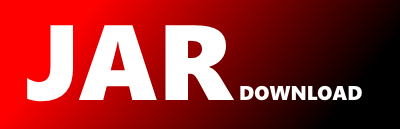
de.sonallux.spotify.api.models.AudioAnalysisTrack Maven / Gradle / Ivy
Show all versions of spotify-web-api-java Show documentation
package de.sonallux.spotify.api.models;
import lombok.*;
/**
*
*/
@Getter
@Setter
@NoArgsConstructor
public class AudioAnalysisTrack {
/**
* The number of channels used for analysis. If 1, all channels are summed together to mono before analysis.
*/
public int analysisChannels;
/**
* The sample rate used to decode and analyze this track. May differ from the actual sample rate of this track available on Spotify.
*/
public int analysisSampleRate;
/**
* A version number for the Echo Nest Musical Fingerprint format used in the codestring field.
*/
public float codeVersion;
/**
* An Echo Nest Musical Fingerprint (ENMFP) codestring for this track.
*/
public String codestring;
/**
* Length of the track in seconds.
*/
public float duration;
/**
* A version number for the EchoPrint format used in the echoprintstring field.
*/
public float echoprintVersion;
/**
* An EchoPrint codestring for this track.
*/
public String echoprintstring;
/**
* The time, in seconds, at which the track's fade-in period ends. If the track has no fade-in, this will be 0.0.
*/
public float endOfFadeIn;
/**
* The key the track is in. Integers map to pitches using standard Pitch Class notation. E.g. 0 = C, 1 = C♯/D♭, 2 = D, and so on. If no key was detected, the value is -1.
*/
public int key;
/**
* The confidence, from 0.0 to 1.0, of the reliability of the key
.
*/
public float keyConfidence;
/**
* The overall loudness of a track in decibels (dB). Loudness values are averaged across the entire track and are useful for comparing relative loudness of tracks. Loudness is the quality of a sound that is the primary psychological correlate of physical strength (amplitude). Values typically range between -60 and 0 db.
*/
public float loudness;
/**
* Mode indicates the modality (major or minor) of a track, the type of scale from which its melodic content is derived. Major is represented by 1 and minor is 0.
*/
public int mode;
/**
* The confidence, from 0.0 to 1.0, of the reliability of the mode
.
*/
public float modeConfidence;
/**
* The exact number of audio samples analyzed from this track. See also analysis_sample_rate
.
*/
public int numSamples;
/**
* An offset to the start of the region of the track that was analyzed. (As the entire track is analyzed, this should always be 0.)
*/
public int offsetSeconds;
/**
* A version number for the Rhythmstring used in the rhythmstring field.
*/
public float rhythmVersion;
/**
* A Rhythmstring for this track. The format of this string is similar to the Synchstring.
*/
public String rhythmstring;
/**
* This field will always contain the empty string.
*/
public String sampleMd5;
/**
* The time, in seconds, at which the track's fade-out period starts. If the track has no fade-out, this should match the track's length.
*/
public float startOfFadeOut;
/**
* A version number for the Synchstring used in the synchstring field.
*/
public float synchVersion;
/**
* A Synchstring for this track.
*/
public String synchstring;
/**
* The overall estimated tempo of a track in beats per minute (BPM). In musical terminology, tempo is the speed or pace of a given piece and derives directly from the average beat duration.
*/
public float tempo;
/**
* The confidence, from 0.0 to 1.0, of the reliability of the tempo
.
*/
public float tempoConfidence;
/**
* An estimated time signature. The time signature (meter) is a notational convention to specify how many beats are in each bar (or measure). The time signature ranges from 3 to 7 indicating time signatures of "3/4", to "7/4".
*/
public int timeSignature;
/**
* The confidence, from 0.0 to 1.0, of the reliability of the time_signature
.
*/
public float timeSignatureConfidence;
/**
* The length of the region of the track was analyzed, if a subset of the track was analyzed. (As the entire track is analyzed, this should always be 0.)
*/
public int windowSeconds;
}