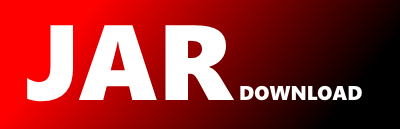
de.uni_hildesheim.sse.util.ArrayIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of locutor Show documentation
Show all versions of locutor Show documentation
The SSE Java native library for accessing operating system level information,
runtime-reinstrumentation etc. Used as part of SPASS-meter.
The newest version!
package de.uni_hildesheim.sse.util;
import java.util.Iterator;
import java.util.NoSuchElementException;
/**
* Defines an array iterator.
*
* @param the type of the elements in the array (iterator)
* @author Holger Eichelberger
* @since 1.00
* @version 1.00
*/
public class ArrayIterator implements Iterator, Iterable {
/**
* The data array to be iterated.
*/
private T[] data;
/**
* The position of the array in the iterator.
*/
private int position;
/**
* Constructs a new iterator.
*
* @param data the data array
*
* @since 1.00
*/
public ArrayIterator(T[] data) {
assert null != data;
this.data = data;
position = 0;
}
/**
* Returns true if the iteration has more elements. (In other
* words, returns true if next would return an element
* rather than throwing an exception.)
*
* @return true if the iterator has more elements.
*/
public boolean hasNext() {
return position < data.length;
}
/**
* Returns the next element in the iteration.
*
* @return the next element in the iteration.
* @exception NoSuchElementException iteration has no more elements.
*/
public T next() {
if (position < 0 || position >= data.length) {
throw new NoSuchElementException();
}
return data[position++];
}
/**
* Removes from the underlying collection the last element returned by the
* iterator (optional operation).
*
* @exception UnsupportedOperationException if the remove
* operation is not supported by this Iterator (always).
*/
public void remove() {
throw new UnsupportedOperationException();
}
/**
* Returns an iterable, i.e. itself.
*
* @return itself
*/
public Iterator iterator() {
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy