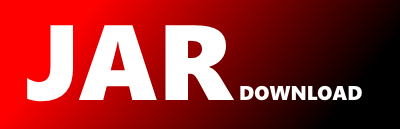
de.schlichtherle.truezip.key.KeyManager Maven / Gradle / Ivy
Show all versions of truezip-driver-zip Show documentation
/*
* Copyright (C) 2005-2013 Schlichtherle IT Services.
* All rights reserved. Use is subject to license terms.
*/
package de.schlichtherle.truezip.key;
import javax.annotation.CheckForNull;
import javax.annotation.Nullable;
import java.net.URI;
import javax.annotation.concurrent.ThreadSafe;
/**
* A container for key providers for reading and writing protected resources.
*
* Implementations must be safe for multi-threading.
*
* @param The type of the secret keys.
* @author Christian Schlichtherle
*/
@ThreadSafe
public interface KeyManager {
/**
* Returns the mapped key provider for the given protected resource.
* If no key provider is mapped yet, a new key provider is created and
* returned.
*
* @param resource the URI of the protected resource.
* @return The key provider mapped for the protected resource.
*/
KeyProvider getKeyProvider(URI resource);
/**
* Moves the mapped key provider from the URI {@code oldResource} to
* {@code newResource}.
*
* @param oldResource the old URI of the protected resource.
* @param newResource the new URI of the protected resource.
* @return The key provider which was previously mapped for the protected
* resource {@code newResource}.
* @throws IllegalArgumentException if {@code oldResource} compares
* {@link URI#equals(Object) equal} to {@code newResource}.
*/
@Nullable KeyProvider moveKeyProvider(URI oldResource, URI newResource);
/**
* Removes the mapped key provider for the given protected resource.
* It is an error to use the returned key provider.
*
* @param resource the URI of the protected resource.
* @return The key provider which was previously mapped for the protected
* resource.
*/
@CheckForNull KeyProvider removeKeyProvider(URI resource);
/**
* Returns a priority to help the key manager service locator.
* The greater number wins!
* The default value should be {@code 0}.
*
* @return A priority to help the key manager service locator.
*/
public int getPriority();
}