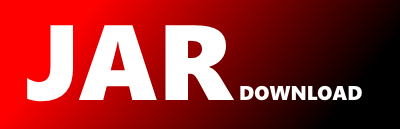
de.uni.freiburg.iig.telematik.sepia.overlap.OverlapCallable Maven / Gradle / Ivy
Show all versions of SEPIA Show documentation
package de.uni.freiburg.iig.telematik.sepia.overlap;
import de.uni.freiburg.iig.telematik.sepia.petrinet.abstr.AbstractFlowRelation;
import de.uni.freiburg.iig.telematik.sepia.petrinet.abstr.AbstractMarking;
import de.uni.freiburg.iig.telematik.sepia.petrinet.abstr.AbstractPlace;
import de.uni.freiburg.iig.telematik.sepia.petrinet.abstr.AbstractTransition;
import de.uni.freiburg.iig.telematik.sepia.petrinet.properties.mg.StateSpaceException;
import de.uni.freiburg.iig.telematik.sepia.petrinet.properties.sequences.MGTraversalResult;
import de.uni.freiburg.iig.telematik.sepia.petrinet.properties.sequences.SequenceGenerationCallable;
import de.uni.freiburg.iig.telematik.sepia.petrinet.properties.sequences.SequenceGenerationCallableGenerator;
import de.uni.freiburg.iig.telematik.sepia.petrinet.properties.threaded.AbstractPNPropertyCheckerCallable;
import de.uni.freiburg.iig.telematik.sepia.replay.ReplayCallable;
import de.uni.freiburg.iig.telematik.sepia.replay.ReplayCallableGenerator;
import de.uni.freiburg.iig.telematik.sepia.replay.ReplayResult;
import de.uni.freiburg.iig.telematik.sewol.log.LogEntry;
public class OverlapCallable< P extends AbstractPlace,
T extends AbstractTransition,
F extends AbstractFlowRelation,
M extends AbstractMarking,
S extends Object,
E extends LogEntry> extends AbstractPNPropertyCheckerCallable
> {
protected OverlapCallable(OverlapCallableGenerator
generator) {
super(generator);
}
@SuppressWarnings("unchecked")
@Override
protected OverlapCallableGenerator
getGenerator() {
return (OverlapCallableGenerator
) super.getGenerator();
}
@Override
protected OverlapResult callRoutine() throws OverlapException, InterruptedException {
// Generate Sequences
MGTraversalResult traversalResult = null;
try {
SequenceGenerationCallable sequenceGeneratorCallable = new SequenceGenerationCallable
(new SequenceGenerationCallableGenerator
(getGenerator()));
traversalResult = sequenceGeneratorCallable.callRoutine();
getGenerator().setMarkingGraph(sequenceGeneratorCallable.getMarkingGraph());
} catch(Exception e){
// Abort when Petri net is unbounded
if(e.getCause() != null && e.getCause() instanceof StateSpaceException){
throw new OverlapException("Cannot generate sequences of unbounded net", e);
}
throw new OverlapException("Exception during marking graph construction", e);
}
// Perform replay
ReplayResult replayResult = null;
try {
ReplayCallable replayingCallable = new ReplayCallable
(new ReplayCallableGenerator
(getGenerator()));
replayResult = replayingCallable.callRoutine();
} catch(Exception e){
throw new OverlapException("Exception during replay", e);
}
return new OverlapResult(traversalResult, replayResult);
}
}