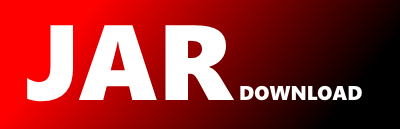
de.schlichtherle.license.wizard.LicensePanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truelicense-swing Show documentation
Show all versions of truelicense-swing Show documentation
Provides an internationalized wizard dialog used to install, verify
and uninstall license keys.
The newest version!
/*
* Copyright (C) 2005-2015 Schlichtherle IT Services.
* All rights reserved. Use is subject to license terms.
*/
package de.schlichtherle.license.wizard;
import com.nexes.wizard.WizardPanelDescriptor;
import de.schlichtherle.license.LicenseContent;
import de.schlichtherle.license.LicenseManager;
import de.schlichtherle.swing.Defaults;
import java.text.DateFormat;
import java.util.Date;
import javax.swing.JPanel;
/**
* @author Christian Schlichtherle
*/
public class LicensePanel extends JPanel {
private static final long serialVersionUID = 1L;
private final LicenseManager manager;
private LicenseContent content;
public LicensePanel(LicenseManager manager) {
this.manager = manager;
initComponents();
}
/**
* Verifies the installed license and updates the panel to display it.
*
* @throws Exception A subclass of this class for various reasons.
* The (localised) detail message should provide more information.
*/
public final void verify()
throws Exception {
content = manager.verify();
updatePanel();
}
/**
* Updates the panel to display the installed license.
*/
protected void updatePanel() {
if (null == content) return;
subjectComponent.setText(toString(content.getSubject()));
holderComponent.setText(toString(content.getHolder()));
infoComponent.setText(toString(content.getInfo()));
consumerComponent.setText(toString(content.getConsumerType()) + " (" + content.getConsumerAmount() + ")");
notBeforeComponent.setText(format(content.getNotBefore()));
notAfterComponent.setText(format(content.getNotAfter()));
issuerComponent.setText(toString(content.getIssuer()));
issuedComponent.setText(format(content.getIssued()));
}
private String format(final Date date) {
return null != date
? DateFormat.getDateTimeInstance(DateFormat.LONG, DateFormat.LONG).format(date)
: "";
}
private String toString(final Object obj) {
return null != obj ? obj.toString() : "";
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
java.awt.GridBagConstraints gridBagConstraints;
holderLabel = new javax.swing.JLabel();
holderScrollPane = new javax.swing.JScrollPane();
holderComponent = new javax.swing.JTextArea();
subjectLabel = new javax.swing.JLabel();
subjectComponent = new javax.swing.JTextArea();
consumerLabel = new javax.swing.JLabel();
consumerComponent = new javax.swing.JTextArea();
notBeforeLabel = new javax.swing.JLabel();
notBeforeComponent = new javax.swing.JTextArea();
notAfterLabel = new javax.swing.JLabel();
notAfterComponent = new javax.swing.JTextArea();
issuerLabel = new javax.swing.JLabel();
issuerScrollPane = new javax.swing.JScrollPane();
issuerComponent = new javax.swing.JTextArea();
issuedLabel = new javax.swing.JLabel();
issuedComponent = new javax.swing.JTextArea();
infoLabel = new javax.swing.JLabel();
infoScrollPane = new javax.swing.JScrollPane();
infoComponent = new javax.swing.JTextArea();
setBorder(javax.swing.BorderFactory.createCompoundBorder(javax.swing.BorderFactory.createTitledBorder(null, Resources.getString("LicensePanel.title"), javax.swing.border.TitledBorder.DEFAULT_JUSTIFICATION, javax.swing.border.TitledBorder.DEFAULT_POSITION, Defaults.labelBoldFont), javax.swing.BorderFactory.createEmptyBorder(10, 10, 10, 10))); // NOI18N
setLayout(new java.awt.GridBagLayout());
holderLabel.setLabelFor(holderComponent);
holderLabel.setText(Resources.getString("LicensePanel.holder.label")); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 0;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.NORTHWEST;
gridBagConstraints.insets = new java.awt.Insets(0, 0, 2, 0);
add(holderLabel, gridBagConstraints);
holderScrollPane.setBorder(javax.swing.BorderFactory.createEtchedBorder());
holderScrollPane.setHorizontalScrollBarPolicy(javax.swing.ScrollPaneConstants.HORIZONTAL_SCROLLBAR_NEVER);
holderScrollPane.setPreferredSize(new java.awt.Dimension(300, 65));
holderComponent.setEditable(false);
holderComponent.setLineWrap(true);
holderComponent.setWrapStyleWord(true);
holderComponent.setBorder(null);
holderComponent.setName("holder");
holderScrollPane.setViewportView(holderComponent);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.weighty = 1.0;
gridBagConstraints.insets = new java.awt.Insets(0, 0, 2, 0);
add(holderScrollPane, gridBagConstraints);
subjectLabel.setLabelFor(subjectComponent);
subjectLabel.setText(Resources.getString("LicensePanel.subject.label")); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 1;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.NORTHWEST;
gridBagConstraints.insets = new java.awt.Insets(2, 0, 2, 0);
add(subjectLabel, gridBagConstraints);
subjectComponent.setEditable(false);
subjectComponent.setBorder(javax.swing.BorderFactory.createEtchedBorder());
subjectComponent.setName("subject");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 1;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
gridBagConstraints.insets = new java.awt.Insets(2, 0, 2, 0);
add(subjectComponent, gridBagConstraints);
consumerLabel.setLabelFor(consumerComponent);
consumerLabel.setText(Resources.getString("LicensePanel.consumer.label")); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 2;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.NORTHWEST;
gridBagConstraints.insets = new java.awt.Insets(2, 0, 2, 0);
add(consumerLabel, gridBagConstraints);
consumerComponent.setEditable(false);
consumerComponent.setBorder(javax.swing.BorderFactory.createEtchedBorder());
consumerComponent.setName("consumer");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 2;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
gridBagConstraints.insets = new java.awt.Insets(2, 0, 2, 0);
add(consumerComponent, gridBagConstraints);
notBeforeLabel.setLabelFor(notBeforeComponent);
notBeforeLabel.setText(Resources.getString("LicensePanel.notBefore.label")); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 4;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.NORTHWEST;
gridBagConstraints.insets = new java.awt.Insets(2, 0, 2, 0);
add(notBeforeLabel, gridBagConstraints);
notBeforeComponent.setEditable(false);
notBeforeComponent.setBorder(javax.swing.BorderFactory.createEtchedBorder());
notBeforeComponent.setName("notBefore");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 4;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
gridBagConstraints.insets = new java.awt.Insets(2, 0, 2, 0);
add(notBeforeComponent, gridBagConstraints);
notAfterLabel.setLabelFor(notAfterComponent);
notAfterLabel.setText(Resources.getString("LicensePanel.notAfter.label")); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 5;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.NORTHWEST;
gridBagConstraints.insets = new java.awt.Insets(2, 0, 2, 0);
add(notAfterLabel, gridBagConstraints);
notAfterComponent.setEditable(false);
notAfterComponent.setBorder(javax.swing.BorderFactory.createEtchedBorder());
notAfterComponent.setName("notAfter");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 5;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
gridBagConstraints.insets = new java.awt.Insets(2, 0, 2, 0);
add(notAfterComponent, gridBagConstraints);
issuerLabel.setLabelFor(issuerComponent);
issuerLabel.setText(Resources.getString("LicensePanel.issuer.label")); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 6;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.NORTHWEST;
gridBagConstraints.insets = new java.awt.Insets(2, 0, 2, 0);
add(issuerLabel, gridBagConstraints);
issuerScrollPane.setBorder(javax.swing.BorderFactory.createEtchedBorder());
issuerScrollPane.setHorizontalScrollBarPolicy(javax.swing.ScrollPaneConstants.HORIZONTAL_SCROLLBAR_NEVER);
issuerScrollPane.setPreferredSize(new java.awt.Dimension(300, 65));
issuerComponent.setEditable(false);
issuerComponent.setLineWrap(true);
issuerComponent.setWrapStyleWord(true);
issuerComponent.setBorder(null);
issuerComponent.setName("issuer");
issuerScrollPane.setViewportView(issuerComponent);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 6;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.weighty = 1.0;
gridBagConstraints.insets = new java.awt.Insets(2, 0, 2, 0);
add(issuerScrollPane, gridBagConstraints);
issuedLabel.setLabelFor(issuedComponent);
issuedLabel.setText(Resources.getString("LicensePanel.issued.label")); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 7;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.NORTHWEST;
gridBagConstraints.insets = new java.awt.Insets(2, 0, 2, 0);
add(issuedLabel, gridBagConstraints);
issuedComponent.setEditable(false);
issuedComponent.setBorder(javax.swing.BorderFactory.createEtchedBorder());
issuedComponent.setName("issued");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 7;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
gridBagConstraints.insets = new java.awt.Insets(2, 0, 2, 0);
add(issuedComponent, gridBagConstraints);
infoLabel.setLabelFor(infoComponent);
infoLabel.setText(Resources.getString("LicensePanel.info.label")); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 8;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.NORTHWEST;
gridBagConstraints.insets = new java.awt.Insets(2, 0, 0, 0);
add(infoLabel, gridBagConstraints);
infoScrollPane.setBorder(javax.swing.BorderFactory.createEtchedBorder());
infoScrollPane.setHorizontalScrollBarPolicy(javax.swing.ScrollPaneConstants.HORIZONTAL_SCROLLBAR_NEVER);
infoScrollPane.setPreferredSize(new java.awt.Dimension(300, 65));
infoComponent.setEditable(false);
infoComponent.setLineWrap(true);
infoComponent.setWrapStyleWord(true);
infoComponent.setBorder(null);
infoComponent.setName("info");
infoScrollPane.setViewportView(infoComponent);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 8;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.weighty = 1.0;
gridBagConstraints.insets = new java.awt.Insets(2, 0, 0, 0);
add(infoScrollPane, gridBagConstraints);
}// //GEN-END:initComponents
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JTextArea consumerComponent;
private javax.swing.JLabel consumerLabel;
private javax.swing.JTextArea holderComponent;
private javax.swing.JLabel holderLabel;
private javax.swing.JScrollPane holderScrollPane;
private javax.swing.JTextArea infoComponent;
private javax.swing.JLabel infoLabel;
private javax.swing.JScrollPane infoScrollPane;
private javax.swing.JTextArea issuedComponent;
private javax.swing.JLabel issuedLabel;
private javax.swing.JTextArea issuerComponent;
private javax.swing.JLabel issuerLabel;
private javax.swing.JScrollPane issuerScrollPane;
private javax.swing.JTextArea notAfterComponent;
private javax.swing.JLabel notAfterLabel;
private javax.swing.JTextArea notBeforeComponent;
private javax.swing.JLabel notBeforeLabel;
private javax.swing.JTextArea subjectComponent;
private javax.swing.JLabel subjectLabel;
// End of variables declaration//GEN-END:variables
public static class Descriptor extends WizardPanelDescriptor {
public static final String IDENTIFIER = "LICENSE_PANEL"; // NOI18N
public Descriptor(LicenseManager manager) {
super(IDENTIFIER, new LicensePanel(manager));
}
public Object getNextPanelDescriptor() {
return FINISH;
}
public Object getBackPanelDescriptor() {
return WelcomePanel.Descriptor.IDENTIFIER;
}
public void aboutToDisplayPanel() {
LicensePanel panel = (LicensePanel)getPanelComponent();
try {
panel.verify();
} catch (final Exception ex) {
Dialogs.showMessageDialog(
panel,
ex.getLocalizedMessage(),
Resources.getString("LicensePanel.failure.title"),
Dialogs.ERROR_MESSAGE);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy