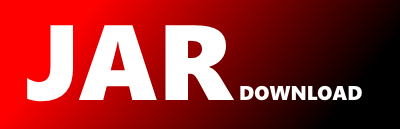
de.uniks.networkparser.parser.excel.ExcelCell Maven / Gradle / Ivy
package de.uniks.networkparser.parser.excel;
/*
NetworkParser
Copyright (c) 2011 - 2016, Stefan Lindel
All rights reserved.
Licensed under the EUPL, Version 1.1 or (as soon they
will be approved by the European Commission) subsequent
versions of the EUPL (the "Licence");
You may not use this work except in compliance with the Licence.
You may obtain a copy of the Licence at:
http://ec.europa.eu/idabc/eupl5
Unless required by applicable law or agreed to in writing, software distributed under the Licence is
distributed on an "AS IS" basis, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the Licence for the specific language governing permissions and limitations under the Licence.
*/
import java.nio.charset.Charset;
import java.util.Comparator;
import de.uniks.networkparser.Pos;
import de.uniks.networkparser.buffer.Buffer;
import de.uniks.networkparser.buffer.CharacterBuffer;
import de.uniks.networkparser.interfaces.BaseItem;
import de.uniks.networkparser.interfaces.Converter;
import de.uniks.networkparser.interfaces.EntityList;
import de.uniks.networkparser.interfaces.SendableEntityCreatorTag;
import de.uniks.networkparser.list.SimpleList;
import de.uniks.networkparser.xml.XMLTokener;
public class ExcelCell implements SendableEntityCreatorTag, EntityList{
public static final String TAG="c";
public static final String TAG_VALUE="v";
public static final String PROPERTY_STYLE="s";
public static final String PROPERTY_TYPE="t";
public static final String PROPERTY_REFERENZ="r";
public static final String[] PROPERTIES={PROPERTY_STYLE, PROPERTY_TYPE, PROPERTY_REFERENZ};
private Pos pos;
private Object content;
private ExcelCell referenceCell;
private String style;
private String type;
private SimpleList children;
@Override
public String[] getProperties() {
return PROPERTIES;
}
@Override
public Object getValue(Object entity, String attribute) {
if(entity instanceof ExcelCell == false) {
return null;
}
if(PROPERTY_STYLE.equals(attribute)) {
return ((ExcelCell)entity).getStyle();
}
if(PROPERTY_TYPE.equals(attribute)) {
return ((ExcelCell)entity).getType();
}
if(PROPERTY_REFERENZ.equals(attribute)) {
return ((ExcelCell)entity).getReferenz();
}
return null;
}
@Override
public boolean setValue(Object entity, String attribute, Object value, String type) {
if(entity instanceof ExcelCell == false) {
return false;
}
ExcelCell item = (ExcelCell) entity;
if(PROPERTY_STYLE.equals(attribute)) {
item.setStyle(""+value);
return true;
}
if(PROPERTY_TYPE.equals(attribute)) {
item.setType(""+value);
return true;
}
if(PROPERTY_REFERENZ.equals(attribute)) {
item.withReferenz(Pos.valueOf(""+value));
return true;
}
if(XMLTokener.CHILDREN.equals(type)) {
item.add(value);
return true;
}
return false;
}
@Override
public Object getSendableInstance(boolean prototyp) {
return new ExcelCell();
}
@Override
public String getTag() {
return TAG;
}
public Pos getReferenz() {
return pos;
}
public ExcelCell withReferenz(Pos referenz) {
this.pos = referenz;
return this;
}
public String getType() {
return type;
}
public String getStyle() {
return style;
}
public Object getContent() {
if(referenceCell != null) {
return referenceCell.getContent();
}
return content;
}
public String getContentAsString() {
if(referenceCell != null) {
return referenceCell.getContentAsString();
}
if(content == null) {
return "";
}
return content.toString();
}
public boolean setContent(Object value) {
if(referenceCell != null) {
return referenceCell.setContent(value);
}
if((this.content == null && value != null) ||
(this.content != null && this.content.equals(value) == false)) {
this.content = value;
return true;
}
return false;
}
public ExcelCell withContent(Object value) {
setContent(value);
return this;
}
public ExcelCell getReferenceCell() {
return referenceCell;
}
public boolean setStyle(String value) {
if((this.style == null && value != null) ||
(this.style != null && this.style.equals(value) == false)) {
this.style = value;
return true;
}
return false;
}
public boolean setType(String value) {
if((this.type == null && value != null) ||
(this.type != null && this.type.equals(value) == false)) {
this.type = value;
return true;
}
return false;
}
@Override
public String toString() {
String ref = "";
if(this.pos != null) {
ref = this.pos.toString();
}
Object context = getContent();
if(context == null){
if(this.children != null) {
CharacterBuffer buffer = new CharacterBuffer();
for(EntityList item : this.children) {
buffer.with(item.toString());
}
return buffer.toString();
}
return "";
}
if(context instanceof Number) {
return ""+context+" ";
}
if(context instanceof Boolean) {
if((Boolean)context) {
return "1 ";
}
return "0 ";
}
return ""+new String(context.toString().getBytes(Charset.forName("UTF-8")), Charset.forName("UTF-8"))+" ";
}
@Override
public String toString(int indentFactor) {
return toString();
}
protected String toString(int indentFactor, int indent) {
return toString();
}
@Override
public String toString(Converter converter) {
return toString();
}
@Override
public boolean add(Object... values) {
if(values == null) {
return false;
}
if(children ==null) {
this.children = new SimpleList();
}
for(Object item : values) {
if(item instanceof EntityList) {
this.children.add((EntityList) item);
}
}
return true;
}
@Override
public BaseItem getNewList(boolean keyValue) {
return new ExcelCell();
}
/**
* Gets the children.
* @param index the Index of Child
* @return the children
*/
public EntityList getChild(int index) {
if (this.children == null || index < 0 || index > this.children.size()) {
return null;
}
return this.children.get(index);
}
public int size() {
return sizeChildren();
}
public int sizeChildren() {
if(this.children == null) {
return 0;
}
return this.children.size();
}
public boolean setReferenceCell(ExcelCell value) {
if((this.referenceCell == null && value != null) ||
(this.referenceCell != null && this.referenceCell.equals(value) == false)) {
this.referenceCell = value;
return true;
}
return false;
}
@Override
public boolean isComparator() {
return false;
}
@Override
public Comparator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy