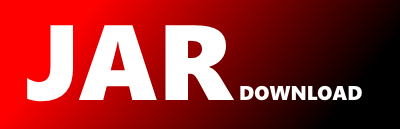
de.sonallux.spotify.api.apis.playlists.AddTracksToPlaylistRequest Maven / Gradle / Ivy
Show all versions of spotify-web-api-java Show documentation
package de.sonallux.spotify.api.apis.playlists;
import com.fasterxml.jackson.core.type.TypeReference;
import de.sonallux.spotify.api.http.ApiCall;
import de.sonallux.spotify.api.http.ApiClient;
import de.sonallux.spotify.api.http.Request;
import de.sonallux.spotify.api.models.*;
/**
* Add Items to Playlist request
*
* Required OAuth scopes
* playlist-modify-public, playlist-modify-private
*
* Response
* A snapshot ID for the playlist
*/
public class AddTracksToPlaylistRequest {
private static final TypeReference RESPONSE_TYPE = new TypeReference<>() {};
private final ApiClient apiClient;
private final Request request;
/**
* Add Items to Playlist request
* @param apiClient The API client
* @param playlistId The Spotify ID of the playlist.
* @param uris A JSON array of the Spotify URIs to add. For example: {"uris": ["spotify:track:4iV5W9uYEdYUVa79Axb7Rh","spotify:track:1301WleyT98MSxVHPZCA6M", "spotify:episode:512ojhOuo1ktJprKbVcKyQ"]}
A maximum of 100 items can be added in one request. Note: if the uris
parameter is present in the query string, any URIs listed here in the body will be ignored.
*/
public AddTracksToPlaylistRequest(ApiClient apiClient, String playlistId, java.util.List uris) {
this.apiClient = apiClient;
this.request = new Request("POST", "/playlists/{playlist_id}/tracks")
.addPathParameter("playlist_id", String.valueOf(playlistId))
.addBodyParameter("uris", uris)
;
}
/**
* @param position The position to insert the items, a zero-based index. For example, to insert the items in the first position: position=0
; to insert the items in the third position: position=2
. If omitted, the items will be appended to the playlist. Items are added in the order they appear in the uris array. For example: {"uris": ["spotify:track:4iV5W9uYEdYUVa79Axb7Rh","spotify:track:1301WleyT98MSxVHPZCA6M"], "position": 3}
* @return this request
*/
public AddTracksToPlaylistRequest position(int position) {
this.request.addBodyParameter("position", position);
return this;
}
/**
* Build the request into an executable api call
* @return an executable api call
*/
public ApiCall build() {
return apiClient.createApiCall(request, RESPONSE_TYPE);
}
}