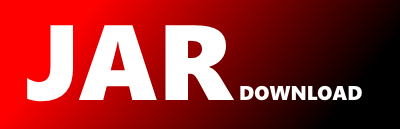
template.ObjectManager_java.txt Maven / Gradle / Ivy
/* Source generated by codegenj (https://github.com/olir/codegenj) - DO NOT EDIT!
*
* https://github.com/olir/codegenj
*
* This generated output is free and unencumbered software released into the public domain.
*
* Anyone is free to copy, modify, publish, use, compile, sell, or
* distribute this software, either in source code form or as a compiled
* binary, for any purpose, commercial or non-commercial, and by any
* means.
*
* In jurisdictions that recognize copyright laws, the author or authors
* of this software dedicate any and all copyright interest in the
* software to the public domain. We make this dedication for the benefit
* of the public at large and to the detriment of our heirs and
* successors. We intend this dedication to be an overt act of
* relinquishment in perpetuity of all present and future rights to this
* software under copyright law.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
* IN NO EVENT SHALL THE AUTHORS BE LIABLE FOR ANY CLAIM, DAMAGES OR
* OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE,
* ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
* OTHER DEALINGS IN THE SOFTWARE.
*
* For more information, please refer to
*/
package de.serviceflow.codegenj;
import java.util.ArrayList;
import java.util.List;
import java.util.HashMap;
import java.util.Map;
import java.util.Collections;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* D-Bus JNI Interface class for ObjectManager
*/
public class ObjectManager {
static {
System.loadLibrary("$$$library$$$"); // Load native library lib$$$library$$$.so at runtime
}
private static ObjectManager instance = null;
private final static Logger LOGGER = Logger.getLogger(ObjectManager.class.getName());
public static ObjectManager getInstance() {
synchronized(ObjectManager.class) {
if (instance==null) {
new ObjectManager();
}
}
return instance;
}
public final static Logger getLogger() {
return LOGGER;
}
/**
* for JNI: <=500 (FINE) triggers logging
*/
private final static int getLogLevelIntValue() {
Logger l = LOGGER;
do {
Level level = l.getLevel();
if (level!=null) {
return level.intValue();
}
l = l.getParent();
} while(l!=null);
return Integer.MAX_VALUE;
}
private Object _client;
private final String objectpath;
private ObjectManager() {
this("/");
instance = this;
initCollectorAPI();
_run();
}
protected ObjectManager(String objectpath) {
if (objectpath.length()<=1 && objectpath.charAt(0)=='/') {
if (instance!=null)
throw new Error("Use getInstance() to obtain System root instance.");
}
if (objectpath.charAt(objectpath.length()-1)!='/') {
objectpath = objectpath + "/";
}
_init(objectpath);
this.objectpath = objectpath;
if (getLogger().isLoggable(Level.FINER))
System.out.println("*** ObjectManager : Direct Buffer with NativeReference : "+ _client);
}
private native void _init(String objectpath);
private native void _run();
public native void _destroy();
public String getObjectPath() {
return objectpath;
}
public native Object [] getProxyObjects(String interfacename, boolean initial);
public native String getObjectPath(Object proxy);
public native void dump();
###for de.serviceflow.codegenj.CollectorAPI###
static private Map $$$vname$$$Map = null;
static public void add$$$cname$$$Object(String path, Object proxy) {
$$$interfacename$$$ n = new $$$interfacename$$$(proxy);
if (path==null)
path = ObjectManager.getInstance().getObjectPath(proxy);
$$$vname$$$Map.put(path, n);
n.setObjectPath(path);
}
static public void remove$$$cname$$$Object(String path, Object proxy) {
if (path==null)
path = ObjectManager.getInstance().getObjectPath(proxy);
$$$interfacename$$$ n = $$$vname$$$Map.get(path);
n._destroy();
$$$vname$$$Map.remove(path);
}
static public void initialize$$$cname$$$Mapping() {
$$$vname$$$Map = Collections.synchronizedMap(new HashMap());
if ($$$opmode$$$) {
Object [] newProxies = ObjectManager.getInstance().getProxyObjects("$$$interfacename$$$", $$$opmode$$$);
for (Object proxy : newProxies) {
add$$$cname$$$Object(null, proxy);
}
System.out.println("*** $$$vname$$$Map initialized, count="+(newProxies.length));
}
}
public List<$$$interfacename$$$> $$$mname$$$() {
if (!$$$opmode$$$) {
$$$vname$$$Map.clear();
Object [] newProxies = ObjectManager.getInstance().getProxyObjects("$$$interfacename$$$", $$$opmode$$$);
for (Object proxy : newProxies) {
add$$$cname$$$Object(null, proxy);
}
System.out.println("*** $$$vname$$$Map initialized, count="+(newProxies.length));
}
List<$$$interfacename$$$> resultList = new ArrayList<$$$interfacename$$$>();
String prefix = objectpath;
if (prefix.length()>0 && prefix.charAt(prefix.length()-1)!='/') {
prefix = prefix + '/';
}
for (Map.Entry entry : $$$vname$$$Map.entrySet()) {
String key = entry.getKey();
if (key.startsWith(prefix)) {
resultList.add(entry.getValue());
}
}
return resultList;
}
###end###
public void initCollectorAPI() {
System.out.println("*** initCollectorAPI() start");
$$$api.init$$$
System.out.println("*** initCollectorAPI() end");
}
public void addObjectToCollection(String objectpath, String interfaceName, Object proxy) {
// System.out.println(" ~ addObjectToCollection("+interfaceName+")");
$$$api.add$$$
}
public void removeObjectFromCollection(String objectpath, String interfaceName, Object proxy) {
$$$api.remove$$$
}
static private List objectManagerSignalListeners =
Collections.synchronizedList(new ArrayList());
static protected void dispatchObjectManagerSignal(String signalname, String objectpath, String interfaceName, Object proxy) {
if ("object-added".equals(signalname)) {
for (ObjectManagerSignalListener l : objectManagerSignalListeners) {
l.objectAdded( getInstance(), objectpath);
}
}
else
if ("object-removed".equals(signalname)) {
for (ObjectManagerSignalListener l : objectManagerSignalListeners) {
l.objectRemoved( getInstance(), objectpath);
}
}
else
if ("interface-added".equals(signalname)) {
getInstance().addObjectToCollection(objectpath, interfaceName, proxy);
for (ObjectManagerSignalListener l : objectManagerSignalListeners) {
l.interfaceAdded( getInstance(), objectpath, interfaceName);
}
}
else
if ("interface-removed".equals(signalname)) {
for (ObjectManagerSignalListener l : objectManagerSignalListeners) {
l.interfaceRemoved( getInstance(), objectpath, interfaceName);
}
getInstance().removeObjectFromCollection(objectpath, interfaceName, proxy);
}
}
public void addObjectManagerSignalListener(ObjectManagerSignalListener l) {
objectManagerSignalListeners.add(l);
}
public void removeObjectManagerSignalListener(ObjectManagerSignalListener l) {
objectManagerSignalListeners.remove(l);
}
public interface ObjectManagerSignalListener {
void interfaceAdded(ObjectManager m, String op, String i);
void interfaceRemoved(ObjectManager m, String op, String i);
void objectAdded(ObjectManager m, String op);
void objectRemoved(ObjectManager m, String op);
}
public static class DBusInterface {
private String objectpath;
protected DBusInterface() {
}
public String getObjectPath() {
return objectpath;
}
public void setObjectPath(String path) {
objectpath = path;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy