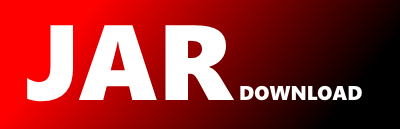
de.schlichtherle.truezip.fs.archive.tar.TarGZipDriver Maven / Gradle / Ivy
Show all versions of truezip-driver-tar Show documentation
/*
* Copyright (C) 2005-2013 Schlichtherle IT Services.
* All rights reserved. Use is subject to license terms.
*/
package de.schlichtherle.truezip.fs.archive.tar;
import de.schlichtherle.truezip.entry.Entry;
import de.schlichtherle.truezip.fs.FsController;
import de.schlichtherle.truezip.fs.FsEntryName;
import de.schlichtherle.truezip.fs.FsModel;
import de.schlichtherle.truezip.fs.FsOutputOption;
import static de.schlichtherle.truezip.fs.FsOutputOption.STORE;
import de.schlichtherle.truezip.io.Streams;
import de.schlichtherle.truezip.socket.IOPoolProvider;
import de.schlichtherle.truezip.socket.OutputSocket;
import de.schlichtherle.truezip.util.BitField;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.zip.Deflater;
import java.util.zip.GZIPInputStream;
import javax.annotation.CheckForNull;
import javax.annotation.concurrent.Immutable;
/**
* An archive driver for GZIP compressed TAR files (TAR.GZIP).
*
* Subclasses must be thread-safe and should be immutable!
*
* @author Christian Schlichtherle
*/
@Immutable
public class TarGZipDriver extends TarDriver {
public TarGZipDriver(IOPoolProvider provider) {
super(provider);
}
/**
* The buffer size used for reading and writing.
* Optimized for performance.
*/
public static final int BUFFER_SIZE = Streams.BUFFER_SIZE;
/**
* Returns the size of the I/O buffer.
*
* The implementation in the class {@link TarGZipDriver} returns
* {@link #BUFFER_SIZE}.
*
* @return The size of the I/O buffer.
*/
public int getBufferSize() {
return BUFFER_SIZE;
}
/**
* Returns the compression level to use when writing a GZIP output stream.
*
* The implementation in the class {@link TarBZip2Driver} returns
* {@link Deflater#BEST_COMPRESSION}.
*
* @return The compression level to use when writing a GZIP output stream.
*/
public int getLevel() {
return Deflater.BEST_COMPRESSION;
}
/**
* Sets {@link FsOutputOption#STORE} in {@code options} before
* forwarding the call to {@code controller}.
*/
@Override
public OutputSocket> getOutputSocket( FsController> controller,
FsEntryName name,
BitField options,
@CheckForNull Entry template) {
return controller.getOutputSocket(name, options.set(STORE), template);
}
@Override
protected TarInputShop newTarInputShop(FsModel model, InputStream in)
throws IOException {
return super.newTarInputShop(model,
new GZIPInputStream(in, getBufferSize()));
}
@Override
protected TarOutputShop newTarOutputShop(
final FsModel model,
final OutputStream out,
final TarInputShop source)
throws IOException {
return super.newTarOutputShop(model,
new GZIPOutputStream(out, getBufferSize(), getLevel()),
source);
}
/** Extends its super class to set the deflater level. */
private static final class GZIPOutputStream
extends java.util.zip.GZIPOutputStream {
GZIPOutputStream(OutputStream out, int size, int level)
throws IOException {
super(out, size);
def.setLevel(level);
}
} // GZIPOutputStream
}