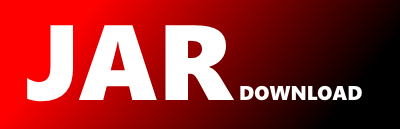
de.unkrig.commons.util.logging.handler.AbstractStreamHandler Maven / Gradle / Ivy
/*
* de.unkrig.commons - A general-purpose Java class library
*
* Copyright (c) 2013, Arno Unkrig
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without modification, are permitted provided that the
* following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice, this list of conditions and the
* following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the
* following disclaimer in the documentation and/or other materials provided with the distribution.
* 3. The name of the author may not be used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED
* TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL
* THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
* BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package de.unkrig.commons.util.logging.handler;
import java.util.logging.Filter;
import java.util.logging.Formatter;
import java.util.logging.Level;
import java.util.logging.LogRecord;
import java.util.logging.SimpleFormatter;
import de.unkrig.commons.nullanalysis.NotNullByDefault;
import de.unkrig.commons.nullanalysis.Nullable;
import de.unkrig.commons.text.expression.EvaluationException;
import de.unkrig.commons.text.parser.ParseException;
import de.unkrig.commons.util.logging.LogUtil;
/**
* Adds an 'autoFlush' feature to the {@link java.util.logging.StreamHandler}, and a one-arg constructor with a
* variable property name prefix.
*/
@NotNullByDefault(false) public abstract
class AbstractStreamHandler extends java.util.logging.StreamHandler {
private static final SimpleFormatter DEFAULT_FORMATTER = new SimpleFormatter();
private boolean autoFlush;
public
AbstractStreamHandler() throws ParseException, EvaluationException {
this(null);
}
/**
* One-arg constructor to be used by derived classes.
*/
public
AbstractStreamHandler(@Nullable String propertyNamePrefix) throws ParseException, EvaluationException {
if (propertyNamePrefix == null) propertyNamePrefix = this.getClass().getName();
// We cannot be sure how the LogManager processes exceptions thrown by this constructor, so we print a stack
// trace to STDERR before we rethrow the exception.
// (The JRE default log manager prints a stack trace, too, so we'll see two.)
try {
this.init(
LogUtil.getLoggingProperty(propertyNamePrefix + ".autoFlush", true),
LogUtil.getLoggingProperty(propertyNamePrefix + ".level", Level.INFO),
LogUtil.getLoggingProperty(propertyNamePrefix + ".filter", Filter.class, null),
LogUtil.getLoggingProperty(
propertyNamePrefix + ".formatter",
Formatter.class,
AbstractStreamHandler.DEFAULT_FORMATTER
),
LogUtil.getLoggingProperty(propertyNamePrefix + ".encoding", (String) null)
);
} catch (ParseException pe) {
pe.printStackTrace();
throw pe;
} catch (EvaluationException ee) {
ee.printStackTrace();
throw ee;
} catch (RuntimeException re) {
re.printStackTrace();
throw re;
}
}
public
AbstractStreamHandler(boolean autoFlush, Level level, Filter filter, Formatter formatter, String encoding) {
this.init(autoFlush, level, filter, formatter, encoding);
}
@Override public synchronized void
publish(LogRecord record) {
super.publish(record);
if (this.autoFlush) this.flush();
}
private void
init(
boolean autoFlush,
Level level,
@Nullable Filter filter,
@Nullable Formatter formatter,
@Nullable String encoding
) {
this.autoFlush = autoFlush;
// Re-configure the underlying 'java.util.logging.StreamHandler' with the CORRECT values.
this.setLevel(level);
this.setFilter(filter);
this.setFormatter(formatter);
try {
this.setEncoding(encoding);
} catch (Exception e) {
try { this.setEncoding(null); } catch (Exception e2) {}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy