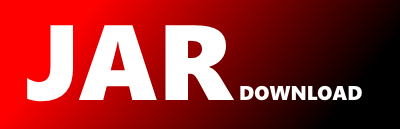
de.schlegel11.lambdadecor.DefaultBehaviour Maven / Gradle / Ivy
package de.schlegel11.lambdadecor;
import java.util.Objects;
import java.util.function.Function;
/**
* @author Marcel Schlegel (schlegel11)
* @since 1.0
*/
public class DefaultBehaviour implements Behaviour {
private final Function, DecorPair> behaviour;
private DefaultBehaviour() {
this(Function.identity());
}
private DefaultBehaviour(Function, DecorPair> behaviour) {
this.behaviour = Objects.requireNonNull(behaviour, "Behaviour is null.");
}
/**
* Creates a new instance.
*
* @param function the {@link Function} that provides an new {@link DefaultBehaviour} instance and returns one.
* @param type for this behaviour
* @return the new instance
* @throws NullPointerException if the {@code function} is null
*/
public static Behaviour newBehaviour(final Function, Behaviour>
function) {
Objects.requireNonNull(function, "Function is null.");
return function.apply(newBehaviour());
}
/**
* Creates a new instance.
*
* @param type for this behaviour
* @return the new instance
*/
public static Behaviour newBehaviour() {
return new DefaultBehaviour<>();
}
public final Behaviour withUnapply(final Function function) {
Objects.requireNonNull(function, "Function is null.");
return new DefaultBehaviour<>(
behaviour.andThen(t -> t.updateUnapply(u -> u.andThen(function.apply(t._Behaviour)))));
}
public final Behaviour with(final Function function) {
Objects.requireNonNull(function, "Function is null.");
return new DefaultBehaviour<>(behaviour.andThen(t -> t.updateBehaviour(function.apply(t._Behaviour))));
}
public final Behaviour merge(final Behaviour other) {
Objects.requireNonNull(other, "Behaviour is null.");
return new DefaultBehaviour<>(behaviour.andThen(p -> {
DecorPair pair = other.apply(p._Behaviour);
return p.updateBehaviour(pair._Behaviour)
.updateUnapply(u -> u.andThen(pair._Unapply));
}));
}
public final DecorPair apply(final T type) {
return behaviour.apply(DecorPair.create(type, Unappliable.EMPTY));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy