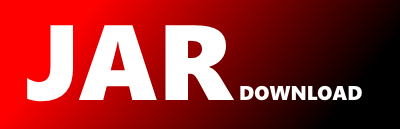
de.schlichtherle.truezip.fs.http.HttpController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truezip-driver-http Show documentation
Show all versions of truezip-driver-http Show documentation
The file system driver for the HTTP(S) scheme.
Add the JAR artifact of this module to the compile-time class path for
dependency injection to the TrueZIP Kernel module or to the run-time
class path for service location in the TrueZIP File* module.
The newest version!
/*
* Copyright (C) 2005-2015 Schlichtherle IT Services.
* All rights reserved. Use is subject to license terms.
*/
package de.schlichtherle.truezip.fs.http;
import de.schlichtherle.truezip.entry.Entry;
import de.schlichtherle.truezip.entry.Entry.Access;
import de.schlichtherle.truezip.entry.Entry.Type;
import static de.schlichtherle.truezip.entry.Entry.Type.FILE;
import de.schlichtherle.truezip.fs.*;
import de.schlichtherle.truezip.socket.IOPool;
import de.schlichtherle.truezip.socket.InputSocket;
import de.schlichtherle.truezip.socket.OutputSocket;
import de.schlichtherle.truezip.util.BitField;
import java.io.IOException;
import javax.annotation.CheckForNull;
import javax.annotation.concurrent.Immutable;
import org.apache.http.HttpResponse;
/**
* A file system controller for the HTTP(S) schemes.
*
* @author Christian Schlichtherle
*/
@Immutable
public class HttpController extends FsAbstractController {
private final HttpDriver driver;
HttpController(final HttpDriver driver, final FsModel model) {
super(model);
if (null != model.getParent())
throw new IllegalArgumentException();
assert null != driver;
this.driver = driver;
}
final IOPool> getPool() {
return driver.getPool();
}
final HttpResponse executeHead(HttpEntry entry) throws IOException {
return driver.executeHead(entry);
}
final HttpResponse executeGet(HttpEntry entry) throws IOException {
return driver.executeGet(entry);
}
protected HttpEntry newEntry(FsEntryName name) {
return new HttpEntry(this, name);
}
final FsPath resolve(FsEntryName name) {
return getMountPoint().resolve(name);
}
@Override
public FsController> getParent() {
return null;
}
@Override
public boolean isReadOnly() throws IOException {
return false;
}
@Override
public HttpEntry getEntry(FsEntryName name) throws IOException {
HttpEntry entry = newEntry(name);
return entry.isType(FILE) ? entry : null;
}
@Override
public boolean isReadable(FsEntryName name) throws IOException {
return null != getEntry(name);
}
@Override
public boolean isWritable(FsEntryName name) throws IOException {
return false;
}
@Override
public void setReadOnly(FsEntryName name) throws IOException {
}
@Override
public boolean setTime(
FsEntryName name,
BitField types,
long value,
BitField options)
throws IOException {
throw new ReadOnlyFileSystemTypeException();
}
@Override
public InputSocket> getInputSocket(
FsEntryName name,
BitField options) {
return newEntry(name).newInputSocket(options);
}
@Override
public OutputSocket> getOutputSocket(
FsEntryName name,
BitField options,
@CheckForNull Entry template) {
return newEntry(name).newOutputSocket(options, template);
}
@Override
public void mknod( final FsEntryName name,
final Type type,
final BitField options,
final @CheckForNull Entry template)
throws IOException {
throw new ReadOnlyFileSystemTypeException();
}
@Override
public void unlink(FsEntryName name, BitField options)
throws IOException {
throw new ReadOnlyFileSystemTypeException();
}
@Override
public void sync(final BitField options) {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy