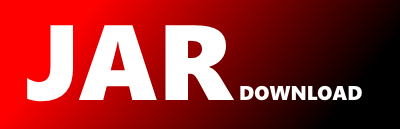
de.sciss.synth.Model.scala Maven / Gradle / Ivy
/*
* Model.scala
* (ScalaCollider)
*
* Copyright (c) 2008-2012 Hanns Holger Rutz. All rights reserved.
*
* This software is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either
* version 2, june 1991 of the License, or (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License (gpl.txt) along with this software; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
*
* For further information, please contact Hanns Holger Rutz at
* [email protected]
*/
package de.sciss.synth
import collection.immutable.Queue
/**
* A Model implements the publish-subscribe pattern for
* generic (untyped) messages. Observers subscribe by
* calling addListener
with an appropriate matcher
* function. When the Model dispatches a message, it
* invokes the apply method of all observers who are
* defined for the given message. Dispatching is
* synchronous, but exceptions are caught.
*/
object Model {
type Listener = PartialFunction[ AnyRef, Unit ]
object EmptyListener extends Listener {
def isDefinedAt( v: AnyRef ) = false
def apply( v: AnyRef ) { throw new MatchError( v )}
}
}
trait Model {
import Model._
private var listeners = Queue.empty[ Listener ]
private val sync = new AnyRef
protected def dispatch( change: AnyRef ) {
listeners foreach { l =>
val t1 = System.currentTimeMillis
try {
if( l.isDefinedAt( change )) l( change )
} catch {
case e: Throwable => e.printStackTrace() // catch, but print
} finally {
val t2 = System.currentTimeMillis
if( (t2 - t1) > 2000 ) println( "" + new java.util.Date() + " WOW listener took long (" + (t2-t1) + ") : " +
change + " -> " + l )
}
}
}
def addListener( l: Listener ) : Listener = {
sync.synchronized {
listeners = listeners.enqueue( l )
}
l
}
def removeListener( l: Listener ) : Listener = {
sync.synchronized {
// multi set diff just removes one instance --
// observers could register more than once if they want
listeners = listeners.diff( List( l ))
}
l
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy