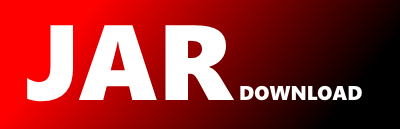
com.alee.utils.CollectionUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of weblaf-core Show documentation
Show all versions of weblaf-core Show documentation
Core components for WebLaf
/*
* This file is part of WebLookAndFeel library.
*
* WebLookAndFeel library is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* WebLookAndFeel library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with WebLookAndFeel library. If not, see .
*/
package com.alee.utils;
import com.alee.utils.collection.IndexedSupplier;
import com.alee.utils.compare.Filter;
import com.alee.utils.text.TextProvider;
import java.util.*;
/**
* This class provides a set of utilities to work with collections.
*
* @author Mikle Garin
*/
public final class CollectionUtils
{
/**
* Returns whether specified collection is empty or not.
*
* @param collection collection to process
* @return true if specified collection is empty, false otherwise
*/
public static boolean isEmpty ( final Collection collection )
{
return collection == null || collection.isEmpty ();
}
/**
* Returns list with limited amount of objects from the initial list.
* Only specified amount of first objects in initial list will be transferred to new list.
*
* @param list initial list
* @param limit objects amount limitation
* @param object type
* @return list with limited amount of objects from the initial list
*/
public static List limit ( final List list, final int limit )
{
return list.size () <= limit ? list : copySubList ( list, 0, limit );
}
/**
* Returns sub list with copied values.
*
* @param list source list
* @param fromIndex start index
* @param toIndex end index
* @param data type
* @return sub list with copied values
*/
public static ArrayList copySubList ( final List list, final int fromIndex, final int toIndex )
{
return new ArrayList ( list.subList ( fromIndex, toIndex ) );
}
/**
* Returns data converted into list.
*
* @param data data
* @param data type
* @return data list
*/
public static ArrayList asList ( final T... data )
{
final ArrayList list = new ArrayList ( data.length );
Collections.addAll ( list, data );
return list;
}
/**
* Returns data converted into list.
*
* @param data data
* @param data type
* @return data list
*/
public static ArrayList asList ( final Iterator data )
{
final ArrayList list = new ArrayList ();
while ( data.hasNext () )
{
list.add ( data.next () );
}
return list;
}
/**
* Returns non-null data converted into list.
*
* @param data data
* @param data type
* @return non-null data list
*/
public static ArrayList asNonNullList ( final T... data )
{
final ArrayList list = new ArrayList ( data.length );
if ( data != null )
{
for ( final T object : data )
{
if ( object != null )
{
list.add ( object );
}
}
}
return list;
}
/**
* Adds all objects into the specified list.
*
* @param collection list to fill
* @param objects objects
* @param objects type
* @return true if list changed as the result of this operation, false otherwise
*/
public static boolean addAll ( final Collection collection, final T... objects )
{
boolean result = false;
for ( final T object : objects )
{
if ( !collection.contains ( object ) )
{
result |= collection.add ( object );
}
}
return result;
}
/**
* Adds all non-null objects into the specified list.
*
* @param collection list to fill
* @param objects objects
* @param objects type
* @return true if list changed as the result of this operation, false otherwise
*/
public static boolean addAllNonNull ( final Collection collection, final T... objects )
{
boolean result = false;
for ( final T object : objects )
{
if ( !collection.contains ( object ) && object != null )
{
result |= collection.add ( object );
}
}
return result;
}
/**
* Adds all objects into the specified list.
*
* @param collection list to fill
* @param objects objects
* @param objects type
* @return true if list changed as the result of this operation, false otherwise
*/
public static boolean addAll ( final Collection collection, final Collection objects )
{
boolean result = false;
for ( final T object : objects )
{
if ( !collection.contains ( object ) )
{
result |= collection.add ( object );
}
}
return result;
}
/**
* Adds all non-null objects into the specified list.
*
* @param collection list to fill
* @param objects objects
* @param objects type
* @return true if list changed as the result of this operation, false otherwise
*/
public static boolean addAllNonNull ( final Collection collection, final Collection objects )
{
boolean result = false;
for ( final T object : objects )
{
if ( !collection.contains ( object ) && object != null )
{
result |= collection.add ( object );
}
}
return result;
}
/**
* Removes all objects from the specified list.
*
* @param collection list to fill
* @param objects objects
* @param objects type
* @return true if list changed as the result of this operation, false otherwise
*/
public static boolean removeAll ( final Collection collection, final T... objects )
{
boolean result = false;
for ( final T object : objects )
{
result |= collection.remove ( object );
}
return result;
}
/**
* Returns collection that contains elements from all specified collections.
* Order in which collection are provided will be preserved.
*
* @param collections collections to join
* @param collection type
* @return collection that contains elements from all specified collections
*/
public static ArrayList join ( final Collection... collections )
{
// Calculating final collection size
int size = 0;
if ( collections != null )
{
for ( final Collection collection : collections )
{
size += collection != null ? collection.size () : 0;
}
}
// Creating joined collection
final ArrayList list = new ArrayList ( size );
if ( collections != null )
{
for ( final Collection collection : collections )
{
if ( !isEmpty ( collection ) )
{
list.addAll ( collection );
}
}
}
return list;
}
/**
* Returns copy of the specified list.
* Note that this method will copy same list values into the new list.
*
* @param collection list to copy
* @param list type
* @return copy of the specified list
*/
public static ArrayList copy ( final Collection collection )
{
if ( collection == null )
{
return null;
}
return new ArrayList ( collection );
}
/**
* Removes all null elements from list.
*
* @param list list to refactor
* @param list type
* @return refactored list
*/
public static List removeNulls ( final List list )
{
if ( list == null )
{
return null;
}
for ( int i = list.size () - 1; i >= 0; i-- )
{
if ( list.get ( i ) == null )
{
list.remove ( i );
}
}
return list;
}
/**
* Returns whether lists are equal or not.
*
* @param list1 first list
* @param list2 second list
* @return true if lists are equal, false otherwise
*/
public static boolean equals ( final List list1, final List list2 )
{
if ( list1 == null && list2 == null )
{
return true;
}
else if ( ( list1 == null || list2 == null ) && list1 != list2 )
{
return false;
}
else
{
if ( list1.size () != list2.size () )
{
return false;
}
else
{
for ( final Object object : list1 )
{
if ( !list2.contains ( object ) )
{
return false;
}
}
return true;
}
}
}
/**
* Returns list of strings extracted from the specified elements list.
*
* @param list elements list
* @param textProvider text provider
* @param elements type
* @return list of strings extracted from the specified elements list
*/
public static ArrayList toStringList ( final List list, final TextProvider textProvider )
{
final ArrayList stringList = new ArrayList ( list.size () );
for ( final T element : list )
{
stringList.add ( textProvider.getText ( element ) );
}
return stringList;
}
/**
* Returns an int array created using Integer list.
*
* @param list Integer list
* @return int array
*/
public static int[] toArray ( final List list )
{
final int[] array = new int[ list.size () ];
for ( int i = 0; i < list.size (); i++ )
{
final Integer integer = list.get ( i );
array[ i ] = integer != null ? integer : 0;
}
return array;
}
/**
* Returns a list of objects converted from array.
*
* @param array data array
* @param data type
* @return data list
*/
public static ArrayList toList ( final T[] array )
{
final ArrayList list = new ArrayList ( array.length );
Collections.addAll ( list, array );
return list;
}
/**
* Returns a list of objects converted from deque.
*
* @param deque data deque
* @param data type
* @return data list
*/
public static ArrayList toList ( final Deque deque )
{
return new ArrayList ( deque );
}
/**
* Returns a vector of objects converted from collection.
*
* @param collection data collection
* @param data type
* @return a vector of objects converted from collection
*/
public static Vector toVector ( final Collection collection )
{
final Vector vector = new Vector ( collection.size () );
for ( final T element : collection )
{
vector.add ( element );
}
return vector;
}
/**
* Returns list of elements filtered from collection.
*
* @param collection collection to filter
* @param filter filter to process
* @param elements type
* @return list of elements filtered from collection
*/
public static ArrayList filter ( final Collection collection, final Filter filter )
{
final ArrayList filtered = new ArrayList ( collection.size () );
for ( final T element : collection )
{
if ( filter.accept ( element ) )
{
filtered.add ( element );
}
}
return filtered;
}
/**
* Returns map keys list.
*
* @param map map to process
* @param key object type
* @param value object type
* @return map keys list
*/
public static ArrayList keysList ( final Map map )
{
return new ArrayList ( map.keySet () );
}
/**
* Returns map values list.
*
* @param map map to process
* @param key object type
* @param value object type
* @return map values list
*/
public static ArrayList valuesList ( final Map map )
{
return new ArrayList ( map.values () );
}
/**
* Returns map values summary list with unique elements only.
*
* @param map map to process
* @param key object type
* @param value object type
* @return map values summary list with unique elements only
*/
public static ArrayList valuesSummaryList ( final Map> map )
{
final ArrayList summary = new ArrayList ( 0 );
for ( final Map.Entry> entry : map.entrySet () )
{
final List list = entry.getValue ();
summary.ensureCapacity ( summary.size () + list.size () );
for ( final V value : list )
{
if ( !summary.contains ( value ) )
{
summary.add ( value );
}
}
}
return summary;
}
/**
* Fills and returns list with data provided by supplier interface implementation.
*
* @param amount amount of list elements
* @param supplier data provider
* @param data type
* @return list filled with data provided by supplier interface implementation
*/
public static List fillList ( final int amount, final IndexedSupplier supplier )
{
final List list = new ArrayList ( amount );
for ( int i = 0; i < amount; i++ )
{
list.add ( supplier.get ( i ) );
}
return list;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy