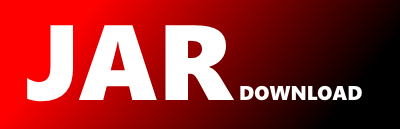
de.unkrig.commons.junit4.AssertLogging Maven / Gradle / Ivy
/*
* de.unkrig.commons - A general-purpose Java class library
*
* Copyright (c) 2014, Arno Unkrig
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without modification, are permitted provided that the
* following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice, this list of conditions and the
* following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the
* following disclaimer in the documentation and/or other materials provided with the distribution.
* 3. The name of the author may not be used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED
* TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL
* THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
* BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package de.unkrig.commons.junit4;
import java.io.File;
import java.text.MessageFormat;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.logging.Handler;
import java.util.logging.Level;
import java.util.logging.LogRecord;
import java.util.logging.Logger;
import org.junit.Assert;
import de.unkrig.commons.lang.AssertionUtil;
import de.unkrig.commons.lang.StringUtil;
import de.unkrig.commons.lang.protocol.RunnableWhichThrows;
import de.unkrig.commons.nullanalysis.Nullable;
/**
* Utility methods related to JUNIT and {@code java.util.logging}.
*/
public final
class AssertLogging {
static { AssertionUtil.enableAssertionsForThisClass(); }
private AssertLogging() {}
/**
* A representation of the log records produced by {@link AssertLogging#collectLogs(Logger, RunnableWhichThrows)}.
*/
public
interface Logs {
/**
* Verifies that a log record with the given {@code message} was logged.
*/
void assertContains(String expected);
/**
* Verifies that no log record with the given {@code message} was logged.
*/
void assertDoesNotContain(String expected);
}
/**
* Temporarily enables logging for the given {@link Logger}, runs the {@code runnable}, and silently collects the
* log records it produces.
*
* @return An object representing the collected log records
* @see Logs
*/
public static Logs
collectLogs(Logger logger, RunnableWhichThrows runnable) throws Exception {
final List messages = Collections.synchronizedList(new ArrayList());
Handler handler = new Handler() {
@Override public void flush() {}
@Override public void close() {}
@Override public void
publish(@Nullable LogRecord lr) {
assert lr != null;
String message = lr.getMessage();
Object[] parameters = lr.getParameters();
if (message.contains("{") && parameters != null && parameters.length > 0) {
try { message = MessageFormat.format(message, parameters); } catch (Exception e) {}
}
messages.add(message.replace(File.separatorChar, '/'));
}
};
Level originalLevel = logger.getLevel();
boolean originalUseParentHandlers = logger.getUseParentHandlers();
logger.addHandler(handler);
logger.setLevel(Level.ALL);
logger.setUseParentHandlers(false);
try {
runnable.run();
return new Logs() {
@Override public void
assertContains(String expected) {
Assert.assertTrue(
"Missing log message '" + expected + "' from\n " + StringUtil.join(messages, "\n "),
messages.contains(expected)
);
}
@Override public void
assertDoesNotContain(String unexpected) {
Assert.assertFalse(unexpected, messages.contains(unexpected));
}
};
} finally {
logger.removeHandler(handler);
logger.setLevel(originalLevel);
logger.setUseParentHandlers(originalUseParentHandlers);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy