for NFA code related to TEMPLATE_TEXT lexical state
*/
private static class TEMPLATE_TEXT {
private static TokenType getNfaNameTEMPLATE_TEXTIndex0(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (ch == '$') {
if (validTypes == null || validTypes.contains(INTERPOLATE)) {
nextStates.set(10);
}
} else if (ch == '<') {
if (validTypes == null || validTypes.contains(USER_DIRECTIVE_OPEN1)) {
nextStates.set(5);
}
if (validTypes == null || validTypes.contains(END_USER_DIRECTIVE2)) {
nextStates.set(24);
}
if (validTypes == null || validTypes.contains(END_DIRECTIVE2)) {
nextStates.set(27);
}
if (validTypes == null || validTypes.contains(FTL_DIRECTIVE_OPEN1)) {
nextStates.set(16);
}
} else if (ch == '[') {
if (validTypes == null || validTypes.contains(END_DIRECTIVE1)) {
nextStates.set(22);
}
if (validTypes == null || validTypes.contains(FTL_DIRECTIVE_OPEN2)) {
nextStates.set(4);
}
if (validTypes == null || validTypes.contains(USER_DIRECTIVE_OPEN2)) {
nextStates.set(12);
}
if (validTypes == null || validTypes.contains(END_USER_DIRECTIVE1)) {
nextStates.set(29);
}
} else if ((ch >= 0x0 && ch <= 0x8) || ((ch == 0xb || ch == '\f') || ((ch >= 0xe && ch <= 0x1f) || ((ch >= '!' && ch <= '#') || ((ch >= '%' && ch <= ';') || ((ch >= '=' && ch <= 'Z') || (ch >= '\\'))))))) {
if (validTypes == null || validTypes.contains(PRINTABLE_CHARS)) {
nextStates.set(11);
type = PRINTABLE_CHARS;
}
}
if (ch == '$') {
if (validTypes == null || validTypes.contains(SPECIAL_CHAR)) {
type = SPECIAL_CHAR;
}
} else if (ch == '<') {
if (validTypes == null || validTypes.contains(SPECIAL_CHAR)) {
type = SPECIAL_CHAR;
}
} else if (ch == '[') {
if (validTypes == null || validTypes.contains(SPECIAL_CHAR)) {
type = SPECIAL_CHAR;
}
} else if ((ch == '\t' || ch == '\n') || ((ch == '\r') || (ch == ' '))) {
if (validTypes == null || validTypes.contains(WHITESPACE)) {
nextStates.set(21);
type = WHITESPACE;
}
}
return type;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex1(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (ch == '\t') {
nextStates.set(2);
} else if (ch == '\n') {
nextStates.set(2);
} else if (ch == '\r') {
nextStates.set(2);
} else if (ch == ' ') {
nextStates.set(2);
} else if (((ch == '$') || ((ch >= 'A' && ch <= 'Z') || ((ch == '_') || (ch >= 'a' && ch <= 'z')))) || (ch >= 128 && checkIntervals(NFA_MOVES_TEMPLATE_TEXT_19, ch))) {
nextStates.set(3);
} else if (ch == '@') {
nextStates.set(3);
} else if (ch == ']') {
type = END_DIRECTIVE1;
}
return type;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex2(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (ch == '\t') {
nextStates.set(2);
} else if (ch == '\n') {
nextStates.set(2);
} else if (ch == '\r') {
nextStates.set(2);
} else if (ch == ' ') {
nextStates.set(2);
} else if (ch == ']') {
type = END_DIRECTIVE1;
}
return type;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex3(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (((ch >= 0x0 && ch <= 0x8) || ((ch >= 0xe && ch <= 0x1b) || ((ch == '$') || ((ch >= '0' && ch <= '9') || ((ch >= 'A' && ch <= 'Z') || ((ch == '_') || ((ch >= 'a' && ch <= 'z') || (ch >= 0x7f && ch <= 0x9f)))))))) || (ch >= 128 && checkIntervals(NFA_MOVES_TEMPLATE_TEXT_21, ch))) {
nextStates.set(3);
} else if (ch == '\t') {
nextStates.set(2);
} else if (ch == '\n') {
nextStates.set(2);
} else if (ch == '\r') {
nextStates.set(2);
} else if (ch == ' ') {
nextStates.set(2);
} else if (ch == '@') {
nextStates.set(3);
} else if (ch == ']') {
type = END_DIRECTIVE1;
}
return type;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex4(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '#') {
return FTL_DIRECTIVE_OPEN2;
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex5(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '@') {
return USER_DIRECTIVE_OPEN1;
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex6(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (ch == '\t') {
nextStates.set(7);
} else if (ch == '\n') {
nextStates.set(7);
} else if (ch == '\r') {
nextStates.set(7);
} else if (ch == ' ') {
nextStates.set(7);
} else if (((ch == '$') || ((ch >= 'A' && ch <= 'Z') || ((ch == '_') || (ch >= 'a' && ch <= 'z')))) || (ch >= 128 && checkIntervals(NFA_MOVES_TEMPLATE_TEXT_60, ch))) {
nextStates.set(8);
} else if (ch == '@') {
nextStates.set(8);
} else if (ch == '>') {
type = END_USER_DIRECTIVE2;
}
return type;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex7(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (ch == '\t') {
nextStates.set(7);
} else if (ch == '\n') {
nextStates.set(7);
} else if (ch == '\r') {
nextStates.set(7);
} else if (ch == ' ') {
nextStates.set(7);
} else if (ch == '>') {
type = END_USER_DIRECTIVE2;
}
return type;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex8(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (((ch >= 0x0 && ch <= 0x8) || ((ch >= 0xe && ch <= 0x1b) || ((ch == '$') || ((ch >= '0' && ch <= '9') || ((ch >= 'A' && ch <= 'Z') || ((ch == '_') || ((ch >= 'a' && ch <= 'z') || (ch >= 0x7f && ch <= 0x9f)))))))) || (ch >= 128 && checkIntervals(NFA_MOVES_TEMPLATE_TEXT_62, ch))) {
nextStates.set(8);
} else if (ch == '\t') {
nextStates.set(7);
} else if (ch == '\n') {
nextStates.set(7);
} else if (ch == '\r') {
nextStates.set(7);
} else if (ch == ' ') {
nextStates.set(7);
} else if (ch == '.') {
nextStates.set(26);
} else if (ch == '@') {
nextStates.set(8);
} else if (ch == '>') {
type = END_USER_DIRECTIVE2;
}
return type;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex9(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (((ch >= 0x0 && ch <= 0x8) || ((ch >= 0xe && ch <= 0x1b) || ((ch == '$') || ((ch >= '0' && ch <= '9') || ((ch >= 'A' && ch <= 'Z') || ((ch == '_') || ((ch >= 'a' && ch <= 'z') || (ch >= 0x7f && ch <= 0x9f)))))))) || (ch >= 128 && checkIntervals(NFA_MOVES_TEMPLATE_TEXT_67, ch))) {
nextStates.set(9);
} else if (ch == '\t') {
nextStates.set(7);
} else if (ch == '\n') {
nextStates.set(7);
} else if (ch == '\r') {
nextStates.set(7);
} else if (ch == ' ') {
nextStates.set(7);
} else if (ch == '.') {
nextStates.set(26);
} else if (ch == '@') {
nextStates.set(9);
} else if (ch == '>') {
type = END_USER_DIRECTIVE2;
}
return type;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex10(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '{') {
return INTERPOLATE;
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex11(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if ((ch >= 0x0 && ch <= 0x8) || ((ch == 0xb || ch == '\f') || ((ch >= 0xe && ch <= 0x1f) || ((ch >= '!' && ch <= '#') || ((ch >= '%' && ch <= ';') || ((ch >= '=' && ch <= 'Z') || (ch >= '\\'))))))) {
nextStates.set(11);
return PRINTABLE_CHARS;
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex12(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '@') {
return USER_DIRECTIVE_OPEN2;
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex13(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (ch == '\t') {
nextStates.set(14);
} else if (ch == '\n') {
nextStates.set(14);
} else if (ch == '\r') {
nextStates.set(14);
} else if (ch == ' ') {
nextStates.set(14);
} else if (((ch == '$') || ((ch >= 'A' && ch <= 'Z') || ((ch == '_') || (ch >= 'a' && ch <= 'z')))) || (ch >= 128 && checkIntervals(NFA_MOVES_TEMPLATE_TEXT_31, ch))) {
nextStates.set(15);
} else if (ch == '@') {
nextStates.set(15);
} else if (ch == '>') {
type = END_DIRECTIVE2;
}
return type;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex14(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (ch == '\t') {
nextStates.set(14);
} else if (ch == '\n') {
nextStates.set(14);
} else if (ch == '\r') {
nextStates.set(14);
} else if (ch == ' ') {
nextStates.set(14);
} else if (ch == '>') {
type = END_DIRECTIVE2;
}
return type;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex15(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (((ch >= 0x0 && ch <= 0x8) || ((ch >= 0xe && ch <= 0x1b) || ((ch == '$') || ((ch >= '0' && ch <= '9') || ((ch >= 'A' && ch <= 'Z') || ((ch == '_') || ((ch >= 'a' && ch <= 'z') || (ch >= 0x7f && ch <= 0x9f)))))))) || (ch >= 128 && checkIntervals(NFA_MOVES_TEMPLATE_TEXT_33, ch))) {
nextStates.set(15);
} else if (ch == '\t') {
nextStates.set(14);
} else if (ch == '\n') {
nextStates.set(14);
} else if (ch == '\r') {
nextStates.set(14);
} else if (ch == ' ') {
nextStates.set(14);
} else if (ch == '@') {
nextStates.set(15);
} else if (ch == '>') {
type = END_DIRECTIVE2;
}
return type;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex16(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '#') {
return FTL_DIRECTIVE_OPEN1;
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex17(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (ch == '\t') {
nextStates.set(18);
} else if (ch == '\n') {
nextStates.set(18);
} else if (ch == '\r') {
nextStates.set(18);
} else if (ch == ' ') {
nextStates.set(18);
} else if (((ch == '$') || ((ch >= 'A' && ch <= 'Z') || ((ch == '_') || (ch >= 'a' && ch <= 'z')))) || (ch >= 128 && checkIntervals(NFA_MOVES_TEMPLATE_TEXT_43, ch))) {
nextStates.set(19);
} else if (ch == '@') {
nextStates.set(19);
} else if (ch == ']') {
type = END_USER_DIRECTIVE1;
}
return type;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex18(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (ch == '\t') {
nextStates.set(18);
} else if (ch == '\n') {
nextStates.set(18);
} else if (ch == '\r') {
nextStates.set(18);
} else if (ch == ' ') {
nextStates.set(18);
} else if (ch == ']') {
type = END_USER_DIRECTIVE1;
}
return type;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex19(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (((ch >= 0x0 && ch <= 0x8) || ((ch >= 0xe && ch <= 0x1b) || ((ch == '$') || ((ch >= '0' && ch <= '9') || ((ch >= 'A' && ch <= 'Z') || ((ch == '_') || ((ch >= 'a' && ch <= 'z') || (ch >= 0x7f && ch <= 0x9f)))))))) || (ch >= 128 && checkIntervals(NFA_MOVES_TEMPLATE_TEXT_45, ch))) {
nextStates.set(19);
} else if (ch == '\t') {
nextStates.set(18);
} else if (ch == '\n') {
nextStates.set(18);
} else if (ch == '\r') {
nextStates.set(18);
} else if (ch == ' ') {
nextStates.set(18);
} else if (ch == '.') {
nextStates.set(31);
} else if (ch == '@') {
nextStates.set(19);
} else if (ch == ']') {
type = END_USER_DIRECTIVE1;
}
return type;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex20(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (((ch >= 0x0 && ch <= 0x8) || ((ch >= 0xe && ch <= 0x1b) || ((ch == '$') || ((ch >= '0' && ch <= '9') || ((ch >= 'A' && ch <= 'Z') || ((ch == '_') || ((ch >= 'a' && ch <= 'z') || (ch >= 0x7f && ch <= 0x9f)))))))) || (ch >= 128 && checkIntervals(NFA_MOVES_TEMPLATE_TEXT_50, ch))) {
nextStates.set(20);
} else if (ch == '\t') {
nextStates.set(18);
} else if (ch == '\n') {
nextStates.set(18);
} else if (ch == '\r') {
nextStates.set(18);
} else if (ch == ' ') {
nextStates.set(18);
} else if (ch == '.') {
nextStates.set(31);
} else if (ch == '@') {
nextStates.set(20);
} else if (ch == ']') {
type = END_USER_DIRECTIVE1;
}
return type;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex21(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if ((ch == '\t' || ch == '\n') || ((ch == '\r') || (ch == ' '))) {
nextStates.set(21);
return WHITESPACE;
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex22(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '/') {
nextStates.set(23);
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex23(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '#') {
nextStates.set(1);
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex24(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '/') {
nextStates.set(25);
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex25(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '@') {
nextStates.set(6);
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex26(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (((ch == '$') || ((ch >= 'A' && ch <= 'Z') || ((ch == '_') || (ch >= 'a' && ch <= 'z')))) || (ch >= 128 && checkIntervals(NFA_MOVES_TEMPLATE_TEXT_65, ch))) {
nextStates.set(9);
} else if (ch == '@') {
nextStates.set(9);
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex27(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '/') {
nextStates.set(28);
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex28(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '#') {
nextStates.set(13);
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex29(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '/') {
nextStates.set(30);
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex30(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '@') {
nextStates.set(17);
}
return null;
}
private static TokenType getNfaNameTEMPLATE_TEXTIndex31(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (((ch == '$') || ((ch >= 'A' && ch <= 'Z') || ((ch == '_') || (ch >= 'a' && ch <= 'z')))) || (ch >= 128 && checkIntervals(NFA_MOVES_TEMPLATE_TEXT_48, ch))) {
nextStates.set(20);
} else if (ch == '@') {
nextStates.set(20);
}
return null;
}
private static final int[] NFA_MOVES_TEMPLATE_TEXT_19 = NFA_MOVES_TEMPLATE_TEXT_19_init();
private static int[] NFA_MOVES_TEMPLATE_TEXT_19_init() {
return new int[] {'$', '$', 'A', 'Z', '_', '_', 'a', 'z', 0xa2, 0xa5,
0xaa, 0xaa, 0xb5, 0xb5, 0xba, 0xba, 0xc0, 0xd6, 0xd8, 0xf6, 0xf8, 0x2c1,
0x2c6, 0x2d1, 0x2e0, 0x2e4, 0x2ec, 0x2ec, 0x2ee, 0x2ee, 0x370, 0x374,
0x376, 0x377, 0x37a, 0x37d, 0x37f, 0x37f, 0x386, 0x386, 0x388, 0x38a,
0x38c, 0x38c, 0x38e, 0x3a1, 0x3a3, 0x3f5, 0x3f7, 0x481, 0x48a, 0x52f,
0x531, 0x556, 0x559, 0x559, 0x560, 0x588, 0x58f, 0x58f, 0x5d0, 0x5ea,
0x5ef, 0x5f2, 0x60b, 0x60b, 0x620, 0x64a, 0x66e, 0x66f, 0x671, 0x6d3,
0x6d5, 0x6d5, 0x6e5, 0x6e6, 0x6ee, 0x6ef, 0x6fa, 0x6fc, 0x6ff, 0x6ff,
0x710, 0x710, 0x712, 0x72f, 0x74d, 0x7a5, 0x7b1, 0x7b1, 0x7ca, 0x7ea,
0x7f4, 0x7f5, 0x7fa, 0x7fa, 0x7fe, 0x815, 0x81a, 0x81a, 0x824, 0x824,
0x828, 0x828, 0x840, 0x858, 0x860, 0x86a, 0x870, 0x887, 0x889, 0x88e,
0x8a0, 0x8c9, 0x904, 0x939, 0x93d, 0x93d, 0x950, 0x950, 0x958, 0x961,
0x971, 0x980, 0x985, 0x98c, 0x98f, 0x990, 0x993, 0x9a8, 0x9aa, 0x9b0,
0x9b2, 0x9b2, 0x9b6, 0x9b9, 0x9bd, 0x9bd, 0x9ce, 0x9ce, 0x9dc, 0x9dd,
0x9df, 0x9e1, 0x9f0, 0x9f3, 0x9fb, 0x9fc, 0xa05, 0xa0a, 0xa0f, 0xa10,
0xa13, 0xa28, 0xa2a, 0xa30, 0xa32, 0xa33, 0xa35, 0xa36, 0xa38, 0xa39,
0xa59, 0xa5c, 0xa5e, 0xa5e, 0xa72, 0xa74, 0xa85, 0xa8d, 0xa8f, 0xa91,
0xa93, 0xaa8, 0xaaa, 0xab0, 0xab2, 0xab3, 0xab5, 0xab9, 0xabd, 0xabd,
0xad0, 0xad0, 0xae0, 0xae1, 0xaf1, 0xaf1, 0xaf9, 0xaf9, 0xb05, 0xb0c,
0xb0f, 0xb10, 0xb13, 0xb28, 0xb2a, 0xb30, 0xb32, 0xb33, 0xb35, 0xb39,
0xb3d, 0xb3d, 0xb5c, 0xb5d, 0xb5f, 0xb61, 0xb71, 0xb71, 0xb83, 0xb83,
0xb85, 0xb8a, 0xb8e, 0xb90, 0xb92, 0xb95, 0xb99, 0xb9a, 0xb9c, 0xb9c,
0xb9e, 0xb9f, 0xba3, 0xba4, 0xba8, 0xbaa, 0xbae, 0xbb9, 0xbd0, 0xbd0,
0xbf9, 0xbf9, 0xc05, 0xc0c, 0xc0e, 0xc10, 0xc12, 0xc28, 0xc2a, 0xc39,
0xc3d, 0xc3d, 0xc58, 0xc5a, 0xc5d, 0xc5d, 0xc60, 0xc61, 0xc80, 0xc80,
0xc85, 0xc8c, 0xc8e, 0xc90, 0xc92, 0xca8, 0xcaa, 0xcb3, 0xcb5, 0xcb9,
0xcbd, 0xcbd, 0xcdd, 0xcde, 0xce0, 0xce1, 0xcf1, 0xcf2, 0xd04, 0xd0c,
0xd0e, 0xd10, 0xd12, 0xd3a, 0xd3d, 0xd3d, 0xd4e, 0xd4e, 0xd54, 0xd56,
0xd5f, 0xd61, 0xd7a, 0xd7f, 0xd85, 0xd96, 0xd9a, 0xdb1, 0xdb3, 0xdbb,
0xdbd, 0xdbd, 0xdc0, 0xdc6, 0xe01, 0xe30, 0xe32, 0xe33, 0xe3f, 0xe46,
0xe81, 0xe82, 0xe84, 0xe84, 0xe86, 0xe8a, 0xe8c, 0xea3, 0xea5, 0xea5,
0xea7, 0xeb0, 0xeb2, 0xeb3, 0xebd, 0xebd, 0xec0, 0xec4, 0xec6, 0xec6,
0xedc, 0xedf, 0xf00, 0xf00, 0xf40, 0xf47, 0xf49, 0xf6c, 0xf88, 0xf8c,
0x1000, 0x102a, 0x103f, 0x103f, 0x1050, 0x1055, 0x105a, 0x105d, 0x1061,
0x1061, 0x1065, 0x1066, 0x106e, 0x1070, 0x1075, 0x1081, 0x108e, 0x108e,
0x10a0, 0x10c5, 0x10c7, 0x10c7, 0x10cd, 0x10cd, 0x10d0, 0x10fa, 0x10fc,
0x1248, 0x124a, 0x124d, 0x1250, 0x1256, 0x1258, 0x1258, 0x125a, 0x125d,
0x1260, 0x1288, 0x128a, 0x128d, 0x1290, 0x12b0, 0x12b2, 0x12b5, 0x12b8,
0x12be, 0x12c0, 0x12c0, 0x12c2, 0x12c5, 0x12c8, 0x12d6, 0x12d8, 0x1310,
0x1312, 0x1315, 0x1318, 0x135a, 0x1380, 0x138f, 0x13a0, 0x13f5, 0x13f8,
0x13fd, 0x1401, 0x166c, 0x166f, 0x167f, 0x1681, 0x169a, 0x16a0, 0x16ea,
0x16ee, 0x16f8, 0x1700, 0x1711, 0x171f, 0x1731, 0x1740, 0x1751, 0x1760,
0x176c, 0x176e, 0x1770, 0x1780, 0x17b3, 0x17d7, 0x17d7, 0x17db, 0x17dc,
0x1820, 0x1878, 0x1880, 0x1884, 0x1887, 0x18a8, 0x18aa, 0x18aa, 0x18b0,
0x18f5, 0x1900, 0x191e, 0x1950, 0x196d, 0x1970, 0x1974, 0x1980, 0x19ab,
0x19b0, 0x19c9, 0x1a00, 0x1a16, 0x1a20, 0x1a54, 0x1aa7, 0x1aa7, 0x1b05,
0x1b33, 0x1b45, 0x1b4c, 0x1b83, 0x1ba0, 0x1bae, 0x1baf, 0x1bba, 0x1be5,
0x1c00, 0x1c23, 0x1c4d, 0x1c4f, 0x1c5a, 0x1c7d, 0x1c80, 0x1c88, 0x1c90,
0x1cba, 0x1cbd, 0x1cbf, 0x1ce9, 0x1cec, 0x1cee, 0x1cf3, 0x1cf5, 0x1cf6,
0x1cfa, 0x1cfa, 0x1d00, 0x1dbf, 0x1e00, 0x1f15, 0x1f18, 0x1f1d, 0x1f20,
0x1f45, 0x1f48, 0x1f4d, 0x1f50, 0x1f57, 0x1f59, 0x1f59, 0x1f5b, 0x1f5b,
0x1f5d, 0x1f5d, 0x1f5f, 0x1f7d, 0x1f80, 0x1fb4, 0x1fb6, 0x1fbc, 0x1fbe,
0x1fbe, 0x1fc2, 0x1fc4, 0x1fc6, 0x1fcc, 0x1fd0, 0x1fd3, 0x1fd6, 0x1fdb,
0x1fe0, 0x1fec, 0x1ff2, 0x1ff4, 0x1ff6, 0x1ffc, 0x203f, 0x2040, 0x2054,
0x2054, 0x2071, 0x2071, 0x207f, 0x207f, 0x2090, 0x209c, 0x20a0, 0x20c0,
0x2102, 0x2102, 0x2107, 0x2107, 0x210a, 0x2113, 0x2115, 0x2115, 0x2119,
0x211d, 0x2124, 0x2124, 0x2126, 0x2126, 0x2128, 0x2128, 0x212a, 0x212d,
0x212f, 0x2139, 0x213c, 0x213f, 0x2145, 0x2149, 0x214e, 0x214e, 0x2160,
0x2188, 0x2c00, 0x2ce4, 0x2ceb, 0x2cee, 0x2cf2, 0x2cf3, 0x2d00, 0x2d25,
0x2d27, 0x2d27, 0x2d2d, 0x2d2d, 0x2d30, 0x2d67, 0x2d6f, 0x2d6f, 0x2d80,
0x2d96, 0x2da0, 0x2da6, 0x2da8, 0x2dae, 0x2db0, 0x2db6, 0x2db8, 0x2dbe,
0x2dc0, 0x2dc6, 0x2dc8, 0x2dce, 0x2dd0, 0x2dd6, 0x2dd8, 0x2dde, 0x2e2f,
0x2e2f, 0x3005, 0x3007, 0x3021, 0x3029, 0x3031, 0x3035, 0x3038, 0x303c,
0x3041, 0x3096, 0x309d, 0x309f, 0x30a1, 0x30fa, 0x30fc, 0x30ff, 0x3105,
0x312f, 0x3131, 0x318e, 0x31a0, 0x31bf, 0x31f0, 0x31ff, 0x3400, 0x4dbf,
0x4e00, 0xa48c, 0xa4d0, 0xa4fd, 0xa500, 0xa60c, 0xa610, 0xa61f, 0xa62a,
0xa62b, 0xa640, 0xa66e, 0xa67f, 0xa69d, 0xa6a0, 0xa6ef, 0xa717, 0xa71f,
0xa722, 0xa788, 0xa78b, 0xa7ca, 0xa7d0, 0xa7d1, 0xa7d3, 0xa7d3, 0xa7d5,
0xa7d9, 0xa7f2, 0xa801, 0xa803, 0xa805, 0xa807, 0xa80a, 0xa80c, 0xa822,
0xa838, 0xa838, 0xa840, 0xa873, 0xa882, 0xa8b3, 0xa8f2, 0xa8f7, 0xa8fb,
0xa8fb, 0xa8fd, 0xa8fe, 0xa90a, 0xa925, 0xa930, 0xa946, 0xa960, 0xa97c,
0xa984, 0xa9b2, 0xa9cf, 0xa9cf, 0xa9e0, 0xa9e4, 0xa9e6, 0xa9ef, 0xa9fa,
0xa9fe, 0xaa00, 0xaa28, 0xaa40, 0xaa42, 0xaa44, 0xaa4b, 0xaa60, 0xaa76,
0xaa7a, 0xaa7a, 0xaa7e, 0xaaaf, 0xaab1, 0xaab1, 0xaab5, 0xaab6, 0xaab9,
0xaabd, 0xaac0, 0xaac0, 0xaac2, 0xaac2, 0xaadb, 0xaadd, 0xaae0, 0xaaea,
0xaaf2, 0xaaf4, 0xab01, 0xab06, 0xab09, 0xab0e, 0xab11, 0xab16, 0xab20,
0xab26, 0xab28, 0xab2e, 0xab30, 0xab5a, 0xab5c, 0xab69, 0xab70, 0xabe2,
0xac00, 0xd7a3, 0xd7b0, 0xd7c6, 0xd7cb, 0xd7fb, 0xf900, 0xfa6d, 0xfa70,
0xfad9, 0xfb00, 0xfb06, 0xfb13, 0xfb17, 0xfb1d, 0xfb1d, 0xfb1f, 0xfb28,
0xfb2a, 0xfb36, 0xfb38, 0xfb3c, 0xfb3e, 0xfb3e, 0xfb40, 0xfb41, 0xfb43,
0xfb44, 0xfb46, 0xfbb1, 0xfbd3, 0xfd3d, 0xfd50, 0xfd8f, 0xfd92, 0xfdc7,
0xfdf0, 0xfdfc, 0xfe33, 0xfe34, 0xfe4d, 0xfe4f, 0xfe69, 0xfe69, 0xfe70,
0xfe74, 0xfe76, 0xfefc, 0xff04, 0xff04, 0xff21, 0xff3a, 0xff3f, 0xff3f,
0xff41, 0xff5a, 0xff66, 0xffbe, 0xffc2, 0xffc7, 0xffca, 0xffcf, 0xffd2,
0xffd7, 0xffda, 0xffdc, 0xffe0, 0xffe1, 0xffe5, 0xffe6, 0x10000, 0x1000b,
0x1000d, 0x10026, 0x10028, 0x1003a, 0x1003c, 0x1003d, 0x1003f, 0x1004d,
0x10050, 0x1005d, 0x10080, 0x100fa, 0x10140, 0x10174, 0x10280, 0x1029c,
0x102a0, 0x102d0, 0x10300, 0x1031f, 0x1032d, 0x1034a, 0x10350, 0x10375,
0x10380, 0x1039d, 0x103a0, 0x103c3, 0x103c8, 0x103cf, 0x103d1, 0x103d5,
0x10400, 0x1049d, 0x104b0, 0x104d3, 0x104d8, 0x104fb, 0x10500, 0x10527,
0x10530, 0x10563, 0x10570, 0x1057a, 0x1057c, 0x1058a, 0x1058c, 0x10592,
0x10594, 0x10595, 0x10597, 0x105a1, 0x105a3, 0x105b1, 0x105b3, 0x105b9,
0x105bb, 0x105bc, 0x10600, 0x10736, 0x10740, 0x10755, 0x10760, 0x10767,
0x10780, 0x10785, 0x10787, 0x107b0, 0x107b2, 0x107ba, 0x10800, 0x10805,
0x10808, 0x10808, 0x1080a, 0x10835, 0x10837, 0x10838, 0x1083c, 0x1083c,
0x1083f, 0x10855, 0x10860, 0x10876, 0x10880, 0x1089e, 0x108e0, 0x108f2,
0x108f4, 0x108f5, 0x10900, 0x10915, 0x10920, 0x10939, 0x10980, 0x109b7,
0x109be, 0x109bf, 0x10a00, 0x10a00, 0x10a10, 0x10a13, 0x10a15, 0x10a17,
0x10a19, 0x10a35, 0x10a60, 0x10a7c, 0x10a80, 0x10a9c, 0x10ac0, 0x10ac7,
0x10ac9, 0x10ae4, 0x10b00, 0x10b35, 0x10b40, 0x10b55, 0x10b60, 0x10b72,
0x10b80, 0x10b91, 0x10c00, 0x10c48, 0x10c80, 0x10cb2, 0x10cc0, 0x10cf2,
0x10d00, 0x10d23, 0x10e80, 0x10ea9, 0x10eb0, 0x10eb1, 0x10f00, 0x10f1c,
0x10f27, 0x10f27, 0x10f30, 0x10f45, 0x10f70, 0x10f81, 0x10fb0, 0x10fc4,
0x10fe0, 0x10ff6, 0x11003, 0x11037, 0x11071, 0x11072, 0x11075, 0x11075,
0x11083, 0x110af, 0x110d0, 0x110e8, 0x11103, 0x11126, 0x11144, 0x11144,
0x11147, 0x11147, 0x11150, 0x11172, 0x11176, 0x11176, 0x11183, 0x111b2,
0x111c1, 0x111c4, 0x111da, 0x111da, 0x111dc, 0x111dc, 0x11200, 0x11211,
0x11213, 0x1122b, 0x11280, 0x11286, 0x11288, 0x11288, 0x1128a, 0x1128d,
0x1128f, 0x1129d, 0x1129f, 0x112a8, 0x112b0, 0x112de, 0x11305, 0x1130c,
0x1130f, 0x11310, 0x11313, 0x11328, 0x1132a, 0x11330, 0x11332, 0x11333,
0x11335, 0x11339, 0x1133d, 0x1133d, 0x11350, 0x11350, 0x1135d, 0x11361,
0x11400, 0x11434, 0x11447, 0x1144a, 0x1145f, 0x11461, 0x11480, 0x114af,
0x114c4, 0x114c5, 0x114c7, 0x114c7, 0x11580, 0x115ae, 0x115d8, 0x115db,
0x11600, 0x1162f, 0x11644, 0x11644, 0x11680, 0x116aa, 0x116b8, 0x116b8,
0x11700, 0x1171a, 0x11740, 0x11746, 0x11800, 0x1182b, 0x118a0, 0x118df,
0x118ff, 0x11906, 0x11909, 0x11909, 0x1190c, 0x11913, 0x11915, 0x11916,
0x11918, 0x1192f, 0x1193f, 0x1193f, 0x11941, 0x11941, 0x119a0, 0x119a7,
0x119aa, 0x119d0, 0x119e1, 0x119e1, 0x119e3, 0x119e3, 0x11a00, 0x11a00,
0x11a0b, 0x11a32, 0x11a3a, 0x11a3a, 0x11a50, 0x11a50, 0x11a5c, 0x11a89,
0x11a9d, 0x11a9d, 0x11ab0, 0x11af8, 0x11c00, 0x11c08, 0x11c0a, 0x11c2e,
0x11c40, 0x11c40, 0x11c72, 0x11c8f, 0x11d00, 0x11d06, 0x11d08, 0x11d09,
0x11d0b, 0x11d30, 0x11d46, 0x11d46, 0x11d60, 0x11d65, 0x11d67, 0x11d68,
0x11d6a, 0x11d89, 0x11d98, 0x11d98, 0x11ee0, 0x11ef2, 0x11fb0, 0x11fb0,
0x11fdd, 0x11fe0, 0x12000, 0x12399, 0x12400, 0x1246e, 0x12480, 0x12543,
0x12f90, 0x12ff0, 0x13000, 0x1342e, 0x14400, 0x14646, 0x16800, 0x16a38,
0x16a40, 0x16a5e, 0x16a70, 0x16abe, 0x16ad0, 0x16aed, 0x16b00, 0x16b2f,
0x16b40, 0x16b43, 0x16b63, 0x16b77, 0x16b7d, 0x16b8f, 0x16e40, 0x16e7f,
0x16f00, 0x16f4a, 0x16f50, 0x16f50, 0x16f93, 0x16f9f, 0x16fe0, 0x16fe1,
0x16fe3, 0x16fe3, 0x17000, 0x187f7, 0x18800, 0x18cd5, 0x18d00, 0x18d08,
0x1aff0, 0x1aff3, 0x1aff5, 0x1affb, 0x1affd, 0x1affe, 0x1b000, 0x1b122,
0x1b150, 0x1b152, 0x1b164, 0x1b167, 0x1b170, 0x1b2fb, 0x1bc00, 0x1bc6a,
0x1bc70, 0x1bc7c, 0x1bc80, 0x1bc88, 0x1bc90, 0x1bc99, 0x1d400, 0x1d454,
0x1d456, 0x1d49c, 0x1d49e, 0x1d49f, 0x1d4a2, 0x1d4a2, 0x1d4a5, 0x1d4a6,
0x1d4a9, 0x1d4ac, 0x1d4ae, 0x1d4b9, 0x1d4bb, 0x1d4bb, 0x1d4bd, 0x1d4c3,
0x1d4c5, 0x1d505, 0x1d507, 0x1d50a, 0x1d50d, 0x1d514, 0x1d516, 0x1d51c,
0x1d51e, 0x1d539, 0x1d53b, 0x1d53e, 0x1d540, 0x1d544, 0x1d546, 0x1d546,
0x1d54a, 0x1d550, 0x1d552, 0x1d6a5, 0x1d6a8, 0x1d6c0, 0x1d6c2, 0x1d6da,
0x1d6dc, 0x1d6fa, 0x1d6fc, 0x1d714, 0x1d716, 0x1d734, 0x1d736, 0x1d74e,
0x1d750, 0x1d76e, 0x1d770, 0x1d788, 0x1d78a, 0x1d7a8, 0x1d7aa, 0x1d7c2,
0x1d7c4, 0x1d7cb, 0x1df00, 0x1df1e, 0x1e100, 0x1e12c, 0x1e137, 0x1e13d,
0x1e14e, 0x1e14e, 0x1e290, 0x1e2ad, 0x1e2c0, 0x1e2eb, 0x1e2ff, 0x1e2ff,
0x1e7e0, 0x1e7e6, 0x1e7e8, 0x1e7eb, 0x1e7ed, 0x1e7ee, 0x1e7f0, 0x1e7fe,
0x1e800, 0x1e8c4, 0x1e900, 0x1e943, 0x1e94b, 0x1e94b, 0x1ecb0, 0x1ecb0,
0x1ee00, 0x1ee03, 0x1ee05, 0x1ee1f, 0x1ee21, 0x1ee22, 0x1ee24, 0x1ee24,
0x1ee27, 0x1ee27, 0x1ee29, 0x1ee32, 0x1ee34, 0x1ee37, 0x1ee39, 0x1ee39,
0x1ee3b, 0x1ee3b, 0x1ee42, 0x1ee42, 0x1ee47, 0x1ee47, 0x1ee49, 0x1ee49,
0x1ee4b, 0x1ee4b, 0x1ee4d, 0x1ee4f, 0x1ee51, 0x1ee52, 0x1ee54, 0x1ee54,
0x1ee57, 0x1ee57, 0x1ee59, 0x1ee59, 0x1ee5b, 0x1ee5b, 0x1ee5d, 0x1ee5d,
0x1ee5f, 0x1ee5f, 0x1ee61, 0x1ee62, 0x1ee64, 0x1ee64, 0x1ee67, 0x1ee6a,
0x1ee6c, 0x1ee72, 0x1ee74, 0x1ee77, 0x1ee79, 0x1ee7c, 0x1ee7e, 0x1ee7e,
0x1ee80, 0x1ee89, 0x1ee8b, 0x1ee9b, 0x1eea1, 0x1eea3, 0x1eea5, 0x1eea9,
0x1eeab, 0x1eebb, 0x20000, 0x2a6df, 0x2a700, 0x2b738, 0x2b740, 0x2b81d,
0x2b820, 0x2cea1, 0x2ceb0, 0x2ebe0, 0x2f800, 0x2fa1d, 0x30000, 0x3134a};
}
private static final int[] NFA_MOVES_TEMPLATE_TEXT_21 = NFA_MOVES_TEMPLATE_TEXT_21_init();
private static int[] NFA_MOVES_TEMPLATE_TEXT_21_init() {
return new int[] {0x0, 0x8, 0xe, 0x1b, '$', '$', '0', '9', 'A', 'Z', '_',
'_', 'a', 'z', 0x7f, 0x9f, 0xa2, 0xa5, 0xaa, 0xaa, 0xad, 0xad, 0xb5, 0xb5,
0xba, 0xba, 0xc0, 0xd6, 0xd8, 0xf6, 0xf8, 0x2c1, 0x2c6, 0x2d1, 0x2e0,
0x2e4, 0x2ec, 0x2ec, 0x2ee, 0x2ee, 0x300, 0x374, 0x376, 0x377, 0x37a,
0x37d, 0x37f, 0x37f, 0x386, 0x386, 0x388, 0x38a, 0x38c, 0x38c, 0x38e,
0x3a1, 0x3a3, 0x3f5, 0x3f7, 0x481, 0x483, 0x487, 0x48a, 0x52f, 0x531,
0x556, 0x559, 0x559, 0x560, 0x588, 0x58f, 0x58f, 0x591, 0x5bd, 0x5bf,
0x5bf, 0x5c1, 0x5c2, 0x5c4, 0x5c5, 0x5c7, 0x5c7, 0x5d0, 0x5ea, 0x5ef,
0x5f2, 0x600, 0x605, 0x60b, 0x60b, 0x610, 0x61a, 0x61c, 0x61c, 0x620,
0x669, 0x66e, 0x6d3, 0x6d5, 0x6dd, 0x6df, 0x6e8, 0x6ea, 0x6fc, 0x6ff,
0x6ff, 0x70f, 0x74a, 0x74d, 0x7b1, 0x7c0, 0x7f5, 0x7fa, 0x7fa, 0x7fd,
0x82d, 0x840, 0x85b, 0x860, 0x86a, 0x870, 0x887, 0x889, 0x88e, 0x890,
0x891, 0x898, 0x963, 0x966, 0x96f, 0x971, 0x983, 0x985, 0x98c, 0x98f,
0x990, 0x993, 0x9a8, 0x9aa, 0x9b0, 0x9b2, 0x9b2, 0x9b6, 0x9b9, 0x9bc,
0x9c4, 0x9c7, 0x9c8, 0x9cb, 0x9ce, 0x9d7, 0x9d7, 0x9dc, 0x9dd, 0x9df,
0x9e3, 0x9e6, 0x9f3, 0x9fb, 0x9fc, 0x9fe, 0x9fe, 0xa01, 0xa03, 0xa05,
0xa0a, 0xa0f, 0xa10, 0xa13, 0xa28, 0xa2a, 0xa30, 0xa32, 0xa33, 0xa35,
0xa36, 0xa38, 0xa39, 0xa3c, 0xa3c, 0xa3e, 0xa42, 0xa47, 0xa48, 0xa4b,
0xa4d, 0xa51, 0xa51, 0xa59, 0xa5c, 0xa5e, 0xa5e, 0xa66, 0xa75, 0xa81,
0xa83, 0xa85, 0xa8d, 0xa8f, 0xa91, 0xa93, 0xaa8, 0xaaa, 0xab0, 0xab2,
0xab3, 0xab5, 0xab9, 0xabc, 0xac5, 0xac7, 0xac9, 0xacb, 0xacd, 0xad0,
0xad0, 0xae0, 0xae3, 0xae6, 0xaef, 0xaf1, 0xaf1, 0xaf9, 0xaff, 0xb01,
0xb03, 0xb05, 0xb0c, 0xb0f, 0xb10, 0xb13, 0xb28, 0xb2a, 0xb30, 0xb32,
0xb33, 0xb35, 0xb39, 0xb3c, 0xb44, 0xb47, 0xb48, 0xb4b, 0xb4d, 0xb55,
0xb57, 0xb5c, 0xb5d, 0xb5f, 0xb63, 0xb66, 0xb6f, 0xb71, 0xb71, 0xb82,
0xb83, 0xb85, 0xb8a, 0xb8e, 0xb90, 0xb92, 0xb95, 0xb99, 0xb9a, 0xb9c,
0xb9c, 0xb9e, 0xb9f, 0xba3, 0xba4, 0xba8, 0xbaa, 0xbae, 0xbb9, 0xbbe,
0xbc2, 0xbc6, 0xbc8, 0xbca, 0xbcd, 0xbd0, 0xbd0, 0xbd7, 0xbd7, 0xbe6,
0xbef, 0xbf9, 0xbf9, 0xc00, 0xc0c, 0xc0e, 0xc10, 0xc12, 0xc28, 0xc2a,
0xc39, 0xc3c, 0xc44, 0xc46, 0xc48, 0xc4a, 0xc4d, 0xc55, 0xc56, 0xc58,
0xc5a, 0xc5d, 0xc5d, 0xc60, 0xc63, 0xc66, 0xc6f, 0xc80, 0xc83, 0xc85,
0xc8c, 0xc8e, 0xc90, 0xc92, 0xca8, 0xcaa, 0xcb3, 0xcb5, 0xcb9, 0xcbc,
0xcc4, 0xcc6, 0xcc8, 0xcca, 0xccd, 0xcd5, 0xcd6, 0xcdd, 0xcde, 0xce0,
0xce3, 0xce6, 0xcef, 0xcf1, 0xcf2, 0xd00, 0xd0c, 0xd0e, 0xd10, 0xd12,
0xd44, 0xd46, 0xd48, 0xd4a, 0xd4e, 0xd54, 0xd57, 0xd5f, 0xd63, 0xd66,
0xd6f, 0xd7a, 0xd7f, 0xd81, 0xd83, 0xd85, 0xd96, 0xd9a, 0xdb1, 0xdb3,
0xdbb, 0xdbd, 0xdbd, 0xdc0, 0xdc6, 0xdca, 0xdca, 0xdcf, 0xdd4, 0xdd6,
0xdd6, 0xdd8, 0xddf, 0xde6, 0xdef, 0xdf2, 0xdf3, 0xe01, 0xe3a, 0xe3f,
0xe4e, 0xe50, 0xe59, 0xe81, 0xe82, 0xe84, 0xe84, 0xe86, 0xe8a, 0xe8c,
0xea3, 0xea5, 0xea5, 0xea7, 0xebd, 0xec0, 0xec4, 0xec6, 0xec6, 0xec8,
0xecd, 0xed0, 0xed9, 0xedc, 0xedf, 0xf00, 0xf00, 0xf18, 0xf19, 0xf20,
0xf29, 0xf35, 0xf35, 0xf37, 0xf37, 0xf39, 0xf39, 0xf3e, 0xf47, 0xf49,
0xf6c, 0xf71, 0xf84, 0xf86, 0xf97, 0xf99, 0xfbc, 0xfc6, 0xfc6, 0x1000,
0x1049, 0x1050, 0x109d, 0x10a0, 0x10c5, 0x10c7, 0x10c7, 0x10cd, 0x10cd,
0x10d0, 0x10fa, 0x10fc, 0x1248, 0x124a, 0x124d, 0x1250, 0x1256, 0x1258,
0x1258, 0x125a, 0x125d, 0x1260, 0x1288, 0x128a, 0x128d, 0x1290, 0x12b0,
0x12b2, 0x12b5, 0x12b8, 0x12be, 0x12c0, 0x12c0, 0x12c2, 0x12c5, 0x12c8,
0x12d6, 0x12d8, 0x1310, 0x1312, 0x1315, 0x1318, 0x135a, 0x135d, 0x135f,
0x1380, 0x138f, 0x13a0, 0x13f5, 0x13f8, 0x13fd, 0x1401, 0x166c, 0x166f,
0x167f, 0x1681, 0x169a, 0x16a0, 0x16ea, 0x16ee, 0x16f8, 0x1700, 0x1715,
0x171f, 0x1734, 0x1740, 0x1753, 0x1760, 0x176c, 0x176e, 0x1770, 0x1772,
0x1773, 0x1780, 0x17d3, 0x17d7, 0x17d7, 0x17db, 0x17dd, 0x17e0, 0x17e9,
0x180b, 0x1819, 0x1820, 0x1878, 0x1880, 0x18aa, 0x18b0, 0x18f5, 0x1900,
0x191e, 0x1920, 0x192b, 0x1930, 0x193b, 0x1946, 0x196d, 0x1970, 0x1974,
0x1980, 0x19ab, 0x19b0, 0x19c9, 0x19d0, 0x19d9, 0x1a00, 0x1a1b, 0x1a20,
0x1a5e, 0x1a60, 0x1a7c, 0x1a7f, 0x1a89, 0x1a90, 0x1a99, 0x1aa7, 0x1aa7,
0x1ab0, 0x1abd, 0x1abf, 0x1ace, 0x1b00, 0x1b4c, 0x1b50, 0x1b59, 0x1b6b,
0x1b73, 0x1b80, 0x1bf3, 0x1c00, 0x1c37, 0x1c40, 0x1c49, 0x1c4d, 0x1c7d,
0x1c80, 0x1c88, 0x1c90, 0x1cba, 0x1cbd, 0x1cbf, 0x1cd0, 0x1cd2, 0x1cd4,
0x1cfa, 0x1d00, 0x1f15, 0x1f18, 0x1f1d, 0x1f20, 0x1f45, 0x1f48, 0x1f4d,
0x1f50, 0x1f57, 0x1f59, 0x1f59, 0x1f5b, 0x1f5b, 0x1f5d, 0x1f5d, 0x1f5f,
0x1f7d, 0x1f80, 0x1fb4, 0x1fb6, 0x1fbc, 0x1fbe, 0x1fbe, 0x1fc2, 0x1fc4,
0x1fc6, 0x1fcc, 0x1fd0, 0x1fd3, 0x1fd6, 0x1fdb, 0x1fe0, 0x1fec, 0x1ff2,
0x1ff4, 0x1ff6, 0x1ffc, 0x200b, 0x200f, 0x202a, 0x202e, 0x203f, 0x2040,
0x2054, 0x2054, 0x2060, 0x2064, 0x2066, 0x206f, 0x2071, 0x2071, 0x207f,
0x207f, 0x2090, 0x209c, 0x20a0, 0x20c0, 0x20d0, 0x20dc, 0x20e1, 0x20e1,
0x20e5, 0x20f0, 0x2102, 0x2102, 0x2107, 0x2107, 0x210a, 0x2113, 0x2115,
0x2115, 0x2119, 0x211d, 0x2124, 0x2124, 0x2126, 0x2126, 0x2128, 0x2128,
0x212a, 0x212d, 0x212f, 0x2139, 0x213c, 0x213f, 0x2145, 0x2149, 0x214e,
0x214e, 0x2160, 0x2188, 0x2c00, 0x2ce4, 0x2ceb, 0x2cf3, 0x2d00, 0x2d25,
0x2d27, 0x2d27, 0x2d2d, 0x2d2d, 0x2d30, 0x2d67, 0x2d6f, 0x2d6f, 0x2d7f,
0x2d96, 0x2da0, 0x2da6, 0x2da8, 0x2dae, 0x2db0, 0x2db6, 0x2db8, 0x2dbe,
0x2dc0, 0x2dc6, 0x2dc8, 0x2dce, 0x2dd0, 0x2dd6, 0x2dd8, 0x2dde, 0x2de0,
0x2dff, 0x2e2f, 0x2e2f, 0x3005, 0x3007, 0x3021, 0x302f, 0x3031, 0x3035,
0x3038, 0x303c, 0x3041, 0x3096, 0x3099, 0x309a, 0x309d, 0x309f, 0x30a1,
0x30fa, 0x30fc, 0x30ff, 0x3105, 0x312f, 0x3131, 0x318e, 0x31a0, 0x31bf,
0x31f0, 0x31ff, 0x3400, 0x4dbf, 0x4e00, 0xa48c, 0xa4d0, 0xa4fd, 0xa500,
0xa60c, 0xa610, 0xa62b, 0xa640, 0xa66f, 0xa674, 0xa67d, 0xa67f, 0xa6f1,
0xa717, 0xa71f, 0xa722, 0xa788, 0xa78b, 0xa7ca, 0xa7d0, 0xa7d1, 0xa7d3,
0xa7d3, 0xa7d5, 0xa7d9, 0xa7f2, 0xa827, 0xa82c, 0xa82c, 0xa838, 0xa838,
0xa840, 0xa873, 0xa880, 0xa8c5, 0xa8d0, 0xa8d9, 0xa8e0, 0xa8f7, 0xa8fb,
0xa8fb, 0xa8fd, 0xa92d, 0xa930, 0xa953, 0xa960, 0xa97c, 0xa980, 0xa9c0,
0xa9cf, 0xa9d9, 0xa9e0, 0xa9fe, 0xaa00, 0xaa36, 0xaa40, 0xaa4d, 0xaa50,
0xaa59, 0xaa60, 0xaa76, 0xaa7a, 0xaac2, 0xaadb, 0xaadd, 0xaae0, 0xaaef,
0xaaf2, 0xaaf6, 0xab01, 0xab06, 0xab09, 0xab0e, 0xab11, 0xab16, 0xab20,
0xab26, 0xab28, 0xab2e, 0xab30, 0xab5a, 0xab5c, 0xab69, 0xab70, 0xabea,
0xabec, 0xabed, 0xabf0, 0xabf9, 0xac00, 0xd7a3, 0xd7b0, 0xd7c6, 0xd7cb,
0xd7fb, 0xf900, 0xfa6d, 0xfa70, 0xfad9, 0xfb00, 0xfb06, 0xfb13, 0xfb17,
0xfb1d, 0xfb28, 0xfb2a, 0xfb36, 0xfb38, 0xfb3c, 0xfb3e, 0xfb3e, 0xfb40,
0xfb41, 0xfb43, 0xfb44, 0xfb46, 0xfbb1, 0xfbd3, 0xfd3d, 0xfd50, 0xfd8f,
0xfd92, 0xfdc7, 0xfdf0, 0xfdfc, 0xfe00, 0xfe0f, 0xfe20, 0xfe2f, 0xfe33,
0xfe34, 0xfe4d, 0xfe4f, 0xfe69, 0xfe69, 0xfe70, 0xfe74, 0xfe76, 0xfefc,
0xfeff, 0xfeff, 0xff04, 0xff04, 0xff10, 0xff19, 0xff21, 0xff3a, 0xff3f,
0xff3f, 0xff41, 0xff5a, 0xff66, 0xffbe, 0xffc2, 0xffc7, 0xffca, 0xffcf,
0xffd2, 0xffd7, 0xffda, 0xffdc, 0xffe0, 0xffe1, 0xffe5, 0xffe6, 0xfff9,
0xfffb, 0x10000, 0x1000b, 0x1000d, 0x10026, 0x10028, 0x1003a, 0x1003c,
0x1003d, 0x1003f, 0x1004d, 0x10050, 0x1005d, 0x10080, 0x100fa, 0x10140,
0x10174, 0x101fd, 0x101fd, 0x10280, 0x1029c, 0x102a0, 0x102d0, 0x102e0,
0x102e0, 0x10300, 0x1031f, 0x1032d, 0x1034a, 0x10350, 0x1037a, 0x10380,
0x1039d, 0x103a0, 0x103c3, 0x103c8, 0x103cf, 0x103d1, 0x103d5, 0x10400,
0x1049d, 0x104a0, 0x104a9, 0x104b0, 0x104d3, 0x104d8, 0x104fb, 0x10500,
0x10527, 0x10530, 0x10563, 0x10570, 0x1057a, 0x1057c, 0x1058a, 0x1058c,
0x10592, 0x10594, 0x10595, 0x10597, 0x105a1, 0x105a3, 0x105b1, 0x105b3,
0x105b9, 0x105bb, 0x105bc, 0x10600, 0x10736, 0x10740, 0x10755, 0x10760,
0x10767, 0x10780, 0x10785, 0x10787, 0x107b0, 0x107b2, 0x107ba, 0x10800,
0x10805, 0x10808, 0x10808, 0x1080a, 0x10835, 0x10837, 0x10838, 0x1083c,
0x1083c, 0x1083f, 0x10855, 0x10860, 0x10876, 0x10880, 0x1089e, 0x108e0,
0x108f2, 0x108f4, 0x108f5, 0x10900, 0x10915, 0x10920, 0x10939, 0x10980,
0x109b7, 0x109be, 0x109bf, 0x10a00, 0x10a03, 0x10a05, 0x10a06, 0x10a0c,
0x10a13, 0x10a15, 0x10a17, 0x10a19, 0x10a35, 0x10a38, 0x10a3a, 0x10a3f,
0x10a3f, 0x10a60, 0x10a7c, 0x10a80, 0x10a9c, 0x10ac0, 0x10ac7, 0x10ac9,
0x10ae6, 0x10b00, 0x10b35, 0x10b40, 0x10b55, 0x10b60, 0x10b72, 0x10b80,
0x10b91, 0x10c00, 0x10c48, 0x10c80, 0x10cb2, 0x10cc0, 0x10cf2, 0x10d00,
0x10d27, 0x10d30, 0x10d39, 0x10e80, 0x10ea9, 0x10eab, 0x10eac, 0x10eb0,
0x10eb1, 0x10f00, 0x10f1c, 0x10f27, 0x10f27, 0x10f30, 0x10f50, 0x10f70,
0x10f85, 0x10fb0, 0x10fc4, 0x10fe0, 0x10ff6, 0x11000, 0x11046, 0x11066,
0x11075, 0x1107f, 0x110ba, 0x110bd, 0x110bd, 0x110c2, 0x110c2, 0x110cd,
0x110cd, 0x110d0, 0x110e8, 0x110f0, 0x110f9, 0x11100, 0x11134, 0x11136,
0x1113f, 0x11144, 0x11147, 0x11150, 0x11173, 0x11176, 0x11176, 0x11180,
0x111c4, 0x111c9, 0x111cc, 0x111ce, 0x111da, 0x111dc, 0x111dc, 0x11200,
0x11211, 0x11213, 0x11237, 0x1123e, 0x1123e, 0x11280, 0x11286, 0x11288,
0x11288, 0x1128a, 0x1128d, 0x1128f, 0x1129d, 0x1129f, 0x112a8, 0x112b0,
0x112ea, 0x112f0, 0x112f9, 0x11300, 0x11303, 0x11305, 0x1130c, 0x1130f,
0x11310, 0x11313, 0x11328, 0x1132a, 0x11330, 0x11332, 0x11333, 0x11335,
0x11339, 0x1133b, 0x11344, 0x11347, 0x11348, 0x1134b, 0x1134d, 0x11350,
0x11350, 0x11357, 0x11357, 0x1135d, 0x11363, 0x11366, 0x1136c, 0x11370,
0x11374, 0x11400, 0x1144a, 0x11450, 0x11459, 0x1145e, 0x11461, 0x11480,
0x114c5, 0x114c7, 0x114c7, 0x114d0, 0x114d9, 0x11580, 0x115b5, 0x115b8,
0x115c0, 0x115d8, 0x115dd, 0x11600, 0x11640, 0x11644, 0x11644, 0x11650,
0x11659, 0x11680, 0x116b8, 0x116c0, 0x116c9, 0x11700, 0x1171a, 0x1171d,
0x1172b, 0x11730, 0x11739, 0x11740, 0x11746, 0x11800, 0x1183a, 0x118a0,
0x118e9, 0x118ff, 0x11906, 0x11909, 0x11909, 0x1190c, 0x11913, 0x11915,
0x11916, 0x11918, 0x11935, 0x11937, 0x11938, 0x1193b, 0x11943, 0x11950,
0x11959, 0x119a0, 0x119a7, 0x119aa, 0x119d7, 0x119da, 0x119e1, 0x119e3,
0x119e4, 0x11a00, 0x11a3e, 0x11a47, 0x11a47, 0x11a50, 0x11a99, 0x11a9d,
0x11a9d, 0x11ab0, 0x11af8, 0x11c00, 0x11c08, 0x11c0a, 0x11c36, 0x11c38,
0x11c40, 0x11c50, 0x11c59, 0x11c72, 0x11c8f, 0x11c92, 0x11ca7, 0x11ca9,
0x11cb6, 0x11d00, 0x11d06, 0x11d08, 0x11d09, 0x11d0b, 0x11d36, 0x11d3a,
0x11d3a, 0x11d3c, 0x11d3d, 0x11d3f, 0x11d47, 0x11d50, 0x11d59, 0x11d60,
0x11d65, 0x11d67, 0x11d68, 0x11d6a, 0x11d8e, 0x11d90, 0x11d91, 0x11d93,
0x11d98, 0x11da0, 0x11da9, 0x11ee0, 0x11ef6, 0x11fb0, 0x11fb0, 0x11fdd,
0x11fe0, 0x12000, 0x12399, 0x12400, 0x1246e, 0x12480, 0x12543, 0x12f90,
0x12ff0, 0x13000, 0x1342e, 0x13430, 0x13438, 0x14400, 0x14646, 0x16800,
0x16a38, 0x16a40, 0x16a5e, 0x16a60, 0x16a69, 0x16a70, 0x16abe, 0x16ac0,
0x16ac9, 0x16ad0, 0x16aed, 0x16af0, 0x16af4, 0x16b00, 0x16b36, 0x16b40,
0x16b43, 0x16b50, 0x16b59, 0x16b63, 0x16b77, 0x16b7d, 0x16b8f, 0x16e40,
0x16e7f, 0x16f00, 0x16f4a, 0x16f4f, 0x16f87, 0x16f8f, 0x16f9f, 0x16fe0,
0x16fe1, 0x16fe3, 0x16fe4, 0x16ff0, 0x16ff1, 0x17000, 0x187f7, 0x18800,
0x18cd5, 0x18d00, 0x18d08, 0x1aff0, 0x1aff3, 0x1aff5, 0x1affb, 0x1affd,
0x1affe, 0x1b000, 0x1b122, 0x1b150, 0x1b152, 0x1b164, 0x1b167, 0x1b170,
0x1b2fb, 0x1bc00, 0x1bc6a, 0x1bc70, 0x1bc7c, 0x1bc80, 0x1bc88, 0x1bc90,
0x1bc99, 0x1bc9d, 0x1bc9e, 0x1bca0, 0x1bca3, 0x1cf00, 0x1cf2d, 0x1cf30,
0x1cf46, 0x1d165, 0x1d169, 0x1d16d, 0x1d182, 0x1d185, 0x1d18b, 0x1d1aa,
0x1d1ad, 0x1d242, 0x1d244, 0x1d400, 0x1d454, 0x1d456, 0x1d49c, 0x1d49e,
0x1d49f, 0x1d4a2, 0x1d4a2, 0x1d4a5, 0x1d4a6, 0x1d4a9, 0x1d4ac, 0x1d4ae,
0x1d4b9, 0x1d4bb, 0x1d4bb, 0x1d4bd, 0x1d4c3, 0x1d4c5, 0x1d505, 0x1d507,
0x1d50a, 0x1d50d, 0x1d514, 0x1d516, 0x1d51c, 0x1d51e, 0x1d539, 0x1d53b,
0x1d53e, 0x1d540, 0x1d544, 0x1d546, 0x1d546, 0x1d54a, 0x1d550, 0x1d552,
0x1d6a5, 0x1d6a8, 0x1d6c0, 0x1d6c2, 0x1d6da, 0x1d6dc, 0x1d6fa, 0x1d6fc,
0x1d714, 0x1d716, 0x1d734, 0x1d736, 0x1d74e, 0x1d750, 0x1d76e, 0x1d770,
0x1d788, 0x1d78a, 0x1d7a8, 0x1d7aa, 0x1d7c2, 0x1d7c4, 0x1d7cb, 0x1d7ce,
0x1d7ff, 0x1da00, 0x1da36, 0x1da3b, 0x1da6c, 0x1da75, 0x1da75, 0x1da84,
0x1da84, 0x1da9b, 0x1da9f, 0x1daa1, 0x1daaf, 0x1df00, 0x1df1e, 0x1e000,
0x1e006, 0x1e008, 0x1e018, 0x1e01b, 0x1e021, 0x1e023, 0x1e024, 0x1e026,
0x1e02a, 0x1e100, 0x1e12c, 0x1e130, 0x1e13d, 0x1e140, 0x1e149, 0x1e14e,
0x1e14e, 0x1e290, 0x1e2ae, 0x1e2c0, 0x1e2f9, 0x1e2ff, 0x1e2ff, 0x1e7e0,
0x1e7e6, 0x1e7e8, 0x1e7eb, 0x1e7ed, 0x1e7ee, 0x1e7f0, 0x1e7fe, 0x1e800,
0x1e8c4, 0x1e8d0, 0x1e8d6, 0x1e900, 0x1e94b, 0x1e950, 0x1e959, 0x1ecb0,
0x1ecb0, 0x1ee00, 0x1ee03, 0x1ee05, 0x1ee1f, 0x1ee21, 0x1ee22, 0x1ee24,
0x1ee24, 0x1ee27, 0x1ee27, 0x1ee29, 0x1ee32, 0x1ee34, 0x1ee37, 0x1ee39,
0x1ee39, 0x1ee3b, 0x1ee3b, 0x1ee42, 0x1ee42, 0x1ee47, 0x1ee47, 0x1ee49,
0x1ee49, 0x1ee4b, 0x1ee4b, 0x1ee4d, 0x1ee4f, 0x1ee51, 0x1ee52, 0x1ee54,
0x1ee54, 0x1ee57, 0x1ee57, 0x1ee59, 0x1ee59, 0x1ee5b, 0x1ee5b, 0x1ee5d,
0x1ee5d, 0x1ee5f, 0x1ee5f, 0x1ee61, 0x1ee62, 0x1ee64, 0x1ee64, 0x1ee67,
0x1ee6a, 0x1ee6c, 0x1ee72, 0x1ee74, 0x1ee77, 0x1ee79, 0x1ee7c, 0x1ee7e,
0x1ee7e, 0x1ee80, 0x1ee89, 0x1ee8b, 0x1ee9b, 0x1eea1, 0x1eea3, 0x1eea5,
0x1eea9, 0x1eeab, 0x1eebb, 0x1fbf0, 0x1fbf9, 0x20000, 0x2a6df, 0x2a700,
0x2b738, 0x2b740, 0x2b81d, 0x2b820, 0x2cea1, 0x2ceb0, 0x2ebe0, 0x2f800,
0x2fa1d, 0x30000, 0x3134a, 0xe0001, 0xe0001, 0xe0020, 0xe007f, 0xe0100,
0xe01ef};
}
private static final int[] NFA_MOVES_TEMPLATE_TEXT_31 = NFA_MOVES_TEMPLATE_TEXT_31_init();
private static int[] NFA_MOVES_TEMPLATE_TEXT_31_init() {
return new int[] {'$', '$', 'A', 'Z', '_', '_', 'a', 'z', 0xa2, 0xa5,
0xaa, 0xaa, 0xb5, 0xb5, 0xba, 0xba, 0xc0, 0xd6, 0xd8, 0xf6, 0xf8, 0x2c1,
0x2c6, 0x2d1, 0x2e0, 0x2e4, 0x2ec, 0x2ec, 0x2ee, 0x2ee, 0x370, 0x374,
0x376, 0x377, 0x37a, 0x37d, 0x37f, 0x37f, 0x386, 0x386, 0x388, 0x38a,
0x38c, 0x38c, 0x38e, 0x3a1, 0x3a3, 0x3f5, 0x3f7, 0x481, 0x48a, 0x52f,
0x531, 0x556, 0x559, 0x559, 0x560, 0x588, 0x58f, 0x58f, 0x5d0, 0x5ea,
0x5ef, 0x5f2, 0x60b, 0x60b, 0x620, 0x64a, 0x66e, 0x66f, 0x671, 0x6d3,
0x6d5, 0x6d5, 0x6e5, 0x6e6, 0x6ee, 0x6ef, 0x6fa, 0x6fc, 0x6ff, 0x6ff,
0x710, 0x710, 0x712, 0x72f, 0x74d, 0x7a5, 0x7b1, 0x7b1, 0x7ca, 0x7ea,
0x7f4, 0x7f5, 0x7fa, 0x7fa, 0x7fe, 0x815, 0x81a, 0x81a, 0x824, 0x824,
0x828, 0x828, 0x840, 0x858, 0x860, 0x86a, 0x870, 0x887, 0x889, 0x88e,
0x8a0, 0x8c9, 0x904, 0x939, 0x93d, 0x93d, 0x950, 0x950, 0x958, 0x961,
0x971, 0x980, 0x985, 0x98c, 0x98f, 0x990, 0x993, 0x9a8, 0x9aa, 0x9b0,
0x9b2, 0x9b2, 0x9b6, 0x9b9, 0x9bd, 0x9bd, 0x9ce, 0x9ce, 0x9dc, 0x9dd,
0x9df, 0x9e1, 0x9f0, 0x9f3, 0x9fb, 0x9fc, 0xa05, 0xa0a, 0xa0f, 0xa10,
0xa13, 0xa28, 0xa2a, 0xa30, 0xa32, 0xa33, 0xa35, 0xa36, 0xa38, 0xa39,
0xa59, 0xa5c, 0xa5e, 0xa5e, 0xa72, 0xa74, 0xa85, 0xa8d, 0xa8f, 0xa91,
0xa93, 0xaa8, 0xaaa, 0xab0, 0xab2, 0xab3, 0xab5, 0xab9, 0xabd, 0xabd,
0xad0, 0xad0, 0xae0, 0xae1, 0xaf1, 0xaf1, 0xaf9, 0xaf9, 0xb05, 0xb0c,
0xb0f, 0xb10, 0xb13, 0xb28, 0xb2a, 0xb30, 0xb32, 0xb33, 0xb35, 0xb39,
0xb3d, 0xb3d, 0xb5c, 0xb5d, 0xb5f, 0xb61, 0xb71, 0xb71, 0xb83, 0xb83,
0xb85, 0xb8a, 0xb8e, 0xb90, 0xb92, 0xb95, 0xb99, 0xb9a, 0xb9c, 0xb9c,
0xb9e, 0xb9f, 0xba3, 0xba4, 0xba8, 0xbaa, 0xbae, 0xbb9, 0xbd0, 0xbd0,
0xbf9, 0xbf9, 0xc05, 0xc0c, 0xc0e, 0xc10, 0xc12, 0xc28, 0xc2a, 0xc39,
0xc3d, 0xc3d, 0xc58, 0xc5a, 0xc5d, 0xc5d, 0xc60, 0xc61, 0xc80, 0xc80,
0xc85, 0xc8c, 0xc8e, 0xc90, 0xc92, 0xca8, 0xcaa, 0xcb3, 0xcb5, 0xcb9,
0xcbd, 0xcbd, 0xcdd, 0xcde, 0xce0, 0xce1, 0xcf1, 0xcf2, 0xd04, 0xd0c,
0xd0e, 0xd10, 0xd12, 0xd3a, 0xd3d, 0xd3d, 0xd4e, 0xd4e, 0xd54, 0xd56,
0xd5f, 0xd61, 0xd7a, 0xd7f, 0xd85, 0xd96, 0xd9a, 0xdb1, 0xdb3, 0xdbb,
0xdbd, 0xdbd, 0xdc0, 0xdc6, 0xe01, 0xe30, 0xe32, 0xe33, 0xe3f, 0xe46,
0xe81, 0xe82, 0xe84, 0xe84, 0xe86, 0xe8a, 0xe8c, 0xea3, 0xea5, 0xea5,
0xea7, 0xeb0, 0xeb2, 0xeb3, 0xebd, 0xebd, 0xec0, 0xec4, 0xec6, 0xec6,
0xedc, 0xedf, 0xf00, 0xf00, 0xf40, 0xf47, 0xf49, 0xf6c, 0xf88, 0xf8c,
0x1000, 0x102a, 0x103f, 0x103f, 0x1050, 0x1055, 0x105a, 0x105d, 0x1061,
0x1061, 0x1065, 0x1066, 0x106e, 0x1070, 0x1075, 0x1081, 0x108e, 0x108e,
0x10a0, 0x10c5, 0x10c7, 0x10c7, 0x10cd, 0x10cd, 0x10d0, 0x10fa, 0x10fc,
0x1248, 0x124a, 0x124d, 0x1250, 0x1256, 0x1258, 0x1258, 0x125a, 0x125d,
0x1260, 0x1288, 0x128a, 0x128d, 0x1290, 0x12b0, 0x12b2, 0x12b5, 0x12b8,
0x12be, 0x12c0, 0x12c0, 0x12c2, 0x12c5, 0x12c8, 0x12d6, 0x12d8, 0x1310,
0x1312, 0x1315, 0x1318, 0x135a, 0x1380, 0x138f, 0x13a0, 0x13f5, 0x13f8,
0x13fd, 0x1401, 0x166c, 0x166f, 0x167f, 0x1681, 0x169a, 0x16a0, 0x16ea,
0x16ee, 0x16f8, 0x1700, 0x1711, 0x171f, 0x1731, 0x1740, 0x1751, 0x1760,
0x176c, 0x176e, 0x1770, 0x1780, 0x17b3, 0x17d7, 0x17d7, 0x17db, 0x17dc,
0x1820, 0x1878, 0x1880, 0x1884, 0x1887, 0x18a8, 0x18aa, 0x18aa, 0x18b0,
0x18f5, 0x1900, 0x191e, 0x1950, 0x196d, 0x1970, 0x1974, 0x1980, 0x19ab,
0x19b0, 0x19c9, 0x1a00, 0x1a16, 0x1a20, 0x1a54, 0x1aa7, 0x1aa7, 0x1b05,
0x1b33, 0x1b45, 0x1b4c, 0x1b83, 0x1ba0, 0x1bae, 0x1baf, 0x1bba, 0x1be5,
0x1c00, 0x1c23, 0x1c4d, 0x1c4f, 0x1c5a, 0x1c7d, 0x1c80, 0x1c88, 0x1c90,
0x1cba, 0x1cbd, 0x1cbf, 0x1ce9, 0x1cec, 0x1cee, 0x1cf3, 0x1cf5, 0x1cf6,
0x1cfa, 0x1cfa, 0x1d00, 0x1dbf, 0x1e00, 0x1f15, 0x1f18, 0x1f1d, 0x1f20,
0x1f45, 0x1f48, 0x1f4d, 0x1f50, 0x1f57, 0x1f59, 0x1f59, 0x1f5b, 0x1f5b,
0x1f5d, 0x1f5d, 0x1f5f, 0x1f7d, 0x1f80, 0x1fb4, 0x1fb6, 0x1fbc, 0x1fbe,
0x1fbe, 0x1fc2, 0x1fc4, 0x1fc6, 0x1fcc, 0x1fd0, 0x1fd3, 0x1fd6, 0x1fdb,
0x1fe0, 0x1fec, 0x1ff2, 0x1ff4, 0x1ff6, 0x1ffc, 0x203f, 0x2040, 0x2054,
0x2054, 0x2071, 0x2071, 0x207f, 0x207f, 0x2090, 0x209c, 0x20a0, 0x20c0,
0x2102, 0x2102, 0x2107, 0x2107, 0x210a, 0x2113, 0x2115, 0x2115, 0x2119,
0x211d, 0x2124, 0x2124, 0x2126, 0x2126, 0x2128, 0x2128, 0x212a, 0x212d,
0x212f, 0x2139, 0x213c, 0x213f, 0x2145, 0x2149, 0x214e, 0x214e, 0x2160,
0x2188, 0x2c00, 0x2ce4, 0x2ceb, 0x2cee, 0x2cf2, 0x2cf3, 0x2d00, 0x2d25,
0x2d27, 0x2d27, 0x2d2d, 0x2d2d, 0x2d30, 0x2d67, 0x2d6f, 0x2d6f, 0x2d80,
0x2d96, 0x2da0, 0x2da6, 0x2da8, 0x2dae, 0x2db0, 0x2db6, 0x2db8, 0x2dbe,
0x2dc0, 0x2dc6, 0x2dc8, 0x2dce, 0x2dd0, 0x2dd6, 0x2dd8, 0x2dde, 0x2e2f,
0x2e2f, 0x3005, 0x3007, 0x3021, 0x3029, 0x3031, 0x3035, 0x3038, 0x303c,
0x3041, 0x3096, 0x309d, 0x309f, 0x30a1, 0x30fa, 0x30fc, 0x30ff, 0x3105,
0x312f, 0x3131, 0x318e, 0x31a0, 0x31bf, 0x31f0, 0x31ff, 0x3400, 0x4dbf,
0x4e00, 0xa48c, 0xa4d0, 0xa4fd, 0xa500, 0xa60c, 0xa610, 0xa61f, 0xa62a,
0xa62b, 0xa640, 0xa66e, 0xa67f, 0xa69d, 0xa6a0, 0xa6ef, 0xa717, 0xa71f,
0xa722, 0xa788, 0xa78b, 0xa7ca, 0xa7d0, 0xa7d1, 0xa7d3, 0xa7d3, 0xa7d5,
0xa7d9, 0xa7f2, 0xa801, 0xa803, 0xa805, 0xa807, 0xa80a, 0xa80c, 0xa822,
0xa838, 0xa838, 0xa840, 0xa873, 0xa882, 0xa8b3, 0xa8f2, 0xa8f7, 0xa8fb,
0xa8fb, 0xa8fd, 0xa8fe, 0xa90a, 0xa925, 0xa930, 0xa946, 0xa960, 0xa97c,
0xa984, 0xa9b2, 0xa9cf, 0xa9cf, 0xa9e0, 0xa9e4, 0xa9e6, 0xa9ef, 0xa9fa,
0xa9fe, 0xaa00, 0xaa28, 0xaa40, 0xaa42, 0xaa44, 0xaa4b, 0xaa60, 0xaa76,
0xaa7a, 0xaa7a, 0xaa7e, 0xaaaf, 0xaab1, 0xaab1, 0xaab5, 0xaab6, 0xaab9,
0xaabd, 0xaac0, 0xaac0, 0xaac2, 0xaac2, 0xaadb, 0xaadd, 0xaae0, 0xaaea,
0xaaf2, 0xaaf4, 0xab01, 0xab06, 0xab09, 0xab0e, 0xab11, 0xab16, 0xab20,
0xab26, 0xab28, 0xab2e, 0xab30, 0xab5a, 0xab5c, 0xab69, 0xab70, 0xabe2,
0xac00, 0xd7a3, 0xd7b0, 0xd7c6, 0xd7cb, 0xd7fb, 0xf900, 0xfa6d, 0xfa70,
0xfad9, 0xfb00, 0xfb06, 0xfb13, 0xfb17, 0xfb1d, 0xfb1d, 0xfb1f, 0xfb28,
0xfb2a, 0xfb36, 0xfb38, 0xfb3c, 0xfb3e, 0xfb3e, 0xfb40, 0xfb41, 0xfb43,
0xfb44, 0xfb46, 0xfbb1, 0xfbd3, 0xfd3d, 0xfd50, 0xfd8f, 0xfd92, 0xfdc7,
0xfdf0, 0xfdfc, 0xfe33, 0xfe34, 0xfe4d, 0xfe4f, 0xfe69, 0xfe69, 0xfe70,
0xfe74, 0xfe76, 0xfefc, 0xff04, 0xff04, 0xff21, 0xff3a, 0xff3f, 0xff3f,
0xff41, 0xff5a, 0xff66, 0xffbe, 0xffc2, 0xffc7, 0xffca, 0xffcf, 0xffd2,
0xffd7, 0xffda, 0xffdc, 0xffe0, 0xffe1, 0xffe5, 0xffe6, 0x10000, 0x1000b,
0x1000d, 0x10026, 0x10028, 0x1003a, 0x1003c, 0x1003d, 0x1003f, 0x1004d,
0x10050, 0x1005d, 0x10080, 0x100fa, 0x10140, 0x10174, 0x10280, 0x1029c,
0x102a0, 0x102d0, 0x10300, 0x1031f, 0x1032d, 0x1034a, 0x10350, 0x10375,
0x10380, 0x1039d, 0x103a0, 0x103c3, 0x103c8, 0x103cf, 0x103d1, 0x103d5,
0x10400, 0x1049d, 0x104b0, 0x104d3, 0x104d8, 0x104fb, 0x10500, 0x10527,
0x10530, 0x10563, 0x10570, 0x1057a, 0x1057c, 0x1058a, 0x1058c, 0x10592,
0x10594, 0x10595, 0x10597, 0x105a1, 0x105a3, 0x105b1, 0x105b3, 0x105b9,
0x105bb, 0x105bc, 0x10600, 0x10736, 0x10740, 0x10755, 0x10760, 0x10767,
0x10780, 0x10785, 0x10787, 0x107b0, 0x107b2, 0x107ba, 0x10800, 0x10805,
0x10808, 0x10808, 0x1080a, 0x10835, 0x10837, 0x10838, 0x1083c, 0x1083c,
0x1083f, 0x10855, 0x10860, 0x10876, 0x10880, 0x1089e, 0x108e0, 0x108f2,
0x108f4, 0x108f5, 0x10900, 0x10915, 0x10920, 0x10939, 0x10980, 0x109b7,
0x109be, 0x109bf, 0x10a00, 0x10a00, 0x10a10, 0x10a13, 0x10a15, 0x10a17,
0x10a19, 0x10a35, 0x10a60, 0x10a7c, 0x10a80, 0x10a9c, 0x10ac0, 0x10ac7,
0x10ac9, 0x10ae4, 0x10b00, 0x10b35, 0x10b40, 0x10b55, 0x10b60, 0x10b72,
0x10b80, 0x10b91, 0x10c00, 0x10c48, 0x10c80, 0x10cb2, 0x10cc0, 0x10cf2,
0x10d00, 0x10d23, 0x10e80, 0x10ea9, 0x10eb0, 0x10eb1, 0x10f00, 0x10f1c,
0x10f27, 0x10f27, 0x10f30, 0x10f45, 0x10f70, 0x10f81, 0x10fb0, 0x10fc4,
0x10fe0, 0x10ff6, 0x11003, 0x11037, 0x11071, 0x11072, 0x11075, 0x11075,
0x11083, 0x110af, 0x110d0, 0x110e8, 0x11103, 0x11126, 0x11144, 0x11144,
0x11147, 0x11147, 0x11150, 0x11172, 0x11176, 0x11176, 0x11183, 0x111b2,
0x111c1, 0x111c4, 0x111da, 0x111da, 0x111dc, 0x111dc, 0x11200, 0x11211,
0x11213, 0x1122b, 0x11280, 0x11286, 0x11288, 0x11288, 0x1128a, 0x1128d,
0x1128f, 0x1129d, 0x1129f, 0x112a8, 0x112b0, 0x112de, 0x11305, 0x1130c,
0x1130f, 0x11310, 0x11313, 0x11328, 0x1132a, 0x11330, 0x11332, 0x11333,
0x11335, 0x11339, 0x1133d, 0x1133d, 0x11350, 0x11350, 0x1135d, 0x11361,
0x11400, 0x11434, 0x11447, 0x1144a, 0x1145f, 0x11461, 0x11480, 0x114af,
0x114c4, 0x114c5, 0x114c7, 0x114c7, 0x11580, 0x115ae, 0x115d8, 0x115db,
0x11600, 0x1162f, 0x11644, 0x11644, 0x11680, 0x116aa, 0x116b8, 0x116b8,
0x11700, 0x1171a, 0x11740, 0x11746, 0x11800, 0x1182b, 0x118a0, 0x118df,
0x118ff, 0x11906, 0x11909, 0x11909, 0x1190c, 0x11913, 0x11915, 0x11916,
0x11918, 0x1192f, 0x1193f, 0x1193f, 0x11941, 0x11941, 0x119a0, 0x119a7,
0x119aa, 0x119d0, 0x119e1, 0x119e1, 0x119e3, 0x119e3, 0x11a00, 0x11a00,
0x11a0b, 0x11a32, 0x11a3a, 0x11a3a, 0x11a50, 0x11a50, 0x11a5c, 0x11a89,
0x11a9d, 0x11a9d, 0x11ab0, 0x11af8, 0x11c00, 0x11c08, 0x11c0a, 0x11c2e,
0x11c40, 0x11c40, 0x11c72, 0x11c8f, 0x11d00, 0x11d06, 0x11d08, 0x11d09,
0x11d0b, 0x11d30, 0x11d46, 0x11d46, 0x11d60, 0x11d65, 0x11d67, 0x11d68,
0x11d6a, 0x11d89, 0x11d98, 0x11d98, 0x11ee0, 0x11ef2, 0x11fb0, 0x11fb0,
0x11fdd, 0x11fe0, 0x12000, 0x12399, 0x12400, 0x1246e, 0x12480, 0x12543,
0x12f90, 0x12ff0, 0x13000, 0x1342e, 0x14400, 0x14646, 0x16800, 0x16a38,
0x16a40, 0x16a5e, 0x16a70, 0x16abe, 0x16ad0, 0x16aed, 0x16b00, 0x16b2f,
0x16b40, 0x16b43, 0x16b63, 0x16b77, 0x16b7d, 0x16b8f, 0x16e40, 0x16e7f,
0x16f00, 0x16f4a, 0x16f50, 0x16f50, 0x16f93, 0x16f9f, 0x16fe0, 0x16fe1,
0x16fe3, 0x16fe3, 0x17000, 0x187f7, 0x18800, 0x18cd5, 0x18d00, 0x18d08,
0x1aff0, 0x1aff3, 0x1aff5, 0x1affb, 0x1affd, 0x1affe, 0x1b000, 0x1b122,
0x1b150, 0x1b152, 0x1b164, 0x1b167, 0x1b170, 0x1b2fb, 0x1bc00, 0x1bc6a,
0x1bc70, 0x1bc7c, 0x1bc80, 0x1bc88, 0x1bc90, 0x1bc99, 0x1d400, 0x1d454,
0x1d456, 0x1d49c, 0x1d49e, 0x1d49f, 0x1d4a2, 0x1d4a2, 0x1d4a5, 0x1d4a6,
0x1d4a9, 0x1d4ac, 0x1d4ae, 0x1d4b9, 0x1d4bb, 0x1d4bb, 0x1d4bd, 0x1d4c3,
0x1d4c5, 0x1d505, 0x1d507, 0x1d50a, 0x1d50d, 0x1d514, 0x1d516, 0x1d51c,
0x1d51e, 0x1d539, 0x1d53b, 0x1d53e, 0x1d540, 0x1d544, 0x1d546, 0x1d546,
0x1d54a, 0x1d550, 0x1d552, 0x1d6a5, 0x1d6a8, 0x1d6c0, 0x1d6c2, 0x1d6da,
0x1d6dc, 0x1d6fa, 0x1d6fc, 0x1d714, 0x1d716, 0x1d734, 0x1d736, 0x1d74e,
0x1d750, 0x1d76e, 0x1d770, 0x1d788, 0x1d78a, 0x1d7a8, 0x1d7aa, 0x1d7c2,
0x1d7c4, 0x1d7cb, 0x1df00, 0x1df1e, 0x1e100, 0x1e12c, 0x1e137, 0x1e13d,
0x1e14e, 0x1e14e, 0x1e290, 0x1e2ad, 0x1e2c0, 0x1e2eb, 0x1e2ff, 0x1e2ff,
0x1e7e0, 0x1e7e6, 0x1e7e8, 0x1e7eb, 0x1e7ed, 0x1e7ee, 0x1e7f0, 0x1e7fe,
0x1e800, 0x1e8c4, 0x1e900, 0x1e943, 0x1e94b, 0x1e94b, 0x1ecb0, 0x1ecb0,
0x1ee00, 0x1ee03, 0x1ee05, 0x1ee1f, 0x1ee21, 0x1ee22, 0x1ee24, 0x1ee24,
0x1ee27, 0x1ee27, 0x1ee29, 0x1ee32, 0x1ee34, 0x1ee37, 0x1ee39, 0x1ee39,
0x1ee3b, 0x1ee3b, 0x1ee42, 0x1ee42, 0x1ee47, 0x1ee47, 0x1ee49, 0x1ee49,
0x1ee4b, 0x1ee4b, 0x1ee4d, 0x1ee4f, 0x1ee51, 0x1ee52, 0x1ee54, 0x1ee54,
0x1ee57, 0x1ee57, 0x1ee59, 0x1ee59, 0x1ee5b, 0x1ee5b, 0x1ee5d, 0x1ee5d,
0x1ee5f, 0x1ee5f, 0x1ee61, 0x1ee62, 0x1ee64, 0x1ee64, 0x1ee67, 0x1ee6a,
0x1ee6c, 0x1ee72, 0x1ee74, 0x1ee77, 0x1ee79, 0x1ee7c, 0x1ee7e, 0x1ee7e,
0x1ee80, 0x1ee89, 0x1ee8b, 0x1ee9b, 0x1eea1, 0x1eea3, 0x1eea5, 0x1eea9,
0x1eeab, 0x1eebb, 0x20000, 0x2a6df, 0x2a700, 0x2b738, 0x2b740, 0x2b81d,
0x2b820, 0x2cea1, 0x2ceb0, 0x2ebe0, 0x2f800, 0x2fa1d, 0x30000, 0x3134a};
}
private static final int[] NFA_MOVES_TEMPLATE_TEXT_33 = NFA_MOVES_TEMPLATE_TEXT_33_init();
private static int[] NFA_MOVES_TEMPLATE_TEXT_33_init() {
return new int[] {0x0, 0x8, 0xe, 0x1b, '$', '$', '0', '9', 'A', 'Z', '_',
'_', 'a', 'z', 0x7f, 0x9f, 0xa2, 0xa5, 0xaa, 0xaa, 0xad, 0xad, 0xb5, 0xb5,
0xba, 0xba, 0xc0, 0xd6, 0xd8, 0xf6, 0xf8, 0x2c1, 0x2c6, 0x2d1, 0x2e0,
0x2e4, 0x2ec, 0x2ec, 0x2ee, 0x2ee, 0x300, 0x374, 0x376, 0x377, 0x37a,
0x37d, 0x37f, 0x37f, 0x386, 0x386, 0x388, 0x38a, 0x38c, 0x38c, 0x38e,
0x3a1, 0x3a3, 0x3f5, 0x3f7, 0x481, 0x483, 0x487, 0x48a, 0x52f, 0x531,
0x556, 0x559, 0x559, 0x560, 0x588, 0x58f, 0x58f, 0x591, 0x5bd, 0x5bf,
0x5bf, 0x5c1, 0x5c2, 0x5c4, 0x5c5, 0x5c7, 0x5c7, 0x5d0, 0x5ea, 0x5ef,
0x5f2, 0x600, 0x605, 0x60b, 0x60b, 0x610, 0x61a, 0x61c, 0x61c, 0x620,
0x669, 0x66e, 0x6d3, 0x6d5, 0x6dd, 0x6df, 0x6e8, 0x6ea, 0x6fc, 0x6ff,
0x6ff, 0x70f, 0x74a, 0x74d, 0x7b1, 0x7c0, 0x7f5, 0x7fa, 0x7fa, 0x7fd,
0x82d, 0x840, 0x85b, 0x860, 0x86a, 0x870, 0x887, 0x889, 0x88e, 0x890,
0x891, 0x898, 0x963, 0x966, 0x96f, 0x971, 0x983, 0x985, 0x98c, 0x98f,
0x990, 0x993, 0x9a8, 0x9aa, 0x9b0, 0x9b2, 0x9b2, 0x9b6, 0x9b9, 0x9bc,
0x9c4, 0x9c7, 0x9c8, 0x9cb, 0x9ce, 0x9d7, 0x9d7, 0x9dc, 0x9dd, 0x9df,
0x9e3, 0x9e6, 0x9f3, 0x9fb, 0x9fc, 0x9fe, 0x9fe, 0xa01, 0xa03, 0xa05,
0xa0a, 0xa0f, 0xa10, 0xa13, 0xa28, 0xa2a, 0xa30, 0xa32, 0xa33, 0xa35,
0xa36, 0xa38, 0xa39, 0xa3c, 0xa3c, 0xa3e, 0xa42, 0xa47, 0xa48, 0xa4b,
0xa4d, 0xa51, 0xa51, 0xa59, 0xa5c, 0xa5e, 0xa5e, 0xa66, 0xa75, 0xa81,
0xa83, 0xa85, 0xa8d, 0xa8f, 0xa91, 0xa93, 0xaa8, 0xaaa, 0xab0, 0xab2,
0xab3, 0xab5, 0xab9, 0xabc, 0xac5, 0xac7, 0xac9, 0xacb, 0xacd, 0xad0,
0xad0, 0xae0, 0xae3, 0xae6, 0xaef, 0xaf1, 0xaf1, 0xaf9, 0xaff, 0xb01,
0xb03, 0xb05, 0xb0c, 0xb0f, 0xb10, 0xb13, 0xb28, 0xb2a, 0xb30, 0xb32,
0xb33, 0xb35, 0xb39, 0xb3c, 0xb44, 0xb47, 0xb48, 0xb4b, 0xb4d, 0xb55,
0xb57, 0xb5c, 0xb5d, 0xb5f, 0xb63, 0xb66, 0xb6f, 0xb71, 0xb71, 0xb82,
0xb83, 0xb85, 0xb8a, 0xb8e, 0xb90, 0xb92, 0xb95, 0xb99, 0xb9a, 0xb9c,
0xb9c, 0xb9e, 0xb9f, 0xba3, 0xba4, 0xba8, 0xbaa, 0xbae, 0xbb9, 0xbbe,
0xbc2, 0xbc6, 0xbc8, 0xbca, 0xbcd, 0xbd0, 0xbd0, 0xbd7, 0xbd7, 0xbe6,
0xbef, 0xbf9, 0xbf9, 0xc00, 0xc0c, 0xc0e, 0xc10, 0xc12, 0xc28, 0xc2a,
0xc39, 0xc3c, 0xc44, 0xc46, 0xc48, 0xc4a, 0xc4d, 0xc55, 0xc56, 0xc58,
0xc5a, 0xc5d, 0xc5d, 0xc60, 0xc63, 0xc66, 0xc6f, 0xc80, 0xc83, 0xc85,
0xc8c, 0xc8e, 0xc90, 0xc92, 0xca8, 0xcaa, 0xcb3, 0xcb5, 0xcb9, 0xcbc,
0xcc4, 0xcc6, 0xcc8, 0xcca, 0xccd, 0xcd5, 0xcd6, 0xcdd, 0xcde, 0xce0,
0xce3, 0xce6, 0xcef, 0xcf1, 0xcf2, 0xd00, 0xd0c, 0xd0e, 0xd10, 0xd12,
0xd44, 0xd46, 0xd48, 0xd4a, 0xd4e, 0xd54, 0xd57, 0xd5f, 0xd63, 0xd66,
0xd6f, 0xd7a, 0xd7f, 0xd81, 0xd83, 0xd85, 0xd96, 0xd9a, 0xdb1, 0xdb3,
0xdbb, 0xdbd, 0xdbd, 0xdc0, 0xdc6, 0xdca, 0xdca, 0xdcf, 0xdd4, 0xdd6,
0xdd6, 0xdd8, 0xddf, 0xde6, 0xdef, 0xdf2, 0xdf3, 0xe01, 0xe3a, 0xe3f,
0xe4e, 0xe50, 0xe59, 0xe81, 0xe82, 0xe84, 0xe84, 0xe86, 0xe8a, 0xe8c,
0xea3, 0xea5, 0xea5, 0xea7, 0xebd, 0xec0, 0xec4, 0xec6, 0xec6, 0xec8,
0xecd, 0xed0, 0xed9, 0xedc, 0xedf, 0xf00, 0xf00, 0xf18, 0xf19, 0xf20,
0xf29, 0xf35, 0xf35, 0xf37, 0xf37, 0xf39, 0xf39, 0xf3e, 0xf47, 0xf49,
0xf6c, 0xf71, 0xf84, 0xf86, 0xf97, 0xf99, 0xfbc, 0xfc6, 0xfc6, 0x1000,
0x1049, 0x1050, 0x109d, 0x10a0, 0x10c5, 0x10c7, 0x10c7, 0x10cd, 0x10cd,
0x10d0, 0x10fa, 0x10fc, 0x1248, 0x124a, 0x124d, 0x1250, 0x1256, 0x1258,
0x1258, 0x125a, 0x125d, 0x1260, 0x1288, 0x128a, 0x128d, 0x1290, 0x12b0,
0x12b2, 0x12b5, 0x12b8, 0x12be, 0x12c0, 0x12c0, 0x12c2, 0x12c5, 0x12c8,
0x12d6, 0x12d8, 0x1310, 0x1312, 0x1315, 0x1318, 0x135a, 0x135d, 0x135f,
0x1380, 0x138f, 0x13a0, 0x13f5, 0x13f8, 0x13fd, 0x1401, 0x166c, 0x166f,
0x167f, 0x1681, 0x169a, 0x16a0, 0x16ea, 0x16ee, 0x16f8, 0x1700, 0x1715,
0x171f, 0x1734, 0x1740, 0x1753, 0x1760, 0x176c, 0x176e, 0x1770, 0x1772,
0x1773, 0x1780, 0x17d3, 0x17d7, 0x17d7, 0x17db, 0x17dd, 0x17e0, 0x17e9,
0x180b, 0x1819, 0x1820, 0x1878, 0x1880, 0x18aa, 0x18b0, 0x18f5, 0x1900,
0x191e, 0x1920, 0x192b, 0x1930, 0x193b, 0x1946, 0x196d, 0x1970, 0x1974,
0x1980, 0x19ab, 0x19b0, 0x19c9, 0x19d0, 0x19d9, 0x1a00, 0x1a1b, 0x1a20,
0x1a5e, 0x1a60, 0x1a7c, 0x1a7f, 0x1a89, 0x1a90, 0x1a99, 0x1aa7, 0x1aa7,
0x1ab0, 0x1abd, 0x1abf, 0x1ace, 0x1b00, 0x1b4c, 0x1b50, 0x1b59, 0x1b6b,
0x1b73, 0x1b80, 0x1bf3, 0x1c00, 0x1c37, 0x1c40, 0x1c49, 0x1c4d, 0x1c7d,
0x1c80, 0x1c88, 0x1c90, 0x1cba, 0x1cbd, 0x1cbf, 0x1cd0, 0x1cd2, 0x1cd4,
0x1cfa, 0x1d00, 0x1f15, 0x1f18, 0x1f1d, 0x1f20, 0x1f45, 0x1f48, 0x1f4d,
0x1f50, 0x1f57, 0x1f59, 0x1f59, 0x1f5b, 0x1f5b, 0x1f5d, 0x1f5d, 0x1f5f,
0x1f7d, 0x1f80, 0x1fb4, 0x1fb6, 0x1fbc, 0x1fbe, 0x1fbe, 0x1fc2, 0x1fc4,
0x1fc6, 0x1fcc, 0x1fd0, 0x1fd3, 0x1fd6, 0x1fdb, 0x1fe0, 0x1fec, 0x1ff2,
0x1ff4, 0x1ff6, 0x1ffc, 0x200b, 0x200f, 0x202a, 0x202e, 0x203f, 0x2040,
0x2054, 0x2054, 0x2060, 0x2064, 0x2066, 0x206f, 0x2071, 0x2071, 0x207f,
0x207f, 0x2090, 0x209c, 0x20a0, 0x20c0, 0x20d0, 0x20dc, 0x20e1, 0x20e1,
0x20e5, 0x20f0, 0x2102, 0x2102, 0x2107, 0x2107, 0x210a, 0x2113, 0x2115,
0x2115, 0x2119, 0x211d, 0x2124, 0x2124, 0x2126, 0x2126, 0x2128, 0x2128,
0x212a, 0x212d, 0x212f, 0x2139, 0x213c, 0x213f, 0x2145, 0x2149, 0x214e,
0x214e, 0x2160, 0x2188, 0x2c00, 0x2ce4, 0x2ceb, 0x2cf3, 0x2d00, 0x2d25,
0x2d27, 0x2d27, 0x2d2d, 0x2d2d, 0x2d30, 0x2d67, 0x2d6f, 0x2d6f, 0x2d7f,
0x2d96, 0x2da0, 0x2da6, 0x2da8, 0x2dae, 0x2db0, 0x2db6, 0x2db8, 0x2dbe,
0x2dc0, 0x2dc6, 0x2dc8, 0x2dce, 0x2dd0, 0x2dd6, 0x2dd8, 0x2dde, 0x2de0,
0x2dff, 0x2e2f, 0x2e2f, 0x3005, 0x3007, 0x3021, 0x302f, 0x3031, 0x3035,
0x3038, 0x303c, 0x3041, 0x3096, 0x3099, 0x309a, 0x309d, 0x309f, 0x30a1,
0x30fa, 0x30fc, 0x30ff, 0x3105, 0x312f, 0x3131, 0x318e, 0x31a0, 0x31bf,
0x31f0, 0x31ff, 0x3400, 0x4dbf, 0x4e00, 0xa48c, 0xa4d0, 0xa4fd, 0xa500,
0xa60c, 0xa610, 0xa62b, 0xa640, 0xa66f, 0xa674, 0xa67d, 0xa67f, 0xa6f1,
0xa717, 0xa71f, 0xa722, 0xa788, 0xa78b, 0xa7ca, 0xa7d0, 0xa7d1, 0xa7d3,
0xa7d3, 0xa7d5, 0xa7d9, 0xa7f2, 0xa827, 0xa82c, 0xa82c, 0xa838, 0xa838,
0xa840, 0xa873, 0xa880, 0xa8c5, 0xa8d0, 0xa8d9, 0xa8e0, 0xa8f7, 0xa8fb,
0xa8fb, 0xa8fd, 0xa92d, 0xa930, 0xa953, 0xa960, 0xa97c, 0xa980, 0xa9c0,
0xa9cf, 0xa9d9, 0xa9e0, 0xa9fe, 0xaa00, 0xaa36, 0xaa40, 0xaa4d, 0xaa50,
0xaa59, 0xaa60, 0xaa76, 0xaa7a, 0xaac2, 0xaadb, 0xaadd, 0xaae0, 0xaaef,
0xaaf2, 0xaaf6, 0xab01, 0xab06, 0xab09, 0xab0e, 0xab11, 0xab16, 0xab20,
0xab26, 0xab28, 0xab2e, 0xab30, 0xab5a, 0xab5c, 0xab69, 0xab70, 0xabea,
0xabec, 0xabed, 0xabf0, 0xabf9, 0xac00, 0xd7a3, 0xd7b0, 0xd7c6, 0xd7cb,
0xd7fb, 0xf900, 0xfa6d, 0xfa70, 0xfad9, 0xfb00, 0xfb06, 0xfb13, 0xfb17,
0xfb1d, 0xfb28, 0xfb2a, 0xfb36, 0xfb38, 0xfb3c, 0xfb3e, 0xfb3e, 0xfb40,
0xfb41, 0xfb43, 0xfb44, 0xfb46, 0xfbb1, 0xfbd3, 0xfd3d, 0xfd50, 0xfd8f,
0xfd92, 0xfdc7, 0xfdf0, 0xfdfc, 0xfe00, 0xfe0f, 0xfe20, 0xfe2f, 0xfe33,
0xfe34, 0xfe4d, 0xfe4f, 0xfe69, 0xfe69, 0xfe70, 0xfe74, 0xfe76, 0xfefc,
0xfeff, 0xfeff, 0xff04, 0xff04, 0xff10, 0xff19, 0xff21, 0xff3a, 0xff3f,
0xff3f, 0xff41, 0xff5a, 0xff66, 0xffbe, 0xffc2, 0xffc7, 0xffca, 0xffcf,
0xffd2, 0xffd7, 0xffda, 0xffdc, 0xffe0, 0xffe1, 0xffe5, 0xffe6, 0xfff9,
0xfffb, 0x10000, 0x1000b, 0x1000d, 0x10026, 0x10028, 0x1003a, 0x1003c,
0x1003d, 0x1003f, 0x1004d, 0x10050, 0x1005d, 0x10080, 0x100fa, 0x10140,
0x10174, 0x101fd, 0x101fd, 0x10280, 0x1029c, 0x102a0, 0x102d0, 0x102e0,
0x102e0, 0x10300, 0x1031f, 0x1032d, 0x1034a, 0x10350, 0x1037a, 0x10380,
0x1039d, 0x103a0, 0x103c3, 0x103c8, 0x103cf, 0x103d1, 0x103d5, 0x10400,
0x1049d, 0x104a0, 0x104a9, 0x104b0, 0x104d3, 0x104d8, 0x104fb, 0x10500,
0x10527, 0x10530, 0x10563, 0x10570, 0x1057a, 0x1057c, 0x1058a, 0x1058c,
0x10592, 0x10594, 0x10595, 0x10597, 0x105a1, 0x105a3, 0x105b1, 0x105b3,
0x105b9, 0x105bb, 0x105bc, 0x10600, 0x10736, 0x10740, 0x10755, 0x10760,
0x10767, 0x10780, 0x10785, 0x10787, 0x107b0, 0x107b2, 0x107ba, 0x10800,
0x10805, 0x10808, 0x10808, 0x1080a, 0x10835, 0x10837, 0x10838, 0x1083c,
0x1083c, 0x1083f, 0x10855, 0x10860, 0x10876, 0x10880, 0x1089e, 0x108e0,
0x108f2, 0x108f4, 0x108f5, 0x10900, 0x10915, 0x10920, 0x10939, 0x10980,
0x109b7, 0x109be, 0x109bf, 0x10a00, 0x10a03, 0x10a05, 0x10a06, 0x10a0c,
0x10a13, 0x10a15, 0x10a17, 0x10a19, 0x10a35, 0x10a38, 0x10a3a, 0x10a3f,
0x10a3f, 0x10a60, 0x10a7c, 0x10a80, 0x10a9c, 0x10ac0, 0x10ac7, 0x10ac9,
0x10ae6, 0x10b00, 0x10b35, 0x10b40, 0x10b55, 0x10b60, 0x10b72, 0x10b80,
0x10b91, 0x10c00, 0x10c48, 0x10c80, 0x10cb2, 0x10cc0, 0x10cf2, 0x10d00,
0x10d27, 0x10d30, 0x10d39, 0x10e80, 0x10ea9, 0x10eab, 0x10eac, 0x10eb0,
0x10eb1, 0x10f00, 0x10f1c, 0x10f27, 0x10f27, 0x10f30, 0x10f50, 0x10f70,
0x10f85, 0x10fb0, 0x10fc4, 0x10fe0, 0x10ff6, 0x11000, 0x11046, 0x11066,
0x11075, 0x1107f, 0x110ba, 0x110bd, 0x110bd, 0x110c2, 0x110c2, 0x110cd,
0x110cd, 0x110d0, 0x110e8, 0x110f0, 0x110f9, 0x11100, 0x11134, 0x11136,
0x1113f, 0x11144, 0x11147, 0x11150, 0x11173, 0x11176, 0x11176, 0x11180,
0x111c4, 0x111c9, 0x111cc, 0x111ce, 0x111da, 0x111dc, 0x111dc, 0x11200,
0x11211, 0x11213, 0x11237, 0x1123e, 0x1123e, 0x11280, 0x11286, 0x11288,
0x11288, 0x1128a, 0x1128d, 0x1128f, 0x1129d, 0x1129f, 0x112a8, 0x112b0,
0x112ea, 0x112f0, 0x112f9, 0x11300, 0x11303, 0x11305, 0x1130c, 0x1130f,
0x11310, 0x11313, 0x11328, 0x1132a, 0x11330, 0x11332, 0x11333, 0x11335,
0x11339, 0x1133b, 0x11344, 0x11347, 0x11348, 0x1134b, 0x1134d, 0x11350,
0x11350, 0x11357, 0x11357, 0x1135d, 0x11363, 0x11366, 0x1136c, 0x11370,
0x11374, 0x11400, 0x1144a, 0x11450, 0x11459, 0x1145e, 0x11461, 0x11480,
0x114c5, 0x114c7, 0x114c7, 0x114d0, 0x114d9, 0x11580, 0x115b5, 0x115b8,
0x115c0, 0x115d8, 0x115dd, 0x11600, 0x11640, 0x11644, 0x11644, 0x11650,
0x11659, 0x11680, 0x116b8, 0x116c0, 0x116c9, 0x11700, 0x1171a, 0x1171d,
0x1172b, 0x11730, 0x11739, 0x11740, 0x11746, 0x11800, 0x1183a, 0x118a0,
0x118e9, 0x118ff, 0x11906, 0x11909, 0x11909, 0x1190c, 0x11913, 0x11915,
0x11916, 0x11918, 0x11935, 0x11937, 0x11938, 0x1193b, 0x11943, 0x11950,
0x11959, 0x119a0, 0x119a7, 0x119aa, 0x119d7, 0x119da, 0x119e1, 0x119e3,
0x119e4, 0x11a00, 0x11a3e, 0x11a47, 0x11a47, 0x11a50, 0x11a99, 0x11a9d,
0x11a9d, 0x11ab0, 0x11af8, 0x11c00, 0x11c08, 0x11c0a, 0x11c36, 0x11c38,
0x11c40, 0x11c50, 0x11c59, 0x11c72, 0x11c8f, 0x11c92, 0x11ca7, 0x11ca9,
0x11cb6, 0x11d00, 0x11d06, 0x11d08, 0x11d09, 0x11d0b, 0x11d36, 0x11d3a,
0x11d3a, 0x11d3c, 0x11d3d, 0x11d3f, 0x11d47, 0x11d50, 0x11d59, 0x11d60,
0x11d65, 0x11d67, 0x11d68, 0x11d6a, 0x11d8e, 0x11d90, 0x11d91, 0x11d93,
0x11d98, 0x11da0, 0x11da9, 0x11ee0, 0x11ef6, 0x11fb0, 0x11fb0, 0x11fdd,
0x11fe0, 0x12000, 0x12399, 0x12400, 0x1246e, 0x12480, 0x12543, 0x12f90,
0x12ff0, 0x13000, 0x1342e, 0x13430, 0x13438, 0x14400, 0x14646, 0x16800,
0x16a38, 0x16a40, 0x16a5e, 0x16a60, 0x16a69, 0x16a70, 0x16abe, 0x16ac0,
0x16ac9, 0x16ad0, 0x16aed, 0x16af0, 0x16af4, 0x16b00, 0x16b36, 0x16b40,
0x16b43, 0x16b50, 0x16b59, 0x16b63, 0x16b77, 0x16b7d, 0x16b8f, 0x16e40,
0x16e7f, 0x16f00, 0x16f4a, 0x16f4f, 0x16f87, 0x16f8f, 0x16f9f, 0x16fe0,
0x16fe1, 0x16fe3, 0x16fe4, 0x16ff0, 0x16ff1, 0x17000, 0x187f7, 0x18800,
0x18cd5, 0x18d00, 0x18d08, 0x1aff0, 0x1aff3, 0x1aff5, 0x1affb, 0x1affd,
0x1affe, 0x1b000, 0x1b122, 0x1b150, 0x1b152, 0x1b164, 0x1b167, 0x1b170,
0x1b2fb, 0x1bc00, 0x1bc6a, 0x1bc70, 0x1bc7c, 0x1bc80, 0x1bc88, 0x1bc90,
0x1bc99, 0x1bc9d, 0x1bc9e, 0x1bca0, 0x1bca3, 0x1cf00, 0x1cf2d, 0x1cf30,
0x1cf46, 0x1d165, 0x1d169, 0x1d16d, 0x1d182, 0x1d185, 0x1d18b, 0x1d1aa,
0x1d1ad, 0x1d242, 0x1d244, 0x1d400, 0x1d454, 0x1d456, 0x1d49c, 0x1d49e,
0x1d49f, 0x1d4a2, 0x1d4a2, 0x1d4a5, 0x1d4a6, 0x1d4a9, 0x1d4ac, 0x1d4ae,
0x1d4b9, 0x1d4bb, 0x1d4bb, 0x1d4bd, 0x1d4c3, 0x1d4c5, 0x1d505, 0x1d507,
0x1d50a, 0x1d50d, 0x1d514, 0x1d516, 0x1d51c, 0x1d51e, 0x1d539, 0x1d53b,
0x1d53e, 0x1d540, 0x1d544, 0x1d546, 0x1d546, 0x1d54a, 0x1d550, 0x1d552,
0x1d6a5, 0x1d6a8, 0x1d6c0, 0x1d6c2, 0x1d6da, 0x1d6dc, 0x1d6fa, 0x1d6fc,
0x1d714, 0x1d716, 0x1d734, 0x1d736, 0x1d74e, 0x1d750, 0x1d76e, 0x1d770,
0x1d788, 0x1d78a, 0x1d7a8, 0x1d7aa, 0x1d7c2, 0x1d7c4, 0x1d7cb, 0x1d7ce,
0x1d7ff, 0x1da00, 0x1da36, 0x1da3b, 0x1da6c, 0x1da75, 0x1da75, 0x1da84,
0x1da84, 0x1da9b, 0x1da9f, 0x1daa1, 0x1daaf, 0x1df00, 0x1df1e, 0x1e000,
0x1e006, 0x1e008, 0x1e018, 0x1e01b, 0x1e021, 0x1e023, 0x1e024, 0x1e026,
0x1e02a, 0x1e100, 0x1e12c, 0x1e130, 0x1e13d, 0x1e140, 0x1e149, 0x1e14e,
0x1e14e, 0x1e290, 0x1e2ae, 0x1e2c0, 0x1e2f9, 0x1e2ff, 0x1e2ff, 0x1e7e0,
0x1e7e6, 0x1e7e8, 0x1e7eb, 0x1e7ed, 0x1e7ee, 0x1e7f0, 0x1e7fe, 0x1e800,
0x1e8c4, 0x1e8d0, 0x1e8d6, 0x1e900, 0x1e94b, 0x1e950, 0x1e959, 0x1ecb0,
0x1ecb0, 0x1ee00, 0x1ee03, 0x1ee05, 0x1ee1f, 0x1ee21, 0x1ee22, 0x1ee24,
0x1ee24, 0x1ee27, 0x1ee27, 0x1ee29, 0x1ee32, 0x1ee34, 0x1ee37, 0x1ee39,
0x1ee39, 0x1ee3b, 0x1ee3b, 0x1ee42, 0x1ee42, 0x1ee47, 0x1ee47, 0x1ee49,
0x1ee49, 0x1ee4b, 0x1ee4b, 0x1ee4d, 0x1ee4f, 0x1ee51, 0x1ee52, 0x1ee54,
0x1ee54, 0x1ee57, 0x1ee57, 0x1ee59, 0x1ee59, 0x1ee5b, 0x1ee5b, 0x1ee5d,
0x1ee5d, 0x1ee5f, 0x1ee5f, 0x1ee61, 0x1ee62, 0x1ee64, 0x1ee64, 0x1ee67,
0x1ee6a, 0x1ee6c, 0x1ee72, 0x1ee74, 0x1ee77, 0x1ee79, 0x1ee7c, 0x1ee7e,
0x1ee7e, 0x1ee80, 0x1ee89, 0x1ee8b, 0x1ee9b, 0x1eea1, 0x1eea3, 0x1eea5,
0x1eea9, 0x1eeab, 0x1eebb, 0x1fbf0, 0x1fbf9, 0x20000, 0x2a6df, 0x2a700,
0x2b738, 0x2b740, 0x2b81d, 0x2b820, 0x2cea1, 0x2ceb0, 0x2ebe0, 0x2f800,
0x2fa1d, 0x30000, 0x3134a, 0xe0001, 0xe0001, 0xe0020, 0xe007f, 0xe0100,
0xe01ef};
}
private static final int[] NFA_MOVES_TEMPLATE_TEXT_43 = NFA_MOVES_TEMPLATE_TEXT_43_init();
private static int[] NFA_MOVES_TEMPLATE_TEXT_43_init() {
return new int[] {'$', '$', 'A', 'Z', '_', '_', 'a', 'z', 0xa2, 0xa5,
0xaa, 0xaa, 0xb5, 0xb5, 0xba, 0xba, 0xc0, 0xd6, 0xd8, 0xf6, 0xf8, 0x2c1,
0x2c6, 0x2d1, 0x2e0, 0x2e4, 0x2ec, 0x2ec, 0x2ee, 0x2ee, 0x370, 0x374,
0x376, 0x377, 0x37a, 0x37d, 0x37f, 0x37f, 0x386, 0x386, 0x388, 0x38a,
0x38c, 0x38c, 0x38e, 0x3a1, 0x3a3, 0x3f5, 0x3f7, 0x481, 0x48a, 0x52f,
0x531, 0x556, 0x559, 0x559, 0x560, 0x588, 0x58f, 0x58f, 0x5d0, 0x5ea,
0x5ef, 0x5f2, 0x60b, 0x60b, 0x620, 0x64a, 0x66e, 0x66f, 0x671, 0x6d3,
0x6d5, 0x6d5, 0x6e5, 0x6e6, 0x6ee, 0x6ef, 0x6fa, 0x6fc, 0x6ff, 0x6ff,
0x710, 0x710, 0x712, 0x72f, 0x74d, 0x7a5, 0x7b1, 0x7b1, 0x7ca, 0x7ea,
0x7f4, 0x7f5, 0x7fa, 0x7fa, 0x7fe, 0x815, 0x81a, 0x81a, 0x824, 0x824,
0x828, 0x828, 0x840, 0x858, 0x860, 0x86a, 0x870, 0x887, 0x889, 0x88e,
0x8a0, 0x8c9, 0x904, 0x939, 0x93d, 0x93d, 0x950, 0x950, 0x958, 0x961,
0x971, 0x980, 0x985, 0x98c, 0x98f, 0x990, 0x993, 0x9a8, 0x9aa, 0x9b0,
0x9b2, 0x9b2, 0x9b6, 0x9b9, 0x9bd, 0x9bd, 0x9ce, 0x9ce, 0x9dc, 0x9dd,
0x9df, 0x9e1, 0x9f0, 0x9f3, 0x9fb, 0x9fc, 0xa05, 0xa0a, 0xa0f, 0xa10,
0xa13, 0xa28, 0xa2a, 0xa30, 0xa32, 0xa33, 0xa35, 0xa36, 0xa38, 0xa39,
0xa59, 0xa5c, 0xa5e, 0xa5e, 0xa72, 0xa74, 0xa85, 0xa8d, 0xa8f, 0xa91,
0xa93, 0xaa8, 0xaaa, 0xab0, 0xab2, 0xab3, 0xab5, 0xab9, 0xabd, 0xabd,
0xad0, 0xad0, 0xae0, 0xae1, 0xaf1, 0xaf1, 0xaf9, 0xaf9, 0xb05, 0xb0c,
0xb0f, 0xb10, 0xb13, 0xb28, 0xb2a, 0xb30, 0xb32, 0xb33, 0xb35, 0xb39,
0xb3d, 0xb3d, 0xb5c, 0xb5d, 0xb5f, 0xb61, 0xb71, 0xb71, 0xb83, 0xb83,
0xb85, 0xb8a, 0xb8e, 0xb90, 0xb92, 0xb95, 0xb99, 0xb9a, 0xb9c, 0xb9c,
0xb9e, 0xb9f, 0xba3, 0xba4, 0xba8, 0xbaa, 0xbae, 0xbb9, 0xbd0, 0xbd0,
0xbf9, 0xbf9, 0xc05, 0xc0c, 0xc0e, 0xc10, 0xc12, 0xc28, 0xc2a, 0xc39,
0xc3d, 0xc3d, 0xc58, 0xc5a, 0xc5d, 0xc5d, 0xc60, 0xc61, 0xc80, 0xc80,
0xc85, 0xc8c, 0xc8e, 0xc90, 0xc92, 0xca8, 0xcaa, 0xcb3, 0xcb5, 0xcb9,
0xcbd, 0xcbd, 0xcdd, 0xcde, 0xce0, 0xce1, 0xcf1, 0xcf2, 0xd04, 0xd0c,
0xd0e, 0xd10, 0xd12, 0xd3a, 0xd3d, 0xd3d, 0xd4e, 0xd4e, 0xd54, 0xd56,
0xd5f, 0xd61, 0xd7a, 0xd7f, 0xd85, 0xd96, 0xd9a, 0xdb1, 0xdb3, 0xdbb,
0xdbd, 0xdbd, 0xdc0, 0xdc6, 0xe01, 0xe30, 0xe32, 0xe33, 0xe3f, 0xe46,
0xe81, 0xe82, 0xe84, 0xe84, 0xe86, 0xe8a, 0xe8c, 0xea3, 0xea5, 0xea5,
0xea7, 0xeb0, 0xeb2, 0xeb3, 0xebd, 0xebd, 0xec0, 0xec4, 0xec6, 0xec6,
0xedc, 0xedf, 0xf00, 0xf00, 0xf40, 0xf47, 0xf49, 0xf6c, 0xf88, 0xf8c,
0x1000, 0x102a, 0x103f, 0x103f, 0x1050, 0x1055, 0x105a, 0x105d, 0x1061,
0x1061, 0x1065, 0x1066, 0x106e, 0x1070, 0x1075, 0x1081, 0x108e, 0x108e,
0x10a0, 0x10c5, 0x10c7, 0x10c7, 0x10cd, 0x10cd, 0x10d0, 0x10fa, 0x10fc,
0x1248, 0x124a, 0x124d, 0x1250, 0x1256, 0x1258, 0x1258, 0x125a, 0x125d,
0x1260, 0x1288, 0x128a, 0x128d, 0x1290, 0x12b0, 0x12b2, 0x12b5, 0x12b8,
0x12be, 0x12c0, 0x12c0, 0x12c2, 0x12c5, 0x12c8, 0x12d6, 0x12d8, 0x1310,
0x1312, 0x1315, 0x1318, 0x135a, 0x1380, 0x138f, 0x13a0, 0x13f5, 0x13f8,
0x13fd, 0x1401, 0x166c, 0x166f, 0x167f, 0x1681, 0x169a, 0x16a0, 0x16ea,
0x16ee, 0x16f8, 0x1700, 0x1711, 0x171f, 0x1731, 0x1740, 0x1751, 0x1760,
0x176c, 0x176e, 0x1770, 0x1780, 0x17b3, 0x17d7, 0x17d7, 0x17db, 0x17dc,
0x1820, 0x1878, 0x1880, 0x1884, 0x1887, 0x18a8, 0x18aa, 0x18aa, 0x18b0,
0x18f5, 0x1900, 0x191e, 0x1950, 0x196d, 0x1970, 0x1974, 0x1980, 0x19ab,
0x19b0, 0x19c9, 0x1a00, 0x1a16, 0x1a20, 0x1a54, 0x1aa7, 0x1aa7, 0x1b05,
0x1b33, 0x1b45, 0x1b4c, 0x1b83, 0x1ba0, 0x1bae, 0x1baf, 0x1bba, 0x1be5,
0x1c00, 0x1c23, 0x1c4d, 0x1c4f, 0x1c5a, 0x1c7d, 0x1c80, 0x1c88, 0x1c90,
0x1cba, 0x1cbd, 0x1cbf, 0x1ce9, 0x1cec, 0x1cee, 0x1cf3, 0x1cf5, 0x1cf6,
0x1cfa, 0x1cfa, 0x1d00, 0x1dbf, 0x1e00, 0x1f15, 0x1f18, 0x1f1d, 0x1f20,
0x1f45, 0x1f48, 0x1f4d, 0x1f50, 0x1f57, 0x1f59, 0x1f59, 0x1f5b, 0x1f5b,
0x1f5d, 0x1f5d, 0x1f5f, 0x1f7d, 0x1f80, 0x1fb4, 0x1fb6, 0x1fbc, 0x1fbe,
0x1fbe, 0x1fc2, 0x1fc4, 0x1fc6, 0x1fcc, 0x1fd0, 0x1fd3, 0x1fd6, 0x1fdb,
0x1fe0, 0x1fec, 0x1ff2, 0x1ff4, 0x1ff6, 0x1ffc, 0x203f, 0x2040, 0x2054,
0x2054, 0x2071, 0x2071, 0x207f, 0x207f, 0x2090, 0x209c, 0x20a0, 0x20c0,
0x2102, 0x2102, 0x2107, 0x2107, 0x210a, 0x2113, 0x2115, 0x2115, 0x2119,
0x211d, 0x2124, 0x2124, 0x2126, 0x2126, 0x2128, 0x2128, 0x212a, 0x212d,
0x212f, 0x2139, 0x213c, 0x213f, 0x2145, 0x2149, 0x214e, 0x214e, 0x2160,
0x2188, 0x2c00, 0x2ce4, 0x2ceb, 0x2cee, 0x2cf2, 0x2cf3, 0x2d00, 0x2d25,
0x2d27, 0x2d27, 0x2d2d, 0x2d2d, 0x2d30, 0x2d67, 0x2d6f, 0x2d6f, 0x2d80,
0x2d96, 0x2da0, 0x2da6, 0x2da8, 0x2dae, 0x2db0, 0x2db6, 0x2db8, 0x2dbe,
0x2dc0, 0x2dc6, 0x2dc8, 0x2dce, 0x2dd0, 0x2dd6, 0x2dd8, 0x2dde, 0x2e2f,
0x2e2f, 0x3005, 0x3007, 0x3021, 0x3029, 0x3031, 0x3035, 0x3038, 0x303c,
0x3041, 0x3096, 0x309d, 0x309f, 0x30a1, 0x30fa, 0x30fc, 0x30ff, 0x3105,
0x312f, 0x3131, 0x318e, 0x31a0, 0x31bf, 0x31f0, 0x31ff, 0x3400, 0x4dbf,
0x4e00, 0xa48c, 0xa4d0, 0xa4fd, 0xa500, 0xa60c, 0xa610, 0xa61f, 0xa62a,
0xa62b, 0xa640, 0xa66e, 0xa67f, 0xa69d, 0xa6a0, 0xa6ef, 0xa717, 0xa71f,
0xa722, 0xa788, 0xa78b, 0xa7ca, 0xa7d0, 0xa7d1, 0xa7d3, 0xa7d3, 0xa7d5,
0xa7d9, 0xa7f2, 0xa801, 0xa803, 0xa805, 0xa807, 0xa80a, 0xa80c, 0xa822,
0xa838, 0xa838, 0xa840, 0xa873, 0xa882, 0xa8b3, 0xa8f2, 0xa8f7, 0xa8fb,
0xa8fb, 0xa8fd, 0xa8fe, 0xa90a, 0xa925, 0xa930, 0xa946, 0xa960, 0xa97c,
0xa984, 0xa9b2, 0xa9cf, 0xa9cf, 0xa9e0, 0xa9e4, 0xa9e6, 0xa9ef, 0xa9fa,
0xa9fe, 0xaa00, 0xaa28, 0xaa40, 0xaa42, 0xaa44, 0xaa4b, 0xaa60, 0xaa76,
0xaa7a, 0xaa7a, 0xaa7e, 0xaaaf, 0xaab1, 0xaab1, 0xaab5, 0xaab6, 0xaab9,
0xaabd, 0xaac0, 0xaac0, 0xaac2, 0xaac2, 0xaadb, 0xaadd, 0xaae0, 0xaaea,
0xaaf2, 0xaaf4, 0xab01, 0xab06, 0xab09, 0xab0e, 0xab11, 0xab16, 0xab20,
0xab26, 0xab28, 0xab2e, 0xab30, 0xab5a, 0xab5c, 0xab69, 0xab70, 0xabe2,
0xac00, 0xd7a3, 0xd7b0, 0xd7c6, 0xd7cb, 0xd7fb, 0xf900, 0xfa6d, 0xfa70,
0xfad9, 0xfb00, 0xfb06, 0xfb13, 0xfb17, 0xfb1d, 0xfb1d, 0xfb1f, 0xfb28,
0xfb2a, 0xfb36, 0xfb38, 0xfb3c, 0xfb3e, 0xfb3e, 0xfb40, 0xfb41, 0xfb43,
0xfb44, 0xfb46, 0xfbb1, 0xfbd3, 0xfd3d, 0xfd50, 0xfd8f, 0xfd92, 0xfdc7,
0xfdf0, 0xfdfc, 0xfe33, 0xfe34, 0xfe4d, 0xfe4f, 0xfe69, 0xfe69, 0xfe70,
0xfe74, 0xfe76, 0xfefc, 0xff04, 0xff04, 0xff21, 0xff3a, 0xff3f, 0xff3f,
0xff41, 0xff5a, 0xff66, 0xffbe, 0xffc2, 0xffc7, 0xffca, 0xffcf, 0xffd2,
0xffd7, 0xffda, 0xffdc, 0xffe0, 0xffe1, 0xffe5, 0xffe6, 0x10000, 0x1000b,
0x1000d, 0x10026, 0x10028, 0x1003a, 0x1003c, 0x1003d, 0x1003f, 0x1004d,
0x10050, 0x1005d, 0x10080, 0x100fa, 0x10140, 0x10174, 0x10280, 0x1029c,
0x102a0, 0x102d0, 0x10300, 0x1031f, 0x1032d, 0x1034a, 0x10350, 0x10375,
0x10380, 0x1039d, 0x103a0, 0x103c3, 0x103c8, 0x103cf, 0x103d1, 0x103d5,
0x10400, 0x1049d, 0x104b0, 0x104d3, 0x104d8, 0x104fb, 0x10500, 0x10527,
0x10530, 0x10563, 0x10570, 0x1057a, 0x1057c, 0x1058a, 0x1058c, 0x10592,
0x10594, 0x10595, 0x10597, 0x105a1, 0x105a3, 0x105b1, 0x105b3, 0x105b9,
0x105bb, 0x105bc, 0x10600, 0x10736, 0x10740, 0x10755, 0x10760, 0x10767,
0x10780, 0x10785, 0x10787, 0x107b0, 0x107b2, 0x107ba, 0x10800, 0x10805,
0x10808, 0x10808, 0x1080a, 0x10835, 0x10837, 0x10838, 0x1083c, 0x1083c,
0x1083f, 0x10855, 0x10860, 0x10876, 0x10880, 0x1089e, 0x108e0, 0x108f2,
0x108f4, 0x108f5, 0x10900, 0x10915, 0x10920, 0x10939, 0x10980, 0x109b7,
0x109be, 0x109bf, 0x10a00, 0x10a00, 0x10a10, 0x10a13, 0x10a15, 0x10a17,
0x10a19, 0x10a35, 0x10a60, 0x10a7c, 0x10a80, 0x10a9c, 0x10ac0, 0x10ac7,
0x10ac9, 0x10ae4, 0x10b00, 0x10b35, 0x10b40, 0x10b55, 0x10b60, 0x10b72,
0x10b80, 0x10b91, 0x10c00, 0x10c48, 0x10c80, 0x10cb2, 0x10cc0, 0x10cf2,
0x10d00, 0x10d23, 0x10e80, 0x10ea9, 0x10eb0, 0x10eb1, 0x10f00, 0x10f1c,
0x10f27, 0x10f27, 0x10f30, 0x10f45, 0x10f70, 0x10f81, 0x10fb0, 0x10fc4,
0x10fe0, 0x10ff6, 0x11003, 0x11037, 0x11071, 0x11072, 0x11075, 0x11075,
0x11083, 0x110af, 0x110d0, 0x110e8, 0x11103, 0x11126, 0x11144, 0x11144,
0x11147, 0x11147, 0x11150, 0x11172, 0x11176, 0x11176, 0x11183, 0x111b2,
0x111c1, 0x111c4, 0x111da, 0x111da, 0x111dc, 0x111dc, 0x11200, 0x11211,
0x11213, 0x1122b, 0x11280, 0x11286, 0x11288, 0x11288, 0x1128a, 0x1128d,
0x1128f, 0x1129d, 0x1129f, 0x112a8, 0x112b0, 0x112de, 0x11305, 0x1130c,
0x1130f, 0x11310, 0x11313, 0x11328, 0x1132a, 0x11330, 0x11332, 0x11333,
0x11335, 0x11339, 0x1133d, 0x1133d, 0x11350, 0x11350, 0x1135d, 0x11361,
0x11400, 0x11434, 0x11447, 0x1144a, 0x1145f, 0x11461, 0x11480, 0x114af,
0x114c4, 0x114c5, 0x114c7, 0x114c7, 0x11580, 0x115ae, 0x115d8, 0x115db,
0x11600, 0x1162f, 0x11644, 0x11644, 0x11680, 0x116aa, 0x116b8, 0x116b8,
0x11700, 0x1171a, 0x11740, 0x11746, 0x11800, 0x1182b, 0x118a0, 0x118df,
0x118ff, 0x11906, 0x11909, 0x11909, 0x1190c, 0x11913, 0x11915, 0x11916,
0x11918, 0x1192f, 0x1193f, 0x1193f, 0x11941, 0x11941, 0x119a0, 0x119a7,
0x119aa, 0x119d0, 0x119e1, 0x119e1, 0x119e3, 0x119e3, 0x11a00, 0x11a00,
0x11a0b, 0x11a32, 0x11a3a, 0x11a3a, 0x11a50, 0x11a50, 0x11a5c, 0x11a89,
0x11a9d, 0x11a9d, 0x11ab0, 0x11af8, 0x11c00, 0x11c08, 0x11c0a, 0x11c2e,
0x11c40, 0x11c40, 0x11c72, 0x11c8f, 0x11d00, 0x11d06, 0x11d08, 0x11d09,
0x11d0b, 0x11d30, 0x11d46, 0x11d46, 0x11d60, 0x11d65, 0x11d67, 0x11d68,
0x11d6a, 0x11d89, 0x11d98, 0x11d98, 0x11ee0, 0x11ef2, 0x11fb0, 0x11fb0,
0x11fdd, 0x11fe0, 0x12000, 0x12399, 0x12400, 0x1246e, 0x12480, 0x12543,
0x12f90, 0x12ff0, 0x13000, 0x1342e, 0x14400, 0x14646, 0x16800, 0x16a38,
0x16a40, 0x16a5e, 0x16a70, 0x16abe, 0x16ad0, 0x16aed, 0x16b00, 0x16b2f,
0x16b40, 0x16b43, 0x16b63, 0x16b77, 0x16b7d, 0x16b8f, 0x16e40, 0x16e7f,
0x16f00, 0x16f4a, 0x16f50, 0x16f50, 0x16f93, 0x16f9f, 0x16fe0, 0x16fe1,
0x16fe3, 0x16fe3, 0x17000, 0x187f7, 0x18800, 0x18cd5, 0x18d00, 0x18d08,
0x1aff0, 0x1aff3, 0x1aff5, 0x1affb, 0x1affd, 0x1affe, 0x1b000, 0x1b122,
0x1b150, 0x1b152, 0x1b164, 0x1b167, 0x1b170, 0x1b2fb, 0x1bc00, 0x1bc6a,
0x1bc70, 0x1bc7c, 0x1bc80, 0x1bc88, 0x1bc90, 0x1bc99, 0x1d400, 0x1d454,
0x1d456, 0x1d49c, 0x1d49e, 0x1d49f, 0x1d4a2, 0x1d4a2, 0x1d4a5, 0x1d4a6,
0x1d4a9, 0x1d4ac, 0x1d4ae, 0x1d4b9, 0x1d4bb, 0x1d4bb, 0x1d4bd, 0x1d4c3,
0x1d4c5, 0x1d505, 0x1d507, 0x1d50a, 0x1d50d, 0x1d514, 0x1d516, 0x1d51c,
0x1d51e, 0x1d539, 0x1d53b, 0x1d53e, 0x1d540, 0x1d544, 0x1d546, 0x1d546,
0x1d54a, 0x1d550, 0x1d552, 0x1d6a5, 0x1d6a8, 0x1d6c0, 0x1d6c2, 0x1d6da,
0x1d6dc, 0x1d6fa, 0x1d6fc, 0x1d714, 0x1d716, 0x1d734, 0x1d736, 0x1d74e,
0x1d750, 0x1d76e, 0x1d770, 0x1d788, 0x1d78a, 0x1d7a8, 0x1d7aa, 0x1d7c2,
0x1d7c4, 0x1d7cb, 0x1df00, 0x1df1e, 0x1e100, 0x1e12c, 0x1e137, 0x1e13d,
0x1e14e, 0x1e14e, 0x1e290, 0x1e2ad, 0x1e2c0, 0x1e2eb, 0x1e2ff, 0x1e2ff,
0x1e7e0, 0x1e7e6, 0x1e7e8, 0x1e7eb, 0x1e7ed, 0x1e7ee, 0x1e7f0, 0x1e7fe,
0x1e800, 0x1e8c4, 0x1e900, 0x1e943, 0x1e94b, 0x1e94b, 0x1ecb0, 0x1ecb0,
0x1ee00, 0x1ee03, 0x1ee05, 0x1ee1f, 0x1ee21, 0x1ee22, 0x1ee24, 0x1ee24,
0x1ee27, 0x1ee27, 0x1ee29, 0x1ee32, 0x1ee34, 0x1ee37, 0x1ee39, 0x1ee39,
0x1ee3b, 0x1ee3b, 0x1ee42, 0x1ee42, 0x1ee47, 0x1ee47, 0x1ee49, 0x1ee49,
0x1ee4b, 0x1ee4b, 0x1ee4d, 0x1ee4f, 0x1ee51, 0x1ee52, 0x1ee54, 0x1ee54,
0x1ee57, 0x1ee57, 0x1ee59, 0x1ee59, 0x1ee5b, 0x1ee5b, 0x1ee5d, 0x1ee5d,
0x1ee5f, 0x1ee5f, 0x1ee61, 0x1ee62, 0x1ee64, 0x1ee64, 0x1ee67, 0x1ee6a,
0x1ee6c, 0x1ee72, 0x1ee74, 0x1ee77, 0x1ee79, 0x1ee7c, 0x1ee7e, 0x1ee7e,
0x1ee80, 0x1ee89, 0x1ee8b, 0x1ee9b, 0x1eea1, 0x1eea3, 0x1eea5, 0x1eea9,
0x1eeab, 0x1eebb, 0x20000, 0x2a6df, 0x2a700, 0x2b738, 0x2b740, 0x2b81d,
0x2b820, 0x2cea1, 0x2ceb0, 0x2ebe0, 0x2f800, 0x2fa1d, 0x30000, 0x3134a};
}
private static final int[] NFA_MOVES_TEMPLATE_TEXT_45 = NFA_MOVES_TEMPLATE_TEXT_45_init();
private static int[] NFA_MOVES_TEMPLATE_TEXT_45_init() {
return new int[] {0x0, 0x8, 0xe, 0x1b, '$', '$', '0', '9', 'A', 'Z', '_',
'_', 'a', 'z', 0x7f, 0x9f, 0xa2, 0xa5, 0xaa, 0xaa, 0xad, 0xad, 0xb5, 0xb5,
0xba, 0xba, 0xc0, 0xd6, 0xd8, 0xf6, 0xf8, 0x2c1, 0x2c6, 0x2d1, 0x2e0,
0x2e4, 0x2ec, 0x2ec, 0x2ee, 0x2ee, 0x300, 0x374, 0x376, 0x377, 0x37a,
0x37d, 0x37f, 0x37f, 0x386, 0x386, 0x388, 0x38a, 0x38c, 0x38c, 0x38e,
0x3a1, 0x3a3, 0x3f5, 0x3f7, 0x481, 0x483, 0x487, 0x48a, 0x52f, 0x531,
0x556, 0x559, 0x559, 0x560, 0x588, 0x58f, 0x58f, 0x591, 0x5bd, 0x5bf,
0x5bf, 0x5c1, 0x5c2, 0x5c4, 0x5c5, 0x5c7, 0x5c7, 0x5d0, 0x5ea, 0x5ef,
0x5f2, 0x600, 0x605, 0x60b, 0x60b, 0x610, 0x61a, 0x61c, 0x61c, 0x620,
0x669, 0x66e, 0x6d3, 0x6d5, 0x6dd, 0x6df, 0x6e8, 0x6ea, 0x6fc, 0x6ff,
0x6ff, 0x70f, 0x74a, 0x74d, 0x7b1, 0x7c0, 0x7f5, 0x7fa, 0x7fa, 0x7fd,
0x82d, 0x840, 0x85b, 0x860, 0x86a, 0x870, 0x887, 0x889, 0x88e, 0x890,
0x891, 0x898, 0x963, 0x966, 0x96f, 0x971, 0x983, 0x985, 0x98c, 0x98f,
0x990, 0x993, 0x9a8, 0x9aa, 0x9b0, 0x9b2, 0x9b2, 0x9b6, 0x9b9, 0x9bc,
0x9c4, 0x9c7, 0x9c8, 0x9cb, 0x9ce, 0x9d7, 0x9d7, 0x9dc, 0x9dd, 0x9df,
0x9e3, 0x9e6, 0x9f3, 0x9fb, 0x9fc, 0x9fe, 0x9fe, 0xa01, 0xa03, 0xa05,
0xa0a, 0xa0f, 0xa10, 0xa13, 0xa28, 0xa2a, 0xa30, 0xa32, 0xa33, 0xa35,
0xa36, 0xa38, 0xa39, 0xa3c, 0xa3c, 0xa3e, 0xa42, 0xa47, 0xa48, 0xa4b,
0xa4d, 0xa51, 0xa51, 0xa59, 0xa5c, 0xa5e, 0xa5e, 0xa66, 0xa75, 0xa81,
0xa83, 0xa85, 0xa8d, 0xa8f, 0xa91, 0xa93, 0xaa8, 0xaaa, 0xab0, 0xab2,
0xab3, 0xab5, 0xab9, 0xabc, 0xac5, 0xac7, 0xac9, 0xacb, 0xacd, 0xad0,
0xad0, 0xae0, 0xae3, 0xae6, 0xaef, 0xaf1, 0xaf1, 0xaf9, 0xaff, 0xb01,
0xb03, 0xb05, 0xb0c, 0xb0f, 0xb10, 0xb13, 0xb28, 0xb2a, 0xb30, 0xb32,
0xb33, 0xb35, 0xb39, 0xb3c, 0xb44, 0xb47, 0xb48, 0xb4b, 0xb4d, 0xb55,
0xb57, 0xb5c, 0xb5d, 0xb5f, 0xb63, 0xb66, 0xb6f, 0xb71, 0xb71, 0xb82,
0xb83, 0xb85, 0xb8a, 0xb8e, 0xb90, 0xb92, 0xb95, 0xb99, 0xb9a, 0xb9c,
0xb9c, 0xb9e, 0xb9f, 0xba3, 0xba4, 0xba8, 0xbaa, 0xbae, 0xbb9, 0xbbe,
0xbc2, 0xbc6, 0xbc8, 0xbca, 0xbcd, 0xbd0, 0xbd0, 0xbd7, 0xbd7, 0xbe6,
0xbef, 0xbf9, 0xbf9, 0xc00, 0xc0c, 0xc0e, 0xc10, 0xc12, 0xc28, 0xc2a,
0xc39, 0xc3c, 0xc44, 0xc46, 0xc48, 0xc4a, 0xc4d, 0xc55, 0xc56, 0xc58,
0xc5a, 0xc5d, 0xc5d, 0xc60, 0xc63, 0xc66, 0xc6f, 0xc80, 0xc83, 0xc85,
0xc8c, 0xc8e, 0xc90, 0xc92, 0xca8, 0xcaa, 0xcb3, 0xcb5, 0xcb9, 0xcbc,
0xcc4, 0xcc6, 0xcc8, 0xcca, 0xccd, 0xcd5, 0xcd6, 0xcdd, 0xcde, 0xce0,
0xce3, 0xce6, 0xcef, 0xcf1, 0xcf2, 0xd00, 0xd0c, 0xd0e, 0xd10, 0xd12,
0xd44, 0xd46, 0xd48, 0xd4a, 0xd4e, 0xd54, 0xd57, 0xd5f, 0xd63, 0xd66,
0xd6f, 0xd7a, 0xd7f, 0xd81, 0xd83, 0xd85, 0xd96, 0xd9a, 0xdb1, 0xdb3,
0xdbb, 0xdbd, 0xdbd, 0xdc0, 0xdc6, 0xdca, 0xdca, 0xdcf, 0xdd4, 0xdd6,
0xdd6, 0xdd8, 0xddf, 0xde6, 0xdef, 0xdf2, 0xdf3, 0xe01, 0xe3a, 0xe3f,
0xe4e, 0xe50, 0xe59, 0xe81, 0xe82, 0xe84, 0xe84, 0xe86, 0xe8a, 0xe8c,
0xea3, 0xea5, 0xea5, 0xea7, 0xebd, 0xec0, 0xec4, 0xec6, 0xec6, 0xec8,
0xecd, 0xed0, 0xed9, 0xedc, 0xedf, 0xf00, 0xf00, 0xf18, 0xf19, 0xf20,
0xf29, 0xf35, 0xf35, 0xf37, 0xf37, 0xf39, 0xf39, 0xf3e, 0xf47, 0xf49,
0xf6c, 0xf71, 0xf84, 0xf86, 0xf97, 0xf99, 0xfbc, 0xfc6, 0xfc6, 0x1000,
0x1049, 0x1050, 0x109d, 0x10a0, 0x10c5, 0x10c7, 0x10c7, 0x10cd, 0x10cd,
0x10d0, 0x10fa, 0x10fc, 0x1248, 0x124a, 0x124d, 0x1250, 0x1256, 0x1258,
0x1258, 0x125a, 0x125d, 0x1260, 0x1288, 0x128a, 0x128d, 0x1290, 0x12b0,
0x12b2, 0x12b5, 0x12b8, 0x12be, 0x12c0, 0x12c0, 0x12c2, 0x12c5, 0x12c8,
0x12d6, 0x12d8, 0x1310, 0x1312, 0x1315, 0x1318, 0x135a, 0x135d, 0x135f,
0x1380, 0x138f, 0x13a0, 0x13f5, 0x13f8, 0x13fd, 0x1401, 0x166c, 0x166f,
0x167f, 0x1681, 0x169a, 0x16a0, 0x16ea, 0x16ee, 0x16f8, 0x1700, 0x1715,
0x171f, 0x1734, 0x1740, 0x1753, 0x1760, 0x176c, 0x176e, 0x1770, 0x1772,
0x1773, 0x1780, 0x17d3, 0x17d7, 0x17d7, 0x17db, 0x17dd, 0x17e0, 0x17e9,
0x180b, 0x1819, 0x1820, 0x1878, 0x1880, 0x18aa, 0x18b0, 0x18f5, 0x1900,
0x191e, 0x1920, 0x192b, 0x1930, 0x193b, 0x1946, 0x196d, 0x1970, 0x1974,
0x1980, 0x19ab, 0x19b0, 0x19c9, 0x19d0, 0x19d9, 0x1a00, 0x1a1b, 0x1a20,
0x1a5e, 0x1a60, 0x1a7c, 0x1a7f, 0x1a89, 0x1a90, 0x1a99, 0x1aa7, 0x1aa7,
0x1ab0, 0x1abd, 0x1abf, 0x1ace, 0x1b00, 0x1b4c, 0x1b50, 0x1b59, 0x1b6b,
0x1b73, 0x1b80, 0x1bf3, 0x1c00, 0x1c37, 0x1c40, 0x1c49, 0x1c4d, 0x1c7d,
0x1c80, 0x1c88, 0x1c90, 0x1cba, 0x1cbd, 0x1cbf, 0x1cd0, 0x1cd2, 0x1cd4,
0x1cfa, 0x1d00, 0x1f15, 0x1f18, 0x1f1d, 0x1f20, 0x1f45, 0x1f48, 0x1f4d,
0x1f50, 0x1f57, 0x1f59, 0x1f59, 0x1f5b, 0x1f5b, 0x1f5d, 0x1f5d, 0x1f5f,
0x1f7d, 0x1f80, 0x1fb4, 0x1fb6, 0x1fbc, 0x1fbe, 0x1fbe, 0x1fc2, 0x1fc4,
0x1fc6, 0x1fcc, 0x1fd0, 0x1fd3, 0x1fd6, 0x1fdb, 0x1fe0, 0x1fec, 0x1ff2,
0x1ff4, 0x1ff6, 0x1ffc, 0x200b, 0x200f, 0x202a, 0x202e, 0x203f, 0x2040,
0x2054, 0x2054, 0x2060, 0x2064, 0x2066, 0x206f, 0x2071, 0x2071, 0x207f,
0x207f, 0x2090, 0x209c, 0x20a0, 0x20c0, 0x20d0, 0x20dc, 0x20e1, 0x20e1,
0x20e5, 0x20f0, 0x2102, 0x2102, 0x2107, 0x2107, 0x210a, 0x2113, 0x2115,
0x2115, 0x2119, 0x211d, 0x2124, 0x2124, 0x2126, 0x2126, 0x2128, 0x2128,
0x212a, 0x212d, 0x212f, 0x2139, 0x213c, 0x213f, 0x2145, 0x2149, 0x214e,
0x214e, 0x2160, 0x2188, 0x2c00, 0x2ce4, 0x2ceb, 0x2cf3, 0x2d00, 0x2d25,
0x2d27, 0x2d27, 0x2d2d, 0x2d2d, 0x2d30, 0x2d67, 0x2d6f, 0x2d6f, 0x2d7f,
0x2d96, 0x2da0, 0x2da6, 0x2da8, 0x2dae, 0x2db0, 0x2db6, 0x2db8, 0x2dbe,
0x2dc0, 0x2dc6, 0x2dc8, 0x2dce, 0x2dd0, 0x2dd6, 0x2dd8, 0x2dde, 0x2de0,
0x2dff, 0x2e2f, 0x2e2f, 0x3005, 0x3007, 0x3021, 0x302f, 0x3031, 0x3035,
0x3038, 0x303c, 0x3041, 0x3096, 0x3099, 0x309a, 0x309d, 0x309f, 0x30a1,
0x30fa, 0x30fc, 0x30ff, 0x3105, 0x312f, 0x3131, 0x318e, 0x31a0, 0x31bf,
0x31f0, 0x31ff, 0x3400, 0x4dbf, 0x4e00, 0xa48c, 0xa4d0, 0xa4fd, 0xa500,
0xa60c, 0xa610, 0xa62b, 0xa640, 0xa66f, 0xa674, 0xa67d, 0xa67f, 0xa6f1,
0xa717, 0xa71f, 0xa722, 0xa788, 0xa78b, 0xa7ca, 0xa7d0, 0xa7d1, 0xa7d3,
0xa7d3, 0xa7d5, 0xa7d9, 0xa7f2, 0xa827, 0xa82c, 0xa82c, 0xa838, 0xa838,
0xa840, 0xa873, 0xa880, 0xa8c5, 0xa8d0, 0xa8d9, 0xa8e0, 0xa8f7, 0xa8fb,
0xa8fb, 0xa8fd, 0xa92d, 0xa930, 0xa953, 0xa960, 0xa97c, 0xa980, 0xa9c0,
0xa9cf, 0xa9d9, 0xa9e0, 0xa9fe, 0xaa00, 0xaa36, 0xaa40, 0xaa4d, 0xaa50,
0xaa59, 0xaa60, 0xaa76, 0xaa7a, 0xaac2, 0xaadb, 0xaadd, 0xaae0, 0xaaef,
0xaaf2, 0xaaf6, 0xab01, 0xab06, 0xab09, 0xab0e, 0xab11, 0xab16, 0xab20,
0xab26, 0xab28, 0xab2e, 0xab30, 0xab5a, 0xab5c, 0xab69, 0xab70, 0xabea,
0xabec, 0xabed, 0xabf0, 0xabf9, 0xac00, 0xd7a3, 0xd7b0, 0xd7c6, 0xd7cb,
0xd7fb, 0xf900, 0xfa6d, 0xfa70, 0xfad9, 0xfb00, 0xfb06, 0xfb13, 0xfb17,
0xfb1d, 0xfb28, 0xfb2a, 0xfb36, 0xfb38, 0xfb3c, 0xfb3e, 0xfb3e, 0xfb40,
0xfb41, 0xfb43, 0xfb44, 0xfb46, 0xfbb1, 0xfbd3, 0xfd3d, 0xfd50, 0xfd8f,
0xfd92, 0xfdc7, 0xfdf0, 0xfdfc, 0xfe00, 0xfe0f, 0xfe20, 0xfe2f, 0xfe33,
0xfe34, 0xfe4d, 0xfe4f, 0xfe69, 0xfe69, 0xfe70, 0xfe74, 0xfe76, 0xfefc,
0xfeff, 0xfeff, 0xff04, 0xff04, 0xff10, 0xff19, 0xff21, 0xff3a, 0xff3f,
0xff3f, 0xff41, 0xff5a, 0xff66, 0xffbe, 0xffc2, 0xffc7, 0xffca, 0xffcf,
0xffd2, 0xffd7, 0xffda, 0xffdc, 0xffe0, 0xffe1, 0xffe5, 0xffe6, 0xfff9,
0xfffb, 0x10000, 0x1000b, 0x1000d, 0x10026, 0x10028, 0x1003a, 0x1003c,
0x1003d, 0x1003f, 0x1004d, 0x10050, 0x1005d, 0x10080, 0x100fa, 0x10140,
0x10174, 0x101fd, 0x101fd, 0x10280, 0x1029c, 0x102a0, 0x102d0, 0x102e0,
0x102e0, 0x10300, 0x1031f, 0x1032d, 0x1034a, 0x10350, 0x1037a, 0x10380,
0x1039d, 0x103a0, 0x103c3, 0x103c8, 0x103cf, 0x103d1, 0x103d5, 0x10400,
0x1049d, 0x104a0, 0x104a9, 0x104b0, 0x104d3, 0x104d8, 0x104fb, 0x10500,
0x10527, 0x10530, 0x10563, 0x10570, 0x1057a, 0x1057c, 0x1058a, 0x1058c,
0x10592, 0x10594, 0x10595, 0x10597, 0x105a1, 0x105a3, 0x105b1, 0x105b3,
0x105b9, 0x105bb, 0x105bc, 0x10600, 0x10736, 0x10740, 0x10755, 0x10760,
0x10767, 0x10780, 0x10785, 0x10787, 0x107b0, 0x107b2, 0x107ba, 0x10800,
0x10805, 0x10808, 0x10808, 0x1080a, 0x10835, 0x10837, 0x10838, 0x1083c,
0x1083c, 0x1083f, 0x10855, 0x10860, 0x10876, 0x10880, 0x1089e, 0x108e0,
0x108f2, 0x108f4, 0x108f5, 0x10900, 0x10915, 0x10920, 0x10939, 0x10980,
0x109b7, 0x109be, 0x109bf, 0x10a00, 0x10a03, 0x10a05, 0x10a06, 0x10a0c,
0x10a13, 0x10a15, 0x10a17, 0x10a19, 0x10a35, 0x10a38, 0x10a3a, 0x10a3f,
0x10a3f, 0x10a60, 0x10a7c, 0x10a80, 0x10a9c, 0x10ac0, 0x10ac7, 0x10ac9,
0x10ae6, 0x10b00, 0x10b35, 0x10b40, 0x10b55, 0x10b60, 0x10b72, 0x10b80,
0x10b91, 0x10c00, 0x10c48, 0x10c80, 0x10cb2, 0x10cc0, 0x10cf2, 0x10d00,
0x10d27, 0x10d30, 0x10d39, 0x10e80, 0x10ea9, 0x10eab, 0x10eac, 0x10eb0,
0x10eb1, 0x10f00, 0x10f1c, 0x10f27, 0x10f27, 0x10f30, 0x10f50, 0x10f70,
0x10f85, 0x10fb0, 0x10fc4, 0x10fe0, 0x10ff6, 0x11000, 0x11046, 0x11066,
0x11075, 0x1107f, 0x110ba, 0x110bd, 0x110bd, 0x110c2, 0x110c2, 0x110cd,
0x110cd, 0x110d0, 0x110e8, 0x110f0, 0x110f9, 0x11100, 0x11134, 0x11136,
0x1113f, 0x11144, 0x11147, 0x11150, 0x11173, 0x11176, 0x11176, 0x11180,
0x111c4, 0x111c9, 0x111cc, 0x111ce, 0x111da, 0x111dc, 0x111dc, 0x11200,
0x11211, 0x11213, 0x11237, 0x1123e, 0x1123e, 0x11280, 0x11286, 0x11288,
0x11288, 0x1128a, 0x1128d, 0x1128f, 0x1129d, 0x1129f, 0x112a8, 0x112b0,
0x112ea, 0x112f0, 0x112f9, 0x11300, 0x11303, 0x11305, 0x1130c, 0x1130f,
0x11310, 0x11313, 0x11328, 0x1132a, 0x11330, 0x11332, 0x11333, 0x11335,
0x11339, 0x1133b, 0x11344, 0x11347, 0x11348, 0x1134b, 0x1134d, 0x11350,
0x11350, 0x11357, 0x11357, 0x1135d, 0x11363, 0x11366, 0x1136c, 0x11370,
0x11374, 0x11400, 0x1144a, 0x11450, 0x11459, 0x1145e, 0x11461, 0x11480,
0x114c5, 0x114c7, 0x114c7, 0x114d0, 0x114d9, 0x11580, 0x115b5, 0x115b8,
0x115c0, 0x115d8, 0x115dd, 0x11600, 0x11640, 0x11644, 0x11644, 0x11650,
0x11659, 0x11680, 0x116b8, 0x116c0, 0x116c9, 0x11700, 0x1171a, 0x1171d,
0x1172b, 0x11730, 0x11739, 0x11740, 0x11746, 0x11800, 0x1183a, 0x118a0,
0x118e9, 0x118ff, 0x11906, 0x11909, 0x11909, 0x1190c, 0x11913, 0x11915,
0x11916, 0x11918, 0x11935, 0x11937, 0x11938, 0x1193b, 0x11943, 0x11950,
0x11959, 0x119a0, 0x119a7, 0x119aa, 0x119d7, 0x119da, 0x119e1, 0x119e3,
0x119e4, 0x11a00, 0x11a3e, 0x11a47, 0x11a47, 0x11a50, 0x11a99, 0x11a9d,
0x11a9d, 0x11ab0, 0x11af8, 0x11c00, 0x11c08, 0x11c0a, 0x11c36, 0x11c38,
0x11c40, 0x11c50, 0x11c59, 0x11c72, 0x11c8f, 0x11c92, 0x11ca7, 0x11ca9,
0x11cb6, 0x11d00, 0x11d06, 0x11d08, 0x11d09, 0x11d0b, 0x11d36, 0x11d3a,
0x11d3a, 0x11d3c, 0x11d3d, 0x11d3f, 0x11d47, 0x11d50, 0x11d59, 0x11d60,
0x11d65, 0x11d67, 0x11d68, 0x11d6a, 0x11d8e, 0x11d90, 0x11d91, 0x11d93,
0x11d98, 0x11da0, 0x11da9, 0x11ee0, 0x11ef6, 0x11fb0, 0x11fb0, 0x11fdd,
0x11fe0, 0x12000, 0x12399, 0x12400, 0x1246e, 0x12480, 0x12543, 0x12f90,
0x12ff0, 0x13000, 0x1342e, 0x13430, 0x13438, 0x14400, 0x14646, 0x16800,
0x16a38, 0x16a40, 0x16a5e, 0x16a60, 0x16a69, 0x16a70, 0x16abe, 0x16ac0,
0x16ac9, 0x16ad0, 0x16aed, 0x16af0, 0x16af4, 0x16b00, 0x16b36, 0x16b40,
0x16b43, 0x16b50, 0x16b59, 0x16b63, 0x16b77, 0x16b7d, 0x16b8f, 0x16e40,
0x16e7f, 0x16f00, 0x16f4a, 0x16f4f, 0x16f87, 0x16f8f, 0x16f9f, 0x16fe0,
0x16fe1, 0x16fe3, 0x16fe4, 0x16ff0, 0x16ff1, 0x17000, 0x187f7, 0x18800,
0x18cd5, 0x18d00, 0x18d08, 0x1aff0, 0x1aff3, 0x1aff5, 0x1affb, 0x1affd,
0x1affe, 0x1b000, 0x1b122, 0x1b150, 0x1b152, 0x1b164, 0x1b167, 0x1b170,
0x1b2fb, 0x1bc00, 0x1bc6a, 0x1bc70, 0x1bc7c, 0x1bc80, 0x1bc88, 0x1bc90,
0x1bc99, 0x1bc9d, 0x1bc9e, 0x1bca0, 0x1bca3, 0x1cf00, 0x1cf2d, 0x1cf30,
0x1cf46, 0x1d165, 0x1d169, 0x1d16d, 0x1d182, 0x1d185, 0x1d18b, 0x1d1aa,
0x1d1ad, 0x1d242, 0x1d244, 0x1d400, 0x1d454, 0x1d456, 0x1d49c, 0x1d49e,
0x1d49f, 0x1d4a2, 0x1d4a2, 0x1d4a5, 0x1d4a6, 0x1d4a9, 0x1d4ac, 0x1d4ae,
0x1d4b9, 0x1d4bb, 0x1d4bb, 0x1d4bd, 0x1d4c3, 0x1d4c5, 0x1d505, 0x1d507,
0x1d50a, 0x1d50d, 0x1d514, 0x1d516, 0x1d51c, 0x1d51e, 0x1d539, 0x1d53b,
0x1d53e, 0x1d540, 0x1d544, 0x1d546, 0x1d546, 0x1d54a, 0x1d550, 0x1d552,
0x1d6a5, 0x1d6a8, 0x1d6c0, 0x1d6c2, 0x1d6da, 0x1d6dc, 0x1d6fa, 0x1d6fc,
0x1d714, 0x1d716, 0x1d734, 0x1d736, 0x1d74e, 0x1d750, 0x1d76e, 0x1d770,
0x1d788, 0x1d78a, 0x1d7a8, 0x1d7aa, 0x1d7c2, 0x1d7c4, 0x1d7cb, 0x1d7ce,
0x1d7ff, 0x1da00, 0x1da36, 0x1da3b, 0x1da6c, 0x1da75, 0x1da75, 0x1da84,
0x1da84, 0x1da9b, 0x1da9f, 0x1daa1, 0x1daaf, 0x1df00, 0x1df1e, 0x1e000,
0x1e006, 0x1e008, 0x1e018, 0x1e01b, 0x1e021, 0x1e023, 0x1e024, 0x1e026,
0x1e02a, 0x1e100, 0x1e12c, 0x1e130, 0x1e13d, 0x1e140, 0x1e149, 0x1e14e,
0x1e14e, 0x1e290, 0x1e2ae, 0x1e2c0, 0x1e2f9, 0x1e2ff, 0x1e2ff, 0x1e7e0,
0x1e7e6, 0x1e7e8, 0x1e7eb, 0x1e7ed, 0x1e7ee, 0x1e7f0, 0x1e7fe, 0x1e800,
0x1e8c4, 0x1e8d0, 0x1e8d6, 0x1e900, 0x1e94b, 0x1e950, 0x1e959, 0x1ecb0,
0x1ecb0, 0x1ee00, 0x1ee03, 0x1ee05, 0x1ee1f, 0x1ee21, 0x1ee22, 0x1ee24,
0x1ee24, 0x1ee27, 0x1ee27, 0x1ee29, 0x1ee32, 0x1ee34, 0x1ee37, 0x1ee39,
0x1ee39, 0x1ee3b, 0x1ee3b, 0x1ee42, 0x1ee42, 0x1ee47, 0x1ee47, 0x1ee49,
0x1ee49, 0x1ee4b, 0x1ee4b, 0x1ee4d, 0x1ee4f, 0x1ee51, 0x1ee52, 0x1ee54,
0x1ee54, 0x1ee57, 0x1ee57, 0x1ee59, 0x1ee59, 0x1ee5b, 0x1ee5b, 0x1ee5d,
0x1ee5d, 0x1ee5f, 0x1ee5f, 0x1ee61, 0x1ee62, 0x1ee64, 0x1ee64, 0x1ee67,
0x1ee6a, 0x1ee6c, 0x1ee72, 0x1ee74, 0x1ee77, 0x1ee79, 0x1ee7c, 0x1ee7e,
0x1ee7e, 0x1ee80, 0x1ee89, 0x1ee8b, 0x1ee9b, 0x1eea1, 0x1eea3, 0x1eea5,
0x1eea9, 0x1eeab, 0x1eebb, 0x1fbf0, 0x1fbf9, 0x20000, 0x2a6df, 0x2a700,
0x2b738, 0x2b740, 0x2b81d, 0x2b820, 0x2cea1, 0x2ceb0, 0x2ebe0, 0x2f800,
0x2fa1d, 0x30000, 0x3134a, 0xe0001, 0xe0001, 0xe0020, 0xe007f, 0xe0100,
0xe01ef};
}
private static final int[] NFA_MOVES_TEMPLATE_TEXT_48 = NFA_MOVES_TEMPLATE_TEXT_48_init();
private static int[] NFA_MOVES_TEMPLATE_TEXT_48_init() {
return new int[] {'$', '$', 'A', 'Z', '_', '_', 'a', 'z', 0xa2, 0xa5,
0xaa, 0xaa, 0xb5, 0xb5, 0xba, 0xba, 0xc0, 0xd6, 0xd8, 0xf6, 0xf8, 0x2c1,
0x2c6, 0x2d1, 0x2e0, 0x2e4, 0x2ec, 0x2ec, 0x2ee, 0x2ee, 0x370, 0x374,
0x376, 0x377, 0x37a, 0x37d, 0x37f, 0x37f, 0x386, 0x386, 0x388, 0x38a,
0x38c, 0x38c, 0x38e, 0x3a1, 0x3a3, 0x3f5, 0x3f7, 0x481, 0x48a, 0x52f,
0x531, 0x556, 0x559, 0x559, 0x560, 0x588, 0x58f, 0x58f, 0x5d0, 0x5ea,
0x5ef, 0x5f2, 0x60b, 0x60b, 0x620, 0x64a, 0x66e, 0x66f, 0x671, 0x6d3,
0x6d5, 0x6d5, 0x6e5, 0x6e6, 0x6ee, 0x6ef, 0x6fa, 0x6fc, 0x6ff, 0x6ff,
0x710, 0x710, 0x712, 0x72f, 0x74d, 0x7a5, 0x7b1, 0x7b1, 0x7ca, 0x7ea,
0x7f4, 0x7f5, 0x7fa, 0x7fa, 0x7fe, 0x815, 0x81a, 0x81a, 0x824, 0x824,
0x828, 0x828, 0x840, 0x858, 0x860, 0x86a, 0x870, 0x887, 0x889, 0x88e,
0x8a0, 0x8c9, 0x904, 0x939, 0x93d, 0x93d, 0x950, 0x950, 0x958, 0x961,
0x971, 0x980, 0x985, 0x98c, 0x98f, 0x990, 0x993, 0x9a8, 0x9aa, 0x9b0,
0x9b2, 0x9b2, 0x9b6, 0x9b9, 0x9bd, 0x9bd, 0x9ce, 0x9ce, 0x9dc, 0x9dd,
0x9df, 0x9e1, 0x9f0, 0x9f3, 0x9fb, 0x9fc, 0xa05, 0xa0a, 0xa0f, 0xa10,
0xa13, 0xa28, 0xa2a, 0xa30, 0xa32, 0xa33, 0xa35, 0xa36, 0xa38, 0xa39,
0xa59, 0xa5c, 0xa5e, 0xa5e, 0xa72, 0xa74, 0xa85, 0xa8d, 0xa8f, 0xa91,
0xa93, 0xaa8, 0xaaa, 0xab0, 0xab2, 0xab3, 0xab5, 0xab9, 0xabd, 0xabd,
0xad0, 0xad0, 0xae0, 0xae1, 0xaf1, 0xaf1, 0xaf9, 0xaf9, 0xb05, 0xb0c,
0xb0f, 0xb10, 0xb13, 0xb28, 0xb2a, 0xb30, 0xb32, 0xb33, 0xb35, 0xb39,
0xb3d, 0xb3d, 0xb5c, 0xb5d, 0xb5f, 0xb61, 0xb71, 0xb71, 0xb83, 0xb83,
0xb85, 0xb8a, 0xb8e, 0xb90, 0xb92, 0xb95, 0xb99, 0xb9a, 0xb9c, 0xb9c,
0xb9e, 0xb9f, 0xba3, 0xba4, 0xba8, 0xbaa, 0xbae, 0xbb9, 0xbd0, 0xbd0,
0xbf9, 0xbf9, 0xc05, 0xc0c, 0xc0e, 0xc10, 0xc12, 0xc28, 0xc2a, 0xc39,
0xc3d, 0xc3d, 0xc58, 0xc5a, 0xc5d, 0xc5d, 0xc60, 0xc61, 0xc80, 0xc80,
0xc85, 0xc8c, 0xc8e, 0xc90, 0xc92, 0xca8, 0xcaa, 0xcb3, 0xcb5, 0xcb9,
0xcbd, 0xcbd, 0xcdd, 0xcde, 0xce0, 0xce1, 0xcf1, 0xcf2, 0xd04, 0xd0c,
0xd0e, 0xd10, 0xd12, 0xd3a, 0xd3d, 0xd3d, 0xd4e, 0xd4e, 0xd54, 0xd56,
0xd5f, 0xd61, 0xd7a, 0xd7f, 0xd85, 0xd96, 0xd9a, 0xdb1, 0xdb3, 0xdbb,
0xdbd, 0xdbd, 0xdc0, 0xdc6, 0xe01, 0xe30, 0xe32, 0xe33, 0xe3f, 0xe46,
0xe81, 0xe82, 0xe84, 0xe84, 0xe86, 0xe8a, 0xe8c, 0xea3, 0xea5, 0xea5,
0xea7, 0xeb0, 0xeb2, 0xeb3, 0xebd, 0xebd, 0xec0, 0xec4, 0xec6, 0xec6,
0xedc, 0xedf, 0xf00, 0xf00, 0xf40, 0xf47, 0xf49, 0xf6c, 0xf88, 0xf8c,
0x1000, 0x102a, 0x103f, 0x103f, 0x1050, 0x1055, 0x105a, 0x105d, 0x1061,
0x1061, 0x1065, 0x1066, 0x106e, 0x1070, 0x1075, 0x1081, 0x108e, 0x108e,
0x10a0, 0x10c5, 0x10c7, 0x10c7, 0x10cd, 0x10cd, 0x10d0, 0x10fa, 0x10fc,
0x1248, 0x124a, 0x124d, 0x1250, 0x1256, 0x1258, 0x1258, 0x125a, 0x125d,
0x1260, 0x1288, 0x128a, 0x128d, 0x1290, 0x12b0, 0x12b2, 0x12b5, 0x12b8,
0x12be, 0x12c0, 0x12c0, 0x12c2, 0x12c5, 0x12c8, 0x12d6, 0x12d8, 0x1310,
0x1312, 0x1315, 0x1318, 0x135a, 0x1380, 0x138f, 0x13a0, 0x13f5, 0x13f8,
0x13fd, 0x1401, 0x166c, 0x166f, 0x167f, 0x1681, 0x169a, 0x16a0, 0x16ea,
0x16ee, 0x16f8, 0x1700, 0x1711, 0x171f, 0x1731, 0x1740, 0x1751, 0x1760,
0x176c, 0x176e, 0x1770, 0x1780, 0x17b3, 0x17d7, 0x17d7, 0x17db, 0x17dc,
0x1820, 0x1878, 0x1880, 0x1884, 0x1887, 0x18a8, 0x18aa, 0x18aa, 0x18b0,
0x18f5, 0x1900, 0x191e, 0x1950, 0x196d, 0x1970, 0x1974, 0x1980, 0x19ab,
0x19b0, 0x19c9, 0x1a00, 0x1a16, 0x1a20, 0x1a54, 0x1aa7, 0x1aa7, 0x1b05,
0x1b33, 0x1b45, 0x1b4c, 0x1b83, 0x1ba0, 0x1bae, 0x1baf, 0x1bba, 0x1be5,
0x1c00, 0x1c23, 0x1c4d, 0x1c4f, 0x1c5a, 0x1c7d, 0x1c80, 0x1c88, 0x1c90,
0x1cba, 0x1cbd, 0x1cbf, 0x1ce9, 0x1cec, 0x1cee, 0x1cf3, 0x1cf5, 0x1cf6,
0x1cfa, 0x1cfa, 0x1d00, 0x1dbf, 0x1e00, 0x1f15, 0x1f18, 0x1f1d, 0x1f20,
0x1f45, 0x1f48, 0x1f4d, 0x1f50, 0x1f57, 0x1f59, 0x1f59, 0x1f5b, 0x1f5b,
0x1f5d, 0x1f5d, 0x1f5f, 0x1f7d, 0x1f80, 0x1fb4, 0x1fb6, 0x1fbc, 0x1fbe,
0x1fbe, 0x1fc2, 0x1fc4, 0x1fc6, 0x1fcc, 0x1fd0, 0x1fd3, 0x1fd6, 0x1fdb,
0x1fe0, 0x1fec, 0x1ff2, 0x1ff4, 0x1ff6, 0x1ffc, 0x203f, 0x2040, 0x2054,
0x2054, 0x2071, 0x2071, 0x207f, 0x207f, 0x2090, 0x209c, 0x20a0, 0x20c0,
0x2102, 0x2102, 0x2107, 0x2107, 0x210a, 0x2113, 0x2115, 0x2115, 0x2119,
0x211d, 0x2124, 0x2124, 0x2126, 0x2126, 0x2128, 0x2128, 0x212a, 0x212d,
0x212f, 0x2139, 0x213c, 0x213f, 0x2145, 0x2149, 0x214e, 0x214e, 0x2160,
0x2188, 0x2c00, 0x2ce4, 0x2ceb, 0x2cee, 0x2cf2, 0x2cf3, 0x2d00, 0x2d25,
0x2d27, 0x2d27, 0x2d2d, 0x2d2d, 0x2d30, 0x2d67, 0x2d6f, 0x2d6f, 0x2d80,
0x2d96, 0x2da0, 0x2da6, 0x2da8, 0x2dae, 0x2db0, 0x2db6, 0x2db8, 0x2dbe,
0x2dc0, 0x2dc6, 0x2dc8, 0x2dce, 0x2dd0, 0x2dd6, 0x2dd8, 0x2dde, 0x2e2f,
0x2e2f, 0x3005, 0x3007, 0x3021, 0x3029, 0x3031, 0x3035, 0x3038, 0x303c,
0x3041, 0x3096, 0x309d, 0x309f, 0x30a1, 0x30fa, 0x30fc, 0x30ff, 0x3105,
0x312f, 0x3131, 0x318e, 0x31a0, 0x31bf, 0x31f0, 0x31ff, 0x3400, 0x4dbf,
0x4e00, 0xa48c, 0xa4d0, 0xa4fd, 0xa500, 0xa60c, 0xa610, 0xa61f, 0xa62a,
0xa62b, 0xa640, 0xa66e, 0xa67f, 0xa69d, 0xa6a0, 0xa6ef, 0xa717, 0xa71f,
0xa722, 0xa788, 0xa78b, 0xa7ca, 0xa7d0, 0xa7d1, 0xa7d3, 0xa7d3, 0xa7d5,
0xa7d9, 0xa7f2, 0xa801, 0xa803, 0xa805, 0xa807, 0xa80a, 0xa80c, 0xa822,
0xa838, 0xa838, 0xa840, 0xa873, 0xa882, 0xa8b3, 0xa8f2, 0xa8f7, 0xa8fb,
0xa8fb, 0xa8fd, 0xa8fe, 0xa90a, 0xa925, 0xa930, 0xa946, 0xa960, 0xa97c,
0xa984, 0xa9b2, 0xa9cf, 0xa9cf, 0xa9e0, 0xa9e4, 0xa9e6, 0xa9ef, 0xa9fa,
0xa9fe, 0xaa00, 0xaa28, 0xaa40, 0xaa42, 0xaa44, 0xaa4b, 0xaa60, 0xaa76,
0xaa7a, 0xaa7a, 0xaa7e, 0xaaaf, 0xaab1, 0xaab1, 0xaab5, 0xaab6, 0xaab9,
0xaabd, 0xaac0, 0xaac0, 0xaac2, 0xaac2, 0xaadb, 0xaadd, 0xaae0, 0xaaea,
0xaaf2, 0xaaf4, 0xab01, 0xab06, 0xab09, 0xab0e, 0xab11, 0xab16, 0xab20,
0xab26, 0xab28, 0xab2e, 0xab30, 0xab5a, 0xab5c, 0xab69, 0xab70, 0xabe2,
0xac00, 0xd7a3, 0xd7b0, 0xd7c6, 0xd7cb, 0xd7fb, 0xf900, 0xfa6d, 0xfa70,
0xfad9, 0xfb00, 0xfb06, 0xfb13, 0xfb17, 0xfb1d, 0xfb1d, 0xfb1f, 0xfb28,
0xfb2a, 0xfb36, 0xfb38, 0xfb3c, 0xfb3e, 0xfb3e, 0xfb40, 0xfb41, 0xfb43,
0xfb44, 0xfb46, 0xfbb1, 0xfbd3, 0xfd3d, 0xfd50, 0xfd8f, 0xfd92, 0xfdc7,
0xfdf0, 0xfdfc, 0xfe33, 0xfe34, 0xfe4d, 0xfe4f, 0xfe69, 0xfe69, 0xfe70,
0xfe74, 0xfe76, 0xfefc, 0xff04, 0xff04, 0xff21, 0xff3a, 0xff3f, 0xff3f,
0xff41, 0xff5a, 0xff66, 0xffbe, 0xffc2, 0xffc7, 0xffca, 0xffcf, 0xffd2,
0xffd7, 0xffda, 0xffdc, 0xffe0, 0xffe1, 0xffe5, 0xffe6, 0x10000, 0x1000b,
0x1000d, 0x10026, 0x10028, 0x1003a, 0x1003c, 0x1003d, 0x1003f, 0x1004d,
0x10050, 0x1005d, 0x10080, 0x100fa, 0x10140, 0x10174, 0x10280, 0x1029c,
0x102a0, 0x102d0, 0x10300, 0x1031f, 0x1032d, 0x1034a, 0x10350, 0x10375,
0x10380, 0x1039d, 0x103a0, 0x103c3, 0x103c8, 0x103cf, 0x103d1, 0x103d5,
0x10400, 0x1049d, 0x104b0, 0x104d3, 0x104d8, 0x104fb, 0x10500, 0x10527,
0x10530, 0x10563, 0x10570, 0x1057a, 0x1057c, 0x1058a, 0x1058c, 0x10592,
0x10594, 0x10595, 0x10597, 0x105a1, 0x105a3, 0x105b1, 0x105b3, 0x105b9,
0x105bb, 0x105bc, 0x10600, 0x10736, 0x10740, 0x10755, 0x10760, 0x10767,
0x10780, 0x10785, 0x10787, 0x107b0, 0x107b2, 0x107ba, 0x10800, 0x10805,
0x10808, 0x10808, 0x1080a, 0x10835, 0x10837, 0x10838, 0x1083c, 0x1083c,
0x1083f, 0x10855, 0x10860, 0x10876, 0x10880, 0x1089e, 0x108e0, 0x108f2,
0x108f4, 0x108f5, 0x10900, 0x10915, 0x10920, 0x10939, 0x10980, 0x109b7,
0x109be, 0x109bf, 0x10a00, 0x10a00, 0x10a10, 0x10a13, 0x10a15, 0x10a17,
0x10a19, 0x10a35, 0x10a60, 0x10a7c, 0x10a80, 0x10a9c, 0x10ac0, 0x10ac7,
0x10ac9, 0x10ae4, 0x10b00, 0x10b35, 0x10b40, 0x10b55, 0x10b60, 0x10b72,
0x10b80, 0x10b91, 0x10c00, 0x10c48, 0x10c80, 0x10cb2, 0x10cc0, 0x10cf2,
0x10d00, 0x10d23, 0x10e80, 0x10ea9, 0x10eb0, 0x10eb1, 0x10f00, 0x10f1c,
0x10f27, 0x10f27, 0x10f30, 0x10f45, 0x10f70, 0x10f81, 0x10fb0, 0x10fc4,
0x10fe0, 0x10ff6, 0x11003, 0x11037, 0x11071, 0x11072, 0x11075, 0x11075,
0x11083, 0x110af, 0x110d0, 0x110e8, 0x11103, 0x11126, 0x11144, 0x11144,
0x11147, 0x11147, 0x11150, 0x11172, 0x11176, 0x11176, 0x11183, 0x111b2,
0x111c1, 0x111c4, 0x111da, 0x111da, 0x111dc, 0x111dc, 0x11200, 0x11211,
0x11213, 0x1122b, 0x11280, 0x11286, 0x11288, 0x11288, 0x1128a, 0x1128d,
0x1128f, 0x1129d, 0x1129f, 0x112a8, 0x112b0, 0x112de, 0x11305, 0x1130c,
0x1130f, 0x11310, 0x11313, 0x11328, 0x1132a, 0x11330, 0x11332, 0x11333,
0x11335, 0x11339, 0x1133d, 0x1133d, 0x11350, 0x11350, 0x1135d, 0x11361,
0x11400, 0x11434, 0x11447, 0x1144a, 0x1145f, 0x11461, 0x11480, 0x114af,
0x114c4, 0x114c5, 0x114c7, 0x114c7, 0x11580, 0x115ae, 0x115d8, 0x115db,
0x11600, 0x1162f, 0x11644, 0x11644, 0x11680, 0x116aa, 0x116b8, 0x116b8,
0x11700, 0x1171a, 0x11740, 0x11746, 0x11800, 0x1182b, 0x118a0, 0x118df,
0x118ff, 0x11906, 0x11909, 0x11909, 0x1190c, 0x11913, 0x11915, 0x11916,
0x11918, 0x1192f, 0x1193f, 0x1193f, 0x11941, 0x11941, 0x119a0, 0x119a7,
0x119aa, 0x119d0, 0x119e1, 0x119e1, 0x119e3, 0x119e3, 0x11a00, 0x11a00,
0x11a0b, 0x11a32, 0x11a3a, 0x11a3a, 0x11a50, 0x11a50, 0x11a5c, 0x11a89,
0x11a9d, 0x11a9d, 0x11ab0, 0x11af8, 0x11c00, 0x11c08, 0x11c0a, 0x11c2e,
0x11c40, 0x11c40, 0x11c72, 0x11c8f, 0x11d00, 0x11d06, 0x11d08, 0x11d09,
0x11d0b, 0x11d30, 0x11d46, 0x11d46, 0x11d60, 0x11d65, 0x11d67, 0x11d68,
0x11d6a, 0x11d89, 0x11d98, 0x11d98, 0x11ee0, 0x11ef2, 0x11fb0, 0x11fb0,
0x11fdd, 0x11fe0, 0x12000, 0x12399, 0x12400, 0x1246e, 0x12480, 0x12543,
0x12f90, 0x12ff0, 0x13000, 0x1342e, 0x14400, 0x14646, 0x16800, 0x16a38,
0x16a40, 0x16a5e, 0x16a70, 0x16abe, 0x16ad0, 0x16aed, 0x16b00, 0x16b2f,
0x16b40, 0x16b43, 0x16b63, 0x16b77, 0x16b7d, 0x16b8f, 0x16e40, 0x16e7f,
0x16f00, 0x16f4a, 0x16f50, 0x16f50, 0x16f93, 0x16f9f, 0x16fe0, 0x16fe1,
0x16fe3, 0x16fe3, 0x17000, 0x187f7, 0x18800, 0x18cd5, 0x18d00, 0x18d08,
0x1aff0, 0x1aff3, 0x1aff5, 0x1affb, 0x1affd, 0x1affe, 0x1b000, 0x1b122,
0x1b150, 0x1b152, 0x1b164, 0x1b167, 0x1b170, 0x1b2fb, 0x1bc00, 0x1bc6a,
0x1bc70, 0x1bc7c, 0x1bc80, 0x1bc88, 0x1bc90, 0x1bc99, 0x1d400, 0x1d454,
0x1d456, 0x1d49c, 0x1d49e, 0x1d49f, 0x1d4a2, 0x1d4a2, 0x1d4a5, 0x1d4a6,
0x1d4a9, 0x1d4ac, 0x1d4ae, 0x1d4b9, 0x1d4bb, 0x1d4bb, 0x1d4bd, 0x1d4c3,
0x1d4c5, 0x1d505, 0x1d507, 0x1d50a, 0x1d50d, 0x1d514, 0x1d516, 0x1d51c,
0x1d51e, 0x1d539, 0x1d53b, 0x1d53e, 0x1d540, 0x1d544, 0x1d546, 0x1d546,
0x1d54a, 0x1d550, 0x1d552, 0x1d6a5, 0x1d6a8, 0x1d6c0, 0x1d6c2, 0x1d6da,
0x1d6dc, 0x1d6fa, 0x1d6fc, 0x1d714, 0x1d716, 0x1d734, 0x1d736, 0x1d74e,
0x1d750, 0x1d76e, 0x1d770, 0x1d788, 0x1d78a, 0x1d7a8, 0x1d7aa, 0x1d7c2,
0x1d7c4, 0x1d7cb, 0x1df00, 0x1df1e, 0x1e100, 0x1e12c, 0x1e137, 0x1e13d,
0x1e14e, 0x1e14e, 0x1e290, 0x1e2ad, 0x1e2c0, 0x1e2eb, 0x1e2ff, 0x1e2ff,
0x1e7e0, 0x1e7e6, 0x1e7e8, 0x1e7eb, 0x1e7ed, 0x1e7ee, 0x1e7f0, 0x1e7fe,
0x1e800, 0x1e8c4, 0x1e900, 0x1e943, 0x1e94b, 0x1e94b, 0x1ecb0, 0x1ecb0,
0x1ee00, 0x1ee03, 0x1ee05, 0x1ee1f, 0x1ee21, 0x1ee22, 0x1ee24, 0x1ee24,
0x1ee27, 0x1ee27, 0x1ee29, 0x1ee32, 0x1ee34, 0x1ee37, 0x1ee39, 0x1ee39,
0x1ee3b, 0x1ee3b, 0x1ee42, 0x1ee42, 0x1ee47, 0x1ee47, 0x1ee49, 0x1ee49,
0x1ee4b, 0x1ee4b, 0x1ee4d, 0x1ee4f, 0x1ee51, 0x1ee52, 0x1ee54, 0x1ee54,
0x1ee57, 0x1ee57, 0x1ee59, 0x1ee59, 0x1ee5b, 0x1ee5b, 0x1ee5d, 0x1ee5d,
0x1ee5f, 0x1ee5f, 0x1ee61, 0x1ee62, 0x1ee64, 0x1ee64, 0x1ee67, 0x1ee6a,
0x1ee6c, 0x1ee72, 0x1ee74, 0x1ee77, 0x1ee79, 0x1ee7c, 0x1ee7e, 0x1ee7e,
0x1ee80, 0x1ee89, 0x1ee8b, 0x1ee9b, 0x1eea1, 0x1eea3, 0x1eea5, 0x1eea9,
0x1eeab, 0x1eebb, 0x20000, 0x2a6df, 0x2a700, 0x2b738, 0x2b740, 0x2b81d,
0x2b820, 0x2cea1, 0x2ceb0, 0x2ebe0, 0x2f800, 0x2fa1d, 0x30000, 0x3134a};
}
private static final int[] NFA_MOVES_TEMPLATE_TEXT_50 = NFA_MOVES_TEMPLATE_TEXT_50_init();
private static int[] NFA_MOVES_TEMPLATE_TEXT_50_init() {
return new int[] {0x0, 0x8, 0xe, 0x1b, '$', '$', '0', '9', 'A', 'Z', '_',
'_', 'a', 'z', 0x7f, 0x9f, 0xa2, 0xa5, 0xaa, 0xaa, 0xad, 0xad, 0xb5, 0xb5,
0xba, 0xba, 0xc0, 0xd6, 0xd8, 0xf6, 0xf8, 0x2c1, 0x2c6, 0x2d1, 0x2e0,
0x2e4, 0x2ec, 0x2ec, 0x2ee, 0x2ee, 0x300, 0x374, 0x376, 0x377, 0x37a,
0x37d, 0x37f, 0x37f, 0x386, 0x386, 0x388, 0x38a, 0x38c, 0x38c, 0x38e,
0x3a1, 0x3a3, 0x3f5, 0x3f7, 0x481, 0x483, 0x487, 0x48a, 0x52f, 0x531,
0x556, 0x559, 0x559, 0x560, 0x588, 0x58f, 0x58f, 0x591, 0x5bd, 0x5bf,
0x5bf, 0x5c1, 0x5c2, 0x5c4, 0x5c5, 0x5c7, 0x5c7, 0x5d0, 0x5ea, 0x5ef,
0x5f2, 0x600, 0x605, 0x60b, 0x60b, 0x610, 0x61a, 0x61c, 0x61c, 0x620,
0x669, 0x66e, 0x6d3, 0x6d5, 0x6dd, 0x6df, 0x6e8, 0x6ea, 0x6fc, 0x6ff,
0x6ff, 0x70f, 0x74a, 0x74d, 0x7b1, 0x7c0, 0x7f5, 0x7fa, 0x7fa, 0x7fd,
0x82d, 0x840, 0x85b, 0x860, 0x86a, 0x870, 0x887, 0x889, 0x88e, 0x890,
0x891, 0x898, 0x963, 0x966, 0x96f, 0x971, 0x983, 0x985, 0x98c, 0x98f,
0x990, 0x993, 0x9a8, 0x9aa, 0x9b0, 0x9b2, 0x9b2, 0x9b6, 0x9b9, 0x9bc,
0x9c4, 0x9c7, 0x9c8, 0x9cb, 0x9ce, 0x9d7, 0x9d7, 0x9dc, 0x9dd, 0x9df,
0x9e3, 0x9e6, 0x9f3, 0x9fb, 0x9fc, 0x9fe, 0x9fe, 0xa01, 0xa03, 0xa05,
0xa0a, 0xa0f, 0xa10, 0xa13, 0xa28, 0xa2a, 0xa30, 0xa32, 0xa33, 0xa35,
0xa36, 0xa38, 0xa39, 0xa3c, 0xa3c, 0xa3e, 0xa42, 0xa47, 0xa48, 0xa4b,
0xa4d, 0xa51, 0xa51, 0xa59, 0xa5c, 0xa5e, 0xa5e, 0xa66, 0xa75, 0xa81,
0xa83, 0xa85, 0xa8d, 0xa8f, 0xa91, 0xa93, 0xaa8, 0xaaa, 0xab0, 0xab2,
0xab3, 0xab5, 0xab9, 0xabc, 0xac5, 0xac7, 0xac9, 0xacb, 0xacd, 0xad0,
0xad0, 0xae0, 0xae3, 0xae6, 0xaef, 0xaf1, 0xaf1, 0xaf9, 0xaff, 0xb01,
0xb03, 0xb05, 0xb0c, 0xb0f, 0xb10, 0xb13, 0xb28, 0xb2a, 0xb30, 0xb32,
0xb33, 0xb35, 0xb39, 0xb3c, 0xb44, 0xb47, 0xb48, 0xb4b, 0xb4d, 0xb55,
0xb57, 0xb5c, 0xb5d, 0xb5f, 0xb63, 0xb66, 0xb6f, 0xb71, 0xb71, 0xb82,
0xb83, 0xb85, 0xb8a, 0xb8e, 0xb90, 0xb92, 0xb95, 0xb99, 0xb9a, 0xb9c,
0xb9c, 0xb9e, 0xb9f, 0xba3, 0xba4, 0xba8, 0xbaa, 0xbae, 0xbb9, 0xbbe,
0xbc2, 0xbc6, 0xbc8, 0xbca, 0xbcd, 0xbd0, 0xbd0, 0xbd7, 0xbd7, 0xbe6,
0xbef, 0xbf9, 0xbf9, 0xc00, 0xc0c, 0xc0e, 0xc10, 0xc12, 0xc28, 0xc2a,
0xc39, 0xc3c, 0xc44, 0xc46, 0xc48, 0xc4a, 0xc4d, 0xc55, 0xc56, 0xc58,
0xc5a, 0xc5d, 0xc5d, 0xc60, 0xc63, 0xc66, 0xc6f, 0xc80, 0xc83, 0xc85,
0xc8c, 0xc8e, 0xc90, 0xc92, 0xca8, 0xcaa, 0xcb3, 0xcb5, 0xcb9, 0xcbc,
0xcc4, 0xcc6, 0xcc8, 0xcca, 0xccd, 0xcd5, 0xcd6, 0xcdd, 0xcde, 0xce0,
0xce3, 0xce6, 0xcef, 0xcf1, 0xcf2, 0xd00, 0xd0c, 0xd0e, 0xd10, 0xd12,
0xd44, 0xd46, 0xd48, 0xd4a, 0xd4e, 0xd54, 0xd57, 0xd5f, 0xd63, 0xd66,
0xd6f, 0xd7a, 0xd7f, 0xd81, 0xd83, 0xd85, 0xd96, 0xd9a, 0xdb1, 0xdb3,
0xdbb, 0xdbd, 0xdbd, 0xdc0, 0xdc6, 0xdca, 0xdca, 0xdcf, 0xdd4, 0xdd6,
0xdd6, 0xdd8, 0xddf, 0xde6, 0xdef, 0xdf2, 0xdf3, 0xe01, 0xe3a, 0xe3f,
0xe4e, 0xe50, 0xe59, 0xe81, 0xe82, 0xe84, 0xe84, 0xe86, 0xe8a, 0xe8c,
0xea3, 0xea5, 0xea5, 0xea7, 0xebd, 0xec0, 0xec4, 0xec6, 0xec6, 0xec8,
0xecd, 0xed0, 0xed9, 0xedc, 0xedf, 0xf00, 0xf00, 0xf18, 0xf19, 0xf20,
0xf29, 0xf35, 0xf35, 0xf37, 0xf37, 0xf39, 0xf39, 0xf3e, 0xf47, 0xf49,
0xf6c, 0xf71, 0xf84, 0xf86, 0xf97, 0xf99, 0xfbc, 0xfc6, 0xfc6, 0x1000,
0x1049, 0x1050, 0x109d, 0x10a0, 0x10c5, 0x10c7, 0x10c7, 0x10cd, 0x10cd,
0x10d0, 0x10fa, 0x10fc, 0x1248, 0x124a, 0x124d, 0x1250, 0x1256, 0x1258,
0x1258, 0x125a, 0x125d, 0x1260, 0x1288, 0x128a, 0x128d, 0x1290, 0x12b0,
0x12b2, 0x12b5, 0x12b8, 0x12be, 0x12c0, 0x12c0, 0x12c2, 0x12c5, 0x12c8,
0x12d6, 0x12d8, 0x1310, 0x1312, 0x1315, 0x1318, 0x135a, 0x135d, 0x135f,
0x1380, 0x138f, 0x13a0, 0x13f5, 0x13f8, 0x13fd, 0x1401, 0x166c, 0x166f,
0x167f, 0x1681, 0x169a, 0x16a0, 0x16ea, 0x16ee, 0x16f8, 0x1700, 0x1715,
0x171f, 0x1734, 0x1740, 0x1753, 0x1760, 0x176c, 0x176e, 0x1770, 0x1772,
0x1773, 0x1780, 0x17d3, 0x17d7, 0x17d7, 0x17db, 0x17dd, 0x17e0, 0x17e9,
0x180b, 0x1819, 0x1820, 0x1878, 0x1880, 0x18aa, 0x18b0, 0x18f5, 0x1900,
0x191e, 0x1920, 0x192b, 0x1930, 0x193b, 0x1946, 0x196d, 0x1970, 0x1974,
0x1980, 0x19ab, 0x19b0, 0x19c9, 0x19d0, 0x19d9, 0x1a00, 0x1a1b, 0x1a20,
0x1a5e, 0x1a60, 0x1a7c, 0x1a7f, 0x1a89, 0x1a90, 0x1a99, 0x1aa7, 0x1aa7,
0x1ab0, 0x1abd, 0x1abf, 0x1ace, 0x1b00, 0x1b4c, 0x1b50, 0x1b59, 0x1b6b,
0x1b73, 0x1b80, 0x1bf3, 0x1c00, 0x1c37, 0x1c40, 0x1c49, 0x1c4d, 0x1c7d,
0x1c80, 0x1c88, 0x1c90, 0x1cba, 0x1cbd, 0x1cbf, 0x1cd0, 0x1cd2, 0x1cd4,
0x1cfa, 0x1d00, 0x1f15, 0x1f18, 0x1f1d, 0x1f20, 0x1f45, 0x1f48, 0x1f4d,
0x1f50, 0x1f57, 0x1f59, 0x1f59, 0x1f5b, 0x1f5b, 0x1f5d, 0x1f5d, 0x1f5f,
0x1f7d, 0x1f80, 0x1fb4, 0x1fb6, 0x1fbc, 0x1fbe, 0x1fbe, 0x1fc2, 0x1fc4,
0x1fc6, 0x1fcc, 0x1fd0, 0x1fd3, 0x1fd6, 0x1fdb, 0x1fe0, 0x1fec, 0x1ff2,
0x1ff4, 0x1ff6, 0x1ffc, 0x200b, 0x200f, 0x202a, 0x202e, 0x203f, 0x2040,
0x2054, 0x2054, 0x2060, 0x2064, 0x2066, 0x206f, 0x2071, 0x2071, 0x207f,
0x207f, 0x2090, 0x209c, 0x20a0, 0x20c0, 0x20d0, 0x20dc, 0x20e1, 0x20e1,
0x20e5, 0x20f0, 0x2102, 0x2102, 0x2107, 0x2107, 0x210a, 0x2113, 0x2115,
0x2115, 0x2119, 0x211d, 0x2124, 0x2124, 0x2126, 0x2126, 0x2128, 0x2128,
0x212a, 0x212d, 0x212f, 0x2139, 0x213c, 0x213f, 0x2145, 0x2149, 0x214e,
0x214e, 0x2160, 0x2188, 0x2c00, 0x2ce4, 0x2ceb, 0x2cf3, 0x2d00, 0x2d25,
0x2d27, 0x2d27, 0x2d2d, 0x2d2d, 0x2d30, 0x2d67, 0x2d6f, 0x2d6f, 0x2d7f,
0x2d96, 0x2da0, 0x2da6, 0x2da8, 0x2dae, 0x2db0, 0x2db6, 0x2db8, 0x2dbe,
0x2dc0, 0x2dc6, 0x2dc8, 0x2dce, 0x2dd0, 0x2dd6, 0x2dd8, 0x2dde, 0x2de0,
0x2dff, 0x2e2f, 0x2e2f, 0x3005, 0x3007, 0x3021, 0x302f, 0x3031, 0x3035,
0x3038, 0x303c, 0x3041, 0x3096, 0x3099, 0x309a, 0x309d, 0x309f, 0x30a1,
0x30fa, 0x30fc, 0x30ff, 0x3105, 0x312f, 0x3131, 0x318e, 0x31a0, 0x31bf,
0x31f0, 0x31ff, 0x3400, 0x4dbf, 0x4e00, 0xa48c, 0xa4d0, 0xa4fd, 0xa500,
0xa60c, 0xa610, 0xa62b, 0xa640, 0xa66f, 0xa674, 0xa67d, 0xa67f, 0xa6f1,
0xa717, 0xa71f, 0xa722, 0xa788, 0xa78b, 0xa7ca, 0xa7d0, 0xa7d1, 0xa7d3,
0xa7d3, 0xa7d5, 0xa7d9, 0xa7f2, 0xa827, 0xa82c, 0xa82c, 0xa838, 0xa838,
0xa840, 0xa873, 0xa880, 0xa8c5, 0xa8d0, 0xa8d9, 0xa8e0, 0xa8f7, 0xa8fb,
0xa8fb, 0xa8fd, 0xa92d, 0xa930, 0xa953, 0xa960, 0xa97c, 0xa980, 0xa9c0,
0xa9cf, 0xa9d9, 0xa9e0, 0xa9fe, 0xaa00, 0xaa36, 0xaa40, 0xaa4d, 0xaa50,
0xaa59, 0xaa60, 0xaa76, 0xaa7a, 0xaac2, 0xaadb, 0xaadd, 0xaae0, 0xaaef,
0xaaf2, 0xaaf6, 0xab01, 0xab06, 0xab09, 0xab0e, 0xab11, 0xab16, 0xab20,
0xab26, 0xab28, 0xab2e, 0xab30, 0xab5a, 0xab5c, 0xab69, 0xab70, 0xabea,
0xabec, 0xabed, 0xabf0, 0xabf9, 0xac00, 0xd7a3, 0xd7b0, 0xd7c6, 0xd7cb,
0xd7fb, 0xf900, 0xfa6d, 0xfa70, 0xfad9, 0xfb00, 0xfb06, 0xfb13, 0xfb17,
0xfb1d, 0xfb28, 0xfb2a, 0xfb36, 0xfb38, 0xfb3c, 0xfb3e, 0xfb3e, 0xfb40,
0xfb41, 0xfb43, 0xfb44, 0xfb46, 0xfbb1, 0xfbd3, 0xfd3d, 0xfd50, 0xfd8f,
0xfd92, 0xfdc7, 0xfdf0, 0xfdfc, 0xfe00, 0xfe0f, 0xfe20, 0xfe2f, 0xfe33,
0xfe34, 0xfe4d, 0xfe4f, 0xfe69, 0xfe69, 0xfe70, 0xfe74, 0xfe76, 0xfefc,
0xfeff, 0xfeff, 0xff04, 0xff04, 0xff10, 0xff19, 0xff21, 0xff3a, 0xff3f,
0xff3f, 0xff41, 0xff5a, 0xff66, 0xffbe, 0xffc2, 0xffc7, 0xffca, 0xffcf,
0xffd2, 0xffd7, 0xffda, 0xffdc, 0xffe0, 0xffe1, 0xffe5, 0xffe6, 0xfff9,
0xfffb, 0x10000, 0x1000b, 0x1000d, 0x10026, 0x10028, 0x1003a, 0x1003c,
0x1003d, 0x1003f, 0x1004d, 0x10050, 0x1005d, 0x10080, 0x100fa, 0x10140,
0x10174, 0x101fd, 0x101fd, 0x10280, 0x1029c, 0x102a0, 0x102d0, 0x102e0,
0x102e0, 0x10300, 0x1031f, 0x1032d, 0x1034a, 0x10350, 0x1037a, 0x10380,
0x1039d, 0x103a0, 0x103c3, 0x103c8, 0x103cf, 0x103d1, 0x103d5, 0x10400,
0x1049d, 0x104a0, 0x104a9, 0x104b0, 0x104d3, 0x104d8, 0x104fb, 0x10500,
0x10527, 0x10530, 0x10563, 0x10570, 0x1057a, 0x1057c, 0x1058a, 0x1058c,
0x10592, 0x10594, 0x10595, 0x10597, 0x105a1, 0x105a3, 0x105b1, 0x105b3,
0x105b9, 0x105bb, 0x105bc, 0x10600, 0x10736, 0x10740, 0x10755, 0x10760,
0x10767, 0x10780, 0x10785, 0x10787, 0x107b0, 0x107b2, 0x107ba, 0x10800,
0x10805, 0x10808, 0x10808, 0x1080a, 0x10835, 0x10837, 0x10838, 0x1083c,
0x1083c, 0x1083f, 0x10855, 0x10860, 0x10876, 0x10880, 0x1089e, 0x108e0,
0x108f2, 0x108f4, 0x108f5, 0x10900, 0x10915, 0x10920, 0x10939, 0x10980,
0x109b7, 0x109be, 0x109bf, 0x10a00, 0x10a03, 0x10a05, 0x10a06, 0x10a0c,
0x10a13, 0x10a15, 0x10a17, 0x10a19, 0x10a35, 0x10a38, 0x10a3a, 0x10a3f,
0x10a3f, 0x10a60, 0x10a7c, 0x10a80, 0x10a9c, 0x10ac0, 0x10ac7, 0x10ac9,
0x10ae6, 0x10b00, 0x10b35, 0x10b40, 0x10b55, 0x10b60, 0x10b72, 0x10b80,
0x10b91, 0x10c00, 0x10c48, 0x10c80, 0x10cb2, 0x10cc0, 0x10cf2, 0x10d00,
0x10d27, 0x10d30, 0x10d39, 0x10e80, 0x10ea9, 0x10eab, 0x10eac, 0x10eb0,
0x10eb1, 0x10f00, 0x10f1c, 0x10f27, 0x10f27, 0x10f30, 0x10f50, 0x10f70,
0x10f85, 0x10fb0, 0x10fc4, 0x10fe0, 0x10ff6, 0x11000, 0x11046, 0x11066,
0x11075, 0x1107f, 0x110ba, 0x110bd, 0x110bd, 0x110c2, 0x110c2, 0x110cd,
0x110cd, 0x110d0, 0x110e8, 0x110f0, 0x110f9, 0x11100, 0x11134, 0x11136,
0x1113f, 0x11144, 0x11147, 0x11150, 0x11173, 0x11176, 0x11176, 0x11180,
0x111c4, 0x111c9, 0x111cc, 0x111ce, 0x111da, 0x111dc, 0x111dc, 0x11200,
0x11211, 0x11213, 0x11237, 0x1123e, 0x1123e, 0x11280, 0x11286, 0x11288,
0x11288, 0x1128a, 0x1128d, 0x1128f, 0x1129d, 0x1129f, 0x112a8, 0x112b0,
0x112ea, 0x112f0, 0x112f9, 0x11300, 0x11303, 0x11305, 0x1130c, 0x1130f,
0x11310, 0x11313, 0x11328, 0x1132a, 0x11330, 0x11332, 0x11333, 0x11335,
0x11339, 0x1133b, 0x11344, 0x11347, 0x11348, 0x1134b, 0x1134d, 0x11350,
0x11350, 0x11357, 0x11357, 0x1135d, 0x11363, 0x11366, 0x1136c, 0x11370,
0x11374, 0x11400, 0x1144a, 0x11450, 0x11459, 0x1145e, 0x11461, 0x11480,
0x114c5, 0x114c7, 0x114c7, 0x114d0, 0x114d9, 0x11580, 0x115b5, 0x115b8,
0x115c0, 0x115d8, 0x115dd, 0x11600, 0x11640, 0x11644, 0x11644, 0x11650,
0x11659, 0x11680, 0x116b8, 0x116c0, 0x116c9, 0x11700, 0x1171a, 0x1171d,
0x1172b, 0x11730, 0x11739, 0x11740, 0x11746, 0x11800, 0x1183a, 0x118a0,
0x118e9, 0x118ff, 0x11906, 0x11909, 0x11909, 0x1190c, 0x11913, 0x11915,
0x11916, 0x11918, 0x11935, 0x11937, 0x11938, 0x1193b, 0x11943, 0x11950,
0x11959, 0x119a0, 0x119a7, 0x119aa, 0x119d7, 0x119da, 0x119e1, 0x119e3,
0x119e4, 0x11a00, 0x11a3e, 0x11a47, 0x11a47, 0x11a50, 0x11a99, 0x11a9d,
0x11a9d, 0x11ab0, 0x11af8, 0x11c00, 0x11c08, 0x11c0a, 0x11c36, 0x11c38,
0x11c40, 0x11c50, 0x11c59, 0x11c72, 0x11c8f, 0x11c92, 0x11ca7, 0x11ca9,
0x11cb6, 0x11d00, 0x11d06, 0x11d08, 0x11d09, 0x11d0b, 0x11d36, 0x11d3a,
0x11d3a, 0x11d3c, 0x11d3d, 0x11d3f, 0x11d47, 0x11d50, 0x11d59, 0x11d60,
0x11d65, 0x11d67, 0x11d68, 0x11d6a, 0x11d8e, 0x11d90, 0x11d91, 0x11d93,
0x11d98, 0x11da0, 0x11da9, 0x11ee0, 0x11ef6, 0x11fb0, 0x11fb0, 0x11fdd,
0x11fe0, 0x12000, 0x12399, 0x12400, 0x1246e, 0x12480, 0x12543, 0x12f90,
0x12ff0, 0x13000, 0x1342e, 0x13430, 0x13438, 0x14400, 0x14646, 0x16800,
0x16a38, 0x16a40, 0x16a5e, 0x16a60, 0x16a69, 0x16a70, 0x16abe, 0x16ac0,
0x16ac9, 0x16ad0, 0x16aed, 0x16af0, 0x16af4, 0x16b00, 0x16b36, 0x16b40,
0x16b43, 0x16b50, 0x16b59, 0x16b63, 0x16b77, 0x16b7d, 0x16b8f, 0x16e40,
0x16e7f, 0x16f00, 0x16f4a, 0x16f4f, 0x16f87, 0x16f8f, 0x16f9f, 0x16fe0,
0x16fe1, 0x16fe3, 0x16fe4, 0x16ff0, 0x16ff1, 0x17000, 0x187f7, 0x18800,
0x18cd5, 0x18d00, 0x18d08, 0x1aff0, 0x1aff3, 0x1aff5, 0x1affb, 0x1affd,
0x1affe, 0x1b000, 0x1b122, 0x1b150, 0x1b152, 0x1b164, 0x1b167, 0x1b170,
0x1b2fb, 0x1bc00, 0x1bc6a, 0x1bc70, 0x1bc7c, 0x1bc80, 0x1bc88, 0x1bc90,
0x1bc99, 0x1bc9d, 0x1bc9e, 0x1bca0, 0x1bca3, 0x1cf00, 0x1cf2d, 0x1cf30,
0x1cf46, 0x1d165, 0x1d169, 0x1d16d, 0x1d182, 0x1d185, 0x1d18b, 0x1d1aa,
0x1d1ad, 0x1d242, 0x1d244, 0x1d400, 0x1d454, 0x1d456, 0x1d49c, 0x1d49e,
0x1d49f, 0x1d4a2, 0x1d4a2, 0x1d4a5, 0x1d4a6, 0x1d4a9, 0x1d4ac, 0x1d4ae,
0x1d4b9, 0x1d4bb, 0x1d4bb, 0x1d4bd, 0x1d4c3, 0x1d4c5, 0x1d505, 0x1d507,
0x1d50a, 0x1d50d, 0x1d514, 0x1d516, 0x1d51c, 0x1d51e, 0x1d539, 0x1d53b,
0x1d53e, 0x1d540, 0x1d544, 0x1d546, 0x1d546, 0x1d54a, 0x1d550, 0x1d552,
0x1d6a5, 0x1d6a8, 0x1d6c0, 0x1d6c2, 0x1d6da, 0x1d6dc, 0x1d6fa, 0x1d6fc,
0x1d714, 0x1d716, 0x1d734, 0x1d736, 0x1d74e, 0x1d750, 0x1d76e, 0x1d770,
0x1d788, 0x1d78a, 0x1d7a8, 0x1d7aa, 0x1d7c2, 0x1d7c4, 0x1d7cb, 0x1d7ce,
0x1d7ff, 0x1da00, 0x1da36, 0x1da3b, 0x1da6c, 0x1da75, 0x1da75, 0x1da84,
0x1da84, 0x1da9b, 0x1da9f, 0x1daa1, 0x1daaf, 0x1df00, 0x1df1e, 0x1e000,
0x1e006, 0x1e008, 0x1e018, 0x1e01b, 0x1e021, 0x1e023, 0x1e024, 0x1e026,
0x1e02a, 0x1e100, 0x1e12c, 0x1e130, 0x1e13d, 0x1e140, 0x1e149, 0x1e14e,
0x1e14e, 0x1e290, 0x1e2ae, 0x1e2c0, 0x1e2f9, 0x1e2ff, 0x1e2ff, 0x1e7e0,
0x1e7e6, 0x1e7e8, 0x1e7eb, 0x1e7ed, 0x1e7ee, 0x1e7f0, 0x1e7fe, 0x1e800,
0x1e8c4, 0x1e8d0, 0x1e8d6, 0x1e900, 0x1e94b, 0x1e950, 0x1e959, 0x1ecb0,
0x1ecb0, 0x1ee00, 0x1ee03, 0x1ee05, 0x1ee1f, 0x1ee21, 0x1ee22, 0x1ee24,
0x1ee24, 0x1ee27, 0x1ee27, 0x1ee29, 0x1ee32, 0x1ee34, 0x1ee37, 0x1ee39,
0x1ee39, 0x1ee3b, 0x1ee3b, 0x1ee42, 0x1ee42, 0x1ee47, 0x1ee47, 0x1ee49,
0x1ee49, 0x1ee4b, 0x1ee4b, 0x1ee4d, 0x1ee4f, 0x1ee51, 0x1ee52, 0x1ee54,
0x1ee54, 0x1ee57, 0x1ee57, 0x1ee59, 0x1ee59, 0x1ee5b, 0x1ee5b, 0x1ee5d,
0x1ee5d, 0x1ee5f, 0x1ee5f, 0x1ee61, 0x1ee62, 0x1ee64, 0x1ee64, 0x1ee67,
0x1ee6a, 0x1ee6c, 0x1ee72, 0x1ee74, 0x1ee77, 0x1ee79, 0x1ee7c, 0x1ee7e,
0x1ee7e, 0x1ee80, 0x1ee89, 0x1ee8b, 0x1ee9b, 0x1eea1, 0x1eea3, 0x1eea5,
0x1eea9, 0x1eeab, 0x1eebb, 0x1fbf0, 0x1fbf9, 0x20000, 0x2a6df, 0x2a700,
0x2b738, 0x2b740, 0x2b81d, 0x2b820, 0x2cea1, 0x2ceb0, 0x2ebe0, 0x2f800,
0x2fa1d, 0x30000, 0x3134a, 0xe0001, 0xe0001, 0xe0020, 0xe007f, 0xe0100,
0xe01ef};
}
private static final int[] NFA_MOVES_TEMPLATE_TEXT_60 = NFA_MOVES_TEMPLATE_TEXT_60_init();
private static int[] NFA_MOVES_TEMPLATE_TEXT_60_init() {
return new int[] {'$', '$', 'A', 'Z', '_', '_', 'a', 'z', 0xa2, 0xa5,
0xaa, 0xaa, 0xb5, 0xb5, 0xba, 0xba, 0xc0, 0xd6, 0xd8, 0xf6, 0xf8, 0x2c1,
0x2c6, 0x2d1, 0x2e0, 0x2e4, 0x2ec, 0x2ec, 0x2ee, 0x2ee, 0x370, 0x374,
0x376, 0x377, 0x37a, 0x37d, 0x37f, 0x37f, 0x386, 0x386, 0x388, 0x38a,
0x38c, 0x38c, 0x38e, 0x3a1, 0x3a3, 0x3f5, 0x3f7, 0x481, 0x48a, 0x52f,
0x531, 0x556, 0x559, 0x559, 0x560, 0x588, 0x58f, 0x58f, 0x5d0, 0x5ea,
0x5ef, 0x5f2, 0x60b, 0x60b, 0x620, 0x64a, 0x66e, 0x66f, 0x671, 0x6d3,
0x6d5, 0x6d5, 0x6e5, 0x6e6, 0x6ee, 0x6ef, 0x6fa, 0x6fc, 0x6ff, 0x6ff,
0x710, 0x710, 0x712, 0x72f, 0x74d, 0x7a5, 0x7b1, 0x7b1, 0x7ca, 0x7ea,
0x7f4, 0x7f5, 0x7fa, 0x7fa, 0x7fe, 0x815, 0x81a, 0x81a, 0x824, 0x824,
0x828, 0x828, 0x840, 0x858, 0x860, 0x86a, 0x870, 0x887, 0x889, 0x88e,
0x8a0, 0x8c9, 0x904, 0x939, 0x93d, 0x93d, 0x950, 0x950, 0x958, 0x961,
0x971, 0x980, 0x985, 0x98c, 0x98f, 0x990, 0x993, 0x9a8, 0x9aa, 0x9b0,
0x9b2, 0x9b2, 0x9b6, 0x9b9, 0x9bd, 0x9bd, 0x9ce, 0x9ce, 0x9dc, 0x9dd,
0x9df, 0x9e1, 0x9f0, 0x9f3, 0x9fb, 0x9fc, 0xa05, 0xa0a, 0xa0f, 0xa10,
0xa13, 0xa28, 0xa2a, 0xa30, 0xa32, 0xa33, 0xa35, 0xa36, 0xa38, 0xa39,
0xa59, 0xa5c, 0xa5e, 0xa5e, 0xa72, 0xa74, 0xa85, 0xa8d, 0xa8f, 0xa91,
0xa93, 0xaa8, 0xaaa, 0xab0, 0xab2, 0xab3, 0xab5, 0xab9, 0xabd, 0xabd,
0xad0, 0xad0, 0xae0, 0xae1, 0xaf1, 0xaf1, 0xaf9, 0xaf9, 0xb05, 0xb0c,
0xb0f, 0xb10, 0xb13, 0xb28, 0xb2a, 0xb30, 0xb32, 0xb33, 0xb35, 0xb39,
0xb3d, 0xb3d, 0xb5c, 0xb5d, 0xb5f, 0xb61, 0xb71, 0xb71, 0xb83, 0xb83,
0xb85, 0xb8a, 0xb8e, 0xb90, 0xb92, 0xb95, 0xb99, 0xb9a, 0xb9c, 0xb9c,
0xb9e, 0xb9f, 0xba3, 0xba4, 0xba8, 0xbaa, 0xbae, 0xbb9, 0xbd0, 0xbd0,
0xbf9, 0xbf9, 0xc05, 0xc0c, 0xc0e, 0xc10, 0xc12, 0xc28, 0xc2a, 0xc39,
0xc3d, 0xc3d, 0xc58, 0xc5a, 0xc5d, 0xc5d, 0xc60, 0xc61, 0xc80, 0xc80,
0xc85, 0xc8c, 0xc8e, 0xc90, 0xc92, 0xca8, 0xcaa, 0xcb3, 0xcb5, 0xcb9,
0xcbd, 0xcbd, 0xcdd, 0xcde, 0xce0, 0xce1, 0xcf1, 0xcf2, 0xd04, 0xd0c,
0xd0e, 0xd10, 0xd12, 0xd3a, 0xd3d, 0xd3d, 0xd4e, 0xd4e, 0xd54, 0xd56,
0xd5f, 0xd61, 0xd7a, 0xd7f, 0xd85, 0xd96, 0xd9a, 0xdb1, 0xdb3, 0xdbb,
0xdbd, 0xdbd, 0xdc0, 0xdc6, 0xe01, 0xe30, 0xe32, 0xe33, 0xe3f, 0xe46,
0xe81, 0xe82, 0xe84, 0xe84, 0xe86, 0xe8a, 0xe8c, 0xea3, 0xea5, 0xea5,
0xea7, 0xeb0, 0xeb2, 0xeb3, 0xebd, 0xebd, 0xec0, 0xec4, 0xec6, 0xec6,
0xedc, 0xedf, 0xf00, 0xf00, 0xf40, 0xf47, 0xf49, 0xf6c, 0xf88, 0xf8c,
0x1000, 0x102a, 0x103f, 0x103f, 0x1050, 0x1055, 0x105a, 0x105d, 0x1061,
0x1061, 0x1065, 0x1066, 0x106e, 0x1070, 0x1075, 0x1081, 0x108e, 0x108e,
0x10a0, 0x10c5, 0x10c7, 0x10c7, 0x10cd, 0x10cd, 0x10d0, 0x10fa, 0x10fc,
0x1248, 0x124a, 0x124d, 0x1250, 0x1256, 0x1258, 0x1258, 0x125a, 0x125d,
0x1260, 0x1288, 0x128a, 0x128d, 0x1290, 0x12b0, 0x12b2, 0x12b5, 0x12b8,
0x12be, 0x12c0, 0x12c0, 0x12c2, 0x12c5, 0x12c8, 0x12d6, 0x12d8, 0x1310,
0x1312, 0x1315, 0x1318, 0x135a, 0x1380, 0x138f, 0x13a0, 0x13f5, 0x13f8,
0x13fd, 0x1401, 0x166c, 0x166f, 0x167f, 0x1681, 0x169a, 0x16a0, 0x16ea,
0x16ee, 0x16f8, 0x1700, 0x1711, 0x171f, 0x1731, 0x1740, 0x1751, 0x1760,
0x176c, 0x176e, 0x1770, 0x1780, 0x17b3, 0x17d7, 0x17d7, 0x17db, 0x17dc,
0x1820, 0x1878, 0x1880, 0x1884, 0x1887, 0x18a8, 0x18aa, 0x18aa, 0x18b0,
0x18f5, 0x1900, 0x191e, 0x1950, 0x196d, 0x1970, 0x1974, 0x1980, 0x19ab,
0x19b0, 0x19c9, 0x1a00, 0x1a16, 0x1a20, 0x1a54, 0x1aa7, 0x1aa7, 0x1b05,
0x1b33, 0x1b45, 0x1b4c, 0x1b83, 0x1ba0, 0x1bae, 0x1baf, 0x1bba, 0x1be5,
0x1c00, 0x1c23, 0x1c4d, 0x1c4f, 0x1c5a, 0x1c7d, 0x1c80, 0x1c88, 0x1c90,
0x1cba, 0x1cbd, 0x1cbf, 0x1ce9, 0x1cec, 0x1cee, 0x1cf3, 0x1cf5, 0x1cf6,
0x1cfa, 0x1cfa, 0x1d00, 0x1dbf, 0x1e00, 0x1f15, 0x1f18, 0x1f1d, 0x1f20,
0x1f45, 0x1f48, 0x1f4d, 0x1f50, 0x1f57, 0x1f59, 0x1f59, 0x1f5b, 0x1f5b,
0x1f5d, 0x1f5d, 0x1f5f, 0x1f7d, 0x1f80, 0x1fb4, 0x1fb6, 0x1fbc, 0x1fbe,
0x1fbe, 0x1fc2, 0x1fc4, 0x1fc6, 0x1fcc, 0x1fd0, 0x1fd3, 0x1fd6, 0x1fdb,
0x1fe0, 0x1fec, 0x1ff2, 0x1ff4, 0x1ff6, 0x1ffc, 0x203f, 0x2040, 0x2054,
0x2054, 0x2071, 0x2071, 0x207f, 0x207f, 0x2090, 0x209c, 0x20a0, 0x20c0,
0x2102, 0x2102, 0x2107, 0x2107, 0x210a, 0x2113, 0x2115, 0x2115, 0x2119,
0x211d, 0x2124, 0x2124, 0x2126, 0x2126, 0x2128, 0x2128, 0x212a, 0x212d,
0x212f, 0x2139, 0x213c, 0x213f, 0x2145, 0x2149, 0x214e, 0x214e, 0x2160,
0x2188, 0x2c00, 0x2ce4, 0x2ceb, 0x2cee, 0x2cf2, 0x2cf3, 0x2d00, 0x2d25,
0x2d27, 0x2d27, 0x2d2d, 0x2d2d, 0x2d30, 0x2d67, 0x2d6f, 0x2d6f, 0x2d80,
0x2d96, 0x2da0, 0x2da6, 0x2da8, 0x2dae, 0x2db0, 0x2db6, 0x2db8, 0x2dbe,
0x2dc0, 0x2dc6, 0x2dc8, 0x2dce, 0x2dd0, 0x2dd6, 0x2dd8, 0x2dde, 0x2e2f,
0x2e2f, 0x3005, 0x3007, 0x3021, 0x3029, 0x3031, 0x3035, 0x3038, 0x303c,
0x3041, 0x3096, 0x309d, 0x309f, 0x30a1, 0x30fa, 0x30fc, 0x30ff, 0x3105,
0x312f, 0x3131, 0x318e, 0x31a0, 0x31bf, 0x31f0, 0x31ff, 0x3400, 0x4dbf,
0x4e00, 0xa48c, 0xa4d0, 0xa4fd, 0xa500, 0xa60c, 0xa610, 0xa61f, 0xa62a,
0xa62b, 0xa640, 0xa66e, 0xa67f, 0xa69d, 0xa6a0, 0xa6ef, 0xa717, 0xa71f,
0xa722, 0xa788, 0xa78b, 0xa7ca, 0xa7d0, 0xa7d1, 0xa7d3, 0xa7d3, 0xa7d5,
0xa7d9, 0xa7f2, 0xa801, 0xa803, 0xa805, 0xa807, 0xa80a, 0xa80c, 0xa822,
0xa838, 0xa838, 0xa840, 0xa873, 0xa882, 0xa8b3, 0xa8f2, 0xa8f7, 0xa8fb,
0xa8fb, 0xa8fd, 0xa8fe, 0xa90a, 0xa925, 0xa930, 0xa946, 0xa960, 0xa97c,
0xa984, 0xa9b2, 0xa9cf, 0xa9cf, 0xa9e0, 0xa9e4, 0xa9e6, 0xa9ef, 0xa9fa,
0xa9fe, 0xaa00, 0xaa28, 0xaa40, 0xaa42, 0xaa44, 0xaa4b, 0xaa60, 0xaa76,
0xaa7a, 0xaa7a, 0xaa7e, 0xaaaf, 0xaab1, 0xaab1, 0xaab5, 0xaab6, 0xaab9,
0xaabd, 0xaac0, 0xaac0, 0xaac2, 0xaac2, 0xaadb, 0xaadd, 0xaae0, 0xaaea,
0xaaf2, 0xaaf4, 0xab01, 0xab06, 0xab09, 0xab0e, 0xab11, 0xab16, 0xab20,
0xab26, 0xab28, 0xab2e, 0xab30, 0xab5a, 0xab5c, 0xab69, 0xab70, 0xabe2,
0xac00, 0xd7a3, 0xd7b0, 0xd7c6, 0xd7cb, 0xd7fb, 0xf900, 0xfa6d, 0xfa70,
0xfad9, 0xfb00, 0xfb06, 0xfb13, 0xfb17, 0xfb1d, 0xfb1d, 0xfb1f, 0xfb28,
0xfb2a, 0xfb36, 0xfb38, 0xfb3c, 0xfb3e, 0xfb3e, 0xfb40, 0xfb41, 0xfb43,
0xfb44, 0xfb46, 0xfbb1, 0xfbd3, 0xfd3d, 0xfd50, 0xfd8f, 0xfd92, 0xfdc7,
0xfdf0, 0xfdfc, 0xfe33, 0xfe34, 0xfe4d, 0xfe4f, 0xfe69, 0xfe69, 0xfe70,
0xfe74, 0xfe76, 0xfefc, 0xff04, 0xff04, 0xff21, 0xff3a, 0xff3f, 0xff3f,
0xff41, 0xff5a, 0xff66, 0xffbe, 0xffc2, 0xffc7, 0xffca, 0xffcf, 0xffd2,
0xffd7, 0xffda, 0xffdc, 0xffe0, 0xffe1, 0xffe5, 0xffe6, 0x10000, 0x1000b,
0x1000d, 0x10026, 0x10028, 0x1003a, 0x1003c, 0x1003d, 0x1003f, 0x1004d,
0x10050, 0x1005d, 0x10080, 0x100fa, 0x10140, 0x10174, 0x10280, 0x1029c,
0x102a0, 0x102d0, 0x10300, 0x1031f, 0x1032d, 0x1034a, 0x10350, 0x10375,
0x10380, 0x1039d, 0x103a0, 0x103c3, 0x103c8, 0x103cf, 0x103d1, 0x103d5,
0x10400, 0x1049d, 0x104b0, 0x104d3, 0x104d8, 0x104fb, 0x10500, 0x10527,
0x10530, 0x10563, 0x10570, 0x1057a, 0x1057c, 0x1058a, 0x1058c, 0x10592,
0x10594, 0x10595, 0x10597, 0x105a1, 0x105a3, 0x105b1, 0x105b3, 0x105b9,
0x105bb, 0x105bc, 0x10600, 0x10736, 0x10740, 0x10755, 0x10760, 0x10767,
0x10780, 0x10785, 0x10787, 0x107b0, 0x107b2, 0x107ba, 0x10800, 0x10805,
0x10808, 0x10808, 0x1080a, 0x10835, 0x10837, 0x10838, 0x1083c, 0x1083c,
0x1083f, 0x10855, 0x10860, 0x10876, 0x10880, 0x1089e, 0x108e0, 0x108f2,
0x108f4, 0x108f5, 0x10900, 0x10915, 0x10920, 0x10939, 0x10980, 0x109b7,
0x109be, 0x109bf, 0x10a00, 0x10a00, 0x10a10, 0x10a13, 0x10a15, 0x10a17,
0x10a19, 0x10a35, 0x10a60, 0x10a7c, 0x10a80, 0x10a9c, 0x10ac0, 0x10ac7,
0x10ac9, 0x10ae4, 0x10b00, 0x10b35, 0x10b40, 0x10b55, 0x10b60, 0x10b72,
0x10b80, 0x10b91, 0x10c00, 0x10c48, 0x10c80, 0x10cb2, 0x10cc0, 0x10cf2,
0x10d00, 0x10d23, 0x10e80, 0x10ea9, 0x10eb0, 0x10eb1, 0x10f00, 0x10f1c,
0x10f27, 0x10f27, 0x10f30, 0x10f45, 0x10f70, 0x10f81, 0x10fb0, 0x10fc4,
0x10fe0, 0x10ff6, 0x11003, 0x11037, 0x11071, 0x11072, 0x11075, 0x11075,
0x11083, 0x110af, 0x110d0, 0x110e8, 0x11103, 0x11126, 0x11144, 0x11144,
0x11147, 0x11147, 0x11150, 0x11172, 0x11176, 0x11176, 0x11183, 0x111b2,
0x111c1, 0x111c4, 0x111da, 0x111da, 0x111dc, 0x111dc, 0x11200, 0x11211,
0x11213, 0x1122b, 0x11280, 0x11286, 0x11288, 0x11288, 0x1128a, 0x1128d,
0x1128f, 0x1129d, 0x1129f, 0x112a8, 0x112b0, 0x112de, 0x11305, 0x1130c,
0x1130f, 0x11310, 0x11313, 0x11328, 0x1132a, 0x11330, 0x11332, 0x11333,
0x11335, 0x11339, 0x1133d, 0x1133d, 0x11350, 0x11350, 0x1135d, 0x11361,
0x11400, 0x11434, 0x11447, 0x1144a, 0x1145f, 0x11461, 0x11480, 0x114af,
0x114c4, 0x114c5, 0x114c7, 0x114c7, 0x11580, 0x115ae, 0x115d8, 0x115db,
0x11600, 0x1162f, 0x11644, 0x11644, 0x11680, 0x116aa, 0x116b8, 0x116b8,
0x11700, 0x1171a, 0x11740, 0x11746, 0x11800, 0x1182b, 0x118a0, 0x118df,
0x118ff, 0x11906, 0x11909, 0x11909, 0x1190c, 0x11913, 0x11915, 0x11916,
0x11918, 0x1192f, 0x1193f, 0x1193f, 0x11941, 0x11941, 0x119a0, 0x119a7,
0x119aa, 0x119d0, 0x119e1, 0x119e1, 0x119e3, 0x119e3, 0x11a00, 0x11a00,
0x11a0b, 0x11a32, 0x11a3a, 0x11a3a, 0x11a50, 0x11a50, 0x11a5c, 0x11a89,
0x11a9d, 0x11a9d, 0x11ab0, 0x11af8, 0x11c00, 0x11c08, 0x11c0a, 0x11c2e,
0x11c40, 0x11c40, 0x11c72, 0x11c8f, 0x11d00, 0x11d06, 0x11d08, 0x11d09,
0x11d0b, 0x11d30, 0x11d46, 0x11d46, 0x11d60, 0x11d65, 0x11d67, 0x11d68,
0x11d6a, 0x11d89, 0x11d98, 0x11d98, 0x11ee0, 0x11ef2, 0x11fb0, 0x11fb0,
0x11fdd, 0x11fe0, 0x12000, 0x12399, 0x12400, 0x1246e, 0x12480, 0x12543,
0x12f90, 0x12ff0, 0x13000, 0x1342e, 0x14400, 0x14646, 0x16800, 0x16a38,
0x16a40, 0x16a5e, 0x16a70, 0x16abe, 0x16ad0, 0x16aed, 0x16b00, 0x16b2f,
0x16b40, 0x16b43, 0x16b63, 0x16b77, 0x16b7d, 0x16b8f, 0x16e40, 0x16e7f,
0x16f00, 0x16f4a, 0x16f50, 0x16f50, 0x16f93, 0x16f9f, 0x16fe0, 0x16fe1,
0x16fe3, 0x16fe3, 0x17000, 0x187f7, 0x18800, 0x18cd5, 0x18d00, 0x18d08,
0x1aff0, 0x1aff3, 0x1aff5, 0x1affb, 0x1affd, 0x1affe, 0x1b000, 0x1b122,
0x1b150, 0x1b152, 0x1b164, 0x1b167, 0x1b170, 0x1b2fb, 0x1bc00, 0x1bc6a,
0x1bc70, 0x1bc7c, 0x1bc80, 0x1bc88, 0x1bc90, 0x1bc99, 0x1d400, 0x1d454,
0x1d456, 0x1d49c, 0x1d49e, 0x1d49f, 0x1d4a2, 0x1d4a2, 0x1d4a5, 0x1d4a6,
0x1d4a9, 0x1d4ac, 0x1d4ae, 0x1d4b9, 0x1d4bb, 0x1d4bb, 0x1d4bd, 0x1d4c3,
0x1d4c5, 0x1d505, 0x1d507, 0x1d50a, 0x1d50d, 0x1d514, 0x1d516, 0x1d51c,
0x1d51e, 0x1d539, 0x1d53b, 0x1d53e, 0x1d540, 0x1d544, 0x1d546, 0x1d546,
0x1d54a, 0x1d550, 0x1d552, 0x1d6a5, 0x1d6a8, 0x1d6c0, 0x1d6c2, 0x1d6da,
0x1d6dc, 0x1d6fa, 0x1d6fc, 0x1d714, 0x1d716, 0x1d734, 0x1d736, 0x1d74e,
0x1d750, 0x1d76e, 0x1d770, 0x1d788, 0x1d78a, 0x1d7a8, 0x1d7aa, 0x1d7c2,
0x1d7c4, 0x1d7cb, 0x1df00, 0x1df1e, 0x1e100, 0x1e12c, 0x1e137, 0x1e13d,
0x1e14e, 0x1e14e, 0x1e290, 0x1e2ad, 0x1e2c0, 0x1e2eb, 0x1e2ff, 0x1e2ff,
0x1e7e0, 0x1e7e6, 0x1e7e8, 0x1e7eb, 0x1e7ed, 0x1e7ee, 0x1e7f0, 0x1e7fe,
0x1e800, 0x1e8c4, 0x1e900, 0x1e943, 0x1e94b, 0x1e94b, 0x1ecb0, 0x1ecb0,
0x1ee00, 0x1ee03, 0x1ee05, 0x1ee1f, 0x1ee21, 0x1ee22, 0x1ee24, 0x1ee24,
0x1ee27, 0x1ee27, 0x1ee29, 0x1ee32, 0x1ee34, 0x1ee37, 0x1ee39, 0x1ee39,
0x1ee3b, 0x1ee3b, 0x1ee42, 0x1ee42, 0x1ee47, 0x1ee47, 0x1ee49, 0x1ee49,
0x1ee4b, 0x1ee4b, 0x1ee4d, 0x1ee4f, 0x1ee51, 0x1ee52, 0x1ee54, 0x1ee54,
0x1ee57, 0x1ee57, 0x1ee59, 0x1ee59, 0x1ee5b, 0x1ee5b, 0x1ee5d, 0x1ee5d,
0x1ee5f, 0x1ee5f, 0x1ee61, 0x1ee62, 0x1ee64, 0x1ee64, 0x1ee67, 0x1ee6a,
0x1ee6c, 0x1ee72, 0x1ee74, 0x1ee77, 0x1ee79, 0x1ee7c, 0x1ee7e, 0x1ee7e,
0x1ee80, 0x1ee89, 0x1ee8b, 0x1ee9b, 0x1eea1, 0x1eea3, 0x1eea5, 0x1eea9,
0x1eeab, 0x1eebb, 0x20000, 0x2a6df, 0x2a700, 0x2b738, 0x2b740, 0x2b81d,
0x2b820, 0x2cea1, 0x2ceb0, 0x2ebe0, 0x2f800, 0x2fa1d, 0x30000, 0x3134a};
}
private static final int[] NFA_MOVES_TEMPLATE_TEXT_62 = NFA_MOVES_TEMPLATE_TEXT_62_init();
private static int[] NFA_MOVES_TEMPLATE_TEXT_62_init() {
return new int[] {0x0, 0x8, 0xe, 0x1b, '$', '$', '0', '9', 'A', 'Z', '_',
'_', 'a', 'z', 0x7f, 0x9f, 0xa2, 0xa5, 0xaa, 0xaa, 0xad, 0xad, 0xb5, 0xb5,
0xba, 0xba, 0xc0, 0xd6, 0xd8, 0xf6, 0xf8, 0x2c1, 0x2c6, 0x2d1, 0x2e0,
0x2e4, 0x2ec, 0x2ec, 0x2ee, 0x2ee, 0x300, 0x374, 0x376, 0x377, 0x37a,
0x37d, 0x37f, 0x37f, 0x386, 0x386, 0x388, 0x38a, 0x38c, 0x38c, 0x38e,
0x3a1, 0x3a3, 0x3f5, 0x3f7, 0x481, 0x483, 0x487, 0x48a, 0x52f, 0x531,
0x556, 0x559, 0x559, 0x560, 0x588, 0x58f, 0x58f, 0x591, 0x5bd, 0x5bf,
0x5bf, 0x5c1, 0x5c2, 0x5c4, 0x5c5, 0x5c7, 0x5c7, 0x5d0, 0x5ea, 0x5ef,
0x5f2, 0x600, 0x605, 0x60b, 0x60b, 0x610, 0x61a, 0x61c, 0x61c, 0x620,
0x669, 0x66e, 0x6d3, 0x6d5, 0x6dd, 0x6df, 0x6e8, 0x6ea, 0x6fc, 0x6ff,
0x6ff, 0x70f, 0x74a, 0x74d, 0x7b1, 0x7c0, 0x7f5, 0x7fa, 0x7fa, 0x7fd,
0x82d, 0x840, 0x85b, 0x860, 0x86a, 0x870, 0x887, 0x889, 0x88e, 0x890,
0x891, 0x898, 0x963, 0x966, 0x96f, 0x971, 0x983, 0x985, 0x98c, 0x98f,
0x990, 0x993, 0x9a8, 0x9aa, 0x9b0, 0x9b2, 0x9b2, 0x9b6, 0x9b9, 0x9bc,
0x9c4, 0x9c7, 0x9c8, 0x9cb, 0x9ce, 0x9d7, 0x9d7, 0x9dc, 0x9dd, 0x9df,
0x9e3, 0x9e6, 0x9f3, 0x9fb, 0x9fc, 0x9fe, 0x9fe, 0xa01, 0xa03, 0xa05,
0xa0a, 0xa0f, 0xa10, 0xa13, 0xa28, 0xa2a, 0xa30, 0xa32, 0xa33, 0xa35,
0xa36, 0xa38, 0xa39, 0xa3c, 0xa3c, 0xa3e, 0xa42, 0xa47, 0xa48, 0xa4b,
0xa4d, 0xa51, 0xa51, 0xa59, 0xa5c, 0xa5e, 0xa5e, 0xa66, 0xa75, 0xa81,
0xa83, 0xa85, 0xa8d, 0xa8f, 0xa91, 0xa93, 0xaa8, 0xaaa, 0xab0, 0xab2,
0xab3, 0xab5, 0xab9, 0xabc, 0xac5, 0xac7, 0xac9, 0xacb, 0xacd, 0xad0,
0xad0, 0xae0, 0xae3, 0xae6, 0xaef, 0xaf1, 0xaf1, 0xaf9, 0xaff, 0xb01,
0xb03, 0xb05, 0xb0c, 0xb0f, 0xb10, 0xb13, 0xb28, 0xb2a, 0xb30, 0xb32,
0xb33, 0xb35, 0xb39, 0xb3c, 0xb44, 0xb47, 0xb48, 0xb4b, 0xb4d, 0xb55,
0xb57, 0xb5c, 0xb5d, 0xb5f, 0xb63, 0xb66, 0xb6f, 0xb71, 0xb71, 0xb82,
0xb83, 0xb85, 0xb8a, 0xb8e, 0xb90, 0xb92, 0xb95, 0xb99, 0xb9a, 0xb9c,
0xb9c, 0xb9e, 0xb9f, 0xba3, 0xba4, 0xba8, 0xbaa, 0xbae, 0xbb9, 0xbbe,
0xbc2, 0xbc6, 0xbc8, 0xbca, 0xbcd, 0xbd0, 0xbd0, 0xbd7, 0xbd7, 0xbe6,
0xbef, 0xbf9, 0xbf9, 0xc00, 0xc0c, 0xc0e, 0xc10, 0xc12, 0xc28, 0xc2a,
0xc39, 0xc3c, 0xc44, 0xc46, 0xc48, 0xc4a, 0xc4d, 0xc55, 0xc56, 0xc58,
0xc5a, 0xc5d, 0xc5d, 0xc60, 0xc63, 0xc66, 0xc6f, 0xc80, 0xc83, 0xc85,
0xc8c, 0xc8e, 0xc90, 0xc92, 0xca8, 0xcaa, 0xcb3, 0xcb5, 0xcb9, 0xcbc,
0xcc4, 0xcc6, 0xcc8, 0xcca, 0xccd, 0xcd5, 0xcd6, 0xcdd, 0xcde, 0xce0,
0xce3, 0xce6, 0xcef, 0xcf1, 0xcf2, 0xd00, 0xd0c, 0xd0e, 0xd10, 0xd12,
0xd44, 0xd46, 0xd48, 0xd4a, 0xd4e, 0xd54, 0xd57, 0xd5f, 0xd63, 0xd66,
0xd6f, 0xd7a, 0xd7f, 0xd81, 0xd83, 0xd85, 0xd96, 0xd9a, 0xdb1, 0xdb3,
0xdbb, 0xdbd, 0xdbd, 0xdc0, 0xdc6, 0xdca, 0xdca, 0xdcf, 0xdd4, 0xdd6,
0xdd6, 0xdd8, 0xddf, 0xde6, 0xdef, 0xdf2, 0xdf3, 0xe01, 0xe3a, 0xe3f,
0xe4e, 0xe50, 0xe59, 0xe81, 0xe82, 0xe84, 0xe84, 0xe86, 0xe8a, 0xe8c,
0xea3, 0xea5, 0xea5, 0xea7, 0xebd, 0xec0, 0xec4, 0xec6, 0xec6, 0xec8,
0xecd, 0xed0, 0xed9, 0xedc, 0xedf, 0xf00, 0xf00, 0xf18, 0xf19, 0xf20,
0xf29, 0xf35, 0xf35, 0xf37, 0xf37, 0xf39, 0xf39, 0xf3e, 0xf47, 0xf49,
0xf6c, 0xf71, 0xf84, 0xf86, 0xf97, 0xf99, 0xfbc, 0xfc6, 0xfc6, 0x1000,
0x1049, 0x1050, 0x109d, 0x10a0, 0x10c5, 0x10c7, 0x10c7, 0x10cd, 0x10cd,
0x10d0, 0x10fa, 0x10fc, 0x1248, 0x124a, 0x124d, 0x1250, 0x1256, 0x1258,
0x1258, 0x125a, 0x125d, 0x1260, 0x1288, 0x128a, 0x128d, 0x1290, 0x12b0,
0x12b2, 0x12b5, 0x12b8, 0x12be, 0x12c0, 0x12c0, 0x12c2, 0x12c5, 0x12c8,
0x12d6, 0x12d8, 0x1310, 0x1312, 0x1315, 0x1318, 0x135a, 0x135d, 0x135f,
0x1380, 0x138f, 0x13a0, 0x13f5, 0x13f8, 0x13fd, 0x1401, 0x166c, 0x166f,
0x167f, 0x1681, 0x169a, 0x16a0, 0x16ea, 0x16ee, 0x16f8, 0x1700, 0x1715,
0x171f, 0x1734, 0x1740, 0x1753, 0x1760, 0x176c, 0x176e, 0x1770, 0x1772,
0x1773, 0x1780, 0x17d3, 0x17d7, 0x17d7, 0x17db, 0x17dd, 0x17e0, 0x17e9,
0x180b, 0x1819, 0x1820, 0x1878, 0x1880, 0x18aa, 0x18b0, 0x18f5, 0x1900,
0x191e, 0x1920, 0x192b, 0x1930, 0x193b, 0x1946, 0x196d, 0x1970, 0x1974,
0x1980, 0x19ab, 0x19b0, 0x19c9, 0x19d0, 0x19d9, 0x1a00, 0x1a1b, 0x1a20,
0x1a5e, 0x1a60, 0x1a7c, 0x1a7f, 0x1a89, 0x1a90, 0x1a99, 0x1aa7, 0x1aa7,
0x1ab0, 0x1abd, 0x1abf, 0x1ace, 0x1b00, 0x1b4c, 0x1b50, 0x1b59, 0x1b6b,
0x1b73, 0x1b80, 0x1bf3, 0x1c00, 0x1c37, 0x1c40, 0x1c49, 0x1c4d, 0x1c7d,
0x1c80, 0x1c88, 0x1c90, 0x1cba, 0x1cbd, 0x1cbf, 0x1cd0, 0x1cd2, 0x1cd4,
0x1cfa, 0x1d00, 0x1f15, 0x1f18, 0x1f1d, 0x1f20, 0x1f45, 0x1f48, 0x1f4d,
0x1f50, 0x1f57, 0x1f59, 0x1f59, 0x1f5b, 0x1f5b, 0x1f5d, 0x1f5d, 0x1f5f,
0x1f7d, 0x1f80, 0x1fb4, 0x1fb6, 0x1fbc, 0x1fbe, 0x1fbe, 0x1fc2, 0x1fc4,
0x1fc6, 0x1fcc, 0x1fd0, 0x1fd3, 0x1fd6, 0x1fdb, 0x1fe0, 0x1fec, 0x1ff2,
0x1ff4, 0x1ff6, 0x1ffc, 0x200b, 0x200f, 0x202a, 0x202e, 0x203f, 0x2040,
0x2054, 0x2054, 0x2060, 0x2064, 0x2066, 0x206f, 0x2071, 0x2071, 0x207f,
0x207f, 0x2090, 0x209c, 0x20a0, 0x20c0, 0x20d0, 0x20dc, 0x20e1, 0x20e1,
0x20e5, 0x20f0, 0x2102, 0x2102, 0x2107, 0x2107, 0x210a, 0x2113, 0x2115,
0x2115, 0x2119, 0x211d, 0x2124, 0x2124, 0x2126, 0x2126, 0x2128, 0x2128,
0x212a, 0x212d, 0x212f, 0x2139, 0x213c, 0x213f, 0x2145, 0x2149, 0x214e,
0x214e, 0x2160, 0x2188, 0x2c00, 0x2ce4, 0x2ceb, 0x2cf3, 0x2d00, 0x2d25,
0x2d27, 0x2d27, 0x2d2d, 0x2d2d, 0x2d30, 0x2d67, 0x2d6f, 0x2d6f, 0x2d7f,
0x2d96, 0x2da0, 0x2da6, 0x2da8, 0x2dae, 0x2db0, 0x2db6, 0x2db8, 0x2dbe,
0x2dc0, 0x2dc6, 0x2dc8, 0x2dce, 0x2dd0, 0x2dd6, 0x2dd8, 0x2dde, 0x2de0,
0x2dff, 0x2e2f, 0x2e2f, 0x3005, 0x3007, 0x3021, 0x302f, 0x3031, 0x3035,
0x3038, 0x303c, 0x3041, 0x3096, 0x3099, 0x309a, 0x309d, 0x309f, 0x30a1,
0x30fa, 0x30fc, 0x30ff, 0x3105, 0x312f, 0x3131, 0x318e, 0x31a0, 0x31bf,
0x31f0, 0x31ff, 0x3400, 0x4dbf, 0x4e00, 0xa48c, 0xa4d0, 0xa4fd, 0xa500,
0xa60c, 0xa610, 0xa62b, 0xa640, 0xa66f, 0xa674, 0xa67d, 0xa67f, 0xa6f1,
0xa717, 0xa71f, 0xa722, 0xa788, 0xa78b, 0xa7ca, 0xa7d0, 0xa7d1, 0xa7d3,
0xa7d3, 0xa7d5, 0xa7d9, 0xa7f2, 0xa827, 0xa82c, 0xa82c, 0xa838, 0xa838,
0xa840, 0xa873, 0xa880, 0xa8c5, 0xa8d0, 0xa8d9, 0xa8e0, 0xa8f7, 0xa8fb,
0xa8fb, 0xa8fd, 0xa92d, 0xa930, 0xa953, 0xa960, 0xa97c, 0xa980, 0xa9c0,
0xa9cf, 0xa9d9, 0xa9e0, 0xa9fe, 0xaa00, 0xaa36, 0xaa40, 0xaa4d, 0xaa50,
0xaa59, 0xaa60, 0xaa76, 0xaa7a, 0xaac2, 0xaadb, 0xaadd, 0xaae0, 0xaaef,
0xaaf2, 0xaaf6, 0xab01, 0xab06, 0xab09, 0xab0e, 0xab11, 0xab16, 0xab20,
0xab26, 0xab28, 0xab2e, 0xab30, 0xab5a, 0xab5c, 0xab69, 0xab70, 0xabea,
0xabec, 0xabed, 0xabf0, 0xabf9, 0xac00, 0xd7a3, 0xd7b0, 0xd7c6, 0xd7cb,
0xd7fb, 0xf900, 0xfa6d, 0xfa70, 0xfad9, 0xfb00, 0xfb06, 0xfb13, 0xfb17,
0xfb1d, 0xfb28, 0xfb2a, 0xfb36, 0xfb38, 0xfb3c, 0xfb3e, 0xfb3e, 0xfb40,
0xfb41, 0xfb43, 0xfb44, 0xfb46, 0xfbb1, 0xfbd3, 0xfd3d, 0xfd50, 0xfd8f,
0xfd92, 0xfdc7, 0xfdf0, 0xfdfc, 0xfe00, 0xfe0f, 0xfe20, 0xfe2f, 0xfe33,
0xfe34, 0xfe4d, 0xfe4f, 0xfe69, 0xfe69, 0xfe70, 0xfe74, 0xfe76, 0xfefc,
0xfeff, 0xfeff, 0xff04, 0xff04, 0xff10, 0xff19, 0xff21, 0xff3a, 0xff3f,
0xff3f, 0xff41, 0xff5a, 0xff66, 0xffbe, 0xffc2, 0xffc7, 0xffca, 0xffcf,
0xffd2, 0xffd7, 0xffda, 0xffdc, 0xffe0, 0xffe1, 0xffe5, 0xffe6, 0xfff9,
0xfffb, 0x10000, 0x1000b, 0x1000d, 0x10026, 0x10028, 0x1003a, 0x1003c,
0x1003d, 0x1003f, 0x1004d, 0x10050, 0x1005d, 0x10080, 0x100fa, 0x10140,
0x10174, 0x101fd, 0x101fd, 0x10280, 0x1029c, 0x102a0, 0x102d0, 0x102e0,
0x102e0, 0x10300, 0x1031f, 0x1032d, 0x1034a, 0x10350, 0x1037a, 0x10380,
0x1039d, 0x103a0, 0x103c3, 0x103c8, 0x103cf, 0x103d1, 0x103d5, 0x10400,
0x1049d, 0x104a0, 0x104a9, 0x104b0, 0x104d3, 0x104d8, 0x104fb, 0x10500,
0x10527, 0x10530, 0x10563, 0x10570, 0x1057a, 0x1057c, 0x1058a, 0x1058c,
0x10592, 0x10594, 0x10595, 0x10597, 0x105a1, 0x105a3, 0x105b1, 0x105b3,
0x105b9, 0x105bb, 0x105bc, 0x10600, 0x10736, 0x10740, 0x10755, 0x10760,
0x10767, 0x10780, 0x10785, 0x10787, 0x107b0, 0x107b2, 0x107ba, 0x10800,
0x10805, 0x10808, 0x10808, 0x1080a, 0x10835, 0x10837, 0x10838, 0x1083c,
0x1083c, 0x1083f, 0x10855, 0x10860, 0x10876, 0x10880, 0x1089e, 0x108e0,
0x108f2, 0x108f4, 0x108f5, 0x10900, 0x10915, 0x10920, 0x10939, 0x10980,
0x109b7, 0x109be, 0x109bf, 0x10a00, 0x10a03, 0x10a05, 0x10a06, 0x10a0c,
0x10a13, 0x10a15, 0x10a17, 0x10a19, 0x10a35, 0x10a38, 0x10a3a, 0x10a3f,
0x10a3f, 0x10a60, 0x10a7c, 0x10a80, 0x10a9c, 0x10ac0, 0x10ac7, 0x10ac9,
0x10ae6, 0x10b00, 0x10b35, 0x10b40, 0x10b55, 0x10b60, 0x10b72, 0x10b80,
0x10b91, 0x10c00, 0x10c48, 0x10c80, 0x10cb2, 0x10cc0, 0x10cf2, 0x10d00,
0x10d27, 0x10d30, 0x10d39, 0x10e80, 0x10ea9, 0x10eab, 0x10eac, 0x10eb0,
0x10eb1, 0x10f00, 0x10f1c, 0x10f27, 0x10f27, 0x10f30, 0x10f50, 0x10f70,
0x10f85, 0x10fb0, 0x10fc4, 0x10fe0, 0x10ff6, 0x11000, 0x11046, 0x11066,
0x11075, 0x1107f, 0x110ba, 0x110bd, 0x110bd, 0x110c2, 0x110c2, 0x110cd,
0x110cd, 0x110d0, 0x110e8, 0x110f0, 0x110f9, 0x11100, 0x11134, 0x11136,
0x1113f, 0x11144, 0x11147, 0x11150, 0x11173, 0x11176, 0x11176, 0x11180,
0x111c4, 0x111c9, 0x111cc, 0x111ce, 0x111da, 0x111dc, 0x111dc, 0x11200,
0x11211, 0x11213, 0x11237, 0x1123e, 0x1123e, 0x11280, 0x11286, 0x11288,
0x11288, 0x1128a, 0x1128d, 0x1128f, 0x1129d, 0x1129f, 0x112a8, 0x112b0,
0x112ea, 0x112f0, 0x112f9, 0x11300, 0x11303, 0x11305, 0x1130c, 0x1130f,
0x11310, 0x11313, 0x11328, 0x1132a, 0x11330, 0x11332, 0x11333, 0x11335,
0x11339, 0x1133b, 0x11344, 0x11347, 0x11348, 0x1134b, 0x1134d, 0x11350,
0x11350, 0x11357, 0x11357, 0x1135d, 0x11363, 0x11366, 0x1136c, 0x11370,
0x11374, 0x11400, 0x1144a, 0x11450, 0x11459, 0x1145e, 0x11461, 0x11480,
0x114c5, 0x114c7, 0x114c7, 0x114d0, 0x114d9, 0x11580, 0x115b5, 0x115b8,
0x115c0, 0x115d8, 0x115dd, 0x11600, 0x11640, 0x11644, 0x11644, 0x11650,
0x11659, 0x11680, 0x116b8, 0x116c0, 0x116c9, 0x11700, 0x1171a, 0x1171d,
0x1172b, 0x11730, 0x11739, 0x11740, 0x11746, 0x11800, 0x1183a, 0x118a0,
0x118e9, 0x118ff, 0x11906, 0x11909, 0x11909, 0x1190c, 0x11913, 0x11915,
0x11916, 0x11918, 0x11935, 0x11937, 0x11938, 0x1193b, 0x11943, 0x11950,
0x11959, 0x119a0, 0x119a7, 0x119aa, 0x119d7, 0x119da, 0x119e1, 0x119e3,
0x119e4, 0x11a00, 0x11a3e, 0x11a47, 0x11a47, 0x11a50, 0x11a99, 0x11a9d,
0x11a9d, 0x11ab0, 0x11af8, 0x11c00, 0x11c08, 0x11c0a, 0x11c36, 0x11c38,
0x11c40, 0x11c50, 0x11c59, 0x11c72, 0x11c8f, 0x11c92, 0x11ca7, 0x11ca9,
0x11cb6, 0x11d00, 0x11d06, 0x11d08, 0x11d09, 0x11d0b, 0x11d36, 0x11d3a,
0x11d3a, 0x11d3c, 0x11d3d, 0x11d3f, 0x11d47, 0x11d50, 0x11d59, 0x11d60,
0x11d65, 0x11d67, 0x11d68, 0x11d6a, 0x11d8e, 0x11d90, 0x11d91, 0x11d93,
0x11d98, 0x11da0, 0x11da9, 0x11ee0, 0x11ef6, 0x11fb0, 0x11fb0, 0x11fdd,
0x11fe0, 0x12000, 0x12399, 0x12400, 0x1246e, 0x12480, 0x12543, 0x12f90,
0x12ff0, 0x13000, 0x1342e, 0x13430, 0x13438, 0x14400, 0x14646, 0x16800,
0x16a38, 0x16a40, 0x16a5e, 0x16a60, 0x16a69, 0x16a70, 0x16abe, 0x16ac0,
0x16ac9, 0x16ad0, 0x16aed, 0x16af0, 0x16af4, 0x16b00, 0x16b36, 0x16b40,
0x16b43, 0x16b50, 0x16b59, 0x16b63, 0x16b77, 0x16b7d, 0x16b8f, 0x16e40,
0x16e7f, 0x16f00, 0x16f4a, 0x16f4f, 0x16f87, 0x16f8f, 0x16f9f, 0x16fe0,
0x16fe1, 0x16fe3, 0x16fe4, 0x16ff0, 0x16ff1, 0x17000, 0x187f7, 0x18800,
0x18cd5, 0x18d00, 0x18d08, 0x1aff0, 0x1aff3, 0x1aff5, 0x1affb, 0x1affd,
0x1affe, 0x1b000, 0x1b122, 0x1b150, 0x1b152, 0x1b164, 0x1b167, 0x1b170,
0x1b2fb, 0x1bc00, 0x1bc6a, 0x1bc70, 0x1bc7c, 0x1bc80, 0x1bc88, 0x1bc90,
0x1bc99, 0x1bc9d, 0x1bc9e, 0x1bca0, 0x1bca3, 0x1cf00, 0x1cf2d, 0x1cf30,
0x1cf46, 0x1d165, 0x1d169, 0x1d16d, 0x1d182, 0x1d185, 0x1d18b, 0x1d1aa,
0x1d1ad, 0x1d242, 0x1d244, 0x1d400, 0x1d454, 0x1d456, 0x1d49c, 0x1d49e,
0x1d49f, 0x1d4a2, 0x1d4a2, 0x1d4a5, 0x1d4a6, 0x1d4a9, 0x1d4ac, 0x1d4ae,
0x1d4b9, 0x1d4bb, 0x1d4bb, 0x1d4bd, 0x1d4c3, 0x1d4c5, 0x1d505, 0x1d507,
0x1d50a, 0x1d50d, 0x1d514, 0x1d516, 0x1d51c, 0x1d51e, 0x1d539, 0x1d53b,
0x1d53e, 0x1d540, 0x1d544, 0x1d546, 0x1d546, 0x1d54a, 0x1d550, 0x1d552,
0x1d6a5, 0x1d6a8, 0x1d6c0, 0x1d6c2, 0x1d6da, 0x1d6dc, 0x1d6fa, 0x1d6fc,
0x1d714, 0x1d716, 0x1d734, 0x1d736, 0x1d74e, 0x1d750, 0x1d76e, 0x1d770,
0x1d788, 0x1d78a, 0x1d7a8, 0x1d7aa, 0x1d7c2, 0x1d7c4, 0x1d7cb, 0x1d7ce,
0x1d7ff, 0x1da00, 0x1da36, 0x1da3b, 0x1da6c, 0x1da75, 0x1da75, 0x1da84,
0x1da84, 0x1da9b, 0x1da9f, 0x1daa1, 0x1daaf, 0x1df00, 0x1df1e, 0x1e000,
0x1e006, 0x1e008, 0x1e018, 0x1e01b, 0x1e021, 0x1e023, 0x1e024, 0x1e026,
0x1e02a, 0x1e100, 0x1e12c, 0x1e130, 0x1e13d, 0x1e140, 0x1e149, 0x1e14e,
0x1e14e, 0x1e290, 0x1e2ae, 0x1e2c0, 0x1e2f9, 0x1e2ff, 0x1e2ff, 0x1e7e0,
0x1e7e6, 0x1e7e8, 0x1e7eb, 0x1e7ed, 0x1e7ee, 0x1e7f0, 0x1e7fe, 0x1e800,
0x1e8c4, 0x1e8d0, 0x1e8d6, 0x1e900, 0x1e94b, 0x1e950, 0x1e959, 0x1ecb0,
0x1ecb0, 0x1ee00, 0x1ee03, 0x1ee05, 0x1ee1f, 0x1ee21, 0x1ee22, 0x1ee24,
0x1ee24, 0x1ee27, 0x1ee27, 0x1ee29, 0x1ee32, 0x1ee34, 0x1ee37, 0x1ee39,
0x1ee39, 0x1ee3b, 0x1ee3b, 0x1ee42, 0x1ee42, 0x1ee47, 0x1ee47, 0x1ee49,
0x1ee49, 0x1ee4b, 0x1ee4b, 0x1ee4d, 0x1ee4f, 0x1ee51, 0x1ee52, 0x1ee54,
0x1ee54, 0x1ee57, 0x1ee57, 0x1ee59, 0x1ee59, 0x1ee5b, 0x1ee5b, 0x1ee5d,
0x1ee5d, 0x1ee5f, 0x1ee5f, 0x1ee61, 0x1ee62, 0x1ee64, 0x1ee64, 0x1ee67,
0x1ee6a, 0x1ee6c, 0x1ee72, 0x1ee74, 0x1ee77, 0x1ee79, 0x1ee7c, 0x1ee7e,
0x1ee7e, 0x1ee80, 0x1ee89, 0x1ee8b, 0x1ee9b, 0x1eea1, 0x1eea3, 0x1eea5,
0x1eea9, 0x1eeab, 0x1eebb, 0x1fbf0, 0x1fbf9, 0x20000, 0x2a6df, 0x2a700,
0x2b738, 0x2b740, 0x2b81d, 0x2b820, 0x2cea1, 0x2ceb0, 0x2ebe0, 0x2f800,
0x2fa1d, 0x30000, 0x3134a, 0xe0001, 0xe0001, 0xe0020, 0xe007f, 0xe0100,
0xe01ef};
}
private static final int[] NFA_MOVES_TEMPLATE_TEXT_65 = NFA_MOVES_TEMPLATE_TEXT_65_init();
private static int[] NFA_MOVES_TEMPLATE_TEXT_65_init() {
return new int[] {'$', '$', 'A', 'Z', '_', '_', 'a', 'z', 0xa2, 0xa5,
0xaa, 0xaa, 0xb5, 0xb5, 0xba, 0xba, 0xc0, 0xd6, 0xd8, 0xf6, 0xf8, 0x2c1,
0x2c6, 0x2d1, 0x2e0, 0x2e4, 0x2ec, 0x2ec, 0x2ee, 0x2ee, 0x370, 0x374,
0x376, 0x377, 0x37a, 0x37d, 0x37f, 0x37f, 0x386, 0x386, 0x388, 0x38a,
0x38c, 0x38c, 0x38e, 0x3a1, 0x3a3, 0x3f5, 0x3f7, 0x481, 0x48a, 0x52f,
0x531, 0x556, 0x559, 0x559, 0x560, 0x588, 0x58f, 0x58f, 0x5d0, 0x5ea,
0x5ef, 0x5f2, 0x60b, 0x60b, 0x620, 0x64a, 0x66e, 0x66f, 0x671, 0x6d3,
0x6d5, 0x6d5, 0x6e5, 0x6e6, 0x6ee, 0x6ef, 0x6fa, 0x6fc, 0x6ff, 0x6ff,
0x710, 0x710, 0x712, 0x72f, 0x74d, 0x7a5, 0x7b1, 0x7b1, 0x7ca, 0x7ea,
0x7f4, 0x7f5, 0x7fa, 0x7fa, 0x7fe, 0x815, 0x81a, 0x81a, 0x824, 0x824,
0x828, 0x828, 0x840, 0x858, 0x860, 0x86a, 0x870, 0x887, 0x889, 0x88e,
0x8a0, 0x8c9, 0x904, 0x939, 0x93d, 0x93d, 0x950, 0x950, 0x958, 0x961,
0x971, 0x980, 0x985, 0x98c, 0x98f, 0x990, 0x993, 0x9a8, 0x9aa, 0x9b0,
0x9b2, 0x9b2, 0x9b6, 0x9b9, 0x9bd, 0x9bd, 0x9ce, 0x9ce, 0x9dc, 0x9dd,
0x9df, 0x9e1, 0x9f0, 0x9f3, 0x9fb, 0x9fc, 0xa05, 0xa0a, 0xa0f, 0xa10,
0xa13, 0xa28, 0xa2a, 0xa30, 0xa32, 0xa33, 0xa35, 0xa36, 0xa38, 0xa39,
0xa59, 0xa5c, 0xa5e, 0xa5e, 0xa72, 0xa74, 0xa85, 0xa8d, 0xa8f, 0xa91,
0xa93, 0xaa8, 0xaaa, 0xab0, 0xab2, 0xab3, 0xab5, 0xab9, 0xabd, 0xabd,
0xad0, 0xad0, 0xae0, 0xae1, 0xaf1, 0xaf1, 0xaf9, 0xaf9, 0xb05, 0xb0c,
0xb0f, 0xb10, 0xb13, 0xb28, 0xb2a, 0xb30, 0xb32, 0xb33, 0xb35, 0xb39,
0xb3d, 0xb3d, 0xb5c, 0xb5d, 0xb5f, 0xb61, 0xb71, 0xb71, 0xb83, 0xb83,
0xb85, 0xb8a, 0xb8e, 0xb90, 0xb92, 0xb95, 0xb99, 0xb9a, 0xb9c, 0xb9c,
0xb9e, 0xb9f, 0xba3, 0xba4, 0xba8, 0xbaa, 0xbae, 0xbb9, 0xbd0, 0xbd0,
0xbf9, 0xbf9, 0xc05, 0xc0c, 0xc0e, 0xc10, 0xc12, 0xc28, 0xc2a, 0xc39,
0xc3d, 0xc3d, 0xc58, 0xc5a, 0xc5d, 0xc5d, 0xc60, 0xc61, 0xc80, 0xc80,
0xc85, 0xc8c, 0xc8e, 0xc90, 0xc92, 0xca8, 0xcaa, 0xcb3, 0xcb5, 0xcb9,
0xcbd, 0xcbd, 0xcdd, 0xcde, 0xce0, 0xce1, 0xcf1, 0xcf2, 0xd04, 0xd0c,
0xd0e, 0xd10, 0xd12, 0xd3a, 0xd3d, 0xd3d, 0xd4e, 0xd4e, 0xd54, 0xd56,
0xd5f, 0xd61, 0xd7a, 0xd7f, 0xd85, 0xd96, 0xd9a, 0xdb1, 0xdb3, 0xdbb,
0xdbd, 0xdbd, 0xdc0, 0xdc6, 0xe01, 0xe30, 0xe32, 0xe33, 0xe3f, 0xe46,
0xe81, 0xe82, 0xe84, 0xe84, 0xe86, 0xe8a, 0xe8c, 0xea3, 0xea5, 0xea5,
0xea7, 0xeb0, 0xeb2, 0xeb3, 0xebd, 0xebd, 0xec0, 0xec4, 0xec6, 0xec6,
0xedc, 0xedf, 0xf00, 0xf00, 0xf40, 0xf47, 0xf49, 0xf6c, 0xf88, 0xf8c,
0x1000, 0x102a, 0x103f, 0x103f, 0x1050, 0x1055, 0x105a, 0x105d, 0x1061,
0x1061, 0x1065, 0x1066, 0x106e, 0x1070, 0x1075, 0x1081, 0x108e, 0x108e,
0x10a0, 0x10c5, 0x10c7, 0x10c7, 0x10cd, 0x10cd, 0x10d0, 0x10fa, 0x10fc,
0x1248, 0x124a, 0x124d, 0x1250, 0x1256, 0x1258, 0x1258, 0x125a, 0x125d,
0x1260, 0x1288, 0x128a, 0x128d, 0x1290, 0x12b0, 0x12b2, 0x12b5, 0x12b8,
0x12be, 0x12c0, 0x12c0, 0x12c2, 0x12c5, 0x12c8, 0x12d6, 0x12d8, 0x1310,
0x1312, 0x1315, 0x1318, 0x135a, 0x1380, 0x138f, 0x13a0, 0x13f5, 0x13f8,
0x13fd, 0x1401, 0x166c, 0x166f, 0x167f, 0x1681, 0x169a, 0x16a0, 0x16ea,
0x16ee, 0x16f8, 0x1700, 0x1711, 0x171f, 0x1731, 0x1740, 0x1751, 0x1760,
0x176c, 0x176e, 0x1770, 0x1780, 0x17b3, 0x17d7, 0x17d7, 0x17db, 0x17dc,
0x1820, 0x1878, 0x1880, 0x1884, 0x1887, 0x18a8, 0x18aa, 0x18aa, 0x18b0,
0x18f5, 0x1900, 0x191e, 0x1950, 0x196d, 0x1970, 0x1974, 0x1980, 0x19ab,
0x19b0, 0x19c9, 0x1a00, 0x1a16, 0x1a20, 0x1a54, 0x1aa7, 0x1aa7, 0x1b05,
0x1b33, 0x1b45, 0x1b4c, 0x1b83, 0x1ba0, 0x1bae, 0x1baf, 0x1bba, 0x1be5,
0x1c00, 0x1c23, 0x1c4d, 0x1c4f, 0x1c5a, 0x1c7d, 0x1c80, 0x1c88, 0x1c90,
0x1cba, 0x1cbd, 0x1cbf, 0x1ce9, 0x1cec, 0x1cee, 0x1cf3, 0x1cf5, 0x1cf6,
0x1cfa, 0x1cfa, 0x1d00, 0x1dbf, 0x1e00, 0x1f15, 0x1f18, 0x1f1d, 0x1f20,
0x1f45, 0x1f48, 0x1f4d, 0x1f50, 0x1f57, 0x1f59, 0x1f59, 0x1f5b, 0x1f5b,
0x1f5d, 0x1f5d, 0x1f5f, 0x1f7d, 0x1f80, 0x1fb4, 0x1fb6, 0x1fbc, 0x1fbe,
0x1fbe, 0x1fc2, 0x1fc4, 0x1fc6, 0x1fcc, 0x1fd0, 0x1fd3, 0x1fd6, 0x1fdb,
0x1fe0, 0x1fec, 0x1ff2, 0x1ff4, 0x1ff6, 0x1ffc, 0x203f, 0x2040, 0x2054,
0x2054, 0x2071, 0x2071, 0x207f, 0x207f, 0x2090, 0x209c, 0x20a0, 0x20c0,
0x2102, 0x2102, 0x2107, 0x2107, 0x210a, 0x2113, 0x2115, 0x2115, 0x2119,
0x211d, 0x2124, 0x2124, 0x2126, 0x2126, 0x2128, 0x2128, 0x212a, 0x212d,
0x212f, 0x2139, 0x213c, 0x213f, 0x2145, 0x2149, 0x214e, 0x214e, 0x2160,
0x2188, 0x2c00, 0x2ce4, 0x2ceb, 0x2cee, 0x2cf2, 0x2cf3, 0x2d00, 0x2d25,
0x2d27, 0x2d27, 0x2d2d, 0x2d2d, 0x2d30, 0x2d67, 0x2d6f, 0x2d6f, 0x2d80,
0x2d96, 0x2da0, 0x2da6, 0x2da8, 0x2dae, 0x2db0, 0x2db6, 0x2db8, 0x2dbe,
0x2dc0, 0x2dc6, 0x2dc8, 0x2dce, 0x2dd0, 0x2dd6, 0x2dd8, 0x2dde, 0x2e2f,
0x2e2f, 0x3005, 0x3007, 0x3021, 0x3029, 0x3031, 0x3035, 0x3038, 0x303c,
0x3041, 0x3096, 0x309d, 0x309f, 0x30a1, 0x30fa, 0x30fc, 0x30ff, 0x3105,
0x312f, 0x3131, 0x318e, 0x31a0, 0x31bf, 0x31f0, 0x31ff, 0x3400, 0x4dbf,
0x4e00, 0xa48c, 0xa4d0, 0xa4fd, 0xa500, 0xa60c, 0xa610, 0xa61f, 0xa62a,
0xa62b, 0xa640, 0xa66e, 0xa67f, 0xa69d, 0xa6a0, 0xa6ef, 0xa717, 0xa71f,
0xa722, 0xa788, 0xa78b, 0xa7ca, 0xa7d0, 0xa7d1, 0xa7d3, 0xa7d3, 0xa7d5,
0xa7d9, 0xa7f2, 0xa801, 0xa803, 0xa805, 0xa807, 0xa80a, 0xa80c, 0xa822,
0xa838, 0xa838, 0xa840, 0xa873, 0xa882, 0xa8b3, 0xa8f2, 0xa8f7, 0xa8fb,
0xa8fb, 0xa8fd, 0xa8fe, 0xa90a, 0xa925, 0xa930, 0xa946, 0xa960, 0xa97c,
0xa984, 0xa9b2, 0xa9cf, 0xa9cf, 0xa9e0, 0xa9e4, 0xa9e6, 0xa9ef, 0xa9fa,
0xa9fe, 0xaa00, 0xaa28, 0xaa40, 0xaa42, 0xaa44, 0xaa4b, 0xaa60, 0xaa76,
0xaa7a, 0xaa7a, 0xaa7e, 0xaaaf, 0xaab1, 0xaab1, 0xaab5, 0xaab6, 0xaab9,
0xaabd, 0xaac0, 0xaac0, 0xaac2, 0xaac2, 0xaadb, 0xaadd, 0xaae0, 0xaaea,
0xaaf2, 0xaaf4, 0xab01, 0xab06, 0xab09, 0xab0e, 0xab11, 0xab16, 0xab20,
0xab26, 0xab28, 0xab2e, 0xab30, 0xab5a, 0xab5c, 0xab69, 0xab70, 0xabe2,
0xac00, 0xd7a3, 0xd7b0, 0xd7c6, 0xd7cb, 0xd7fb, 0xf900, 0xfa6d, 0xfa70,
0xfad9, 0xfb00, 0xfb06, 0xfb13, 0xfb17, 0xfb1d, 0xfb1d, 0xfb1f, 0xfb28,
0xfb2a, 0xfb36, 0xfb38, 0xfb3c, 0xfb3e, 0xfb3e, 0xfb40, 0xfb41, 0xfb43,
0xfb44, 0xfb46, 0xfbb1, 0xfbd3, 0xfd3d, 0xfd50, 0xfd8f, 0xfd92, 0xfdc7,
0xfdf0, 0xfdfc, 0xfe33, 0xfe34, 0xfe4d, 0xfe4f, 0xfe69, 0xfe69, 0xfe70,
0xfe74, 0xfe76, 0xfefc, 0xff04, 0xff04, 0xff21, 0xff3a, 0xff3f, 0xff3f,
0xff41, 0xff5a, 0xff66, 0xffbe, 0xffc2, 0xffc7, 0xffca, 0xffcf, 0xffd2,
0xffd7, 0xffda, 0xffdc, 0xffe0, 0xffe1, 0xffe5, 0xffe6, 0x10000, 0x1000b,
0x1000d, 0x10026, 0x10028, 0x1003a, 0x1003c, 0x1003d, 0x1003f, 0x1004d,
0x10050, 0x1005d, 0x10080, 0x100fa, 0x10140, 0x10174, 0x10280, 0x1029c,
0x102a0, 0x102d0, 0x10300, 0x1031f, 0x1032d, 0x1034a, 0x10350, 0x10375,
0x10380, 0x1039d, 0x103a0, 0x103c3, 0x103c8, 0x103cf, 0x103d1, 0x103d5,
0x10400, 0x1049d, 0x104b0, 0x104d3, 0x104d8, 0x104fb, 0x10500, 0x10527,
0x10530, 0x10563, 0x10570, 0x1057a, 0x1057c, 0x1058a, 0x1058c, 0x10592,
0x10594, 0x10595, 0x10597, 0x105a1, 0x105a3, 0x105b1, 0x105b3, 0x105b9,
0x105bb, 0x105bc, 0x10600, 0x10736, 0x10740, 0x10755, 0x10760, 0x10767,
0x10780, 0x10785, 0x10787, 0x107b0, 0x107b2, 0x107ba, 0x10800, 0x10805,
0x10808, 0x10808, 0x1080a, 0x10835, 0x10837, 0x10838, 0x1083c, 0x1083c,
0x1083f, 0x10855, 0x10860, 0x10876, 0x10880, 0x1089e, 0x108e0, 0x108f2,
0x108f4, 0x108f5, 0x10900, 0x10915, 0x10920, 0x10939, 0x10980, 0x109b7,
0x109be, 0x109bf, 0x10a00, 0x10a00, 0x10a10, 0x10a13, 0x10a15, 0x10a17,
0x10a19, 0x10a35, 0x10a60, 0x10a7c, 0x10a80, 0x10a9c, 0x10ac0, 0x10ac7,
0x10ac9, 0x10ae4, 0x10b00, 0x10b35, 0x10b40, 0x10b55, 0x10b60, 0x10b72,
0x10b80, 0x10b91, 0x10c00, 0x10c48, 0x10c80, 0x10cb2, 0x10cc0, 0x10cf2,
0x10d00, 0x10d23, 0x10e80, 0x10ea9, 0x10eb0, 0x10eb1, 0x10f00, 0x10f1c,
0x10f27, 0x10f27, 0x10f30, 0x10f45, 0x10f70, 0x10f81, 0x10fb0, 0x10fc4,
0x10fe0, 0x10ff6, 0x11003, 0x11037, 0x11071, 0x11072, 0x11075, 0x11075,
0x11083, 0x110af, 0x110d0, 0x110e8, 0x11103, 0x11126, 0x11144, 0x11144,
0x11147, 0x11147, 0x11150, 0x11172, 0x11176, 0x11176, 0x11183, 0x111b2,
0x111c1, 0x111c4, 0x111da, 0x111da, 0x111dc, 0x111dc, 0x11200, 0x11211,
0x11213, 0x1122b, 0x11280, 0x11286, 0x11288, 0x11288, 0x1128a, 0x1128d,
0x1128f, 0x1129d, 0x1129f, 0x112a8, 0x112b0, 0x112de, 0x11305, 0x1130c,
0x1130f, 0x11310, 0x11313, 0x11328, 0x1132a, 0x11330, 0x11332, 0x11333,
0x11335, 0x11339, 0x1133d, 0x1133d, 0x11350, 0x11350, 0x1135d, 0x11361,
0x11400, 0x11434, 0x11447, 0x1144a, 0x1145f, 0x11461, 0x11480, 0x114af,
0x114c4, 0x114c5, 0x114c7, 0x114c7, 0x11580, 0x115ae, 0x115d8, 0x115db,
0x11600, 0x1162f, 0x11644, 0x11644, 0x11680, 0x116aa, 0x116b8, 0x116b8,
0x11700, 0x1171a, 0x11740, 0x11746, 0x11800, 0x1182b, 0x118a0, 0x118df,
0x118ff, 0x11906, 0x11909, 0x11909, 0x1190c, 0x11913, 0x11915, 0x11916,
0x11918, 0x1192f, 0x1193f, 0x1193f, 0x11941, 0x11941, 0x119a0, 0x119a7,
0x119aa, 0x119d0, 0x119e1, 0x119e1, 0x119e3, 0x119e3, 0x11a00, 0x11a00,
0x11a0b, 0x11a32, 0x11a3a, 0x11a3a, 0x11a50, 0x11a50, 0x11a5c, 0x11a89,
0x11a9d, 0x11a9d, 0x11ab0, 0x11af8, 0x11c00, 0x11c08, 0x11c0a, 0x11c2e,
0x11c40, 0x11c40, 0x11c72, 0x11c8f, 0x11d00, 0x11d06, 0x11d08, 0x11d09,
0x11d0b, 0x11d30, 0x11d46, 0x11d46, 0x11d60, 0x11d65, 0x11d67, 0x11d68,
0x11d6a, 0x11d89, 0x11d98, 0x11d98, 0x11ee0, 0x11ef2, 0x11fb0, 0x11fb0,
0x11fdd, 0x11fe0, 0x12000, 0x12399, 0x12400, 0x1246e, 0x12480, 0x12543,
0x12f90, 0x12ff0, 0x13000, 0x1342e, 0x14400, 0x14646, 0x16800, 0x16a38,
0x16a40, 0x16a5e, 0x16a70, 0x16abe, 0x16ad0, 0x16aed, 0x16b00, 0x16b2f,
0x16b40, 0x16b43, 0x16b63, 0x16b77, 0x16b7d, 0x16b8f, 0x16e40, 0x16e7f,
0x16f00, 0x16f4a, 0x16f50, 0x16f50, 0x16f93, 0x16f9f, 0x16fe0, 0x16fe1,
0x16fe3, 0x16fe3, 0x17000, 0x187f7, 0x18800, 0x18cd5, 0x18d00, 0x18d08,
0x1aff0, 0x1aff3, 0x1aff5, 0x1affb, 0x1affd, 0x1affe, 0x1b000, 0x1b122,
0x1b150, 0x1b152, 0x1b164, 0x1b167, 0x1b170, 0x1b2fb, 0x1bc00, 0x1bc6a,
0x1bc70, 0x1bc7c, 0x1bc80, 0x1bc88, 0x1bc90, 0x1bc99, 0x1d400, 0x1d454,
0x1d456, 0x1d49c, 0x1d49e, 0x1d49f, 0x1d4a2, 0x1d4a2, 0x1d4a5, 0x1d4a6,
0x1d4a9, 0x1d4ac, 0x1d4ae, 0x1d4b9, 0x1d4bb, 0x1d4bb, 0x1d4bd, 0x1d4c3,
0x1d4c5, 0x1d505, 0x1d507, 0x1d50a, 0x1d50d, 0x1d514, 0x1d516, 0x1d51c,
0x1d51e, 0x1d539, 0x1d53b, 0x1d53e, 0x1d540, 0x1d544, 0x1d546, 0x1d546,
0x1d54a, 0x1d550, 0x1d552, 0x1d6a5, 0x1d6a8, 0x1d6c0, 0x1d6c2, 0x1d6da,
0x1d6dc, 0x1d6fa, 0x1d6fc, 0x1d714, 0x1d716, 0x1d734, 0x1d736, 0x1d74e,
0x1d750, 0x1d76e, 0x1d770, 0x1d788, 0x1d78a, 0x1d7a8, 0x1d7aa, 0x1d7c2,
0x1d7c4, 0x1d7cb, 0x1df00, 0x1df1e, 0x1e100, 0x1e12c, 0x1e137, 0x1e13d,
0x1e14e, 0x1e14e, 0x1e290, 0x1e2ad, 0x1e2c0, 0x1e2eb, 0x1e2ff, 0x1e2ff,
0x1e7e0, 0x1e7e6, 0x1e7e8, 0x1e7eb, 0x1e7ed, 0x1e7ee, 0x1e7f0, 0x1e7fe,
0x1e800, 0x1e8c4, 0x1e900, 0x1e943, 0x1e94b, 0x1e94b, 0x1ecb0, 0x1ecb0,
0x1ee00, 0x1ee03, 0x1ee05, 0x1ee1f, 0x1ee21, 0x1ee22, 0x1ee24, 0x1ee24,
0x1ee27, 0x1ee27, 0x1ee29, 0x1ee32, 0x1ee34, 0x1ee37, 0x1ee39, 0x1ee39,
0x1ee3b, 0x1ee3b, 0x1ee42, 0x1ee42, 0x1ee47, 0x1ee47, 0x1ee49, 0x1ee49,
0x1ee4b, 0x1ee4b, 0x1ee4d, 0x1ee4f, 0x1ee51, 0x1ee52, 0x1ee54, 0x1ee54,
0x1ee57, 0x1ee57, 0x1ee59, 0x1ee59, 0x1ee5b, 0x1ee5b, 0x1ee5d, 0x1ee5d,
0x1ee5f, 0x1ee5f, 0x1ee61, 0x1ee62, 0x1ee64, 0x1ee64, 0x1ee67, 0x1ee6a,
0x1ee6c, 0x1ee72, 0x1ee74, 0x1ee77, 0x1ee79, 0x1ee7c, 0x1ee7e, 0x1ee7e,
0x1ee80, 0x1ee89, 0x1ee8b, 0x1ee9b, 0x1eea1, 0x1eea3, 0x1eea5, 0x1eea9,
0x1eeab, 0x1eebb, 0x20000, 0x2a6df, 0x2a700, 0x2b738, 0x2b740, 0x2b81d,
0x2b820, 0x2cea1, 0x2ceb0, 0x2ebe0, 0x2f800, 0x2fa1d, 0x30000, 0x3134a};
}
private static final int[] NFA_MOVES_TEMPLATE_TEXT_67 = NFA_MOVES_TEMPLATE_TEXT_67_init();
private static int[] NFA_MOVES_TEMPLATE_TEXT_67_init() {
return new int[] {0x0, 0x8, 0xe, 0x1b, '$', '$', '0', '9', 'A', 'Z', '_',
'_', 'a', 'z', 0x7f, 0x9f, 0xa2, 0xa5, 0xaa, 0xaa, 0xad, 0xad, 0xb5, 0xb5,
0xba, 0xba, 0xc0, 0xd6, 0xd8, 0xf6, 0xf8, 0x2c1, 0x2c6, 0x2d1, 0x2e0,
0x2e4, 0x2ec, 0x2ec, 0x2ee, 0x2ee, 0x300, 0x374, 0x376, 0x377, 0x37a,
0x37d, 0x37f, 0x37f, 0x386, 0x386, 0x388, 0x38a, 0x38c, 0x38c, 0x38e,
0x3a1, 0x3a3, 0x3f5, 0x3f7, 0x481, 0x483, 0x487, 0x48a, 0x52f, 0x531,
0x556, 0x559, 0x559, 0x560, 0x588, 0x58f, 0x58f, 0x591, 0x5bd, 0x5bf,
0x5bf, 0x5c1, 0x5c2, 0x5c4, 0x5c5, 0x5c7, 0x5c7, 0x5d0, 0x5ea, 0x5ef,
0x5f2, 0x600, 0x605, 0x60b, 0x60b, 0x610, 0x61a, 0x61c, 0x61c, 0x620,
0x669, 0x66e, 0x6d3, 0x6d5, 0x6dd, 0x6df, 0x6e8, 0x6ea, 0x6fc, 0x6ff,
0x6ff, 0x70f, 0x74a, 0x74d, 0x7b1, 0x7c0, 0x7f5, 0x7fa, 0x7fa, 0x7fd,
0x82d, 0x840, 0x85b, 0x860, 0x86a, 0x870, 0x887, 0x889, 0x88e, 0x890,
0x891, 0x898, 0x963, 0x966, 0x96f, 0x971, 0x983, 0x985, 0x98c, 0x98f,
0x990, 0x993, 0x9a8, 0x9aa, 0x9b0, 0x9b2, 0x9b2, 0x9b6, 0x9b9, 0x9bc,
0x9c4, 0x9c7, 0x9c8, 0x9cb, 0x9ce, 0x9d7, 0x9d7, 0x9dc, 0x9dd, 0x9df,
0x9e3, 0x9e6, 0x9f3, 0x9fb, 0x9fc, 0x9fe, 0x9fe, 0xa01, 0xa03, 0xa05,
0xa0a, 0xa0f, 0xa10, 0xa13, 0xa28, 0xa2a, 0xa30, 0xa32, 0xa33, 0xa35,
0xa36, 0xa38, 0xa39, 0xa3c, 0xa3c, 0xa3e, 0xa42, 0xa47, 0xa48, 0xa4b,
0xa4d, 0xa51, 0xa51, 0xa59, 0xa5c, 0xa5e, 0xa5e, 0xa66, 0xa75, 0xa81,
0xa83, 0xa85, 0xa8d, 0xa8f, 0xa91, 0xa93, 0xaa8, 0xaaa, 0xab0, 0xab2,
0xab3, 0xab5, 0xab9, 0xabc, 0xac5, 0xac7, 0xac9, 0xacb, 0xacd, 0xad0,
0xad0, 0xae0, 0xae3, 0xae6, 0xaef, 0xaf1, 0xaf1, 0xaf9, 0xaff, 0xb01,
0xb03, 0xb05, 0xb0c, 0xb0f, 0xb10, 0xb13, 0xb28, 0xb2a, 0xb30, 0xb32,
0xb33, 0xb35, 0xb39, 0xb3c, 0xb44, 0xb47, 0xb48, 0xb4b, 0xb4d, 0xb55,
0xb57, 0xb5c, 0xb5d, 0xb5f, 0xb63, 0xb66, 0xb6f, 0xb71, 0xb71, 0xb82,
0xb83, 0xb85, 0xb8a, 0xb8e, 0xb90, 0xb92, 0xb95, 0xb99, 0xb9a, 0xb9c,
0xb9c, 0xb9e, 0xb9f, 0xba3, 0xba4, 0xba8, 0xbaa, 0xbae, 0xbb9, 0xbbe,
0xbc2, 0xbc6, 0xbc8, 0xbca, 0xbcd, 0xbd0, 0xbd0, 0xbd7, 0xbd7, 0xbe6,
0xbef, 0xbf9, 0xbf9, 0xc00, 0xc0c, 0xc0e, 0xc10, 0xc12, 0xc28, 0xc2a,
0xc39, 0xc3c, 0xc44, 0xc46, 0xc48, 0xc4a, 0xc4d, 0xc55, 0xc56, 0xc58,
0xc5a, 0xc5d, 0xc5d, 0xc60, 0xc63, 0xc66, 0xc6f, 0xc80, 0xc83, 0xc85,
0xc8c, 0xc8e, 0xc90, 0xc92, 0xca8, 0xcaa, 0xcb3, 0xcb5, 0xcb9, 0xcbc,
0xcc4, 0xcc6, 0xcc8, 0xcca, 0xccd, 0xcd5, 0xcd6, 0xcdd, 0xcde, 0xce0,
0xce3, 0xce6, 0xcef, 0xcf1, 0xcf2, 0xd00, 0xd0c, 0xd0e, 0xd10, 0xd12,
0xd44, 0xd46, 0xd48, 0xd4a, 0xd4e, 0xd54, 0xd57, 0xd5f, 0xd63, 0xd66,
0xd6f, 0xd7a, 0xd7f, 0xd81, 0xd83, 0xd85, 0xd96, 0xd9a, 0xdb1, 0xdb3,
0xdbb, 0xdbd, 0xdbd, 0xdc0, 0xdc6, 0xdca, 0xdca, 0xdcf, 0xdd4, 0xdd6,
0xdd6, 0xdd8, 0xddf, 0xde6, 0xdef, 0xdf2, 0xdf3, 0xe01, 0xe3a, 0xe3f,
0xe4e, 0xe50, 0xe59, 0xe81, 0xe82, 0xe84, 0xe84, 0xe86, 0xe8a, 0xe8c,
0xea3, 0xea5, 0xea5, 0xea7, 0xebd, 0xec0, 0xec4, 0xec6, 0xec6, 0xec8,
0xecd, 0xed0, 0xed9, 0xedc, 0xedf, 0xf00, 0xf00, 0xf18, 0xf19, 0xf20,
0xf29, 0xf35, 0xf35, 0xf37, 0xf37, 0xf39, 0xf39, 0xf3e, 0xf47, 0xf49,
0xf6c, 0xf71, 0xf84, 0xf86, 0xf97, 0xf99, 0xfbc, 0xfc6, 0xfc6, 0x1000,
0x1049, 0x1050, 0x109d, 0x10a0, 0x10c5, 0x10c7, 0x10c7, 0x10cd, 0x10cd,
0x10d0, 0x10fa, 0x10fc, 0x1248, 0x124a, 0x124d, 0x1250, 0x1256, 0x1258,
0x1258, 0x125a, 0x125d, 0x1260, 0x1288, 0x128a, 0x128d, 0x1290, 0x12b0,
0x12b2, 0x12b5, 0x12b8, 0x12be, 0x12c0, 0x12c0, 0x12c2, 0x12c5, 0x12c8,
0x12d6, 0x12d8, 0x1310, 0x1312, 0x1315, 0x1318, 0x135a, 0x135d, 0x135f,
0x1380, 0x138f, 0x13a0, 0x13f5, 0x13f8, 0x13fd, 0x1401, 0x166c, 0x166f,
0x167f, 0x1681, 0x169a, 0x16a0, 0x16ea, 0x16ee, 0x16f8, 0x1700, 0x1715,
0x171f, 0x1734, 0x1740, 0x1753, 0x1760, 0x176c, 0x176e, 0x1770, 0x1772,
0x1773, 0x1780, 0x17d3, 0x17d7, 0x17d7, 0x17db, 0x17dd, 0x17e0, 0x17e9,
0x180b, 0x1819, 0x1820, 0x1878, 0x1880, 0x18aa, 0x18b0, 0x18f5, 0x1900,
0x191e, 0x1920, 0x192b, 0x1930, 0x193b, 0x1946, 0x196d, 0x1970, 0x1974,
0x1980, 0x19ab, 0x19b0, 0x19c9, 0x19d0, 0x19d9, 0x1a00, 0x1a1b, 0x1a20,
0x1a5e, 0x1a60, 0x1a7c, 0x1a7f, 0x1a89, 0x1a90, 0x1a99, 0x1aa7, 0x1aa7,
0x1ab0, 0x1abd, 0x1abf, 0x1ace, 0x1b00, 0x1b4c, 0x1b50, 0x1b59, 0x1b6b,
0x1b73, 0x1b80, 0x1bf3, 0x1c00, 0x1c37, 0x1c40, 0x1c49, 0x1c4d, 0x1c7d,
0x1c80, 0x1c88, 0x1c90, 0x1cba, 0x1cbd, 0x1cbf, 0x1cd0, 0x1cd2, 0x1cd4,
0x1cfa, 0x1d00, 0x1f15, 0x1f18, 0x1f1d, 0x1f20, 0x1f45, 0x1f48, 0x1f4d,
0x1f50, 0x1f57, 0x1f59, 0x1f59, 0x1f5b, 0x1f5b, 0x1f5d, 0x1f5d, 0x1f5f,
0x1f7d, 0x1f80, 0x1fb4, 0x1fb6, 0x1fbc, 0x1fbe, 0x1fbe, 0x1fc2, 0x1fc4,
0x1fc6, 0x1fcc, 0x1fd0, 0x1fd3, 0x1fd6, 0x1fdb, 0x1fe0, 0x1fec, 0x1ff2,
0x1ff4, 0x1ff6, 0x1ffc, 0x200b, 0x200f, 0x202a, 0x202e, 0x203f, 0x2040,
0x2054, 0x2054, 0x2060, 0x2064, 0x2066, 0x206f, 0x2071, 0x2071, 0x207f,
0x207f, 0x2090, 0x209c, 0x20a0, 0x20c0, 0x20d0, 0x20dc, 0x20e1, 0x20e1,
0x20e5, 0x20f0, 0x2102, 0x2102, 0x2107, 0x2107, 0x210a, 0x2113, 0x2115,
0x2115, 0x2119, 0x211d, 0x2124, 0x2124, 0x2126, 0x2126, 0x2128, 0x2128,
0x212a, 0x212d, 0x212f, 0x2139, 0x213c, 0x213f, 0x2145, 0x2149, 0x214e,
0x214e, 0x2160, 0x2188, 0x2c00, 0x2ce4, 0x2ceb, 0x2cf3, 0x2d00, 0x2d25,
0x2d27, 0x2d27, 0x2d2d, 0x2d2d, 0x2d30, 0x2d67, 0x2d6f, 0x2d6f, 0x2d7f,
0x2d96, 0x2da0, 0x2da6, 0x2da8, 0x2dae, 0x2db0, 0x2db6, 0x2db8, 0x2dbe,
0x2dc0, 0x2dc6, 0x2dc8, 0x2dce, 0x2dd0, 0x2dd6, 0x2dd8, 0x2dde, 0x2de0,
0x2dff, 0x2e2f, 0x2e2f, 0x3005, 0x3007, 0x3021, 0x302f, 0x3031, 0x3035,
0x3038, 0x303c, 0x3041, 0x3096, 0x3099, 0x309a, 0x309d, 0x309f, 0x30a1,
0x30fa, 0x30fc, 0x30ff, 0x3105, 0x312f, 0x3131, 0x318e, 0x31a0, 0x31bf,
0x31f0, 0x31ff, 0x3400, 0x4dbf, 0x4e00, 0xa48c, 0xa4d0, 0xa4fd, 0xa500,
0xa60c, 0xa610, 0xa62b, 0xa640, 0xa66f, 0xa674, 0xa67d, 0xa67f, 0xa6f1,
0xa717, 0xa71f, 0xa722, 0xa788, 0xa78b, 0xa7ca, 0xa7d0, 0xa7d1, 0xa7d3,
0xa7d3, 0xa7d5, 0xa7d9, 0xa7f2, 0xa827, 0xa82c, 0xa82c, 0xa838, 0xa838,
0xa840, 0xa873, 0xa880, 0xa8c5, 0xa8d0, 0xa8d9, 0xa8e0, 0xa8f7, 0xa8fb,
0xa8fb, 0xa8fd, 0xa92d, 0xa930, 0xa953, 0xa960, 0xa97c, 0xa980, 0xa9c0,
0xa9cf, 0xa9d9, 0xa9e0, 0xa9fe, 0xaa00, 0xaa36, 0xaa40, 0xaa4d, 0xaa50,
0xaa59, 0xaa60, 0xaa76, 0xaa7a, 0xaac2, 0xaadb, 0xaadd, 0xaae0, 0xaaef,
0xaaf2, 0xaaf6, 0xab01, 0xab06, 0xab09, 0xab0e, 0xab11, 0xab16, 0xab20,
0xab26, 0xab28, 0xab2e, 0xab30, 0xab5a, 0xab5c, 0xab69, 0xab70, 0xabea,
0xabec, 0xabed, 0xabf0, 0xabf9, 0xac00, 0xd7a3, 0xd7b0, 0xd7c6, 0xd7cb,
0xd7fb, 0xf900, 0xfa6d, 0xfa70, 0xfad9, 0xfb00, 0xfb06, 0xfb13, 0xfb17,
0xfb1d, 0xfb28, 0xfb2a, 0xfb36, 0xfb38, 0xfb3c, 0xfb3e, 0xfb3e, 0xfb40,
0xfb41, 0xfb43, 0xfb44, 0xfb46, 0xfbb1, 0xfbd3, 0xfd3d, 0xfd50, 0xfd8f,
0xfd92, 0xfdc7, 0xfdf0, 0xfdfc, 0xfe00, 0xfe0f, 0xfe20, 0xfe2f, 0xfe33,
0xfe34, 0xfe4d, 0xfe4f, 0xfe69, 0xfe69, 0xfe70, 0xfe74, 0xfe76, 0xfefc,
0xfeff, 0xfeff, 0xff04, 0xff04, 0xff10, 0xff19, 0xff21, 0xff3a, 0xff3f,
0xff3f, 0xff41, 0xff5a, 0xff66, 0xffbe, 0xffc2, 0xffc7, 0xffca, 0xffcf,
0xffd2, 0xffd7, 0xffda, 0xffdc, 0xffe0, 0xffe1, 0xffe5, 0xffe6, 0xfff9,
0xfffb, 0x10000, 0x1000b, 0x1000d, 0x10026, 0x10028, 0x1003a, 0x1003c,
0x1003d, 0x1003f, 0x1004d, 0x10050, 0x1005d, 0x10080, 0x100fa, 0x10140,
0x10174, 0x101fd, 0x101fd, 0x10280, 0x1029c, 0x102a0, 0x102d0, 0x102e0,
0x102e0, 0x10300, 0x1031f, 0x1032d, 0x1034a, 0x10350, 0x1037a, 0x10380,
0x1039d, 0x103a0, 0x103c3, 0x103c8, 0x103cf, 0x103d1, 0x103d5, 0x10400,
0x1049d, 0x104a0, 0x104a9, 0x104b0, 0x104d3, 0x104d8, 0x104fb, 0x10500,
0x10527, 0x10530, 0x10563, 0x10570, 0x1057a, 0x1057c, 0x1058a, 0x1058c,
0x10592, 0x10594, 0x10595, 0x10597, 0x105a1, 0x105a3, 0x105b1, 0x105b3,
0x105b9, 0x105bb, 0x105bc, 0x10600, 0x10736, 0x10740, 0x10755, 0x10760,
0x10767, 0x10780, 0x10785, 0x10787, 0x107b0, 0x107b2, 0x107ba, 0x10800,
0x10805, 0x10808, 0x10808, 0x1080a, 0x10835, 0x10837, 0x10838, 0x1083c,
0x1083c, 0x1083f, 0x10855, 0x10860, 0x10876, 0x10880, 0x1089e, 0x108e0,
0x108f2, 0x108f4, 0x108f5, 0x10900, 0x10915, 0x10920, 0x10939, 0x10980,
0x109b7, 0x109be, 0x109bf, 0x10a00, 0x10a03, 0x10a05, 0x10a06, 0x10a0c,
0x10a13, 0x10a15, 0x10a17, 0x10a19, 0x10a35, 0x10a38, 0x10a3a, 0x10a3f,
0x10a3f, 0x10a60, 0x10a7c, 0x10a80, 0x10a9c, 0x10ac0, 0x10ac7, 0x10ac9,
0x10ae6, 0x10b00, 0x10b35, 0x10b40, 0x10b55, 0x10b60, 0x10b72, 0x10b80,
0x10b91, 0x10c00, 0x10c48, 0x10c80, 0x10cb2, 0x10cc0, 0x10cf2, 0x10d00,
0x10d27, 0x10d30, 0x10d39, 0x10e80, 0x10ea9, 0x10eab, 0x10eac, 0x10eb0,
0x10eb1, 0x10f00, 0x10f1c, 0x10f27, 0x10f27, 0x10f30, 0x10f50, 0x10f70,
0x10f85, 0x10fb0, 0x10fc4, 0x10fe0, 0x10ff6, 0x11000, 0x11046, 0x11066,
0x11075, 0x1107f, 0x110ba, 0x110bd, 0x110bd, 0x110c2, 0x110c2, 0x110cd,
0x110cd, 0x110d0, 0x110e8, 0x110f0, 0x110f9, 0x11100, 0x11134, 0x11136,
0x1113f, 0x11144, 0x11147, 0x11150, 0x11173, 0x11176, 0x11176, 0x11180,
0x111c4, 0x111c9, 0x111cc, 0x111ce, 0x111da, 0x111dc, 0x111dc, 0x11200,
0x11211, 0x11213, 0x11237, 0x1123e, 0x1123e, 0x11280, 0x11286, 0x11288,
0x11288, 0x1128a, 0x1128d, 0x1128f, 0x1129d, 0x1129f, 0x112a8, 0x112b0,
0x112ea, 0x112f0, 0x112f9, 0x11300, 0x11303, 0x11305, 0x1130c, 0x1130f,
0x11310, 0x11313, 0x11328, 0x1132a, 0x11330, 0x11332, 0x11333, 0x11335,
0x11339, 0x1133b, 0x11344, 0x11347, 0x11348, 0x1134b, 0x1134d, 0x11350,
0x11350, 0x11357, 0x11357, 0x1135d, 0x11363, 0x11366, 0x1136c, 0x11370,
0x11374, 0x11400, 0x1144a, 0x11450, 0x11459, 0x1145e, 0x11461, 0x11480,
0x114c5, 0x114c7, 0x114c7, 0x114d0, 0x114d9, 0x11580, 0x115b5, 0x115b8,
0x115c0, 0x115d8, 0x115dd, 0x11600, 0x11640, 0x11644, 0x11644, 0x11650,
0x11659, 0x11680, 0x116b8, 0x116c0, 0x116c9, 0x11700, 0x1171a, 0x1171d,
0x1172b, 0x11730, 0x11739, 0x11740, 0x11746, 0x11800, 0x1183a, 0x118a0,
0x118e9, 0x118ff, 0x11906, 0x11909, 0x11909, 0x1190c, 0x11913, 0x11915,
0x11916, 0x11918, 0x11935, 0x11937, 0x11938, 0x1193b, 0x11943, 0x11950,
0x11959, 0x119a0, 0x119a7, 0x119aa, 0x119d7, 0x119da, 0x119e1, 0x119e3,
0x119e4, 0x11a00, 0x11a3e, 0x11a47, 0x11a47, 0x11a50, 0x11a99, 0x11a9d,
0x11a9d, 0x11ab0, 0x11af8, 0x11c00, 0x11c08, 0x11c0a, 0x11c36, 0x11c38,
0x11c40, 0x11c50, 0x11c59, 0x11c72, 0x11c8f, 0x11c92, 0x11ca7, 0x11ca9,
0x11cb6, 0x11d00, 0x11d06, 0x11d08, 0x11d09, 0x11d0b, 0x11d36, 0x11d3a,
0x11d3a, 0x11d3c, 0x11d3d, 0x11d3f, 0x11d47, 0x11d50, 0x11d59, 0x11d60,
0x11d65, 0x11d67, 0x11d68, 0x11d6a, 0x11d8e, 0x11d90, 0x11d91, 0x11d93,
0x11d98, 0x11da0, 0x11da9, 0x11ee0, 0x11ef6, 0x11fb0, 0x11fb0, 0x11fdd,
0x11fe0, 0x12000, 0x12399, 0x12400, 0x1246e, 0x12480, 0x12543, 0x12f90,
0x12ff0, 0x13000, 0x1342e, 0x13430, 0x13438, 0x14400, 0x14646, 0x16800,
0x16a38, 0x16a40, 0x16a5e, 0x16a60, 0x16a69, 0x16a70, 0x16abe, 0x16ac0,
0x16ac9, 0x16ad0, 0x16aed, 0x16af0, 0x16af4, 0x16b00, 0x16b36, 0x16b40,
0x16b43, 0x16b50, 0x16b59, 0x16b63, 0x16b77, 0x16b7d, 0x16b8f, 0x16e40,
0x16e7f, 0x16f00, 0x16f4a, 0x16f4f, 0x16f87, 0x16f8f, 0x16f9f, 0x16fe0,
0x16fe1, 0x16fe3, 0x16fe4, 0x16ff0, 0x16ff1, 0x17000, 0x187f7, 0x18800,
0x18cd5, 0x18d00, 0x18d08, 0x1aff0, 0x1aff3, 0x1aff5, 0x1affb, 0x1affd,
0x1affe, 0x1b000, 0x1b122, 0x1b150, 0x1b152, 0x1b164, 0x1b167, 0x1b170,
0x1b2fb, 0x1bc00, 0x1bc6a, 0x1bc70, 0x1bc7c, 0x1bc80, 0x1bc88, 0x1bc90,
0x1bc99, 0x1bc9d, 0x1bc9e, 0x1bca0, 0x1bca3, 0x1cf00, 0x1cf2d, 0x1cf30,
0x1cf46, 0x1d165, 0x1d169, 0x1d16d, 0x1d182, 0x1d185, 0x1d18b, 0x1d1aa,
0x1d1ad, 0x1d242, 0x1d244, 0x1d400, 0x1d454, 0x1d456, 0x1d49c, 0x1d49e,
0x1d49f, 0x1d4a2, 0x1d4a2, 0x1d4a5, 0x1d4a6, 0x1d4a9, 0x1d4ac, 0x1d4ae,
0x1d4b9, 0x1d4bb, 0x1d4bb, 0x1d4bd, 0x1d4c3, 0x1d4c5, 0x1d505, 0x1d507,
0x1d50a, 0x1d50d, 0x1d514, 0x1d516, 0x1d51c, 0x1d51e, 0x1d539, 0x1d53b,
0x1d53e, 0x1d540, 0x1d544, 0x1d546, 0x1d546, 0x1d54a, 0x1d550, 0x1d552,
0x1d6a5, 0x1d6a8, 0x1d6c0, 0x1d6c2, 0x1d6da, 0x1d6dc, 0x1d6fa, 0x1d6fc,
0x1d714, 0x1d716, 0x1d734, 0x1d736, 0x1d74e, 0x1d750, 0x1d76e, 0x1d770,
0x1d788, 0x1d78a, 0x1d7a8, 0x1d7aa, 0x1d7c2, 0x1d7c4, 0x1d7cb, 0x1d7ce,
0x1d7ff, 0x1da00, 0x1da36, 0x1da3b, 0x1da6c, 0x1da75, 0x1da75, 0x1da84,
0x1da84, 0x1da9b, 0x1da9f, 0x1daa1, 0x1daaf, 0x1df00, 0x1df1e, 0x1e000,
0x1e006, 0x1e008, 0x1e018, 0x1e01b, 0x1e021, 0x1e023, 0x1e024, 0x1e026,
0x1e02a, 0x1e100, 0x1e12c, 0x1e130, 0x1e13d, 0x1e140, 0x1e149, 0x1e14e,
0x1e14e, 0x1e290, 0x1e2ae, 0x1e2c0, 0x1e2f9, 0x1e2ff, 0x1e2ff, 0x1e7e0,
0x1e7e6, 0x1e7e8, 0x1e7eb, 0x1e7ed, 0x1e7ee, 0x1e7f0, 0x1e7fe, 0x1e800,
0x1e8c4, 0x1e8d0, 0x1e8d6, 0x1e900, 0x1e94b, 0x1e950, 0x1e959, 0x1ecb0,
0x1ecb0, 0x1ee00, 0x1ee03, 0x1ee05, 0x1ee1f, 0x1ee21, 0x1ee22, 0x1ee24,
0x1ee24, 0x1ee27, 0x1ee27, 0x1ee29, 0x1ee32, 0x1ee34, 0x1ee37, 0x1ee39,
0x1ee39, 0x1ee3b, 0x1ee3b, 0x1ee42, 0x1ee42, 0x1ee47, 0x1ee47, 0x1ee49,
0x1ee49, 0x1ee4b, 0x1ee4b, 0x1ee4d, 0x1ee4f, 0x1ee51, 0x1ee52, 0x1ee54,
0x1ee54, 0x1ee57, 0x1ee57, 0x1ee59, 0x1ee59, 0x1ee5b, 0x1ee5b, 0x1ee5d,
0x1ee5d, 0x1ee5f, 0x1ee5f, 0x1ee61, 0x1ee62, 0x1ee64, 0x1ee64, 0x1ee67,
0x1ee6a, 0x1ee6c, 0x1ee72, 0x1ee74, 0x1ee77, 0x1ee79, 0x1ee7c, 0x1ee7e,
0x1ee7e, 0x1ee80, 0x1ee89, 0x1ee8b, 0x1ee9b, 0x1eea1, 0x1eea3, 0x1eea5,
0x1eea9, 0x1eeab, 0x1eebb, 0x1fbf0, 0x1fbf9, 0x20000, 0x2a6df, 0x2a700,
0x2b738, 0x2b740, 0x2b81d, 0x2b820, 0x2cea1, 0x2ceb0, 0x2ebe0, 0x2f800,
0x2fa1d, 0x30000, 0x3134a, 0xe0001, 0xe0001, 0xe0020, 0xe007f, 0xe0100,
0xe01ef};
}
private static void NFA_FUNCTIONS_init() {
NfaFunction[] functions = new NfaFunction[] {TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex0,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex1, TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex2,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex3, TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex4,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex5, TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex6,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex7, TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex8,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex9, TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex10,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex11, TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex12,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex13, TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex14,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex15, TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex16,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex17, TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex18,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex19, TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex20,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex21, TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex22,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex23, TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex24,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex25, TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex26,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex27, TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex28,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex29, TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex30,
TEMPLATE_TEXT::getNfaNameTEMPLATE_TEXTIndex31};
functionTableMap.put(LexicalState.TEMPLATE_TEXT, functions);
}
}
/**
* Holder class for NFA code related to FTL_EXPRESSION lexical state
*/
private static class FTL_EXPRESSION {
private static TokenType getNfaNameFTL_EXPRESSIONIndex0(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (ch == '\t') {
if (validTypes == null || validTypes.contains(CLOSE_EMPTY_TAG)) {
nextStates.set(53);
}
} else if (ch == '\n') {
if (validTypes == null || validTypes.contains(CLOSE_EMPTY_TAG)) {
nextStates.set(53);
}
} else if (ch == '\r') {
if (validTypes == null || validTypes.contains(CLOSE_EMPTY_TAG)) {
nextStates.set(53);
}
} else if (ch == ' ') {
if (validTypes == null || validTypes.contains(CLOSE_EMPTY_TAG)) {
nextStates.set(53);
}
} else if (ch == '!') {
if (validTypes == null || validTypes.contains(NOT_EQUALS)) {
nextStates.set(23);
}
} else if (ch == '"') {
if (validTypes == null || validTypes.contains(STRING_LITERAL)) {
nextStates.set(16);
}
} else if (ch == '&') {
if (validTypes == null || validTypes.contains(AND2)) {
nextStates.set(14);
}
} else if (ch == '\'') {
if (validTypes == null || validTypes.contains(STRING_LITERAL)) {
nextStates.set(20);
}
} else if (ch == '.') {
if (validTypes == null || validTypes.contains(ELLIPSIS)) {
nextStates.set(37);
}
if (validTypes == null || validTypes.contains(DOT_DOT)) {
nextStates.set(10);
}
if (validTypes == null || validTypes.contains(DOT_DOT_EXCLUSIVE)) {
nextStates.set(52);
}
if (validTypes == null || validTypes.contains(DOT_DOT_LENGTH)) {
nextStates.set(69);
}
} else if (ch == '/') {
if (validTypes == null || validTypes.contains(CLOSE_EMPTY_TAG)) {
nextStates.set(19);
}
} else if (ch >= '0' && ch <= '9') {
if (validTypes == null || validTypes.contains(DECIMAL)) {
nextStates.set(45);
}
} else if (ch == ':') {
if (validTypes == null || validTypes.contains(DOUBLE_COLON)) {
nextStates.set(9);
}
} else if (ch == '<') {
if (validTypes == null || validTypes.contains(_TOKEN_2)) {
nextStates.set(49);
}
if (validTypes == null || validTypes.contains(_TOKEN_1)) {
nextStates.set(67);
}
if (validTypes == null || validTypes.contains(LTE)) {
nextStates.set(33);
}
} else if (ch == '=') {
if (validTypes == null || validTypes.contains(DOUBLE_EQUALS)) {
nextStates.set(17);
}
} else if (ch == '>') {
if (validTypes == null || validTypes.contains(GTE)) {
nextStates.set(24);
}
} else if (ch == '?') {
if (validTypes == null || validTypes.contains(EXISTS_OPERATOR)) {
nextStates.set(2);
}
} else if (ch == '[') {
if (validTypes == null || validTypes.contains(_TOKEN_3)) {
nextStates.set(58);
}
} else if (ch == 'a') {
if (validTypes == null || validTypes.contains(AS)) {
nextStates.set(13);
}
if (validTypes == null || validTypes.contains(ASCENDING)) {
nextStates.set(55);
}
} else if (ch == 'd') {
if (validTypes == null || validTypes.contains(DESCENDING)) {
nextStates.set(43);
}
} else if (ch == 'f') {
if (validTypes == null || validTypes.contains(FALSE)) {
nextStates.set(38);
}
if (validTypes == null || validTypes.contains(FILTER)) {
nextStates.set(70);
}
} else if (ch == 'i') {
if (validTypes == null || validTypes.contains(IN)) {
nextStates.set(28);
}
} else if (ch == 'l') {
if (validTypes == null || validTypes.contains(LIMIT)) {
nextStates.set(60);
}
} else if (ch == 'n') {
if (validTypes == null || validTypes.contains(NULL)) {
nextStates.set(35);
}
} else if (ch == 's') {
if (validTypes == null || validTypes.contains(SORTED)) {
nextStates.set(63);
}
} else if (ch == 't') {
if (validTypes == null || validTypes.contains(TRUE)) {
nextStates.set(56);
}
} else if (ch == 'u') {
if (validTypes == null || validTypes.contains(USING)) {
nextStates.set(46);
}
} else if (ch == 'w') {
if (validTypes == null || validTypes.contains(WITH)) {
nextStates.set(41);
}
} else if (ch == '|') {
if (validTypes == null || validTypes.contains(OR2)) {
nextStates.set(3);
}
}
if (((ch == '$') || ((ch >= 'A' && ch <= 'Z') || ((ch == '_') || (ch >= 'a' && ch <= 'z')))) || (ch >= 128 && checkIntervals(NFA_MOVES_FTL_EXPRESSION_131, ch))) {
if (validTypes == null || validTypes.contains(IDENTIFIER)) {
nextStates.set(26);
type = IDENTIFIER;
}
} else if (ch == '@') {
if (validTypes == null || validTypes.contains(IDENTIFIER)) {
nextStates.set(26);
type = IDENTIFIER;
}
} else if (ch >= '0' && ch <= '9') {
if (validTypes == null || validTypes.contains(INTEGER)) {
nextStates.set(22);
type = INTEGER;
}
} else if (ch == 0x2265) {
if (validTypes == null || validTypes.contains(GTE)) {
type = GTE;
}
} else if (ch == 0x2264) {
if (validTypes == null || validTypes.contains(LTE)) {
type = LTE;
}
} else if (ch == 0x2203) {
if (validTypes == null || validTypes.contains(EXISTS_OPERATOR)) {
type = EXISTS_OPERATOR;
}
} else if (ch == 0x2260) {
if (validTypes == null || validTypes.contains(NOT_EQUALS)) {
type = NOT_EQUALS;
}
} else if (ch == '^') {
if (validTypes == null || validTypes.contains(XOR)) {
type = XOR;
}
} else if (ch == 0x22bb) {
if (validTypes == null || validTypes.contains(XOR)) {
type = XOR;
}
} else if (ch == '&') {
if (validTypes == null || validTypes.contains(AND)) {
type = AND;
}
} else if (ch == 0x2227) {
if (validTypes == null || validTypes.contains(AND)) {
type = AND;
}
} else if (ch == '|') {
if (validTypes == null || validTypes.contains(OR)) {
type = OR;
}
} else if (ch == 0x2228) {
if (validTypes == null || validTypes.contains(OR)) {
type = OR;
}
} else if (ch == '/') {
if (validTypes == null || validTypes.contains(DIVIDE)) {
type = DIVIDE;
}
} else if (ch == 0xf7) {
if (validTypes == null || validTypes.contains(DIVIDE)) {
type = DIVIDE;
}
} else if (ch == '*') {
if (validTypes == null || validTypes.contains(TIMES)) {
type = TIMES;
}
} else if (ch == 0xd7) {
if (validTypes == null || validTypes.contains(TIMES)) {
type = TIMES;
}
} else if (ch == '\t') {
if (validTypes == null || validTypes.contains(EXP_WHITE_SPACE)) {
nextStates.set(8);
type = EXP_WHITE_SPACE;
}
} else if (ch == '\n') {
if (validTypes == null || validTypes.contains(EXP_WHITE_SPACE)) {
nextStates.set(8);
type = EXP_WHITE_SPACE;
}
} else if (ch == '\r') {
if (validTypes == null || validTypes.contains(EXP_WHITE_SPACE)) {
nextStates.set(8);
type = EXP_WHITE_SPACE;
}
} else if (ch == ' ') {
if (validTypes == null || validTypes.contains(EXP_WHITE_SPACE)) {
nextStates.set(8);
type = EXP_WHITE_SPACE;
}
} else if (ch == '?') {
if (validTypes == null || validTypes.contains(BUILT_IN)) {
type = BUILT_IN;
}
} else if (ch == 0xac) {
if (validTypes == null || validTypes.contains(NOT)) {
type = NOT;
}
} else if (ch == '!') {
if (validTypes == null || validTypes.contains(EXCLAM)) {
type = EXCLAM;
}
} else if (ch == ';') {
if (validTypes == null || validTypes.contains(SEMICOLON)) {
type = SEMICOLON;
}
} else if (ch == ':') {
if (validTypes == null || validTypes.contains(COLON)) {
type = COLON;
}
} else if (ch == ',') {
if (validTypes == null || validTypes.contains(COMMA)) {
type = COMMA;
}
} else if (ch == '>') {
if (validTypes == null || validTypes.contains(GT)) {
type = GT;
}
} else if (ch == '<') {
if (validTypes == null || validTypes.contains(LT)) {
type = LT;
}
} else if (ch == '%') {
if (validTypes == null || validTypes.contains(PERCENT)) {
type = PERCENT;
}
} else if (ch == '-') {
if (validTypes == null || validTypes.contains(MINUS)) {
type = MINUS;
}
} else if (ch == '+') {
if (validTypes == null || validTypes.contains(PLUS)) {
type = PLUS;
}
} else if (ch == '.') {
if (validTypes == null || validTypes.contains(DOT)) {
type = DOT;
}
} else if (ch == '=') {
if (validTypes == null || validTypes.contains(EQUALS)) {
type = EQUALS;
}
} else if (ch == '}') {
if (validTypes == null || validTypes.contains(CLOSE_BRACE)) {
type = CLOSE_BRACE;
}
} else if (ch == '{') {
if (validTypes == null || validTypes.contains(OPEN_BRACE)) {
type = OPEN_BRACE;
}
} else if (ch == ']') {
if (validTypes == null || validTypes.contains(CLOSE_BRACKET)) {
type = CLOSE_BRACKET;
}
} else if (ch == '[') {
if (validTypes == null || validTypes.contains(OPEN_BRACKET)) {
type = OPEN_BRACKET;
}
} else if (ch == ')') {
if (validTypes == null || validTypes.contains(CLOSE_PAREN)) {
type = CLOSE_PAREN;
}
} else if (ch == '(') {
if (validTypes == null || validTypes.contains(OPEN_PAREN)) {
type = OPEN_PAREN;
}
}
return type;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex1(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'l') {
return NULL;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex2(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '?') {
return EXISTS_OPERATOR;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex3(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '|') {
return OR2;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex4(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '.') {
return ELLIPSIS;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex5(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'e') {
return FALSE;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex6(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'h') {
return WITH;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex7(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'c') {
return DESCENDING;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex8(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (ch == '\t') {
nextStates.set(8);
type = EXP_WHITE_SPACE;
} else if (ch == '\n') {
nextStates.set(8);
type = EXP_WHITE_SPACE;
} else if (ch == '\r') {
nextStates.set(8);
type = EXP_WHITE_SPACE;
} else if (ch == ' ') {
nextStates.set(8);
type = EXP_WHITE_SPACE;
}
return type;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex9(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == ':') {
return DOUBLE_COLON;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex10(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '.') {
return DOT_DOT;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex11(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch >= '0' && ch <= '9') {
nextStates.set(11);
return DECIMAL;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex12(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'g') {
return USING;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex13(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 's') {
return AS;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex14(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '&') {
return AND2;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex15(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '-') {
return _TOKEN_2;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex16(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if ((ch >= 0x0 && ch <= '!') || ((ch >= '#' && ch <= '[') || (ch >= ']'))) {
nextStates.set(16);
} else if (ch == '\\') {
nextStates.set(51);
} else if (ch == '"') {
type = STRING_LITERAL;
}
return type;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex17(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '=') {
return DOUBLE_EQUALS;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex18(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '<') {
return DOT_DOT_EXCLUSIVE;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex19(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (ch == '>') {
type = CLOSE_EMPTY_TAG;
} else if (ch == ']') {
type = CLOSE_EMPTY_TAG;
}
return type;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex20(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if ((ch >= 0x0 && ch <= '&') || ((ch >= '(' && ch <= '[') || (ch >= ']'))) {
nextStates.set(20);
} else if (ch == '\\') {
nextStates.set(54);
} else if (ch == '\'') {
type = STRING_LITERAL;
}
return type;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex21(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'c') {
return ASCENDING;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex22(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch >= '0' && ch <= '9') {
nextStates.set(22);
return INTEGER;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex23(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '=') {
return NOT_EQUALS;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex24(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '=') {
return GTE;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex25(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'e') {
return TRUE;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex26(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (((ch >= 0x0 && ch <= 0x8) || ((ch >= 0xe && ch <= 0x1b) || ((ch == '$') || ((ch >= '0' && ch <= '9') || ((ch >= 'A' && ch <= 'Z') || ((ch == '_') || ((ch >= 'a' && ch <= 'z') || (ch >= 0x7f && ch <= 0x9f)))))))) || (ch >= 128 && checkIntervals(NFA_MOVES_FTL_EXPRESSION_133, ch))) {
nextStates.set(26);
type = IDENTIFIER;
} else if (ch == '@') {
nextStates.set(26);
type = IDENTIFIER;
}
return type;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex27(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '-') {
return _TOKEN_3;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex28(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'n') {
return IN;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex29(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 't') {
return LIMIT;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex30(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'd') {
return SORTED;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex31(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '-') {
return _TOKEN_1;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex32(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '*') {
return DOT_DOT_LENGTH;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex33(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '=') {
return LTE;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex34(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'r') {
return FILTER;
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex35(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'u') {
nextStates.set(36);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex36(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'l') {
nextStates.set(1);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex37(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '.') {
nextStates.set(4);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex38(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'a') {
nextStates.set(39);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex39(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'l') {
nextStates.set(40);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex40(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 's') {
nextStates.set(5);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex41(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'i') {
nextStates.set(42);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex42(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 't') {
nextStates.set(6);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex43(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'e') {
nextStates.set(44);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex44(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 's') {
nextStates.set(7);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex45(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '.') {
nextStates.set(11);
} else if (ch >= '0' && ch <= '9') {
nextStates.set(45);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex46(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 's') {
nextStates.set(47);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex47(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'i') {
nextStates.set(48);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex48(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'n') {
nextStates.set(12);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex49(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '!') {
nextStates.set(50);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex50(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '-') {
nextStates.set(15);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex51(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch >= 0x0) {
nextStates.set(16);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex52(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '.') {
nextStates.set(18);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex53(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '\t') {
nextStates.set(53);
} else if (ch == '\n') {
nextStates.set(53);
} else if (ch == '\r') {
nextStates.set(53);
} else if (ch == ' ') {
nextStates.set(53);
} else if (ch == '/') {
nextStates.set(19);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex54(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch >= 0x0) {
nextStates.set(20);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex55(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 's') {
nextStates.set(21);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex56(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'r') {
nextStates.set(57);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex57(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'u') {
nextStates.set(25);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex58(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '#') {
nextStates.set(59);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex59(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '-') {
nextStates.set(27);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex60(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'i') {
nextStates.set(61);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex61(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'm') {
nextStates.set(62);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex62(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'i') {
nextStates.set(29);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex63(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'o') {
nextStates.set(64);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex64(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'r') {
nextStates.set(65);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex65(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 't') {
nextStates.set(66);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex66(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'e') {
nextStates.set(30);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex67(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '#') {
nextStates.set(68);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex68(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '-') {
nextStates.set(31);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex69(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '.') {
nextStates.set(32);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex70(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'i') {
nextStates.set(71);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex71(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'l') {
nextStates.set(72);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex72(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 't') {
nextStates.set(73);
}
return null;
}
private static TokenType getNfaNameFTL_EXPRESSIONIndex73(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'e') {
nextStates.set(34);
}
return null;
}
private static final int[] NFA_MOVES_FTL_EXPRESSION_131 = NFA_MOVES_FTL_EXPRESSION_131_init();
private static int[] NFA_MOVES_FTL_EXPRESSION_131_init() {
return new int[] {'$', '$', 'A', 'Z', '_', '_', 'a', 'z', 0xa2, 0xa5,
0xaa, 0xaa, 0xb5, 0xb5, 0xba, 0xba, 0xc0, 0xd6, 0xd8, 0xf6, 0xf8, 0x2c1,
0x2c6, 0x2d1, 0x2e0, 0x2e4, 0x2ec, 0x2ec, 0x2ee, 0x2ee, 0x370, 0x374,
0x376, 0x377, 0x37a, 0x37d, 0x37f, 0x37f, 0x386, 0x386, 0x388, 0x38a,
0x38c, 0x38c, 0x38e, 0x3a1, 0x3a3, 0x3f5, 0x3f7, 0x481, 0x48a, 0x52f,
0x531, 0x556, 0x559, 0x559, 0x560, 0x588, 0x58f, 0x58f, 0x5d0, 0x5ea,
0x5ef, 0x5f2, 0x60b, 0x60b, 0x620, 0x64a, 0x66e, 0x66f, 0x671, 0x6d3,
0x6d5, 0x6d5, 0x6e5, 0x6e6, 0x6ee, 0x6ef, 0x6fa, 0x6fc, 0x6ff, 0x6ff,
0x710, 0x710, 0x712, 0x72f, 0x74d, 0x7a5, 0x7b1, 0x7b1, 0x7ca, 0x7ea,
0x7f4, 0x7f5, 0x7fa, 0x7fa, 0x7fe, 0x815, 0x81a, 0x81a, 0x824, 0x824,
0x828, 0x828, 0x840, 0x858, 0x860, 0x86a, 0x870, 0x887, 0x889, 0x88e,
0x8a0, 0x8c9, 0x904, 0x939, 0x93d, 0x93d, 0x950, 0x950, 0x958, 0x961,
0x971, 0x980, 0x985, 0x98c, 0x98f, 0x990, 0x993, 0x9a8, 0x9aa, 0x9b0,
0x9b2, 0x9b2, 0x9b6, 0x9b9, 0x9bd, 0x9bd, 0x9ce, 0x9ce, 0x9dc, 0x9dd,
0x9df, 0x9e1, 0x9f0, 0x9f3, 0x9fb, 0x9fc, 0xa05, 0xa0a, 0xa0f, 0xa10,
0xa13, 0xa28, 0xa2a, 0xa30, 0xa32, 0xa33, 0xa35, 0xa36, 0xa38, 0xa39,
0xa59, 0xa5c, 0xa5e, 0xa5e, 0xa72, 0xa74, 0xa85, 0xa8d, 0xa8f, 0xa91,
0xa93, 0xaa8, 0xaaa, 0xab0, 0xab2, 0xab3, 0xab5, 0xab9, 0xabd, 0xabd,
0xad0, 0xad0, 0xae0, 0xae1, 0xaf1, 0xaf1, 0xaf9, 0xaf9, 0xb05, 0xb0c,
0xb0f, 0xb10, 0xb13, 0xb28, 0xb2a, 0xb30, 0xb32, 0xb33, 0xb35, 0xb39,
0xb3d, 0xb3d, 0xb5c, 0xb5d, 0xb5f, 0xb61, 0xb71, 0xb71, 0xb83, 0xb83,
0xb85, 0xb8a, 0xb8e, 0xb90, 0xb92, 0xb95, 0xb99, 0xb9a, 0xb9c, 0xb9c,
0xb9e, 0xb9f, 0xba3, 0xba4, 0xba8, 0xbaa, 0xbae, 0xbb9, 0xbd0, 0xbd0,
0xbf9, 0xbf9, 0xc05, 0xc0c, 0xc0e, 0xc10, 0xc12, 0xc28, 0xc2a, 0xc39,
0xc3d, 0xc3d, 0xc58, 0xc5a, 0xc5d, 0xc5d, 0xc60, 0xc61, 0xc80, 0xc80,
0xc85, 0xc8c, 0xc8e, 0xc90, 0xc92, 0xca8, 0xcaa, 0xcb3, 0xcb5, 0xcb9,
0xcbd, 0xcbd, 0xcdd, 0xcde, 0xce0, 0xce1, 0xcf1, 0xcf2, 0xd04, 0xd0c,
0xd0e, 0xd10, 0xd12, 0xd3a, 0xd3d, 0xd3d, 0xd4e, 0xd4e, 0xd54, 0xd56,
0xd5f, 0xd61, 0xd7a, 0xd7f, 0xd85, 0xd96, 0xd9a, 0xdb1, 0xdb3, 0xdbb,
0xdbd, 0xdbd, 0xdc0, 0xdc6, 0xe01, 0xe30, 0xe32, 0xe33, 0xe3f, 0xe46,
0xe81, 0xe82, 0xe84, 0xe84, 0xe86, 0xe8a, 0xe8c, 0xea3, 0xea5, 0xea5,
0xea7, 0xeb0, 0xeb2, 0xeb3, 0xebd, 0xebd, 0xec0, 0xec4, 0xec6, 0xec6,
0xedc, 0xedf, 0xf00, 0xf00, 0xf40, 0xf47, 0xf49, 0xf6c, 0xf88, 0xf8c,
0x1000, 0x102a, 0x103f, 0x103f, 0x1050, 0x1055, 0x105a, 0x105d, 0x1061,
0x1061, 0x1065, 0x1066, 0x106e, 0x1070, 0x1075, 0x1081, 0x108e, 0x108e,
0x10a0, 0x10c5, 0x10c7, 0x10c7, 0x10cd, 0x10cd, 0x10d0, 0x10fa, 0x10fc,
0x1248, 0x124a, 0x124d, 0x1250, 0x1256, 0x1258, 0x1258, 0x125a, 0x125d,
0x1260, 0x1288, 0x128a, 0x128d, 0x1290, 0x12b0, 0x12b2, 0x12b5, 0x12b8,
0x12be, 0x12c0, 0x12c0, 0x12c2, 0x12c5, 0x12c8, 0x12d6, 0x12d8, 0x1310,
0x1312, 0x1315, 0x1318, 0x135a, 0x1380, 0x138f, 0x13a0, 0x13f5, 0x13f8,
0x13fd, 0x1401, 0x166c, 0x166f, 0x167f, 0x1681, 0x169a, 0x16a0, 0x16ea,
0x16ee, 0x16f8, 0x1700, 0x1711, 0x171f, 0x1731, 0x1740, 0x1751, 0x1760,
0x176c, 0x176e, 0x1770, 0x1780, 0x17b3, 0x17d7, 0x17d7, 0x17db, 0x17dc,
0x1820, 0x1878, 0x1880, 0x1884, 0x1887, 0x18a8, 0x18aa, 0x18aa, 0x18b0,
0x18f5, 0x1900, 0x191e, 0x1950, 0x196d, 0x1970, 0x1974, 0x1980, 0x19ab,
0x19b0, 0x19c9, 0x1a00, 0x1a16, 0x1a20, 0x1a54, 0x1aa7, 0x1aa7, 0x1b05,
0x1b33, 0x1b45, 0x1b4c, 0x1b83, 0x1ba0, 0x1bae, 0x1baf, 0x1bba, 0x1be5,
0x1c00, 0x1c23, 0x1c4d, 0x1c4f, 0x1c5a, 0x1c7d, 0x1c80, 0x1c88, 0x1c90,
0x1cba, 0x1cbd, 0x1cbf, 0x1ce9, 0x1cec, 0x1cee, 0x1cf3, 0x1cf5, 0x1cf6,
0x1cfa, 0x1cfa, 0x1d00, 0x1dbf, 0x1e00, 0x1f15, 0x1f18, 0x1f1d, 0x1f20,
0x1f45, 0x1f48, 0x1f4d, 0x1f50, 0x1f57, 0x1f59, 0x1f59, 0x1f5b, 0x1f5b,
0x1f5d, 0x1f5d, 0x1f5f, 0x1f7d, 0x1f80, 0x1fb4, 0x1fb6, 0x1fbc, 0x1fbe,
0x1fbe, 0x1fc2, 0x1fc4, 0x1fc6, 0x1fcc, 0x1fd0, 0x1fd3, 0x1fd6, 0x1fdb,
0x1fe0, 0x1fec, 0x1ff2, 0x1ff4, 0x1ff6, 0x1ffc, 0x203f, 0x2040, 0x2054,
0x2054, 0x2071, 0x2071, 0x207f, 0x207f, 0x2090, 0x209c, 0x20a0, 0x20c0,
0x2102, 0x2102, 0x2107, 0x2107, 0x210a, 0x2113, 0x2115, 0x2115, 0x2119,
0x211d, 0x2124, 0x2124, 0x2126, 0x2126, 0x2128, 0x2128, 0x212a, 0x212d,
0x212f, 0x2139, 0x213c, 0x213f, 0x2145, 0x2149, 0x214e, 0x214e, 0x2160,
0x2188, 0x2c00, 0x2ce4, 0x2ceb, 0x2cee, 0x2cf2, 0x2cf3, 0x2d00, 0x2d25,
0x2d27, 0x2d27, 0x2d2d, 0x2d2d, 0x2d30, 0x2d67, 0x2d6f, 0x2d6f, 0x2d80,
0x2d96, 0x2da0, 0x2da6, 0x2da8, 0x2dae, 0x2db0, 0x2db6, 0x2db8, 0x2dbe,
0x2dc0, 0x2dc6, 0x2dc8, 0x2dce, 0x2dd0, 0x2dd6, 0x2dd8, 0x2dde, 0x2e2f,
0x2e2f, 0x3005, 0x3007, 0x3021, 0x3029, 0x3031, 0x3035, 0x3038, 0x303c,
0x3041, 0x3096, 0x309d, 0x309f, 0x30a1, 0x30fa, 0x30fc, 0x30ff, 0x3105,
0x312f, 0x3131, 0x318e, 0x31a0, 0x31bf, 0x31f0, 0x31ff, 0x3400, 0x4dbf,
0x4e00, 0xa48c, 0xa4d0, 0xa4fd, 0xa500, 0xa60c, 0xa610, 0xa61f, 0xa62a,
0xa62b, 0xa640, 0xa66e, 0xa67f, 0xa69d, 0xa6a0, 0xa6ef, 0xa717, 0xa71f,
0xa722, 0xa788, 0xa78b, 0xa7ca, 0xa7d0, 0xa7d1, 0xa7d3, 0xa7d3, 0xa7d5,
0xa7d9, 0xa7f2, 0xa801, 0xa803, 0xa805, 0xa807, 0xa80a, 0xa80c, 0xa822,
0xa838, 0xa838, 0xa840, 0xa873, 0xa882, 0xa8b3, 0xa8f2, 0xa8f7, 0xa8fb,
0xa8fb, 0xa8fd, 0xa8fe, 0xa90a, 0xa925, 0xa930, 0xa946, 0xa960, 0xa97c,
0xa984, 0xa9b2, 0xa9cf, 0xa9cf, 0xa9e0, 0xa9e4, 0xa9e6, 0xa9ef, 0xa9fa,
0xa9fe, 0xaa00, 0xaa28, 0xaa40, 0xaa42, 0xaa44, 0xaa4b, 0xaa60, 0xaa76,
0xaa7a, 0xaa7a, 0xaa7e, 0xaaaf, 0xaab1, 0xaab1, 0xaab5, 0xaab6, 0xaab9,
0xaabd, 0xaac0, 0xaac0, 0xaac2, 0xaac2, 0xaadb, 0xaadd, 0xaae0, 0xaaea,
0xaaf2, 0xaaf4, 0xab01, 0xab06, 0xab09, 0xab0e, 0xab11, 0xab16, 0xab20,
0xab26, 0xab28, 0xab2e, 0xab30, 0xab5a, 0xab5c, 0xab69, 0xab70, 0xabe2,
0xac00, 0xd7a3, 0xd7b0, 0xd7c6, 0xd7cb, 0xd7fb, 0xf900, 0xfa6d, 0xfa70,
0xfad9, 0xfb00, 0xfb06, 0xfb13, 0xfb17, 0xfb1d, 0xfb1d, 0xfb1f, 0xfb28,
0xfb2a, 0xfb36, 0xfb38, 0xfb3c, 0xfb3e, 0xfb3e, 0xfb40, 0xfb41, 0xfb43,
0xfb44, 0xfb46, 0xfbb1, 0xfbd3, 0xfd3d, 0xfd50, 0xfd8f, 0xfd92, 0xfdc7,
0xfdf0, 0xfdfc, 0xfe33, 0xfe34, 0xfe4d, 0xfe4f, 0xfe69, 0xfe69, 0xfe70,
0xfe74, 0xfe76, 0xfefc, 0xff04, 0xff04, 0xff21, 0xff3a, 0xff3f, 0xff3f,
0xff41, 0xff5a, 0xff66, 0xffbe, 0xffc2, 0xffc7, 0xffca, 0xffcf, 0xffd2,
0xffd7, 0xffda, 0xffdc, 0xffe0, 0xffe1, 0xffe5, 0xffe6, 0x10000, 0x1000b,
0x1000d, 0x10026, 0x10028, 0x1003a, 0x1003c, 0x1003d, 0x1003f, 0x1004d,
0x10050, 0x1005d, 0x10080, 0x100fa, 0x10140, 0x10174, 0x10280, 0x1029c,
0x102a0, 0x102d0, 0x10300, 0x1031f, 0x1032d, 0x1034a, 0x10350, 0x10375,
0x10380, 0x1039d, 0x103a0, 0x103c3, 0x103c8, 0x103cf, 0x103d1, 0x103d5,
0x10400, 0x1049d, 0x104b0, 0x104d3, 0x104d8, 0x104fb, 0x10500, 0x10527,
0x10530, 0x10563, 0x10570, 0x1057a, 0x1057c, 0x1058a, 0x1058c, 0x10592,
0x10594, 0x10595, 0x10597, 0x105a1, 0x105a3, 0x105b1, 0x105b3, 0x105b9,
0x105bb, 0x105bc, 0x10600, 0x10736, 0x10740, 0x10755, 0x10760, 0x10767,
0x10780, 0x10785, 0x10787, 0x107b0, 0x107b2, 0x107ba, 0x10800, 0x10805,
0x10808, 0x10808, 0x1080a, 0x10835, 0x10837, 0x10838, 0x1083c, 0x1083c,
0x1083f, 0x10855, 0x10860, 0x10876, 0x10880, 0x1089e, 0x108e0, 0x108f2,
0x108f4, 0x108f5, 0x10900, 0x10915, 0x10920, 0x10939, 0x10980, 0x109b7,
0x109be, 0x109bf, 0x10a00, 0x10a00, 0x10a10, 0x10a13, 0x10a15, 0x10a17,
0x10a19, 0x10a35, 0x10a60, 0x10a7c, 0x10a80, 0x10a9c, 0x10ac0, 0x10ac7,
0x10ac9, 0x10ae4, 0x10b00, 0x10b35, 0x10b40, 0x10b55, 0x10b60, 0x10b72,
0x10b80, 0x10b91, 0x10c00, 0x10c48, 0x10c80, 0x10cb2, 0x10cc0, 0x10cf2,
0x10d00, 0x10d23, 0x10e80, 0x10ea9, 0x10eb0, 0x10eb1, 0x10f00, 0x10f1c,
0x10f27, 0x10f27, 0x10f30, 0x10f45, 0x10f70, 0x10f81, 0x10fb0, 0x10fc4,
0x10fe0, 0x10ff6, 0x11003, 0x11037, 0x11071, 0x11072, 0x11075, 0x11075,
0x11083, 0x110af, 0x110d0, 0x110e8, 0x11103, 0x11126, 0x11144, 0x11144,
0x11147, 0x11147, 0x11150, 0x11172, 0x11176, 0x11176, 0x11183, 0x111b2,
0x111c1, 0x111c4, 0x111da, 0x111da, 0x111dc, 0x111dc, 0x11200, 0x11211,
0x11213, 0x1122b, 0x11280, 0x11286, 0x11288, 0x11288, 0x1128a, 0x1128d,
0x1128f, 0x1129d, 0x1129f, 0x112a8, 0x112b0, 0x112de, 0x11305, 0x1130c,
0x1130f, 0x11310, 0x11313, 0x11328, 0x1132a, 0x11330, 0x11332, 0x11333,
0x11335, 0x11339, 0x1133d, 0x1133d, 0x11350, 0x11350, 0x1135d, 0x11361,
0x11400, 0x11434, 0x11447, 0x1144a, 0x1145f, 0x11461, 0x11480, 0x114af,
0x114c4, 0x114c5, 0x114c7, 0x114c7, 0x11580, 0x115ae, 0x115d8, 0x115db,
0x11600, 0x1162f, 0x11644, 0x11644, 0x11680, 0x116aa, 0x116b8, 0x116b8,
0x11700, 0x1171a, 0x11740, 0x11746, 0x11800, 0x1182b, 0x118a0, 0x118df,
0x118ff, 0x11906, 0x11909, 0x11909, 0x1190c, 0x11913, 0x11915, 0x11916,
0x11918, 0x1192f, 0x1193f, 0x1193f, 0x11941, 0x11941, 0x119a0, 0x119a7,
0x119aa, 0x119d0, 0x119e1, 0x119e1, 0x119e3, 0x119e3, 0x11a00, 0x11a00,
0x11a0b, 0x11a32, 0x11a3a, 0x11a3a, 0x11a50, 0x11a50, 0x11a5c, 0x11a89,
0x11a9d, 0x11a9d, 0x11ab0, 0x11af8, 0x11c00, 0x11c08, 0x11c0a, 0x11c2e,
0x11c40, 0x11c40, 0x11c72, 0x11c8f, 0x11d00, 0x11d06, 0x11d08, 0x11d09,
0x11d0b, 0x11d30, 0x11d46, 0x11d46, 0x11d60, 0x11d65, 0x11d67, 0x11d68,
0x11d6a, 0x11d89, 0x11d98, 0x11d98, 0x11ee0, 0x11ef2, 0x11fb0, 0x11fb0,
0x11fdd, 0x11fe0, 0x12000, 0x12399, 0x12400, 0x1246e, 0x12480, 0x12543,
0x12f90, 0x12ff0, 0x13000, 0x1342e, 0x14400, 0x14646, 0x16800, 0x16a38,
0x16a40, 0x16a5e, 0x16a70, 0x16abe, 0x16ad0, 0x16aed, 0x16b00, 0x16b2f,
0x16b40, 0x16b43, 0x16b63, 0x16b77, 0x16b7d, 0x16b8f, 0x16e40, 0x16e7f,
0x16f00, 0x16f4a, 0x16f50, 0x16f50, 0x16f93, 0x16f9f, 0x16fe0, 0x16fe1,
0x16fe3, 0x16fe3, 0x17000, 0x187f7, 0x18800, 0x18cd5, 0x18d00, 0x18d08,
0x1aff0, 0x1aff3, 0x1aff5, 0x1affb, 0x1affd, 0x1affe, 0x1b000, 0x1b122,
0x1b150, 0x1b152, 0x1b164, 0x1b167, 0x1b170, 0x1b2fb, 0x1bc00, 0x1bc6a,
0x1bc70, 0x1bc7c, 0x1bc80, 0x1bc88, 0x1bc90, 0x1bc99, 0x1d400, 0x1d454,
0x1d456, 0x1d49c, 0x1d49e, 0x1d49f, 0x1d4a2, 0x1d4a2, 0x1d4a5, 0x1d4a6,
0x1d4a9, 0x1d4ac, 0x1d4ae, 0x1d4b9, 0x1d4bb, 0x1d4bb, 0x1d4bd, 0x1d4c3,
0x1d4c5, 0x1d505, 0x1d507, 0x1d50a, 0x1d50d, 0x1d514, 0x1d516, 0x1d51c,
0x1d51e, 0x1d539, 0x1d53b, 0x1d53e, 0x1d540, 0x1d544, 0x1d546, 0x1d546,
0x1d54a, 0x1d550, 0x1d552, 0x1d6a5, 0x1d6a8, 0x1d6c0, 0x1d6c2, 0x1d6da,
0x1d6dc, 0x1d6fa, 0x1d6fc, 0x1d714, 0x1d716, 0x1d734, 0x1d736, 0x1d74e,
0x1d750, 0x1d76e, 0x1d770, 0x1d788, 0x1d78a, 0x1d7a8, 0x1d7aa, 0x1d7c2,
0x1d7c4, 0x1d7cb, 0x1df00, 0x1df1e, 0x1e100, 0x1e12c, 0x1e137, 0x1e13d,
0x1e14e, 0x1e14e, 0x1e290, 0x1e2ad, 0x1e2c0, 0x1e2eb, 0x1e2ff, 0x1e2ff,
0x1e7e0, 0x1e7e6, 0x1e7e8, 0x1e7eb, 0x1e7ed, 0x1e7ee, 0x1e7f0, 0x1e7fe,
0x1e800, 0x1e8c4, 0x1e900, 0x1e943, 0x1e94b, 0x1e94b, 0x1ecb0, 0x1ecb0,
0x1ee00, 0x1ee03, 0x1ee05, 0x1ee1f, 0x1ee21, 0x1ee22, 0x1ee24, 0x1ee24,
0x1ee27, 0x1ee27, 0x1ee29, 0x1ee32, 0x1ee34, 0x1ee37, 0x1ee39, 0x1ee39,
0x1ee3b, 0x1ee3b, 0x1ee42, 0x1ee42, 0x1ee47, 0x1ee47, 0x1ee49, 0x1ee49,
0x1ee4b, 0x1ee4b, 0x1ee4d, 0x1ee4f, 0x1ee51, 0x1ee52, 0x1ee54, 0x1ee54,
0x1ee57, 0x1ee57, 0x1ee59, 0x1ee59, 0x1ee5b, 0x1ee5b, 0x1ee5d, 0x1ee5d,
0x1ee5f, 0x1ee5f, 0x1ee61, 0x1ee62, 0x1ee64, 0x1ee64, 0x1ee67, 0x1ee6a,
0x1ee6c, 0x1ee72, 0x1ee74, 0x1ee77, 0x1ee79, 0x1ee7c, 0x1ee7e, 0x1ee7e,
0x1ee80, 0x1ee89, 0x1ee8b, 0x1ee9b, 0x1eea1, 0x1eea3, 0x1eea5, 0x1eea9,
0x1eeab, 0x1eebb, 0x20000, 0x2a6df, 0x2a700, 0x2b738, 0x2b740, 0x2b81d,
0x2b820, 0x2cea1, 0x2ceb0, 0x2ebe0, 0x2f800, 0x2fa1d, 0x30000, 0x3134a};
}
private static final int[] NFA_MOVES_FTL_EXPRESSION_133 = NFA_MOVES_FTL_EXPRESSION_133_init();
private static int[] NFA_MOVES_FTL_EXPRESSION_133_init() {
return new int[] {0x0, 0x8, 0xe, 0x1b, '$', '$', '0', '9', 'A', 'Z', '_',
'_', 'a', 'z', 0x7f, 0x9f, 0xa2, 0xa5, 0xaa, 0xaa, 0xad, 0xad, 0xb5, 0xb5,
0xba, 0xba, 0xc0, 0xd6, 0xd8, 0xf6, 0xf8, 0x2c1, 0x2c6, 0x2d1, 0x2e0,
0x2e4, 0x2ec, 0x2ec, 0x2ee, 0x2ee, 0x300, 0x374, 0x376, 0x377, 0x37a,
0x37d, 0x37f, 0x37f, 0x386, 0x386, 0x388, 0x38a, 0x38c, 0x38c, 0x38e,
0x3a1, 0x3a3, 0x3f5, 0x3f7, 0x481, 0x483, 0x487, 0x48a, 0x52f, 0x531,
0x556, 0x559, 0x559, 0x560, 0x588, 0x58f, 0x58f, 0x591, 0x5bd, 0x5bf,
0x5bf, 0x5c1, 0x5c2, 0x5c4, 0x5c5, 0x5c7, 0x5c7, 0x5d0, 0x5ea, 0x5ef,
0x5f2, 0x600, 0x605, 0x60b, 0x60b, 0x610, 0x61a, 0x61c, 0x61c, 0x620,
0x669, 0x66e, 0x6d3, 0x6d5, 0x6dd, 0x6df, 0x6e8, 0x6ea, 0x6fc, 0x6ff,
0x6ff, 0x70f, 0x74a, 0x74d, 0x7b1, 0x7c0, 0x7f5, 0x7fa, 0x7fa, 0x7fd,
0x82d, 0x840, 0x85b, 0x860, 0x86a, 0x870, 0x887, 0x889, 0x88e, 0x890,
0x891, 0x898, 0x963, 0x966, 0x96f, 0x971, 0x983, 0x985, 0x98c, 0x98f,
0x990, 0x993, 0x9a8, 0x9aa, 0x9b0, 0x9b2, 0x9b2, 0x9b6, 0x9b9, 0x9bc,
0x9c4, 0x9c7, 0x9c8, 0x9cb, 0x9ce, 0x9d7, 0x9d7, 0x9dc, 0x9dd, 0x9df,
0x9e3, 0x9e6, 0x9f3, 0x9fb, 0x9fc, 0x9fe, 0x9fe, 0xa01, 0xa03, 0xa05,
0xa0a, 0xa0f, 0xa10, 0xa13, 0xa28, 0xa2a, 0xa30, 0xa32, 0xa33, 0xa35,
0xa36, 0xa38, 0xa39, 0xa3c, 0xa3c, 0xa3e, 0xa42, 0xa47, 0xa48, 0xa4b,
0xa4d, 0xa51, 0xa51, 0xa59, 0xa5c, 0xa5e, 0xa5e, 0xa66, 0xa75, 0xa81,
0xa83, 0xa85, 0xa8d, 0xa8f, 0xa91, 0xa93, 0xaa8, 0xaaa, 0xab0, 0xab2,
0xab3, 0xab5, 0xab9, 0xabc, 0xac5, 0xac7, 0xac9, 0xacb, 0xacd, 0xad0,
0xad0, 0xae0, 0xae3, 0xae6, 0xaef, 0xaf1, 0xaf1, 0xaf9, 0xaff, 0xb01,
0xb03, 0xb05, 0xb0c, 0xb0f, 0xb10, 0xb13, 0xb28, 0xb2a, 0xb30, 0xb32,
0xb33, 0xb35, 0xb39, 0xb3c, 0xb44, 0xb47, 0xb48, 0xb4b, 0xb4d, 0xb55,
0xb57, 0xb5c, 0xb5d, 0xb5f, 0xb63, 0xb66, 0xb6f, 0xb71, 0xb71, 0xb82,
0xb83, 0xb85, 0xb8a, 0xb8e, 0xb90, 0xb92, 0xb95, 0xb99, 0xb9a, 0xb9c,
0xb9c, 0xb9e, 0xb9f, 0xba3, 0xba4, 0xba8, 0xbaa, 0xbae, 0xbb9, 0xbbe,
0xbc2, 0xbc6, 0xbc8, 0xbca, 0xbcd, 0xbd0, 0xbd0, 0xbd7, 0xbd7, 0xbe6,
0xbef, 0xbf9, 0xbf9, 0xc00, 0xc0c, 0xc0e, 0xc10, 0xc12, 0xc28, 0xc2a,
0xc39, 0xc3c, 0xc44, 0xc46, 0xc48, 0xc4a, 0xc4d, 0xc55, 0xc56, 0xc58,
0xc5a, 0xc5d, 0xc5d, 0xc60, 0xc63, 0xc66, 0xc6f, 0xc80, 0xc83, 0xc85,
0xc8c, 0xc8e, 0xc90, 0xc92, 0xca8, 0xcaa, 0xcb3, 0xcb5, 0xcb9, 0xcbc,
0xcc4, 0xcc6, 0xcc8, 0xcca, 0xccd, 0xcd5, 0xcd6, 0xcdd, 0xcde, 0xce0,
0xce3, 0xce6, 0xcef, 0xcf1, 0xcf2, 0xd00, 0xd0c, 0xd0e, 0xd10, 0xd12,
0xd44, 0xd46, 0xd48, 0xd4a, 0xd4e, 0xd54, 0xd57, 0xd5f, 0xd63, 0xd66,
0xd6f, 0xd7a, 0xd7f, 0xd81, 0xd83, 0xd85, 0xd96, 0xd9a, 0xdb1, 0xdb3,
0xdbb, 0xdbd, 0xdbd, 0xdc0, 0xdc6, 0xdca, 0xdca, 0xdcf, 0xdd4, 0xdd6,
0xdd6, 0xdd8, 0xddf, 0xde6, 0xdef, 0xdf2, 0xdf3, 0xe01, 0xe3a, 0xe3f,
0xe4e, 0xe50, 0xe59, 0xe81, 0xe82, 0xe84, 0xe84, 0xe86, 0xe8a, 0xe8c,
0xea3, 0xea5, 0xea5, 0xea7, 0xebd, 0xec0, 0xec4, 0xec6, 0xec6, 0xec8,
0xecd, 0xed0, 0xed9, 0xedc, 0xedf, 0xf00, 0xf00, 0xf18, 0xf19, 0xf20,
0xf29, 0xf35, 0xf35, 0xf37, 0xf37, 0xf39, 0xf39, 0xf3e, 0xf47, 0xf49,
0xf6c, 0xf71, 0xf84, 0xf86, 0xf97, 0xf99, 0xfbc, 0xfc6, 0xfc6, 0x1000,
0x1049, 0x1050, 0x109d, 0x10a0, 0x10c5, 0x10c7, 0x10c7, 0x10cd, 0x10cd,
0x10d0, 0x10fa, 0x10fc, 0x1248, 0x124a, 0x124d, 0x1250, 0x1256, 0x1258,
0x1258, 0x125a, 0x125d, 0x1260, 0x1288, 0x128a, 0x128d, 0x1290, 0x12b0,
0x12b2, 0x12b5, 0x12b8, 0x12be, 0x12c0, 0x12c0, 0x12c2, 0x12c5, 0x12c8,
0x12d6, 0x12d8, 0x1310, 0x1312, 0x1315, 0x1318, 0x135a, 0x135d, 0x135f,
0x1380, 0x138f, 0x13a0, 0x13f5, 0x13f8, 0x13fd, 0x1401, 0x166c, 0x166f,
0x167f, 0x1681, 0x169a, 0x16a0, 0x16ea, 0x16ee, 0x16f8, 0x1700, 0x1715,
0x171f, 0x1734, 0x1740, 0x1753, 0x1760, 0x176c, 0x176e, 0x1770, 0x1772,
0x1773, 0x1780, 0x17d3, 0x17d7, 0x17d7, 0x17db, 0x17dd, 0x17e0, 0x17e9,
0x180b, 0x1819, 0x1820, 0x1878, 0x1880, 0x18aa, 0x18b0, 0x18f5, 0x1900,
0x191e, 0x1920, 0x192b, 0x1930, 0x193b, 0x1946, 0x196d, 0x1970, 0x1974,
0x1980, 0x19ab, 0x19b0, 0x19c9, 0x19d0, 0x19d9, 0x1a00, 0x1a1b, 0x1a20,
0x1a5e, 0x1a60, 0x1a7c, 0x1a7f, 0x1a89, 0x1a90, 0x1a99, 0x1aa7, 0x1aa7,
0x1ab0, 0x1abd, 0x1abf, 0x1ace, 0x1b00, 0x1b4c, 0x1b50, 0x1b59, 0x1b6b,
0x1b73, 0x1b80, 0x1bf3, 0x1c00, 0x1c37, 0x1c40, 0x1c49, 0x1c4d, 0x1c7d,
0x1c80, 0x1c88, 0x1c90, 0x1cba, 0x1cbd, 0x1cbf, 0x1cd0, 0x1cd2, 0x1cd4,
0x1cfa, 0x1d00, 0x1f15, 0x1f18, 0x1f1d, 0x1f20, 0x1f45, 0x1f48, 0x1f4d,
0x1f50, 0x1f57, 0x1f59, 0x1f59, 0x1f5b, 0x1f5b, 0x1f5d, 0x1f5d, 0x1f5f,
0x1f7d, 0x1f80, 0x1fb4, 0x1fb6, 0x1fbc, 0x1fbe, 0x1fbe, 0x1fc2, 0x1fc4,
0x1fc6, 0x1fcc, 0x1fd0, 0x1fd3, 0x1fd6, 0x1fdb, 0x1fe0, 0x1fec, 0x1ff2,
0x1ff4, 0x1ff6, 0x1ffc, 0x200b, 0x200f, 0x202a, 0x202e, 0x203f, 0x2040,
0x2054, 0x2054, 0x2060, 0x2064, 0x2066, 0x206f, 0x2071, 0x2071, 0x207f,
0x207f, 0x2090, 0x209c, 0x20a0, 0x20c0, 0x20d0, 0x20dc, 0x20e1, 0x20e1,
0x20e5, 0x20f0, 0x2102, 0x2102, 0x2107, 0x2107, 0x210a, 0x2113, 0x2115,
0x2115, 0x2119, 0x211d, 0x2124, 0x2124, 0x2126, 0x2126, 0x2128, 0x2128,
0x212a, 0x212d, 0x212f, 0x2139, 0x213c, 0x213f, 0x2145, 0x2149, 0x214e,
0x214e, 0x2160, 0x2188, 0x2c00, 0x2ce4, 0x2ceb, 0x2cf3, 0x2d00, 0x2d25,
0x2d27, 0x2d27, 0x2d2d, 0x2d2d, 0x2d30, 0x2d67, 0x2d6f, 0x2d6f, 0x2d7f,
0x2d96, 0x2da0, 0x2da6, 0x2da8, 0x2dae, 0x2db0, 0x2db6, 0x2db8, 0x2dbe,
0x2dc0, 0x2dc6, 0x2dc8, 0x2dce, 0x2dd0, 0x2dd6, 0x2dd8, 0x2dde, 0x2de0,
0x2dff, 0x2e2f, 0x2e2f, 0x3005, 0x3007, 0x3021, 0x302f, 0x3031, 0x3035,
0x3038, 0x303c, 0x3041, 0x3096, 0x3099, 0x309a, 0x309d, 0x309f, 0x30a1,
0x30fa, 0x30fc, 0x30ff, 0x3105, 0x312f, 0x3131, 0x318e, 0x31a0, 0x31bf,
0x31f0, 0x31ff, 0x3400, 0x4dbf, 0x4e00, 0xa48c, 0xa4d0, 0xa4fd, 0xa500,
0xa60c, 0xa610, 0xa62b, 0xa640, 0xa66f, 0xa674, 0xa67d, 0xa67f, 0xa6f1,
0xa717, 0xa71f, 0xa722, 0xa788, 0xa78b, 0xa7ca, 0xa7d0, 0xa7d1, 0xa7d3,
0xa7d3, 0xa7d5, 0xa7d9, 0xa7f2, 0xa827, 0xa82c, 0xa82c, 0xa838, 0xa838,
0xa840, 0xa873, 0xa880, 0xa8c5, 0xa8d0, 0xa8d9, 0xa8e0, 0xa8f7, 0xa8fb,
0xa8fb, 0xa8fd, 0xa92d, 0xa930, 0xa953, 0xa960, 0xa97c, 0xa980, 0xa9c0,
0xa9cf, 0xa9d9, 0xa9e0, 0xa9fe, 0xaa00, 0xaa36, 0xaa40, 0xaa4d, 0xaa50,
0xaa59, 0xaa60, 0xaa76, 0xaa7a, 0xaac2, 0xaadb, 0xaadd, 0xaae0, 0xaaef,
0xaaf2, 0xaaf6, 0xab01, 0xab06, 0xab09, 0xab0e, 0xab11, 0xab16, 0xab20,
0xab26, 0xab28, 0xab2e, 0xab30, 0xab5a, 0xab5c, 0xab69, 0xab70, 0xabea,
0xabec, 0xabed, 0xabf0, 0xabf9, 0xac00, 0xd7a3, 0xd7b0, 0xd7c6, 0xd7cb,
0xd7fb, 0xf900, 0xfa6d, 0xfa70, 0xfad9, 0xfb00, 0xfb06, 0xfb13, 0xfb17,
0xfb1d, 0xfb28, 0xfb2a, 0xfb36, 0xfb38, 0xfb3c, 0xfb3e, 0xfb3e, 0xfb40,
0xfb41, 0xfb43, 0xfb44, 0xfb46, 0xfbb1, 0xfbd3, 0xfd3d, 0xfd50, 0xfd8f,
0xfd92, 0xfdc7, 0xfdf0, 0xfdfc, 0xfe00, 0xfe0f, 0xfe20, 0xfe2f, 0xfe33,
0xfe34, 0xfe4d, 0xfe4f, 0xfe69, 0xfe69, 0xfe70, 0xfe74, 0xfe76, 0xfefc,
0xfeff, 0xfeff, 0xff04, 0xff04, 0xff10, 0xff19, 0xff21, 0xff3a, 0xff3f,
0xff3f, 0xff41, 0xff5a, 0xff66, 0xffbe, 0xffc2, 0xffc7, 0xffca, 0xffcf,
0xffd2, 0xffd7, 0xffda, 0xffdc, 0xffe0, 0xffe1, 0xffe5, 0xffe6, 0xfff9,
0xfffb, 0x10000, 0x1000b, 0x1000d, 0x10026, 0x10028, 0x1003a, 0x1003c,
0x1003d, 0x1003f, 0x1004d, 0x10050, 0x1005d, 0x10080, 0x100fa, 0x10140,
0x10174, 0x101fd, 0x101fd, 0x10280, 0x1029c, 0x102a0, 0x102d0, 0x102e0,
0x102e0, 0x10300, 0x1031f, 0x1032d, 0x1034a, 0x10350, 0x1037a, 0x10380,
0x1039d, 0x103a0, 0x103c3, 0x103c8, 0x103cf, 0x103d1, 0x103d5, 0x10400,
0x1049d, 0x104a0, 0x104a9, 0x104b0, 0x104d3, 0x104d8, 0x104fb, 0x10500,
0x10527, 0x10530, 0x10563, 0x10570, 0x1057a, 0x1057c, 0x1058a, 0x1058c,
0x10592, 0x10594, 0x10595, 0x10597, 0x105a1, 0x105a3, 0x105b1, 0x105b3,
0x105b9, 0x105bb, 0x105bc, 0x10600, 0x10736, 0x10740, 0x10755, 0x10760,
0x10767, 0x10780, 0x10785, 0x10787, 0x107b0, 0x107b2, 0x107ba, 0x10800,
0x10805, 0x10808, 0x10808, 0x1080a, 0x10835, 0x10837, 0x10838, 0x1083c,
0x1083c, 0x1083f, 0x10855, 0x10860, 0x10876, 0x10880, 0x1089e, 0x108e0,
0x108f2, 0x108f4, 0x108f5, 0x10900, 0x10915, 0x10920, 0x10939, 0x10980,
0x109b7, 0x109be, 0x109bf, 0x10a00, 0x10a03, 0x10a05, 0x10a06, 0x10a0c,
0x10a13, 0x10a15, 0x10a17, 0x10a19, 0x10a35, 0x10a38, 0x10a3a, 0x10a3f,
0x10a3f, 0x10a60, 0x10a7c, 0x10a80, 0x10a9c, 0x10ac0, 0x10ac7, 0x10ac9,
0x10ae6, 0x10b00, 0x10b35, 0x10b40, 0x10b55, 0x10b60, 0x10b72, 0x10b80,
0x10b91, 0x10c00, 0x10c48, 0x10c80, 0x10cb2, 0x10cc0, 0x10cf2, 0x10d00,
0x10d27, 0x10d30, 0x10d39, 0x10e80, 0x10ea9, 0x10eab, 0x10eac, 0x10eb0,
0x10eb1, 0x10f00, 0x10f1c, 0x10f27, 0x10f27, 0x10f30, 0x10f50, 0x10f70,
0x10f85, 0x10fb0, 0x10fc4, 0x10fe0, 0x10ff6, 0x11000, 0x11046, 0x11066,
0x11075, 0x1107f, 0x110ba, 0x110bd, 0x110bd, 0x110c2, 0x110c2, 0x110cd,
0x110cd, 0x110d0, 0x110e8, 0x110f0, 0x110f9, 0x11100, 0x11134, 0x11136,
0x1113f, 0x11144, 0x11147, 0x11150, 0x11173, 0x11176, 0x11176, 0x11180,
0x111c4, 0x111c9, 0x111cc, 0x111ce, 0x111da, 0x111dc, 0x111dc, 0x11200,
0x11211, 0x11213, 0x11237, 0x1123e, 0x1123e, 0x11280, 0x11286, 0x11288,
0x11288, 0x1128a, 0x1128d, 0x1128f, 0x1129d, 0x1129f, 0x112a8, 0x112b0,
0x112ea, 0x112f0, 0x112f9, 0x11300, 0x11303, 0x11305, 0x1130c, 0x1130f,
0x11310, 0x11313, 0x11328, 0x1132a, 0x11330, 0x11332, 0x11333, 0x11335,
0x11339, 0x1133b, 0x11344, 0x11347, 0x11348, 0x1134b, 0x1134d, 0x11350,
0x11350, 0x11357, 0x11357, 0x1135d, 0x11363, 0x11366, 0x1136c, 0x11370,
0x11374, 0x11400, 0x1144a, 0x11450, 0x11459, 0x1145e, 0x11461, 0x11480,
0x114c5, 0x114c7, 0x114c7, 0x114d0, 0x114d9, 0x11580, 0x115b5, 0x115b8,
0x115c0, 0x115d8, 0x115dd, 0x11600, 0x11640, 0x11644, 0x11644, 0x11650,
0x11659, 0x11680, 0x116b8, 0x116c0, 0x116c9, 0x11700, 0x1171a, 0x1171d,
0x1172b, 0x11730, 0x11739, 0x11740, 0x11746, 0x11800, 0x1183a, 0x118a0,
0x118e9, 0x118ff, 0x11906, 0x11909, 0x11909, 0x1190c, 0x11913, 0x11915,
0x11916, 0x11918, 0x11935, 0x11937, 0x11938, 0x1193b, 0x11943, 0x11950,
0x11959, 0x119a0, 0x119a7, 0x119aa, 0x119d7, 0x119da, 0x119e1, 0x119e3,
0x119e4, 0x11a00, 0x11a3e, 0x11a47, 0x11a47, 0x11a50, 0x11a99, 0x11a9d,
0x11a9d, 0x11ab0, 0x11af8, 0x11c00, 0x11c08, 0x11c0a, 0x11c36, 0x11c38,
0x11c40, 0x11c50, 0x11c59, 0x11c72, 0x11c8f, 0x11c92, 0x11ca7, 0x11ca9,
0x11cb6, 0x11d00, 0x11d06, 0x11d08, 0x11d09, 0x11d0b, 0x11d36, 0x11d3a,
0x11d3a, 0x11d3c, 0x11d3d, 0x11d3f, 0x11d47, 0x11d50, 0x11d59, 0x11d60,
0x11d65, 0x11d67, 0x11d68, 0x11d6a, 0x11d8e, 0x11d90, 0x11d91, 0x11d93,
0x11d98, 0x11da0, 0x11da9, 0x11ee0, 0x11ef6, 0x11fb0, 0x11fb0, 0x11fdd,
0x11fe0, 0x12000, 0x12399, 0x12400, 0x1246e, 0x12480, 0x12543, 0x12f90,
0x12ff0, 0x13000, 0x1342e, 0x13430, 0x13438, 0x14400, 0x14646, 0x16800,
0x16a38, 0x16a40, 0x16a5e, 0x16a60, 0x16a69, 0x16a70, 0x16abe, 0x16ac0,
0x16ac9, 0x16ad0, 0x16aed, 0x16af0, 0x16af4, 0x16b00, 0x16b36, 0x16b40,
0x16b43, 0x16b50, 0x16b59, 0x16b63, 0x16b77, 0x16b7d, 0x16b8f, 0x16e40,
0x16e7f, 0x16f00, 0x16f4a, 0x16f4f, 0x16f87, 0x16f8f, 0x16f9f, 0x16fe0,
0x16fe1, 0x16fe3, 0x16fe4, 0x16ff0, 0x16ff1, 0x17000, 0x187f7, 0x18800,
0x18cd5, 0x18d00, 0x18d08, 0x1aff0, 0x1aff3, 0x1aff5, 0x1affb, 0x1affd,
0x1affe, 0x1b000, 0x1b122, 0x1b150, 0x1b152, 0x1b164, 0x1b167, 0x1b170,
0x1b2fb, 0x1bc00, 0x1bc6a, 0x1bc70, 0x1bc7c, 0x1bc80, 0x1bc88, 0x1bc90,
0x1bc99, 0x1bc9d, 0x1bc9e, 0x1bca0, 0x1bca3, 0x1cf00, 0x1cf2d, 0x1cf30,
0x1cf46, 0x1d165, 0x1d169, 0x1d16d, 0x1d182, 0x1d185, 0x1d18b, 0x1d1aa,
0x1d1ad, 0x1d242, 0x1d244, 0x1d400, 0x1d454, 0x1d456, 0x1d49c, 0x1d49e,
0x1d49f, 0x1d4a2, 0x1d4a2, 0x1d4a5, 0x1d4a6, 0x1d4a9, 0x1d4ac, 0x1d4ae,
0x1d4b9, 0x1d4bb, 0x1d4bb, 0x1d4bd, 0x1d4c3, 0x1d4c5, 0x1d505, 0x1d507,
0x1d50a, 0x1d50d, 0x1d514, 0x1d516, 0x1d51c, 0x1d51e, 0x1d539, 0x1d53b,
0x1d53e, 0x1d540, 0x1d544, 0x1d546, 0x1d546, 0x1d54a, 0x1d550, 0x1d552,
0x1d6a5, 0x1d6a8, 0x1d6c0, 0x1d6c2, 0x1d6da, 0x1d6dc, 0x1d6fa, 0x1d6fc,
0x1d714, 0x1d716, 0x1d734, 0x1d736, 0x1d74e, 0x1d750, 0x1d76e, 0x1d770,
0x1d788, 0x1d78a, 0x1d7a8, 0x1d7aa, 0x1d7c2, 0x1d7c4, 0x1d7cb, 0x1d7ce,
0x1d7ff, 0x1da00, 0x1da36, 0x1da3b, 0x1da6c, 0x1da75, 0x1da75, 0x1da84,
0x1da84, 0x1da9b, 0x1da9f, 0x1daa1, 0x1daaf, 0x1df00, 0x1df1e, 0x1e000,
0x1e006, 0x1e008, 0x1e018, 0x1e01b, 0x1e021, 0x1e023, 0x1e024, 0x1e026,
0x1e02a, 0x1e100, 0x1e12c, 0x1e130, 0x1e13d, 0x1e140, 0x1e149, 0x1e14e,
0x1e14e, 0x1e290, 0x1e2ae, 0x1e2c0, 0x1e2f9, 0x1e2ff, 0x1e2ff, 0x1e7e0,
0x1e7e6, 0x1e7e8, 0x1e7eb, 0x1e7ed, 0x1e7ee, 0x1e7f0, 0x1e7fe, 0x1e800,
0x1e8c4, 0x1e8d0, 0x1e8d6, 0x1e900, 0x1e94b, 0x1e950, 0x1e959, 0x1ecb0,
0x1ecb0, 0x1ee00, 0x1ee03, 0x1ee05, 0x1ee1f, 0x1ee21, 0x1ee22, 0x1ee24,
0x1ee24, 0x1ee27, 0x1ee27, 0x1ee29, 0x1ee32, 0x1ee34, 0x1ee37, 0x1ee39,
0x1ee39, 0x1ee3b, 0x1ee3b, 0x1ee42, 0x1ee42, 0x1ee47, 0x1ee47, 0x1ee49,
0x1ee49, 0x1ee4b, 0x1ee4b, 0x1ee4d, 0x1ee4f, 0x1ee51, 0x1ee52, 0x1ee54,
0x1ee54, 0x1ee57, 0x1ee57, 0x1ee59, 0x1ee59, 0x1ee5b, 0x1ee5b, 0x1ee5d,
0x1ee5d, 0x1ee5f, 0x1ee5f, 0x1ee61, 0x1ee62, 0x1ee64, 0x1ee64, 0x1ee67,
0x1ee6a, 0x1ee6c, 0x1ee72, 0x1ee74, 0x1ee77, 0x1ee79, 0x1ee7c, 0x1ee7e,
0x1ee7e, 0x1ee80, 0x1ee89, 0x1ee8b, 0x1ee9b, 0x1eea1, 0x1eea3, 0x1eea5,
0x1eea9, 0x1eeab, 0x1eebb, 0x1fbf0, 0x1fbf9, 0x20000, 0x2a6df, 0x2a700,
0x2b738, 0x2b740, 0x2b81d, 0x2b820, 0x2cea1, 0x2ceb0, 0x2ebe0, 0x2f800,
0x2fa1d, 0x30000, 0x3134a, 0xe0001, 0xe0001, 0xe0020, 0xe007f, 0xe0100,
0xe01ef};
}
private static void NFA_FUNCTIONS_init() {
NfaFunction[] functions = new NfaFunction[] {FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex0,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex1, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex2,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex3, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex4,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex5, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex6,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex7, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex8,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex9, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex10,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex11, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex12,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex13, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex14,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex15, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex16,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex17, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex18,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex19, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex20,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex21, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex22,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex23, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex24,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex25, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex26,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex27, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex28,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex29, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex30,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex31, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex32,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex33, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex34,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex35, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex36,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex37, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex38,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex39, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex40,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex41, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex42,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex43, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex44,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex45, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex46,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex47, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex48,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex49, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex50,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex51, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex52,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex53, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex54,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex55, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex56,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex57, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex58,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex59, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex60,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex61, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex62,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex63, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex64,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex65, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex66,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex67, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex68,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex69, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex70,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex71, FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex72,
FTL_EXPRESSION::getNfaNameFTL_EXPRESSIONIndex73};
functionTableMap.put(LexicalState.FTL_EXPRESSION, functions);
}
}
/**
* Holder class for NFA code related to EXPRESSION_COMMENT lexical state
*/
private static class EXPRESSION_COMMENT {
private static TokenType getNfaNameEXPRESSION_COMMENTIndex0(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (ch == '-') {
if (validTypes == null || validTypes.contains(_TOKEN_4)) {
nextStates.set(3);
}
if (validTypes == null || validTypes.contains(_TOKEN_5)) {
nextStates.set(4);
}
}
if (ch >= 0x0) {
if (validTypes == null || validTypes.contains(_TOKEN_74)) {
type = _TOKEN_74;
}
}
return type;
}
private static TokenType getNfaNameEXPRESSION_COMMENTIndex1(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '>') {
return _TOKEN_4;
}
return null;
}
private static TokenType getNfaNameEXPRESSION_COMMENTIndex2(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == ']') {
return _TOKEN_5;
}
return null;
}
private static TokenType getNfaNameEXPRESSION_COMMENTIndex3(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '-') {
nextStates.set(1);
}
return null;
}
private static TokenType getNfaNameEXPRESSION_COMMENTIndex4(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == '-') {
nextStates.set(2);
}
return null;
}
private static void NFA_FUNCTIONS_init() {
NfaFunction[] functions = new NfaFunction[] {EXPRESSION_COMMENT::getNfaNameEXPRESSION_COMMENTIndex0,
EXPRESSION_COMMENT::getNfaNameEXPRESSION_COMMENTIndex1, EXPRESSION_COMMENT::getNfaNameEXPRESSION_COMMENTIndex2,
EXPRESSION_COMMENT::getNfaNameEXPRESSION_COMMENTIndex3, EXPRESSION_COMMENT::getNfaNameEXPRESSION_COMMENTIndex4};
functionTableMap.put(LexicalState.EXPRESSION_COMMENT, functions);
}
}
/**
* Holder class for NFA code related to FTL_DIRECTIVE lexical state
*/
private static class FTL_DIRECTIVE {
private static TokenType getNfaNameFTL_DIRECTIVEIndex0(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (ch == '\t') {
if (validTypes == null || validTypes.contains(CLOSE_EMPTY_TAG)) {
nextStates.set(33);
}
if (validTypes == null || validTypes.contains(CLOSE_TAG)) {
nextStates.set(17);
}
} else if (ch == '\n') {
if (validTypes == null || validTypes.contains(CLOSE_EMPTY_TAG)) {
nextStates.set(33);
}
if (validTypes == null || validTypes.contains(CLOSE_TAG)) {
nextStates.set(17);
}
} else if (ch == '\r') {
if (validTypes == null || validTypes.contains(CLOSE_EMPTY_TAG)) {
nextStates.set(33);
}
if (validTypes == null || validTypes.contains(CLOSE_TAG)) {
nextStates.set(17);
}
} else if (ch == ' ') {
if (validTypes == null || validTypes.contains(CLOSE_EMPTY_TAG)) {
nextStates.set(33);
}
if (validTypes == null || validTypes.contains(CLOSE_TAG)) {
nextStates.set(17);
}
} else if (ch == '-') {
if (validTypes == null || validTypes.contains(COMMENT)) {
nextStates.set(7);
}
} else if (ch == '/') {
if (validTypes == null || validTypes.contains(CLOSE_EMPTY_TAG)) {
nextStates.set(3);
}
} else if (ch == 'a') {
if (validTypes == null || validTypes.contains(ASSIGN)) {
nextStates.set(29);
}
} else if (ch == 'b') {
if (validTypes == null || validTypes.contains(BREAK)) {
nextStates.set(26);
}
if (validTypes == null || validTypes.contains(BRICK)) {
nextStates.set(62);
}
} else if (ch == 'c') {
if (validTypes == null || validTypes.contains(CASE)) {
nextStates.set(76);
}
} else if (ch == 'd') {
if (validTypes == null || validTypes.contains(DEFAULT)) {
nextStates.set(71);
}
} else if (ch == 'e') {
if (validTypes == null || validTypes.contains(ELSEIF)) {
nextStates.set(79);
}
if (validTypes == null || validTypes.contains(ELSE)) {
nextStates.set(83);
}
} else if (ch == 'f') {
if (validTypes == null || validTypes.contains(FUNCTION)) {
nextStates.set(65);
}
} else if (ch == 'g') {
if (validTypes == null || validTypes.contains(GLOBAL)) {
nextStates.set(34);
}
} else if (ch == 'i') {
if (validTypes == null || validTypes.contains(_INCLUDE)) {
nextStates.set(38);
}
if (validTypes == null || validTypes.contains(IF)) {
nextStates.set(8);
}
if (validTypes == null || validTypes.contains(IMPORT)) {
nextStates.set(88);
}
} else if (ch == 'l') {
if (validTypes == null || validTypes.contains(LIST)) {
nextStates.set(47);
}
if (validTypes == null || validTypes.contains(LOCAL)) {
nextStates.set(49);
}
} else if (ch == 'm') {
if (validTypes == null || validTypes.contains(MACRO)) {
nextStates.set(85);
}
} else if (ch == 'n') {
if (validTypes == null || validTypes.contains(NESTED)) {
nextStates.set(92);
}
} else if (ch == 'o') {
if (validTypes == null || validTypes.contains(OUTPUT_FORMAT)) {
nextStates.set(52);
}
} else if (ch == 'r') {
if (validTypes == null || validTypes.contains(RETURN)) {
nextStates.set(102);
}
} else if (ch == 's') {
if (validTypes == null || validTypes.contains(SWITCH)) {
nextStates.set(43);
}
if (validTypes == null || validTypes.contains(SET)) {
nextStates.set(78);
}
if (validTypes == null || validTypes.contains(SETTING)) {
nextStates.set(96);
}
} else if (ch == 'v') {
if (validTypes == null || validTypes.contains(VAR)) {
nextStates.set(101);
}
} else if (ch == '>') {
if (validTypes == null || validTypes.contains(CLOSE_TAG)) {
type = CLOSE_TAG;
}
} else if (ch == ']') {
if (validTypes == null || validTypes.contains(CLOSE_TAG)) {
type = CLOSE_TAG;
}
}
if (ch == '\t') {
if (validTypes == null || validTypes.contains(BLANK)) {
type = BLANK;
}
} else if (ch == '\n') {
if (validTypes == null || validTypes.contains(BLANK)) {
type = BLANK;
}
} else if (ch == '\r') {
if (validTypes == null || validTypes.contains(BLANK)) {
type = BLANK;
}
} else if (ch == ' ') {
if (validTypes == null || validTypes.contains(BLANK)) {
type = BLANK;
}
}
return type;
}
private static TokenType getNfaNameFTL_DIRECTIVEIndex1(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'k') {
return BREAK;
}
return null;
}
private static TokenType getNfaNameFTL_DIRECTIVEIndex2(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'n') {
return ASSIGN;
}
return null;
}
private static TokenType getNfaNameFTL_DIRECTIVEIndex3(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
TokenType type = null;
if (ch == '>') {
type = CLOSE_EMPTY_TAG;
} else if (ch == ']') {
type = CLOSE_EMPTY_TAG;
}
return type;
}
private static TokenType getNfaNameFTL_DIRECTIVEIndex4(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'l') {
return GLOBAL;
}
return null;
}
private static TokenType getNfaNameFTL_DIRECTIVEIndex5(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'e') {
return _INCLUDE;
}
return null;
}
private static TokenType getNfaNameFTL_DIRECTIVEIndex6(int ch, BitSet nextStates, EnumSet validTypes, EnumSet alreadyMatchedTypes) {
if (ch == 'h') {
return SWITCH;
}
return null;
}
private static TokenType getNfaNameFTL_DIRECTIVEIndex7(int ch, BitSet nextStates, EnumSet