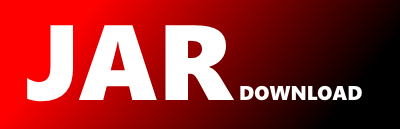
de.uni.freiburg.iig.telematik.sewol.log.LogEntryUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of SEWOL Show documentation
Show all versions of SEWOL Show documentation
SEWOL provides support for the handling of workflow traces. Specifically it allows to specify the shape and content of process traces in terms of entries representing the execution of a specific workflow activity. SEWOL also allows to write these traces on disk as a log file with the help of a special file writer for process logs. Currently it supports plain text, Petrify, MXML and XES log file types. In order to specify security-related context information, SEWOL provides access control models such as access control lists (ACL) and role-based access control models (RBAC). All types of models can be conveniently edited with the help of appropriate dialogs.
package de.uni.freiburg.iig.telematik.sewol.log;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import de.invation.code.toval.validate.ParameterException;
import de.invation.code.toval.validate.ParameterException.ErrorCode;
import de.invation.code.toval.validate.Validate;
public class LogEntryUtils {
public static void lockFieldForEntries(EntryField field, String reason, Collection entries) throws ParameterException{
Validate.notNull(field);
Validate.notNull(entries);
for(E entry: entries){
if(entry != null){
entry.lockField(field, reason);
} else {
throw new ParameterException(ErrorCode.NULLPOINTER);
}
}
}
public static List getEntriesWithLockedField(List entries, EntryField field) throws ParameterException{
validateEntries(entries);
Validate.notNull(field);
List entriesWithLockedField = new ArrayList();
for(E entry: entries){
if(entry.isFieldLocked(field)){
entriesWithLockedField.add(entry);
}
}
return entriesWithLockedField;
}
public static List getEntriesWithoutLockedField(List entries, EntryField field) throws ParameterException{
validateEntries(entries);
Validate.notNull(field);
List entriesWithoutLockedField = new ArrayList();
for(E entry: entries){
if(!entry.isFieldLocked(field)){
entriesWithoutLockedField.add(entry);
}
}
return entriesWithoutLockedField;
}
public static List getFieldValues(List entries, EntryField field) throws ParameterException{
validateEntries(entries);
Validate.notNull(field);
List values = new ArrayList();
for(E entry: entries){
values.add(entry.getFieldValue(field).toString());
}
return values;
}
public static List getEntriesWithActivity(List entries, String activity) throws ParameterException{
validateEntries(entries);
Validate.notNull(activity);
List result = new ArrayList();
for(E entry: entries){
if(entry.getActivity().equals(activity)){
result.add(entry);
}
}
return result;
}
public static Map> clusterEntriesAccordingToActivities(List entries) throws ParameterException{
validateEntries(entries);
Map> result = new HashMap>();
for(E entry: entries){
if(!result.keySet().contains(entry.getActivity())){
result.put(entry.getActivity(), new ArrayList());
}
result.get(entry.getActivity()).add(entry);
}
return result;
}
public static Map> clusterOriginatorsAccordingToActivity(List entries) throws ParameterException{
validateEntries(entries);
Map> originatorSets = new HashMap>();
for(E entry: entries){
if(!originatorSets.containsKey(entry.getActivity())){
originatorSets.put(entry.getActivity(), new HashSet());
}
originatorSets.get(entry.getActivity()).add(entry.getOriginator());
}
return originatorSets;
}
public static Set getDistinctValuesForField(List entries, EntryField field) throws ParameterException{
validateEntries(entries);
Validate.notNull(field);
Set result = new HashSet();
for(E entry: entries){
result.add(entry.getFieldValue(field).toString());
}
return result;
}
public static void setOriginatorForEntries(String originator, List entries) throws ParameterException{
validateEntries(entries);
try {
for(E entry: entries){
entry.setOriginator(originator);
}
} catch (ParameterException e) {
e.printStackTrace();
} catch (LockingException e) {
e.printStackTrace();
}
}
public static void setRoleForEntries(String role, List entries) throws ParameterException{
validateEntries(entries);
try {
for(E entry: entries) {
entry.setRole(role);
}
} catch (ParameterException e) {
e.printStackTrace();
} catch (LockingException e) {
e.printStackTrace();
}
}
protected static void validateEntries(Collection entries) throws ParameterException{
Validate.notNull(entries);
Validate.notEmpty(entries);
Validate.noNullElements(entries);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy