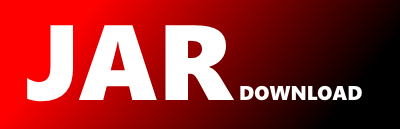
de.sonallux.spotify.api.apis.TracksApi Maven / Gradle / Ivy
Show all versions of spotify-web-api-java Show documentation
package de.sonallux.spotify.api.apis;
import de.sonallux.spotify.api.http.ApiClient;
import de.sonallux.spotify.api.apis.tracks.*;
import lombok.RequiredArgsConstructor;
/**
* Tracks
*/
@RequiredArgsConstructor
public class TracksApi {
private final ApiClient apiClient;
/**
* Check User's Saved Tracks
* Check if one or more tracks is already saved in the current Spotify user's 'Your Music' library.
* @param ids A comma-separated list of the Spotify IDs. For example: ids=4iV5W9uYEdYUVa79Axb7Rh,1301WleyT98MSxVHPZCA6M
. Maximum: 50 IDs.
* @return a {@link CheckUsersSavedTracksRequest} object to build and execute the request
*/
public CheckUsersSavedTracksRequest checkUsersSavedTracks(String ids) {
return new CheckUsersSavedTracksRequest(apiClient, ids);
}
/**
* Get Track's Audio Analysis
* Get a low-level audio analysis for a track in the Spotify catalog. The audio analysis describes the track’s structure and musical content, including rhythm, pitch, and timbre.
* @param id The Spotify IDfor the track.
* @return a {@link GetAudioAnalysisRequest} object to build and execute the request
*/
public GetAudioAnalysisRequest getAudioAnalysis(String id) {
return new GetAudioAnalysisRequest(apiClient, id);
}
/**
* Get Track's Audio Features
* Get audio feature information for a single track identified by its unique Spotify ID.
* @param id The Spotify ID for the track.
* @return a {@link GetAudioFeaturesRequest} object to build and execute the request
*/
public GetAudioFeaturesRequest getAudioFeatures(String id) {
return new GetAudioFeaturesRequest(apiClient, id);
}
/**
* Get Recommendations
* Recommendations are generated based on the available information for a given seed entity and matched against similar artists and tracks. If there is sufficient information about the provided seeds, a list of tracks will be returned together with pool size details.
For artists and tracks that are very new or obscure there might not be enough data to generate a list of tracks.
* @return a {@link GetRecommendationsRequest} object to build and execute the request
*/
public GetRecommendationsRequest getRecommendations() {
return new GetRecommendationsRequest(apiClient);
}
/**
* Get Several Tracks' Audio Features
* Get audio features for multiple tracks based on their Spotify IDs.
* @param ids A comma-separated list of the Spotify IDsfor the tracks. Maximum: 100 IDs.
* @return a {@link GetSeveralAudioFeaturesRequest} object to build and execute the request
*/
public GetSeveralAudioFeaturesRequest getSeveralAudioFeatures(String ids) {
return new GetSeveralAudioFeaturesRequest(apiClient, ids);
}
/**
* Get Several Tracks
* Get Spotify catalog information for multiple tracks based on their Spotify IDs.
* @param ids A comma-separated list of the Spotify IDs. For example: ids=4iV5W9uYEdYUVa79Axb7Rh,1301WleyT98MSxVHPZCA6M
. Maximum: 50 IDs.
* @return a {@link GetSeveralTracksRequest} object to build and execute the request
*/
public GetSeveralTracksRequest getSeveralTracks(String ids) {
return new GetSeveralTracksRequest(apiClient, ids);
}
/**
* Get Track
* Get Spotify catalog information for a single track identified by its unique Spotify ID.
* @param id The Spotify IDfor the track.
* @return a {@link GetTrackRequest} object to build and execute the request
*/
public GetTrackRequest getTrack(String id) {
return new GetTrackRequest(apiClient, id);
}
/**
* Get User's Saved Tracks
* Get a list of the songs saved in the current Spotify user's 'Your Music' library.
* @return a {@link GetUsersSavedTracksRequest} object to build and execute the request
*/
public GetUsersSavedTracksRequest getUsersSavedTracks() {
return new GetUsersSavedTracksRequest(apiClient);
}
/**
* Remove User's Saved Tracks
* Remove one or more tracks from the current user's 'Your Music' library.
* @param ids A JSON array of the Spotify IDs. For example: ["4iV5W9uYEdYUVa79Axb7Rh", "1301WleyT98MSxVHPZCA6M"]
A maximum of 50 items can be specified in one request. Note: if the ids
parameter is present in the query string, any IDs listed here in the body will be ignored.
* @return a {@link RemoveTracksUserRequest} object to build and execute the request
*/
public RemoveTracksUserRequest removeTracksUser(java.util.List ids) {
return new RemoveTracksUserRequest(apiClient, ids);
}
/**
* Save Tracks for Current User
* Save one or more tracks to the current user's 'Your Music' library.
* @param ids A JSON array of the Spotify IDs. For example: ["4iV5W9uYEdYUVa79Axb7Rh", "1301WleyT98MSxVHPZCA6M"]
A maximum of 50 items can be specified in one request. Note: if the ids
parameter is present in the query string, any IDs listed here in the body will be ignored.
* @return a {@link SaveTracksUserRequest} object to build and execute the request
*/
public SaveTracksUserRequest saveTracksUser(java.util.List ids) {
return new SaveTracksUserRequest(apiClient, ids);
}
}