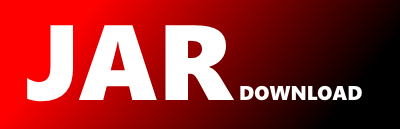
de.unkrig.notemplate.util.Entities Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of no-template-core Show documentation
Show all versions of no-template-core Show documentation
A super-small Java library for templating, i.e. generating text files (HTML, XML, whatever) from a "template" text file and dynamic data.
It is based on the concept that the templates are bare Java classes.
The newest version!
/*
* No-Template - an extremely light-weight templating framework
*
* Copyright (c) 2015, Arno Unkrig
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without modification, are permitted provided that the
* following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice, this list of conditions and the
* following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the
* following disclaimer in the documentation and/or other materials provided with the distribution.
* 3. Neither the name of the copyright holder nor the names of its contributors may be used to endorse or promote
* products derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES,
* INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package de.unkrig.notemplate.util;
import java.io.InputStream;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Properties;
import de.unkrig.commons.lang.AssertionUtil;
/**
* Utility functionality which deals with XML and HTML "entities".
*
* @see HTML and XML character
* entities
*/
public final
class Entities {
static { AssertionUtil.enableAssertionsForThisClass(); }
private Entities() {} // Make the constructor of this utility class inaccessible.
/**
* Replaces the five XML "special characters" with the respective "predefined entities".
*
* Predefined entities in XML
* Name Character Unicode code point (decimal) Description
* quot " U+0022 (34) double quotation mark
* amp & U+0026 (38) ampersand
* apos ' U+0027 (39) apostrophe (apostrophe-quote)
* lt < U+003C (60) less-than sign
* gt > U+003E (62) greater-than sign
*
*/
public static String
replaceXmlSpecialCharactersWithPredefinedEntities(String s) {
int len = s.length();
int idx;
// Optimization for the special case when the string contains no characters that need to be replaced.
NEEDS_ENCODING: {
for (idx = 0; idx < len; idx++) {
char c = s.charAt(idx);
if (c == '"' || c == '&' || c == '\'' || c == '<' || c == '>') break NEEDS_ENCODING;
}
return s;
}
// At this point, "idx" is the index of the first character within "s" than needs to be escaped as an SGML
// entity.
StringBuilder sb = new StringBuilder(s.substring(0, idx));
for (; idx < len; idx++) {
char c = s.charAt(idx);
switch (c) {
case '"': sb.append("""); break;
case '&': sb.append("&"); break;
case '\'': sb.append("'"); break;
case '<': sb.append("<"); break;
case '>': sb.append(">"); break;
default: sb.append(c); break;
}
}
return sb.toString();
}
/**
* Replaces all characters with unicode code point 128 and greater either with
*
* -
* An "HTML character entity reference" (like "{@code ä}" for "ä"), if defined for the codepoint, or
*
* -
* A "numeric character reference" (like "{@code 〹}" for the character for codepoint 12345).
*
*
*
* Particularly, double quotes, ampersands, single quotes, less-than signs and greater-than signs (also known as
* "XML special characters") are not replaced!
*
*
* @see #replaceXmlSpecialCharactersWithPredefinedEntities(String)
*/
public static String
replaceNonAsciiCharactersWithHtmlCharacterReferences(String s) {
int len = s.length();
// Optimization for the special case when the string contains no characters that need to be replaced.
int idx;
NEEDS_ENCODING: {
for (idx = 0; idx < len; idx++) {
if (s.charAt(idx) >= 128) break NEEDS_ENCODING;
}
return s;
}
// At this point, "idx" is the index of the first character within "s" than needs to be replaced.
StringBuilder sb = new StringBuilder(s.substring(0, idx));
for (; idx < len; idx++) {
char c = s.charAt(idx);
if (c <= 127) {
sb.append(c);
continue;
}
String entity = Entities.HTML_CHARACTER_ENTITIES.get(c);
if (entity != null) {
sb.append('&').append(entity).append(';');
continue;
}
sb.append("").append((int) c).append(';');
}
return sb.toString();
}
/**
* Replaces all characters with unicode code point 128 and greater with "numeric character references" (like
* "{@code 〹}" for the character for codepoint 12345).
*
* @see #replaceXmlSpecialCharactersWithPredefinedEntities(String)
*/
public static String
replaceNonAsciiCharactersWithNumericCharacterReferences(String s) {
int len = s.length();
// Optimization for the special case when the string contains no characters that need to be replaced.
int idx;
NEEDS_ENCODING: {
for (idx = 0; idx < len; idx++) {
if (s.charAt(idx) >= 128) break NEEDS_ENCODING;
}
return s;
}
// At this point, "idx" is the index of the first character within "s" than needs to be escaped as an SGML
// entity.
StringBuilder sb = new StringBuilder(s.substring(0, idx));
for (; idx < len; idx++) {
char c = s.charAt(idx);
if (c <= 127) {
sb.append(c);
} else {
sb.append("").append((int) c).append(';');
}
}
return sb.toString();
}
private static final Map HTML_CHARACTER_ENTITIES;
static {
try {
InputStream is = Entities.class.getResourceAsStream("html-character-entities.properties");
assert is != null : "Resource \"html-character-entities.properties\" not found";
Properties p = new Properties();
p.load(is);
is.close();
Map m = new HashMap();
for (Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy