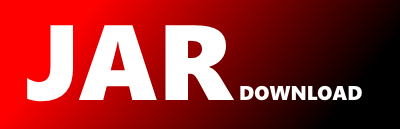
de.uni.freiburg.iig.telematik.sepia.parser.pnml.PNMLParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of SEPIA Show documentation
Show all versions of SEPIA Show documentation
SEPIA provides implementations for various types of Petri nets. Along Place/Transition-nets, it supports Petri nets with distinguishable token colors and defines coloured workflow nets, where coloured tokens are interpreted as data elements used during process execution. To support information flow analysis of processes, SEPIA defines so-called IF-Nets, tailored for security-oriented workflow modeling which enable users to assign security-levels (HIGH, LOW) to transitions, data elements and persons/agents participating in the process execution.
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy