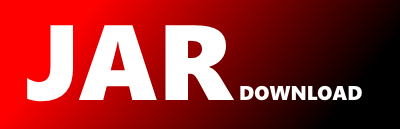
de.sstoehr.pustefix.i18n.model.Message Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pustefix-i18n-maven-plugin Show documentation
Show all versions of pustefix-i18n-maven-plugin Show documentation
A maven plugin to work with PO translation files with Pustefix framework
package de.sstoehr.pustefix.i18n.model;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class Message {
private final String messageId;
private List translations = new ArrayList<>();
public Message(final String messageId) {
if (messageId == null) {
throw new IllegalArgumentException("messageId has to be specified");
}
this.messageId = messageId;
}
public void add(MessageTranslation translation) {
if (translation == null) {
throw new IllegalArgumentException("translation has to be specified");
}
this.translations.add(translation);
}
public String getMessageId() {
return messageId;
}
public List getTranslations() {
List values = new ArrayList<>(translations);
return Collections.unmodifiableList(values);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Message message = (Message) o;
if (!messageId.equals(message.messageId)) {
return false;
}
return true;
}
@Override
public int hashCode() {
return messageId.hashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy