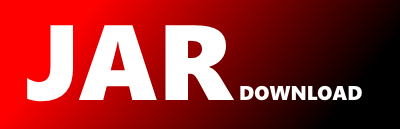
template.skeleton_c.txt Maven / Gradle / Ivy
/* Source generated by codegenj (https://github.com/olir/codegenj) - DO NOT EDIT!
*
* https://github.com/olir/codegenj
*
* This generated output is free and unencumbered software released into the public domain.
*
* Anyone is free to copy, modify, publish, use, compile, sell, or
* distribute this software, either in source code form or as a compiled
* binary, for any purpose, commercial or non-commercial, and by any
* means.
*
* In jurisdictions that recognize copyright laws, the author or authors
* of this software dedicate any and all copyright interest in the
* software to the public domain. We make this dedication for the benefit
* of the public at large and to the detriment of our heirs and
* successors. We intend this dedication to be an overt act of
* relinquishment in perpetuity of all present and future rights to this
* software under copyright law.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
* IN NO EVENT SHALL THE AUTHORS BE LIABLE FOR ANY CLAIM, DAMAGES OR
* OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE,
* ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
* OTHER DEALINGS IN THE SOFTWARE.
*
* For more information, please refer to
*/
#include
#include
#include "$$$interface.jname$$$.h"
#include "$$$library$$$-codegen.h"
// from olir/codegenj/ObjectManager.c
JNIEnv *attachCurrentThread();
void detachCurrentThread();
struct NativeReferenceStructure;
typedef struct NativeReferenceStructure {
GObject *value;
} NativeReference;
GThread *export_thread;
GMainLoop *export_loop;
struct ExportThreadDataStructure;
typedef struct ExportThreadDataStructure {
// $$$interface.uname$$$ *gskeleton;
jobject *javaInstance;
const gchar *gpath;
} ExportThreadData;
/*
##for method##
void on_handle_$$$interface.jname$$$_$$$method.jname$$$ ($$$interface.uname$$$ *skeleton,
GDBusObject *object, gpointer user_data) {
jobject *thisObj = (jobject *)user_data;
g_print (" * on_handle_$$$interface.jname$$$_$$$method.jname$$$() called.\n");
JNIEnv *env = attachCurrentThread();
// return forward_$$$interface.jname$$$_$$$method.jname$$$($$$method.skeleton.jniparams$$$);
// return error_message(conn, msg,
// "org.bluez.Error.Rejected", "???");
detachCurrentThread();
}
##end##
*/
static GVariant *
handle_get_property (GDBusConnection *connection,
const gchar *sender,
const gchar *object_path,
const gchar *interface_name,
const gchar *property_name,
GError **error,
gpointer user_data) {
return NULL;
}
static gboolean
handle_set_property (GDBusConnection *connection,
const gchar *sender,
const gchar *object_path,
const gchar *interface_name,
const gchar *property_name,
GVariant *value,
GError **error,
gpointer user_data) {
return TRUE;
}
static void
handle_method_call (GDBusConnection *connection,
const gchar *sender,
const gchar *object_path,
const gchar *interface_name,
const gchar *method_name,
GVariant *parameters,
GDBusMethodInvocation *invocation,
gpointer data) {
// NEVER CALLED - BUG !?
g_print ( " ++ handle_method_call: %s\n", method_name );
jobject *callbackObj = ((ExportThreadData *) data)->javaInstance;
JNIEnv *env = attachCurrentThread();
// TODO: lookup and call java methods related to method_name within new thread, deliver return value async
// jclass cls = (*env)->FindClass(env, "olir/codegenj/ObjectManager"); - todo generate class name
//jmethodID mid = (*env)->GetStaticMethodID(env, cls, "dispatchObjectManagerSignal",
// "(Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;Ljava/lang/Object;)V");
// (*env)->CallVoidMethod(env, cls, mid, _signalname, _objectpath, NULL, NULL); -- use callbackObj
detachCurrentThread();
g_dbus_method_invocation_return_value (invocation, NULL);
}
static const GDBusInterfaceVTable interface_vtable =
{
handle_method_call,
handle_get_property,
handle_set_property
};
gpointer exit_export_thread (void *data) {
g_main_loop_quit (export_loop);
export_thread = NULL;
return NULL;
}
void alert() {
g_print ( "+++ ALERT +++\n" );
}
void on_bus_acquired(GDBusConnection *connection, const gchar *name, gpointer data) {
if (connection==NULL) {
g_print ( "ERROR: on_bus_acquired(): no connection\n" );
return;
}
// register objects here
// $$$interface.uname$$$ *skeleton = ((ExportThreadData *) data)->gskeleton;
// jobject *callbackObj = ((ExportThreadData *) data)->javaInstance;
const gchar *_path = ((ExportThreadData *) data)->gpath;
g_print ( "Registering object at %s\n", _path );
GDBusInterfaceInfo *info = agent1_interface_info();
guint registration_id;
GError *_error = NULL;
###for method###
// g_signal_connect (skeleton, "handle-$$$method.sname$$$", G_CALLBACK (alert), data);
###end###
registration_id = g_dbus_connection_register_object (connection,
_path,
info, // introspection_data->interfaces[0],
&interface_vtable,
data, // user_data
NULL, // user_data_free_func
&_error);
if (registration_id<=0) {
g_print ( "ERROR: on_bus_acquired(): g_dbus_connection_register_object() failed: %s\n", _error->message );
return;
}
/*
gboolean _success = g_dbus_interface_skeleton_export((GDBusInterfaceSkeleton *)skeleton, connection, _path, &_error);
if (!_success) {
g_print ( "ERROR: on_bus_acquired(): g_dbus_interface_skeleton_export() failed: %s\n", _error->message );
return;
}
*/
g_print ( "on_bus_acquired(): succeeded: %d\n", registration_id);
}
void on_name_acquired(GDBusConnection *connection, const gchar *name, gpointer data) {
g_print (" * name_acquired\n");
g_thread_new(NULL, exit_export_thread, NULL);
}
void on_name_lost(GDBusConnection *connection, const gchar *name, gpointer data) {
g_print (" * name_lost\n");
g_thread_new(NULL, exit_export_thread, NULL);
}
gpointer start_export_thread (void *data) {
export_loop = g_main_loop_new(NULL, FALSE);
g_bus_own_name (G_BUS_TYPE_SESSION, // G_BUS_TYPE_SYSTEM
"de.serviceflow.codegenj.$$$interface.uname$$$",
G_BUS_NAME_OWNER_FLAGS_ALLOW_REPLACEMENT, // G_BUS_NAME_OWNER_FLAGS_NONE,
on_bus_acquired,
on_name_acquired,
on_name_lost,
data,
NULL); // TODO: Add free function
g_print (" starting export loop...\n");
g_main_loop_run(export_loop);
return NULL;
}
JNIEXPORT jobject JNICALL Java_$$$interface.jname$$$__1init(JNIEnv *env, jobject thisObj, jstring path) {
// int handler_id;
//##for method##
// handler_id = g_signal_connect (skeleton, "$$$method.jname$$$", (GCallback) on_handle_$$$interface.jname$$$_$$$method.jname$$$, &thisObj);
// if (handler_id <= 0) {
// jclass _eClass = (*env)->FindClass(env, "java/io/IOException");
// (*env)->ThrowNew(env, _eClass, "Failed to connect signal handler for signal '$$$method.jname$$$'.");
// (*env)->DeleteLocalRef(env, _eClass);
// }
//##end##
const gchar *_path = (*env)->GetStringUTFChars(env, path, NULL);
/*
// $$$interface.uname$$$ *skeleton = $$$interface.cname$$$_skeleton_new();
GError *_error = NULL;
$$$interface.uname$$$ *skeleton = $$$interface.cname$$$_proxy_new_for_bus_sync (
G_BUS_TYPE_SYSTEM,
G_DBUS_PROXY_FLAGS_NONE,
"$$$busname$$$", // bus name
_path, // object path
NULL, // GCancellable*
&_error);
if (_error!=NULL) {
jclass _eClass = (*env)->FindClass(env, "java/lang/Error");
(*env)->ThrowNew(env, _eClass, _error->message);
(*env)->DeleteLocalRef(env, _eClass);
g_error_free(_error);
}
*/
// Start export Thread
ExportThreadData *td = malloc( sizeof(*td) );
//td->gskeleton = skeleton;
td->javaInstance = &thisObj;
td->gpath = _path;
export_thread = g_thread_new(NULL, start_export_thread, td);
NativeReference *_nref = malloc( sizeof(*_nref) );
//_nref->value = (GObject *)skeleton;
jobject _bb = (*env)->NewDirectByteBuffer(env, (void*)_nref, sizeof(NativeReference));
return _bb;
}
JNIEXPORT void JNICALL Java_$$$interface.jname$$$__1destroy(JNIEnv *env, jobject thisObj) {
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy