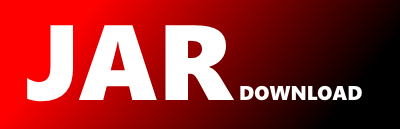
de.uniks.networkparser.graph.Clazz Maven / Gradle / Ivy
package de.uniks.networkparser.graph;
import de.uniks.networkparser.buffer.CharacterBuffer;
import de.uniks.networkparser.graph.util.AssociationSet;
import de.uniks.networkparser.graph.util.AttributeSet;
import de.uniks.networkparser.graph.util.ClazzSet;
import de.uniks.networkparser.graph.util.MethodSet;
import de.uniks.networkparser.interfaces.Condition;
import de.uniks.networkparser.list.SimpleSet;
public class Clazz extends GraphEntity {
public static final StringFilter NAME = new StringFilter(GraphMember.PROPERTY_NAME);
public static final String PROPERTY_PACKAGENAME = "packageName";
public static final String PROPERTY_FULLNAME = "fullName";
public static final String PROPERTY_VISIBILITY = "visibility";
public static final String PROPERTY_MODIFIERS = "modifiers";
public static final String PROPERTY_TYPE = "type";
public static final String PROPERTY_SUPERCLAZZ = "superclazz";
public static final String PROPERTY_IMPLEMENTS = "implements";
private ClazzType type = ClazzType.CLAZZ;
Clazz() {
}
/**
* Constructor with Name of Clazz
* @param name Name of Clazz
*/
public Clazz(String name) {
this.with(name);
}
public Clazz(Class> name) {
if(name != null) {
with(name.getName().replace("$", "."));
}
}
@Override
public Clazz with(String name) {
super.with(name);
return this;
}
@Override
public Clazz withId(String id) {
super.withId(id);
return this;
}
public Clazz with(ClazzType clazzType) {
this.type = clazzType;
return this;
}
public Clazz enableInterface() {
this.with(ClazzType.INTERFACE);
return this;
}
public Clazz enableEnumeration() {
this.with(ClazzType.ENUMERATION);
return this;
}
public Clazz enableEnumeration(String... literals) {
this.with(ClazzType.ENUMERATION);
if(literals == null) {
return this;
}
for(String item : literals) {
this.with(new Literal(item));
}
return this;
}
public Clazz enableEnumeration(Literal... literals) {
this.with(ClazzType.ENUMERATION);
if(literals == null) {
return this;
}
for(Literal item : literals) {
this.with(item);
}
return this;
}
public ClazzType getType() {
return type;
}
@Override
protected String getFullId() {
if(this.getId() != null) {
return this.getId();
}
return super.getFullId();
}
@Override
public Clazz withExternal(boolean value) {
super.withExternal(value);
return this;
}
@Override
public Modifier getModifier() {
Modifier modifier = super.getModifier();
if(modifier == null) {
modifier = new Modifier(Modifier.PUBLIC);
super.withChildren(modifier);
}
return modifier;
}
public Clazz with(Association... values) {
super.with(values);
return this;
}
public Clazz with(Modifier... values) {
super.withModifier(values);
return this;
}
public Clazz with(Attribute... values) {
super.withChildren(values);
return this;
}
public Clazz with(Method... values) {
super.withChildren(values);
return this;
}
public Clazz with(Annotation value) {
super.with(value);
return this;
}
public Clazz with(GraphImage... values) {
super.withChildren(values);
return this;
}
public Clazz with(Literal... values) {
super.withChildren(values);
return this;
}
public SimpleSet getValues() {
SimpleSet collection = new SimpleSet();
if(this.children == null) {
return collection;
}
if(this.children instanceof Literal) {
collection.add((Literal)this.children);
return collection;
}
if(this.children instanceof GraphSimpleSet) {
GraphSimpleSet list = (GraphSimpleSet) this.children;
for(GraphMember item : list) {
if(item instanceof Literal) {
collection.add((Literal)item);
}
}
}
return collection;
}
public Clazz without(Association... values) {
super.without(values);
return this;
}
public Clazz without(Modifier... values) {
super.without(values);
return this;
}
public Clazz without(Attribute... values) {
super.without(values);
return this;
}
public Clazz without(Method... values) {
super.without(values);
return this;
}
public Clazz without(Annotation value) {
super.without(value);
return this;
}
public Clazz without(GraphImage... values) {
super.without(values);
return this;
}
public Clazz without(Literal... values) {
super.without(values);
return this;
}
/**
* ********************************************************************
*
* %srcCardinality% %tgtCardinality%
* Clazz -------------------------------------- %tgtClass%
* %srcRoleName% %tgtRoleName%
*
*
* create a Bidirectional Association
*
* @param tgtClass The target Clazz
* @param tgtRoleName The Targetrolename
* @param tgtCardinality The Targetcardinality
* @param srcRoleName The sourcerolename
* @param srcCardinality The sourcecardinality
* @return The Clazz Instance
*/
public Clazz withBidirectional(Clazz tgtClass, String tgtRoleName, Cardinality tgtCardinality, String srcRoleName, Cardinality srcCardinality) {
// Target
Association assocTarget = new Association(tgtClass).with(tgtCardinality).with(tgtRoleName);
// Source
Association assocSource = new Association(this).with(srcCardinality).with(srcRoleName);
assocSource.with(assocTarget);
tgtClass.with(assocTarget);
this.with(assocSource);
return this;
}
/**
* ********************************************************************
*
* %tgtCardinality%
* Clazz ----------------------------------- %tgtClass%
* %tgtRoleName%
*
*
* create a Undirectional Association
*
* @param tgtClass The target Clazz
* @param tgtRoleName The Targetrolename
* @param tgtCardinality The Targetcardinality
* @return The Clazz Instance
*/
public Clazz withUniDirectional(Clazz tgtClass, String tgtRoleName, Cardinality tgtCardinality) {
// Target
Association assocTarget = new Association(tgtClass).with(tgtCardinality).with(AssociationTypes.UNDIRECTIONAL).with(tgtRoleName);
// Source
Association assocSource = new Association(this).with(AssociationTypes.EDGE).with(assocTarget);
tgtClass.with(assocTarget);
this.with(assocSource);
return this;
}
/**
* Get All Interfaces
* @param transitive Get all Interfaces or direct Interfaces
* @return all Interfaces of a Clazz
*
* one many
* Clazz ----------------------------------- Clazz
* clazz Interfaces
*
*/
public ClazzSet getInterfaces(boolean transitive) {
repairAssociations();
AssociationTypes type = AssociationTypes.IMPLEMENTS;
if(this.getType()==ClazzType.INTERFACE) {
type = AssociationTypes.GENERALISATION;
}
ClazzSet collection = getEdgeClazzes(type, null);
if(!transitive) {
return collection;
}
int size = collection.size();
for(int i=0;i
* one many
* Clazz ----------------------------------- Clazz
* clazz superClazzes
*
*/
public ClazzSet getSuperClazzes(boolean transitive) {
repairAssociations();
ClazzSet collection = getEdgeClazzes(AssociationTypes.GENERALISATION, null);
if(!transitive) {
return collection;
}
int size = collection.size();
for(int i=0;i* Clazz --------------------- Attributes * one many **/ public AttributeSet getAttributes(Condition>... filters) { AttributeSet collection = new AttributeSet(); if(this.children == null) { return collection; } ClazzSet superClasses= new ClazzSet(); if(this.children instanceof Attribute) { if(check((Attribute)this.children, filters)) { collection.add((Attribute)this.children); } return collection; } else if(this.children instanceof Association) { Association assoc = (Association) this.children; if(assoc.getType()==AssociationTypes.GENERALISATION || assoc.getType() == AssociationTypes.IMPLEMENTS) { superClasses.add(assoc.getOtherClazz()); } } if(this.children instanceof GraphSimpleSet) { GraphSimpleSet list = (GraphSimpleSet) this.children; for(GraphMember item : list) { if(item instanceof Attribute) { if(check(item, filters)) { collection.add((Attribute)item); } } else if(item instanceof Association) { Association assoc = (Association) item; if(assoc.getType()==AssociationTypes.GENERALISATION || assoc.getType() == AssociationTypes.IMPLEMENTS) { superClasses.add(assoc.getOtherClazz()); } } } } boolean isInterface = getType() == ClazzType.INTERFACE; boolean isAbstract = getModifier().has(Modifier.ABSTRACT); if(isInterface || isAbstract) { return collection; } // ALL SUPERMETHODS AttributeSet newAttribute = new AttributeSet(); AttributeSet foundAttribute = new AttributeSet(); for(int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy