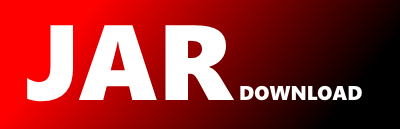
de.uni.freiburg.iig.telematik.sepia.traversal.PNTraversalUtils Maven / Gradle / Ivy
Show all versions of SEPIA Show documentation
package de.uni.freiburg.iig.telematik.sepia.traversal;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.List;
import de.invation.code.toval.validate.ParameterException;
import de.invation.code.toval.validate.Validate;
import de.uni.freiburg.iig.telematik.sepia.exception.PNException;
import de.uni.freiburg.iig.telematik.sepia.petrinet.abstr.AbstractFlowRelation;
import de.uni.freiburg.iig.telematik.sepia.petrinet.abstr.AbstractMarking;
import de.uni.freiburg.iig.telematik.sepia.petrinet.abstr.AbstractPetriNet;
import de.uni.freiburg.iig.telematik.sepia.petrinet.abstr.AbstractPlace;
import de.uni.freiburg.iig.telematik.sepia.petrinet.abstr.AbstractTransition;
public class PNTraversalUtils {
/**
* Simulates the given Petri net the given number of times and returns all distinct observed traces.
* This method uses a random Petri net traverser.
* @param net The Petri net to simulate.
* @param runs The nubmer of times to simulate the net.
* @return A set of distinct observed traces.
* @throws ParameterException If the given net is null
*/
public static ,
T extends AbstractTransition,
F extends AbstractFlowRelation,
M extends AbstractMarking,
S extends Object>
Collection> testTraces(AbstractPetriNet net, int runs, int maxEventsPerTrace){
return testTraces(net, runs, maxEventsPerTrace, false, false, false);
}
/**
* Simulates the given Petri net the given number of times and returns all distinct observed traces.
* This method uses a random Petri net traverser.
* @param net The Petri net to simulate.
* @param runs The number of times to simulate the net.
* @param printOut Indicates, if new distinct traces are printed out.
* @return A set of distinct observed traces.
* @throws ParameterException If the given net is null
*/
public static
,
T extends AbstractTransition,
F extends AbstractFlowRelation,
M extends AbstractMarking,
S extends Object>
Collection> testTraces(AbstractPetriNet net, int runs, int maxEventsPerTrace, boolean printOut, boolean onlyDistinctTraces, boolean useLabelNames){
Validate.notNull(net);
Collection> traces = null;
if(onlyDistinctTraces){
traces = new HashSet>();
} else {
traces = new ArrayList>();
}
List newTrace = null;
PNTraverser traverser = new RandomPNTraverser(net);
for(int i = 0; i();
net.reset();
int c = 0;
while(net.hasEnabledTransitions() && c++ < maxEventsPerTrace){
T nextTransition = traverser.chooseNextTransition(net.getEnabledTransitions());
String descriptor = useLabelNames ? nextTransition.getLabel() : nextTransition.getName();
if(!nextTransition.isSilent()){
newTrace.add(descriptor);
}
try {
net.fire(nextTransition.getName());
} catch (PNException e) {
e.printStackTrace();
}
}
if(traces.add(newTrace) && printOut)
System.out.println(newTrace);
}
return traces;
}
}