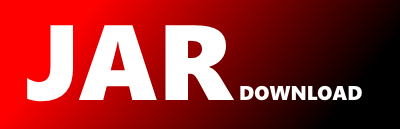
de.team33.patterns.arbitrary.mimas.Charging Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arbitrary-mimas Show documentation
Show all versions of arbitrary-mimas Show documentation
Provides classes that support the generation of arbitrary values of virtually any type.
The newest version!
package de.team33.patterns.arbitrary.mimas;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.lang.reflect.Type;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.BinaryOperator;
import java.util.function.Consumer;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import static java.lang.String.format;
final class Charging extends Supplying {
private static final String METHOD_NOT_APPLICABLE = Util.load(Charging.class, "setterMethodNotApplicable.txt");
private static final String NO_SUPPLIER = Util.load(Charging.class, "noSupplierMethodFound.txt");
private static final Map, List> SETTERS = new ConcurrentHashMap<>(0);
private final T target;
private final Class> targetType;
Charging(final S source, final T target, final Collection ignore) {
super(source, ignore);
this.target = target;
this.targetType = target.getClass();
}
private static List newSettersOf(final Class> targetType) {
return Methods.publicSetters(targetType)
.collect(Collectors.toList());
}
private Stream desiredSetters() {
return SETTERS.computeIfAbsent(targetType, Charging::newSettersOf)
.stream()
.filter(desired);
}
private Consumer
© 2015 - 2024 Weber Informatics LLC | Privacy Policy