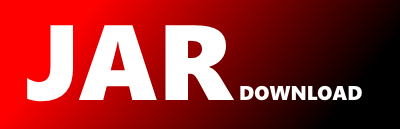
de.unkrig.notemplate.javadocish.templates.AbstractSummaryHtml Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of no-template-core Show documentation
Show all versions of no-template-core Show documentation
A super-small Java library for templating, i.e. generating text files (HTML, XML, whatever) from a "template" text file and dynamic data.
It is based on the concept that the templates are bare Java classes.
The newest version!
/*
* No-Template - an extremely light-weight templating framework
*
* Copyright (c) 2015, Arno Unkrig
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without modification, are permitted provided that the
* following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice, this list of conditions and the
* following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the
* following disclaimer in the documentation and/or other materials provided with the distribution.
* 3. Neither the name of the copyright holder nor the names of its contributors may be used to endorse or promote
* products derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES,
* INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
// SUPPRESS CHECKSTYLE Wrap|LineLength:9999
package de.unkrig.notemplate.javadocish.templates;
import java.util.ArrayList;
import java.util.List;
import de.unkrig.commons.lang.protocol.Producer;
import de.unkrig.commons.lang.protocol.ProducerUtil;
import de.unkrig.commons.nullanalysis.Nullable;
import de.unkrig.notemplate.javadocish.Options;
/**
* Base class for rendering HTML documents similar to JAVADOC's "summary" pages.
*
* Examples:
*
*
*/
public
class AbstractSummaryHtml extends AbstractRightFrameHtml {
/**
* Representation of a section on the "summary page". E.g. on the JAVADOC package summary page, the sections are
* "Interface Summary", "Class Summary", "Enum Summary", "Exception Summary", "Error Summary", "Annotation Type
* Summary".
*/
public static
class Section {
@Nullable public final String anchor;
public final String title;
@Nullable public final String summary;
public final String firstColumnHeading;
public final List items = new ArrayList<>();
public
Section(@Nullable String anchor, String title, @Nullable String summary, String firstColumnHeading) {
this.anchor = anchor;
this.title = title;
this.summary = summary;
this.firstColumnHeading = firstColumnHeading;
}
}
/**
* Representation of an item in a {@link Section}. E.g. on the JAVADOC package summary page, the items of the
* "Interface Summary" section are the individual interfaces declared in the package.
*/
public static
class SectionItem {
public final String link;
@Nullable public final String name;
public final String summary;
public
SectionItem(String link, @Nullable String name, String summary) {
this.link = link;
this.name = name;
this.summary = summary;
}
}
/**
* @param epilog Renders the "description" at the bottom of the page
*
* @see AbstractRightFrameHtml#rRightFrameHtml(String, Options, String[], String[], String[], String[], String[],
* String[], String[], Runnable)
*/
protected void
rSummary(
String windowTitle,
Options options,
@Nullable String[] stylesheetLinks,
@Nullable String[] nav1,
@Nullable String[] nav2,
@Nullable String[] nav3,
@Nullable String[] nav4,
Runnable[] headers,
@Nullable Runnable epilog,
List sections
) {
super.rRightFrameHtml(
windowTitle, // windowTitle
options, // options
stylesheetLinks, // stylesheetLinks
nav1, // nav1
nav2, // nav2
nav3, // nav3
nav4, // nav4
null, // nav5
null, // nav6
() -> { // renderBody
for (Runnable header : headers) {
if (header == null) continue;
this.l(
" "
);
header.run();
this.l(
" "
);
}
this.l(
" ",
" "
);
for (Section section : sections) {
if (section.items.isEmpty()) continue;
if (section.anchor != null) {
this.l(
" ",
" ",
" "
);
}
this.l(
" - ",
"
",
" " + section.title + " ",
" ",
" " + section.firstColumnHeading + " ",
" Description ",
" ",
" "
);
Producer trClass = ProducerUtil.alternate("altColor", "rowColor");
for (SectionItem item : section.items) {
this.l(
" ",
" " + item.name + " "
);
if (item.summary.isEmpty()) {
this.l(
" "
);
} else {
this.l(
" ",
" " + item.summary + "",
" "
);
}
this.l(
" "
);
}
this.l(
" ",
"
",
" "
);
}
this.l(
"
"
);
if (epilog != null) epilog.run();
this.l(
" "
);
}
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy