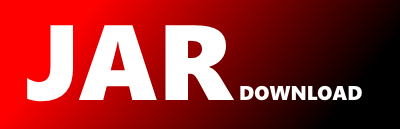
de.skuzzle.test.snapshots.normalize.ObjectMemberAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snapshot-tests-normalize Show documentation
Show all versions of snapshot-tests-normalize Show documentation
Travers/Clean/Normalize object instances
package de.skuzzle.test.snapshots.normalize;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.regex.Pattern;
import java.util.stream.Stream;
/**
* An action that can be applied to a {@link ObjectMember} which is matched by a
* {@link Predicate}.
*
* @author Simon Taddiken
*/
public final class ObjectMemberAction {
private final Predicate predicate;
private final Consumer action;
private ObjectMemberAction(Predicate predicate, Consumer action) {
this.predicate = predicate;
this.action = action;
}
public static ChoosePredicateBuilder members() {
return new ChoosePredicateBuilder();
}
Stream applyTo(Stream stream) {
return stream.peek(objectMember -> {
if (predicate.test(objectMember)) {
action.accept(objectMember);
}
});
}
public static class ChoosePredicateBuilder {
public ChooseActionBuilder withValueTypeCompatibleTo(Class> type) {
return where(objectMember -> objectMember.hasTypeCompatibleTo(type));
}
public ChooseActionBuilder withValueEqualTo(Object obj) {
return where(objectMember -> Objects.equals(obj, objectMember.value()));
}
public ChooseActionBuilder withStringValueMatching(Pattern pattern) {
return where(objectMember -> objectMember.hasTypeCompatibleTo(String.class)
&& objectMember.value() != null
&& pattern.matcher(objectMember.value().toString()).matches());
}
public ChooseActionBuilder withStringValueMatching(String pattern) {
return withStringValueMatching(Pattern.compile(pattern));
}
public ChooseActionBuilder any() {
return where(objectMember -> true);
}
public ChooseActionBuilder where(Predicate predicate) {
return new ChooseActionBuilder(predicate);
}
}
public static class ChooseActionBuilder {
private final Predicate predicate;
private ChooseActionBuilder(Predicate predicate) {
this.predicate = Objects.requireNonNull(predicate, "predicate must not be null");
}
public ObjectMemberAction consumeWith(Consumer action) {
return new ObjectMemberAction(predicate, Objects.requireNonNull(action, "action must not be null"));
}
public ObjectMemberAction mapValueTo(Function super Object, ? extends Object> transformer) {
return consumeWith(objectMember -> objectMember.setValue(transformer.apply(objectMember.value())));
}
public ObjectMemberAction setValueTo(Object value) {
return consumeWith(objectMember -> objectMember.setValue(value));
}
public ObjectMemberAction setValueToNull() {
return setValueTo(null);
}
public ObjectMemberAction setToEmptyValue() {
return consumeWith(objectMember -> {
final Object emptyValue = SpecialTypesAndValues.getEmptyValueForType(objectMember.valueType());
if (emptyValue != null) {
objectMember.setValue(emptyValue);
}
});
}
public ObjectMemberAction removeFromParent() {
return consumeWith(objectMember -> {
final Object containerCollection = objectMember.collectionParent().orElse(null);
if (containerCollection == null) {
objectMember.setValue(null);
} else if (containerCollection instanceof Collection>) {
((Collection>) containerCollection).remove(objectMember.parent());
}
});
}
public ObjectMemberAction consistentlyReplaceWith(
BiFunction generator) {
final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy