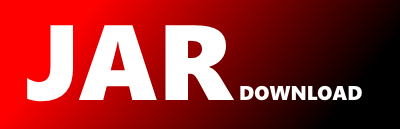
de.sonallux.spotify.api.apis.SearchApi Maven / Gradle / Ivy
Show all versions of spotify-web-api-java Show documentation
package de.sonallux.spotify.api.apis;
import de.sonallux.spotify.api.http.ApiClient;
import de.sonallux.spotify.api.apis.search.*;
import lombok.RequiredArgsConstructor;
/**
* Search
*/
@RequiredArgsConstructor
public class SearchApi {
private final ApiClient apiClient;
/**
* Search for Item
* Get Spotify catalog information about albums, artists, playlists, tracks, shows, episodes or audiobooks that match a keyword string. Audiobooks are only available within the US, UK, Canada, Ireland, New Zealand and Australia markets.
* @param q Your search query.
You can narrow down your search using field filters. The available filters are album
, artist
, track
, year
, upc
, tag:hipster
, tag:new
, isrc
, and genre
. Each field filter only applies to certain result types.
The artist
and year
filters can be used while searching albums, artists and tracks. You can filter on a single year
or a range (e.g. 1955-1960).
The album
filter can be used while searching albums and tracks.
The genre
filter can be used while searching artists and tracks.
The isrc
and track
filters can be used while searching tracks.
The upc
, tag:new
and tag:hipster
filters can only be used while searching albums. The tag:new
filter will return albums released in the past two weeks and tag:hipster
can be used to return only albums with the lowest 10% popularity.
* @param type A comma-separated list of item types to search across. Search results include hits from all the specified item types. For example: q=abacab&type=album,track
returns both albums and tracks matching "abacab".
* @return a {@link SearchRequest} object to build and execute the request
*/
public SearchRequest search(String q, java.util.List type) {
return new SearchRequest(apiClient, q, type);
}
}