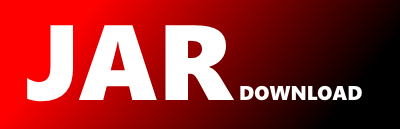
streams.weka.QFactor Maven / Gradle / Ivy
/**
*
*/
package streams.weka;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import stream.AbstractProcessor;
import stream.Data;
import stream.annotations.Parameter;
import stream.data.Statistics;
/**
* This implementation of a processor will compute the *q-factor*, i.e. the
* efficiency of the class prediction for the positive class. The true label of
* the instances is expected to be found as key `@label` whereas the predicted
* value is assumed to be available as `@prediction`.
*
* @author Christian Bockermann <[email protected]>
*
*/
public class QFactor extends AbstractProcessor {
static Logger log = LoggerFactory.getLogger(QFactor.class);
@Parameter(description = "The key under which the true label of the item is found.", required = false, defaultValue = "@label")
String label = "@label";
@Parameter(description = "The key under which the predicted class value is found.", required = false, defaultValue = "@prediction")
String prediction = "@prediction";
final Statistics statistics = new Statistics();
/**
* @see stream.Processor#process(stream.Data)
*/
@Override
public Data process(Data input) {
if (input.containsKey(prediction) && input.containsKey(label)) {
String label = input.get(this.label).toString();
String pred = input.get(this.prediction).toString();
if (label.equals("gamma")) {
if (label.equals(pred)) {
statistics.add("true-positive", 1.0d);
} else {
statistics.add("false-positive", 1.0d);
}
} else {
if (label.equals(pred)) {
statistics.add("true-negative", 1.0d);
} else {
statistics.add("false-negative", 1.0d);
}
}
}
return input;
}
/**
* @see stream.AbstractProcessor#finish()
*/
@Override
public void finish() throws Exception {
super.finish();
Double tp = value("true-positive");
Double fp = value("false-positive");
Double tn = value("true-negative");
Double fn = value("false-negative");
Double q = qfactor(tp, fp, tn, fn);
log.info("+----- Q-Factor -----");
log.info("| TP = {}", tp);
log.info("| FP = {}", fp);
log.info("| TN = {}", tn);
log.info("| FN = {}", fn);
log.info("|");
log.info("| q-Factor: {}", q);
log.info("+----- -------- -----");
}
/**
* @return the label
*/
public String getLabel() {
return label;
}
/**
* @param label
* the label to set
*/
public void setLabel(String label) {
this.label = label;
}
/**
* @return the prediction
*/
public String getPrediction() {
return prediction;
}
/**
* @param prediction
* the prediction to set
*/
public void setPrediction(String prediction) {
this.prediction = prediction;
}
protected Double value(String key) {
Double val = statistics.get(key);
if (val == null) {
return 0.0;
} else {
return val;
}
}
public static double qfactor(double tp, double fp, double tn, double fn) {
return (tp / (tp + fn)) / Math.sqrt(fp / (fp + tn));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy