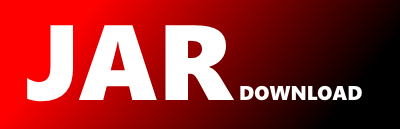
stream.runtime.setup.handler.DProcessElementHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of streams-spark Show documentation
Show all versions of streams-spark Show documentation
A streams extension for the execution on an Apache Spark cluster.
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy