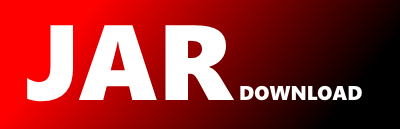
de.uni.freiburg.iig.telematik.sepia.graphic.netgraphics.NodeGraphics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of SEPIA Show documentation
Show all versions of SEPIA Show documentation
SEPIA provides implementations for various types of Petri nets. Along Place/Transition-nets, it supports Petri nets with distinguishable token colors and defines coloured workflow nets, where coloured tokens are interpreted as data elements used during process execution. To support information flow analysis of processes, SEPIA defines so-called IF-Nets, tailored for security-oriented workflow modeling which enable users to assign security-levels (HIGH, LOW) to transitions, data elements and persons/agents participating in the process execution.
The newest version!
package de.uni.freiburg.iig.telematik.sepia.graphic.netgraphics;
import de.invation.code.toval.validate.Validate;
import de.uni.freiburg.iig.telematik.sepia.graphic.netgraphics.attributes.Dimension;
import de.uni.freiburg.iig.telematik.sepia.graphic.netgraphics.attributes.Fill;
import de.uni.freiburg.iig.telematik.sepia.graphic.netgraphics.attributes.Line;
import de.uni.freiburg.iig.telematik.sepia.graphic.netgraphics.attributes.Position;
/**
*
* Node graphics attribute class containing the attributes position, dimension, fill and line for places and transitions.
*
*
* @author Adrian Lange
*/
public class NodeGraphics extends AbstractObjectGraphics {
/** Default position */
public static final Position DEFAULT_POSITION = new Position();
/** Default dimension */
public static final Dimension DEFAULT_DIMENSION = new Dimension();
/** Default fill */
public static final Fill DEFAULT_FILL = new Fill();
/** Default line */
public static final Line DEFAULT_LINE = new Line();
private Position position = null;
private Dimension dimension = null;
private Fill fill = null;
private Line line = null;
/**
* Create new node graphic object with default values.
*/
public NodeGraphics() {
setPosition(DEFAULT_POSITION);
setDimension(DEFAULT_DIMENSION);
setFill(DEFAULT_FILL);
setLine(DEFAULT_LINE);
}
/**
* Create new node graphic object with the specified values.
*/
public NodeGraphics(Position position, Dimension dimension, Fill fill, Line line) {
setPosition(position);
setDimension(dimension);
setFill(fill);
setLine(line);
}
/**
* @return the position
*/
public Position getPosition() {
return position;
}
/**
* @return the dimension
*/
public Dimension getDimension() {
return dimension;
}
/**
* @return the fill
*/
public Fill getFill() {
return fill;
}
/**
* @return the line
*/
public Line getLine() {
return line;
}
/**
* @param position
* the position to set
*/
public void setPosition(Position position) {
Validate.notNull(position);
this.position = position;
}
/**
* @param dimension
* the dimension to set
*/
public void setDimension(Dimension dimension) {
Validate.notNull(dimension);
this.dimension = dimension;
}
/**
* @param fill
* the fill to set
*/
public void setFill(Fill fill) {
Validate.notNull(fill);
this.fill = fill;
}
/**
* @param line
* the line to set
*/
public void setLine(Line line) {
Validate.notNull(line);
this.line = line;
}
@Override
public boolean hasContent() {
return position.hasContent() || dimension.hasContent() || line.hasContent() || fill.hasContent();
}
@Override
public String toString() {
StringBuilder str = new StringBuilder();
boolean empty = true;
str.append("[");
if (position != DEFAULT_POSITION) {
str.append(position);
empty = false;
}
if (dimension != DEFAULT_DIMENSION) {
if (!empty)
str.append(",");
str.append(dimension);
empty = false;
}
if (fill != DEFAULT_FILL) {
if (!empty)
str.append(",");
str.append(fill);
empty = false;
}
if (line != DEFAULT_LINE) {
if (!empty)
str.append(",");
str.append(line);
}
str.append("]");
return str.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy