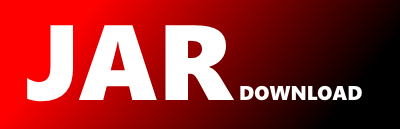
de.terrestris.shoguncore.rest.PluginRestController Maven / Gradle / Ivy
package de.terrestris.shoguncore.rest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
import de.terrestris.shoguncore.dao.PluginDao;
import de.terrestris.shoguncore.model.Plugin;
import de.terrestris.shoguncore.service.PluginService;
/**
* @author Nils Bühner
*/
@RestController
@RequestMapping("/plugins")
public class PluginRestController, S extends PluginService>
extends AbstractRestController {
/**
* Default constructor, which calls the type-constructor
*/
@SuppressWarnings("unchecked")
public PluginRestController() {
this((Class) Plugin.class);
}
/**
* Constructor that sets the concrete entity class for the controller.
* Subclasses MUST call this constructor.
*/
protected PluginRestController(Class entityClass) {
super(entityClass);
}
/**
* @return
*/
@RequestMapping(value = "/{simpleClassName}.js", method = RequestMethod.GET)
public @ResponseBody
ResponseEntity getExternalPluginSource(
@PathVariable String simpleClassName) {
LOG.debug("Requested to return the sourcecode for plugin " + simpleClassName);
ResponseEntity responseEntity = null;
HttpHeaders responseHeaders = new HttpHeaders();
try {
responseHeaders.add("Content-Type", "text/javascript; charset=utf-8");
String classCode = service.getPluginSource(simpleClassName);
responseEntity = new ResponseEntity(
classCode,
responseHeaders,
HttpStatus.OK
);
} catch (Exception e) {
responseHeaders.add("Content-Type", "text/*");
String errMsg = "Error while returning the class code for "
+ "external plugin: " + e.getMessage();
LOG.error(errMsg);
responseEntity = new ResponseEntity(
errMsg,
responseHeaders,
HttpStatus.INTERNAL_SERVER_ERROR
);
}
return responseEntity;
}
/**
* We have to use {@link Qualifier} to define the correct service here.
* Otherwise, spring can not decide which service has to be autowired here
* as there are multiple candidates.
*/
@Override
@Autowired
@Qualifier("pluginService")
public void setService(S service) {
this.service = service;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy