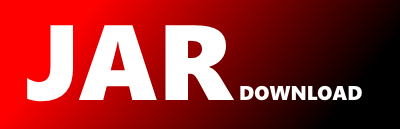
de.sonallux.spotify.api.models.ChapterBase Maven / Gradle / Ivy
Show all versions of spotify-web-api-java Show documentation
package de.sonallux.spotify.api.models;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import lombok.*;
/**
* ChapterBase
*/
@Getter
@Setter
@NoArgsConstructor
@JsonTypeInfo(use = JsonTypeInfo.Id.NONE) // Disable deserialization based on @JsonTypeInfo
public class ChapterBase extends BaseObject {
/**
* A URL to a 30 second preview (MP3 format) of the chapter. null
if not available.
*/
public String audioPreviewUrl;
/**
* A list of the countries in which the chapter can be played, identified by their ISO 3166-1 alpha-2 code.
*/
public java.util.List availableMarkets;
/**
* The number of the chapter
*/
public int chapterNumber;
/**
* A description of the chapter. HTML tags are stripped away from this field, use html_description
field in case HTML tags are needed.
*/
public String description;
/**
* The chapter length in milliseconds.
*/
public int durationMs;
/**
* Whether or not the chapter has explicit content (true = yes it does; false = no it does not OR unknown).
*/
public boolean explicit;
/**
* External URLs for this chapter.
*/
public ExternalUrl externalUrls;
/**
* A description of the chapter. This field may contain HTML tags.
*/
public String htmlDescription;
/**
* The cover art for the chapter in various sizes, widest first.
*/
public java.util.List images;
/**
* True if the chapter is playable in the given market. Otherwise false.
*/
public boolean isPlayable;
/**
* A list of the languages used in the chapter, identified by their ISO 639-1 code.
*/
public java.util.List languages;
/**
* The name of the chapter.
*/
public String name;
/**
* The date the chapter was first released, for example "1981-12-15"
. Depending on the precision, it might be shown as "1981"
or "1981-12"
.
*/
public String releaseDate;
/**
* The precision with which release_date
value is known.
*/
public String releaseDatePrecision;
/**
* Included in the response when a content restriction is applied.
*/
public ChapterRestriction restrictions;
/**
* The user's most recent position in the chapter. Set if the supplied access token is a user token and has the scope 'user-read-playback-position'.
*/
public ResumePoint resumePoint;
}