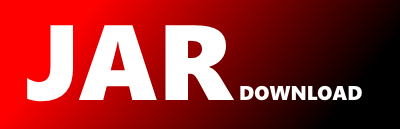
de.sonallux.spotify.api.apis.PlayerApi Maven / Gradle / Ivy
Show all versions of spotify-web-api-java Show documentation
package de.sonallux.spotify.api.apis;
import de.sonallux.spotify.api.http.ApiClient;
import de.sonallux.spotify.api.apis.player.*;
import lombok.RequiredArgsConstructor;
/**
* Player
*/
@RequiredArgsConstructor
public class PlayerApi {
private final ApiClient apiClient;
/**
* Add Item to Playback Queue
* Add an item to the end of the user's current playback queue. This API only works for users who have Spotify Premium. The order of execution is not guaranteed when you use this API with other Player API endpoints.
* @param uri The uri of the item to add to the queue. Must be a track or an episode uri.
* @return a {@link AddToQueueRequest} object to build and execute the request
*/
public AddToQueueRequest addToQueue(String uri) {
return new AddToQueueRequest(apiClient, uri);
}
/**
* Get Playback State
* Get information about the user’s current playback state, including track or episode, progress, and active device.
* @return a {@link GetInformationAboutUsersCurrentPlaybackRequest} object to build and execute the request
*/
public GetInformationAboutUsersCurrentPlaybackRequest getInformationAboutUsersCurrentPlayback() {
return new GetInformationAboutUsersCurrentPlaybackRequest(apiClient);
}
/**
* Get the User's Queue
* Get the list of objects that make up the user's queue.
* @return a {@link GetQueueRequest} object to build and execute the request
*/
public GetQueueRequest getQueue() {
return new GetQueueRequest(apiClient);
}
/**
* Get Recently Played Tracks
* Get tracks from the current user's recently played tracks. Note: Currently doesn't support podcast episodes.
* @return a {@link GetRecentlyPlayedRequest} object to build and execute the request
*/
public GetRecentlyPlayedRequest getRecentlyPlayed() {
return new GetRecentlyPlayedRequest(apiClient);
}
/**
* Get Available Devices
* Get information about a user’s available Spotify Connect devices. Some device models are not supported and will not be listed in the API response.
* @return a {@link GetUsersAvailableDevicesRequest} object to build and execute the request
*/
public GetUsersAvailableDevicesRequest getUsersAvailableDevices() {
return new GetUsersAvailableDevicesRequest(apiClient);
}
/**
* Get Currently Playing Track
* Get the object currently being played on the user's Spotify account.
* @return a {@link GetUsersCurrentlyPlayingTrackRequest} object to build and execute the request
*/
public GetUsersCurrentlyPlayingTrackRequest getUsersCurrentlyPlayingTrack() {
return new GetUsersCurrentlyPlayingTrackRequest(apiClient);
}
/**
* Pause Playback
* Pause playback on the user's account. This API only works for users who have Spotify Premium. The order of execution is not guaranteed when you use this API with other Player API endpoints.
* @return a {@link PauseUsersPlaybackRequest} object to build and execute the request
*/
public PauseUsersPlaybackRequest pauseUsersPlayback() {
return new PauseUsersPlaybackRequest(apiClient);
}
/**
* Seek To Position
* Seeks to the given position in the user’s currently playing track. This API only works for users who have Spotify Premium. The order of execution is not guaranteed when you use this API with other Player API endpoints.
* @param positionMs The position in milliseconds to seek to. Must be a positive number. Passing in a position that is greater than the length of the track will cause the player to start playing the next song.
* @return a {@link SeekToPositionInCurrentlyPlayingTrackRequest} object to build and execute the request
*/
public SeekToPositionInCurrentlyPlayingTrackRequest seekToPositionInCurrentlyPlayingTrack(int positionMs) {
return new SeekToPositionInCurrentlyPlayingTrackRequest(apiClient, positionMs);
}
/**
* Set Repeat Mode
* Set the repeat mode for the user's playback. This API only works for users who have Spotify Premium. The order of execution is not guaranteed when you use this API with other Player API endpoints.
* @param state track, context or off.
track will repeat the current track.
context will repeat the current context.
off will turn repeat off.
* @return a {@link SetRepeatModeOnUsersPlaybackRequest} object to build and execute the request
*/
public SetRepeatModeOnUsersPlaybackRequest setRepeatModeOnUsersPlayback(String state) {
return new SetRepeatModeOnUsersPlaybackRequest(apiClient, state);
}
/**
* Set Playback Volume
* Set the volume for the user’s current playback device. This API only works for users who have Spotify Premium. The order of execution is not guaranteed when you use this API with other Player API endpoints.
* @param volumePercent The volume to set. Must be a value from 0 to 100 inclusive.
* @return a {@link SetVolumeForUsersPlaybackRequest} object to build and execute the request
*/
public SetVolumeForUsersPlaybackRequest setVolumeForUsersPlayback(int volumePercent) {
return new SetVolumeForUsersPlaybackRequest(apiClient, volumePercent);
}
/**
* Skip To Next
* Skips to next track in the user’s queue. This API only works for users who have Spotify Premium. The order of execution is not guaranteed when you use this API with other Player API endpoints.
* @return a {@link SkipUsersPlaybackToNextTrackRequest} object to build and execute the request
*/
public SkipUsersPlaybackToNextTrackRequest skipUsersPlaybackToNextTrack() {
return new SkipUsersPlaybackToNextTrackRequest(apiClient);
}
/**
* Skip To Previous
* Skips to previous track in the user’s queue. This API only works for users who have Spotify Premium. The order of execution is not guaranteed when you use this API with other Player API endpoints.
* @return a {@link SkipUsersPlaybackToPreviousTrackRequest} object to build and execute the request
*/
public SkipUsersPlaybackToPreviousTrackRequest skipUsersPlaybackToPreviousTrack() {
return new SkipUsersPlaybackToPreviousTrackRequest(apiClient);
}
/**
* Start/Resume Playback
* Start a new context or resume current playback on the user's active device. This API only works for users who have Spotify Premium. The order of execution is not guaranteed when you use this API with other Player API endpoints.
* @return a {@link StartUsersPlaybackRequest} object to build and execute the request
*/
public StartUsersPlaybackRequest startUsersPlayback() {
return new StartUsersPlaybackRequest(apiClient);
}
/**
* Toggle Playback Shuffle
* Toggle shuffle on or off for user’s playback. This API only works for users who have Spotify Premium. The order of execution is not guaranteed when you use this API with other Player API endpoints.
* @param state true : Shuffle user's playback.
false : Do not shuffle user's playback.
* @return a {@link ToggleShuffleForUsersPlaybackRequest} object to build and execute the request
*/
public ToggleShuffleForUsersPlaybackRequest toggleShuffleForUsersPlayback(boolean state) {
return new ToggleShuffleForUsersPlaybackRequest(apiClient, state);
}
/**
* Transfer Playback
* Transfer playback to a new device and optionally begin playback. This API only works for users who have Spotify Premium. The order of execution is not guaranteed when you use this API with other Player API endpoints.
* @param deviceIds A JSON array containing the ID of the device on which playback should be started/transferred.
For example:{device_ids:["74ASZWbe4lXaubB36ztrGX"]}
Note: Although an array is accepted, only a single device_id is currently supported. Supplying more than one will return 400 Bad Request
* @return a {@link TransferUsersPlaybackRequest} object to build and execute the request
*/
public TransferUsersPlaybackRequest transferUsersPlayback(java.util.List deviceIds) {
return new TransferUsersPlaybackRequest(apiClient, deviceIds);
}
}