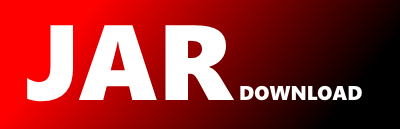
de.team33.libs.exceptional.v3.Wrapping Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lib-exceptional Show documentation
Show all versions of lib-exceptional Show documentation
Provides wrapping and unwrapping checked exceptions.
package de.team33.libs.exceptional.v3;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
/**
* A tool that can turn certain functional constructs that may throw checked exceptions into others that do
* not.
*/
public class Wrapping {
private final XBiFunction delegate;
private Wrapping(final XBiFunction delegate) {
this.delegate = delegate;
}
/**
* Wraps an {@link XRunnable} as {@link Runnable} that, when executed, wraps any occurring
* checked exception as {@link WrappedException}.
*/
public static Runnable runnable(final XRunnable> xRunnable) {
return new Wrapping<>(toBiFunction(xRunnable)).toRunnable();
}
/**
* Wraps an {@link XConsumer} as {@link Consumer} that, when executed, wraps any occurring
* checked exception as {@link WrappedException}.
*/
public static Consumer consumer(final XConsumer xConsumer) {
return new Wrapping<>(toBiFunction(xConsumer)).toConsumer();
}
/**
* Wraps an {@link XBiConsumer} as {@link BiConsumer} that, when executed, wraps any occurring
* checked exception as {@link WrappedException}.
*/
public static BiConsumer biConsumer(final XBiConsumer xBiConsumer) {
return new Wrapping<>(toBiFunction(xBiConsumer)).toBiConsumer();
}
/**
* Wraps an {@link XSupplier} as {@link Supplier} that, when executed, wraps any occurring
* checked exception as {@link WrappedException}.
*/
public static Supplier supplier(final XSupplier xSupplier) {
return new Wrapping<>(toBiFunction(xSupplier)).toSupplier();
}
/**
* Wraps an {@link XFunction} as {@link Function} that, when executed, wraps any occurring
* checked exception as {@link WrappedException}.
*/
public static Function function(final XFunction xFunction) {
return new Wrapping<>(toBiFunction(xFunction)).toFunction();
}
/**
* Wraps an {@link XBiFunction} as {@link BiFunction} that, when executed, wraps any occurring
* checked exception as {@link WrappedException}.
*/
public static BiFunction biFunction(final XBiFunction xBiFunction) {
return new Wrapping<>(xBiFunction).toBiFunction();
}
private static XBiFunction toBiFunction(final XRunnable> xRunnable) {
return (t, u) -> {
xRunnable.run();
return null;
};
}
private static XBiFunction toBiFunction(final XConsumer xConsumer) {
return (t, u) -> {
xConsumer.accept(t);
return null;
};
}
private static XBiFunction toBiFunction(final XBiConsumer xBiConsumer) {
return (t, u) -> {
xBiConsumer.accept(t, u);
return null;
};
}
private static XBiFunction toBiFunction(final XSupplier xSupplier) {
return (t, u) -> xSupplier.get();
}
private static XBiFunction toBiFunction(final XFunction xFunction) {
return (t, u) -> xFunction.apply(t);
}
private R exec(final T t, final U u) {
try {
return delegate.apply(t, u);
} catch (final RuntimeException caught) {
throw caught;
} catch (final Exception caught) {
throw new WrappedException(caught);
}
}
private Runnable toRunnable() {
return () -> exec(null, null);
}
private Consumer toConsumer() {
return t -> exec(t, null);
}
private BiConsumer toBiConsumer() {
return this::exec;
}
private Supplier toSupplier() {
return () -> exec(null, null);
}
private Function toFunction() {
return t -> exec(t, null);
}
private BiFunction toBiFunction() {
return this::exec;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy