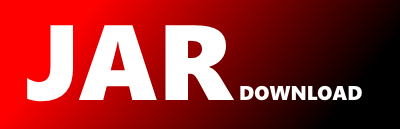
de.team33.patterns.collection.ceres.Collecting Maven / Gradle / Ivy
package de.team33.patterns.collection.ceres;
import de.team33.patterns.building.elara.LateBuilder;
import java.util.AbstractCollection;
import java.util.AbstractList;
import java.util.AbstractSet;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Set;
import java.util.function.Predicate;
import java.util.function.Supplier;
import java.util.stream.Stream;
import static java.util.Arrays.asList;
/**
* {@linkplain Collections Additional} convenience methods to deal with Collections.
*/
@SuppressWarnings({"ProhibitedExceptionCaught", "unused", "ClassWithTooManyMethods"})
public final class Collecting {
private static final Object[] EMPTY_ARRAY = {};
private Collecting() {
}
/**
* Just like {@link Collection#add(Object) subject.add(element)}, but returns the subject.
*
* @throws UnsupportedOperationException if {@link Collection#add(Object)} is not supported by the subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the specified element is {@code null} and the subject
* does not permit {@code null} elements.
* @throws ClassCastException if the class of the specified element prevents it from being
* added to the subject
* (may occur only if used raw or forced in a mismatched class context).
* @throws IllegalArgumentException if some property of the element prevents it from being added to
* the subject.
* @throws IllegalStateException if the element cannot be added at this time due to
* the subject's insertion restrictions (if any).
* @see Collection#add(Object)
* @see Collecting#add(Collection, Object, Object, Object[])
* @see Collecting#addAll(Collection, Collection)
* @see Collecting#addAll(Collection, Iterable)
* @see Collecting#addAll(Collection, Iterator)
* @see Collecting#addAll(Collection, Stream)
* @see Collecting#addAll(Collection, Object[])
*/
public static > C add(final C subject, final E element) {
subject.add(element);
return subject;
}
/**
* Similar to {@link Collecting#add(Collection, Object)}, but allows to add two or more elements at once.
*
* @throws UnsupportedOperationException if {@link Collection#add(Object)} is not supported by the subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@code array} of more elements is {@code null} or ...
* @throws NullPointerException if any of the specified elements is {@code null} and the
* subject does not permit {@code null} elements.
* @throws ClassCastException if the class of the specified elements prevents them from being
* added to the subject
* (may occur only if used raw or forced in a mismatched class context).
* @throws IllegalArgumentException if some property of some elements prevents them from being
* added to the subject.
* @throws IllegalStateException if the elements cannot be added at this time due to
* the subject's insertion restrictions (if any).
* @see Collection#add(Object)
* @see Collecting#add(Collection, Object)
* @see Collecting#addAll(Collection, Collection)
* @see Collecting#addAll(Collection, Iterable)
* @see Collecting#addAll(Collection, Iterator)
* @see Collecting#addAll(Collection, Stream)
* @see Collecting#addAll(Collection, Object[])
*/
@SafeVarargs
public static > C add(final C subject,
final E element0, final E element1, final E... more) {
return addAll(subject, Stream.concat(Stream.of(element0, element1), Stream.of(more)));
}
/**
* Just like {@link Collection#addAll(Collection) subject.addAll(elements)}, but returns the subject.
*
* @throws UnsupportedOperationException if {@link Collection#addAll(Collection)} is not supported by the
* subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Collection} of elements is {@code null} or ...
* @throws NullPointerException if any of the specified elements is {@code null} and the
* subject does not permit {@code null} elements.
* @throws ClassCastException if the class of the elements prevents them from being added to the
* subject
* (may occur only if used raw or forced in a mismatched class context).
* @throws IllegalArgumentException if some property of some elements prevents them from being added
* to the subject.
* @throws IllegalStateException if the elements cannot be added at this time due to the
* subject's insertion restrictions (if any).
* @see Collection#addAll(Collection)
* @see Collecting#add(Collection, Object)
* @see Collecting#add(Collection, Object, Object, Object[])
* @see Collecting#addAll(Collection, Stream)
* @see Collecting#addAll(Collection, Iterable)
* @see Collecting#addAll(Collection, Iterator)
* @see Collecting#addAll(Collection, Object[])
*/
public static > C addAll(final C subject, final Collection extends E> elements) {
subject.addAll(elements);
return subject;
}
/**
* Similar to {@link Collecting#addAll(Collection, Collection)}, but takes a {@link Stream} as second argument.
*
* @throws UnsupportedOperationException if {@link Collection#add(Object)} is not supported by the subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Stream} of elements is {@code null} or ...
* @throws NullPointerException if any of the streamed elements is {@code null} and the
* subject does not permit {@code null} elements.
* @throws ClassCastException if the class of the elements prevents them from being added to the
* subject
* (may occur only if used raw or forced in a mismatched class context).
* @throws IllegalArgumentException if some property of some elements prevents them from being added
* to the subject.
* @throws IllegalStateException if the elements cannot be added at this time due to the
* subject's insertion restrictions (if any).
* @see Collection#add(Object)
* @see Collecting#add(Collection, Object)
* @see Collecting#add(Collection, Object, Object, Object[])
* @see Collecting#addAll(Collection, Collection)
* @see Collecting#addAll(Collection, Iterable)
* @see Collecting#addAll(Collection, Iterator)
* @see Collecting#addAll(Collection, Object[])
*/
public static > C addAll(final C subject, final Stream extends E> elements) {
elements.forEach(subject::add);
return subject;
}
/**
* Similar to {@link Collecting#addAll(Collection, Collection)}, but takes an {@link Iterable} as second argument.
*
* @throws UnsupportedOperationException if {@link Collection#add(Object)} or {@link Collection#addAll(Collection)}
* is not supported by the subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Iterable} of elements is {@code null} or ...
* @throws NullPointerException if any of the specified elements is {@code null} and the
* subject does not permit {@code null} elements.
* @throws ClassCastException if the class of the elements prevents them from being added to the
* subject
* (may occur only if used raw or forced in a mismatched class context).
* @throws IllegalArgumentException if some property of some elements prevents them from being added
* to the subject.
* @throws IllegalStateException if the elements cannot be added at this time due to the
* subject's insertion restrictions (if any).
* @see Collection#add(Object)
* @see Collection#addAll(Collection)
* @see Collecting#add(Collection, Object)
* @see Collecting#add(Collection, Object, Object, Object[])
* @see Collecting#addAll(Collection, Collection)
* @see Collecting#addAll(Collection, Stream)
* @see Collecting#addAll(Collection, Iterator)
* @see Collecting#addAll(Collection, Object[])
*/
public static > C addAll(final C subject, final Iterable extends E> elements) {
return (elements instanceof Collection>)
? addAll(subject, (Collection extends E>) elements)
: addAll(subject, elements.iterator());
}
/**
* Similar to {@link Collecting#addAll(Collection, Collection)}, but takes an {@link Iterator} as second argument.
*
* @throws UnsupportedOperationException if {@link Collection#add(Object)} is not supported by the subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Iterator} of elements is {@code null} or ...
* @throws NullPointerException if any of the iterated elements is {@code null} and the
* subject does not permit {@code null} elements.
* @throws ClassCastException if the class of the elements prevents them from being added to the
* subject
* (may occur only if used raw or forced in a mismatched class context).
* @throws IllegalArgumentException if some property of some elements prevents them from being added
* to the subject.
* @throws IllegalStateException if the elements cannot be added at this time due to the
* subject's insertion restrictions (if any).
* @see Collection#add(Object)
* @see Collecting#add(Collection, Object)
* @see Collecting#add(Collection, Object, Object, Object[])
* @see Collecting#addAll(Collection, Collection)
* @see Collecting#addAll(Collection, Stream)
* @see Collecting#addAll(Collection, Iterable)
* @see Collecting#addAll(Collection, Object[])
*/
public static > C addAll(final C subject, final Iterator extends E> elements) {
while (elements.hasNext()) {
subject.add(elements.next());
}
return subject;
}
/**
* Similar to {@link Collecting#addAll(Collection, Collection)}, but takes an {@code array} as second argument.
*
* @throws UnsupportedOperationException if {@link Collection#addAll(Collection)} is not supported by the
* subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@code array} of elements is {@code null} or ...
* @throws NullPointerException if any of the specified elements is {@code null} and the
* subject does not permit {@code null} elements.
* @throws ClassCastException if the class of the elements prevents them from being added to the
* subject
* (may occur only if used raw or forced in a mismatched class context).
* @throws IllegalArgumentException if some property of some elements prevents them from being added
* to the subject.
* @throws IllegalStateException if the elements cannot be added at this time due to the
* subject's insertion restrictions (if any).
* @see Collection#add(Object)
* @see Collecting#add(Collection, Object)
* @see Collecting#add(Collection, Object, Object, Object[])
* @see Collecting#addAll(Collection, Collection)
* @see Collecting#addAll(Collection, Stream)
* @see Collecting#addAll(Collection, Iterable)
* @see Collecting#addAll(Collection, Iterator)
*/
public static > C addAll(final C subject, final E[] elements) {
return addAll(subject, Arrays.asList(elements));
}
/**
* Just like {@link Collection#clear() subject.clear()}, but returns the subject.
*
* @throws NullPointerException if subject is {@code null}.
* @throws UnsupportedOperationException if {@link Collection#clear()} is not supported by the subject.
* @see Collection#clear()
*/
public static > C clear(final C subject) {
subject.clear();
return subject;
}
/**
* Similar to {@link Collection#remove(Object) subject.remove(element)}, but returns the subject.
*
* If subject contains the element several times, each occurrence will be removed!
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#remove(Object)} when the subject does not support the requested element.
*
* @throws UnsupportedOperationException if {@link Collection#removeAll(Collection)} is not supported by the
* subject.
* @throws NullPointerException if subject is {@code null}.
* @see Collection#remove(Object)
* @see Collection#removeAll(Collection)
* @see Collecting#remove(Collection, Object, Object, Object...)
* @see Collecting#removeAll(Collection, Collection)
* @see Collecting#removeAll(Collection, Stream)
* @see Collecting#removeAll(Collection, Iterable)
* @see Collecting#removeAll(Collection, Iterator)
* @see Collecting#removeAll(Collection, Object[])
*/
public static > C remove(final C subject, final Object element) {
try {
subject.removeAll(Collections.singleton(element));
} catch (final NullPointerException | ClassCastException caught) {
if (null == subject) {
throw caught; // expected to be a NullPointerException
}
// --> can not contain
// --> simply does not contain
// --> Nothing else to do.
}
return subject;
}
/**
* Similar to {@link Collecting#remove(Collection, Object)}, but allows to remove two or more elements.
*
* If subject contains some of the elements several times, each occurrence will be removed!
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#remove(Object)} or {@link Collection#removeAll(Collection)} when the subject does not
* support some requested elements.
*
* @throws NullPointerException if subject is {@code null} or if the {@code array} of {@code more}
* elements is {@code null}.
* @throws UnsupportedOperationException if {@link Collection#removeAll(Collection)} is not supported by the
* subject.
* @see Collection#remove(Object)
* @see Collection#removeAll(Collection)
* @see Collecting#remove(Collection, Object)
* @see Collecting#removeAll(Collection, Collection)
* @see Collecting#removeAll(Collection, Stream)
* @see Collecting#removeAll(Collection, Iterable)
* @see Collecting#removeAll(Collection, Iterator)
* @see Collecting#removeAll(Collection, Object[])
*/
public static > C remove(final C subject,
final Object element0,
final Object element1,
final Object... more) {
return removeAll(subject, Stream.concat(Stream.of(element0, element1), Stream.of(more)));
}
/**
* Just like {@link Collection#removeAll(Collection) subject.removeAll(elements)}, but returns the subject.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#removeAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws UnsupportedOperationException if {@link Collection#removeAll(Collection)} is not supported by the
* subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Collection} of elements is {@code null}.
* @see Collection#removeAll(Collection)
* @see Collecting#remove(Collection, Object)
* @see Collecting#remove(Collection, Object, Object, Object...)
* @see Collecting#removeAll(Collection, Stream)
* @see Collecting#removeAll(Collection, Iterable)
* @see Collecting#removeAll(Collection, Iterator)
* @see Collecting#removeAll(Collection, Object[])
*/
public static > C removeAll(final C subject, final Collection> elements) {
try {
subject.removeAll(elements);
} catch (final NullPointerException | ClassCastException caught) {
if ((null == subject) || (null == elements)) {
throw caught; // expected to be a NullPointerException
} else {
// --> or can not contain an element
// --> or simply does not contain that element
// --> removal may be incomplete, retry in a more secure way ...
removeAll(subject, retainAll(new HashSet<>(elements), subject));
}
}
return subject;
}
/**
* Similar to {@link Collecting#removeAll(Collection, Collection)}, but takes a {@link Stream} as second argument.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#removeAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws UnsupportedOperationException if {@link Collection#removeAll(Collection)} is not supported by the
* subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Stream} of elements is {@code null}.
* @see Collection#removeAll(Collection)
* @see Collecting#remove(Collection, Object)
* @see Collecting#remove(Collection, Object, Object, Object...)
* @see Collecting#removeAll(Collection, Collection)
* @see Collecting#removeAll(Collection, Iterable)
* @see Collecting#removeAll(Collection, Iterator)
* @see Collecting#removeAll(Collection, Object[])
*/
public static > C removeAll(final C subject, final Stream> elements) {
return removeAll(subject, addAll(new HashSet<>(), elements.filter(subject::contains)));
}
/**
* Similar to {@link Collecting#removeAll(Collection, Collection)}, but takes an {@link Iterable} as second
* argument.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#removeAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws UnsupportedOperationException if {@link Collection#removeAll(Collection)} is not supported by the
* subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Iterable} of elements is {@code null}.
* @see Collection#removeAll(Collection)
* @see Collecting#remove(Collection, Object)
* @see Collecting#remove(Collection, Object, Object, Object...)
* @see Collecting#removeAll(Collection, Collection)
* @see Collecting#removeAll(Collection, Stream)
* @see Collecting#removeAll(Collection, Iterator)
* @see Collecting#removeAll(Collection, Object[])
*/
public static > C removeAll(final C subject, final Iterable> elements) {
return (elements instanceof Collection>)
? removeAll(subject, (Collection>) elements)
: removeAll(subject, elements.iterator());
}
/**
* Similar to {@link Collecting#removeAll(Collection, Collection)}, but takes an {@link Iterator} as second
* argument.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#removeAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws UnsupportedOperationException if {@link Collection#removeAll(Collection)} is not supported by the
* subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Iterator} of elements is {@code null}.
* @see Collection#removeAll(Collection)
* @see Collecting#remove(Collection, Object)
* @see Collecting#remove(Collection, Object, Object, Object...)
* @see Collecting#removeAll(Collection, Collection)
* @see Collecting#removeAll(Collection, Iterable)
* @see Collecting#removeAll(Collection, Stream)
* @see Collecting#removeAll(Collection, Object[])
*/
public static > C removeAll(final C subject, final Iterator> elements) {
return removeAll(subject, addAll(new HashSet<>(), elements));
}
/**
* Similar to {@link Collecting#removeAll(Collection, Collection)}, but takes an {@code array} as second
* argument.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#removeAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws UnsupportedOperationException if {@link Collection#removeAll(Collection)} is not supported by the
* subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@code array} of elements is {@code null}.
* @see Collection#removeAll(Collection)
* @see Collecting#remove(Collection, Object)
* @see Collecting#remove(Collection, Object, Object, Object...)
* @see Collecting#removeAll(Collection, Collection)
* @see Collecting#removeAll(Collection, Stream)
* @see Collecting#removeAll(Collection, Iterable)
* @see Collecting#removeAll(Collection, Iterator)
*/
public static > C removeAll(final C subject, final Object[] elements) {
return removeAll(subject, asList(elements));
}
/**
* Just like {@link Collection#removeIf(Predicate) subject.removeIf(filter)}, but returns the subject.
*
* @throws UnsupportedOperationException if {@link Collection#removeIf(Predicate)} is not supported by the
* subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the filter is {@code null}.
* @see Collection#removeIf(Predicate)
*/
public static > C removeIf(final C subject, final Predicate super E> filter) {
subject.removeIf(filter);
return subject;
}
/**
* Just like {@link Collection#retainAll(Collection) subject.retainAll(elements)}, but returns the subject.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#retainAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws UnsupportedOperationException if {@link Collection#retainAll(Collection)} is not supported by the
* subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Collection} of elements is {@code null}.
* @see Collection#retainAll(Collection)
* @see Collecting#retainAll(Collection, Stream)
* @see Collecting#retainAll(Collection, Iterable)
* @see Collecting#retainAll(Collection, Iterator)
* @see Collecting#retainAll(Collection, Object[])
*/
public static > C retainAll(final C subject, final Collection> elements) {
try {
subject.retainAll(elements);
} catch (final NullPointerException | ClassCastException caught) {
if ((null == subject) || (null == elements)) {
throw caught; // expected to be a NullPointerException
} else {
// --> or can not contain an element
// --> or simply does not contain that element
// --> removal may be incomplete, retry in a more secure way ...
retainAll(subject, retainAll(new HashSet<>(elements), subject));
}
}
return subject;
}
/**
* Similar to {@link Collecting#retainAll(Collection, Collection)}, but takes a {@link Stream} as second argument.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#retainAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws UnsupportedOperationException if {@link Collection#retainAll(Collection)} is not supported by the
* subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Stream} of elements is {@code null}.
* @see Collection#retainAll(Collection)
* @see Collecting#retainAll(Collection, Collection)
* @see Collecting#retainAll(Collection, Iterable)
* @see Collecting#retainAll(Collection, Iterator)
* @see Collecting#retainAll(Collection, Object[])
*/
public static > C retainAll(final C subject, final Stream> elements) {
return retainAll(subject, addAll(new HashSet<>(), elements));
}
/**
* Similar to {@link Collecting#retainAll(Collection, Collection)},
* but takes an {@link Iterable} as second argument.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#retainAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws UnsupportedOperationException if {@link Collection#retainAll(Collection)} is not supported by the
* subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Iterable} of elements is {@code null}.
* @see Collection#retainAll(Collection)
* @see Collecting#retainAll(Collection, Collection)
* @see Collecting#retainAll(Collection, Stream)
* @see Collecting#retainAll(Collection, Iterator)
* @see Collecting#retainAll(Collection, Object[])
*/
public static > C retainAll(final C subject, final Iterable> elements) {
return (elements instanceof Collection>)
? retainAll(subject, (Collection>) elements)
: retainAll(subject, elements.iterator());
}
/**
* Similar to {@link Collecting#retainAll(Collection, Collection)},
* but takes an {@link Iterator} as second argument.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#retainAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws UnsupportedOperationException if {@link Collection#retainAll(Collection)} is not supported by the
* subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Iterator} of elements is {@code null}.
* @see Collection#retainAll(Collection)
* @see Collecting#retainAll(Collection, Collection)
* @see Collecting#retainAll(Collection, Stream)
* @see Collecting#retainAll(Collection, Iterable)
* @see Collecting#retainAll(Collection, Object[])
*/
public static > C retainAll(final C subject, final Iterator> elements) {
return retainAll(subject, addAll(new HashSet<>(), elements));
}
/**
* Similar to {@link Collecting#retainAll(Collection, Collection)}, but takes an {@code array} as second
* argument.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#retainAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws UnsupportedOperationException if {@link Collection#retainAll(Collection)} is not supported by the
* subject.
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@code array} of elements is {@code null}.
* @see Collection#retainAll(Collection)
* @see Collecting#retainAll(Collection, Collection)
* @see Collecting#retainAll(Collection, Stream)
* @see Collecting#retainAll(Collection, Iterable)
* @see Collecting#retainAll(Collection, Iterator)
*/
public static > C retainAll(final C subject, final Object[] elements) {
return retainAll(subject, asList(elements));
}
/**
* Just like {@link Collection#contains(Object) subject.contains(element)}.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#contains(Object)} when the subject does not support the requested element.
*
* @throws NullPointerException if subject is {@code null}.
* @see Collection#contains(Object)
* @see Collection#containsAll(Collection)
* @see Collecting#contains(Collection, Object, Object, Object...)
* @see Collecting#containsAll(Collection, Collection)
* @see Collecting#containsAll(Collection, Stream)
* @see Collecting#containsAll(Collection, Iterable)
* @see Collecting#containsAll(Collection, Iterator)
* @see Collecting#containsAll(Collection, Object[])
*/
@SuppressWarnings("OverloadedMethodsWithSameNumberOfParameters")
public static boolean contains(final Collection> subject, final Object element) {
try {
return subject.contains(element);
} catch (final NullPointerException | ClassCastException caught) {
if (null == subject) {
throw caught; // expected to be a NullPointerException
} else {
// --> can not contain
// --> simply does not contain ...
return false;
}
}
}
/**
* Similar to {@link Collecting#contains(Collection, Object)}, but allows to test two or more elements.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#containsAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@code array} of {@code more} elements is {@code null}.
* @see Collection#contains(Object)
* @see Collection#containsAll(Collection)
* @see Collecting#contains(Collection, Object)
* @see Collecting#containsAll(Collection, Collection)
* @see Collecting#containsAll(Collection, Stream)
* @see Collecting#containsAll(Collection, Iterable)
* @see Collecting#containsAll(Collection, Iterator)
* @see Collecting#containsAll(Collection, Object[])
*/
public static boolean contains(final Collection> subject,
final Object element0, final Object element1, final Object... more) {
return containsAll(subject, Stream.concat(Stream.of(element0, element1), Stream.of(more)));
}
/**
* Just like {@link Collection#containsAll(Collection) subject.containsAll(elements)},
* but returns the subject.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#containsAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Collection} of elements is {@code null}.
* @see Collection#contains(Object)
* @see Collection#containsAll(Collection)
* @see Collecting#contains(Collection, Object)
* @see Collecting#contains(Collection, Object, Object, Object...)
* @see Collecting#containsAll(Collection, Stream)
* @see Collecting#containsAll(Collection, Iterable)
* @see Collecting#containsAll(Collection, Iterator)
* @see Collecting#containsAll(Collection, Object[])
*/
public static boolean containsAll(final Collection> subject, final Collection> elements) {
try {
return subject.containsAll(elements);
} catch (final NullPointerException | ClassCastException caught) {
if ((null == subject) || (null == elements)) {
// is expected to be a NullPointerException ...
throw caught;
} else {
// --> can not contain all
// --> simply does not contain all ...
return false;
}
}
}
/**
* Similar to {@link Collecting#containsAll(Collection, Collection)}, but takes a {@link Stream} as second argument.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#containsAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Collection} of elements is {@code null}.
* @see Collection#contains(Object)
* @see Collection#containsAll(Collection)
* @see Collecting#contains(Collection, Object)
* @see Collecting#contains(Collection, Object, Object, Object...)
* @see Collecting#containsAll(Collection, Collection)
* @see Collecting#containsAll(Collection, Iterable)
* @see Collecting#containsAll(Collection, Iterator)
* @see Collecting#containsAll(Collection, Object[])
*/
public static boolean containsAll(final Collection> subject, final Stream> elements) {
return containsAll(subject, addAll(new HashSet<>(), elements));
}
/**
* Similar to {@link Collecting#containsAll(Collection, Collection)},
* but takes an {@link Iterable} as second argument.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#containsAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Collection} of elements is {@code null}.
* @see Collection#contains(Object)
* @see Collection#containsAll(Collection)
* @see Collecting#contains(Collection, Object)
* @see Collecting#contains(Collection, Object, Object, Object...)
* @see Collecting#containsAll(Collection, Collection)
* @see Collecting#containsAll(Collection, Stream)
* @see Collecting#containsAll(Collection, Iterator)
* @see Collecting#containsAll(Collection, Object[])
*/
public static boolean containsAll(final Collection> subject, final Iterable> elements) {
return (elements instanceof Collection>)
? containsAll(subject, (Collection>) elements)
: containsAll(subject, elements.iterator());
}
/**
* Similar to {@link Collecting#containsAll(Collection, Collection)},
* but takes an {@link Iterator} as second argument.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#containsAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Collection} of elements is {@code null}.
* @see Collection#contains(Object)
* @see Collection#containsAll(Collection)
* @see Collecting#contains(Collection, Object)
* @see Collecting#contains(Collection, Object, Object, Object...)
* @see Collecting#containsAll(Collection, Collection)
* @see Collecting#containsAll(Collection, Stream)
* @see Collecting#containsAll(Collection, Iterable)
* @see Collecting#containsAll(Collection, Object[])
*/
public static boolean containsAll(final Collection> subject, final Iterator> elements) {
return containsAll(subject, addAll(new HashSet<>(), elements));
}
/**
* Similar to {@link Collecting#containsAll(Collection, Collection)}, but takes an {@code array} as second argument.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#containsAll(Collection)} when the subject does not support some requested
* elements.
*
* @throws NullPointerException if subject is {@code null} or ...
* @throws NullPointerException if the {@link Collection} of elements is {@code null}.
* @see Collection#contains(Object)
* @see Collection#containsAll(Collection)
* @see Collecting#contains(Collection, Object)
* @see Collecting#contains(Collection, Object, Object, Object...)
* @see Collecting#containsAll(Collection, Collection)
* @see Collecting#containsAll(Collection, Stream)
* @see Collecting#containsAll(Collection, Iterable)
* @see Collecting#containsAll(Collection, Iterator)
*/
public static boolean containsAll(final Collection> subject, final Object[] elements) {
return containsAll(subject, asList(elements));
}
/**
* Supplies a proxy for a given subject that may be used to implement some {@link Collection}-specific
* methods, e.g.:
*
* - {@link Collection#toArray()}
* - {@link Collection#toArray(Object[])}
* - {@link Object#toString()}
* - ...
*
*
* @param subject A {@link Collection}, that at least provides independently ...
*
* - {@link Collection#iterator()}
* - {@link Collection#size()}
*
*/
@SuppressWarnings("ReturnOfInnerClass")
public static Collection proxy(final Collection subject) {
// noinspection AnonymousInnerClass
return new AbstractCollection() {
@Override
public Iterator iterator() {
return subject.iterator();
}
@Override
public int size() {
return subject.size();
}
};
}
/**
* Supplies a proxy for a given {@link List subject} that may be used to implement some {@link List}-specific
* methods, e.g.:
*
* - {@link List#toArray()}
* - {@link List#toArray(Object[])}
* - {@link Object#toString()}
* - {@link List#equals(Object)}
* - {@link List#hashCode()}
* - ...
*
*
* @param subject A {@link List}, that at least provides independently ...
*
* - {@link List#get(int)}
* - {@link List#size()}
*
*/
@SuppressWarnings("ReturnOfInnerClass")
public static List proxy(final List subject) {
// noinspection AnonymousInnerClass
return new AbstractList() {
@Override
public E get(final int index) {
return subject.get(index);
}
@Override
public int size() {
return subject.size();
}
};
}
/**
* Supplies a proxy for a given {@link Set subject} that may be used to implement some {@link Set}-specific
* methods, e.g.:
*
* - {@link Set#toArray()}
* - {@link Set#toArray(Object[])}
* - {@link Object#toString()}
* - {@link Set#equals(Object)}
* - {@link Set#hashCode()}
* - ...
*
*
* @param subject A {@link Set}, that at least provides independently ...
*
* - {@link Set#iterator()}
* - {@link Set#size()}
*
*/
@SuppressWarnings("ReturnOfInnerClass")
public static Set proxy(final Set subject) {
// noinspection AnonymousInnerClass
return new AbstractSet() {
@Override
public Iterator iterator() {
return subject.iterator();
}
@Override
public int size() {
return subject.size();
}
};
}
@SuppressWarnings("MethodOnlyUsedFromInnerClass")
private static Collection nullAsEmpty(final Collection nullable) {
return (null == nullable) ? Collections.emptySet() : nullable;
}
@SuppressWarnings("MethodOnlyUsedFromInnerClass")
private static Stream nullAsEmpty(final Stream nullable) {
return (null == nullable) ? Stream.empty() : nullable;
}
@SuppressWarnings("MethodOnlyUsedFromInnerClass")
private static Iterable nullAsEmpty(final Iterable nullable) {
return (null == nullable) ? Collections.emptySet() : nullable;
}
@SuppressWarnings("MethodOnlyUsedFromInnerClass")
private static Iterator nullAsEmpty(final Iterator nullable) {
return (null == nullable) ? Collections.emptyIterator() : nullable;
}
@SuppressWarnings({"unchecked", "MethodOnlyUsedFromInnerClass", "SuspiciousArrayCast"})
private static E[] nullAsEmpty(final E[] nullable) {
return (null == nullable) ? (E[]) EMPTY_ARRAY : nullable;
}
/**
* Returns a new {@link Builder} for target instances as supplied by the given {@link Supplier}.
*
* @param The element type.
* @param The final type of the target instances, at least {@link Collection}.
*/
public static > Builder builder(final Supplier newTarget) {
return new Builder<>(newTarget, Builder.class);
}
/**
* Returns a new {@link Charger} for a given target instance.
*
* @param The element type.
* @param The final type of the target instances, at least {@link Collection}.
*/
public static > Charger charger(final C target) {
return new Charger<>(target, Charger.class);
}
/**
* Utility interface to set up a target instance of {@link Collection}.
*
* @param The element type.
* @param The final type of the target instance, at least {@link Collection}.
* @param The final type of the Setup implementation.
*/
@SuppressWarnings("ClassNameSameAsAncestorName")
@FunctionalInterface
public interface Setup, S extends Setup>
extends de.team33.patterns.building.elara.Setup {
/**
* Adds an element to the instance to be set up.
*
* @throws UnsupportedOperationException if {@link Collection#add(Object)} is not supported by the instance
* to be set up.
* @throws NullPointerException if the specified element is {@code null} and the instance
* to be set up does not permit {@code null} elements.
* @throws ClassCastException if the class of the specified element prevents it from being
* added to the instance to be set up
* (may occur only if used raw or forced in a mismatched class context).
* @throws IllegalArgumentException if some property of the element prevents it from being added
* to the instance to be set up.
* @throws IllegalStateException if the element cannot be added at this time due to
* insertion restrictions on the instance to be set up (if any).
* @see Collection#add(Object)
* @see Collecting#add(Collection, Object)
*/
default S add(final E element) {
return setup(target -> Collecting.add(target, element));
}
/**
* Adds two or more elements to the instance to be set up.
*
* If the {@code array} of more elements is {@code null} it will be treated as an empty {@code array}.
*
* @throws UnsupportedOperationException if {@link Collection#add(Object)} is not supported by the instance
* to be set up.
* @throws NullPointerException if any of the specified elements is {@code null} and the
* instance to be set up does not permit {@code null} elements.
* @throws ClassCastException if the class of any specified elements
* prevents them from being added to the instance to be set up
* (may occur only if used raw or forced in a mismatched class context).
* @throws IllegalArgumentException if some property of any elements prevents
* them from being added to the instance to be set up.
* @throws IllegalStateException if any of the elements cannot be added at this time
* due to insertion restrictions of the instance to be set up (if any).
* @see #add(Object)
* @see Collecting#add(Collection, Object, Object, Object[])
*/
@SuppressWarnings("unchecked")
default S add(final E element0, final E element1, final E... more) {
return setup(target -> Collecting.add(target, element0, element1, nullAsEmpty(more)));
}
/**
* Adds multiple elements to the instance to be set up.
*
* If the {@link Collection} of elements is {@code null} it will be treated as an empty
* {@link Collection}.
*
* @throws UnsupportedOperationException if {@link Collection#addAll(Collection)} is not supported by the
* instance to be set up.
* @throws NullPointerException if any of the specified elements is {@code null} and the
* instance to be set up does not permit {@code null} elements.
* @throws ClassCastException if the class of any specified elements
* prevents them from being added to the instance to be set up
* (may occur only if used raw or forced in a mismatched class context).
* @throws IllegalArgumentException if some property of any elements prevents
* them from being added to the instance to be set up.
* @throws IllegalStateException if any of the elements cannot be added at this time
* due to insertion restrictions of the instance to be set up (if any).
* @see Collection#addAll(Collection)
* @see Collecting#addAll(Collection, Collection)
* @see #addAll(Stream)
* @see #addAll(Iterable)
* @see #addAll(Iterator)
* @see #addAll(Object[])
*/
default S addAll(final Collection extends E> elements) {
return setup(target -> Collecting.addAll(target, nullAsEmpty(elements)));
}
/**
* Adds multiple elements to the instance to be set up.
*
* If the {@link Stream} of elements is {@code null} it will be treated as an empty {@link Stream}.
*
* @throws UnsupportedOperationException if {@link Collection#add(Object)} is not supported by the
* instance to be set up.
* @throws NullPointerException if any of the specified elements is {@code null} and the
* instance to be set up does not permit {@code null} elements.
* @throws ClassCastException if the class of any specified elements
* prevents them from being added to the instance to be set up
* (may occur only if used raw or forced in a mismatched class context).
* @throws IllegalArgumentException if some property of any elements prevents
* them from being added to the instance to be set up.
* @throws IllegalStateException if any of the elements cannot be added at this time
* due to insertion restrictions of the instance to be set up (if any).
* @see Collecting#addAll(Collection, Stream)
* @see #addAll(Collection)
* @see #addAll(Iterable)
* @see #addAll(Iterator)
* @see #addAll(Object[])
*/
default S addAll(final Stream extends E> elements) {
return setup(target -> Collecting.addAll(target, nullAsEmpty(elements)));
}
/**
* Adds multiple elements to the instance to be set up.
*
* Treats a {@code null}-argument just like an empty {@link Iterable}.
*
* @throws UnsupportedOperationException if {@link Collection#add(Object)} or
* if {@link Collection#addAll(Collection)} is not supported by the
* instance to be set up.
* @throws NullPointerException if any of the specified elements is {@code null} and the
* instance to be set up does not permit {@code null} elements.
* @throws ClassCastException if the class of any specified elements
* prevents them from being added to the instance to be set up
* (may occur only if used raw or forced in a mismatched class context).
* @throws IllegalArgumentException if some property of any elements prevents
* them from being added to the instance to be set up.
* @throws IllegalStateException if any of the elements cannot be added at this time
* due to insertion restrictions of the instance to be set up (if any).
* @see Collecting#addAll(Collection, Iterable)
* @see #addAll(Collection)
* @see #addAll(Stream)
* @see #addAll(Iterator)
* @see #addAll(Object[])
*/
default S addAll(final Iterable extends E> elements) {
return setup(target -> Collecting.addAll(target, nullAsEmpty(elements)));
}
/**
* Adds multiple elements to the instance to be set up.
*
* Treats a {@code null}-argument just like an empty {@link Iterator}.
*
* @throws UnsupportedOperationException if {@link Collection#add(Object)} or
* if {@link Collection#addAll(Collection)} is not supported by the
* instance to be set up.
* @throws NullPointerException if any of the specified elements is {@code null} and the
* instance to be set up does not permit {@code null} elements.
* @throws ClassCastException if the class of any specified elements
* prevents them from being added to the instance to be set up
* (may occur only if used raw or forced in a mismatched class context).
* @throws IllegalArgumentException if some property of any elements prevents
* them from being added to the instance to be set up.
* @throws IllegalStateException if any of the elements cannot be added at this time
* due to insertion restrictions of the instance to be set up (if any).
* @see Collecting#addAll(Collection, Iterable)
* @see #addAll(Collection)
* @see #addAll(Stream)
* @see #addAll(Iterable)
* @see #addAll(Object[])
*/
default S addAll(final Iterator extends E> elements) {
return setup(target -> Collecting.addAll(target, nullAsEmpty(elements)));
}
/**
* Adds multiple elements to the instance to be set up.
*
* Treats a {@code null}-argument just like an empty {@code array}.
*
* @throws UnsupportedOperationException if {@link Collection#add(Object)} or
* if {@link Collection#addAll(Collection)} is not supported by the
* instance to be set up.
* @throws NullPointerException if any of the specified elements is {@code null} and the
* instance to be set up does not permit {@code null} elements.
* @throws ClassCastException if the class of any specified elements
* prevents them from being added to the instance to be set up
* (may occur only if used raw or forced in a mismatched class context).
* @throws IllegalArgumentException if some property of any elements prevents
* them from being added to the instance to be set up.
* @throws IllegalStateException if any of the elements cannot be added at this time
* due to insertion restrictions of the instance to be set up (if any).
* @see Collecting#addAll(Collection, Iterable)
* @see #addAll(Collection)
* @see #addAll(Stream)
* @see #addAll(Iterable)
* @see #addAll(Iterator)
*/
default S addAll(final E[] elements) {
return setup(target -> Collecting.addAll(target, nullAsEmpty(elements)));
}
/**
* Removes an element from the instance to be set up.
*
* If the instance to be set up contains the element several times, each occurrence will be removed!
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#remove(Object)} or {@link Collection#removeAll(Collection)} when the instance to be set up
* does not support the requested element.
*
* @throws UnsupportedOperationException if {@link Collection#remove(Object)} or
* if {@link Collection#removeAll(Collection)} is not supported by the
* instance to be set up.
* @see Collection#remove(Object)
* @see Collecting#remove(Collection, Object)
*/
default S remove(final Object element) {
return setup(target -> Collecting.remove(target, element));
}
/**
* Removes two or more elements from the instance to be set up.
*
* If the instance to be set up contains an element several times, each occurrence will be removed!
*
* @throws UnsupportedOperationException if {@link Collection#remove(Object)} or
* if {@link Collection#removeAll(Collection)} is not supported by the
* instance to be set up.
* @see #remove(Object)
* @see Collecting#remove(Collection, Object, Object, Object[])
*/
default S remove(final Object element0, final Object element1, final Object... more) {
return setup(target -> Collecting.remove(target, element0, element1, nullAsEmpty(more)));
}
/**
* Removes multiple elements from the instance to be set up.
*
* If the instance to be set up contains an element several times, each occurrence will be removed!
*
* Treats a {@code null}-argument just like an empty {@link Collection}.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#remove(Object)} or {@link Collection#removeAll(Collection)} when the instance to be set up
* does not support the requested elements.
*
* @throws UnsupportedOperationException if {@link Collection#remove(Object)} or
* if {@link Collection#removeAll(Collection)} is not supported by the
* instance to be set up.
* @see Collection#removeAll(Collection)
* @see Collecting#removeAll(Collection, Collection)
* @see #removeAll(Stream)
* @see #removeAll(Iterable)
* @see #removeAll(Iterator)
* @see #removeAll(Object[])
*/
default S removeAll(final Collection> elements) {
return setup(target -> Collecting.removeAll(target, nullAsEmpty(elements)));
}
/**
* Removes multiple elements from the instance to be set up.
*
* If the instance to be set up contains an element several times, each occurrence will be removed!
*
* Treats a {@code null}-argument just like an empty {@link Stream}.
*
* @throws UnsupportedOperationException if {@link Collection#remove(Object)} or
* if {@link Collection#removeAll(Collection)} is not supported by the
* instance to be set up.
* @see Collecting#removeAll(Collection, Stream)
* @see #removeAll(Collection)
* @see #removeAll(Iterable)
* @see #removeAll(Iterator)
* @see #removeAll(Object[])
*/
default S removeAll(final Stream> elements) {
return setup(target -> Collecting.removeAll(target, nullAsEmpty(elements)));
}
/**
* Removes multiple elements from the instance to be set up.
*
* If the instance to be set up contains an element several times, each occurrence will be removed!
*
* Treats a {@code null}-argument just like an empty {@link Iterable}.
*
* @throws UnsupportedOperationException if {@link Collection#remove(Object)} or
* if {@link Collection#removeAll(Collection)} is not supported by the
* instance to be set up.
* @see Collecting#removeAll(Collection, Iterable)
* @see #removeAll(Collection)
* @see #removeAll(Stream)
* @see #removeAll(Iterator)
* @see #removeAll(Object[])
*/
default S removeAll(final Iterable> elements) {
return setup(target -> Collecting.removeAll(target, nullAsEmpty(elements)));
}
/**
* Removes multiple elements from the instance to be set up.
*
* If the instance to be set up contains an element several times, each occurrence will be removed!
*
* Treats a {@code null}-argument just like an empty {@link Iterator}.
*
* @throws UnsupportedOperationException if {@link Collection#remove(Object)} or
* if {@link Collection#removeAll(Collection)} is not supported by the
* instance to be set up.
* @see Collecting#removeAll(Collection, Iterable)
* @see #removeAll(Collection)
* @see #removeAll(Stream)
* @see #removeAll(Iterable)
* @see #removeAll(Object[])
*/
default S removeAll(final Iterator> elements) {
return setup(target -> Collecting.removeAll(target, nullAsEmpty(elements)));
}
/**
* Removes multiple elements from the instance to be set up.
*
* If the instance to be set up contains an element several times, each occurrence will be removed!
*
* Treats a {@code null}-argument just like an empty {@code array}.
*
* @throws UnsupportedOperationException if {@link Collection#remove(Object)} or
* if {@link Collection#removeAll(Collection)} is not supported by the
* instance to be set up.
* @see Collecting#removeAll(Collection, Object[])
* @see #removeAll(Collection)
* @see #removeAll(Stream)
* @see #removeAll(Iterable)
* @see #removeAll(Iterator)
*/
default S removeAll(final Object[] elements) {
return setup(target -> Collecting.removeAll(target, nullAsEmpty(elements)));
}
/**
* Removes multiple elements from the instance to be set up.
*
* @throws UnsupportedOperationException if {@link Collection#removeIf(Predicate)} is not supported by the
* instance to be set up.
* @throws NullPointerException if the filter is {@code null}.
* @see Collection#removeIf(Predicate)
* @see Collecting#removeIf(Collection, Predicate)
*/
default S removeIf(final Predicate super E> filter) {
return setup(target -> Collecting.removeIf(target, filter));
}
/**
* Retains multiple elements by removing all others from the instance to be set up.
*
* Treats a {@code null}-argument just like an empty {@link Collection}.
*
* Avoids an unnecessary {@link ClassCastException} or {@link NullPointerException} which might be caused by
* {@link Collection#retainAll(Collection)} when the instance to be set up does not support the requested
* elements.
*
* @throws UnsupportedOperationException if {@link Collection#retainAll(Collection)} is not supported by the
* instance to be set up.
* @see Collection#retainAll(Collection)
* @see Collecting#retainAll(Collection, Collection)
* @see #retainAll(Stream)
* @see #retainAll(Iterable)
* @see #retainAll(Iterator)
* @see #retainAll(Object[])
*/
default S retainAll(final Collection> elements) {
return setup(target -> Collecting.retainAll(target, nullAsEmpty(elements)));
}
/**
* Retains multiple elements by removing all others from the instance to be set up.
*
* Treats a {@code null}-argument just like an empty {@link Stream}.
*
* @throws UnsupportedOperationException if {@link Collection#retainAll(Collection)} is not supported by the
* instance to be set up.
* @see Collecting#retainAll(Collection, Stream)
* @see #retainAll(Collection)
* @see #retainAll(Iterable)
* @see #retainAll(Iterator)
* @see #retainAll(Object[])
*/
default S retainAll(final Stream> elements) {
return setup(target -> Collecting.retainAll(target, nullAsEmpty(elements)));
}
/**
* Retains multiple elements by removing all others from the instance to be set up.
*
* Treats a {@code null}-argument just like an empty {@link Iterable}.
*
* @throws UnsupportedOperationException if {@link Collection#retainAll(Collection)} is not supported by the
* instance to be set up.
* @see Collecting#retainAll(Collection, Iterable)
* @see #retainAll(Collection)
* @see #retainAll(Stream)
* @see #retainAll(Iterator)
* @see #retainAll(Object[])
*/
default S retainAll(final Iterable> elements) {
return setup(target -> Collecting.retainAll(target, nullAsEmpty(elements)));
}
/**
* Retains multiple elements by removing all others from the instance to be set up.
*
* Treats a {@code null}-argument just like an empty {@link Iterator}.
*
* @throws UnsupportedOperationException if {@link Collection#retainAll(Collection)} is not supported by the
* instance to be set up.
* @see Collecting#retainAll(Collection, Iterable)
* @see #retainAll(Collection)
* @see #retainAll(Stream)
* @see #retainAll(Iterable)
* @see #retainAll(Object[])
*/
default S retainAll(final Iterator> elements) {
return setup(target -> Collecting.retainAll(target, nullAsEmpty(elements)));
}
/**
* Retains multiple elements by removing all others from the instance to be set up.
*
* Treats a {@code null}-argument just like an empty {@code array}.
*
* @throws UnsupportedOperationException if {@link Collection#retainAll(Collection)} is not supported by the
* instance to be set up.
* @see Collecting#retainAll(Collection, Object[])
* @see #retainAll(Collection)
* @see #retainAll(Stream)
* @see #retainAll(Iterable)
* @see #retainAll(Iterator)
*/
default S retainAll(final Object[] elements) {
return setup(target -> Collecting.retainAll(target, nullAsEmpty(elements)));
}
/**
* Removes all elements from the instance to be set up.
*
* @throws UnsupportedOperationException if {@link Collection#clear()} is not supported by the instance to be
* set up.
* @see Collection#clear()
* @see Collecting#clear(Collection)
*/
default S clear() {
return setup(Collecting::clear);
}
}
/**
* Builder implementation to build target instances of {@link Collection}.
*
* Use {@link #builder(Supplier)} to get an instance.
*
* @param The element type.
* @param The final type of the target instances, at least {@link Collection}.
*/
public static class Builder>
extends LateBuilder>
implements Setup> {
@SuppressWarnings({"rawtypes", "unchecked"})
private Builder(final Supplier newResult, final Class builderClass) {
super(newResult, builderClass);
}
}
/**
* Charger implementation to charge target instances of {@link Collection}.
*
* Use {@link #charger(Collection)} to get an instance.
*
* @param The element type.
* @param The final type of the target instances, at least {@link Collection}.
*/
@SuppressWarnings("ClassNameSameAsAncestorName")
public static class Charger>
extends de.team33.patterns.building.elara.Charger>
implements Setup> {
@SuppressWarnings({"rawtypes", "unchecked"})
private Charger(final C target, final Class builderClass) {
super(target, builderClass);
}
}
}