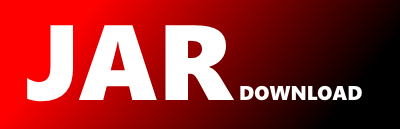
de.schlichtherle.truezip.key.PromptingKeyManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truezip-driver-zip Show documentation
Show all versions of truezip-driver-zip Show documentation
The file system driver family for ZIP and related archive file types.
Add the JAR artifact of this module to the run time class path to
make its file system drivers available for service location in the
client API modules.
/*
* Copyright (C) 2005-2013 Schlichtherle IT Services.
* All rights reserved. Use is subject to license terms.
*/
package de.schlichtherle.truezip.key;
import de.schlichtherle.truezip.key.PromptingKeyProvider.View;
import java.net.URI;
import javax.annotation.Nullable;
import javax.annotation.concurrent.ThreadSafe;
/**
* A key manager which prompts the user for a key if required.
*
* @param The type of the keys.
* @see PromptingKeyProvider
* @author Christian Schlichtherle
*/
@ThreadSafe
public final class PromptingKeyManager>
extends SafeKeyManager> {
private final View view;
/**
* Constructs a new prompting key manager.
*
* @param view the view instance for prompting for keys.
*/
public PromptingKeyManager(final View view) {
if (null == view)
throw new NullPointerException();
this.view = view;
}
final View getView() {
return view;
}
/**
* Returns a new prompting key provider.
*
* @return A new prompting key provider.
* @since TrueZIP 7.2
*/
@Override
protected PromptingKeyProvider newKeyProvider() {
return new PromptingKeyProvider(this);
}
@Override
public synchronized PromptingKeyProvider getKeyProvider(URI resource) {
final PromptingKeyProvider provider = super.getKeyProvider(resource);
provider.setResource(resource);
return provider;
}
@Override
public synchronized @Nullable PromptingKeyProvider getMappedKeyProvider(URI resource) {
final PromptingKeyProvider provider = super.getMappedKeyProvider(resource);
if (null != provider)
provider.setResource(resource);
return provider;
}
@Override
public synchronized PromptingKeyProvider moveKeyProvider(URI oldResource, URI newResource) {
final PromptingKeyProvider
oldProvider = super.moveKeyProvider(oldResource, newResource);
if (null != oldProvider)
oldProvider.setResource(null);
final PromptingKeyProvider
newProvider = super.getMappedKeyProvider(newResource);
if (null != newProvider)
newProvider.setResource(newResource);
return oldProvider;
}
@Override
public synchronized PromptingKeyProvider removeKeyProvider(URI resource) {
final PromptingKeyProvider
provider = super.removeKeyProvider(resource);
if (null != provider)
provider.setResource(null);
return provider;
}
/**
* Returns a string representation of this object for debugging and logging
* purposes.
*/
@Override
public String toString() {
return String.format("%s[view=%s, priority=%d]",
getClass().getName(),
getView(),
getPriority());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy