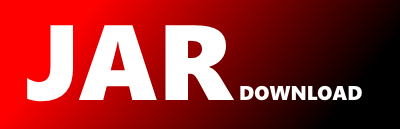
de.sonallux.spotify.api.apis.player.StartUsersPlaybackRequest Maven / Gradle / Ivy
Show all versions of spotify-web-api-java Show documentation
package de.sonallux.spotify.api.apis.player;
import com.fasterxml.jackson.core.type.TypeReference;
import de.sonallux.spotify.api.http.ApiCall;
import de.sonallux.spotify.api.http.ApiClient;
import de.sonallux.spotify.api.http.Request;
import de.sonallux.spotify.api.models.*;
/**
* Start/Resume Playback request
*
* Required OAuth scopes
* user-modify-playback-state
*
* Response
* Playback started
*/
public class StartUsersPlaybackRequest {
private static final TypeReference RESPONSE_TYPE = new TypeReference<>() {};
private final ApiClient apiClient;
private final Request request;
/**
* Start/Resume Playback request
* @param apiClient The API client
*/
public StartUsersPlaybackRequest(ApiClient apiClient) {
this.apiClient = apiClient;
this.request = new Request("PUT", "/me/player/play")
;
}
/**
* @param deviceId The id of the device this command is targeting. If not supplied, the user's currently active device is the target.
* @return this request
*/
public StartUsersPlaybackRequest deviceId(String deviceId) {
this.request.addQueryParameter("device_id", String.valueOf(deviceId));
return this;
}
/**
* @param contextUri Optional. Spotify URI of the context to play. Valid contexts are albums, artists & playlists. {context_uri:"spotify:album:1Je1IMUlBXcx1Fz0WE7oPT"}
* @return this request
*/
public StartUsersPlaybackRequest contextUri(String contextUri) {
this.request.addBodyParameter("context_uri", contextUri);
return this;
}
/**
* @param uris Optional. A JSON array of the Spotify track URIs to play. For example: {"uris": ["spotify:track:4iV5W9uYEdYUVa79Axb7Rh", "spotify:track:1301WleyT98MSxVHPZCA6M"]}
* @return this request
*/
public StartUsersPlaybackRequest uris(java.util.List uris) {
this.request.addBodyParameter("uris", uris);
return this;
}
/**
* @param offset Optional. Indicates from where in the context playback should start. Only available when context_uri corresponds to an album or playlist object "position" is zero based and can’t be negative. Example: "offset": {"position": 5}
"uri" is a string representing the uri of the item to start at. Example: "offset": {"uri": "spotify:track:1301WleyT98MSxVHPZCA6M"}
* @return this request
*/
public StartUsersPlaybackRequest offset(java.util.Map offset) {
this.request.addBodyParameter("offset", offset);
return this;
}
/**
* @param positionMs Indicates from what position to start playback. Must be a positive number. Passing in a position that is greater than the length of the track will cause the player to start playing the next song.
* @return this request
*/
public StartUsersPlaybackRequest positionMs(int positionMs) {
this.request.addBodyParameter("position_ms", positionMs);
return this;
}
/**
* Build the request into an executable api call
* @return an executable api call
*/
public ApiCall build() {
return apiClient.createApiCall(request, RESPONSE_TYPE);
}
}