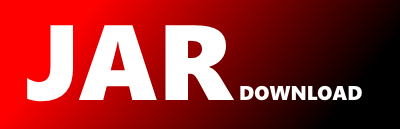
de.sonallux.spotify.api.apis.AlbumsApi Maven / Gradle / Ivy
Show all versions of spotify-web-api-java Show documentation
package de.sonallux.spotify.api.apis;
import de.sonallux.spotify.api.http.ApiClient;
import de.sonallux.spotify.api.apis.albums.*;
import lombok.RequiredArgsConstructor;
/**
* Albums
*/
@RequiredArgsConstructor
public class AlbumsApi {
private final ApiClient apiClient;
/**
* Check User's Saved Albums
* Check if one or more albums is already saved in the current Spotify user's 'Your Music' library.
* @param ids A comma-separated list of the Spotify IDs for the albums. Maximum: 20 IDs.
* @return a {@link CheckUsersSavedAlbumsRequest} object to build and execute the request
*/
public CheckUsersSavedAlbumsRequest checkUsersSavedAlbums(String ids) {
return new CheckUsersSavedAlbumsRequest(apiClient, ids);
}
/**
* Get Album
* Get Spotify catalog information for a single album.
* @param id The Spotify ID of the album.
* @return a {@link GetAlbumRequest} object to build and execute the request
*/
public GetAlbumRequest getAlbum(String id) {
return new GetAlbumRequest(apiClient, id);
}
/**
* Get Album Tracks
* Get Spotify catalog information about an album’s tracks. Optional parameters can be used to limit the number of tracks returned.
* @param id The Spotify ID of the album.
* @return a {@link GetAlbumsTracksRequest} object to build and execute the request
*/
public GetAlbumsTracksRequest getAlbumsTracks(String id) {
return new GetAlbumsTracksRequest(apiClient, id);
}
/**
* Get Several Albums
* Get Spotify catalog information for multiple albums identified by their Spotify IDs.
* @param ids A comma-separated list of the Spotify IDs for the albums. Maximum: 20 IDs.
* @return a {@link GetMultipleAlbumsRequest} object to build and execute the request
*/
public GetMultipleAlbumsRequest getMultipleAlbums(String ids) {
return new GetMultipleAlbumsRequest(apiClient, ids);
}
/**
* Get New Releases
* Get a list of new album releases featured in Spotify (shown, for example, on a Spotify player’s “Browse” tab).
* @return a {@link GetNewReleasesRequest} object to build and execute the request
*/
public GetNewReleasesRequest getNewReleases() {
return new GetNewReleasesRequest(apiClient);
}
/**
* Get User's Saved Albums
* Get a list of the albums saved in the current Spotify user's 'Your Music' library.
* @return a {@link GetUsersSavedAlbumsRequest} object to build and execute the request
*/
public GetUsersSavedAlbumsRequest getUsersSavedAlbums() {
return new GetUsersSavedAlbumsRequest(apiClient);
}
/**
* Remove Users' Saved Albums
* Remove one or more albums from the current user's 'Your Music' library.
* @param ids A JSON array of the Spotify IDs. For example: ["4iV5W9uYEdYUVa79Axb7Rh", "1301WleyT98MSxVHPZCA6M"]
A maximum of 50 items can be specified in one request. Note: if the ids
parameter is present in the query string, any IDs listed here in the body will be ignored.
* @return a {@link RemoveAlbumsUserRequest} object to build and execute the request
*/
public RemoveAlbumsUserRequest removeAlbumsUser(java.util.List ids) {
return new RemoveAlbumsUserRequest(apiClient, ids);
}
/**
* Save Albums for Current User
* Save one or more albums to the current user's 'Your Music' library.
* @param ids A JSON array of the Spotify IDs. For example: ["4iV5W9uYEdYUVa79Axb7Rh", "1301WleyT98MSxVHPZCA6M"]
A maximum of 50 items can be specified in one request. Note: if the ids
parameter is present in the query string, any IDs listed here in the body will be ignored.
* @return a {@link SaveAlbumsUserRequest} object to build and execute the request
*/
public SaveAlbumsUserRequest saveAlbumsUser(java.util.List ids) {
return new SaveAlbumsUserRequest(apiClient, ids);
}
}