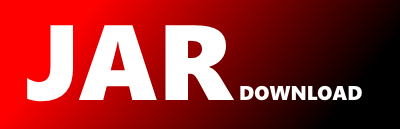
template.ObjectManager_c.txt Maven / Gradle / Ivy
/* Source generated by codegenj (https://github.com/olir/codegenj) - DO NOT EDIT!
*
* https://github.com/olir/codegenj
*
* This generated output is free and unencumbered software released into the public domain.
*
* Anyone is free to copy, modify, publish, use, compile, sell, or
* distribute this software, either in source code form or as a compiled
* binary, for any purpose, commercial or non-commercial, and by any
* means.
*
* In jurisdictions that recognize copyright laws, the author or authors
* of this software dedicate any and all copyright interest in the
* software to the public domain. We make this dedication for the benefit
* of the public at large and to the detriment of our heirs and
* successors. We intend this dedication to be an overt act of
* relinquishment in perpetuity of all present and future rights to this
* software under copyright law.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
* IN NO EVENT SHALL THE AUTHORS BE LIABLE FOR ANY CLAIM, DAMAGES OR
* OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE,
* ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
* OTHER DEALINGS IN THE SOFTWARE.
*
* For more information, please refer to
*/
#include
#include
#include
#include
#include
#include "de_serviceflow_codegenj_ObjectManager.h"
#include "$$$library$$$-codegen.h"
struct NativeReferenceStructure;
typedef struct NativeReferenceStructure {
GObject *value;
} NativeReference;
struct ThreadDataStructure;
typedef struct ThreadDataStructure {
GDBusObjectManager *gmanager;
jobject *jmanager;
} ThreadData;
JavaVM *g_jvm = NULL;
JNIEnv *g_env;
int loglevel; // read from java
const int FINE = 500; // log level <500 triggers logging.
const int FINER =400;
const int FINEST =300;
GThread *manager_thread;
GMainLoop *main_loop;
GList *initial_objects; // used only during initialization. is not updated by signals!
JNIEnv *attachCurrentThread() {
// double check it's all ok
int getEnvStat = (*g_jvm)->GetEnv(g_jvm, (void **) &g_env, JNI_VERSION_1_6);
if (getEnvStat == JNI_EDETACHED) {
if (loglevel<=FINER)
g_print ( "GetEnv: not attached yet ... " );
if ((*g_jvm)->AttachCurrentThread(g_jvm, (void **) &g_env, NULL) != 0) {
g_print ( "Failed to attach thread!\n" );
}
else {
if (loglevel<=FINER)
g_print ( "done.\n" );
}
} else if (getEnvStat == JNI_OK) {
//
} else if (getEnvStat == JNI_EVERSION) {
g_print ( "GetEnv: version not supported\n" );
}
return g_env;
}
void detachCurrentThread() {
if ((*g_env)->ExceptionCheck(g_env)) {
(*g_env)->ExceptionDescribe(g_env);
}
g_env = NULL;
(*g_jvm)->DetachCurrentThread(g_jvm);
}
void on_interface_added (GDBusObject *object,
GDBusInterface *interface, gpointer user_data) {
if (loglevel<=FINER)
g_print (" * on_interface_added()\n");
const gchar *objectpath = g_dbus_object_get_object_path (G_DBUS_OBJECT (object));
const gchar *name = g_dbus_proxy_get_interface_name (G_DBUS_PROXY (interface));
JNIEnv *env = attachCurrentThread();
jstring _signalname = (*env)->NewStringUTF(env, "interface-added");
jstring _objectpath = (*env)->NewStringUTF(env, objectpath);
jstring _name = (*env)->NewStringUTF(env, name);
NativeReference *_nref = malloc( sizeof(*_nref) );
_nref->value = (GObject *)interface;
jobject _bb = (*env)->NewDirectByteBuffer(env, (void*)_nref, sizeof(NativeReference));
jclass cls = (*env)->FindClass(env, "de/serviceflow/codegenj/ObjectManager");
jmethodID mid = (*env)->GetStaticMethodID(env, cls, "dispatchObjectManagerSignal", "(Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;Ljava/lang/Object;)V");
(*env)->CallStaticVoidMethod(env, cls, mid, _signalname, _objectpath, _name, _bb);
detachCurrentThread();
}
void on_interface_removed (GDBusObject *object,
GDBusInterface *interface, gpointer user_data) {
if (loglevel<=FINER)
g_print (" * on_interface_rmoved()\n");
const gchar *objectpath = g_dbus_object_get_object_path (G_DBUS_OBJECT (object));
const gchar *name = g_dbus_proxy_get_interface_name (G_DBUS_PROXY (interface));
JNIEnv *env = attachCurrentThread();
jstring _signalname = (*env)->NewStringUTF(env, "interface-removed");
jstring _objectpath = (*env)->NewStringUTF(env, objectpath);
jstring _name = (*env)->NewStringUTF(env, name);
NativeReference *_nref = malloc( sizeof(*_nref) );
_nref->value = (GObject *)interface;
jobject _bb = (*env)->NewDirectByteBuffer(env, (void*)_nref, sizeof(NativeReference));
jclass cls = (*env)->FindClass(env, "de/serviceflow/codegenj/ObjectManager");
jmethodID mid = (*env)->GetStaticMethodID(env, cls, "dispatchObjectManagerSignal", "(Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;Ljava/lang/Object;)V");
(*env)->CallStaticVoidMethod(env, cls, mid, _signalname, _objectpath, _name, _bb);
detachCurrentThread();
}
void on_object_added (GDBusObjectManager *manager,
GDBusObject *object, gpointer user_data) {
if (loglevel<=FINER)
g_print (" * on_object_added()\n");
const gchar *objectpath = g_dbus_object_get_object_path (G_DBUS_OBJECT (object));
JNIEnv *env = attachCurrentThread();
jstring _signalname = (*env)->NewStringUTF(env, "object-added");
jstring _objectpath = (*env)->NewStringUTF(env, objectpath);
jclass cls = (*env)->FindClass(env, "de/serviceflow/codegenj/ObjectManager");
jmethodID mid = (*env)->GetStaticMethodID(env, cls, "dispatchObjectManagerSignal", "(Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;Ljava/lang/Object;)V");
(*env)->CallStaticVoidMethod(env, cls, mid, _signalname, _objectpath, NULL, NULL);
detachCurrentThread();
GList *l, *interfaces = g_dbus_object_get_interfaces(object);
for(l = interfaces; l != NULL; l = l->next) {
on_interface_added(object, (GDBusInterface *)l->data, user_data);
}
g_list_free_full(interfaces, g_object_unref);
}
void on_object_removed (GDBusObjectManager *manager,
GDBusObject *object, gpointer user_data) {
if (loglevel<=FINER)
g_print (" * on_object_removed()\n");
const gchar *objectpath = g_dbus_object_get_object_path (G_DBUS_OBJECT (object));
JNIEnv *env = attachCurrentThread();
jstring _signalname = (*env)->NewStringUTF(env, "object-removed");
jstring _objectpath = (*env)->NewStringUTF(env, objectpath);
jclass cls = (*env)->FindClass(env, "de/serviceflow/codegenj/ObjectManager");
jmethodID mid = (*env)->GetStaticMethodID(env, cls, "dispatchObjectManagerSignal",
"(Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;Ljava/lang/Object;)V");
(*env)->CallStaticVoidMethod(env, cls, mid, _signalname, _objectpath, NULL, NULL);
detachCurrentThread();
GList *l, *interfaces = g_dbus_object_get_interfaces(object);
for(l = interfaces; l != NULL; l = l->next)
on_interface_removed(object, (GDBusInterface *)l->data, user_data);
g_list_free_full(interfaces, g_object_unref);
}
gpointer start_thread (void *data) {
GDBusObjectManager *_client = ((ThreadData *) data)->gmanager;
jobject *callbackObj = ((ThreadData *) data)->jmanager;
main_loop = g_main_loop_new(NULL, FALSE);
g_signal_connect(_client,
"interface-added",
G_CALLBACK(on_interface_added),
callbackObj);
g_signal_connect(_client,
"interface-removed",
G_CALLBACK(on_interface_removed),
callbackObj);
g_signal_connect(_client,
"object-added",
G_CALLBACK(on_object_added),
callbackObj);
g_signal_connect(_client,
"object-removed",
G_CALLBACK(on_object_removed),
callbackObj);
if (loglevel<=FINEST)
g_print (" starting main loop...\n");
g_main_loop_run(main_loop);
return NULL;
}
gpointer exit_thread (void *data) {
g_main_loop_quit (main_loop);
manager_thread = NULL;
return NULL;
}
JNIEXPORT void JNICALL Java_de_serviceflow_codegenj_ObjectManager__1init(JNIEnv *env, jobject thisObj, jstring objectpath) {
jclass cls = (*env)->FindClass(env, "de/serviceflow/codegenj/ObjectManager");
jmethodID mid = (*env)->GetStaticMethodID(env, cls, "getLogLevelIntValue", "()I");
loglevel = (*env)->CallStaticIntMethod(env, cls, mid);
int status = (*env)->GetJavaVM(env, &g_jvm);
if(status != 0) {
g_print ("\nFailed to get vm pointer!!!\n");
}
const gchar *_objectpath = (*env)->GetStringUTFChars(env, objectpath, 0);
GError *_error = NULL;
GDBusObjectManager *_client = object_manager_client_new_for_bus_sync (
G_BUS_TYPE_SYSTEM,
G_DBUS_OBJECT_MANAGER_CLIENT_FLAGS_NONE,
"$$$busname$$$", // bus name
_objectpath, // object path
NULL, // GCancellable*
&_error);
(*env)->ReleaseStringUTFChars(env, objectpath, _objectpath);
if (_error!=NULL) {
jclass _eClass = (*env)->FindClass(env, "java/lang/Error");
(*env)->ThrowNew(env, _eClass, _error->message);
(*env)->DeleteLocalRef(env, _eClass);
g_error_free(_error);
}
if (_client == NULL) {
jclass _eClass = (*env)->FindClass(env, "java/lang/Error");
(*env)->ThrowNew(env, _eClass, "Error getting object manager client. ");
}
NativeReference *_nref = malloc( sizeof(*_nref) );
_nref->value = (GObject *)_client;
jobject _bb = (*env)->NewDirectByteBuffer(env, (void*)_nref, sizeof(NativeReference));
jclass _cls = (*env)->GetObjectClass(env, thisObj);
jfieldID _fid = (*env)->GetFieldID(env, _cls, "_client", "Ljava/lang/Object;");
(*env)->SetObjectField(env, thisObj, _fid, _bb);
g_object_ref(_client);
// get top-level objects
if (loglevel<=FINER) {
const gchar *path = g_dbus_object_manager_get_object_path (_client);
g_print (" - GDBusObjectManager at %s\n", path);
}
initial_objects = g_dbus_object_manager_get_objects(_client);
}
JNIEXPORT void JNICALL Java_de_serviceflow_codegenj_ObjectManager__1run(JNIEnv *env, jobject thisObj) {
g_list_free_full (initial_objects, g_object_unref);
jclass _cls = (*env)->GetObjectClass(env, thisObj);
jfieldID _fid = (*env)->GetFieldID(env, _cls, "_client", "Ljava/lang/Object;");
jobject _bb = (*env)->GetObjectField(env, thisObj, _fid);
NativeReference* _nref = (NativeReference*) (*env)->GetDirectBufferAddress(env, _bb);
GDBusObjectManager *_client = (GDBusObjectManager *)_nref->value;
// Start update Thread
ThreadData *td = malloc( sizeof(*td) );
td->gmanager = _client;
td->jmanager = &thisObj;
manager_thread = g_thread_new(NULL, start_thread, td);
}
JNIEXPORT void JNICALL Java_de_serviceflow_codegenj_ObjectManager__1destroy(JNIEnv *env, jobject thisObj) {
g_thread_new(NULL, exit_thread, NULL);
jclass _cls = (*env)->GetObjectClass(env, thisObj);
jfieldID _fid = (*env)->GetFieldID(env, _cls, "_client", "Ljava/lang/Object;");
jobject _bb = (*env)->GetObjectField(env, thisObj, _fid);
NativeReference *_nref = (NativeReference*) (*env)->GetDirectBufferAddress(env, _bb);
GDBusObjectManager *_client = (GDBusObjectManager *)_nref->value;
g_object_unref(_client);
free(_nref);
}
JNIEXPORT jobjectArray JNICALL Java_de_serviceflow_codegenj_ObjectManager_getProxyObjects(JNIEnv *env, jobject thisObj, jstring interfacename, jboolean initial) {
const gchar *_interfacename = (*env)->GetStringUTFChars(env, interfacename, 0);
jclass _cls = (*env)->GetObjectClass(env, thisObj);
jfieldID _fid = (*env)->GetFieldID(env, _cls, "_client", "Ljava/lang/Object;");
jobject _bb = (*env)->GetObjectField(env, thisObj, _fid);
NativeReference* _nref = (NativeReference*) (*env)->GetDirectBufferAddress(env, _bb);
GDBusObjectManager *_client = (GDBusObjectManager *)_nref->value;
jobjectArray ret;
int count = 0;
GList *l;
GList *objects;
if (initial) {
objects = initial_objects;
}
else {
objects = g_dbus_object_manager_get_objects(_client);
}
// guint ocount = g_list_length (objects);
// g_print (" [giob 1] objects.length = %d\n", ocount);
const gchar *objectpath;
for (l = g_list_first (objects); l != NULL; l = l->next) {
GDBusObject *object = l->data;
GList *interfaces;
GList *ll;
objectpath = g_dbus_object_get_object_path (G_DBUS_OBJECT (object));
if (loglevel<=FINE)
g_print (" - Object at %s\n", objectpath);
interfaces = g_dbus_object_get_interfaces (G_DBUS_OBJECT (object));
for (ll = interfaces; ll != NULL; ll = ll->next) {
GDBusInterface *interface = G_DBUS_INTERFACE (ll->data);
const gchar *name = g_dbus_proxy_get_interface_name (G_DBUS_PROXY (interface));
if (loglevel<=FINE)
g_print (" - Interface %s\n", name );
if (strcmp(_interfacename, name) == 0) {
count++;
}
}
g_list_free_full (interfaces, g_object_unref);
}
if (loglevel<=FINER)
g_print (" - interface match count: %d\n", count);
ret= (jobjectArray)(*env)->NewObjectArray(env,
count,
(*env)->FindClass(env, "java/lang/Object"),
NULL);
int i = 0;
for (l = objects; l != NULL; l = l->next) {
GDBusObject *object = l->data;
GList *interfaces;
GList *ll;
objectpath = g_dbus_object_get_object_path (G_DBUS_OBJECT (object));
interfaces = g_dbus_object_get_interfaces (G_DBUS_OBJECT (object));
for (ll = interfaces; ll != NULL; ll = ll->next) {
GDBusInterface *interface = G_DBUS_INTERFACE (ll->data);
const gchar *name = g_dbus_proxy_get_interface_name (G_DBUS_PROXY (interface));
if (strcmp(_interfacename, name) == 0) {
NativeReference *_nref = malloc( sizeof(*_nref) );
_nref->value = (GObject *)g_dbus_object_get_interface (G_DBUS_OBJECT (object), _interfacename);
jobject _bb = (*env)->NewDirectByteBuffer(env, (void*)_nref, sizeof(NativeReference));
(*env)->SetObjectArrayElement(env, ret, i++, _bb);
}
}
g_list_free_full (interfaces, g_object_unref);
}
(*env)->ReleaseStringUTFChars(env, interfacename, _interfacename);
return(ret);
}
JNIEXPORT jstring JNICALL Java_de_serviceflow_codegenj_ObjectManager_getObjectPath(JNIEnv *env, jobject thisObj, jobject bb) {
NativeReference* _nref = (NativeReference*) (*env)->GetDirectBufferAddress(env, bb);
GDBusInterface *_client = (GDBusInterface *)_nref->value;
// const gchar *name = g_dbus_proxy_get_interface_name (G_DBUS_PROXY (_client));
GDBusObject *object = g_dbus_interface_get_object (_client);
const gchar *path = g_dbus_object_get_object_path (object);
return (*env)->NewStringUTF(env, path);
}
JNIEXPORT void JNICALL Java_de_serviceflow_codegenj_ObjectManager_dump(JNIEnv *env, jobject thisObj) {
jclass _cls = (*env)->GetObjectClass(env, thisObj);
jfieldID _fid = (*env)->GetFieldID(env, _cls, "_client", "Ljava/lang/Object;");
jobject _bb = (*env)->GetObjectField(env, thisObj, _fid);
NativeReference* _nref = (NativeReference*) (*env)->GetDirectBufferAddress(env, _bb);
GDBusObjectManager *_client = (GDBusObjectManager *)_nref->value;
GList *objects = g_dbus_object_manager_get_objects(_client);
GList *l;
const gchar *objectpath;
for (l = g_list_first (objects); l != NULL; l = l->next) {
GDBusObject *object = l->data;
GList *interfaces;
GList *ll;
objectpath = g_dbus_object_get_object_path (G_DBUS_OBJECT (object));
g_print ("+ Object [%s]\n", objectpath);
interfaces = g_dbus_object_get_interfaces (G_DBUS_OBJECT (object));
for (ll = interfaces; ll != NULL; ll = ll->next) {
GDBusInterface *interface = G_DBUS_INTERFACE (ll->data);
const gchar *name = g_dbus_proxy_get_interface_name (G_DBUS_PROXY (interface));
g_print (" - Interface %s\n", name );
}
g_list_free_full (interfaces, g_object_unref);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy