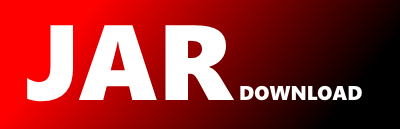
de.undercouch.gradle.tasks.download.DownloadSpec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-download-task Show documentation
Show all versions of gradle-download-task Show documentation
Adds a download task to Gradle that displays progress information
// Copyright 2013 Michel Kraemer
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package de.undercouch.gradle.tasks.download;
import java.io.File;
import java.net.MalformedURLException;
import java.util.Map;
import org.apache.http.HttpRequestInterceptor;
import org.apache.http.HttpResponseInterceptor;
import org.apache.http.auth.AuthScheme;
import org.apache.http.auth.Credentials;
/**
* An interface for classes that perform file downloads
* @author Michel Kraemer
*/
public interface DownloadSpec {
/**
* Sets the download source URL
* @param src the URL
* @throws MalformedURLException if the download source is not a URL
*/
void src(Object src) throws MalformedURLException;
/**
* Sets the download destination
* @param dest a file or directory where to store the retrieved file
*/
void dest(Object dest);
/**
* Sets the quiet flag
* @param quiet true if download progress should not be logged
*/
void quiet(boolean quiet);
/**
* Sets the overwrite flag
* @param overwrite true if existing files should be overwritten, false otherwise
*/
void overwrite(boolean overwrite);
/**
* Sets the onlyIfModified flag
* @param onlyIfModified true if the file should only be downloaded if it
* has been modified on the server since the last download
*/
void onlyIfModified(boolean onlyIfModified);
/**
* Sets the onlyIfNewer flag. This method is an alias for
* {@link #onlyIfModified(boolean)}.
* @param onlyIfNewer true if the file should only be downloaded if it
* has been modified on the server since the last download
*/
void onlyIfNewer(boolean onlyIfNewer);
/**
* Specifies if compression should be used during download
* @param compress true if compression should be enabled
*/
void compress(boolean compress);
/**
* Sets the username for Basic
or Digest
* authentication
* @param username the username
*/
void username(String username);
/**
* Sets the password for Basic
or Digest
* authentication
* @param password the password
*/
void password(String password);
/**
* Sets the authentication scheme to use. This method accepts
* either a String
(valid values are "Basic"
* and "Digest"
) or an instance of {@link AuthScheme}.
* If username
and password
are set this
* method will only accept "Basic"
or "Digest"
* as valid values. The default value will be "Basic"
in
* this case.
* @param authScheme the authentication scheme
*/
void authScheme(Object authScheme);
/**
* Sets the credentials used for authentication. Can be called as an
* alternative to {@link #username(String)} and {@link #password(String)}.
* Allows for setting credentials for authentication schemes other than
* Basic
or Digest
.
* @param credentials the credentials
*/
void credentials(Credentials credentials);
/**
* Sets the HTTP request headers to use when downloading
* @param headers a Map of header names to values
*/
void headers(Map headers);
/**
* Sets an HTTP request header to use when downloading
* @param name name of the HTTP header
* @param value value of the HTTP header
*/
void header(String name, String value);
/**
* Specifies if HTTPS certificate verification errors should be ignored
* and any certificate (even an invalid one) should be accepted. By default
* certificates are validated and errors are not being ignored.
* @param accept true if certificate errors should be ignored (default: false)
*/
void acceptAnyCertificate(boolean accept);
/**
* Specifies a timeout in milliseconds which is the maximum time to wait
* until a connection is established or until the server returns data. A
* value of zero means infinite timeout. A negative value is interpreted
* as undefined.
* @param milliseconds the timeout in milliseconds (default: -1)
*/
void timeout(int milliseconds);
/**
* Specifies the directory where gradle-download-task stores information
* that should persist between builds
* @param dir the directory (default: ${buildDir}/gradle-download-task)
*/
void downloadTaskDir(Object dir);
/**
* Specifies whether the file should be downloaded to a temporary location
* and, upon successful execution, moved to the final location. If the
* overwrite flag is set to false, this flag is useful to avoid partially
* downloaded files if Gradle is forcefully closed or the system crashes.
* Note that the plugin always deletes partial downloads on connection
* errors, regardless of the value of this flag. The default temporary
* location can be configured with the {@link #downloadTaskDir(Object)};
* @param tempAndMove true if the file should be downloaded to a temporary
* location and, upon successful execution, moved to the final location
* (default: false)
*/
void tempAndMove(boolean tempAndMove);
/**
* Sets the useETag
flag. Possible values are:
*
* true
: check if the entity tag (ETag) of a downloaded
* file has changed and issue a warning if a weak ETag was encountered
* false
: Do not use entity tags (ETags) at all
* "all"
: Use all ETags but do not issue a warning for weak ones
* "strongOnly"
: Use only strong ETags
*
* Note that this flag is only effective if onlyIfModified
is
* true
.
* @param useETag the flag's new value
*/
void useETag(Object useETag);
/**
* Sets the location of the file that keeps entity tags (ETags) received
* from the server
* @param location the location (default: ${downloadTaskDir}/etags.json)
*/
void cachedETagsFile(Object location);
/**
* Specifies an interceptor that will be called when a request is about
* to be sent to the server. This is useful if you want to manipulate
* a request beyond the capabilities of the download task.
* @param interceptor the interceptor to set
*/
void requestInterceptor(HttpRequestInterceptor interceptor);
/**
* Specifies an interceptor that will be called when a response has been
* received from the server. This is useful if you want to manipulate
* incoming data before it is handled by the download task.
* @param interceptor the interceptor to set
*/
void responseInterceptor(HttpResponseInterceptor interceptor);
/**
* @return the download source(s), either a URL or a list of URLs
*/
Object getSrc();
/**
* @return the download destination
*/
File getDest();
/**
* @return the quiet flag
*/
boolean isQuiet();
/**
* @return the overwrite flag
*/
boolean isOverwrite();
/**
* @return the onlyIfModified flag
*/
boolean isOnlyIfModified();
/**
* Get the onlyIfNewer
flag. This method is an alias for
* {@link #isOnlyIfModified()}.
* @return the onlyIfNewer flag
*/
boolean isOnlyIfNewer();
/**
* @return true if compression is enabled
*/
boolean isCompress();
/**
* @return the username for Basic
or Digest
* authentication
*/
String getUsername();
/**
* @return the password for Basic
or Digest
* authentication
*/
String getPassword();
/**
* @return the authentication scheme used (or null
if
* no authentication is performed)
*/
AuthScheme getAuthScheme();
/**
* @return the credentials used for authentication (or null
if
* no authentication is performed)
*/
Credentials getCredentials();
/**
* @return the HTTP request headers to use when downloading
*/
Map getHeaders();
/**
* @param name name of the HTTP header
* @return the value of the HTTP header
*/
String getHeader(String name);
/**
* @return true if HTTPS certificate verification errors should be ignored,
* default value is false
*/
boolean isAcceptAnyCertificate();
/**
* @return the timeout in milliseconds which is the maximum time to wait
* until a connection is established or until the server returns data.
*/
int getTimeout();
/**
* @return the directory where gradle-download-task stores information
* that should persist between builds
*/
File getDownloadTaskDir();
/**
* @return true of if the file should be downloaded to a temporary
* location and, upon successful execution, moved to the final
* location.
*/
boolean isTempAndMove();
/**
* @return the value of the useETag
flag
* @see #useETag(Object)
*/
Object getUseETag();
/**
* @return the location of the file that keeps entity tags (ETags) received
* from the server
*/
File getCachedETagsFile();
/**
* @return an interceptor that will be called when a request is about to
* be sent to the server (or null
if no interceptor is specified)
*/
HttpRequestInterceptor getRequestInterceptor();
/**
* @return an interceptor that will be called when a response has been
* received from the server (or null
if no interceptor is specified)
*/
HttpResponseInterceptor getResponseInterceptor();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy