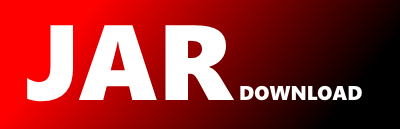
de.tum.in.naturals.set.PowerNatBitSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of naturals-util Show documentation
Show all versions of naturals-util Show documentation
Datastructures and utility classes for non-negative integers
The newest version!
/*
* Copyright (C) 2017 Tobias Meggendorfer
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package de.tum.in.naturals.set;
import it.unimi.dsi.fastutil.Size64;
import it.unimi.dsi.fastutil.ints.IntCollection;
import java.util.AbstractSet;
import java.util.Iterator;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.annotation.Nullable;
class PowerNatBitSet extends AbstractSet implements Size64 {
private final NatBitSet baseSet;
private final int baseSize;
PowerNatBitSet(NatBitSet baseSet) {
assert !baseSet.isEmpty();
this.baseSet = NatBitSets.compact(baseSet, true);
baseSize = this.baseSet.size();
}
@Override
public boolean isEmpty() {
return false;
}
@Override
public boolean contains(@Nullable Object obj) {
return obj instanceof IntCollection && baseSet.containsAll((IntCollection) obj);
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj instanceof PowerNatBitSet) {
PowerNatBitSet other = (PowerNatBitSet) obj;
return baseSet.equals(other.baseSet);
}
Logger.getLogger(PowerNatBitSet.class.getName()).log(Level.WARNING, "Calling equals on PowerNatBitSet");
return super.equals(obj);
}
@Override
public int hashCode() {
Logger.getLogger(PowerNatBitSet.class.getName()).log(Level.WARNING, "Calling hashCode on PowerNatBitSet");
return super.hashCode();
}
/**
* Returns an iterator over the power set.
* Warning: To avoid repeated allocation, the returned set is modified in-place!
*/
@Override
public Iterator iterator() {
return new PowerNatBitSetIterator(baseSet);
}
@SuppressWarnings("deprecation")
@Override
public int size() {
return baseSize >= Integer.SIZE ? Integer.MAX_VALUE : 1 << baseSize;
}
@Override
public long size64() {
return 1L << baseSize;
}
@Override
public String toString() {
return String.format("powerSet(%s)", baseSet);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy