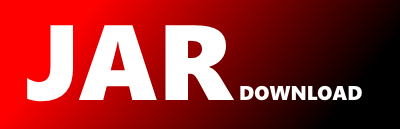
streams.weka.RandomGuess Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of streams-weka Show documentation
Show all versions of streams-weka Show documentation
Weka adaption for streaming processes in streams.
The newest version!
/**
*
*/
package streams.weka;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import weka.classifiers.AbstractClassifier;
import weka.core.Attribute;
import weka.core.Instance;
import weka.core.Instances;
/**
* @author chris
*
*/
public class RandomGuess extends AbstractClassifier {
/** The unique class ID */
private static final long serialVersionUID = -5074227619992360588L;
static Logger log = LoggerFactory.getLogger(RandomGuess.class);
boolean regression = false;
Double min = 0.0;
Double max = 1.0;
int numClasses = 0;
String[] classes;
/**
* @see weka.classifiers.Classifier#buildClassifier(weka.core.Instances)
*/
@Override
public void buildClassifier(Instances data) throws Exception {
Attribute labelAttribute = data.classAttribute();
if (labelAttribute.isNumeric()) {
regression = true;
for (int i = 0; i < data.size(); i++) {
Instance ex = data.instance(i);
Double val = ex.value(labelAttribute);
min = Math.min(min, val);
max = Math.max(max, val);
}
// log.info("Creating regression RandomGuess model for [{},{}]",
// min,
// max);
} else {
numClasses = labelAttribute.numValues();
classes = new String[numClasses];
// log.info("Creating RandomGuess model with {} classes",
// numClasses);
for (int i = 0; i < numClasses; i++) {
String str = labelAttribute.value(i);
classes[i] = str;
}
}
}
/**
* @see weka.classifiers.AbstractClassifier#classifyInstance(weka.core.Instance)
*/
@Override
public double classifyInstance(Instance instance) throws Exception {
if (regression) {
double ret = Math.random() * (max - min);
// log.info("Random regression value: {}", ret);
return ret;
}
double[] dist = this.distributionForInstance(instance);
int max = 0;
for (int i = 1; i < dist.length; i++) {
if (dist[max] < dist[i]) {
max = i;
}
}
// log.info("Random prediction value: {} ({})", classes[max], max);
return (double) max;
}
/*
*/
@Override
public double[] distributionForInstance(Instance instance) throws Exception {
double sum = 0.0;
double[] dist = new double[numClasses];
for (int i = 0; i < classes.length; i++) {
dist[i] = Math.random();
sum += dist[i];
}
for (int i = 0; i < dist.length; i++) {
dist[i] = dist[i] / sum;
}
return dist;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy