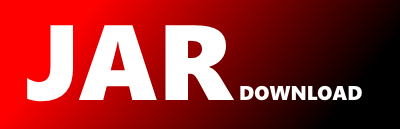
de.uni.freiburg.iig.telematik.jagal.graph.GraphUtils Maven / Gradle / Ivy
package de.uni.freiburg.iig.telematik.jagal.graph;
import java.util.HashSet;
import java.util.Set;
import java.util.concurrent.ArrayBlockingQueue;
import de.uni.freiburg.iig.telematik.jagal.graph.abstr.AbstractGraph;
import de.uni.freiburg.iig.telematik.jagal.graph.exception.EdgeNotFoundException;
import de.uni.freiburg.iig.telematik.jagal.graph.exception.GraphException;
public class GraphUtils {
private final static ArrayBlockingQueue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy