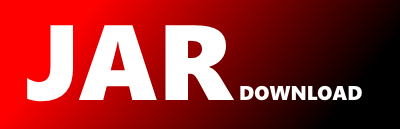
de.sonallux.spotify.api.apis.UsersApi Maven / Gradle / Ivy
Show all versions of spotify-web-api-java Show documentation
package de.sonallux.spotify.api.apis;
import de.sonallux.spotify.api.http.ApiClient;
import de.sonallux.spotify.api.apis.users.*;
import lombok.RequiredArgsConstructor;
/**
* Users
*/
@RequiredArgsConstructor
public class UsersApi {
private final ApiClient apiClient;
/**
* Check If User Follows Artists or Users
* Check to see if the current user is following one or more artists or other Spotify users.
* @param type The ID type: either artist
or user
.
* @param ids A comma-separated list of the artist or the user Spotify IDs to check. For example: ids=74ASZWbe4lXaubB36ztrGX,08td7MxkoHQkXnWAYD8d6Q
. A maximum of 50 IDs can be sent in one request.
* @return a {@link CheckCurrentUserFollowsRequest} object to build and execute the request
*/
public CheckCurrentUserFollowsRequest checkCurrentUserFollows(String type, String ids) {
return new CheckCurrentUserFollowsRequest(apiClient, type, ids);
}
/**
* Check if Current User Follows Playlist
* Check to see if the current user is following a specified playlist.
* @param playlistId The Spotify ID of the playlist.
* @return a {@link CheckIfUserFollowsPlaylistRequest} object to build and execute the request
*/
public CheckIfUserFollowsPlaylistRequest checkIfUserFollowsPlaylist(String playlistId) {
return new CheckIfUserFollowsPlaylistRequest(apiClient, playlistId);
}
/**
* Follow Artists or Users
* Add the current user as a follower of one or more artists or other Spotify users.
* @param type The ID type.
* @param ids A JSON array of the artist or user Spotify IDs. For example: {ids:["74ASZWbe4lXaubB36ztrGX", "08td7MxkoHQkXnWAYD8d6Q"]}
. A maximum of 50 IDs can be sent in one request. Note: if the ids
parameter is present in the query string, any IDs listed here in the body will be ignored.
* @return a {@link FollowArtistsUsersRequest} object to build and execute the request
*/
public FollowArtistsUsersRequest followArtistsUsers(String type, java.util.List ids) {
return new FollowArtistsUsersRequest(apiClient, type, ids);
}
/**
* Follow Playlist
* Add the current user as a follower of a playlist.
* @param playlistId The Spotify ID of the playlist.
* @return a {@link FollowPlaylistRequest} object to build and execute the request
*/
public FollowPlaylistRequest followPlaylist(String playlistId) {
return new FollowPlaylistRequest(apiClient, playlistId);
}
/**
* Get Current User's Profile
* Get detailed profile information about the current user (including the current user's username).
* @return a {@link GetCurrentUsersProfileRequest} object to build and execute the request
*/
public GetCurrentUsersProfileRequest getCurrentUsersProfile() {
return new GetCurrentUsersProfileRequest(apiClient);
}
/**
* Get Followed Artists
* Get the current user's followed artists.
* @param type The ID type: currently only artist
is supported.
* @return a {@link GetFollowedRequest} object to build and execute the request
*/
public GetFollowedRequest getFollowed(String type) {
return new GetFollowedRequest(apiClient, type);
}
/**
* Get User's Profile
* Get public profile information about a Spotify user.
* @param userId The user's Spotify user ID.
* @return a {@link GetUsersProfileRequest} object to build and execute the request
*/
public GetUsersProfileRequest getUsersProfile(String userId) {
return new GetUsersProfileRequest(apiClient, userId);
}
/**
* Get User's Top Artists
* Get the current user's top artists based on calculated affinity.
* @return a {@link GetUsersTopArtistsRequest} object to build and execute the request
*/
public GetUsersTopArtistsRequest getUsersTopArtists() {
return new GetUsersTopArtistsRequest(apiClient);
}
/**
* Get User's Top Tracks
* Get the current user's top tracks based on calculated affinity.
* @return a {@link GetUsersTopTracksRequest} object to build and execute the request
*/
public GetUsersTopTracksRequest getUsersTopTracks() {
return new GetUsersTopTracksRequest(apiClient);
}
/**
* Unfollow Artists or Users
* Remove the current user as a follower of one or more artists or other Spotify users.
* @param type The ID type: either artist
or user
.
* @param ids A JSON array of the artist or user Spotify IDs. For example: {ids:["74ASZWbe4lXaubB36ztrGX", "08td7MxkoHQkXnWAYD8d6Q"]}
. A maximum of 50 IDs can be sent in one request. Note: if the ids
parameter is present in the query string, any IDs listed here in the body will be ignored.
* @return a {@link UnfollowArtistsUsersRequest} object to build and execute the request
*/
public UnfollowArtistsUsersRequest unfollowArtistsUsers(String type, java.util.List ids) {
return new UnfollowArtistsUsersRequest(apiClient, type, ids);
}
/**
* Unfollow Playlist
* Remove the current user as a follower of a playlist.
* @param playlistId The Spotify ID of the playlist.
* @return a {@link UnfollowPlaylistRequest} object to build and execute the request
*/
public UnfollowPlaylistRequest unfollowPlaylist(String playlistId) {
return new UnfollowPlaylistRequest(apiClient, playlistId);
}
}